在IT领域,文件传输是日常工作中非常常见的任务。本文将深入探讨如何实现“文件传输代码”,特别是基于TCP协议的简单实现。TCP(Transmission Control Protocol)是一种面向连接的、可靠的、基于字节流的传输层通信协议,非常适合用于文件传输。 理解TCP的基本原理至关重要。TCP通过三次握手建立连接,确保双方都有能力进行数据交换。然后,数据被分割成多个数据段,并通过确认机制确保数据的正确接收。通过四次挥手断开连接。在文件传输中,TCP提供了一种保证数据完整性的机制,因为即使在网络不稳定的情况下,也能通过重传机制确保文件的无损传输。 要实现简单的TCP文件传输,我们首先需要创建一个服务器端,它监听特定的端口,等待客户端的连接请求。在服务器端,我们需要使用socket编程接口来创建一个套接字,绑定到指定的IP地址和端口号,并调用listen()函数开始监听。一旦有客户端连接,accept()函数会返回一个新的套接字用于与该客户端进行通信。 在客户端,同样需要创建一个socket对象,并使用connect()函数连接到服务器的IP地址和端口号。连接成功后,客户端可以开始读取本地文件内容并发送到服务器。文件内容通常需要按字节流进行处理,以便通过TCP套接字发送。 在服务器端,接收文件时,需要持续接收来自客户端的数据,直到文件传输完毕。可以设定一个缓冲区大小,每次读取缓冲区大小的数据,然后写入到本地文件。为了确定文件传输的结束,可以约定一个特殊的结束标记,或者预先知道文件的大小,在接收完预期的字节数后关闭连接。 文件传输过程中,可能需要处理各种异常情况,如网络中断、文件过大等。因此,良好的错误处理机制是必要的。例如,可以设置超时机制,当连接长时间无响应时自动断开,防止程序挂起。 在实际应用中,还可以考虑增加其他功能,如断点续传、进度显示、多线程处理等,以提高用户体验。此外,考虑到安全性,可能需要对传输的数据进行加密,如使用SSL/TLS协议。 总结起来,简单的TCP文件传输代码涉及的关键知识点包括: 1. TCP协议的基础概念:连接建立、数据传输、连接关闭。 2. Socket编程接口的使用:创建套接字、绑定、监听、连接、读写数据。 3. 文件操作:读取、写入文件内容。 4. 错误处理:网络异常、文件处理异常等。 5. 可能的优化:断点续传、进度显示、多线程、加密传输。 以上就是关于“文件传输代码”的简要介绍,通过理解和实现这些技术,我们可以构建一个基本的文件传输系统。在实际项目中,还需要根据具体需求进行调整和扩展。
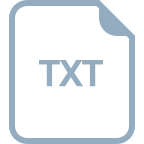
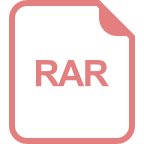
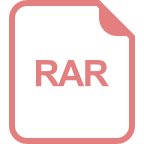
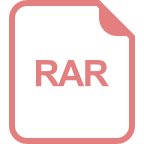
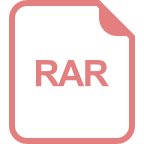
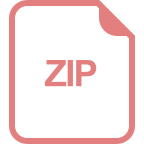
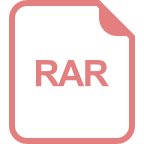
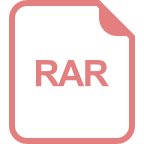
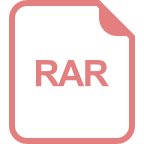
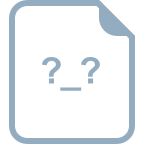
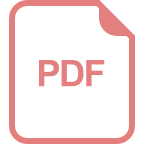
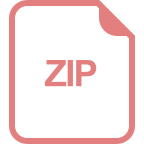
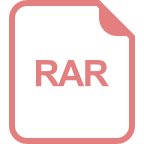

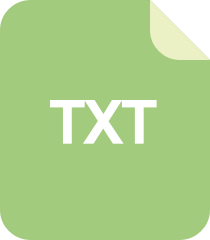
- 1
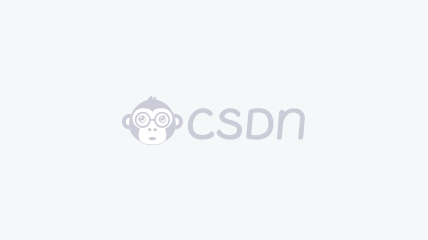

- 粉丝: 1
- 资源: 2
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

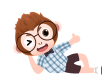
最新资源
- 考试教材中例题和实验代码
- C语言算法详解:经典算法及其应用实例
- 西门子1214 PID 通信模板 西门子PLC 1214和多台G120西门子变频器Modbud RTU通讯 (1)西门子触摸屏; (2)变频器参数 Modbus通讯报文详细讲解; (3)PID自写
- 案例-剪刀石头布小游戏- 源代码
- 基于滑模控制的永磁同步电机矢量控制的matlab仿真模型(smc-pmsm)
- Java综合项目实训-俄罗斯方块
- 【C++】PC微信机器人,实现获取通讯录,发送文本、图片、文件等消息,封装COM接口供Python.zip
- 【c#】基于C#开发的天蓝蓝(aikaobukao)考试管理系统.zip
- 【C#】基于C#+ASP.NetCore实现的在线考试系统,数据库操作使用EnityFrameworkCore框架.zip
- simulink仿真模型 燃料电池 电池 超级电容复合能量管理策略 1、传统PI; 2、等效燃油(氢)耗最低(ECMS); 3、等效能耗最低(EEMS); 4、分频解耦 适用于混合储能能量管理方向
- 【go语言】《Go语言四十二章经》详细讲述Go语言规范与语法细节及开发中常见的误区.zip
- 【C语言】2019年南航计算机学院操作系统课程的实验代码-实验心得-上机考试练习-笔试复习笔记.zip
- 【Golang设计模式】使用Golang泛型实现的设计模式(大话设计模式).zip
- 【go语言】golang钉钉机器人客户端支持文本、链接、Markdown、ActionCard、FeedCard类型消息的发送.zip
- 【java】QQ官方机器人Java-JVM-kotlinSDKQQbotsdkqq机器人sdk.zip
- 【java】ssm+jsp+mysql+LD算法在线考试系统.zip

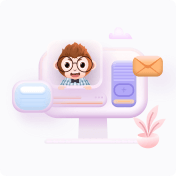
