Qt中用qwt实现动态绘制二维曲线
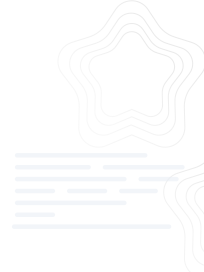


在Qt开发环境中,Qwt库是一个非常实用的工具,它为开发者提供了丰富的科学绘图功能,包括绘制二维曲线、图表等。本篇文章将详细介绍如何在Qt 5.5.1中利用Qwt 6.1.2实现动态、实时的二维曲线绘制,以及如何实现坐标轴缩放和曲线缩放功能。 你需要安装Qwt库。通常,你可以从Qwt官网下载源代码,然后在Qt环境中配置编译。确保你的Qt版本与Qwt版本兼容。在完成编译和安装后,将Qwt库添加到你的项目.pro文件中,例如: ```pro QT += core gui greaterThan(QT_MAJOR_VERSION, 4): QT += widgets TARGET = 2DPlot CONFIG += console CONFIG -= app_bundle CONFIG += qwt SOURCES += main.cpp \ widget.cpp HEADERS += widget.h FORMS += widget.ui ``` 接着,创建一个QWidget子类,用于绘制曲线。在`widget.h`中定义这个类,包括必要的成员变量和方法: ```cpp #include <QWidget> #include <QwtPlot> #include <QwtPlotCurve> class Widget : public QWidget { Q_OBJECT public: Widget(QWidget *parent = nullptr); ~Widget(); private: QwtPlot *plot; QwtPlotCurve *curve; private slots: void replot(); }; ``` 在`widget.cpp`中实现类的构造函数和方法: ```cpp Widget::Widget(QWidget *parent) : QWidget(parent) { plot = new QwtPlot(this); plot->setCanvasBackground(Qt::white); curve = new QwtPlotCurve("Curve"); curve->setPen(QPen(Qt::blue, 2, Qt::SolidLine)); // 添加曲线到图例 plot->legend()->addEntry(curve, curve->title().text()); // 设置坐标轴标签 plot->axisTitle(QwtPlot::xBottom)->setText("X Axis"); plot->axisTitle(QwtPlot::yLeft)->setText("Y Axis"); // 将曲线添加到绘图区域 curve->attach(plot); // 设置坐标轴范围 plot->setAxisScale(QwtPlot::xBottom, 0, 100); plot->setAxisScale(QwtPlot::yLeft, 0, 100); // 设置坐标轴缩放行为 plot->setAxisAutoScale(QwtPlot::xBottom, true); plot->setAxisAutoScale(QwtPlot::yLeft, true); // 布局调整 plot->insertLegend(plot->legend(), QwtPlot::TopRight); // 连接信号与槽,实时更新曲线 connect(plot, SIGNAL(xAxisAutoScaleChanged()), this, SLOT(replot())); connect(plot, SIGNAL(yAxisAutoScaleChanged()), this, SLOT(replot())); setCentralWidget(plot); } Widget::~Widget() { delete plot; delete curve; } void Widget::replot() { // 更新曲线数据并重绘 curve->setSamples(// 这里根据实际情况填充数据点); plot->replot(); } ``` 现在,你可以实现实时数据更新。在`main.cpp`中,创建一个`Widget`实例并模拟数据生成,不断调用`replot()`方法更新曲线: ```cpp #include "widget.h" int main(int argc, char *argv[]) { QApplication app(argc, argv); Widget w; w.show(); // 模拟实时数据更新 QTimer *timer = new QTimer(&w); connect(timer, &QTimer::timeout, &w, &Widget::replot); timer->start(1000); // 每秒更新一次 return app.exec(); } ``` 为了实现鼠标滚轮缩放功能,你需要监听滚轮事件并调用`QwtPlot`的相应方法。在`widget.cpp`中添加以下代码: ```cpp bool Widget::eventFilter(QObject *obj, QEvent *event) { if (event->type() == QEvent::Wheel && obj == plot) { QWheelEvent *wheelEvent = static_cast<QWheelEvent*>(event); if (wheelEvent->modifiers().testFlag(Qt::ControlModifier)) { if (wheelEvent->delta() > 0) { plot->zoomIn(); } else { plot->zoomOut(); } } else { // 滚动垂直坐标轴 plot->setAxisScale(QwtPlot::yLeft, plot->axisScaleDiv(QwtPlot::yLeft).lowerBound() - wheelEvent->delta() / 100.0, plot->axisScaleDiv(QwtPlot::yLeft).upperBound()); } event->accept(); return true; } return QWidget::eventFilter(obj, event); } void Widget::installEventFilter() { plot->installEventFilter(this); } ``` 在`Widget`构造函数中调用`installEventFilter()`,使事件过滤器生效。 通过以上步骤,你已经成功实现了在Qt中利用Qwt绘制动态二维曲线的功能,同时也支持了坐标轴和曲线的滚轮缩放。这使得你可以方便地在Qt应用程序中展示实时数据变化,适用于各种科学计算、数据分析或监控系统。记得根据实际需求调整数据生成和更新逻辑,以及坐标轴的范围和缩放行为。
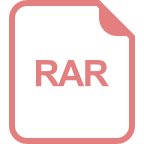
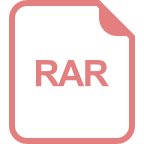
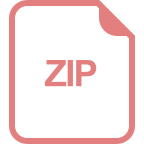
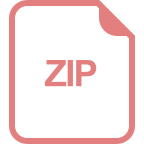
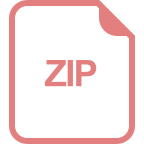
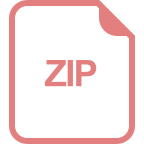
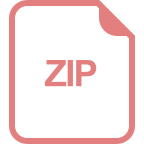
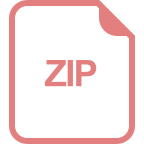
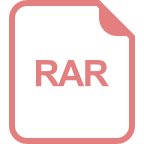
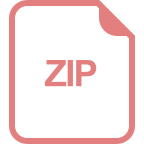
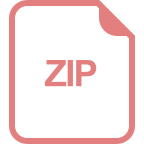
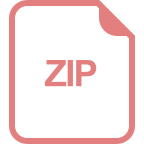


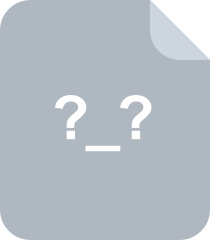
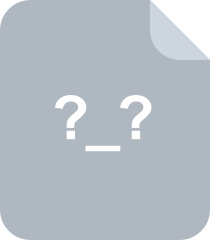
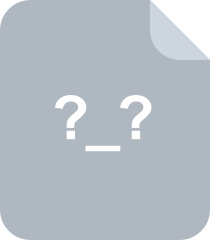
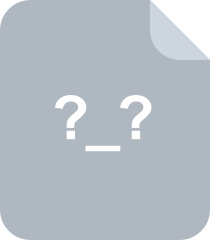
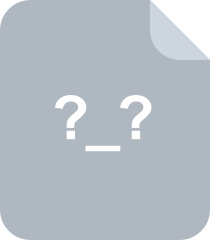
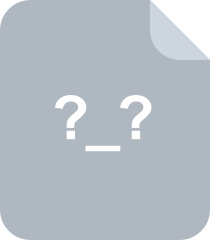
- 1
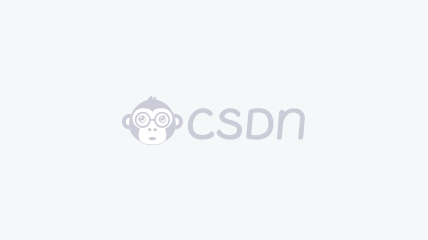
- one-rabbit2020-08-14实时,坐标轴为时间;先不说能不能运行;并不是按时间更新的,有出入

- 粉丝: 0
- 资源: 2
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

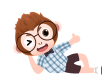
最新资源

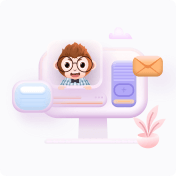
