### C#实现文件压缩知识点详解 #### 一、概述 在C#开发中,文件压缩是一项常见的需求,尤其是在处理大量文件或需要节省存储空间时。本文档将详细讲解如何使用C#来压缩多个文件到一个ZIP文件中,特别强调的是只压缩指定文件夹中的文件而不包括子文件夹中的文件。 #### 二、核心代码解析 我们来看一下关键的代码片段: ```csharp using System; using System.Collections.Generic; using System.IO; using System.IO.Compression; namespace Test { public class MoreZipFile { /// <summary> /// Compresses files from a source folder into a single ZIP file without including subfolders. /// </summary> /// <param name="sourceFolder">The location of the files to include in the ZIP file (excluding subfolders).</param> /// <param name="destFolder">Folder to write the ZIP file into.</param> /// <param name="zipFileName">Name of the ZIP file to write.</param> /// <returns>A GZipResult object containing the compression result.</returns> public static GZipResult CompressWithoutSubfolders(string sourceFolder, string destFolder, string zipFileName) { return Compress(sourceFolder, "*.*", SearchOption.TopDirectoryOnly, destFolder, zipFileName, true); } /// <summary> /// Compresses files based on search pattern and options. /// </summary> /// <param name="sourceFolder">The location of the files to include in the ZIP file.</param> /// <param name="searchPattern">Search pattern (e.g., "*.*" or "*.txt" or "*.gif") to identify what files in sourceFolder to include in the ZIP file.</param> /// <param name="searchOption">Only files in sourceFolder or include files in subfolders also.</param> /// <param name="destFolder">Folder to write the ZIP file into.</param> /// <param name="zipFileName">Name of the ZIP file to write.</param> /// <param name="deleteTempFile">Boolean, true deletes the intermediate temp file, false leaves the temp file in destFolder (for debugging).</param> /// <returns>A GZipResult object containing the compression result.</returns> public static GZipResult Compress(string sourceFolder, string searchPattern, SearchOption searchOption, string destFolder, string zipFileName, bool deleteTempFile) { DirectoryInfo di = new DirectoryInfo(sourceFolder); FileInfo[] files = di.GetFiles(searchPattern, searchOption); return Compress(files, sourceFolder, destFolder, zipFileName, deleteTempFile); } /// <summary> /// Compresses an array of FileInfo objects into a ZIP file. /// </summary> /// <param name="files">Array of FileInfo objects to be included in the ZIP file.</param> /// <param name="sourceFolder">The original folder where the files are located.</param> /// <param name="destFolder">Folder to write the ZIP file into.</param> /// <param name="zipFileName">Name of the ZIP file to write.</param> /// <param name="deleteTempFile">Boolean, true deletes the intermediate temp file, false leaves the temp file in destFolder (for debugging).</param> /// <returns>A GZipResult object containing the compression result.</returns> public static GZipResult Compress(FileInfo[] files, string sourceFolder, string destFolder, string zipFileName, bool deleteTempFile) { // Implementation details for compressing the files into a ZIP archive... } } /// <summary> /// Represents the result of a file compression operation. /// </summary> public class GZipResult { public bool Success { get; set; } public string ErrorMessage { get; set; } public string ZipFilePath { get; set; } } } ``` #### 三、知识点分析 ##### 1. 使用`System.IO.Compression`命名空间 C#提供了内置的支持来处理文件压缩和解压缩操作,这些功能主要包含在`System.IO.Compression`命名空间中。在这个示例中,我们没有直接使用这个命名空间中的类来进行压缩,而是通过自定义的方法来封装压缩逻辑。 ##### 2. `DirectoryInfo` 和 `FileInfo` 类 - **`DirectoryInfo`**: 用于获取目录的信息。 - **`FileInfo`**: 用于获取文件的信息。 这两个类提供了丰富的属性和方法来操作文件系统。 ##### 3. 文件搜索模式和选项 - **搜索模式**:如 `"*.*"` 表示搜索所有类型的文件,`"*.txt"` 表示仅搜索文本文件等。 - **搜索选项**:`SearchOption.AllDirectories` 表示递归搜索所有子目录;`SearchOption.TopDirectoryOnly` 表示只搜索当前目录。 在此示例中,为了只压缩顶层文件,使用了`SearchOption.TopDirectoryOnly`。 ##### 4. 压缩结果对象 为了更好地返回压缩结果,定义了一个`GZipResult`类,该类包含了压缩是否成功、错误消息(如果有的话)以及最终ZIP文件的路径等信息。 ##### 5. 文件压缩实现 实际的文件压缩逻辑并未在提供的代码中给出,但在实际应用中,可以使用`ZipFile.CreateFromDirectory`方法来创建ZIP文件,或者使用`ZipArchive`类进行更高级的操作。 #### 四、总结 本文详细介绍了如何使用C#来实现文件压缩的功能,并且特别关注了如何只压缩指定文件夹中的文件而不包括子文件夹中的文件。通过使用`System.IO`和`System.IO.Compression`命名空间,我们可以轻松地实现这一目标。此外,还定义了一个自定义的结果类来方便地返回压缩操作的结果信息。
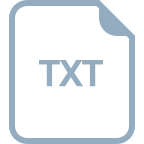
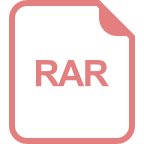
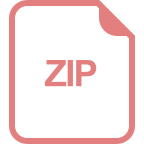
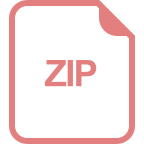
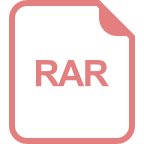
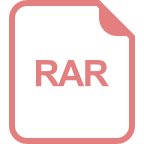
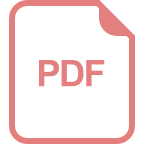
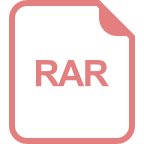
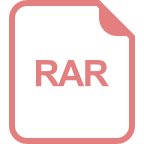
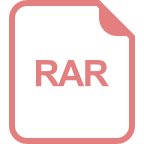
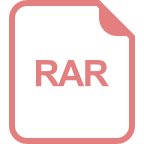
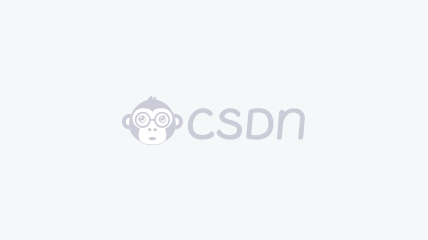
- yellowabul2014-05-02挺不错的,相比于我写的
- wolipengbo2013-08-21不错不错,非常有用
- nycgwqd2013-06-25还不错,有参考价值
- nianduhao2014-01-21有参考价值,谢谢分享

- 粉丝: 0
- 资源: 4
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

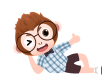
最新资源

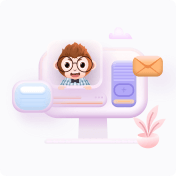
