sprintboot框架访问mysql数据库
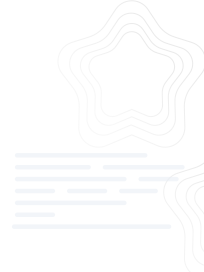

SpringBoot框架是基于Java开发的一款轻量级应用框架,它简化了Spring应用程序的初始搭建以及开发过程。在SpringBoot中整合MyBatis框架,可以实现高效、便捷地操作数据库。以下将详细介绍如何在SpringBoot项目中配置并使用MyBatis来访问MySQL数据库。 1. **依赖管理** 我们需要在`pom.xml`文件中添加SpringBoot的起步依赖(Starter)和MyBatis的相关依赖。SpringBoot提供了`spring-boot-starter-data-jpa`,但在这里我们使用MyBatis,所以需要引入`spring-boot-starter-mybatis`,同时还要包含MySQL的驱动依赖: ```xml <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> </dependency> <dependency> <groupId>org.mybatis.spring.boot</groupId> <artifactId>mybatis-spring-boot-starter</artifactId> <version>2.1.4</version> </dependency> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> </dependency> ``` 2. **数据库配置** 在`application.properties`或`application.yml`中配置MySQL数据库的相关信息,如数据库URL、用户名、密码等: ```properties # application.properties 示例 spring.datasource.url=jdbc:mysql://localhost:3306/mydatabase?useSSL=false&serverTimezone=UTC spring.datasource.username=root spring.datasource.password=root spring.datasource.driver-class-name=com.mysql.jdbc.Driver spring.jpa.hibernate.ddl-auto=none ``` 3. **MyBatis配置** SpringBoot会自动配置MyBatis,但我们可以自定义配置以满足特定需求。在`src/main/resources`目录下创建`mybatis-config.xml`文件,配置MyBatis的全局属性,例如类型别名包、日志工厂等: ```xml <?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd"> <configuration> <typeAliases> <package name="com.example.demo.entity"/> </typeAliases> </configuration> ``` 4. **Mapper接口与XML文件** 创建Mapper接口,定义SQL查询方法。这些接口通常位于`com.example.demo.mapper`包下。例如,一个UserMapper接口: ```java package com.example.demo.mapper; public interface UserMapper { User selectUserById(Long id); } ``` 对应的Mapper XML文件(`UserMapper.xml`)放在`src/main/resources/mapper`目录下,编写SQL语句: ```xml <?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <mapper namespace="com.example.demo.mapper.UserMapper"> <select id="selectUserById" resultType="com.example.demo.entity.User"> SELECT * FROM users WHERE id = #{id} </select> </mapper> ``` 5. **Service层** 创建Service类,注入Mapper接口,实现业务逻辑。例如,UserService类: ```java package com.example.demo.service; import com.example.demo.entity.User; import com.example.demo.mapper.UserMapper; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; @Service public class UserService { private final UserMapper userMapper; @Autowired public UserService(UserMapper userMapper) { this.userMapper = userMapper; } public User getUserById(Long id) { return userMapper.selectUserById(id); } } ``` 6. **Controller层** 创建Controller类,处理HTTP请求,并调用Service层的方法。例如,UserController类: ```java package com.example.demo.controller; import com.example.demo.entity.User; import com.example.demo.service.UserService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RestController; @RestController public class UserController { private final UserService userService; @Autowired public UserController(UserService userService) { this.userService = userService; } @GetMapping("/users/{id}") public User getUser(@PathVariable Long id) { return userService.getUserById(id); } } ``` 7. **运行与测试** 编译并运行SpringBoot项目,通过浏览器访问`http://localhost:8080/users/1`(假设ID为1的用户存在),即可查看到查询结果。此外,可以使用JUnit或其他测试框架对Service层进行单元测试。 通过以上步骤,你已经成功地在SpringBoot项目中集成了MyBatis,实现了对MySQL数据库的访问。这种方式允许开发者专注于业务逻辑,而不必关心底层数据访问的细节,极大地提高了开发效率。
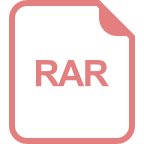
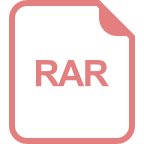
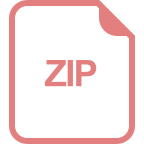
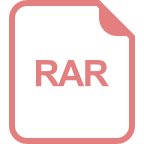
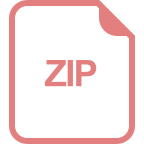
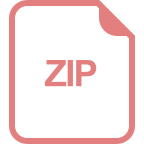
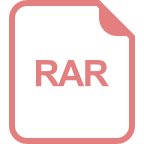
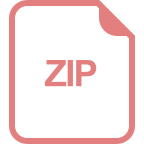
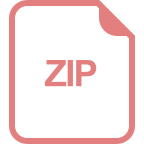
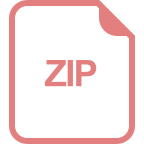
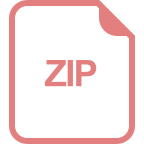
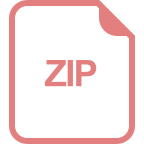
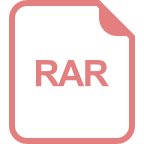
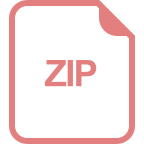
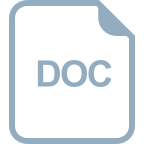
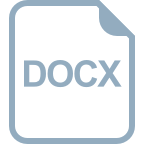
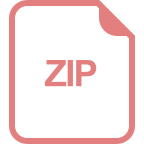
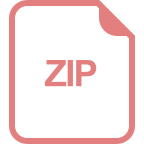
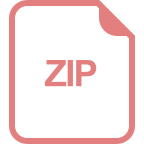
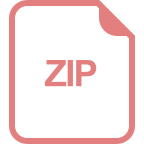
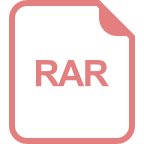
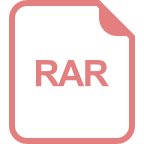


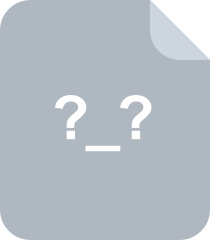







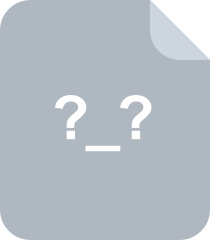
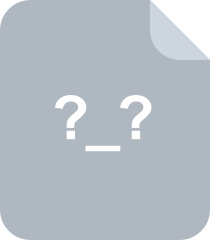
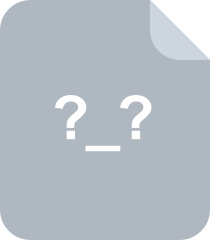
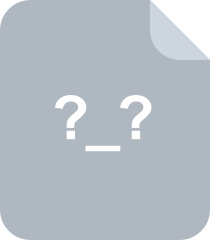




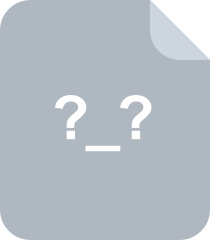
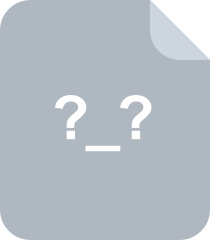

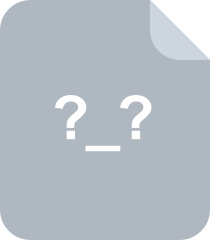

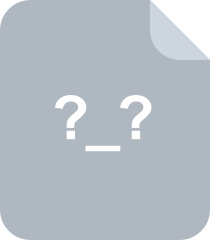

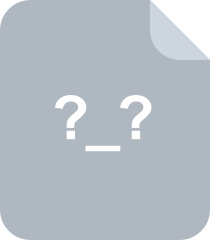

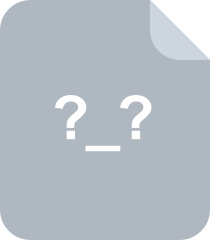

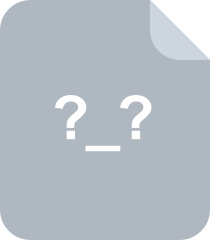
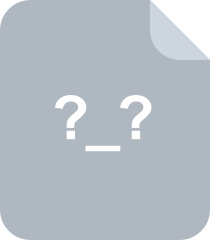
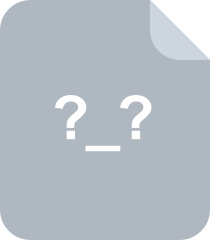






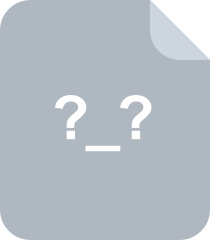

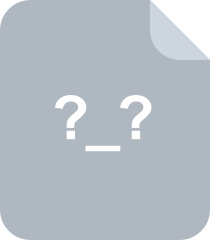





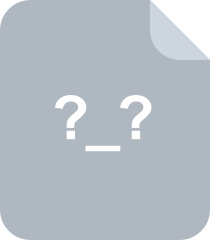
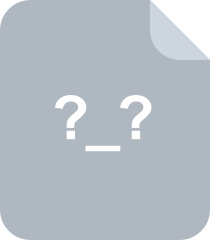

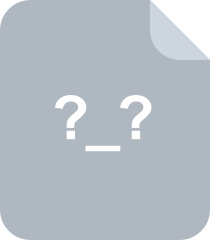

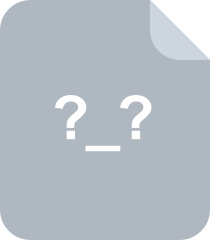

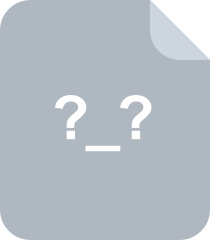
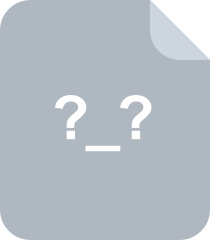
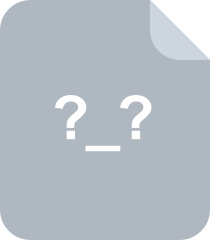
- 1
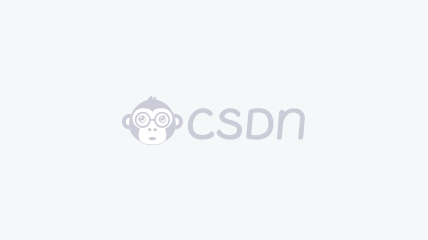

- 粉丝: 7
- 资源: 9
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

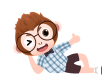
最新资源
- (源码)基于Spring Boot框架的博客系统.zip
- (源码)基于Spring Boot框架的博客管理系统.zip
- (源码)基于ESP8266和Blynk的IR设备控制系统.zip
- (源码)基于Java和JSP的校园论坛系统.zip
- (源码)基于ROS Kinetic框架的AGV激光雷达导航与SLAM系统.zip
- (源码)基于PythonDjango框架的资产管理系统.zip
- (源码)基于计算机系统原理与Arduino技术的学习平台.zip
- (源码)基于SSM框架的大学消息通知系统服务端.zip
- (源码)基于Java Servlet的学生信息管理系统.zip
- (源码)基于Qt和AVR的FestosMechatronics系统终端.zip

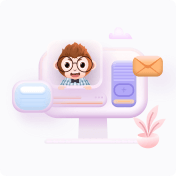
