## Transition 过渡动画
> **组件名:uni-transition**
> 代码块: `uTransition`
元素过渡动画
> **注意事项**
> 为了避免错误使用,给大家带来不好的开发体验,请在使用组件前仔细阅读下面的注意事项,可以帮你避免一些错误。
> - 组件需要依赖 `sass` 插件 ,请自行手动安装
> - rotate 旋转动画不需要填写 deg 单位,在小程序上填写单位动画不会执行
> - NVUE 下修改宽高动画,不能定位到中心点
> - 百度小程序下修改宽高 ,可能会影响其他动画,需注意
> - nvue 不支持 costom-class , 请使用 styles
> - 如使用过程中有任何问题,或者您对uni-ui有一些好的建议,欢迎加入 uni-ui 交流群:871950839
### 安装方式
本组件符合[easycom](https://uniapp.dcloud.io/collocation/pages?id=easycom)规范,`HBuilderX 2.5.5`起,只需将本组件导入项目,在页面`template`中即可直接使用,无需在页面中`import`和注册`components`。
如需通过`npm`方式使用`uni-ui`组件,另见文档:[https://ext.dcloud.net.cn/plugin?id=55](https://ext.dcloud.net.cn/plugin?id=55)
### 基本用法
在 ``template`` 中使用组件
```html
<template>
<view>
<button type="primary" @click="open">fade</button>
<uni-transition mode-class="fade" :styles="{'width':'100px','height':'100px','backgroundColor':'red'}" :show="show" @change="change" />
</view>
</template>
<script>
export default {
data() {
return {
show: false,
}
},
onLoad() {},
methods: {
open(mode) {
this.show = !this.show
},
change() {
console.log('触发动画')
}
}
}
</script>
```
### 样式覆盖
**注意:`nvue` 不支持 `custom-class` 属性 ,需要使用 `styles` 属性进行兼容**
使用 `custom-class` 属性绑定样式,可以自定义 `uni-transition` 的样式
```html
<template>
<view class="content">
<uni-transition custom-class="custom-transition" mode-class="fade" :duration="0" :show="true" />
</view>
</template>
<style>
/* 常规样式覆盖 */
.content >>> .custom-transition {
width:100px;
height:100px;
background-color:red;
}
</style>
<style lang="scss">
/* 如果使用 scss 需要使用 /deep/ */
.content /deep/ .custom-transition {
width:100px;
height:100px;
background-color:red;
}
</style>
```
如果使用 `styles` 注意带’-‘连接符的属性需要使用小驼峰写法如:`backgroundColor:red`
```html
<template>
<view class="content">
<uni-transition :styles="styles" mode-class="fade" :duration="0" :show="true" />
</view>
</template>
<script>
export default {
data() {
return {
styles:{
'width':'100px',
'height':'100px',
'backgroundColor':'red'
}
}
}
}
</script>
```
### 自定义动画
当内置动画类型不能满足需求的时候 ,可以使用 `step()` 和 `run()` 自定义动画,入参以及具体用法参考下方属性说明
`init()` 方法可以覆盖默认配置
```html
<template>
<view>
<button type="primary" @click="run">执行动画</button>
<uni-transition ref="ani" :styles="{'width':'100px','height':'100px','backgroundColor':'red'}" :show="show" />
</view>
</template>
<script>
export default {
data() {
return {
show: true,
}
},
onReady() {
this.$refs.ani.init({
duration: 1000,
timingFunction: 'linear',
transformOrigin: '50% 50%',
delay: 500
})
},
methods: {
run() {
// 同时右平移到 100px,旋转 360 读
this.$refs.ani.step({
translateX: '100px',
rotate: '360'
})
// 上面的动画执行完成后,等待200毫秒平移到 0px,旋转到 0 读
this.$refs.ani.step({
translateX: '0px',
rotate: '0'
},
{
timingFunction: 'ease-in',
duration: 200
})
// 开始执行动画
this.$refs.ani.run(()=>{
console.log('动画支持完毕')
})
}
}
}
</script>
```
## API
### Transition Props
|属性名 |类型 |默认值 |说明 |
|:-: |:-: |:-: |:-:|
|show |Boolean|false |控制组件显示或隐藏 |
|mode-class |Array/String |- |内置过渡动画类型 |
|custom-class |String |- |自定义类名 |
|duration |Number |300 |过渡动画持续时间 |
|styles |Object |- |组件样式,同 css 样式,注意带’-‘连接符的属性需要使用小驼峰写法如:`backgroundColor:red` |
#### mode-class 内置过渡动画类型说明
**格式为** :`'fade'` 或者 `['fade','slide-top']`
|属性名 |说明 |
|:-: |:-: |
|fade |渐隐渐出过渡 |
|slide-top |由上至下过渡 |
|slide-right |由右至左过渡 |
|slide-bottom |由下至上过渡 |
|slide-left |由左至右过渡 |
|zoom-in |由小到大过渡 |
|zoom-out |由大到小过渡 |
**注意**
组合使用时,同一种类型相反的过渡动画如(slide-top、slide-bottom)同时使用时,只有最后一个生效
### Transition Events
|事件名 |说明 |返回值 |
|:-: |:-: |:-: |
|click |点击组件触发 |- |
|change |过渡动画结束时触发 | e = {detail:true} |
### Transition Methons
|方法名|说明|参数|
|:-:|:-:|:-:|
|init()|手动初始化配置|Function(OBJECT:config)|
|step()|动画队列|Function(OBJECT:type,OBJECT:config)|
|run()|执行动画|Function(FUNCTION:callback) |
### init(OBJECT:config)
**通过 ref 调用方法**
手动设置动画配置,需要在页面渲染完毕后调用
```javascript
this.$refs.ani.init({
duration: 1000,
timingFunction:'ease',
delay:500,
transformOrigin:'left center'
})
```
### step(OBJECT:type,OBJECT:config) 动画队列
**通过 ref 调用方法**
调用 `step()` 来表示一组动画完成,`step` 第一个参数可以传入任意多个动画方法,一组动画中的所有动画会同时开始,一组动画完成后才会进行下一组动画。`step` 第二个参数可以传入一个跟 `uni.createAnimation()` 一样的配置参数用于指定当前组动画的配置。
Tips
- 第一个参数支持的动画参考下面的 `支持的动画`
- 第二个参数参考下面的 `动画配置`,可省略,如果省略继承`init`的配置
```javascript
this.$refs.ani.step({
translateX: '100px'
},{
duration: 1000,
timingFunction:'ease',
delay:500,
transformOrigin:'left center'
})
```
### run(FUNCTION:callback) 执行动画
**通过 ref 调用方法**
在执行 `step()` 后,需要调用 `run()` 来运行动画 ,否则动画会一直等待
`run()` 方法可以传入一个 `callback` 函数 ,会在所有动画执行完毕后回调
```javascript
this.$refs.ani.step({
translateX: '100px'
})
this.$refs.ani.run(()=>{
console.log('动画执行完毕')
})
```
### 动画配置
动画配置 , `init()` 与 `step()` 第二个参数配置相同 ,如果配置`step() `第二个参数,将会覆盖 `init()` 的配置
|属性名|值|必填|默认值|说明|平台差异|
|:-:|:-:|:-:|:-:|:-:|:-:|
|duration|Number|否|400|动画持续时间,单位ms|-|
|timingFunction|String|否|"linear"|定义动画的效果|-|
|delay|Number|否|0|动画延迟时间,单位 ms|-|
|needLayout|Boolean|否|false |动画执行是否影响布局|仅 nvue 支持|
|transformOrigin|String |否|"center center"|设置 [transform-origin](https://developer.mozilla.org/en-US/docs/Web/CSS/transform-origin)|-|
### timingFunction 属性说明
|值|说明|平台差异|
|:-:|:-:|:-:|
|linear|动画从头到尾的速度是相同的|-|
|ease|动画以低速开始,然后加快,在结束前变慢|-|
|ease-in| 动画以低速开始|-|
|ease-in-out| 动画以低速开始和结束|-|
|ease-out|动画以低速结束|-|
|step-start|动画第一帧就跳至结束状态直到结束|nvue不支持|
|step-end|动画一直保持开始状态,最后一帧跳到结束状态|nvue不支持|
```javascript
// init 配置
this.$refs.ani.init({
duration: 1000,
timingFunction:'ease',
delay:500,
transformOrigin:'left center'
})
// step 配置
this.$refs.ani.step({
translateX: '100px'
},{
duration: 1000,
timingFunction:'ease',
delay:500
没有合适的资源?快使用搜索试试~ 我知道了~
开源H5盲盒商城源码系统4.0-vue+TP5php框架开发开源网站+安装教程
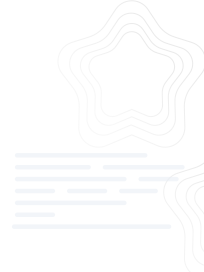
共2000个文件
js:1227个
png:763个
json:99个


温馨提示
需要搭建请私信我 开源H5盲盒商城源码系统4.0|vue+TP5php框架开发开源网站+安装教程 vue+tp5框架编写,H5网页,前后端分离,开源无加密无授权,可以二开使用。 含充值3级分销,盲盒回收成余/额功能/晒图/盲盒转增功能。 带微信无限回调登录、易支付码支付通用聚合接口、短信宝短信、阿里云oss
资源推荐
资源详情
资源评论
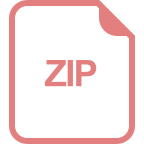
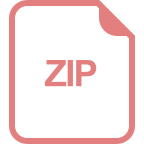
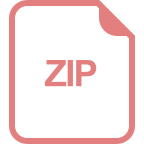
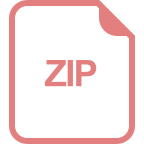
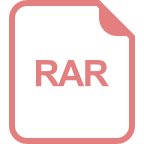
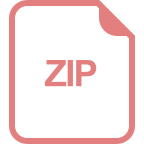
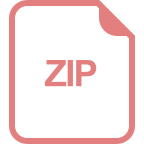
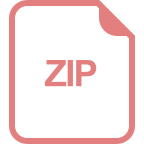
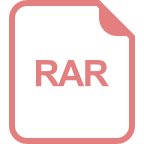
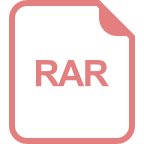
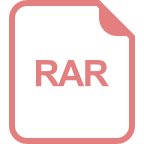
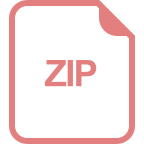
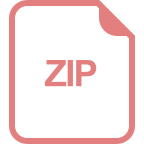
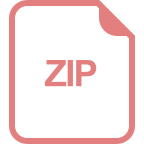
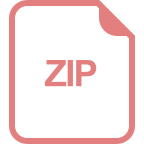
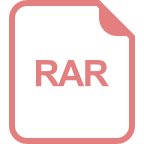
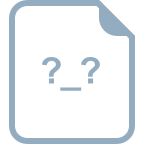
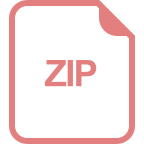
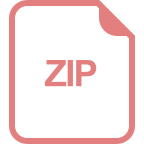
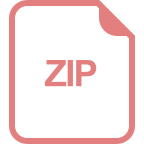
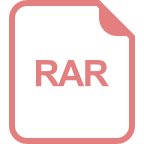
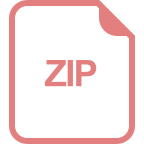
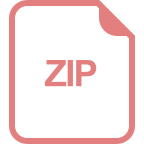
收起资源包目录

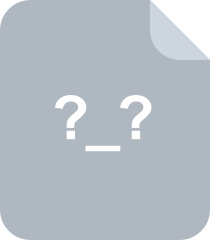
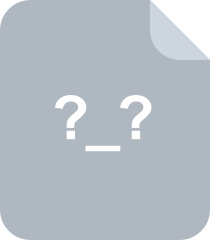
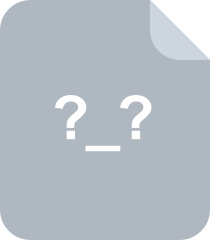
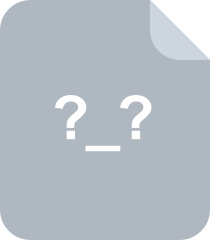
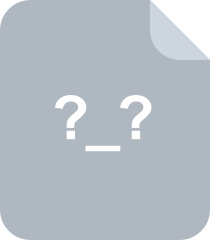
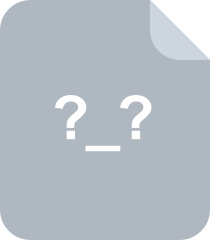
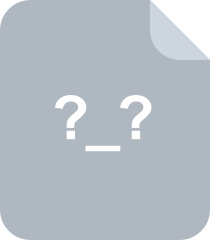
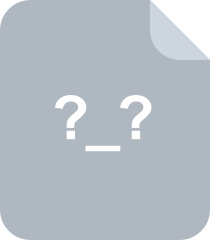
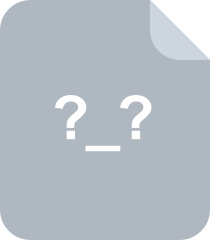
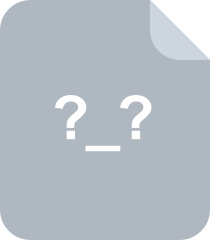
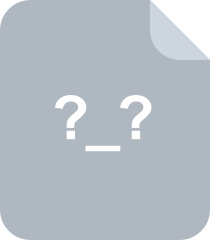
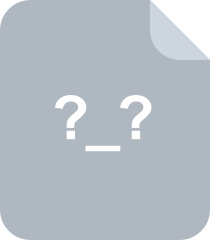
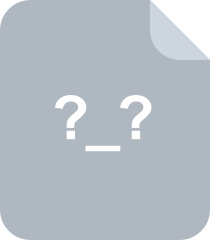
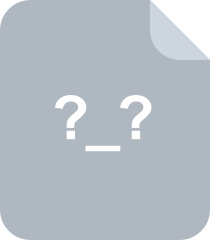
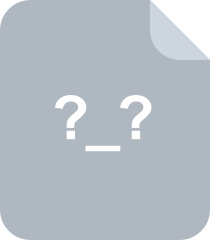
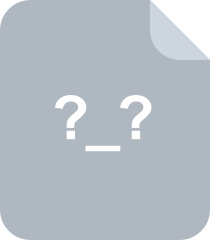
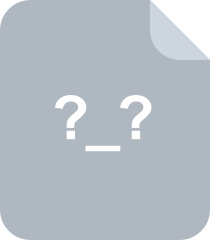
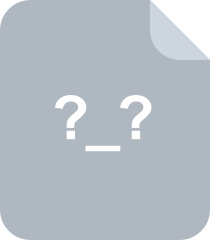
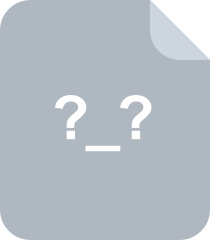
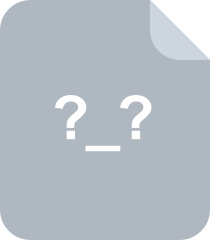
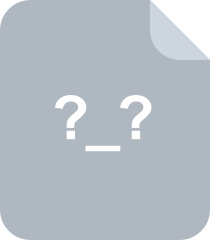
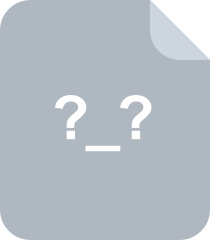
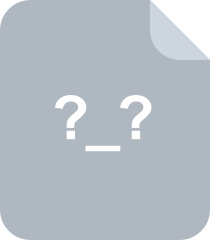
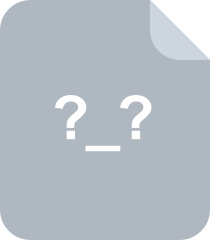
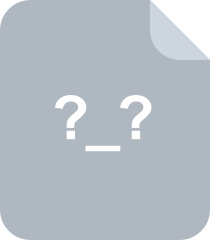
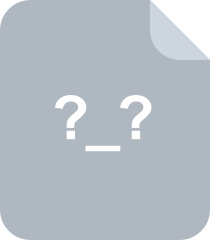
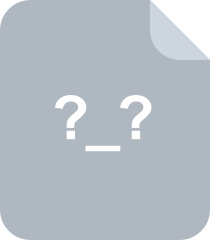
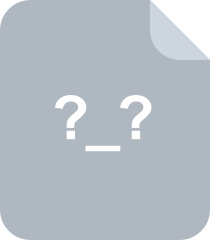
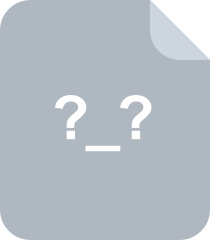
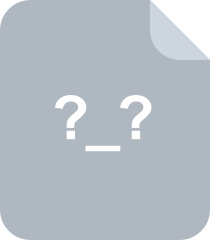
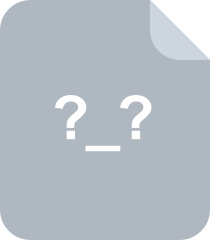
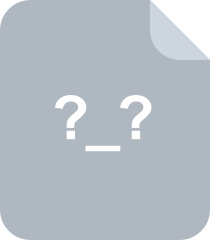
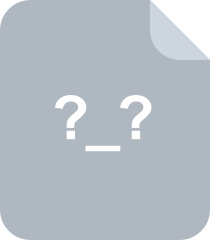
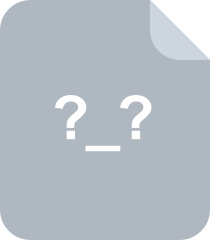
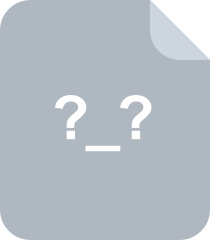
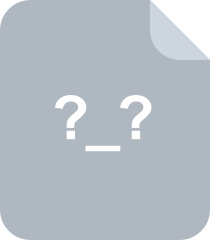
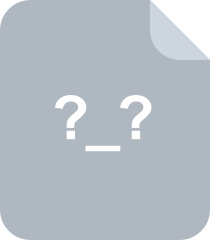
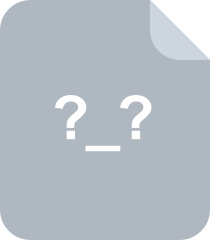
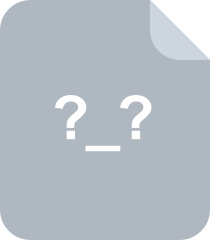
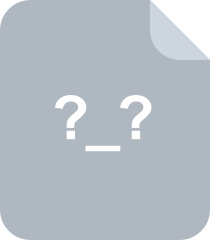
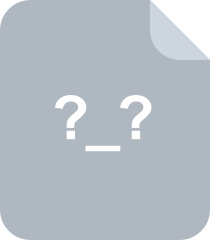
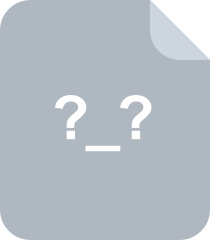
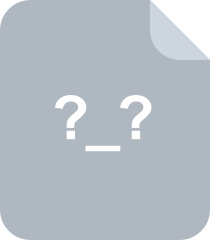
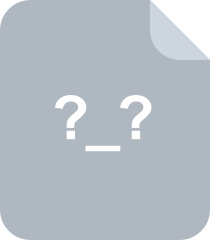
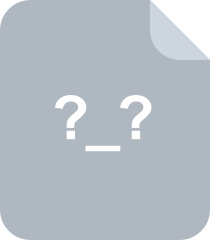
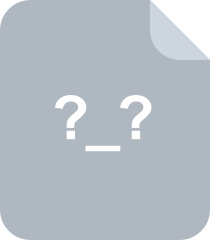
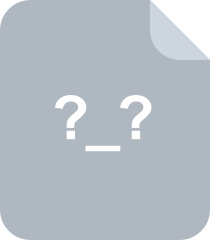
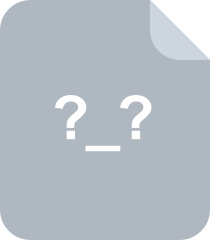
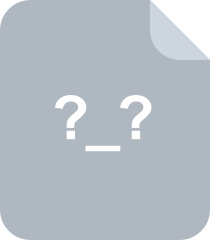
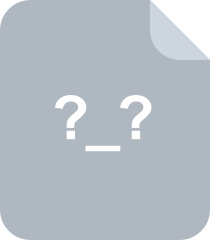
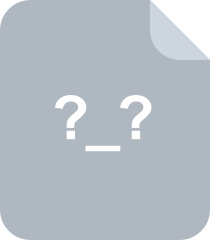
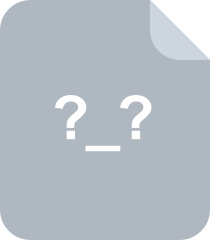
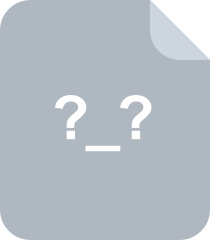
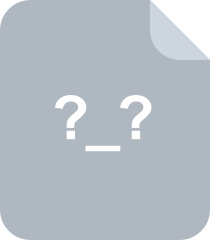
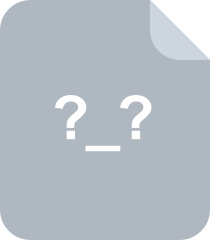
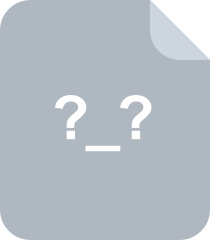
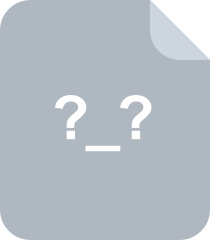
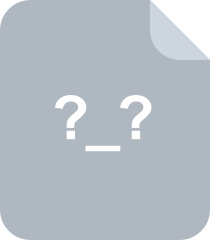
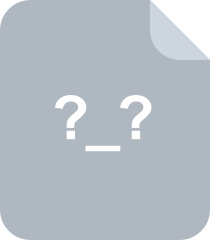
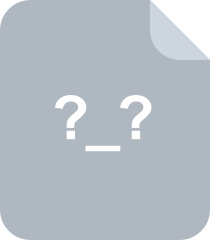
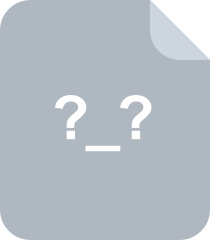
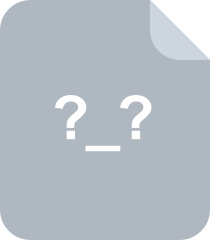
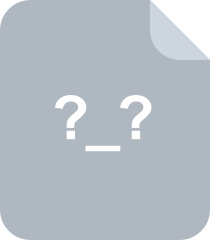
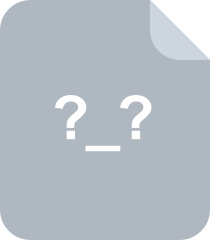
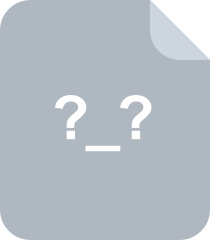
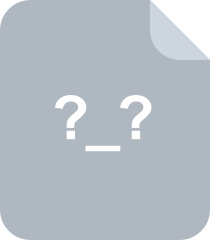
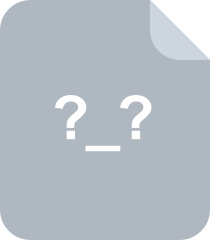
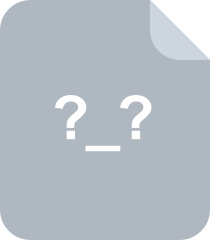
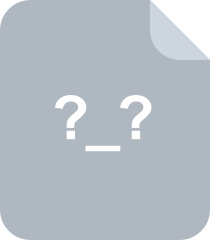
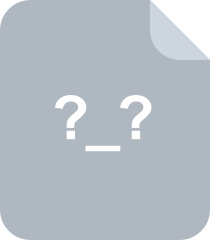
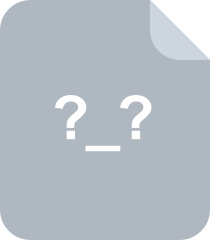
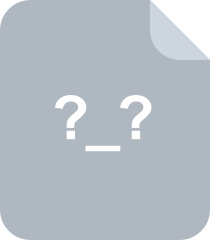
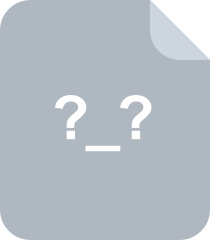
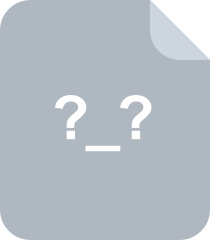
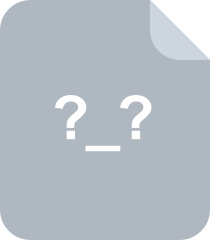
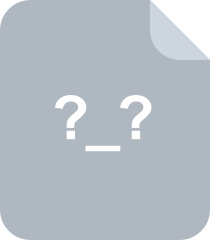
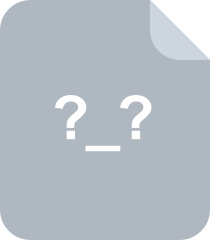
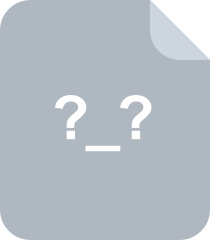
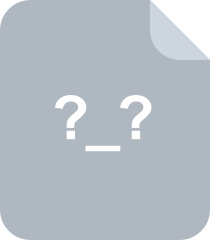
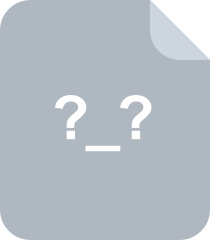
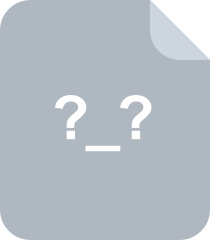
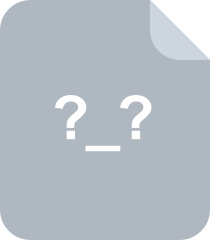
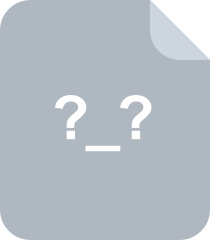
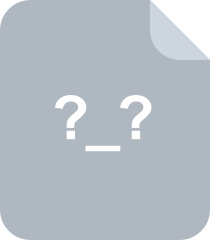
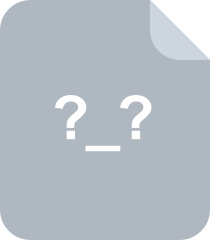
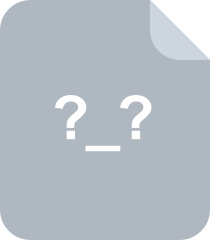
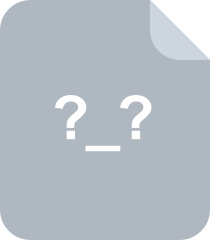
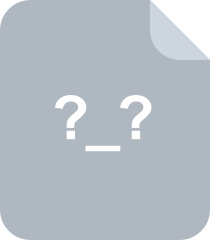
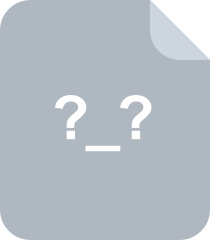
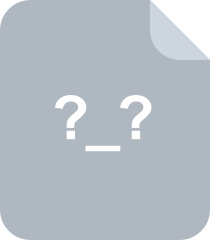
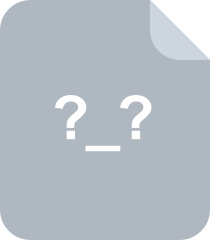
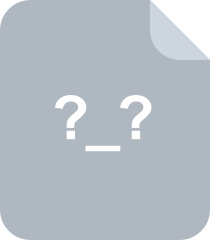
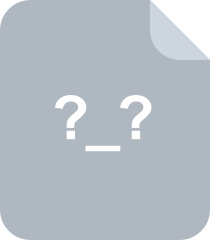
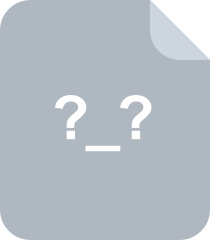
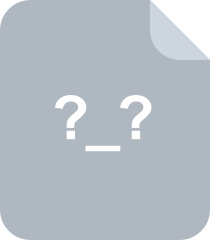
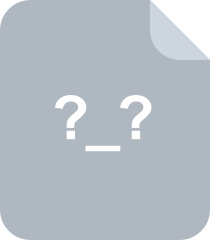
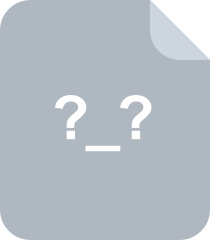
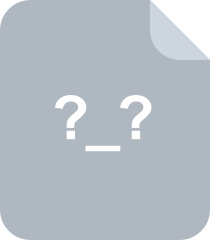
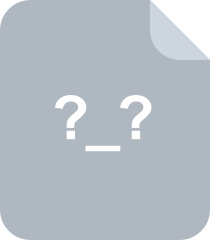
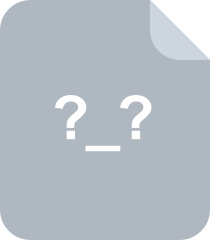
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
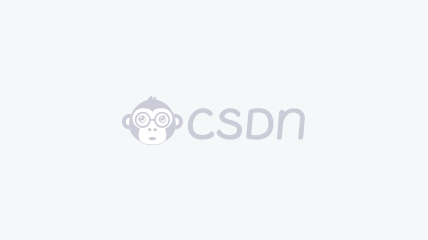

百创科技
- 粉丝: 2042
- 资源: 261
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

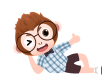
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


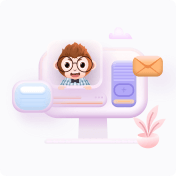
安全验证
文档复制为VIP权益,开通VIP直接复制
