springboot实现邮件发送
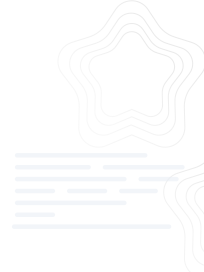


在Spring Boot框架中,实现邮件发送功能是一项常见的需求,它能够帮助我们自动化处理通知、确认信息等业务场景。本文将详细讲解如何利用Spring Boot的JavaMailSender接口和相关的配置来实现各种类型的邮件发送,包括文本邮件、HTML邮件、带有附件的邮件、包含图片的邮件以及使用模板的邮件。 我们需要在Spring Boot项目中引入`spring-boot-starter-mail`依赖,这可以通过在`pom.xml`文件中添加以下依赖来完成: ```xml <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-mail</artifactId> </dependency> ``` 接下来,配置邮件服务的相关属性。在`application.properties`或`application.yml`文件中,添加如下配置: ```properties # application.properties 示例 spring.mail.host=smtp.example.com spring.mail.port=587 spring.mail.username=your-email@example.com spring.mail.password=your-password spring.mail.protocol=smtp spring.mail.properties.mail.smtp.auth=true spring.mail.properties.mail.smtp.starttls.enable=true ``` 现在,我们可以创建一个邮件服务类,使用`JavaMailSender`接口来实现邮件发送。创建`MailService`类: ```java import org.springframework.beans.factory.annotation.Autowired; import org.springframework.mail.SimpleMailMessage; import org.springframework.mail.javamail.JavaMailSender; import org.springframework.stereotype.Service; @Service public class MailService { @Autowired private JavaMailSender javaMailSender; // 发送简单文本邮件 public void sendSimpleMail(String to, String subject, String text) { SimpleMailMessage message = new SimpleMailMessage(); message.setTo(to); message.setSubject(subject); message.setText(text); javaMailSender.send(message); } // 发送HTML邮件 public void sendHtmlMail(String to, String subject, String htmlContent) { SimpleMailMessage message = new SimpleMailMessage(); message.setTo(to); message.setSubject(subject); message.setText(htmlContent, true); // 第二个参数设为true表示是HTML内容 javaMailSender.send(message); } // 发送带附件的邮件 public void sendMailWithAttachment(String to, String subject, String text, File attachment) { MimeMessage message = javaMailSender.createMimeMessage(); MimeMessageHelper helper = new MimeMessageHelper(message, true); helper.setTo(to); helper.setSubject(subject); helper.setText(text, true); helper.addAttachment(attachment.getName(), attachment); javaMailSender.send(message); } // 发送包含图片的邮件 public void sendMailWithInlineImage(String to, String subject, String htmlContent, byte[] imageData, String cid) { MimeMessage message = javaMailSender.createMimeMessage(); MimeMessageHelper helper = new MimeMessageHelper(message, true); helper.setTo(to); helper.setSubject(subject); helper.setText(htmlContent, true); helper.addInline(cid, new ByteArrayResource(imageData), "image/jpeg"); javaMailSender.send(message); } // 使用模板发送邮件 public void sendTemplateMail(String to, String subject, Map<String, Object> model, String templatePath) { // 假设你有一个模板引擎如Thymeleaf Context context = new Context(); context.setVariables(model); String processedTemplate = templateEngine.process(templatePath, context); sendHtmlMail(to, subject, processedTemplate); } } ``` 在上述代码中,我们定义了发送不同类型邮件的方法,包括简单文本邮件、HTML邮件、带附件的邮件、包含图片的邮件以及使用模板的邮件。在实际项目中,你可能需要根据具体需求调整这些方法,例如添加更多的参数或使用不同的模板引擎。 为了测试这些功能,你可以创建一个控制器或者命令行工具,调用`MailService`中的相应方法,传入必要的参数。例如,创建一个`MailController`类,然后在其中添加测试用例: ```java import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.PostMapping; import org.springframework.web.bind.annotation.RequestParam; import org.springframework.web.multipart.MultipartFile; import javax.mail.MessagingException; import java.io.File; import java.io.IOException; @RestController public class MailController { @Autowired private MailService mailService; // 测试发送简单文本邮件 @PostMapping("/send-simple") public void sendSimpleMail(@RequestParam("to") String to, @RequestParam("subject") String subject, @RequestParam("text") String text) { mailService.sendSimpleMail(to, subject, text); } // 测试发送HTML邮件 @PostMapping("/send-html") public void sendHtmlMail(@RequestParam("to") String to, @RequestParam("subject") String subject, @RequestParam("htmlContent") String htmlContent) { mailService.sendHtmlMail(to, subject, htmlContent); } // 测试发送带附件的邮件 @PostMapping("/send-attachment") public void sendMailWithAttachment(@RequestParam("to") String to, @RequestParam("subject") String subject, @RequestParam("text") String text, @RequestParam("file") MultipartFile file) throws IOException { File tempFile = File.createTempFile("attachment", file.getOriginalFilename()); file.transferTo(tempFile); mailService.sendMailWithAttachment(to, subject, text, tempFile); tempFile.delete(); } // 测试发送包含图片的邮件 @PostMapping("/send-inline-image") public void sendMailWithInlineImage(@RequestParam("to") String to, @RequestParam("subject") String subject, @RequestParam("htmlContent") String htmlContent, @RequestParam("imageData") byte[] imageData, @RequestParam("cid") String cid) { mailService.sendMailWithInlineImage(to, subject, htmlContent, imageData, cid); } // 测试使用模板发送邮件 @PostMapping("/send-template") public void sendTemplateMail(@RequestParam("to") String to, @RequestParam("subject") String subject, @RequestParam("modelJson") String modelJson, @RequestParam("templatePath") String templatePath) throws IOException { // 假设modelJson是JSON字符串,你需要将其转换为Map对象 Map<String, Object> model = ...; // JSON转Map mailService.sendTemplateMail(to, subject, model, templatePath); } } ``` 通过上述步骤,你已经成功地在Spring Boot项目中实现了邮件发送功能,包括各种类型和复杂场景。现在你可以使用提供的测试用例进行实际操作,验证邮件发送的正确性。如果需要更复杂的邮件功能,例如邮件队列、定时发送等,可以考虑引入额外的库或服务,如Spring Integration、SendGrid等。
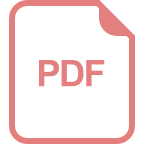
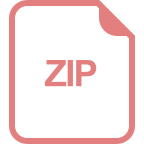
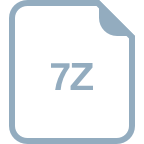
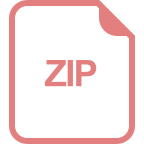
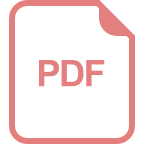
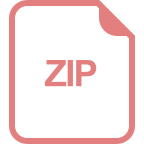
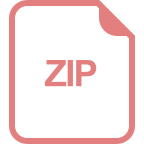
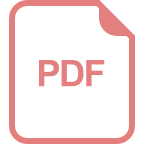
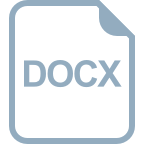
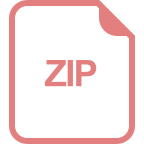
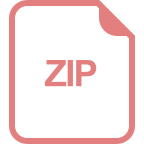
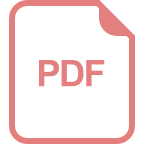
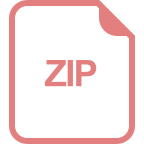
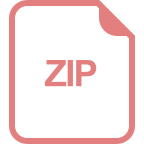


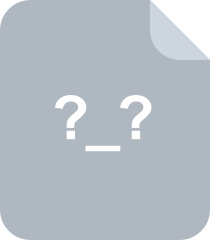
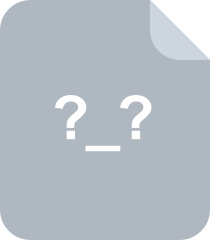





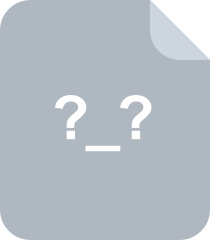





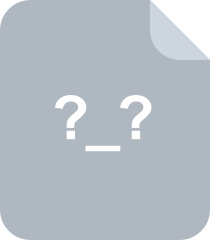
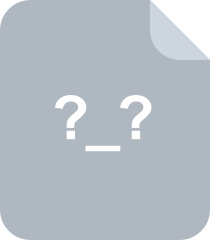
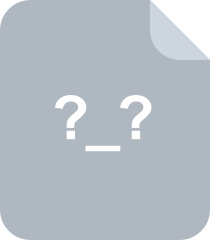
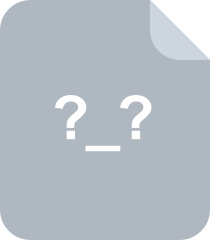

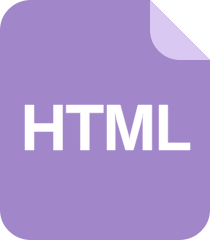



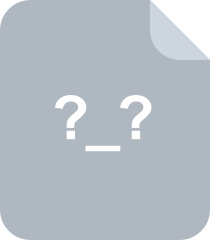
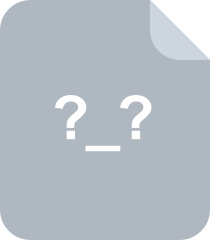

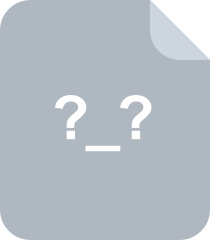

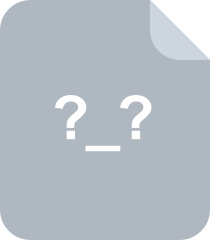
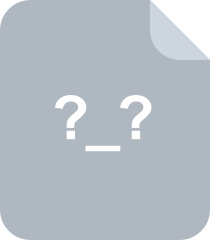
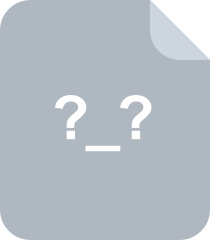
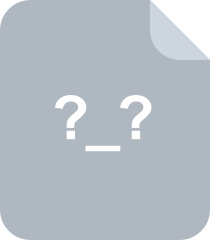






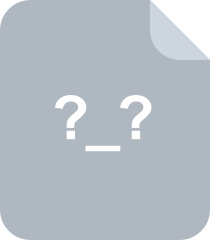


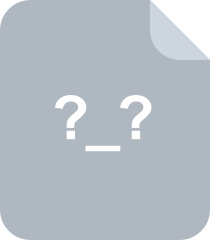

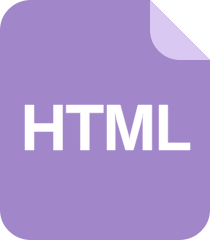





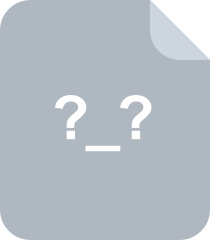
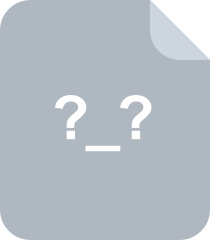
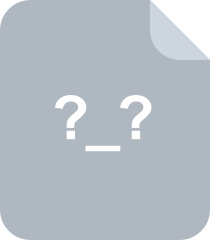
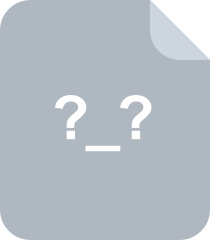
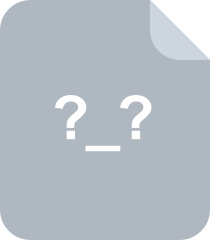


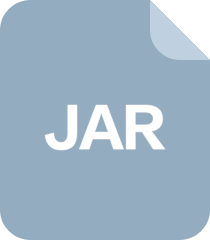
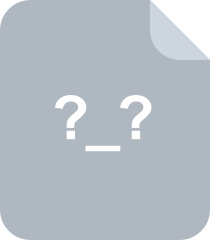
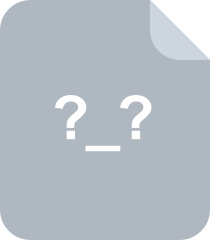
- 1
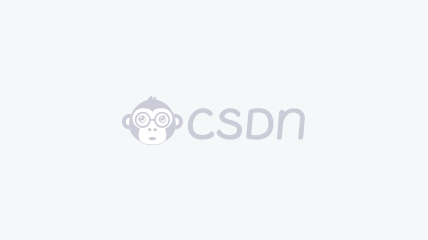
- DTcloud_ysj2021-06-15垃圾 很少的代码 都是基础的代码 没有参考价值

- 粉丝: 91
- 资源: 20
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

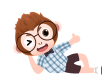
