# Copyright 2017 The TensorFlow Authors. All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# ==============================================================================
"""Tests for object_detection.utils.ops."""
import numpy as np
import tensorflow as tf
from object_detection.core import standard_fields as fields
from object_detection.utils import ops
class NormalizedToImageCoordinatesTest(tf.test.TestCase):
def test_normalized_to_image_coordinates(self):
normalized_boxes = tf.placeholder(tf.float32, shape=(None, 1, 4))
normalized_boxes_np = np.array([[[0.0, 0.0, 1.0, 1.0]],
[[0.5, 0.5, 1.0, 1.0]]])
image_shape = tf.convert_to_tensor([1, 4, 4, 3], dtype=tf.int32)
absolute_boxes = ops.normalized_to_image_coordinates(normalized_boxes,
image_shape,
parallel_iterations=2)
expected_boxes = np.array([[[0, 0, 4, 4]],
[[2, 2, 4, 4]]])
with self.test_session() as sess:
absolute_boxes = sess.run(absolute_boxes,
feed_dict={normalized_boxes:
normalized_boxes_np})
self.assertAllEqual(absolute_boxes, expected_boxes)
class MeshgridTest(tf.test.TestCase):
def test_meshgrid_numpy_comparison(self):
"""Tests meshgrid op with vectors, for which it should match numpy."""
x = np.arange(4)
y = np.arange(6)
exp_xgrid, exp_ygrid = np.meshgrid(x, y)
xgrid, ygrid = ops.meshgrid(x, y)
with self.test_session() as sess:
xgrid_output, ygrid_output = sess.run([xgrid, ygrid])
self.assertAllEqual(xgrid_output, exp_xgrid)
self.assertAllEqual(ygrid_output, exp_ygrid)
def test_meshgrid_multidimensional(self):
np.random.seed(18)
x = np.random.rand(4, 1, 2).astype(np.float32)
y = np.random.rand(2, 3).astype(np.float32)
xgrid, ygrid = ops.meshgrid(x, y)
grid_shape = list(y.shape) + list(x.shape)
self.assertEqual(xgrid.get_shape().as_list(), grid_shape)
self.assertEqual(ygrid.get_shape().as_list(), grid_shape)
with self.test_session() as sess:
xgrid_output, ygrid_output = sess.run([xgrid, ygrid])
# Check the shape of the output grids
self.assertEqual(xgrid_output.shape, tuple(grid_shape))
self.assertEqual(ygrid_output.shape, tuple(grid_shape))
# Check a few elements
test_elements = [((3, 0, 0), (1, 2)),
((2, 0, 1), (0, 0)),
((0, 0, 0), (1, 1))]
for xind, yind in test_elements:
# These are float equality tests, but the meshgrid op should not introduce
# rounding.
self.assertEqual(xgrid_output[yind + xind], x[xind])
self.assertEqual(ygrid_output[yind + xind], y[yind])
class OpsTestPadToMultiple(tf.test.TestCase):
def test_zero_padding(self):
tensor = tf.constant([[[[0.], [0.]], [[0.], [0.]]]])
padded_tensor = ops.pad_to_multiple(tensor, 1)
with self.test_session() as sess:
padded_tensor_out = sess.run(padded_tensor)
self.assertEqual((1, 2, 2, 1), padded_tensor_out.shape)
def test_no_padding(self):
tensor = tf.constant([[[[0.], [0.]], [[0.], [0.]]]])
padded_tensor = ops.pad_to_multiple(tensor, 2)
with self.test_session() as sess:
padded_tensor_out = sess.run(padded_tensor)
self.assertEqual((1, 2, 2, 1), padded_tensor_out.shape)
def test_padding(self):
tensor = tf.constant([[[[0.], [0.]], [[0.], [0.]]]])
padded_tensor = ops.pad_to_multiple(tensor, 4)
with self.test_session() as sess:
padded_tensor_out = sess.run(padded_tensor)
self.assertEqual((1, 4, 4, 1), padded_tensor_out.shape)
class OpsTestPaddedOneHotEncoding(tf.test.TestCase):
def test_correct_one_hot_tensor_with_no_pad(self):
indices = tf.constant([1, 2, 3, 5])
one_hot_tensor = ops.padded_one_hot_encoding(indices, depth=6, left_pad=0)
expected_tensor = np.array([[0, 1, 0, 0, 0, 0],
[0, 0, 1, 0, 0, 0],
[0, 0, 0, 1, 0, 0],
[0, 0, 0, 0, 0, 1]], np.float32)
with self.test_session() as sess:
out_one_hot_tensor = sess.run(one_hot_tensor)
self.assertAllClose(out_one_hot_tensor, expected_tensor, rtol=1e-10,
atol=1e-10)
def test_correct_one_hot_tensor_with_pad_one(self):
indices = tf.constant([1, 2, 3, 5])
one_hot_tensor = ops.padded_one_hot_encoding(indices, depth=6, left_pad=1)
expected_tensor = np.array([[0, 0, 1, 0, 0, 0, 0],
[0, 0, 0, 1, 0, 0, 0],
[0, 0, 0, 0, 1, 0, 0],
[0, 0, 0, 0, 0, 0, 1]], np.float32)
with self.test_session() as sess:
out_one_hot_tensor = sess.run(one_hot_tensor)
self.assertAllClose(out_one_hot_tensor, expected_tensor, rtol=1e-10,
atol=1e-10)
def test_correct_one_hot_tensor_with_pad_three(self):
indices = tf.constant([1, 2, 3, 5])
one_hot_tensor = ops.padded_one_hot_encoding(indices, depth=6, left_pad=3)
expected_tensor = np.array([[0, 0, 0, 0, 1, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 1, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 1, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 1]], np.float32)
with self.test_session() as sess:
out_one_hot_tensor = sess.run(one_hot_tensor)
self.assertAllClose(out_one_hot_tensor, expected_tensor, rtol=1e-10,
atol=1e-10)
def test_correct_padded_one_hot_tensor_with_empty_indices(self):
depth = 6
pad = 2
indices = tf.constant([])
one_hot_tensor = ops.padded_one_hot_encoding(
indices, depth=depth, left_pad=pad)
expected_tensor = np.zeros((0, depth + pad))
with self.test_session() as sess:
out_one_hot_tensor = sess.run(one_hot_tensor)
self.assertAllClose(out_one_hot_tensor, expected_tensor, rtol=1e-10,
atol=1e-10)
def test_return_none_on_zero_depth(self):
indices = tf.constant([1, 2, 3, 4, 5])
one_hot_tensor = ops.padded_one_hot_encoding(indices, depth=0, left_pad=2)
self.assertEqual(one_hot_tensor, None)
def test_raise_value_error_on_rank_two_input(self):
indices = tf.constant(1.0, shape=(2, 3))
with self.assertRaises(ValueError):
ops.padded_one_hot_encoding(indices, depth=6, left_pad=2)
def test_raise_value_error_on_negative_pad(self):
indices = tf.constant(1.0, shape=(2, 3))
with self.assertRaises(ValueError):
ops.padded_one_hot_encoding(indices, depth=6, left_pad=-1)
def test_raise_value_error_on_float_pad(self):
indices = tf.constant(1.0, shape=(2, 3))
with self.assertRaises(ValueError):
ops.padded_one_hot_encoding(indices, depth=6, left_pad=0.1)
def test_raise_value_error_on_float_depth(self):
indices = tf.constant(1.0, shape=(2, 3))
with self.assertRaises(ValueError):
ops.padded_one_hot_encoding(indices, depth=0.1, left_pad=2)
class OpsDenseToSparseBoxesTest(tf.test.TestCase):
def test_return_all_boxes_when_all_input_boxes_are_valid(self):
num_classes = 4
num_valid_boxes = 3
code_size = 4
dense_location_placeholder = tf.placeholder(tf.float32,
shape=(num_valid_boxes,
没有合适的资源?快使用搜索试试~ 我知道了~
python在线目标识别系统
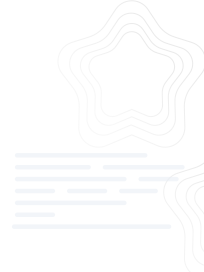
共69个文件
py:49个
jpg:8个
html:2个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 96 浏览量
2023-06-27
15:43:13
上传
评论
收藏 3.83MB ZIP 举报
温馨提示
python在线目标识别系统
资源推荐
资源详情
资源评论
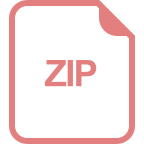
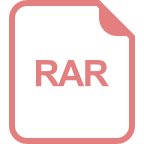
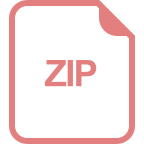
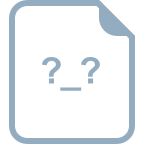
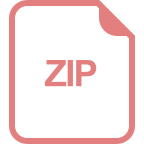
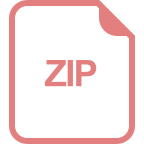
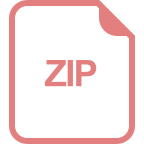
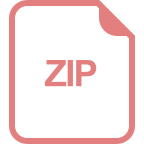
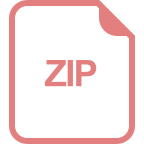
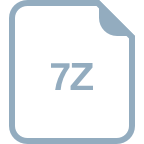
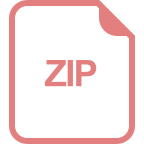
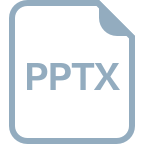
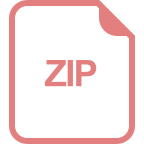
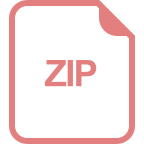
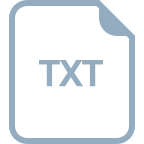
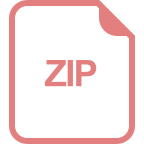
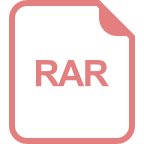
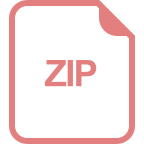
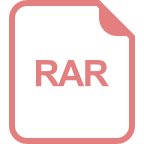
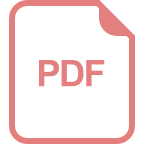
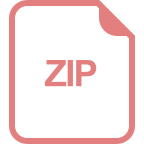
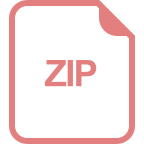
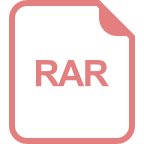
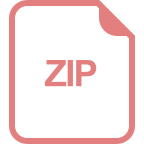
收起资源包目录




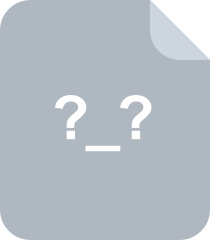



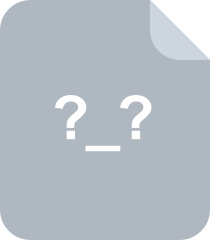

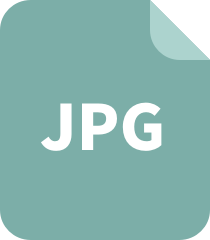
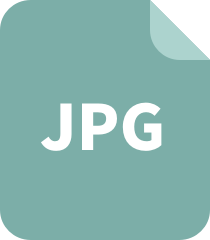
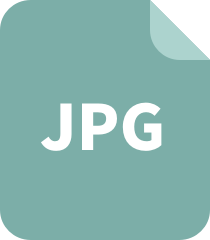
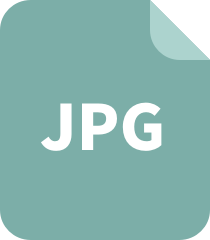
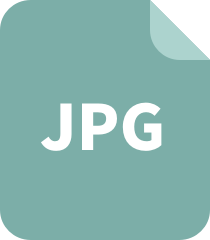
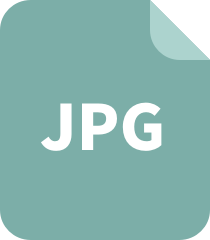
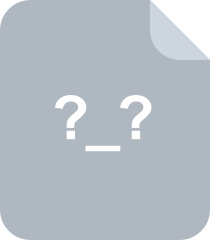

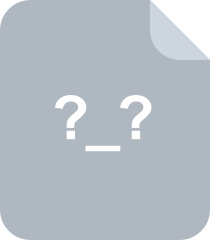

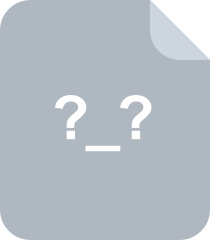
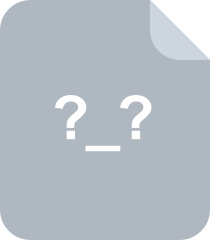
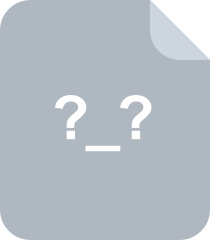
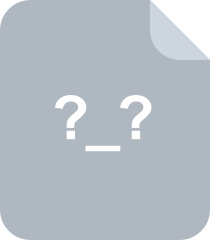
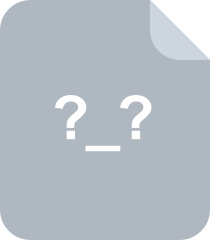
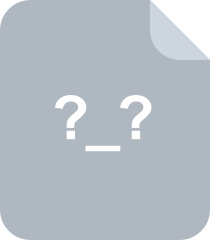
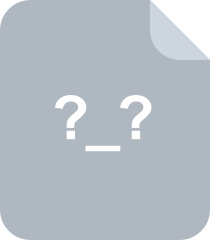
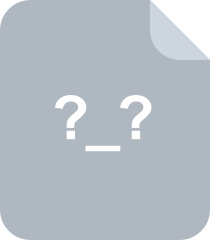
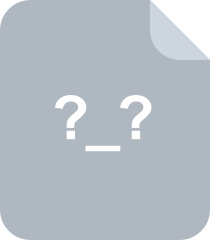
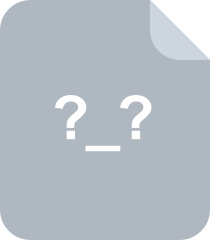
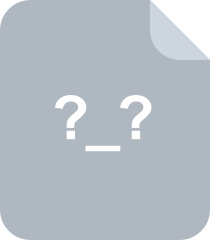
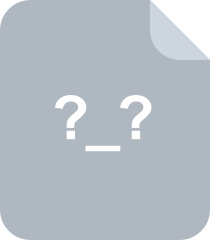
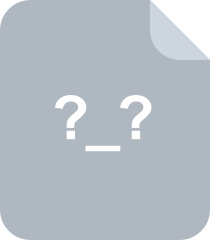
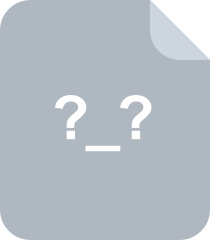
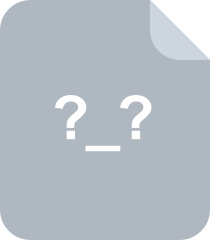
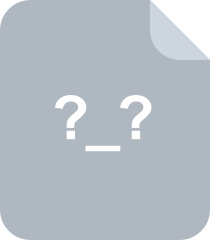
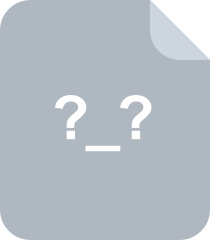
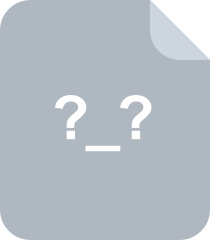
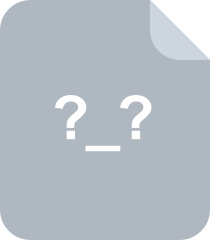
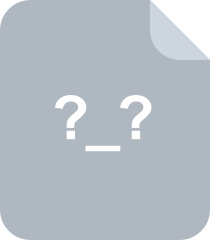
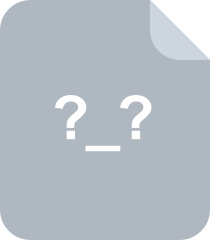
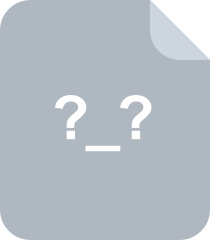
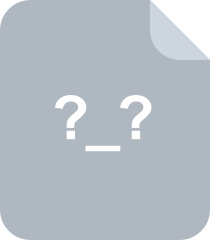
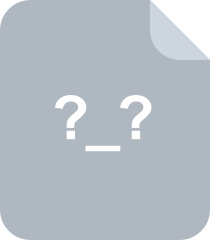
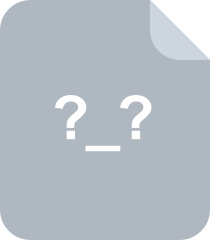
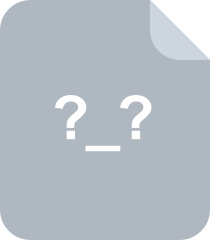
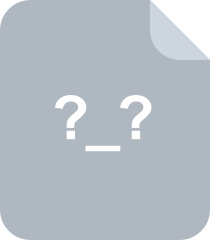
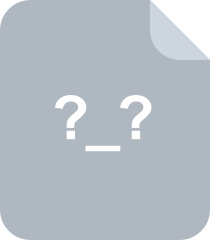
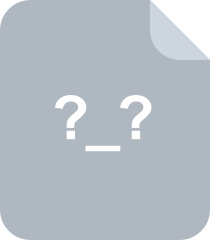
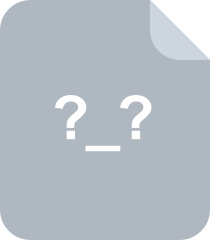
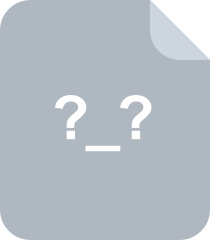
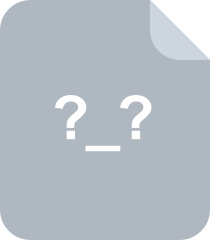
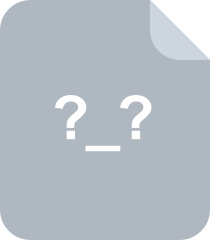
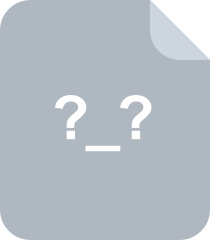
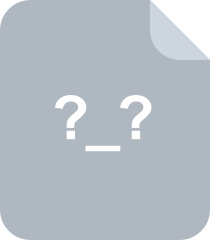
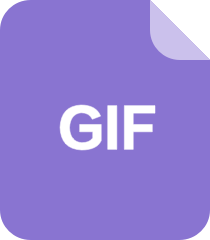
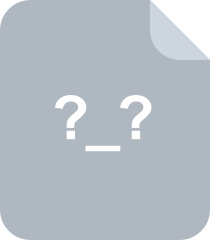
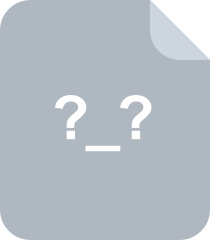

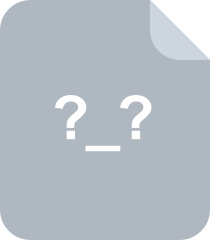
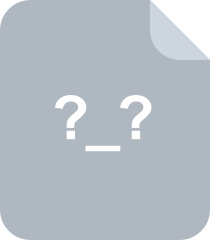

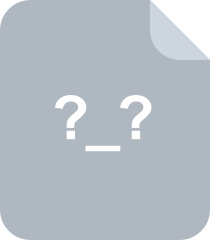
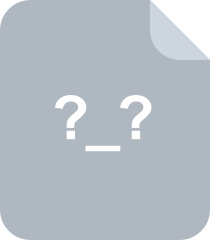
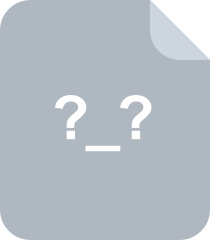
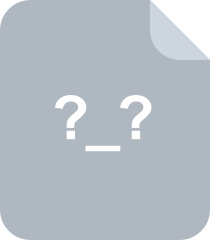


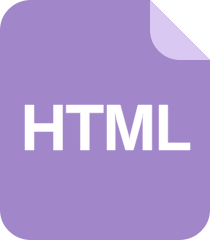
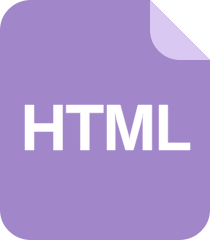
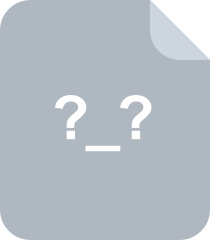



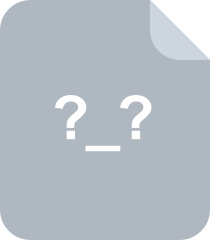

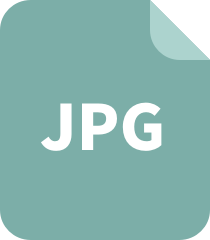
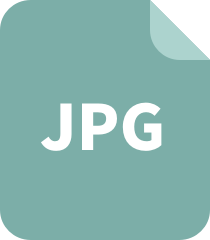

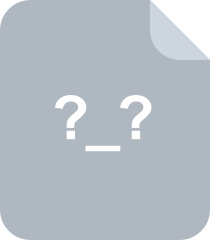
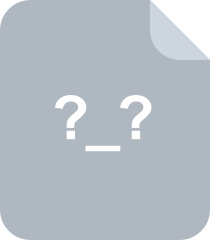
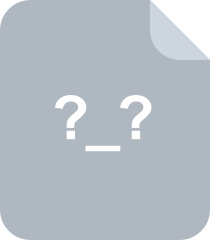

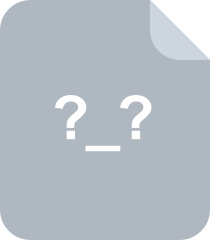
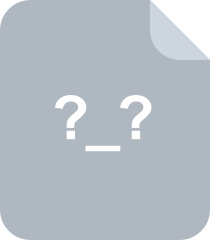

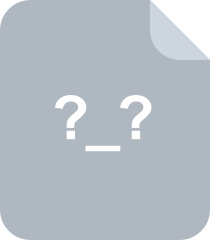

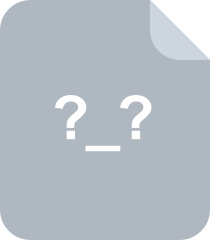
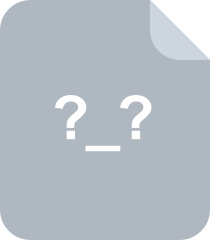
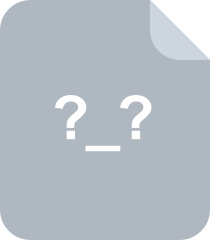
共 69 条
- 1
资源评论
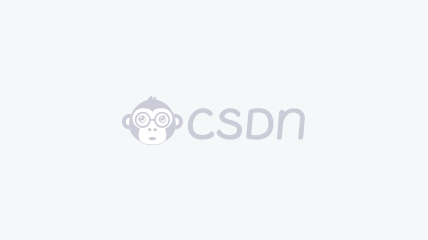

GeekyGuru
- 粉丝: 1750
- 资源: 1099
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

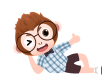
安全验证
文档复制为VIP权益,开通VIP直接复制
