/****************************************************************************
**
** Copyright (C) 2016 The Qt Company Ltd.
** Contact: https://www.qt.io/licensing/
**
** This file is part of the Qt Quick Controls module of the Qt Toolkit.
**
** $QT_BEGIN_LICENSE:LGPL$
** Commercial License Usage
** Licensees holding valid commercial Qt licenses may use this file in
** accordance with the commercial license agreement provided with the
** Software or, alternatively, in accordance with the terms contained in
** a written agreement between you and The Qt Company. For licensing terms
** and conditions see https://www.qt.io/terms-conditions. For further
** information use the contact form at https://www.qt.io/contact-us.
**
** GNU Lesser General Public License Usage
** Alternatively, this file may be used under the terms of the GNU Lesser
** General Public License version 3 as published by the Free Software
** Foundation and appearing in the file LICENSE.LGPL3 included in the
** packaging of this file. Please review the following information to
** ensure the GNU Lesser General Public License version 3 requirements
** will be met: https://www.gnu.org/licenses/lgpl-3.0.html.
**
** GNU General Public License Usage
** Alternatively, this file may be used under the terms of the GNU
** General Public License version 2.0 or (at your option) the GNU General
** Public license version 3 or any later version approved by the KDE Free
** Qt Foundation. The licenses are as published by the Free Software
** Foundation and appearing in the file LICENSE.GPL2 and LICENSE.GPL3
** included in the packaging of this file. Please review the following
** information to ensure the GNU General Public License requirements will
** be met: https://www.gnu.org/licenses/gpl-2.0.html and
** https://www.gnu.org/licenses/gpl-3.0.html.
**
** $QT_END_LICENSE$
**
****************************************************************************/
.pragma library
var daysInAWeek = 7;
var monthsInAYear = 12;
// Not the number of weeks per month, but the number of weeks that are
// shown on a typical calendar.
var weeksOnACalendarMonth = 6;
// Can't create year 1 directly...
var minimumCalendarDate = new Date(-1, 0, 1);
minimumCalendarDate.setFullYear(minimumCalendarDate.getFullYear() + 2);
var maximumCalendarDate = new Date(275759, 9, 25);
function daysInMonth(date) {
// Passing 0 as the day will give us the previous month, which will be
// date.getMonth() since we added 1 to it.
return new Date(date.getFullYear(), date.getMonth() + 1, 0).getDate();
}
/*!
Returns a copy of \a date with its month set to \a month, keeping the same
day if possible. Does not modify \a date.
*/
function setMonth(date, month) {
var oldDay = date.getDate();
var newDate = new Date(date);
// Set the day first, because setting the month could cause it to skip ahead
// a month if the day is larger than the latest day in that month.
newDate.setDate(1);
newDate.setMonth(month);
// We'd like to have the previous day still selected when we change
// months, but it might not be possible, so use the smallest of the two.
newDate.setDate(Math.min(oldDay, daysInMonth(newDate)));
return newDate;
}
/*!
Returns the cell rectangle for the cell at the given \a index, assuming
that the grid has a number of columns equal to \a columns and rows
equal to \a rows, with an available width of \a availableWidth and height
of \a availableHeight.
If \a gridLineWidth is greater than \c 0, the cell rectangle will be
calculated under the assumption that there is a grid between the cells:
31 | 1 | 2 | 3 | 4 | 5 | 6
--------------------------------
7 | 8 | 9 | 10 | 11 | 12 | 13
--------------------------------
14 | 15 | 16 | 17 | 18 | 19 | 20
--------------------------------
21 | 22 | 23 | 24 | 25 | 26 | 27
--------------------------------
28 | 29 | 30 | 31 | 1 | 2 | 3
--------------------------------
4 | 5 | 6 | 7 | 8 | 9 | 10
*/
function cellRectAt(index, columns, rows, availableWidth, availableHeight, gridLineWidth) {
var col = Math.floor(index % columns);
var row = Math.floor(index / columns);
var availableWidthMinusGridLines = availableWidth - ((columns - 1) * gridLineWidth);
var availableHeightMinusGridLines = availableHeight - ((rows - 1) * gridLineWidth);
var remainingHorizontalSpace = Math.floor(availableWidthMinusGridLines % columns);
var remainingVerticalSpace = Math.floor(availableHeightMinusGridLines % rows);
var baseCellWidth = Math.floor(availableWidthMinusGridLines / columns);
var baseCellHeight = Math.floor(availableHeightMinusGridLines / rows);
var rect = Qt.rect(0, 0, 0, 0);
rect.x = baseCellWidth * col;
rect.width = baseCellWidth;
if (remainingHorizontalSpace > 0) {
if (col < remainingHorizontalSpace) {
++rect.width;
}
// This cell's x position should be increased by 1 for every column above it.
rect.x += Math.min(remainingHorizontalSpace, col);
}
rect.y = baseCellHeight * row;
rect.height = baseCellHeight;
if (remainingVerticalSpace > 0) {
if (row < remainingVerticalSpace) {
++rect.height;
}
// This cell's y position should be increased by 1 for every row above it.
rect.y += Math.min(remainingVerticalSpace, row);
}
rect.x += col * gridLineWidth;
rect.y += row * gridLineWidth;
return rect;
}
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
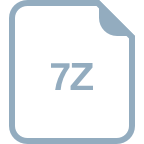
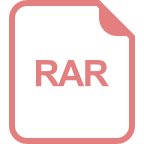
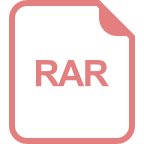
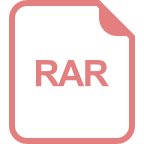
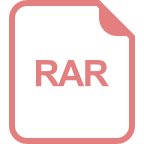
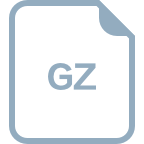
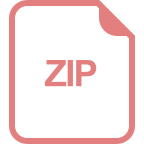
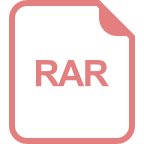
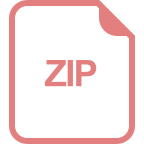
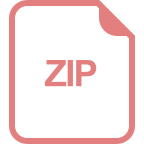
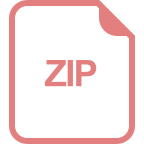
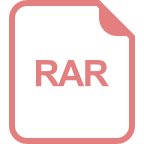
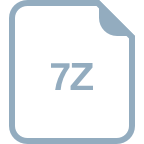
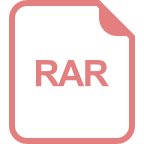
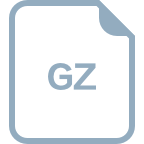
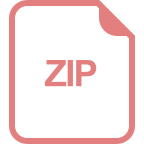
收起资源包目录

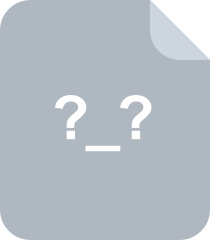
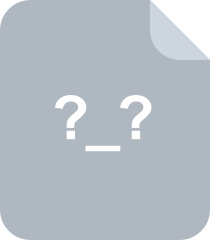
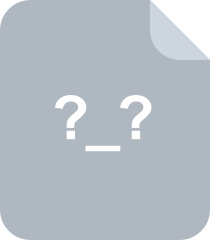
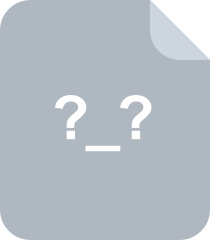
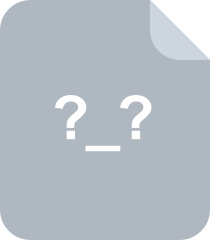
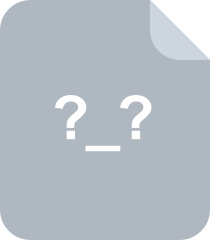
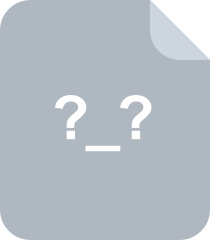
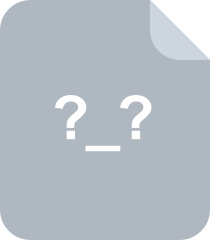
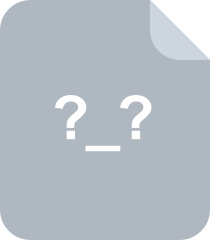
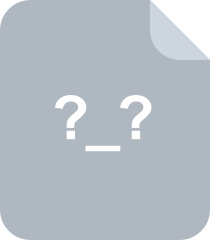
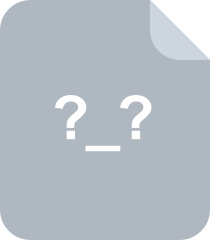
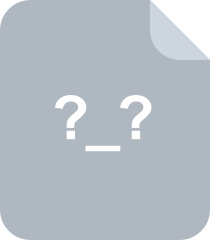
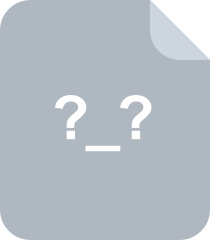
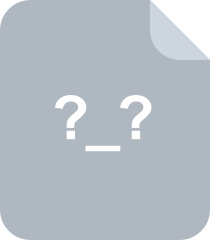
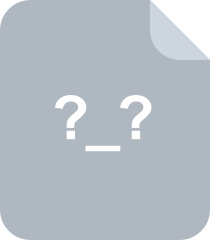
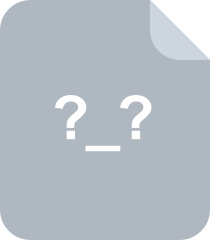
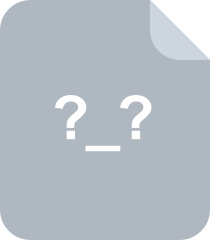
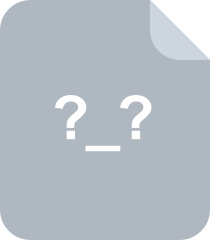
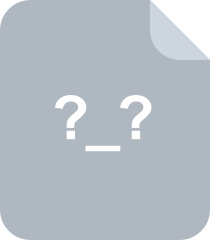
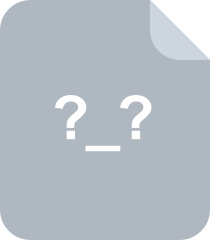
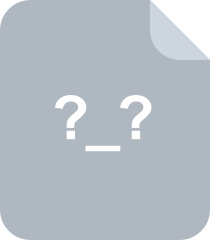
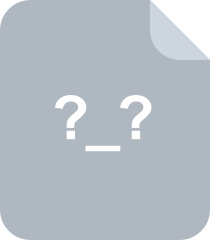
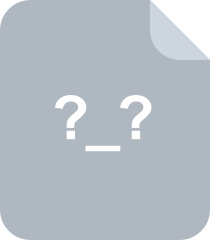
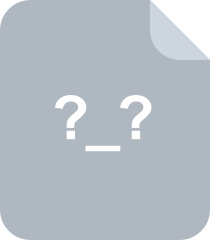
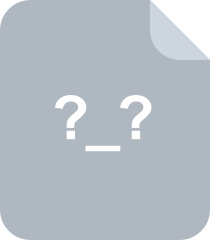
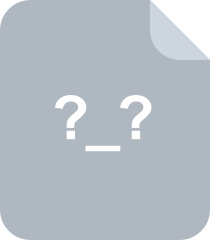
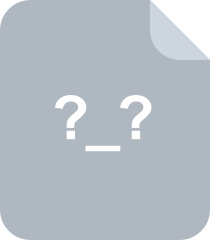
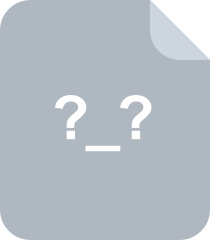
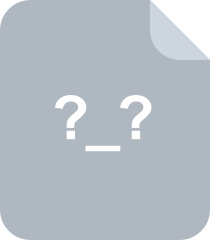
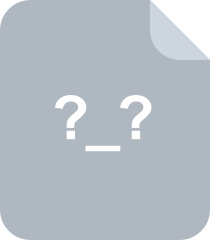
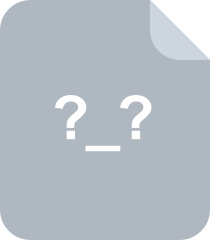
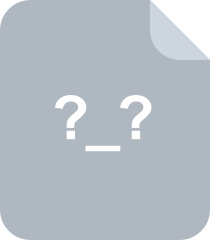
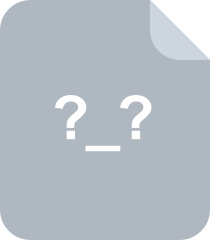
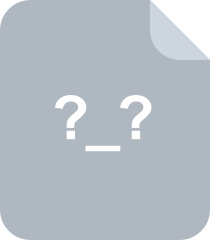
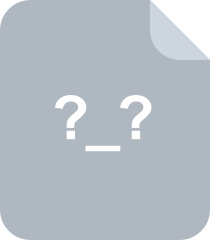
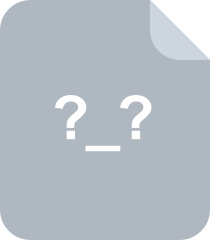
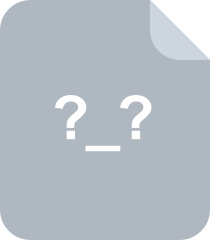
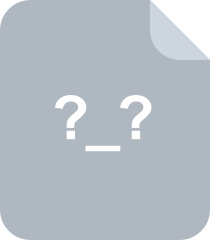
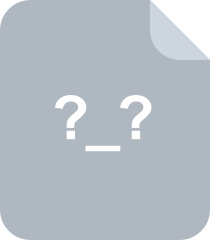
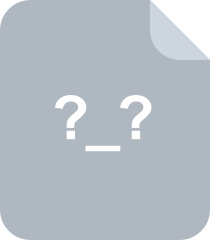
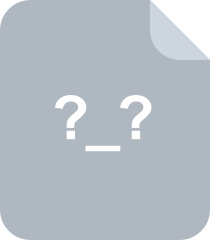
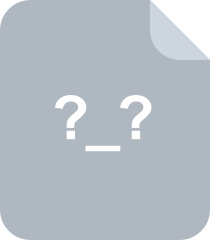
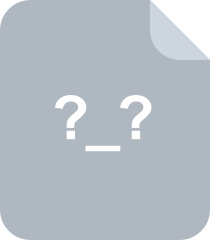
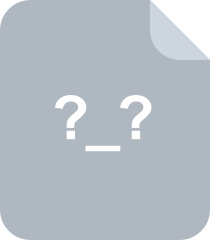
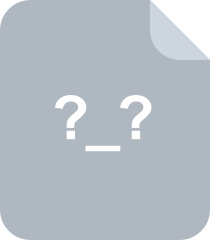
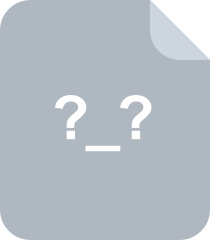
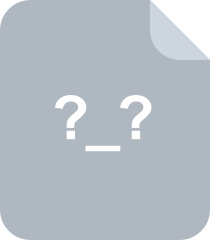
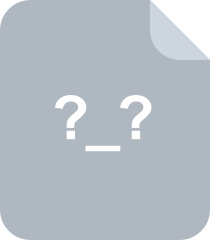
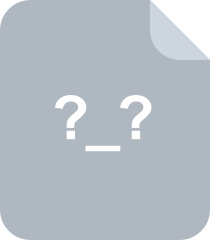
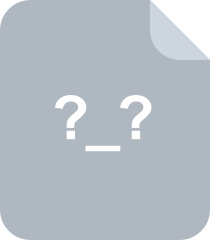
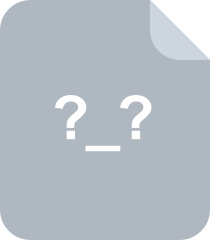
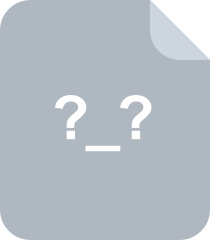
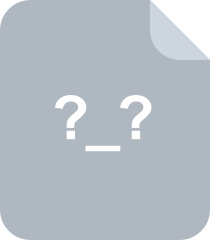
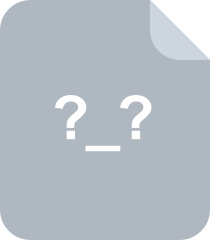
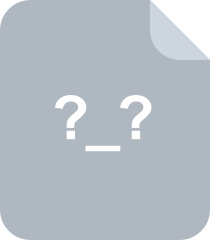
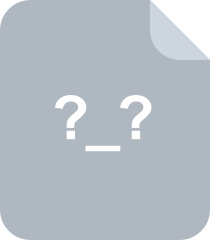
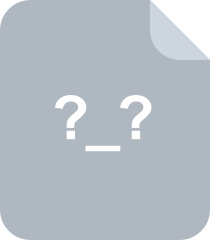
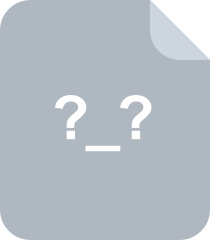
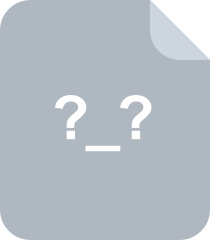
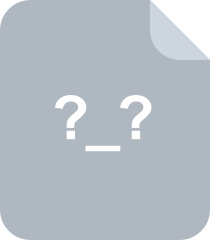
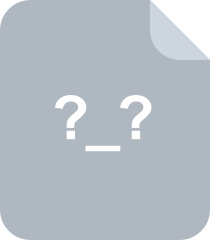
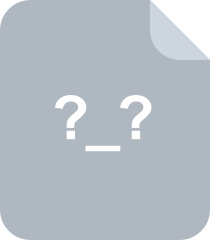
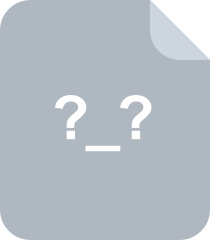
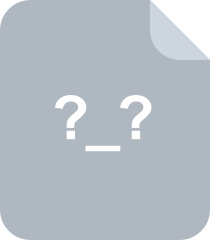
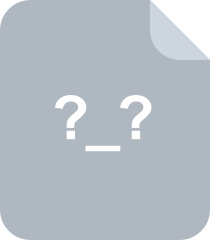
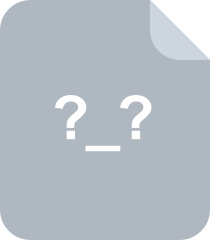
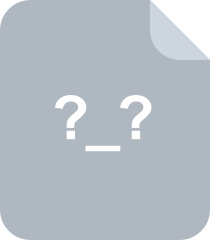
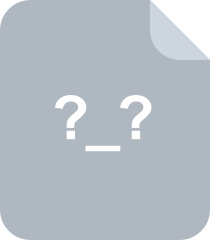
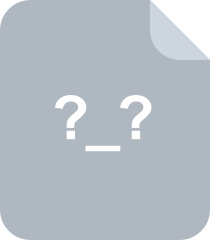
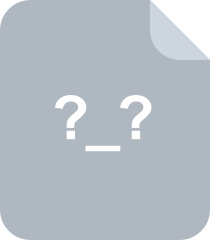
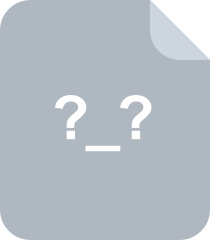
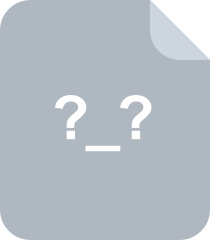
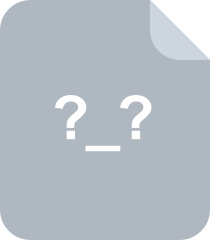
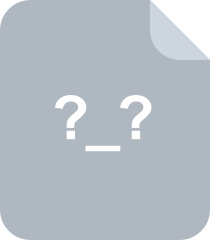
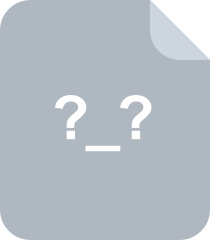
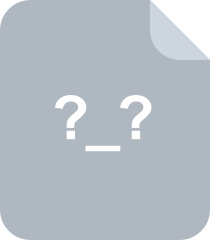
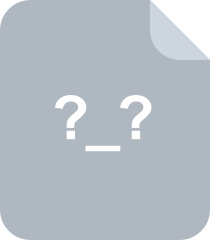
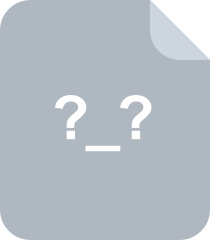
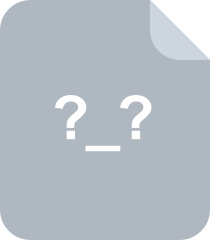
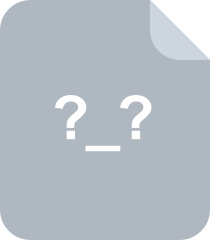
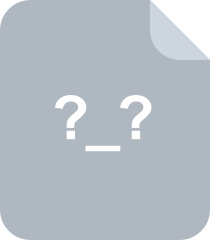
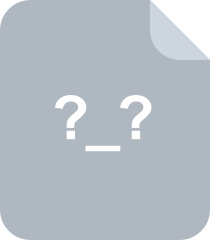
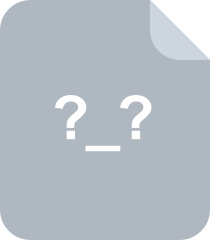
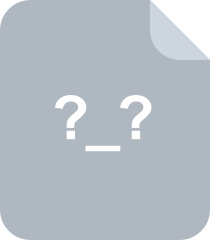
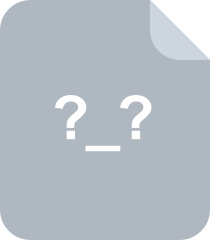
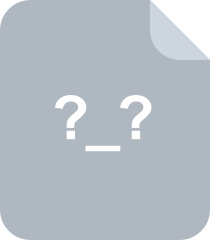
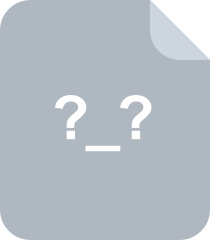
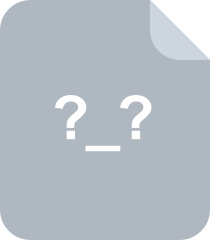
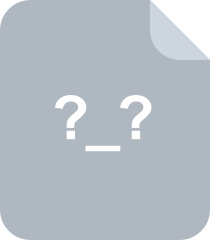
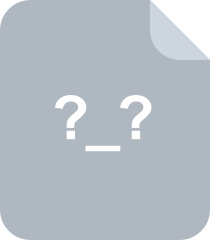
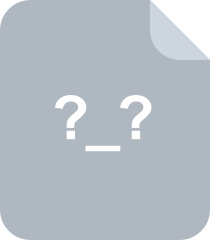
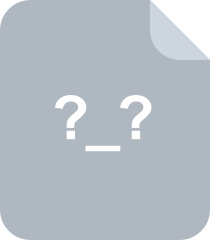
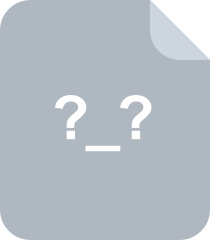
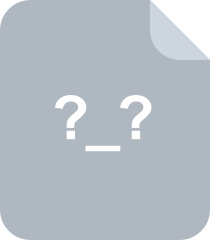
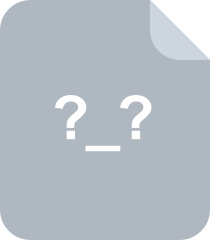
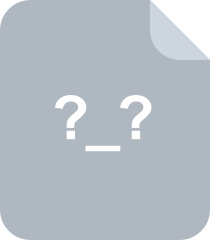
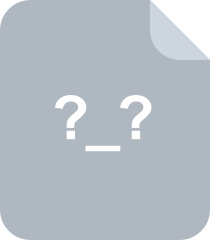
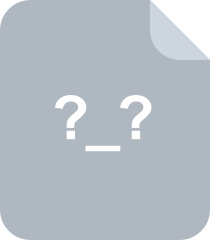
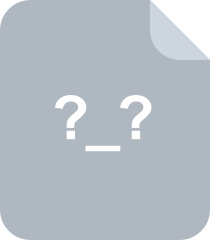
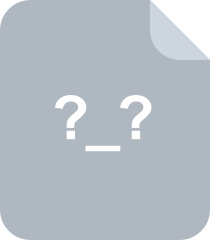
共 1389 条
- 1
- 2
- 3
- 4
- 5
- 6
- 14
资源评论
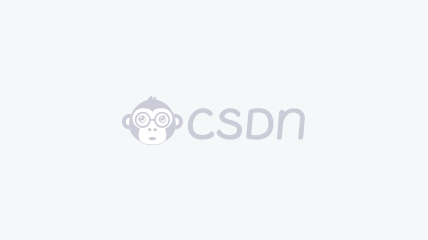


长沙红胖子Qt(技术Q群4597637)
- 粉丝: 14w+
- 资源: 218
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

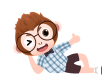
最新资源
- 36 -公司员工手册范本234.doc
- 46 -宏达利员工手册范本.doc
- 48 -华为员工手册-范本.doc
- 65 -美容院员工手册(范例).doc
- 63 -美容店员工手册范本.doc
- 69 -某公司员工手册-范本1.doc
- 76 -某食品公司连锁运营部员工守则.doc
- 75 -某软件公司员工手册.doc
- 83 -企业员工手册范本 (1).doc
- 103 -微软公司的员工手册.doc
- 154 -员工手册范本(公司类).doc
- 172 -员工手册和规章制度范本.doc
- 182 -云支付和云付通的员工手册.doc
- 185 -中小公司员工手册-经典.doc
- 190 -足疗店员工手册范本.doc
- 126 -员工手册 (1).docx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


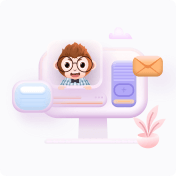
安全验证
文档复制为VIP权益,开通VIP直接复制
