/*
SLdm2318
QVertexBlendAnimation (Qt3DAnimation)
`QVertexBlendAnimation` 类是 Qt3DAnimation 模块中的一个类,用于在 Qt 3D 应用程序中实现顶点混合动画。顶点混合动画是一种在三维场景中控制模型表现形状和姿势的技术,通常用于实现角色动画、形变动画等效果。以下是关于 `QVertexBlendAnimation` 类的功能介绍:
1. **顶点混合动画**:`QVertexBlendAnimation` 类实现了顶点混合动画功能,可以通过控制顶点的权重和位置来实现模型的形变和动画效果。
2. **关键帧动画**:`QVertexBlendAnimation` 类支持关键帧动画,可以定义多个关键帧,每个关键帧包含不同的顶点权重和位置信息,从而实现平滑的动画过渡。
3. **权重调整**:`QVertexBlendAnimation` 类允许用户调整顶点的权重,从而控制不同部位的变形程度,实现更加自然和灵活的动画效果。
4. **动画控制**:`QVertexBlendAnimation` 类提供了控制动画播放速度、循环、暂停等功能,方便用户对顶点混合动画进行控制。
5. **与其他 Qt3D 类集成**:`QVertexBlendAnimation` 类与 Qt3D 中的其他类(如 `QMesh`、`QSkeleton` 等)紧密集成,可以方便地在 Qt3D 应用程序中实现复杂的顶点混合动画效果。
6. **性能优化**:`QVertexBlendAnimation` 类在实现顶点混合动画时做了性能优化,保证动画的流畅性和效率。
7. **易于使用**:`QVertexBlendAnimation` 类提供了简单易用的接口,方便开发人员在 Qt3D 应用程序中实现顶点混合动画效果。
总的来说,`QVertexBlendAnimation` 类是 Qt3DAnimation 模块中用于实现顶点混合动画的类,提供了丰富的功能和接口,方便开发人员在三维场景中实现复杂的角色动画、形变动画等效果。希望这些信息对您有所帮助!如果您有任何其他问题,请随时告诉我。我很乐意为您提供帮助。
*/
#include <Qt3DAnimation/QVertexBlendAnimation>
#include <Qt3DCore/QEntity>
#include <Qt3DCore/QTransform>
#include <Qt3DExtras/QPhongMaterial>
#include <Qt3DExtras/QSphereMesh>
#include <Qt3DRender/QCamera>
#include <Qt3DRender/QPointLight>
#include <Qt3DRender/QRenderAspect>
#include <Qt3DRender/QViewport>
#include <Qt3DRender/QRenderSettings>
#include <Qt3DExtras/QFirstPersonCameraController>
#include <Qt3DExtras/Qt3DWindow>
int main(int argc, char *argv[])
{
// 创建 Qt3D 应用程序
QGuiApplication app(argc, argv);
Qt3DExtras::Qt3DWindow view;
// 创建 Qt3D 实体
Qt3DCore::QEntity *rootEntity = new Qt3DCore::QEntity();
view.setRootEntity(rootEntity);
// 创建一个球体网格和材质
Qt3DCore::QEntity *sphereEntity = new Qt3DCore::QEntity(rootEntity);
Qt3DExtras::QSphereMesh *sphereMesh = new Qt3DExtras::QSphereMesh();
sphereMesh->setRadius(1.0f);
Qt3DExtras::QPhongMaterial *sphereMaterial = new Qt3DExtras::QPhongMaterial();
sphereEntity->addComponent(sphereMesh);
sphereEntity->addComponent(sphereMaterial);
// 创建顶点混合动画
Qt3DAnimation::QVertexBlendAnimation *vertexBlendAnimation = new Qt3DAnimation::QVertexBlendAnimation(rootEntity);
vertexBlendAnimation->setDuration(2000);
vertexBlendAnimation->setLoopCount(-1); // 无限循环
// 设置动画目标对象和属性
vertexBlendAnimation->setTarget(sphereEntity);
vertexBlendAnimation->setPropertyName("vertexBlend");
// 添加动画插值
QVariantAnimation *animation = new QVariantAnimation();
animation->setKeyValues({0.0f, 1.0f});
animation->setDuration(2000);
vertexBlendAnimation->addAnimation(animation);
// 设置摄像机和视图参数
Qt3DRender::QCamera *cameraEntity = view.camera();
cameraEntity->lens()->setPerspectiveProjection(45.0f, 16.0f/9.0f, 0.1f, 100.0f);
cameraEntity->setPosition(QVector3D(0, 0, 10));
cameraEntity->setUpVector(QVector3D(0, 1, 0));
cameraEntity->setViewCenter(QVector3D(0, 0, 0));
// 创建光源
Qt3DRender::QPointLight *lightEntity = new Qt3DRender::QPointLight(rootEntity);
lightEntity->setColor("white");
lightEntity->setIntensity(1);
lightEntity->setLinearAttenuation(0.01);
rootEntity->addComponent(lightEntity);
// 创建渲染设置
Qt3DRender::QRenderSettings *renderSettings = view.renderSettings();
Qt3DRender::QRenderAspect *renderAspect = new Qt3DRender::QRenderAspect();
renderSettings->setActiveFrameGraph(renderAspect);
// 创建第一人称摄像机控制器
Qt3DExtras::QFirstPersonCameraController *camController = new Qt3DExtras::QFirstPersonCameraController(rootEntity);
camController->setCamera(cameraEntity);
// 显示窗口
view.show();
return app.exec();
}


徐子宸
- 粉丝: 113
- 资源: 35
最新资源
- 【创新无忧】基于鹈鹕优化算法POA优化相关向量机RVM实现北半球光伏数据预测附matlab代码.rar
- 【创新无忧】基于天鹰优化算法AO优化广义神经网络GRNN实现电机故障诊断附matlab代码.rar
- 【创新无忧】基于天鹰优化算法AO优化广义神经网络GRNN实现数据回归预测附matlab代码.rar
- 【创新无忧】基于天鹰优化算法AO优化广义神经网络GRNN实现光伏预测附matlab代码.rar
- 【创新无忧】基于天鹰优化算法AO优化极限学习机KELM实现故障诊断附matlab代码.rar
- 【创新无忧】基于天鹰优化算法AO优化相关向量机RVM实现北半球光伏数据预测附matlab代码.rar
- 【创新无忧】基于天鹰优化算法AO优化极限学习机ELM实现乳腺肿瘤诊断附matlab代码.rar
- 【创新无忧】基于秃鹰优化算法BES优化广义神经网络GRNN实现电机故障诊断附matlab代码.rar
- 【创新无忧】基于天鹰优化算法AO优化相关向量机RVM实现数据多输入单输出回归预测附matlab代码.rar
- 【创新无忧】基于秃鹰优化算法BES优化广义神经网络GRNN实现光伏预测附matlab代码.rar
- 【创新无忧】基于秃鹰优化算法BES优化广义神经网络GRNN实现数据回归预测附matlab代码.rar
- 【创新无忧】基于秃鹰优化算法BES优化极限学习机ELM实现乳腺肿瘤诊断附matlab代码.rar
- 【创新无忧】基于秃鹰优化算法BES优化极限学习机KELM实现故障诊断附matlab代码.rar
- 【创新无忧】基于秃鹰优化算法BES优化相关向量机RVM实现北半球光伏数据预测附matlab代码.rar
- 【创新无忧】基于秃鹰优化算法BES优化相关向量机RVM实现数据多输入单输出回归预测附matlab代码.rar
- 【创新无忧】基于雾凇优化算法RIME优化广义神经网络GRNN实现光伏预测附matlab代码.rar
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


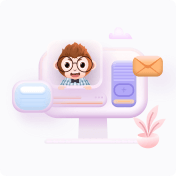