/*
* The Apache Software License, Version 1.1
*
*
* Copyright (c) 1999 The Apache Software Foundation. All rights
* reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
* 1. Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
*
* 2. Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in
* the documentation and/or other materials provided with the
* distribution.
*
* 3. The end-user documentation included with the redistribution,
* if any, must include the following acknowledgment:
* "This product includes software developed by the
* Apache Software Foundation (http://www.apache.org/)."
* Alternately, this acknowledgment may appear in the software itself,
* if and wherever such third-party acknowledgments normally appear.
*
* 4. The names "Xalan" and "Apache Software Foundation" must
* not be used to endorse or promote products derived from this
* software without prior written permission. For written
* permission, please contact apache@apache.org.
*
* 5. Products derived from this software may not be called "Apache",
* nor may "Apache" appear in their name, without prior written
* permission of the Apache Software Foundation.
*
* THIS SOFTWARE IS PROVIDED ``AS IS'' AND ANY EXPRESSED OR IMPLIED
* WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES
* OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* DISCLAIMED. IN NO EVENT SHALL THE APACHE SOFTWARE FOUNDATION OR
* ITS CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
* SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT
* LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF
* USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND
* ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY,
* OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT
* OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF
* SUCH DAMAGE.
* ====================================================================
*
* This software consists of voluntary contributions made by many
* individuals on behalf of the Apache Software Foundation and was
* originally based on software copyright (c) 1999, Lotus
* Development Corporation., http://www.lotus.com. For more
* information on the Apache Software Foundation, please see
* <http://www.apache.org/>.
*/
package servlet;
import java.io.*;
import java.util.*;
import javax.servlet.*;
import javax.servlet.http.*;
import java.net.URL;
import java.net.MalformedURLException;
import java.net.URLConnection;
import javax.xml.transform.OutputKeys;
import org.apache.xalan.templates.Constants;
import org.apache.xalan.templates.StylesheetRoot;
import org.apache.xalan.templates.OutputProperties;
// SAX2 Imports
import org.xml.sax.ContentHandler;
import org.xml.sax.SAXException;
import org.xml.sax.XMLReader;
import org.xml.sax.Locator;
import org.xml.sax.helpers.XMLReaderFactory;
import org.xml.sax.ext.DeclHandler;
import org.xml.sax.ext.LexicalHandler;
import org.xml.sax.SAXNotRecognizedException;
import org.xml.sax.SAXNotSupportedException;
import org.w3c.dom.*;
import javax.xml.transform.*;
import javax.xml.transform.stream.*;
import javax.xml.transform.sax.SAXTransformerFactory;
import org.apache.xalan.transformer.TransformerImpl;
import org.apache.xpath.objects.XObject;
import org.apache.xpath.objects.XString;
import org.apache.xalan.processor.*;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import org.xml.sax.XMLReader;
import org.xml.sax.helpers.XMLReaderFactory;
import org.xml.sax.helpers.XMLFilterImpl;
/*****************************************************************************************************
*
* ApplyXSLT supplies the basic
* functions for transforming XML data using XSL stylesheets.
*
* @author Spencer Shepard (sshepard@us.ibm.com)
* @author R. Adam King (rak@us.ibm.com)
* @author Tom Rowe (trowe@us.ibm.com)
* @author Don Leslie (donald_leslie@lotus.com)
*
*****************************************************************************************************/
public class ApplyXSLT extends HttpServlet
{
/**
* Operational parameters for this class.
* <p>Request-time values override init-time values which override class defaults.</p>
* @see #init
* @serial
*/
protected ApplyXSLTProperties ourDefaultParameters = null;
private boolean useDefaultTemplates = false;
private Templates defaultTemplates = null;
/**
* String representing the end of line characters for the System.
*/
public final static String EOL = System.getProperty("line.separator");
/**
* String representing the file separator characters for the System.
*/
public final static String FS = System.getProperty("file.separator");
/**
* String representing the current directory for properties files. See init().
*/
public final static String ROOT = System.getProperty("server.root");
public static String CURRENTDIR;
/**
* Initialize operational parameters from the configuration.
* @param config Configuration
* @exception ServletException Never thrown
*/
public void init(ServletConfig config)
throws ServletException
{
super.init(config);
// If the server.root property --see above-- is null, use current working directory
// as default location for media.properties.
if (ROOT != null)
CURRENTDIR= ROOT + FS + "servlets" + FS;
else
CURRENTDIR = System.getProperty("user.dir")+ FS;
setDefaultParameters(config);
setMediaProps(config.getInitParameter("mediaURL"));
String defaultXSL = config.getInitParameter("xslURL");
try
{
if (defaultXSL !=null && defaultXSL.length() > 0)
compileXSL(defaultXSL);
}
catch (Exception e){}
}
/**
* If a default setting exists for xslURL, create a Templates object
* for rapid transformations.
*/
protected void compileXSL(String defaultXSL)
throws TransformerConfigurationException
{
TransformerFactory tFactory = TransformerFactory.newInstance();
defaultTemplates = tFactory.newTemplates(new StreamSource(defaultXSL));
useDefaultTemplates = true;
}
/**
* Sets the default parameters for the servlet from the configuration.
* Also sets required system properties until we figure out why servlet
* sometimess fails to read properties from properties files.
* @param config Configuration
*/
protected void setDefaultParameters(ServletConfig config)
{
ourDefaultParameters = new DefaultApplyXSLTProperties(config);
}
/**
* Loads the media properties file specified by the given string.
* @param mediaURLstring Location of the media properties file. Can be either a full URL or a path relative
* to the System's server.root /servlets directory. If this parameter is null,
* server.root/servlets/media.properties will be used.
* @see ApplyXSL#CURRENTDIR
*/
protected void setMediaProps(String mediaURLstring)
{
if (mediaURLstring != null)
{
URL url = null;
try
{
url = new URL(mediaURLstring);
}
catch (MalformedURLException mue1)
{
try
{
url = new URL("file", "", CURRENTDIR + mediaURLstring);
}
catch (MalformedURLException mue2)
{
writeLog("Unable to find the media properties file based on parameter 'mediaURL' = "
+ mediaURL
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
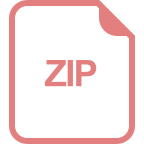
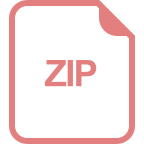
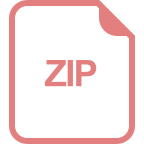
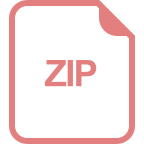
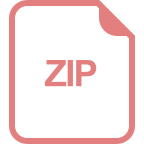
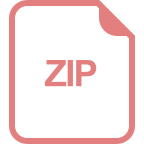
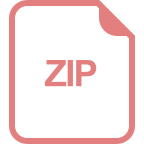
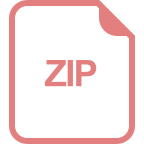
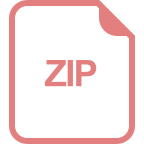
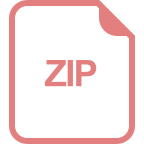
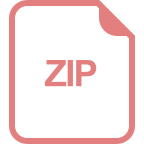
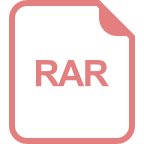
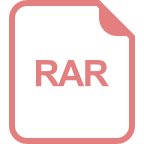
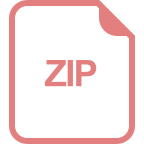
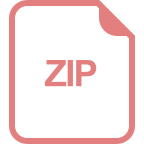
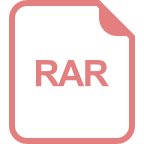
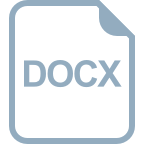
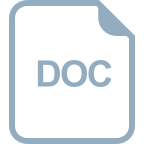
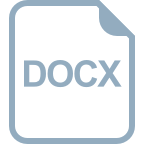
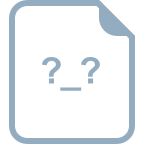
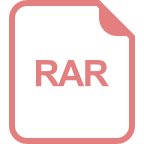
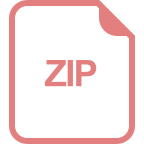
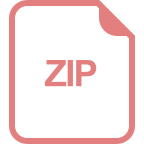
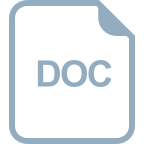
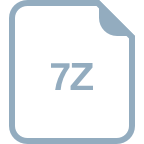
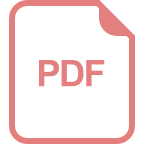
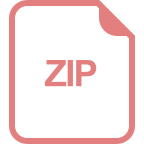
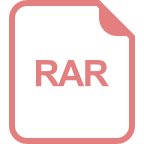
收起资源包目录

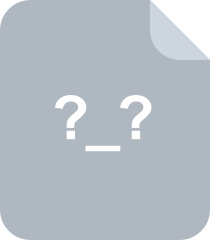
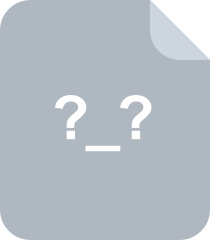
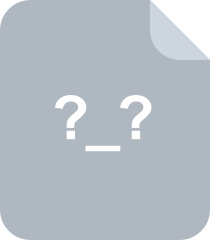
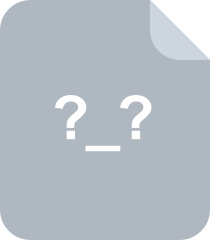
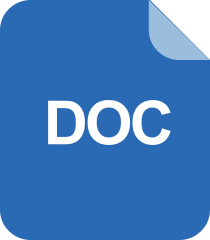
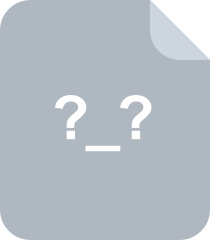
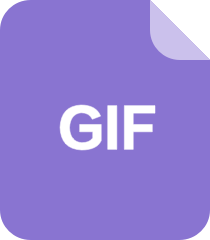
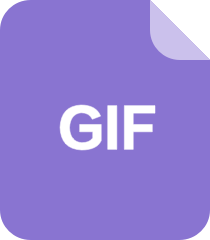
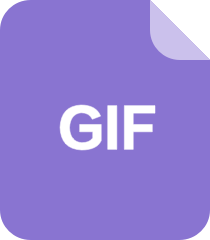
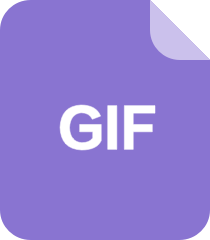
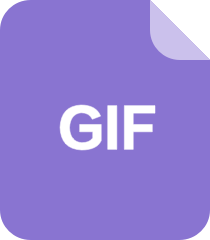
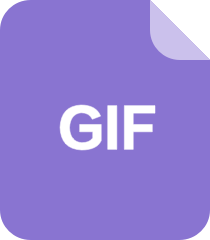
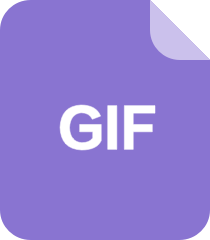
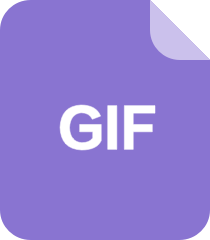
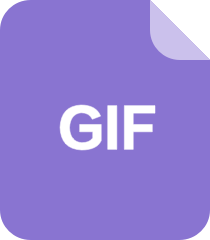
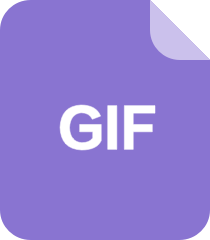
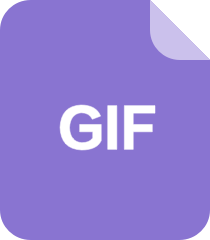
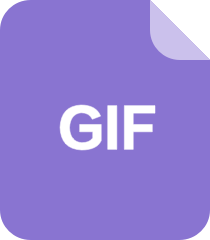
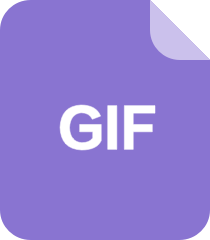
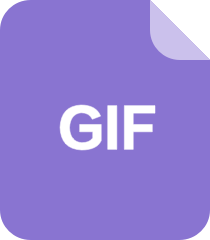
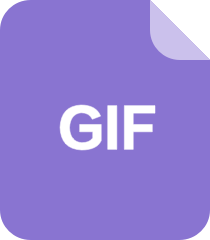
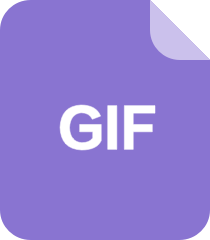
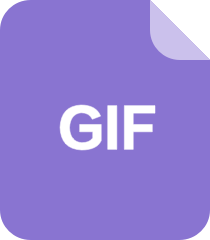
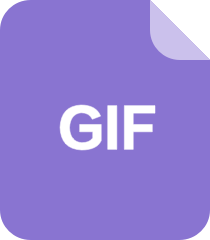
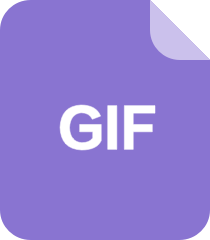
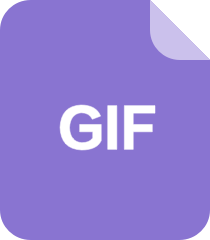
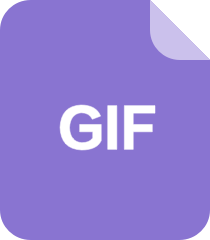
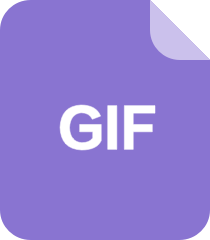
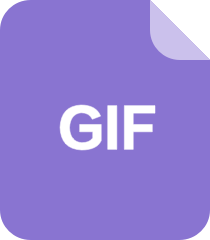
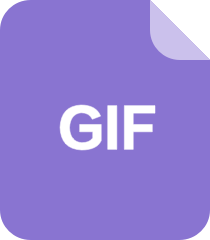
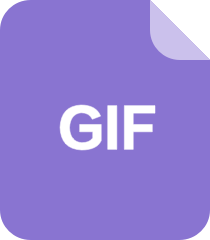
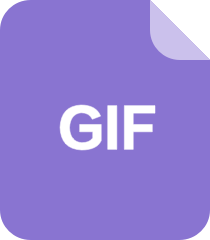
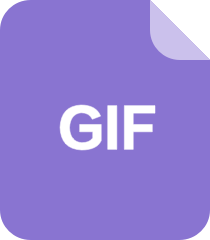
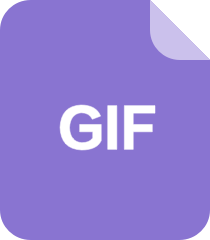
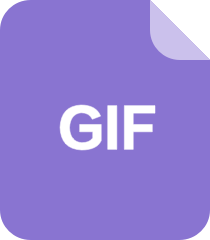
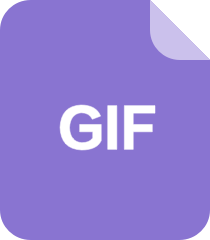
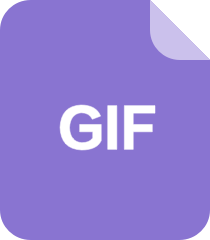
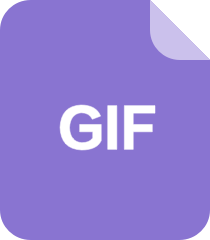
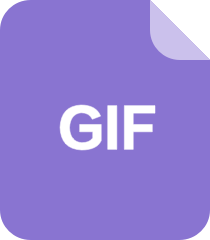
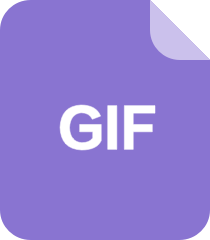
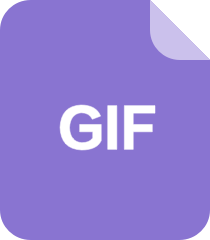
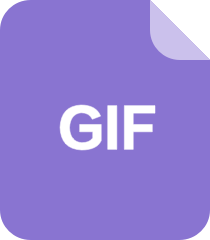
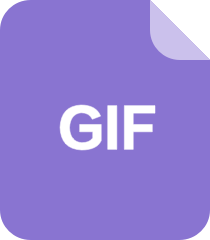
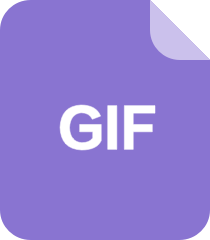
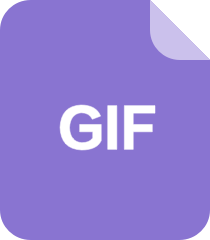
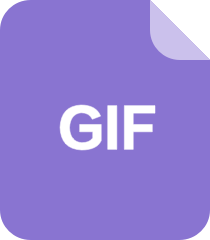
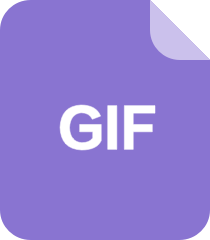
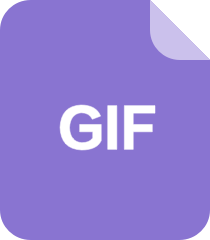
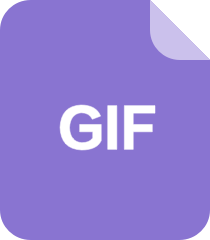
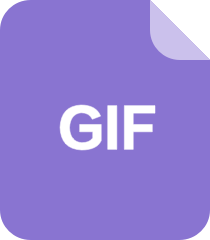
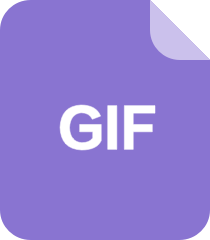
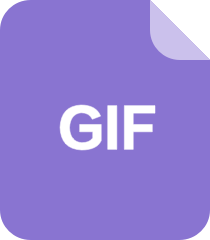
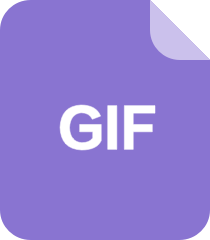
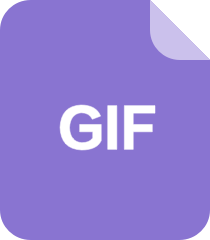
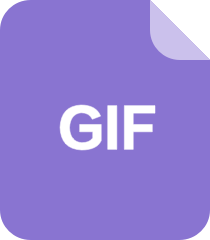
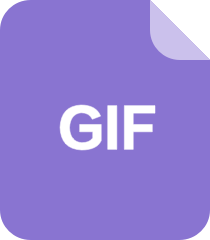
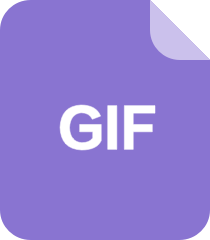
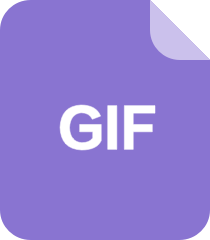
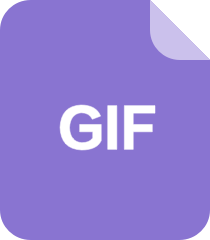
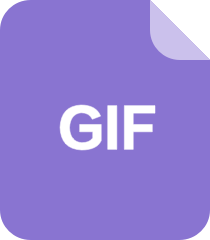
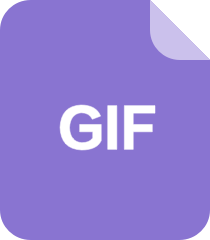
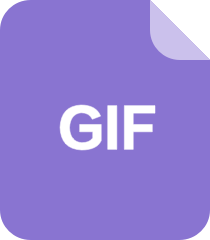
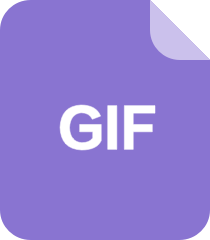
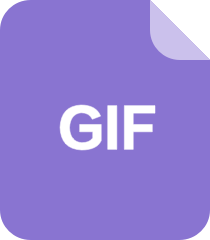
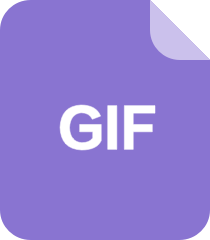
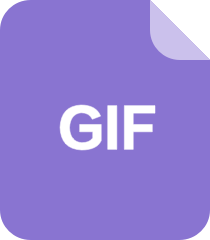
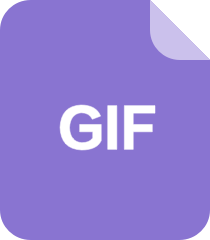
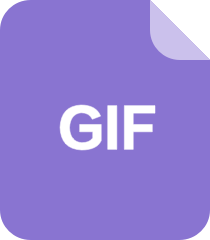
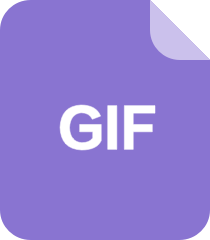
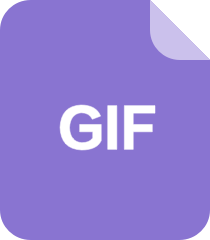
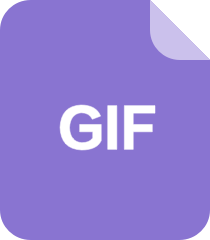
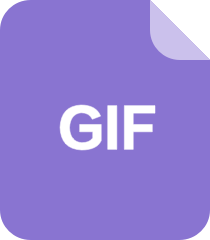
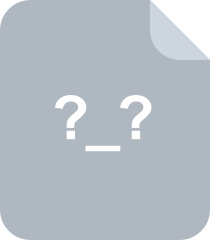
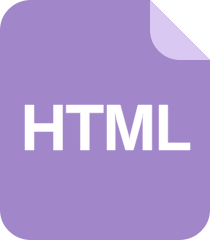
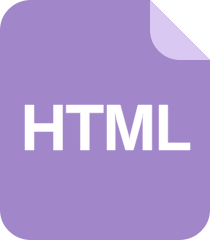
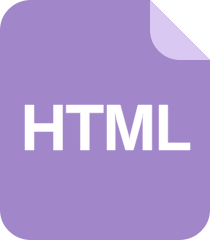
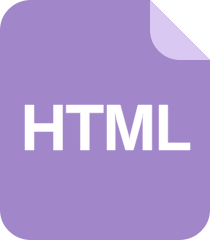
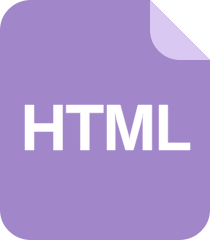
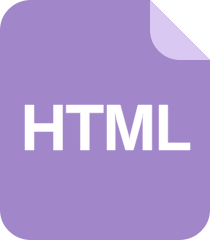
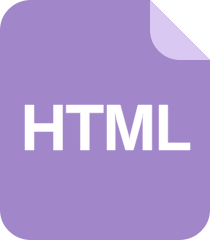
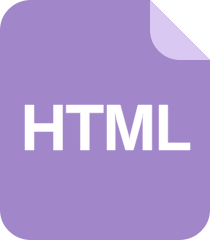
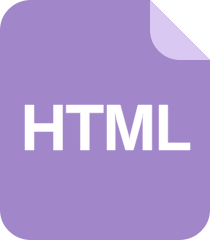
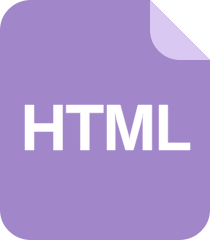
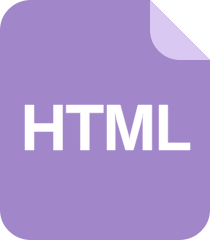
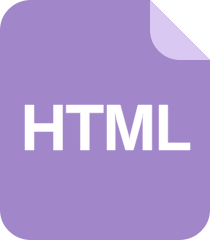
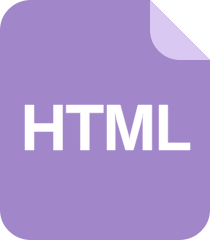
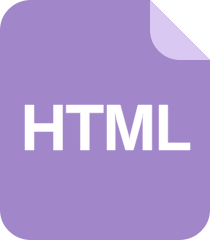
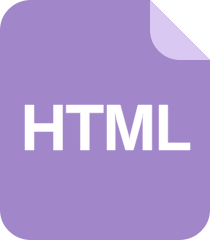
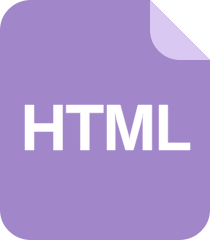
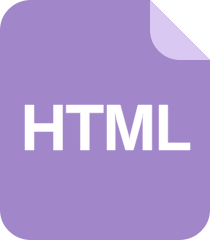
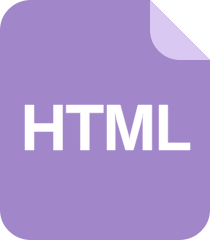
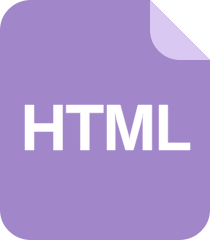
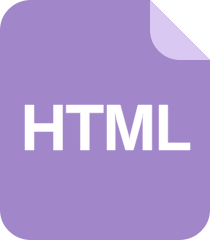
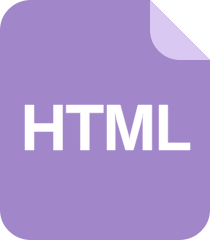
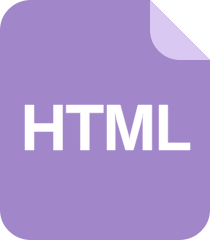
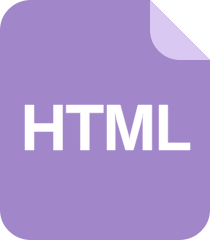
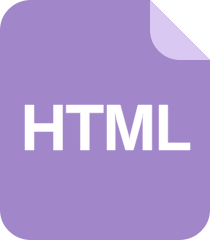
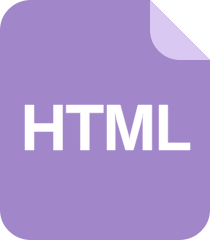
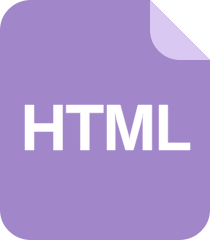
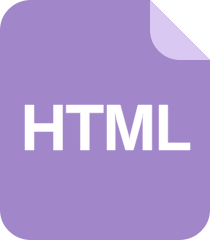
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
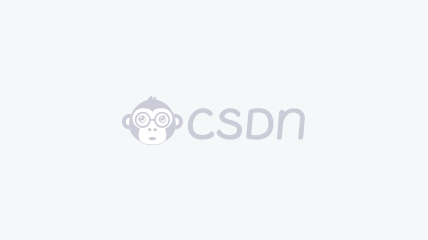

段子手-168
- 粉丝: 4380
- 资源: 2745
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

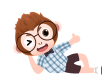
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


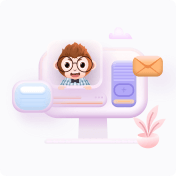
安全验证
文档复制为VIP权益,开通VIP直接复制
