package com.loveplusplus.weixin;
import java.io.IOException;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.jsoup.Connection.Method;
import org.jsoup.Connection.Response;
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
import org.jsoup.select.Elements;
import com.google.gson.Gson;
import com.google.gson.reflect.TypeToken;
public class Main {
public static String TOKEN;
public static String LOGIN_URL = "http://mp.weixin.qq.com/cgi-bin/login?lang=zh_CN";
public static String SEND_MSG = "http://mp.weixin.qq.com/cgi-bin/singlesend?t=ajax-response&lang=zh_CN";
/**
* 获取登录session
*/
public static Map<String, String> auth(String username, String password)
throws IOException {
Map<String, String> map = new HashMap<String, String>();
map.put("username", username);
map.put("pwd", MD5.getMD5(password.getBytes())
.toUpperCase());
map.put("f", "json");
Response response = Jsoup.connect(LOGIN_URL).ignoreContentType(true)
.method(Method.POST).data(map).execute();
Map<String, String> cookies = response.cookies();
//新添加代码
String json=response.body();
System.out.println(json);
Gson gson=new Gson();
WeiXinResponse weiXinResponse=gson.fromJson(json, WeiXinResponse.class);
String errMsg=weiXinResponse.getErrMsg();
TOKEN=errMsg.substring(errMsg.lastIndexOf("=")+1, errMsg.length());
cookies= response.cookies();
return cookies;
}
/**
* 获取关注列表
*
* @param cookie
* @return
* @throws IOException
*/
public static List<WeiXinFans> getFans(Map<String, String> cookie)
throws IOException {
String FANS_URL = "http://mp.weixin.qq.com/cgi-bin/contactmanagepage?t=wxm-friend&token="+TOKEN+"&lang=zh_CN&pagesize=10&pageidx=0&type=0&groupid=0";
Document document = Jsoup.connect(FANS_URL).cookies(cookie).post();
Elements eles = document.select("#json-friendList");
Element element = eles.get(0);
String json = element.data();
Gson gson = new Gson();
return gson.fromJson(json, new TypeToken<List<WeiXinFans>>() {
}.getType());
}
/**
* 发送消息
*/
public static void sendMsg(Map<String, String> cookie, String content,
String fakeId) throws IOException {
HashMap<String, String> map = new HashMap<String, String>();
map.put("tofakeid", fakeId);
map.put("content", content);
map.put("error", "false");
map.put("token", TOKEN);
map.put("type", "1");
map.put("ajax", "1");
String referrerUrl="http://mp.weixin.qq.com/cgi-bin/singlemsgpage?token="+TOKEN+"&fromfakeid="+fakeId+"&msgid=&source=&count=20&t=wxm-singlechat&lang=zh_CN";
Document document = Jsoup.connect(SEND_MSG).referrer(referrerUrl).data(map).cookies(cookie)
.post();
Element body = document.body();
System.out.println(body.text());
}
public static void main(String[] args) throws IOException {
Map<String, String> cookie = auth("账号", "密码");//自行填写
//获取粉丝列表
//List<WeiXinFans> list = getFans(cookie);
//群发
//for (WeiXinFans fans : list) {
//System.out.println(fans);
//sendMsg(cookie, "2013测试", fans.getFakeId());
//}
sendMsg(cookie, "非常抱歉,昨天服务器出现问题,今天已经修复,现在可以正常查询。", "粉丝ID");//ID填写
}
}

MaxCrazy
- 粉丝: 1
- 资源: 1
最新资源
- 基于一款语音交互智能家居机器人全部资料+详细文档+优秀项目.zip
- CuZnAl合金焊接方法 - .pdf
- Cu异种金属冷金属过渡熔钎焊接头显微组织与性能 - .pdf
- D406A钢电子束焊接头组织及性能分析 - .pdf
- 基于语音识别的智能家居控制方案研究与设计全部资料+详细文档+优秀项目.zip
- DB21T 2700-2016 焊接绝热气瓶充装站安全技术条件.pdf
- DG1427-2001钢制压力容器产品焊接试板的力学性能检验.pdf
- DIN 928-2000 焊接方螺母 Square weld nuts.pdf
- DIN 1910-1-1983 焊接 第1部分 焊接概念焊接方法分类.pdf
- DIN 1910-2-1977 焊接 金属焊接 工艺.pdf
- DIN 1910-5-1986 焊接.金属焊接.电阻焊接.方法.pdf
- DIN 1912-1 1976 焊接.钎焊图样表示法.焊接接头.焊接坡口及焊缝的概念与名称.pdf
- DIN 1912-2 1977 焊接.钎焊图样表示法.工作位置.焊缝倾角.焊缝旋转角度.PDF
- DIN 1910-11-1979 焊接.金属焊接材料术语.pdf
- DIN 2393-1-1994 有特殊尺寸精度的精密焊接钢管 尺寸.PDF
- DIN 6700-1-2001 中文版 铁路车辆及车辆部件的焊接.第1部分基本概念,基本规则.pdf
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


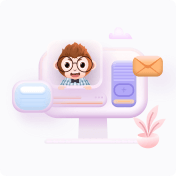
- 1
- 2
- 3
- 4
- 5
- 6
前往页