### C++代码优化 #### 一、引言与优化成本考量 C++作为一种高性能的编程语言,在许多领域都有着广泛的应用,比如游戏开发、系统软件、高性能计算等。然而,为了充分发挥其性能优势,开发者需要对代码进行优化。《C++代码优化》这本书详细介绍了在Windows、Linux和Mac平台上的C++代码优化方法。本书开篇就提到了优化的成本问题,即在优化过程中需要权衡的时间和资源消耗。 #### 二、选择最优平台 ##### 2.1 硬件平台的选择 不同的硬件平台对于程序性能有着直接影响。例如,服务器级的CPU通常具有更高的核心数和更大的缓存,这使得它们更适合处理并发任务和大数据量的运算。 ##### 2.2 微处理器的选择 微处理器的选择也至关重要。不同品牌(如Intel、AMD)的CPU在架构上存在差异,这些差异会影响到程序的执行效率。此外,即使是同一品牌的CPU,不同系列之间也有着显著的性能差异。 ##### 2.3 操作系统的选取 操作系统的选择同样重要。不同操作系统对于底层硬件的支持程度不同,提供的API和工具也不一样。例如,Linux提供了丰富的命令行工具和库支持,使得性能调优更为便捷。 ##### 2.4 编程语言的选择 尽管本书主要讨论的是C++,但选择合适的编程语言也是提高性能的关键之一。某些特定的任务可能更适合用其他语言实现,例如Python在数据分析方面非常强大。 ##### 2.5 编译器的选择 编译器的选择对于最终生成的机器码质量有着直接影响。不同编译器在优化选项和策略上存在差异,因此选择适合特定场景的编译器非常重要。 ##### 2.6 用户界面框架的选择 用户界面框架的选择不仅影响到用户体验,也会影响到程序的整体性能。轻量级的UI框架通常会带来更好的性能表现。 ##### 2.7 克服C++语言的局限性 C++虽然功能强大,但也存在一些局限性,如内存管理复杂、模板元编程难以调试等。了解并克服这些局限性是优化过程中的关键步骤。 #### 三、寻找最大的时间消耗者 理解程序中哪些部分耗时最长是优化的第一步。这包括但不限于: - **如何衡量一个周期的时间?** - **使用性能分析工具来定位热点**。 - **程序安装与加载**。 - **文件访问**。 - **数据库操作**。 - **网络通信**。 - **内存访问**。 - **上下文切换**。 - **依赖链分析**。 - **执行单元吞吐量**。 #### 四、性能与可用性 性能优化不能以牺牲用户体验为代价。在进行优化时,必须考虑到用户体验和程序的易用性。 #### 五、选择最优算法 算法的选择对于程序性能至关重要。不同的数据结构和算法适用于不同的场景,选择正确的算法可以极大地提升程序的运行速度。 #### 六、不同C++构造的不同效率 这部分详细讨论了各种C++语言特性及其对性能的影响,包括但不限于: - **不同类型的变量存储方式**。 - **整型变量与操作符**。 - **浮点数变量与操作符**。 - **枚举类型**。 - **布尔类型**。 - **指针与引用**。 - **函数指针**。 - **成员指针**。 - **数组**。 - **类型转换**。 - **分支与开关语句**。 - **循环**。 - **函数**。 - **结构体与类**。 - **类数据成员**。 - **类成员函数**。 - **虚函数**。 - **运行时类型识别(RTTI)**。 - **继承**。 - **构造函数与析构函数**。 - **联合体**。 - **位字段**。 - **重载函数**。 - **重载操作符**。 - **模板**。 - **线程**。 - **异常处理**。 - **预处理指令**。 #### 七、编译器优化 这一部分探讨了编译器如何进行优化以及不同编译器之间的比较,并讨论了阻碍编译器优化的因素,包括编译器优化选项和优化指导原则等。 #### 八、优化内存访问 优化内存访问是提高程序性能的重要手段之一。这部分讨论了代码和数据的缓存机制,以及如何通过合理布局函数和数据结构来减少内存访问延迟等问题。 通过上述各个方面的深入探讨,《C++代码优化》这本书为我们提供了一套全面而系统的优化指南,帮助开发者在实际工作中更好地理解和应用优化技术,从而提高程序的执行效率。
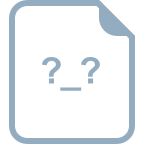
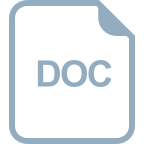
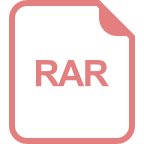
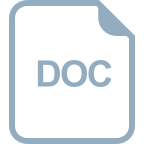
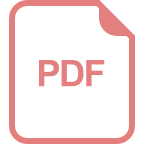
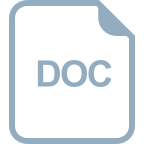
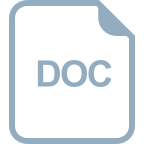
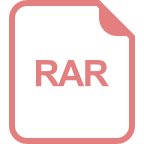
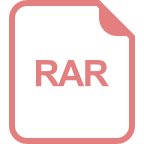
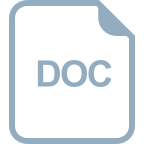
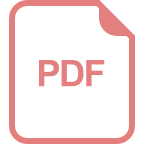
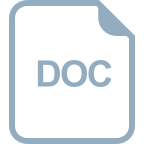
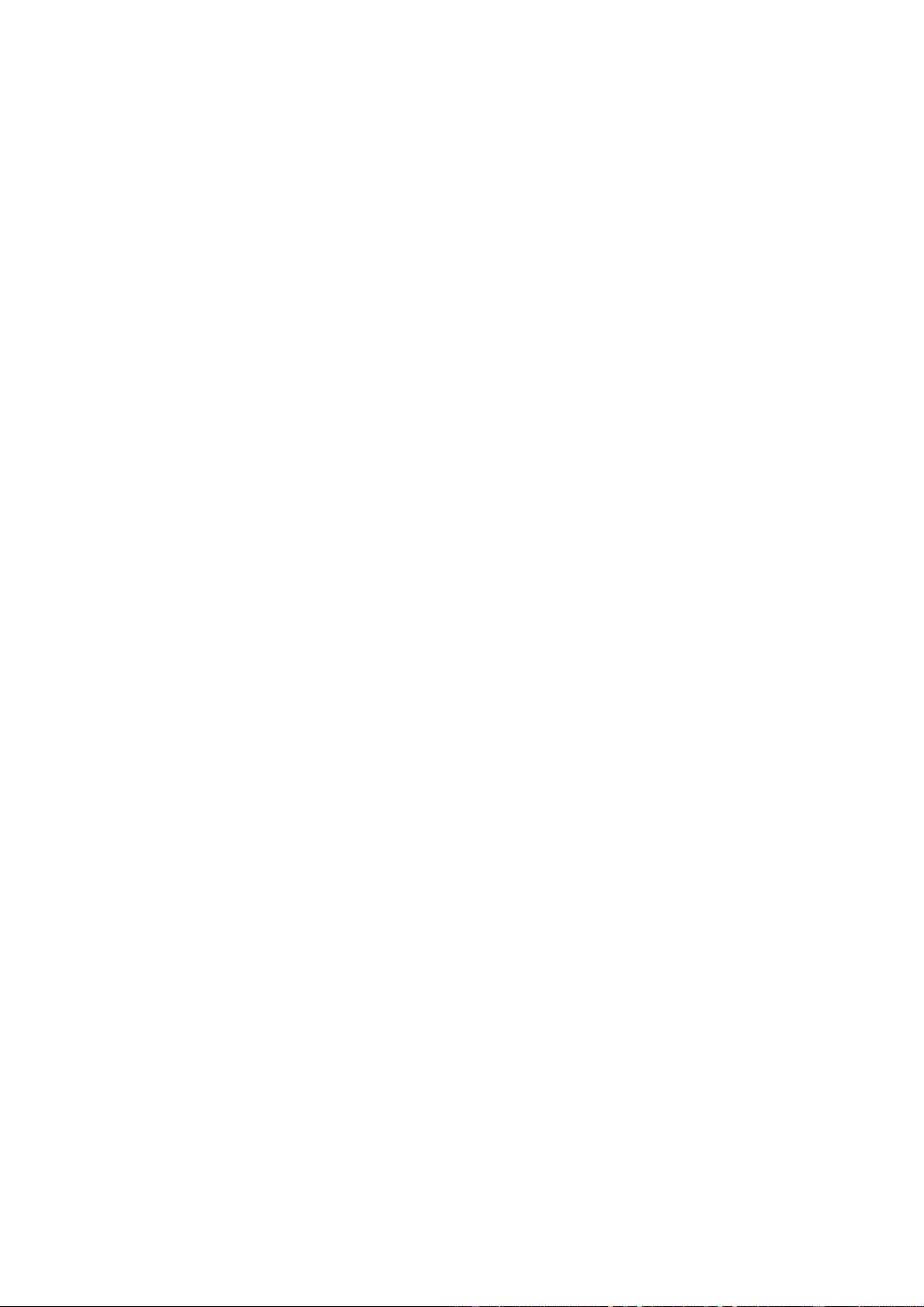
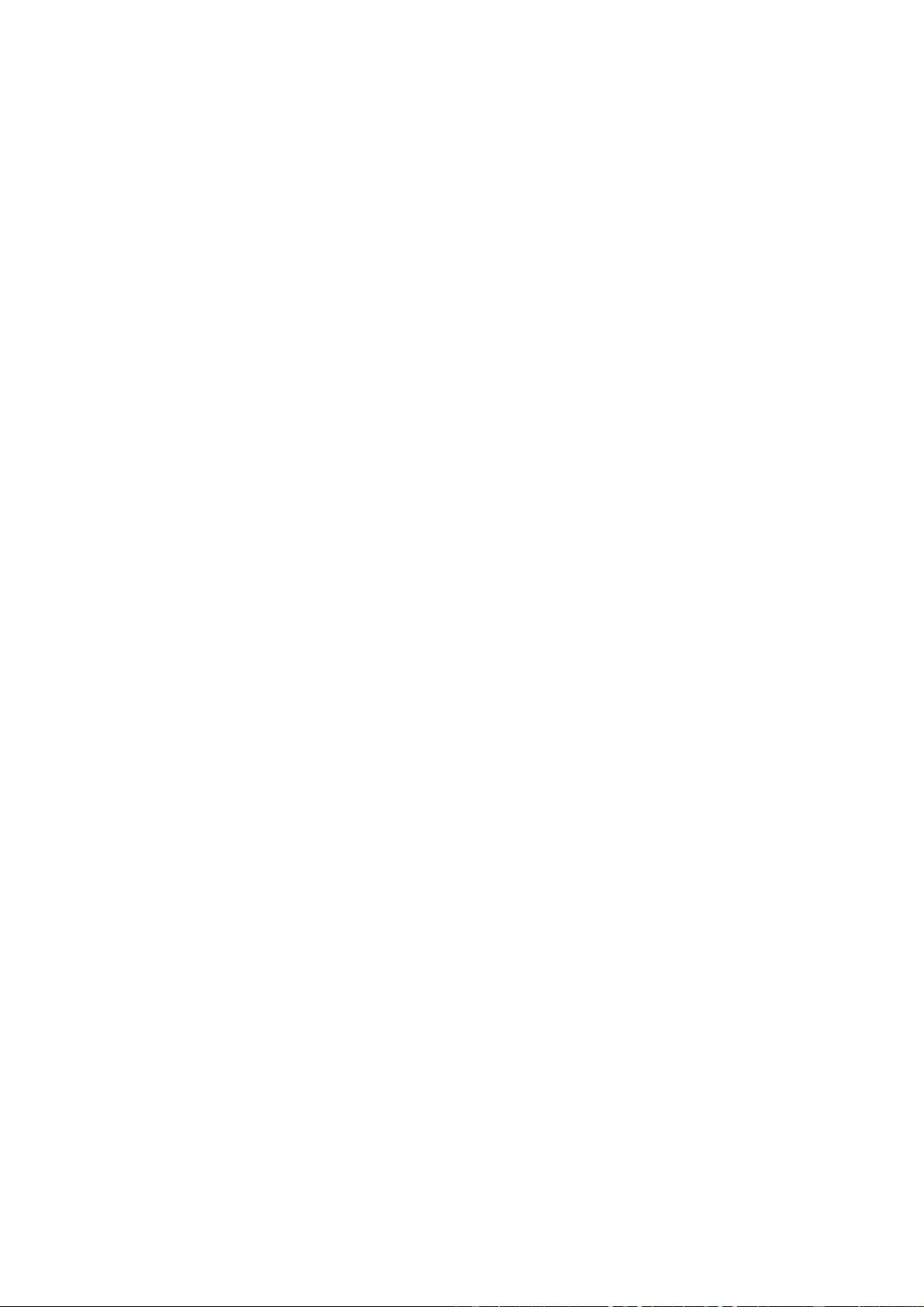
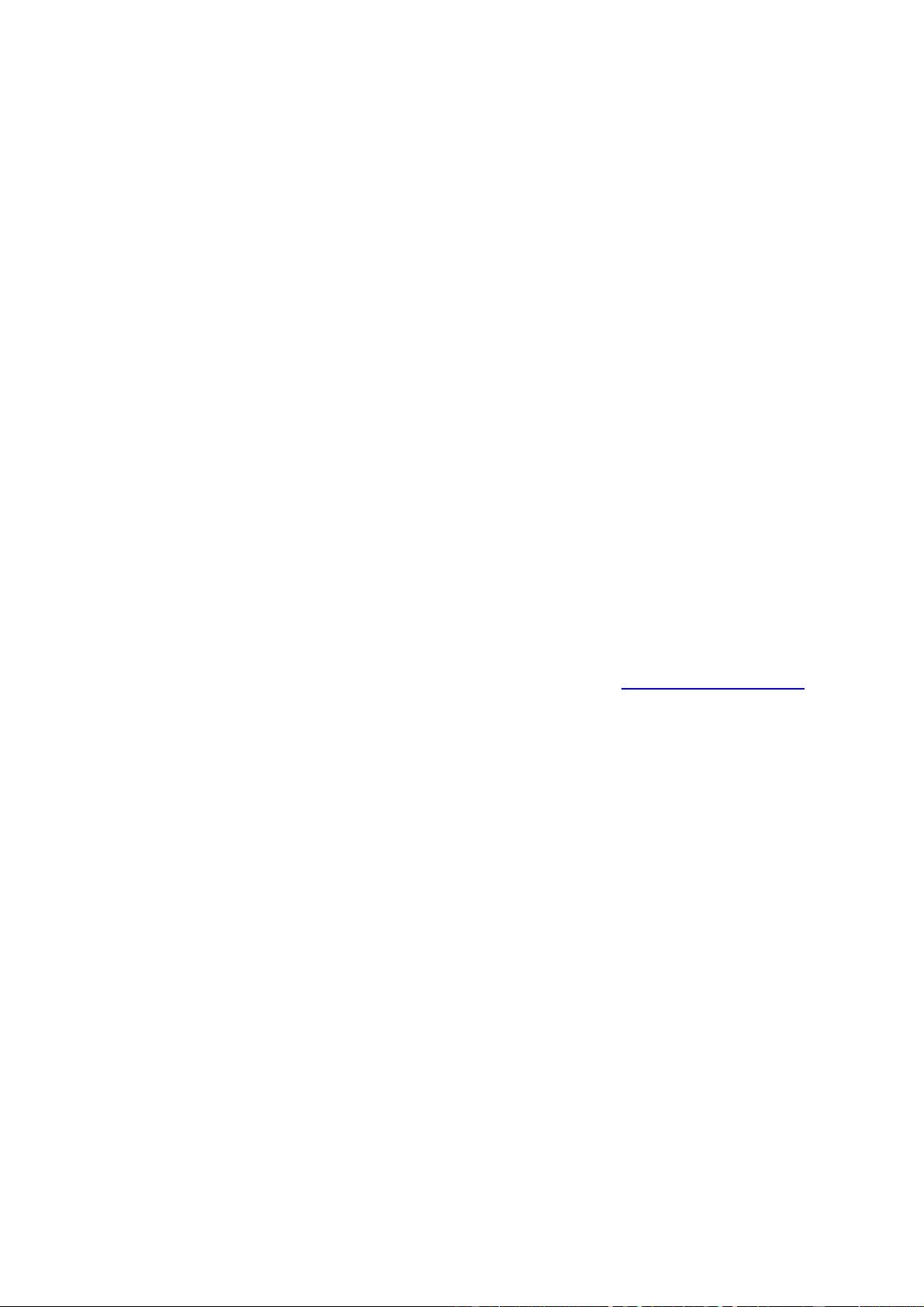
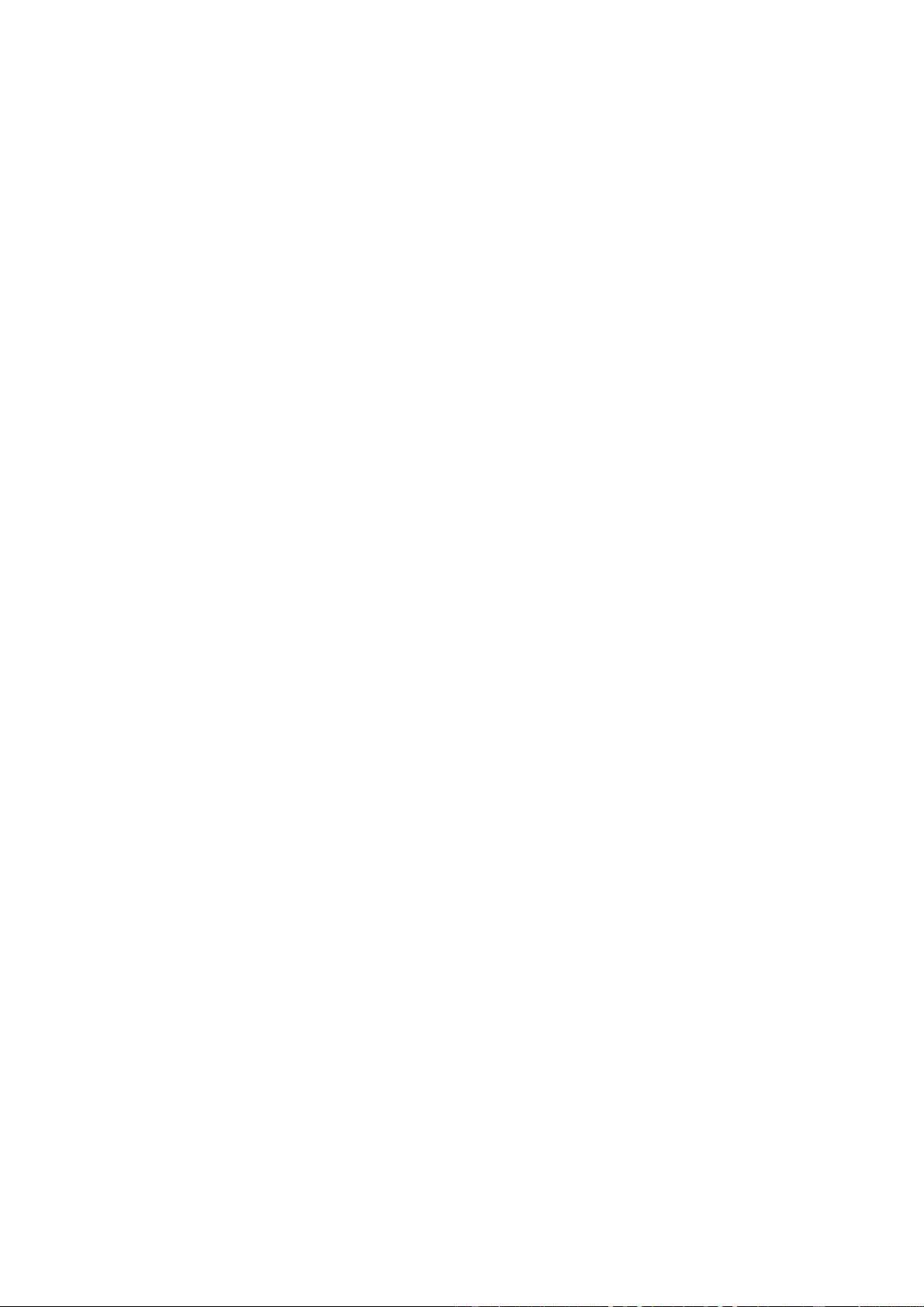
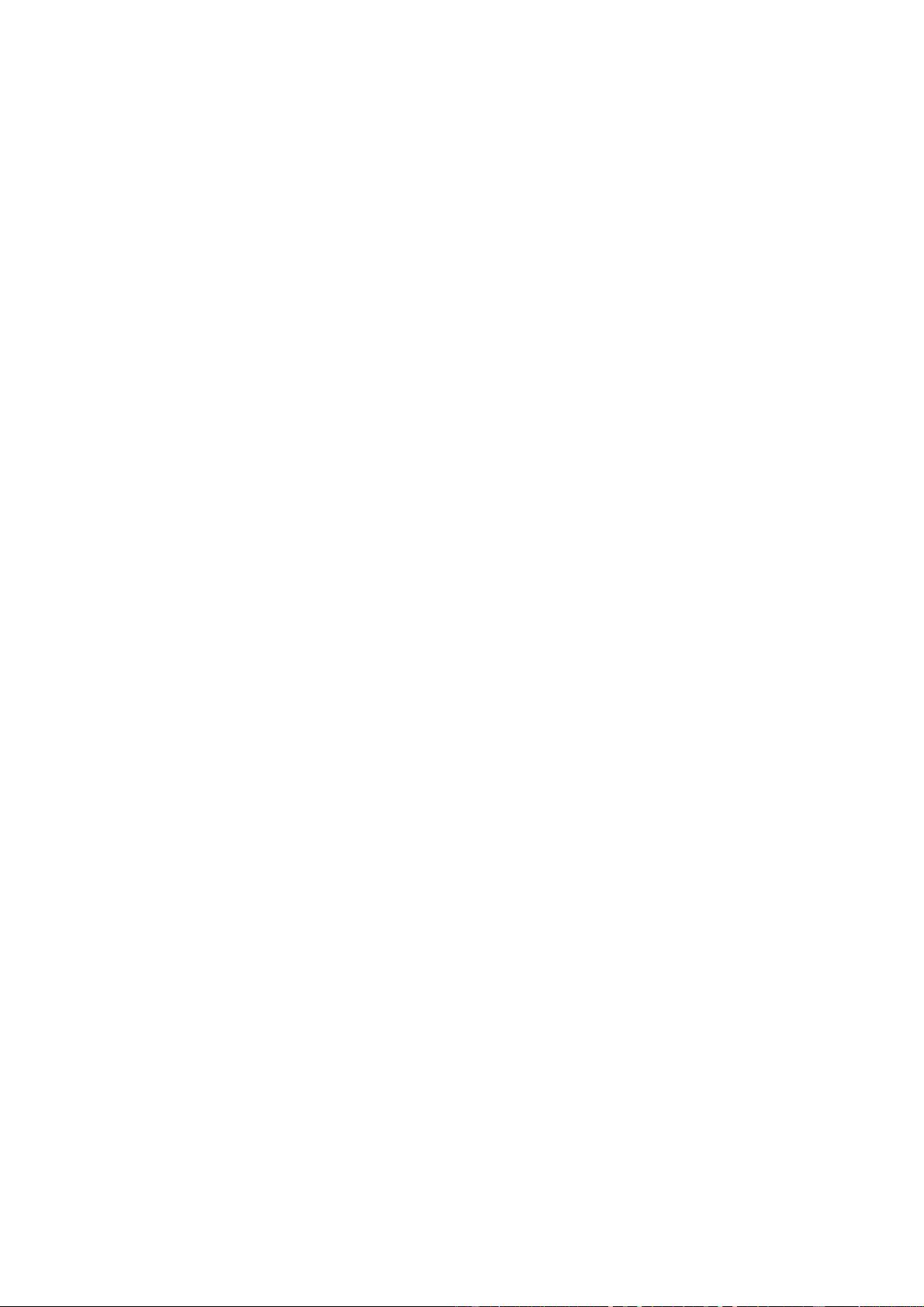
剩余132页未读,继续阅读
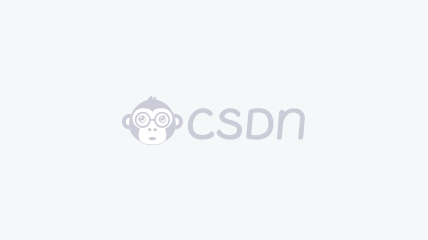

- 粉丝: 15
- 资源: 92
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

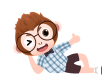
最新资源
- ABAQUS高速铁路板式无砟轨道耦合动力学模型
- 短路电流计算 Matlab编程计算 针对常见的四种短路故障(单相接地短路,两相相间短路,两相接地短路,三相短路),可采取三种方法进行计算: 1.实用短路电流计算 2.对称分量法计算 3
- 优化算法改进 Matlab 麻雀搜索算法,粒子群优化算法,鲸鱼优化算法,灰狼优化算法,黏菌优化算法等优化算法,提供算法改进点 改进后的优化算法也可应用于支持向量机,最小二乘支持向量机,随机森林,核
- 遗传算法优化极限学习机做预测,运行直接出图,包含真实值,ELM,GA-ELM对比,方便比较 智能优化算法,粒子群算法,花授粉算法,麻雀算法,鲸鱼算法,灰狼算法等,优化BP神经网络,支持向量机,极限学
- FX3U,FX5U,控制IO卡 ,STM32F407ZET6工控板,包括pcb,原理图 , PLC STMF32F407ZET6 FX-3U PCB生产方案 板载资源介绍 1. 8路高速脉冲加方向
- 利用matlab和simulink搭建的纯跟踪控制器用于单移线轨迹跟踪,效果如图 版本各为2018b和2019 拿后内容包含: 1、simulink模型 2、纯跟踪算法的纯matlab代码,便于理解
- 三相光伏并网逆变器设计,原理图,PCB,以及源代码 主要包括以下板卡: 1)主控DSP板, 负责逆变器的逆变及保护控制 原理图为pdf. pcb为AD文件 2)接口板,负责信号采集、处理,以及
- 考虑气电联合需求响应的 气电综合能源配网系统协调优化运行 该文提出气电综合能源配网系统最优潮流的凸优化方法,即利用二阶锥规划方法对配电网潮流方 程约束进行处理,并提出运用增强二阶锥规划与泰勒级数展开相
- 光子晶体BIC,OAM激发 若需第二幅图中本征态以及三维Q等计算额外
- 基于共享储能电站的工业用户日前优化经济调度,通过协调各用户使用共享储能电站进行充放电,实现日运行最优 代码环境:matlab+yalmip+cplex gurobi ,注释详尽,结果正确 对学习储
- 三相PWM整流器simulink仿真模型,采用双闭关PI控制,SVPWM调制策略,可以实现很好的整流效果,交流侧谐波含量低,可以很好的应对负载突变等复杂工况
- 红外遥控器+红外一体化接收头部分的仿真 带程序 红外线编码是数据传输和家用电器遥控常用的一种通讯方法,其实质是一种脉宽调制的串行通讯 家电遥控中常用的红外线编码电路有μPD6121G型HT622型和
- 新能源系统低碳优化调度(用Matlab) 包含各类分布式电源消纳、热电联产、电锅炉、储能电池、天然气等新能源元素,实现系统中各种成本的优化,调度 若有需要,我也有matlab
- Matlab 遗传算法解决0-1背包问题(装包问题) 源码+详细注释 问题描述:已知不同物品质量与不同背包最大载重,求取最优值使得所有背包所装得的物品质量总和最大 可以改物品质量与背包载重数据
- 信捷plc控制3轴机械臂调试程序,只是调试程序,包含信捷plc程序,信捷触摸屏程序,手机组态软件程序,含手机组态软件 程序自己写的,后期还会增加相关项目 触摸屏示教程序写好,可以任意示教完成全部动
- ABS制动系统开发 PID控制 开关控制 matlab simulink carsim联合仿真,下面视频为pid控制效果和不带ABS的对比 滑移率控制目标20% 分离路面制动

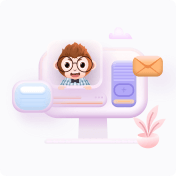
