/*
* Copyright 2010 The myBatis Team
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.mybatis.spring;
import static org.junit.Assert.assertEquals;
import static org.junit.Assert.assertNotSame;
import static org.junit.Assert.fail;
import org.apache.ibatis.exceptions.PersistenceException;
import org.apache.ibatis.mapping.Environment;
import org.apache.ibatis.session.ExecutorType;
import org.apache.ibatis.session.SqlSession;
import org.apache.ibatis.transaction.jdbc.JdbcTransactionFactory;
import org.junit.After;
import org.junit.Test;
import org.springframework.dao.DataAccessException;
import org.springframework.dao.TransientDataAccessResourceException;
import org.springframework.transaction.TransactionStatus;
import org.springframework.transaction.support.DefaultTransactionDefinition;
import com.mockrunner.mock.jdbc.MockDataSource;
import com.mockrunner.mock.jdbc.MockPreparedStatement;
/**
* @version $Id$
*/
public final class MyBatisSpringTest extends AbstractMyBatisSpringTest {
private SqlSession session;
@After
public void validateSessionClose() {
// assume if the Executor is closed, the Session is too
if ((session != null) && !executorInterceptor.isExecutorClosed()) {
session = null;
fail("SqlSession is not closed");
} else {
session = null;
}
}
// ensure MyBatis API still works with SpringManagedTransaction
@Test
public void testMyBatisAPI() {
session = sqlSessionFactory.openSession();
session.getMapper(TestMapper.class).findTest();
session.close();
assertNoCommit();
assertSingleConnection();
assertExecuteCount(1);
}
@Test
public void testMyBatisAPIWithCommit() {
session = sqlSessionFactory.openSession();
session.getMapper(TestMapper.class).findTest();
session.commit(true);
session.close();
assertCommit();
assertSingleConnection();
assertExecuteCount(1);
}
@Test
public void testMyBatisAPIWithRollback() {
session = sqlSessionFactory.openSession();
session.getMapper(TestMapper.class).findTest();
session.rollback(true);
session.close();
assertRollback();
assertSingleConnection();
assertExecuteCount(1);
}
// basic tests using SqlSessionUtils instead of using the MyBatis API directly
@Test
public void testSpringAPI() {
session = SqlSessionUtils.getSqlSession(sqlSessionFactory);
session.getMapper(TestMapper.class).findTest();
SqlSessionUtils.closeSqlSession(session, sqlSessionFactory);
assertNoCommit();
assertSingleConnection();
assertExecuteCount(1);
}
@Test
public void testSpringAPIWithCommit() {
session = SqlSessionUtils.getSqlSession(sqlSessionFactory);
session.getMapper(TestMapper.class).findTest();
session.commit(true);
SqlSessionUtils.closeSqlSession(session, sqlSessionFactory);
assertCommit();
assertSingleConnection();
}
@Test
public void testSpringAPIWithRollback() {
session = SqlSessionUtils.getSqlSession(sqlSessionFactory);
session.getMapper(TestMapper.class).findTest();
session.rollback(true);
SqlSessionUtils.closeSqlSession(session, sqlSessionFactory);
assertRollback();
assertSingleConnection();
}
@Test
public void testSpringAPIWithMyBatisClose() {
// This is a programming error and could lead to connection leak if there is a transaction
// in progress. But, the API allows it, so make sure it at least works without a tx.
session = SqlSessionUtils.getSqlSession(sqlSessionFactory);
session.getMapper(TestMapper.class).findTest();
session.close();
assertNoCommit();
assertSingleConnection();
}
// Spring API should work with a MyBatis TransactionFactories, as long as there is not a Spring
// TX is progress
@Test
public void testWithNonSpringTransactionFactory() {
Environment original = sqlSessionFactory.getConfiguration().getEnvironment();
Environment nonSpring = new Environment("non-spring", new JdbcTransactionFactory(), dataSource);
sqlSessionFactory.getConfiguration().setEnvironment(nonSpring);
try {
session = SqlSessionUtils.getSqlSession(sqlSessionFactory);
session.getMapper(TestMapper.class).findTest();
SqlSessionUtils.closeSqlSession(session, sqlSessionFactory);
// users need to manually call commit, rollback and close, just like with normal MyBatis
// API usage
assertNoCommit();
assertSingleConnection();
} finally {
sqlSessionFactory.getConfiguration().setEnvironment(original);
}
}
@Test(expected = TransientDataAccessResourceException.class)
public void testNonSpringTxFactoryWithTx() throws Exception {
Environment original = sqlSessionFactory.getConfiguration().getEnvironment();
Environment nonSpring = new Environment("non-spring", new JdbcTransactionFactory(), dataSource);
sqlSessionFactory.getConfiguration().setEnvironment(nonSpring);
TransactionStatus status = null;
try {
status = txManager.getTransaction(new DefaultTransactionDefinition());
session = SqlSessionUtils.getSqlSession(sqlSessionFactory);
fail("should not be able to get an SqlSession using non-Spring tx manager when there is an active Spring tx");
} finally {
// rollback required to close connection
txManager.rollback(status);
sqlSessionFactory.getConfiguration().setEnvironment(original);
}
}
// TODO should this pass?
/*
* this is an edge case - completely separate DataSource, non-Spring TXManager but with an
* existing Spring TX. Technically, this could be allowed, but the current implementation fails
* because DataSourceUtils.getConnection(DataSource) pulls _any_ Connection into the current tx.
* To fix, however, SqlSessionTemplate.execute() would need to run more checks
* (DataSourceUtils.isConnectionTransactional() and unwrapping TransactionAwareDataSourceProxy)
* which would increase the code path for all transactions.
*/
@Test(expected = TransientDataAccessResourceException.class)
public void testNonSpringTxFactoryNonSpringDSWithTx() {
Environment original = sqlSessionFactory.getConfiguration().getEnvironment();
MockDataSource mockDataSource = new MockDataSource();
mockDataSource.setupConnection(createMockConnection());
Environment nonSpring = new Environment("non-spring", new JdbcTransactionFactory(), mockDataSource);
sqlSessionFactory.getConfiguration().setEnvironment(nonSpring);
TransactionStatus status = null;
try {
status = txManager.getTransaction(new DefaultTransactionDefinition());
session = SqlSessionUtils.getSqlSession(sqlSessionFactory);
fail("should not be able to get an SqlSession using non-Spring tx manager when there is an active Spring tx");
} finally {
// rollback required to close connection
txManager.rollback(status);
sqlSessionFactory.getConfiguration().set
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
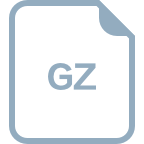
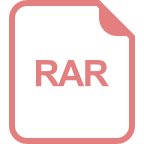
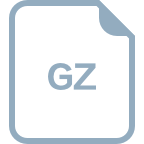
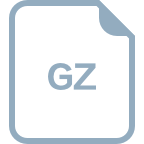
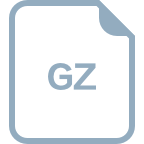
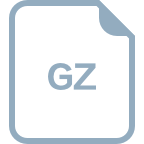
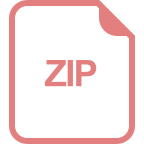
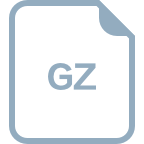
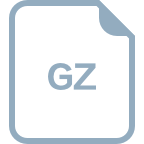
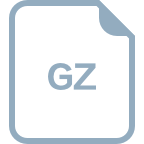
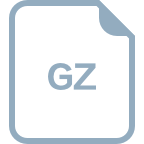
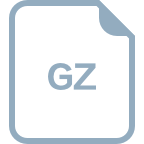
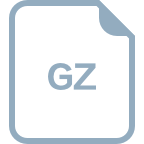
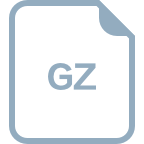
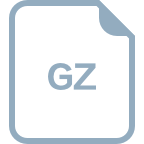
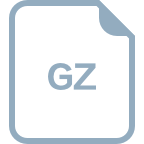
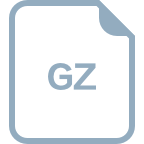
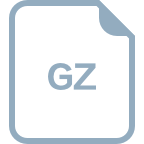
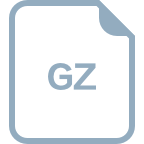
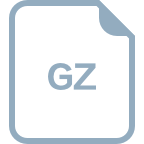
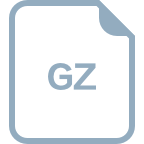
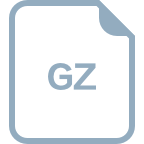
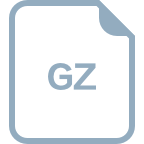
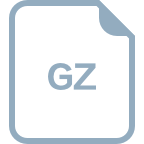
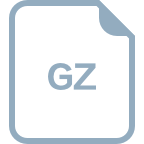
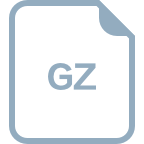
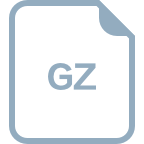
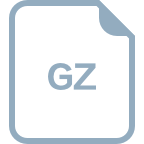
收起资源包目录


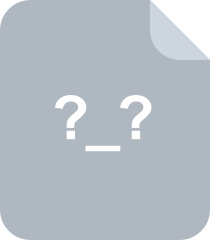


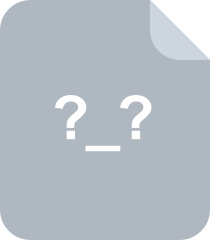
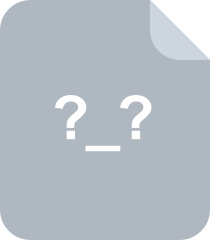
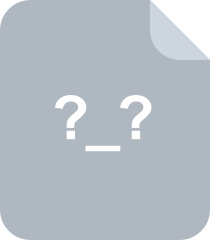
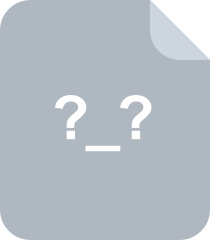
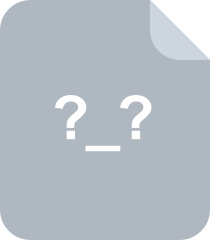
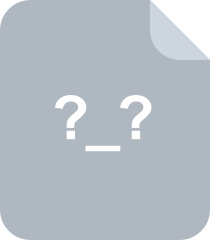
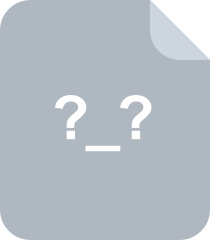
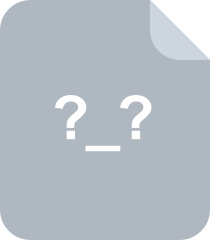
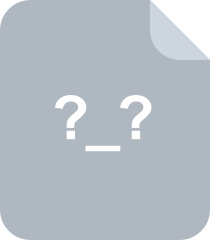

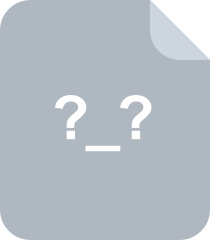


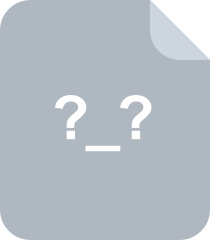





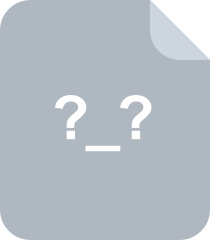
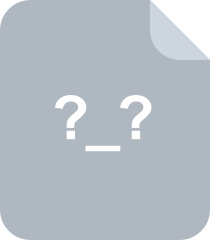
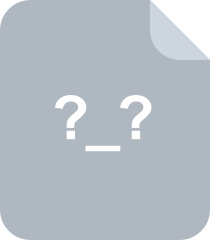
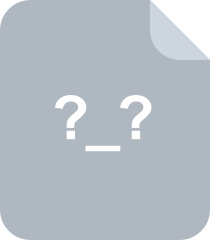
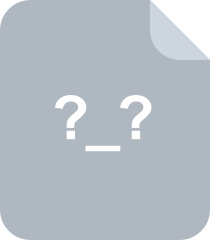
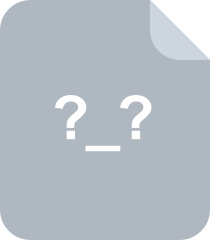
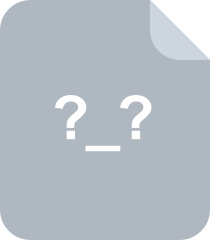

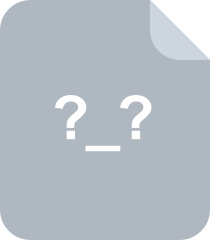
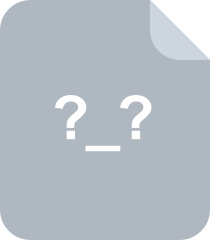
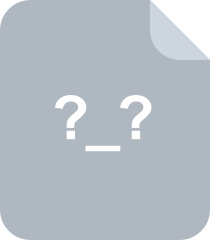
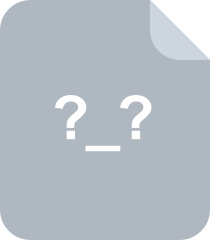




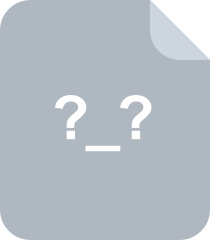
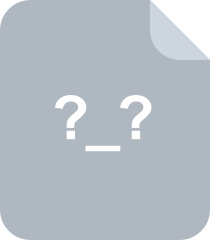
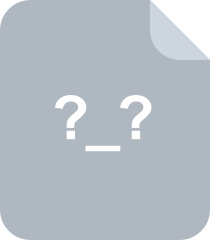


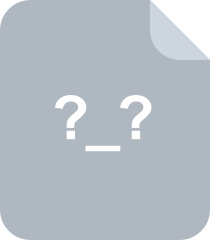
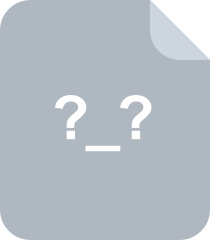
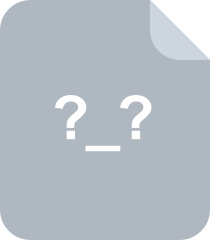
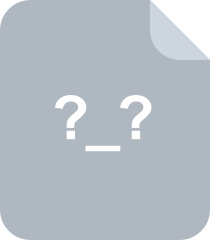
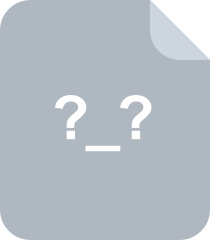
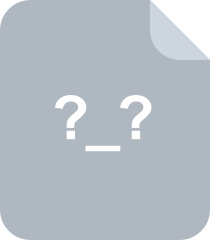
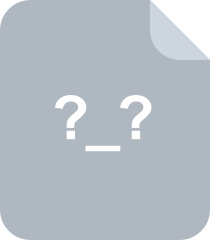
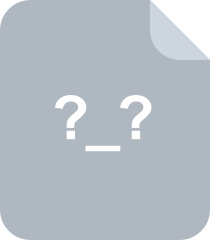
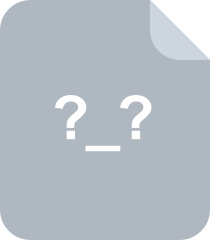

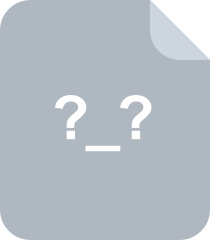

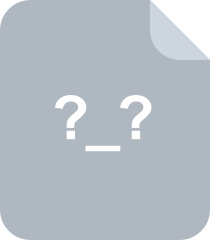
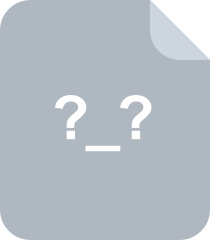

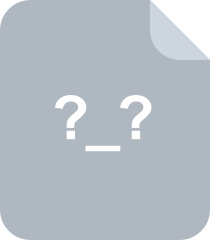
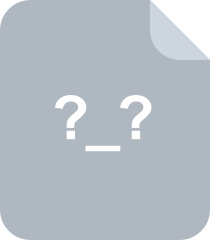
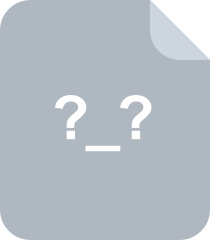

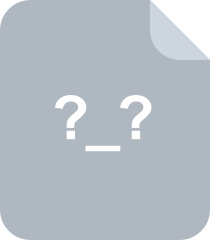
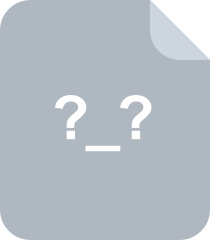
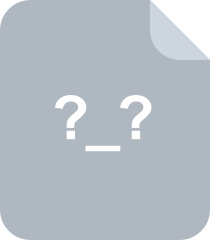
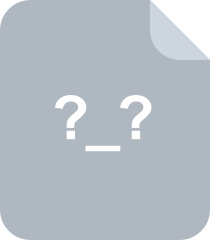
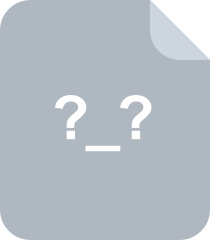

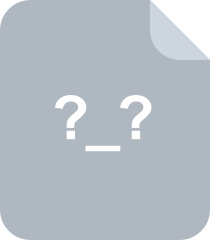

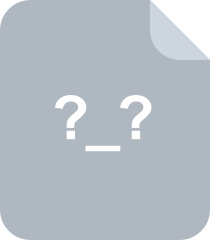

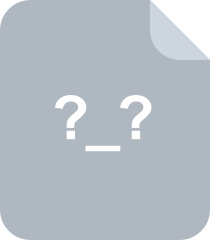
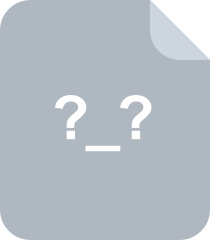
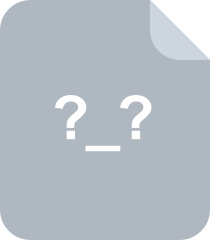
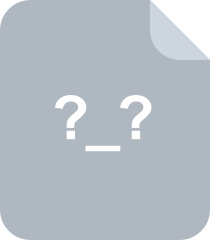
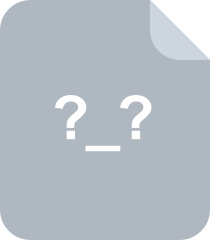





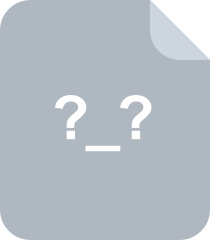
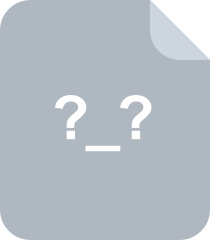
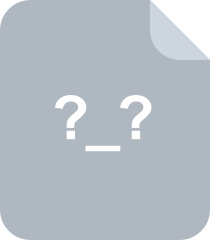
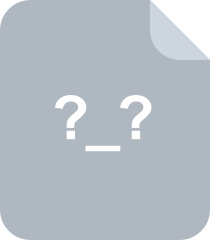

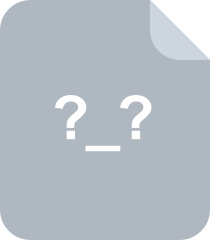
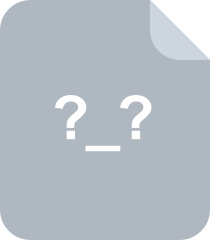
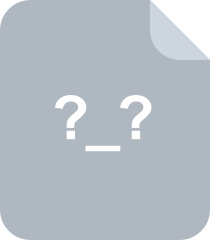
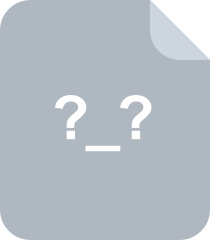

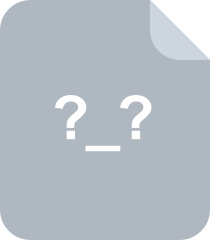
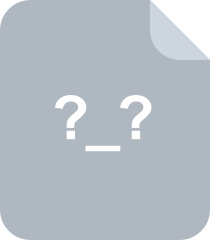

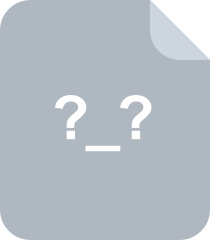
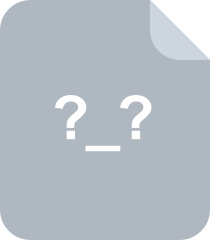
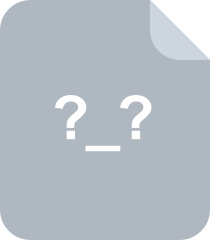
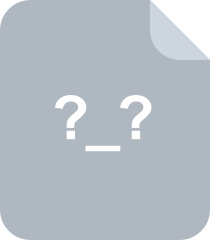
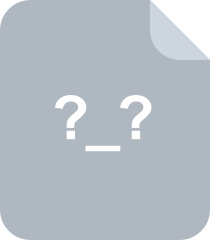
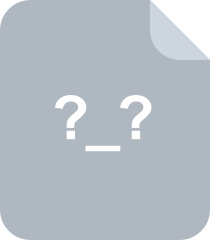
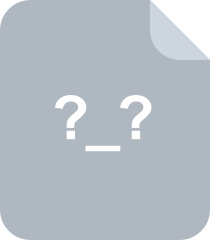
共 70 条
- 1
资源评论
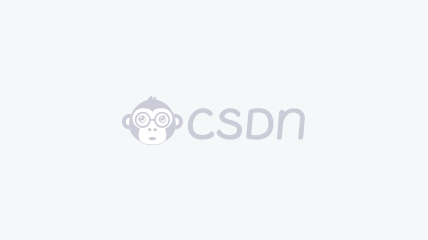

段子手-168
- 粉丝: 4483
- 资源: 2745
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

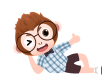
最新资源
- 黑色多页式轻芒杂志消息的微信小程序模板下载.rar
- 黑色仿抖音小视频的微信小程序页面源码.rar
- 黑色仿百度地图精准地图定位的微信小程序模板源码下载.zip
- 黑色购物节抽奖活动的微信小程序模板下载.rar
- 黑色分答简历信息的微信小程序模板下载.zip
- 黑色放肆狂欢水果商城的微信小程序页面模板源码下载.zip
- 黑色古觉居家在线销售奢侈品的微信小程序页面源码.zip
- 黑色好吃实惠水果在线水果商城的微信小程序模板下载.zip
- 黑色好吃水果商城的微信小程序页面源码.zip
- 黑色黑白风DFS微商的微信小程序模板下载.zip
- 黑色横向图文排版商品介绍的微信小程序模板下载.zip
- 黑色花礼网鲜花的微信小程序模板源码下载.rar
- 网上书店(struts+hibernate+css+mysql).zip
- 黑色汇汇生活的微信小程序网页模板源码下载.rar
- 黑色简便四则运算在线计算器的微信小程序模板源码下载.zip
- 黑色婚庆啦婚礼策划摄影的微信小程序页面源码.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


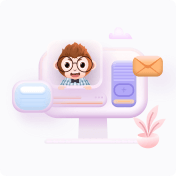
安全验证
文档复制为VIP权益,开通VIP直接复制
