<?php
namespace com;
// --------------------------------------------------------------------------------
// PhpConcept Library - Zip Module 2.8.2
// --------------------------------------------------------------------------------
// License GNU/LGPL - Vincent Blavet - August 2009
// http://www.phpconcept.net
// --------------------------------------------------------------------------------
//
// Presentation :
// PclZip is a PHP library that manage ZIP archives.
// So far tests show that archives generated by PclZip are readable by
// WinZip application and other tools.
//
// Description :
// See readme.txt and http://www.phpconcept.net
//
// Warning :
// This library and the associated files are non commercial, non professional
// work.
// It should not have unexpected results. However if any damage is caused by
// this software the author can not be responsible.
// The use of this software is at the risk of the user.
//
// --------------------------------------------------------------------------------
// $Id: pclzip.lib.php,v 1.60 2009/09/30 21:01:04 vblavet Exp $
// --------------------------------------------------------------------------------
// ----- Constants
if (!defined('PCLZIP_READ_BLOCK_SIZE')) {
define( 'PCLZIP_READ_BLOCK_SIZE', 2048 );
}
// ----- File list separator
// In version 1.x of PclZip, the separator for file list is a space
// (which is not a very smart choice, specifically for windows paths !).
// A better separator should be a comma (,). This constant gives you the
// abilty to change that.
// However notice that changing this value, may have impact on existing
// scripts, using space separated filenames.
// Recommanded values for compatibility with older versions :
//define( 'PCLZIP_SEPARATOR', ' ' );
// Recommanded values for smart separation of filenames.
if (!defined('PCLZIP_SEPARATOR')) {
define( 'PCLZIP_SEPARATOR', ',' );
}
// ----- Error configuration
// 0 : PclZip Class integrated error handling
// 1 : PclError external library error handling. By enabling this
// you must ensure that you have included PclError library.
// [2,...] : reserved for futur use
if (!defined('PCLZIP_ERROR_EXTERNAL')) {
define( 'PCLZIP_ERROR_EXTERNAL', 0 );
}
// ----- Optional static temporary directory
// By default temporary files are generated in the script current
// path.
// If defined :
// - MUST BE terminated by a '/'.
// - MUST be a valid, already created directory
// Samples :
// define( 'PCLZIP_TEMPORARY_DIR', '/temp/' );
// define( 'PCLZIP_TEMPORARY_DIR', 'C:/Temp/' );
if (!defined('PCLZIP_TEMPORARY_DIR')) {
define( 'PCLZIP_TEMPORARY_DIR', '' );
}
// ----- Optional threshold ratio for use of temporary files
// Pclzip sense the size of the file to add/extract and decide to
// use or not temporary file. The algorythm is looking for
// memory_limit of PHP and apply a ratio.
// threshold = memory_limit * ratio.
// Recommended values are under 0.5. Default 0.47.
// Samples :
// define( 'PCLZIP_TEMPORARY_FILE_RATIO', 0.5 );
if (!defined('PCLZIP_TEMPORARY_FILE_RATIO')) {
define( 'PCLZIP_TEMPORARY_FILE_RATIO', 0.47 );
}
// --------------------------------------------------------------------------------
// ***** UNDER THIS LINE NOTHING NEEDS TO BE MODIFIED *****
// --------------------------------------------------------------------------------
// ----- Global variables
$g_pclzip_version = "2.8.2";
// ----- Error codes
// -1 : Unable to open file in binary write mode
// -2 : Unable to open file in binary read mode
// -3 : Invalid parameters
// -4 : File does not exist
// -5 : Filename is too long (max. 255)
// -6 : Not a valid zip file
// -7 : Invalid extracted file size
// -8 : Unable to create directory
// -9 : Invalid archive extension
// -10 : Invalid archive format
// -11 : Unable to delete file (unlink)
// -12 : Unable to rename file (rename)
// -13 : Invalid header checksum
// -14 : Invalid archive size
define( 'PCLZIP_ERR_USER_ABORTED', 2 );
define( 'PCLZIP_ERR_NO_ERROR', 0 );
define( 'PCLZIP_ERR_WRITE_OPEN_FAIL', -1 );
define( 'PCLZIP_ERR_READ_OPEN_FAIL', -2 );
define( 'PCLZIP_ERR_INVALID_PARAMETER', -3 );
define( 'PCLZIP_ERR_MISSING_FILE', -4 );
define( 'PCLZIP_ERR_FILENAME_TOO_LONG', -5 );
define( 'PCLZIP_ERR_INVALID_ZIP', -6 );
define( 'PCLZIP_ERR_BAD_EXTRACTED_FILE', -7 );
define( 'PCLZIP_ERR_DIR_CREATE_FAIL', -8 );
define( 'PCLZIP_ERR_BAD_EXTENSION', -9 );
define( 'PCLZIP_ERR_BAD_FORMAT', -10 );
define( 'PCLZIP_ERR_DELETE_FILE_FAIL', -11 );
define( 'PCLZIP_ERR_RENAME_FILE_FAIL', -12 );
define( 'PCLZIP_ERR_BAD_CHECKSUM', -13 );
define( 'PCLZIP_ERR_INVALID_ARCHIVE_ZIP', -14 );
define( 'PCLZIP_ERR_MISSING_OPTION_VALUE', -15 );
define( 'PCLZIP_ERR_INVALID_OPTION_VALUE', -16 );
define( 'PCLZIP_ERR_ALREADY_A_DIRECTORY', -17 );
define( 'PCLZIP_ERR_UNSUPPORTED_COMPRESSION', -18 );
define( 'PCLZIP_ERR_UNSUPPORTED_ENCRYPTION', -19 );
define( 'PCLZIP_ERR_INVALID_ATTRIBUTE_VALUE', -20 );
define( 'PCLZIP_ERR_DIRECTORY_RESTRICTION', -21 );
// ----- Options values
define( 'PCLZIP_OPT_PATH', 77001 );
define( 'PCLZIP_OPT_ADD_PATH', 77002 );
define( 'PCLZIP_OPT_REMOVE_PATH', 77003 );
define( 'PCLZIP_OPT_REMOVE_ALL_PATH', 77004 );
define( 'PCLZIP_OPT_SET_CHMOD', 77005 );
define( 'PCLZIP_OPT_EXTRACT_AS_STRING', 77006 );
define( 'PCLZIP_OPT_NO_COMPRESSION', 77007 );
define( 'PCLZIP_OPT_BY_NAME', 77008 );
define( 'PCLZIP_OPT_BY_INDEX', 77009 );
define( 'PCLZIP_OPT_BY_EREG', 77010 );
define( 'PCLZIP_OPT_BY_PREG', 77011 );
define( 'PCLZIP_OPT_COMMENT', 77012 );
define( 'PCLZIP_OPT_ADD_COMMENT', 77013 );
define( 'PCLZIP_OPT_PREPEND_COMMENT', 77014 );
define( 'PCLZIP_OPT_EXTRACT_IN_OUTPUT', 77015 );
define( 'PCLZIP_OPT_REPLACE_NEWER', 77016 );
define( 'PCLZIP_OPT_STOP_ON_ERROR', 77017 );
// Having big trouble with crypt. Need to multiply 2 long int
// which is not correctly supported by PHP ...
//define( 'PCLZIP_OPT_CRYPT', 77018 );
define( 'PCLZIP_OPT_EXTRACT_DIR_RESTRICTION', 77019 );
define( 'PCLZIP_OPT_TEMP_FILE_THRESHOLD', 77020 );
define( 'PCLZIP_OPT_ADD_TEMP_FILE_THRESHOLD', 77020 ); // alias
define( 'PCLZIP_OPT_TEMP_FILE_ON', 77021 );
define( 'PCLZIP_OPT_ADD_TEMP_FILE_ON', 77021 ); // alias
define( 'PCLZIP_OPT_TEMP_FILE_OFF', 77022 );
define( 'PCLZIP_OPT_ADD_TEMP_FILE_OFF', 77022 ); // alias
// ----- File description attributes
define( 'PCLZIP_ATT_FILE_NAME', 79001 );
define( 'PCLZIP_ATT_FILE_NEW_SHORT_NAME', 79002 );
define( 'PCLZIP_ATT_FILE_NEW_FULL_NAME', 79003 );
define( 'PCLZIP_ATT_FILE_MTIME', 79004 );
define( 'PCLZIP_ATT_FILE_CONTENT', 79005 );
define( 'PCLZIP_ATT_FILE_COMMENT', 79006 );
// ----- Call backs values
define( 'PCLZIP_CB_PRE_EXTRACT', 78001 );
define( 'PCLZIP_CB_POST_EXTRACT', 78002 );
define( 'PCLZIP_CB_PRE_ADD', 78003 );
define( 'PCLZIP_CB_POST_ADD', 78004 );
/* For futur use
define( 'PCLZIP_CB_PRE_LIST', 78005 );
define( 'PCLZIP_CB_POST_LIST', 78006 );
define( 'PCLZIP_CB_PRE_DELETE', 78007 );
define( 'PCLZIP_CB_POST_DELETE', 78008 );
*/
// --------------------------------------------------------------------------------
// Class : PclZip
// Description :
// PclZip is the class that represent a Zip archive.
// The public methods allow the manipulation of the archive.
// Attributes :
// Attributes must not be accessed directly.
// Methods :
// PclZip() : Object creator
// create() : Creates the Zip archive
// listContent() : List the content of the Zip archive
// extract() : Extract the content of t
没有合适的资源?快使用搜索试试~ 我知道了~
杰迅CMS全国城市分站系统
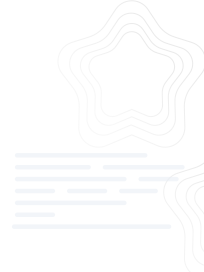
共1257个文件
php:336个
js:220个
png:192个

需积分: 5 4 下载量 86 浏览量
2023-08-29
14:49:14
上传
评论 1
收藏 33.5MB RAR 举报
温馨提示
杰迅CMS全国城市分站系统 杰迅CMS是一款基于TP5.0框架为核心开发的一套免费+开源的城市分站内容管理系统。团队自2013年起自主研发城市分站内容管理系统,5年来,修复升级上千个功能性问题 1、适用场景 城市分站系统:适用于生产型企业,本地服务类型的公司 站群系统:适用于中大型集团,学校,政府机关单位,招商加盟类型的公司 2、功能 城市分站系统: 1)一个主域名,无限二级域名或二级目录城市分站 2)城市分站栏目不能独立,内容可以独立也可以和主站内容一致加上地区前缀 3)主站和分站的模板都是同一个 站群系统 1)一个主域名,子站域名不限制 2)子站数据可以复制主站,也可以独立栏目和数据自成站点 3)主站和子站的模板可以不是同一个,每个子站的模板都可以不一样 3、配套 城市分站系统 1)多站点管理平台:开通后可以使用SEO监控系统(收录、排名),文章组合自动发布,文章采集自动发布,外链发布等多个SEO自动化功能 2)saas小程序平台:开通后可以在线创建微信+百度+支付宝+抖音+头条+QQ六大小程序平台,数据完全同步网站和电脑网站+平板网站+手机网站+微官网形成
资源推荐
资源详情
资源评论
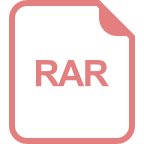
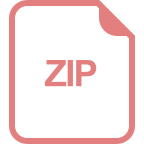
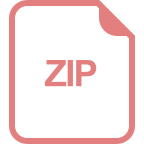
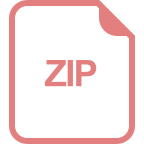
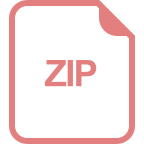
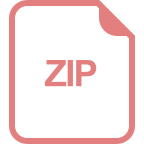
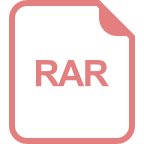
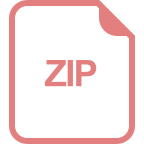
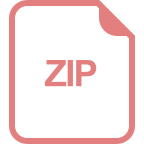
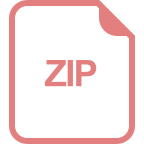
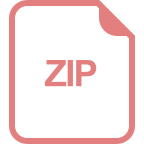
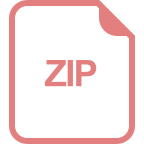
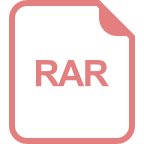
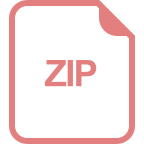
收起资源包目录

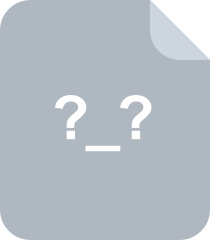
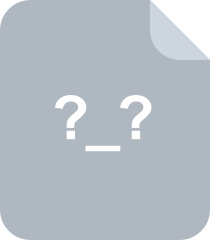
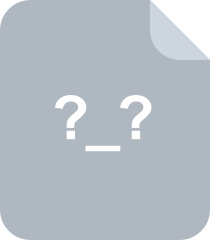
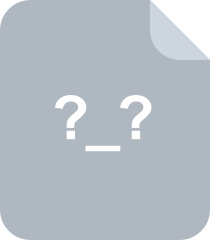
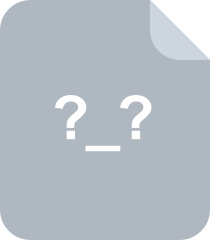
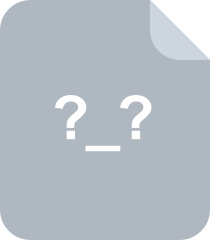
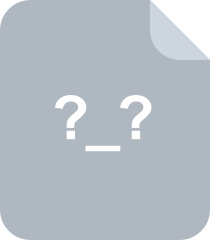
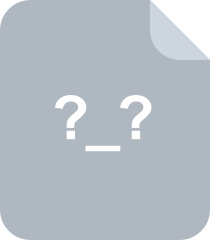
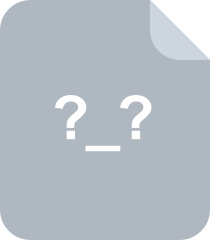
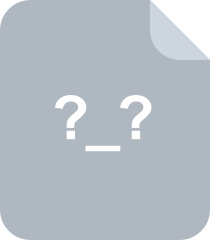
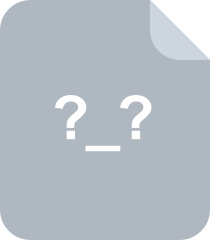
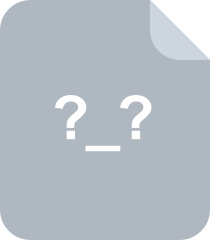
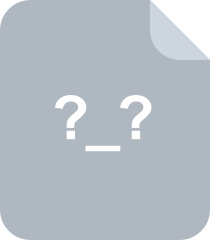
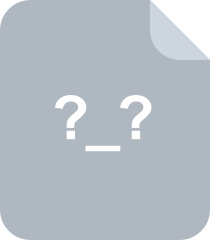
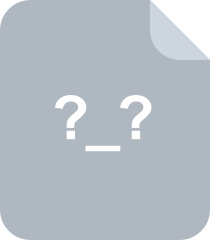
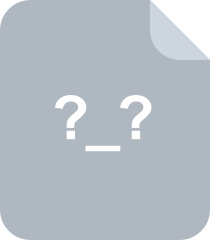
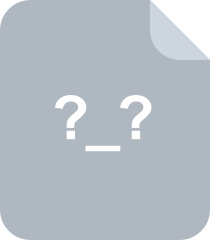
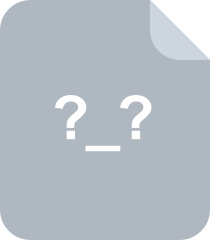
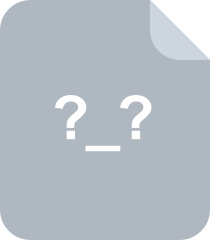
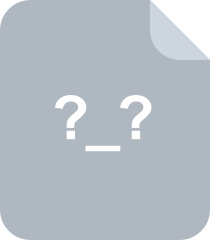
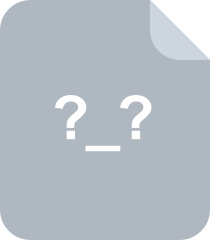
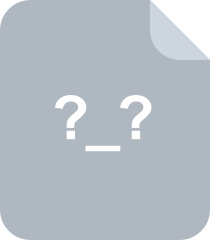
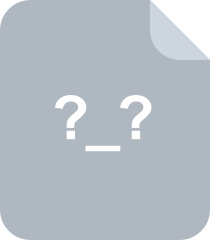
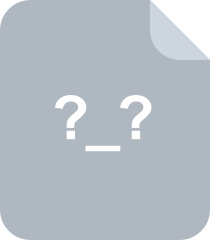
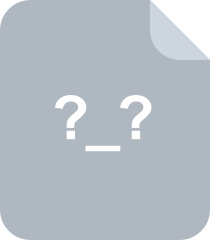
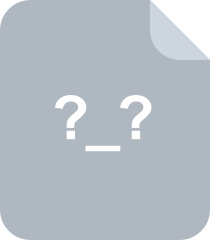
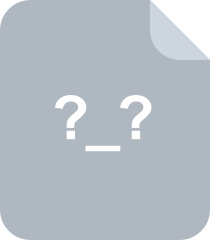
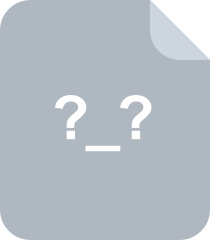
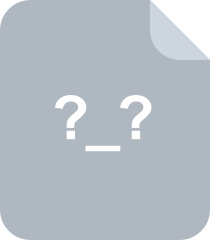
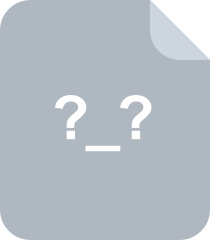
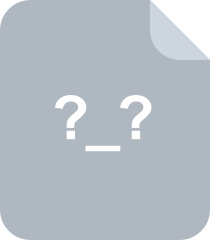
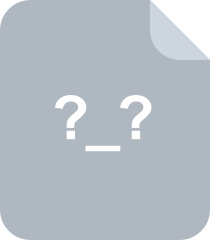
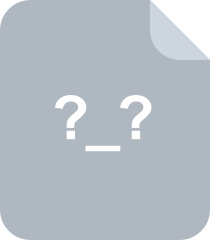
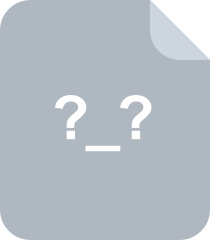
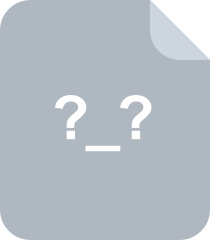
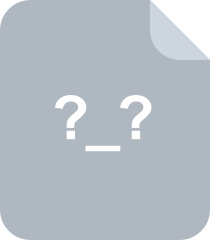
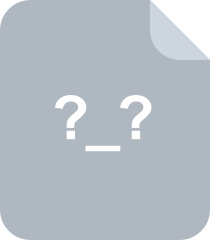
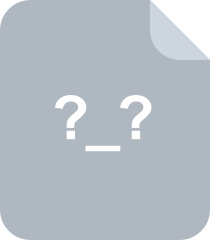
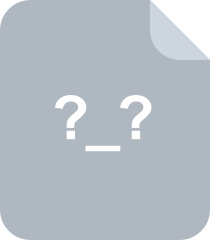
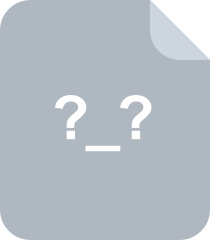
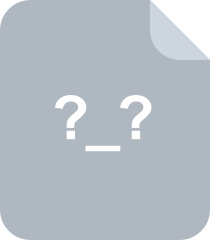
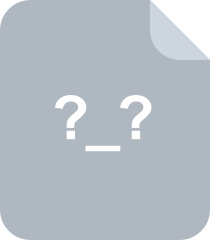
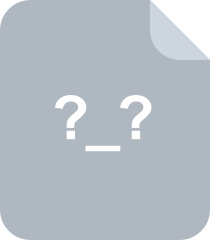
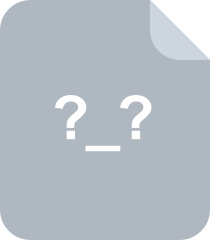
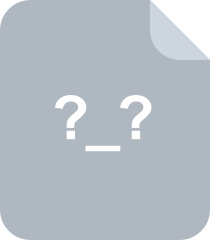
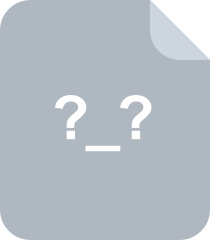
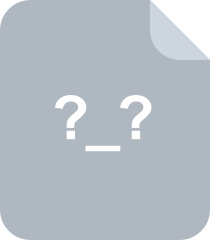
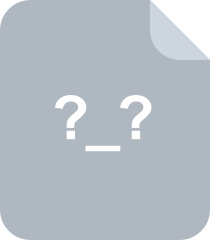
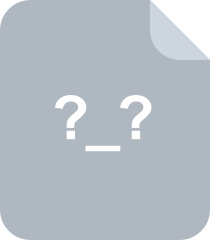
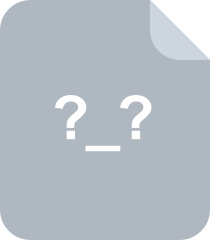
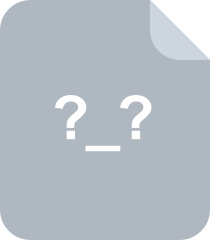
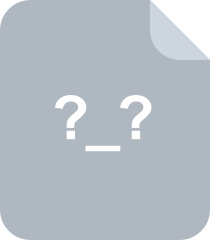
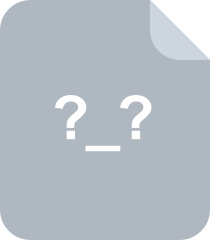
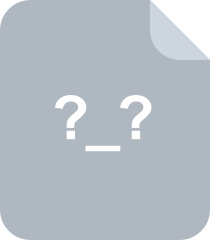
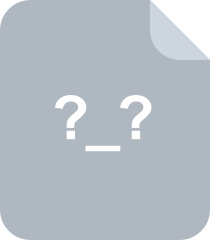
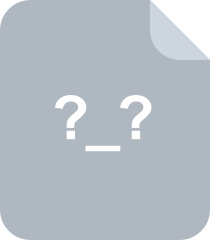
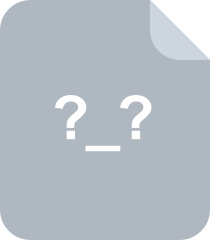
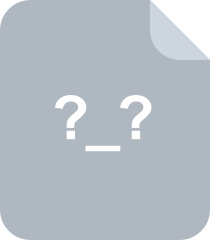
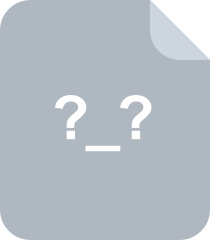
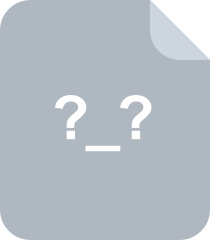
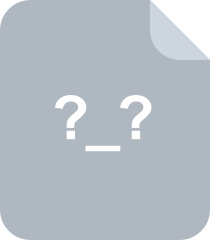
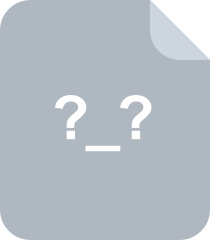
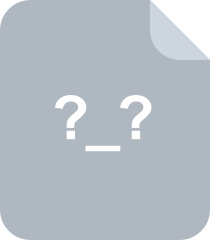
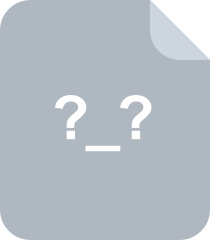
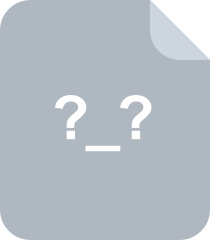
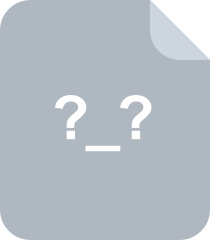
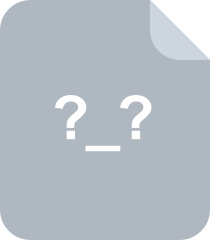
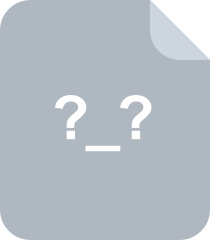
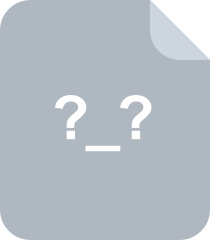
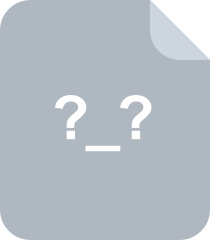
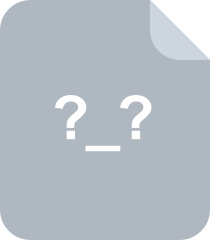
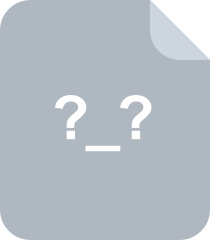
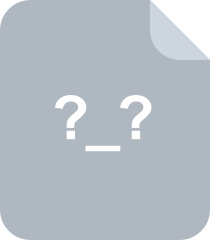
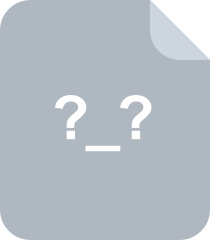
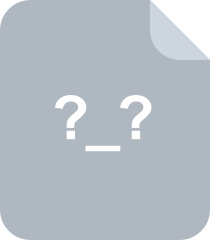
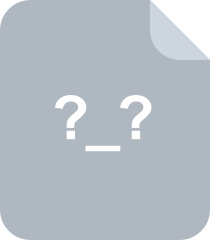
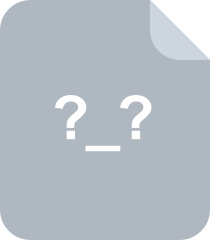
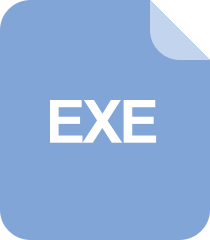
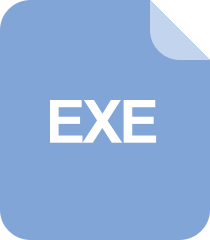
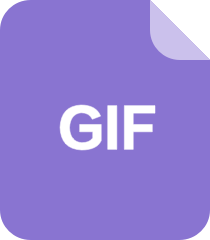
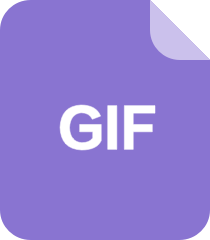
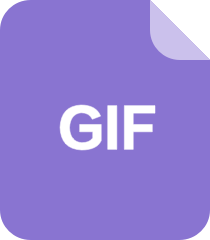
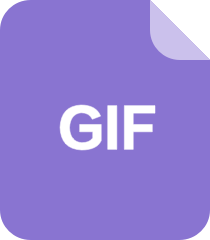
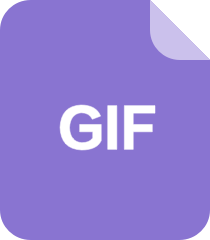
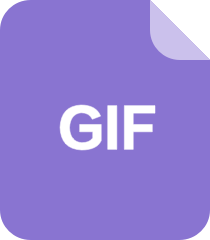
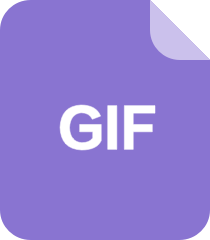
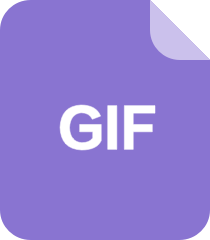
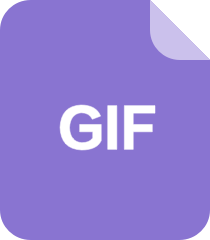
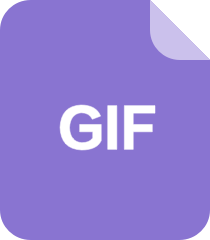
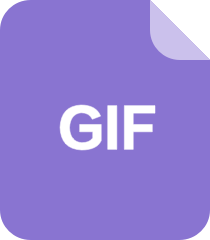
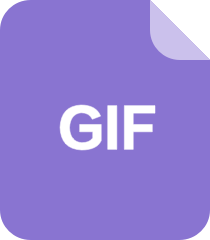
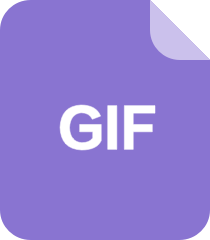
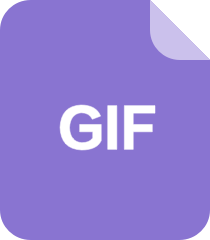
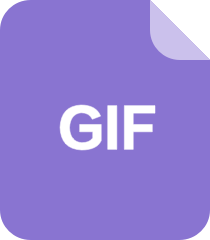
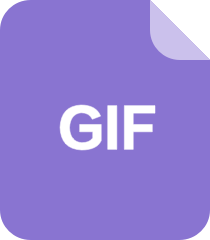
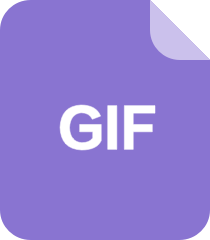
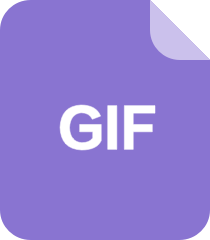
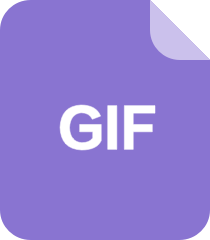
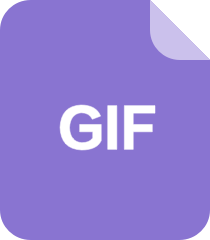
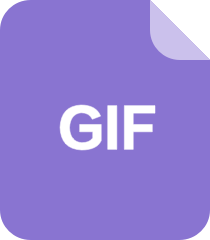
共 1257 条
- 1
- 2
- 3
- 4
- 5
- 6
- 13
资源评论
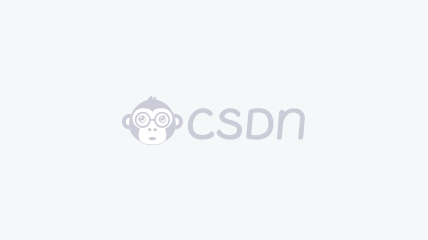

小杰jace
- 粉丝: 533
- 资源: 5
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

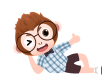
最新资源
- 使用NetBeans连接SQLserver2008数据库教程中文WORD版最新版本
- XPath实例中文WORD版最新版本
- XPath语法规则中文WORD版最新版本
- XPath入门教程中文WORD版最新版本
- ORACLE数据库管理系统体系结构中文WORD版最新版本
- Sybase数据库安装以及新建数据库中文WORD版最新版本
- tomcat6.0配置oracle数据库连接池中文WORD版最新版本
- hibernate连接oracle数据库中文WORD版最新版本
- MyEclipse连接MySQL的方法中文WORD版最新版本
- MyEclipse中配置Hibernate连接Oracle中文WORD版最新版本
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


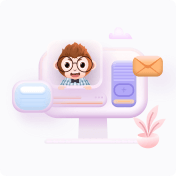
安全验证
文档复制为VIP权益,开通VIP直接复制
