### Unity3D图片滑动效果实现详解 #### 一、概述 在Unity3D开发过程中,实现图片滑动效果是一项常见的需求,特别是在制作游戏UI时。本文将通过一个具体的示例代码来详细介绍如何在Unity3D中实现图片的滑动效果,并对其中的关键技术和参数进行解析。 #### 二、关键概念和技术 1. **GUI系统**:Unity中的GUI系统提供了一系列工具和API用于创建用户界面,如按钮、文本框等。 2. **Touch输入**:用于检测触摸屏上的用户输入事件,例如触摸开始、移动和结束。 3. **ScrollView**:GUI系统中的滚动视图组件,可以用来创建可滚动的界面区域。 4. **物理与数学函数**:Unity提供了丰富的物理引擎支持以及数学计算函数,用于处理各种动态效果。 #### 三、示例代码分析 本节将对提供的代码进行详细解读: ```csharp using UnityEngine; using System.Collections; public class TestUI : MonoBehaviour { public Vector2 scrollPosition = Vector2.zero; public float scrollVelocity = 0f; public float timeTouchPhaseEnded = 0f; public float inertiaDuration = 0.5f; public Vector2 lastDeltaPos; // 初始化 void Start() {} void OnGUI() { scrollPosition = GUI.BeginScrollView(new Rect(100, 40, 600, 400), scrollPosition, new Rect(0, 0, 500, 1600), false, true); for (int i = 0; i < 32; i++) { GUI.Button(new Rect(0, i * 50, 400, 50), "Button" + i); } GUI.EndScrollView(); } // 每帧调用一次 void Update() { if (Input.touchCount > 0) { if (Input.GetTouch(0).phase == TouchPhase.Moved) { scrollPosition.y += Input.GetTouch(0).deltaPosition.y; lastDeltaPos = Input.GetTouch(0).deltaPosition; } else if (Input.GetTouch(0).phase == TouchPhase.Ended) { print("End:" + lastDeltaPos.y + "|" + Input.GetTouch(0).deltaTime); if (Mathf.Abs(lastDeltaPos.y) > 20.0f) { scrollVelocity = (int)(lastDeltaPos.y * 0.5 / Input.GetTouch(0).deltaTime); print(scrollVelocity); } timeTouchPhaseEnded = Time.time; } } else { if (scrollVelocity != 0.0f) { // 减速 float t = (Time.time - timeTouchPhaseEnded) / inertiaDuration; float frameVelocity = Mathf.Lerp(scrollVelocity, 0, t); scrollPosition.y += frameVelocity * Time.deltaTime; if (t >= inertiaDuration) scrollVelocity = 0; } } } } ``` ##### 1. 变量定义 - **scrollPosition**:存储当前滚动位置的二维向量。 - **scrollVelocity**:记录滚动速度。 - **timeTouchPhaseEnded**:记录触摸结束的时间。 - **inertiaDuration**:惯性持续时间。 - **lastDeltaPos**:记录最后一次触摸移动的距离。 ##### 2. 方法详解 - **Start()**:初始化方法,在脚本被加载时执行。 - **OnGUI()**:在每帧绘制GUI元素时调用的方法。 - 调用`GUI.BeginScrollView`创建一个可滚动的区域,参数包括:滚动视图的位置、大小、内容区域大小以及是否允许水平/垂直滚动。 - 循环创建按钮,并设置其位置。 - 使用`GUI.EndScrollView()`关闭滚动视图。 - **Update()**:每帧调用一次,用于处理逻辑更新。 - 检测触摸事件: - 如果检测到触摸并且处于移动阶段,则更新滚动位置。 - 如果触摸结束,根据移动距离计算滚动速度,并记录时间。 - 如果没有触摸输入且存在滚动速度,则模拟惯性效果。 #### 四、扩展技术 除了上述基础实现外,还可以进一步扩展功能,比如: - **多点触控支持**:处理多指同时滑动的情况。 - **边界限制**:防止滚动超出边界。 - **平滑滚动**:使用平滑算法优化滚动体验。 #### 五、总结 本文详细介绍了如何在Unity3D中实现图片滑动效果,并通过具体代码示例对关键技术点进行了讲解。掌握这些技术对于提升Unity项目的交互性和用户体验有着重要意义。希望本文能帮助开发者更好地理解和应用这些技术。
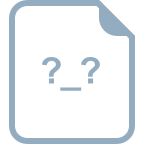
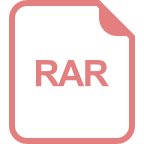
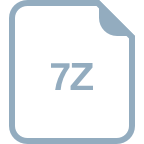
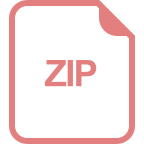
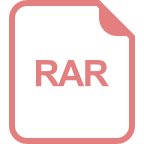
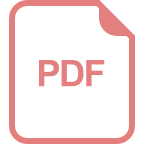
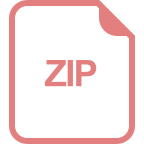
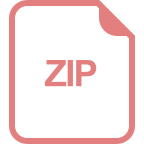
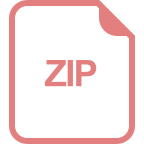
using System.Collections;
public class TestUI : MonoBehaviour {
public Vector2 scrollPosition = Vector2.zero;
public float scrollVelocity = 0f;
public float timeTouchPhaseEnded = 0f;
public float inertiaDuration = 0.5f;
public Vector2 lastDeltaPos;
// Use this for initialization
void Start () {
}
void OnGUI()
{
scrollPosition = GUI.BeginScrollView(new Rect(100, 40, 600, 400), scrollPosition, new Rect(0, 0, 500, 1600), false, true);
for (int i = 0; i < 32; i++)
{
GUI.Button(new Rect(0, i*50, 400, 50), "Button"+i);
}
GUI.EndScrollView();
}
// Update is called once per frame

- 粉丝: 0
- 资源: 6
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

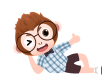
最新资源

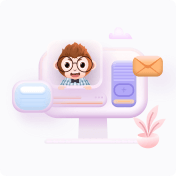

- 1
- 2
前往页