//日志管理类实现
#include "MyLog.h"
//////////////////////////////////////////////////////////////////////////////////////
// 静态变量 //
//////////////////////////////////////////////////////////////////////////////////////
std::string CMyLog::m_strLogPath; //日志文件路径
std::string CMyLog::m_strSingleLogName; //单日志文件名称
std::string CMyLog::m_strCurrentLog; //当前日志文件名称
EMyLogModle CMyLog::m_eModle; //日志文件类型
std::queue<std::string> CMyLog::m_qLogManager; //日志管理队列
pthread_mutex_t CMyLog::m_mutexQueue; //日志队列锁
FILE* CMyLog::m_Filelog; //日志文件
char CMyLog::m_cTmp[1024]; //缓冲区
CMyLog::CMyLog(){}
CMyLog::~CMyLog(){}
//////////////////////////////////////////////////////////////////////////////////////
// 对外接口 //
//////////////////////////////////////////////////////////////////////////////////////
//初始化日志管理类相关成员
void CMyLog::Init(std::string strLogPath)
{
//默认为每天一个日志文件
m_eModle = EL_EVERY_DAY;
//清空日志队列
while (m_qLogManager.size() > 0)
{
m_qLogManager.pop();
}
//初始化互斥锁
pthread_mutex_init(&m_mutexQueue,NULL);
//初始化日志文件
m_Filelog = NULL;
m_strLogPath = strLogPath;
m_strSingleLogName = "";
m_strCurrentLog = " ";
memset(m_cTmp,0,1024);
//启动日记线程
pthread_t threadID;
pthread_create(&threadID,NULL,CreateLogFile,NULL);//启动服务端接收线程
}
//设置日志文件模式
void CMyLog::SetLogFileModle( EMyLogModle eMod ,std::string strFileName)
{
m_eModle = eMod;
if (eMod == EL_ONLYONE)
{
m_strSingleLogName = strFileName;
}
}
//写入日志
std::string CMyLog::NewLog( std::string strLog )
{
std::string strTmp = GetTime();
strTmp += strLog;
pthread_mutex_lock(&m_mutexQueue);
m_qLogManager.push(strTmp);//strLog
pthread_mutex_unlock(&m_mutexQueue);
return strLog;
}
//写入日志
std::string CMyLog::NewLog( const char* pszFormat,... )
{
//LINUX
va_list args;
va_start( args, pszFormat );
memset(m_cTmp,0,1024);
int nRes = vsprintf(m_cTmp, pszFormat, args );
va_end(args);
std::string strTmp =(std::string)m_cTmp;
NewLog(strTmp);
return strTmp;
}
//////////////////////////////////////////////////////////////////////////////////////
// 内部成员函数 //
//////////////////////////////////////////////////////////////////////////////////////
//日志出队
std::string CMyLog::LogOutQueue()
{
std::string strLog = "";
pthread_mutex_lock(&m_mutexQueue);
strLog = m_qLogManager.front();
m_qLogManager.pop();
pthread_mutex_unlock(&m_mutexQueue);
return strLog;
}
//创建日志文件:后台线程
void* CMyLog::CreateLogFile(void*)
{
while(1)
{
if (m_qLogManager.size() == 0)
{
sleep(1);
}else
{
//获取日志信息
std::string strLog = LogOutQueue();
//获取日志文件信息
GetLogFile();
//日志写入日志文件
if (m_Filelog != NULL)
{
fwrite(strLog.c_str(),strLog.length(),1,m_Filelog);
fflush(m_Filelog);
/*fclose(m_Filelog);
m_Filelog = NULL;*/
}
}
}
}
//获取日志文件指针
void CMyLog::GetLogFile()
{
if (m_eModle == EL_ONLYONE)//单一日志模式
{
if (m_Filelog == NULL)
{
std::string strFileNameOnly = m_strLogPath + m_strSingleLogName;
m_Filelog = fopen(strFileNameOnly.c_str(),"a+");
}else
return;
}else//其他模式
{
//获取当天日期
time_t now;
struct tm* timeinfo;
time(&now);
timeinfo = localtime(&now);
std::string strTmp = "";
char cTmp[15];
memset(cTmp,0,15);
//根据模式判断是否需要更换日志文件
if (m_eModle == EL_EVERY_DAY )
{
sprintf(cTmp,"%4d%02d.log",(int)1900+timeinfo->tm_year,(int)1+timeinfo->tm_mon);
}else if (m_eModle == EL_EVERY_MON)
{
sprintf(cTmp,"%4d%02d%02d.log",(int)1900+timeinfo->tm_year,(int)1+timeinfo->tm_mon,(int)timeinfo->tm_mday);
}else
return;
if (IsNeedNewFile(cTmp))//需要重新生成日志文件
{
if (m_Filelog != NULL)//如果原始日志文件未关闭
{
fclose(m_Filelog);
m_Filelog = NULL;
}
//重新生成日志文件
m_strCurrentLog = (std::string)cTmp;
std::string strFileName = m_strLogPath + m_strCurrentLog;
m_Filelog = fopen(strFileName.c_str(),"a+");
}else
return;
}
return;
}
//判断是否需要新的日志文件
bool CMyLog::IsNeedNewFile(std::string strFile)
{
if (m_Filelog == NULL)//日志指针为空
{
return true;
}else
{
if (m_strCurrentLog.compare(" ") == 0)//还没有生成过日志文件
{
return true;
}else//生成过日志文件
{
if (m_strCurrentLog.compare(strFile.c_str()) == 0)//跟当前日期相同,不需要重新生成
{
return false;
}else//跟当前日期不同,需要重新生成
return true;
}
}
}
//获取时间
std::string CMyLog::GetTime()
{
time_t tmNow = time(NULL);
struct tm *local;
local = localtime(&tmNow);
char cTmp[22] = {0};
sprintf(cTmp,"%d-%d-%d %d:%d:%d ",local->tm_year+1900,local->tm_mon+1,local->tm_mday,local->tm_hour,local->tm_min,local->tm_sec);
return (std::string)cTmp;
}
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
Linux系统下,使用C++编写的日志类,不是linux自动的日志系统。 资源包括3个文件: MyLog.h是日志类的头文件 MyLog.cpp是日志类的实现文件 main.cpp是日志接口的测试文件 日志文件模式:每天一个日志文件,每月一个日志文件,只有一个日志文件。 日志通过线程实现,添加日志时,日志添加到队列中,子线程从队列中取日志字符串并写入文件。 支持像printf函数一样拼接日志字符串。 供参考。
资源推荐
资源详情
资源评论
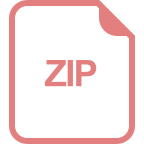
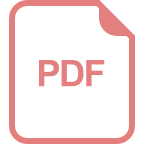
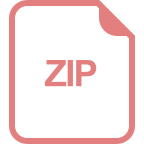
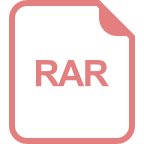
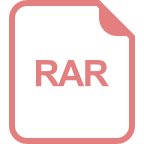
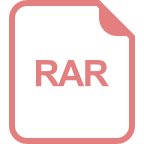
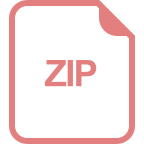
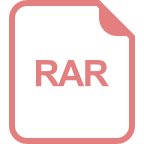
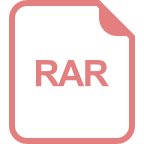
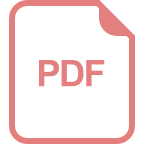
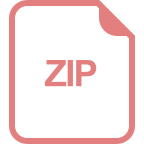
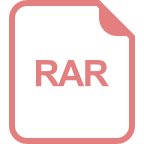
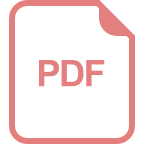
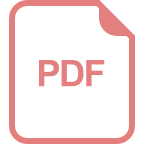
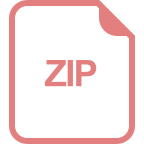
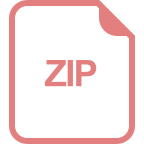
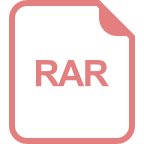
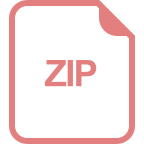
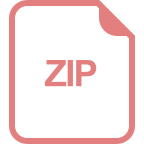
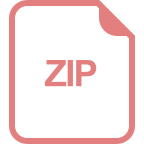
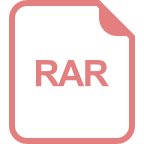
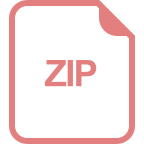
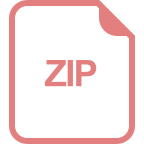
收起资源包目录


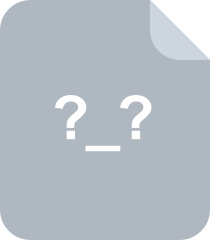
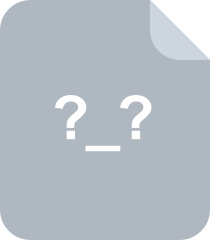
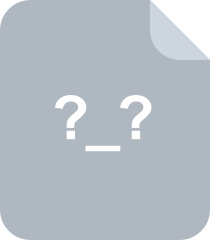
共 3 条
- 1
资源评论
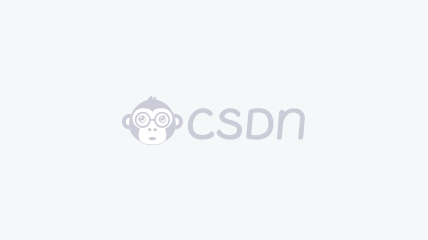

qfl_sdu
- 粉丝: 4292
- 资源: 20
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

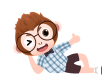
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


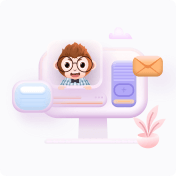
安全验证
文档复制为VIP权益,开通VIP直接复制
