在Android开发中,自定义UI控件是一种常见的需求,它能帮助开发者实现独特且符合应用需求的交互效果。本教程将深入讲解如何通过继承ViewGroup并利用Scroller组件,实现手动拖拽滚屏以及自动滚屏功能的自定义UI控件。 `Scroller`是Android中的一个关键类,它并不直接控制View的滚动,而是提供了平滑滚动的计算逻辑。当你需要进行动画滚动,比如在用户手指离开屏幕后继续滚动一段距离,或者在程序逻辑中实现平滑滚动时,Scroller就派上了用场。Scroller的工作方式是通过`startScroll()`方法设置初始位置和滚动目标,然后通过`computeScrollOffset()`方法获取当前滚动的位置,最后在`onDraw()`或`computeScroll()`中更新视图的位置。 现在,我们来看如何在自定义的ViewGroup中实现手动拖拽滚屏: 1. **创建自定义ViewGroup**:你需要创建一个新的Java类,继承自ViewGroup。在这个类中,你需要重写一些关键方法,如`onTouchEvent()`来处理触摸事件,`onLayout()`来确定子View的位置,以及`onDraw()`来绘制视图。 2. **处理触摸事件**:在`onTouchEvent()`中,你需要捕获用户的触摸动作。当用户按下屏幕时记录初始位置,当用户移动手指时,根据手指的移动距离更新ViewGroup的位置。同时,你需要跟踪用户的滑动是否超过了边界,以便后续的自动滚屏操作。 3. **应用Scroller**:在用户松开手指后,你可以使用Scroller的`startScroll()`方法,根据用户滑动的距离和方向来启动一个滚动动画。Scroller会计算出平滑滚动的过程,你需要在`computeScroll()`方法中持续检查Scroller的状态,并更新ViewGroup的位置。 4. **自动滚屏**:如果需要实现自动滚屏,可以在特定条件下(比如达到边界)调用Scroller的`fling()`方法,传入起始位置、结束位置、速度等参数,让Scroller自动执行一个快速的滚动动画。 5. **重绘与滚动更新**:在每次改变ViewGroup位置后,记得调用`invalidate()`来请求重新绘制,以便系统能够及时更新视图。同时,你可能需要在`onComputeScroll()`中处理Scroller的滚动过程,确保在Scroller完成滚动动画后正确地更新View的位置。 6. **AllAppScroller.java**:这个文件很可能是实现上述功能的具体代码。通过查看源码,你可以学习到如何在实际项目中应用这些概念和方法,理解每个部分的作用和它们之间的协作。 通过以上步骤,你可以创建一个既支持手动拖拽也支持自动滚屏的自定义UI控件。这样的控件在许多应用场景中都非常有用,例如在长列表或者轮播图中,可以提供更加流畅的用户体验。同时,这也是Android开发中的一个重要技能,有助于提升你的编程能力。
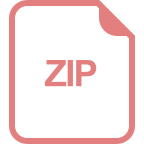
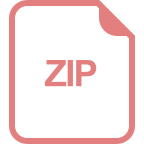
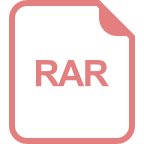
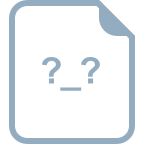
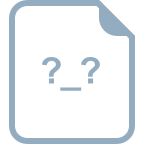
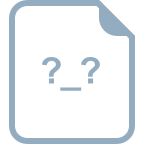
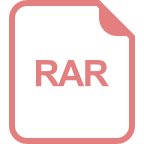
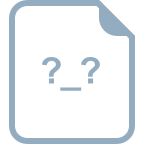
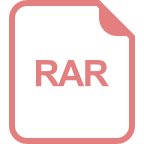
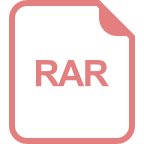
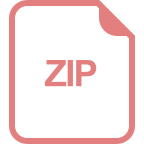
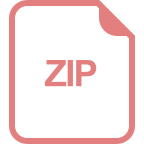
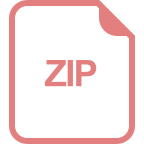
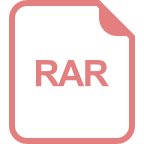
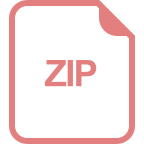
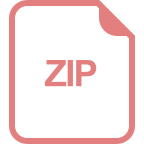
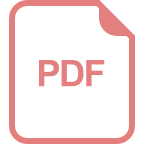
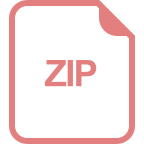
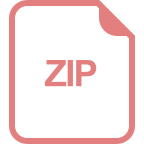
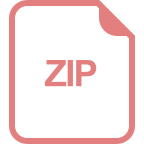
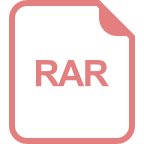
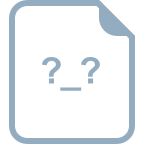
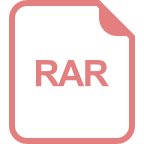
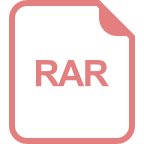

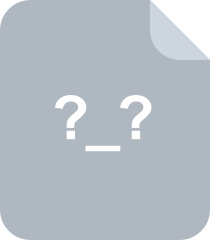
- 1
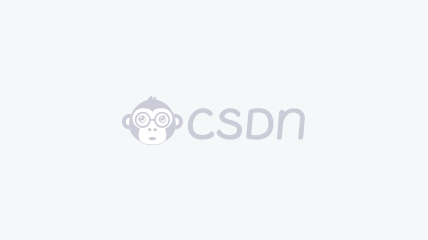
- 甘磊62662015-08-31还行吧不错的
- 巨盛2013-11-04好东西,学习对触摸屏的动作
- sinat_217353432014-12-04好东西,谢谢楼主分享
- Arrow5205202014-12-30自定义控件,可以下载学习看

- 粉丝: 1
- 资源: 29
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

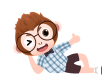
最新资源
- 基于小程序的校园疫情防控管理平台小程序源代码(java+小程序+mysql+LW).zip
- 【MPSK通信】基于matlab宽带信道上MPSK通信仿真【含Matlab源码 9932期】.zip
- 【机器臂控制】基于matlab运动学的四自由度机械臂末端稳定性控制【含Matlab源码 9948期】.zip
- 【编码解码】基于matlab罗利衰落信道编解码器设计【含Matlab源码 9930期】.zip
- 【手势识别】基于matlab神经元网络结构和移动加速度计传感器手势识别【含Matlab源码 9936期】.zip
- 【目标检测】基于matlab局部强度和梯度特性LIG红外小目标检测【含Matlab源码 9940期】.zip
- 【生物学】基于matlab果蝇幼虫感觉神经元TRP通道动力学的突发和尖峰冷温编码【含Matlab源码 9931期】.zip
- 【图像边缘检测】基于matlab Canny算法自适应阈值边缘检测【含Matlab源码 9944期】.zip
- 【手写数字识别】基于matlab BP神经网络数字识别(含课程报告+PPT)【含Matlab源码 9941期】.zip
- 【数字信号调制】基于matlab AWGN信道上模拟不同调制技术(BPSK、QPSK、8PSK、BFSK、16QAM)【含Matlab源码 9933期】.zip
- 【图像分割】基于matlab图像纹理分割【含Matlab源码 9945期】复现.zip
- 【图像分割】基于matlab动态阈值结合全局阈值算法图像分割【含Matlab源码 9943期】.zip
- 【图像去噪】基于matlab PolSAR GWLS滤波器图像去噪【含Matlab源码 9937期】.zip
- 【图像融合】基于matlab RGB和最佳波段图像融合的两尺度图像融合【含Matlab源码 9947期】.zip
- 【图像去噪】基于matlab分裂BregmanTV全变分各向同性各向异性去噪【含Matlab源码 9942期】.zip
- 【图像去噪】基于matlab模糊逻辑方法图像去噪【含Matlab源码 9949期】.zip

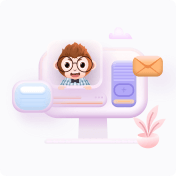
