### XSLT简便教程 #### XSLT简介与核心概念 XSLT(Extensible Stylesheet Language Transformations)是一种用于转换XML文档的标准语言。通过XSLT,开发人员能够将一个XML文档转换成另一种格式的文档,如HTML、PDF或其他类型的XML文档。这在处理动态数据、生成报告或构建网站时特别有用。 #### XSLT与XPath的关系 XSLT紧密地与XPath标准配合使用。XPath提供了一种查询和定位XML文档中元素和属性的方法,而XSLT则利用这些查询结果来定义如何转换源文档。简单来说,XPath告诉你“选什么”,而XSLT告诉你“怎么做”。 #### XSLT的加工机制 XSLT的加工过程是基于树结构的。它将源XML文档解析为一棵节点树,并按照XSLT样式表中的规则对这棵树进行操作,最终生成新的节点树作为输出。这个过程通常包括以下几个步骤: 1. **加载源文档**:XSLT处理器读取并解析输入的XML文档。 2. **应用样式表**:处理器根据提供的XSLT样式表中的指令执行转换。 3. **生成结果文档**:处理器根据样式表中的规则生成输出文档。 #### 单模板样式表 单模板样式表是最简单的XSLT样式表类型,它只包含一个模板,通常用于处理整个文档或者指定的特定节点。例如,可以创建一个模板来转换整个XML文档为HTML格式。 #### 理解输入和输出选项 在XSLT加工过程中,理解输入和输出的选项非常重要。这包括选择合适的编码方式、设置缩进和空白字符等。通过控制这些选项,开发人员可以更精细地控制输出文档的格式和外观。 #### 多模板提高灵活性 多模板样式表允许在一个样式表中定义多个模板,每个模板可以处理不同的节点类型。这种灵活性使得XSLT能够根据源文档的不同部分执行不同的转换逻辑。例如,一个模板可能专门用于处理标题,而另一个模板用于处理列表。 #### Oracle XSLT处理器的应用 在实际开发中,Oracle提供了强大的XSLT处理器支持。通过命令行工具`oraxsl`、程序接口API以及XSQL页面中的`<?xml-stylesheet?>`指令,开发人员可以灵活地集成XSLT到他们的应用程序中。例如,在之前的章节中已经展示了如何使用Oracle XSLT处理器将数据库驱动的XML转换为HTML页面、特定词汇的XML数据报、SQL脚本甚至是电子邮件。 #### 实例分析 考虑以下简单的XML文档示例: ```xml <!-- Emp.xml --> <ROWSET> <ROW num="1"> <EMPNO>7839</EMPNO> <!-- 更多节点 --> </ROW> <!-- 更多ROW节点 --> </ROWSET> ``` 为了将这个文档转换为HTML格式,我们可以创建一个简单的XSLT样式表: ```xslt <xsl:stylesheet version="1.0" xmlns:xsl="http://www.w3.org/1999/XSL/Transform"> <xsl:template match="/"> <html> <body> <xsl:apply-templates select="/ROWSET/ROW"/> </body> </html> </xsl:template> <xsl:template match="ROW"> <p>Employee Number: <xsl:value-of select="EMPNO"/></p> </xsl:template> </xsl:stylesheet> ``` 在这个样式表中,我们定义了两个模板:一个用于整个文档的转换,另一个用于处理每个`ROW`节点。通过这种方式,我们能够将XML文档中的员工编号转换为HTML格式显示。 #### 结论 XSLT作为一种强大且灵活的工具,对于任何希望利用XML文档的强大功能的人来说都是不可或缺的。无论是在Web开发中还是在数据处理领域,掌握XSLT都能够极大地提高工作效率并简化复杂的数据转换任务。通过深入了解XSLT的加工机制和高级特性,开发人员可以更好地利用这一工具解决实际问题。
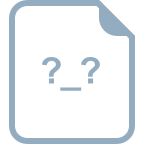
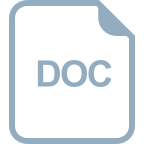
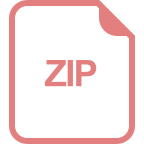
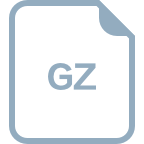
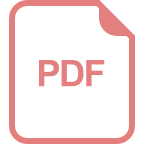
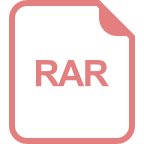
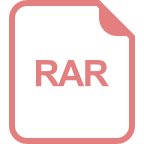
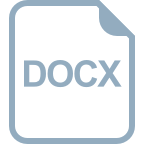
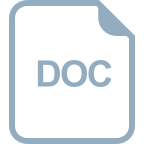
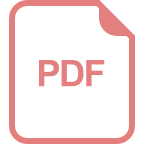
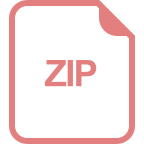
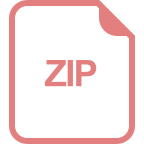
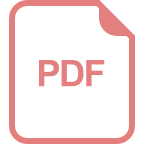
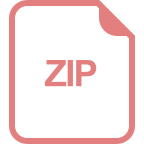
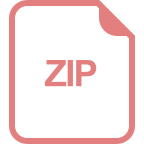
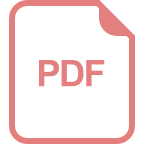
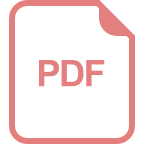
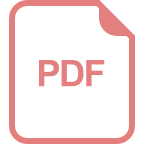
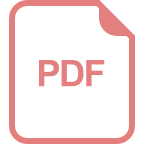
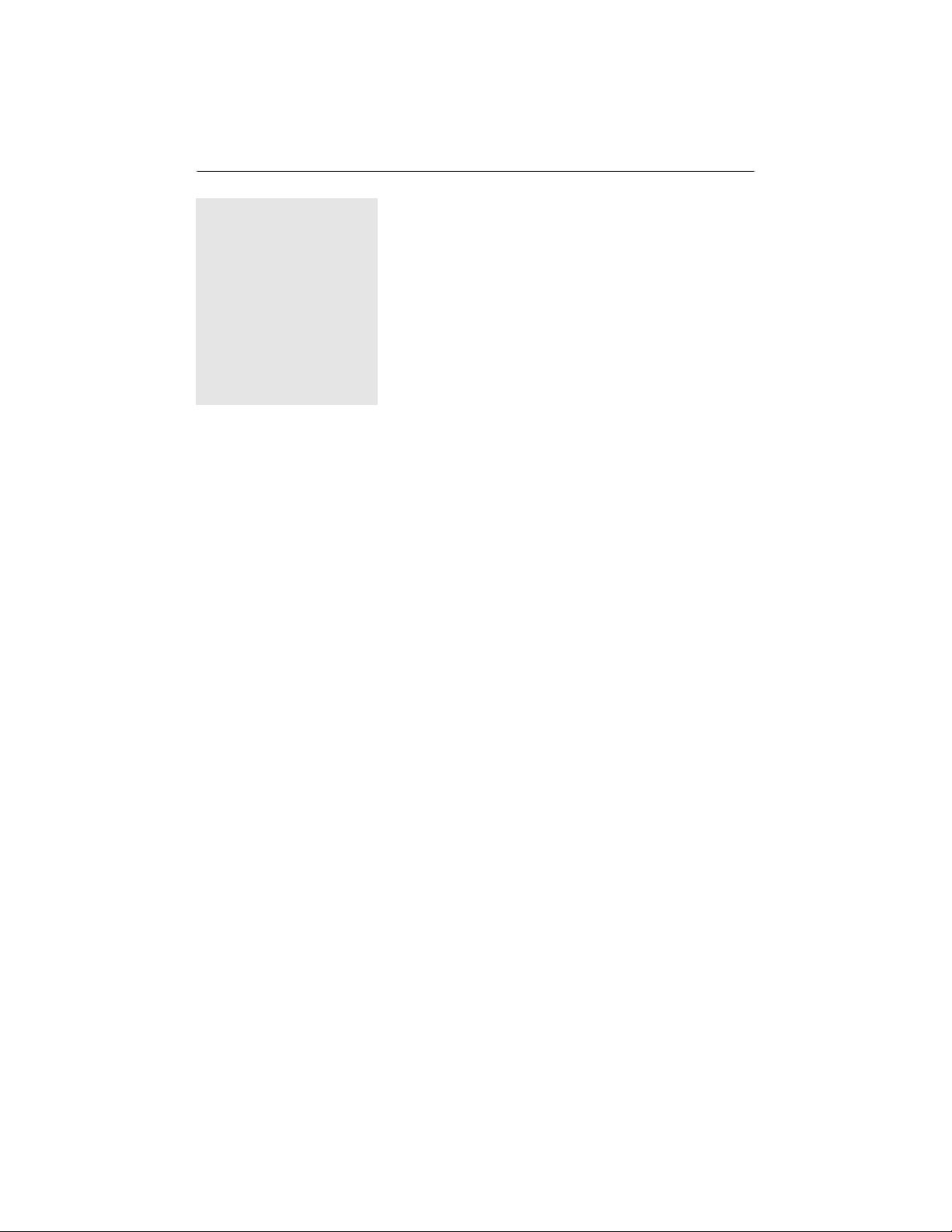
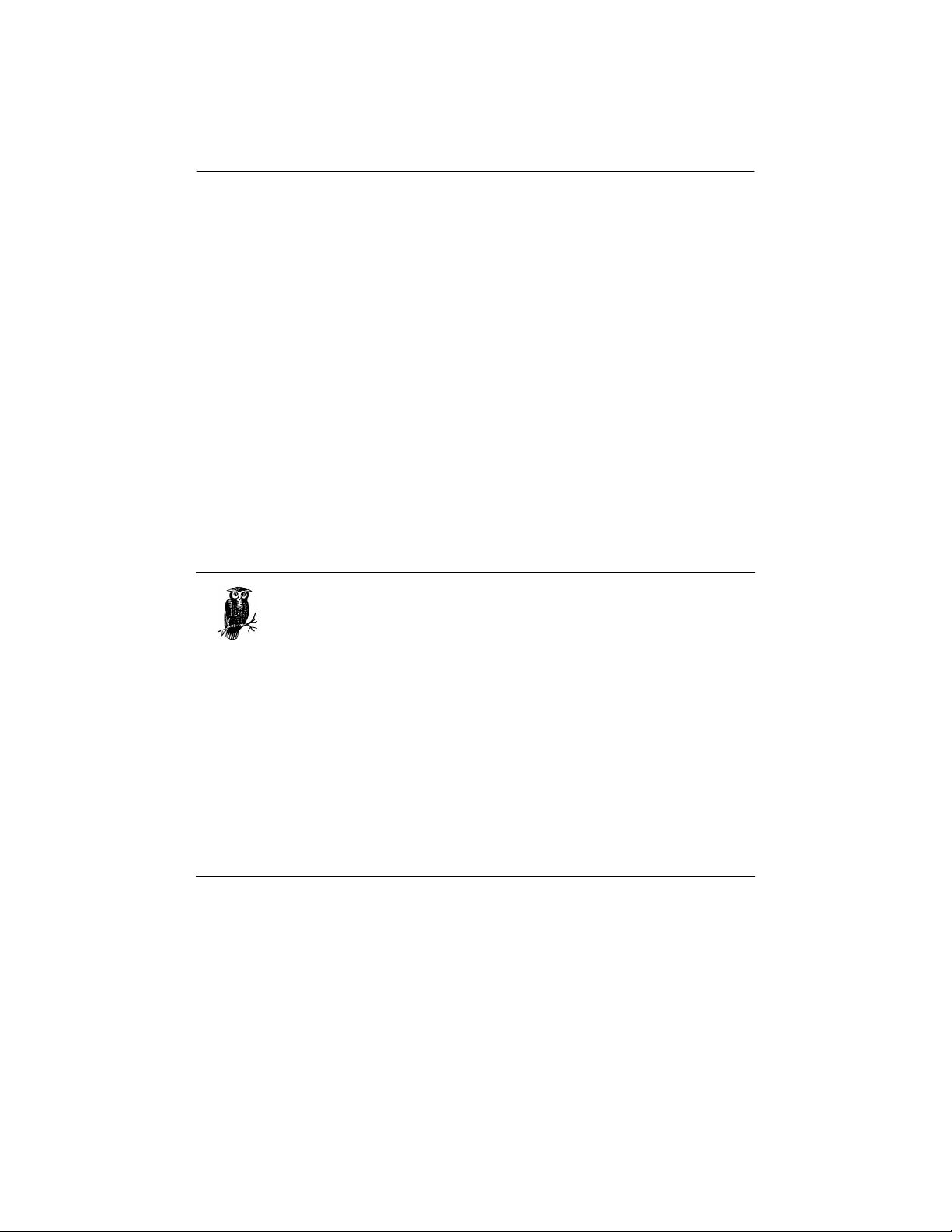
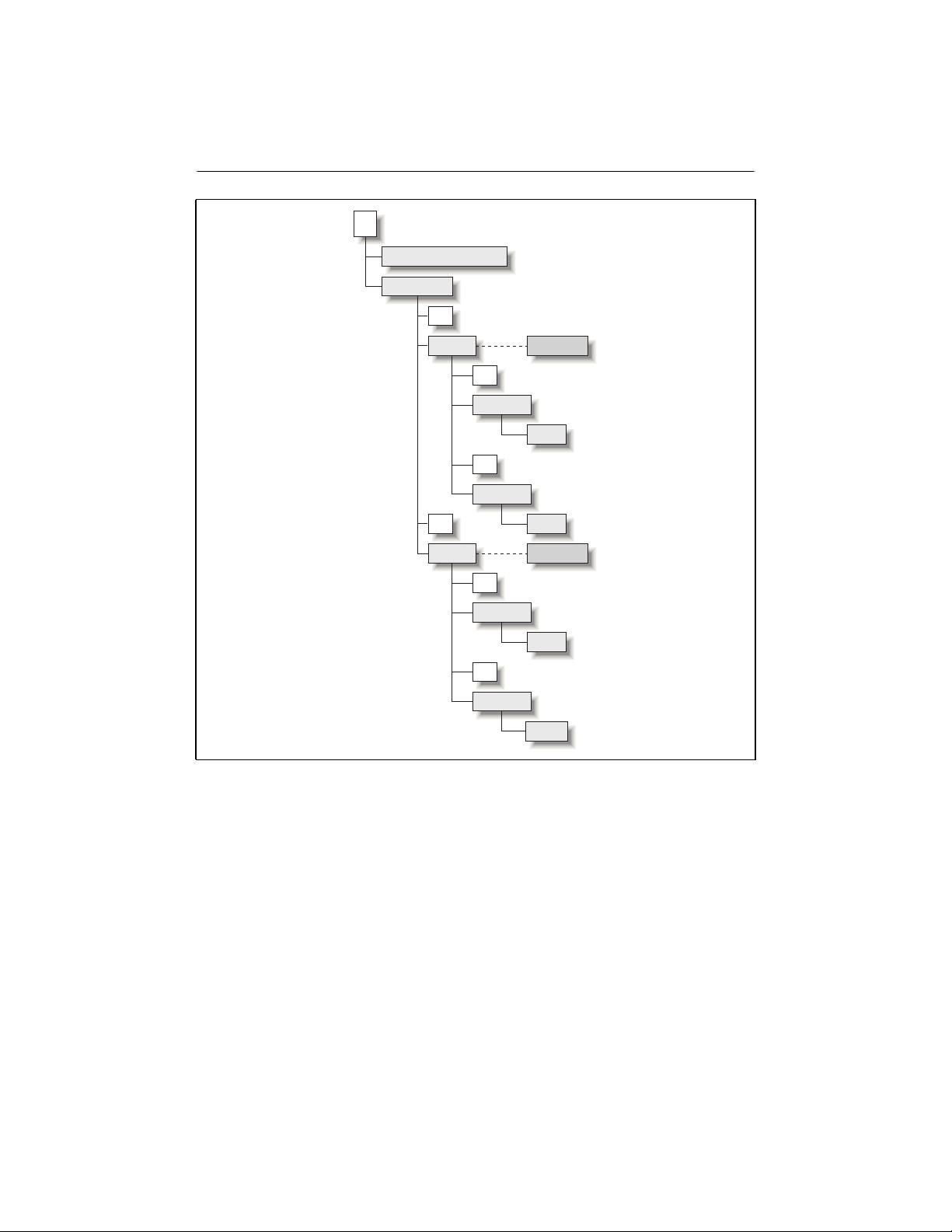
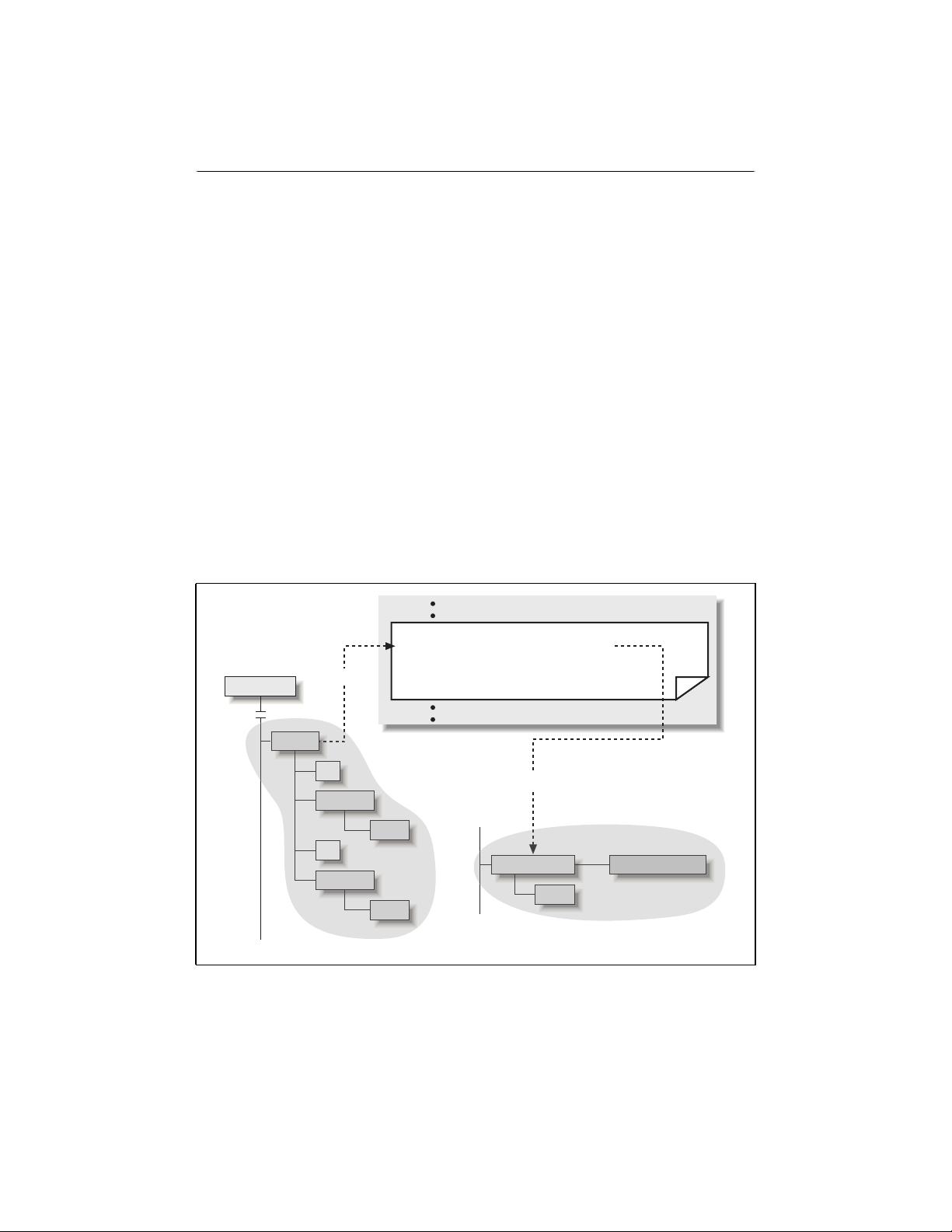
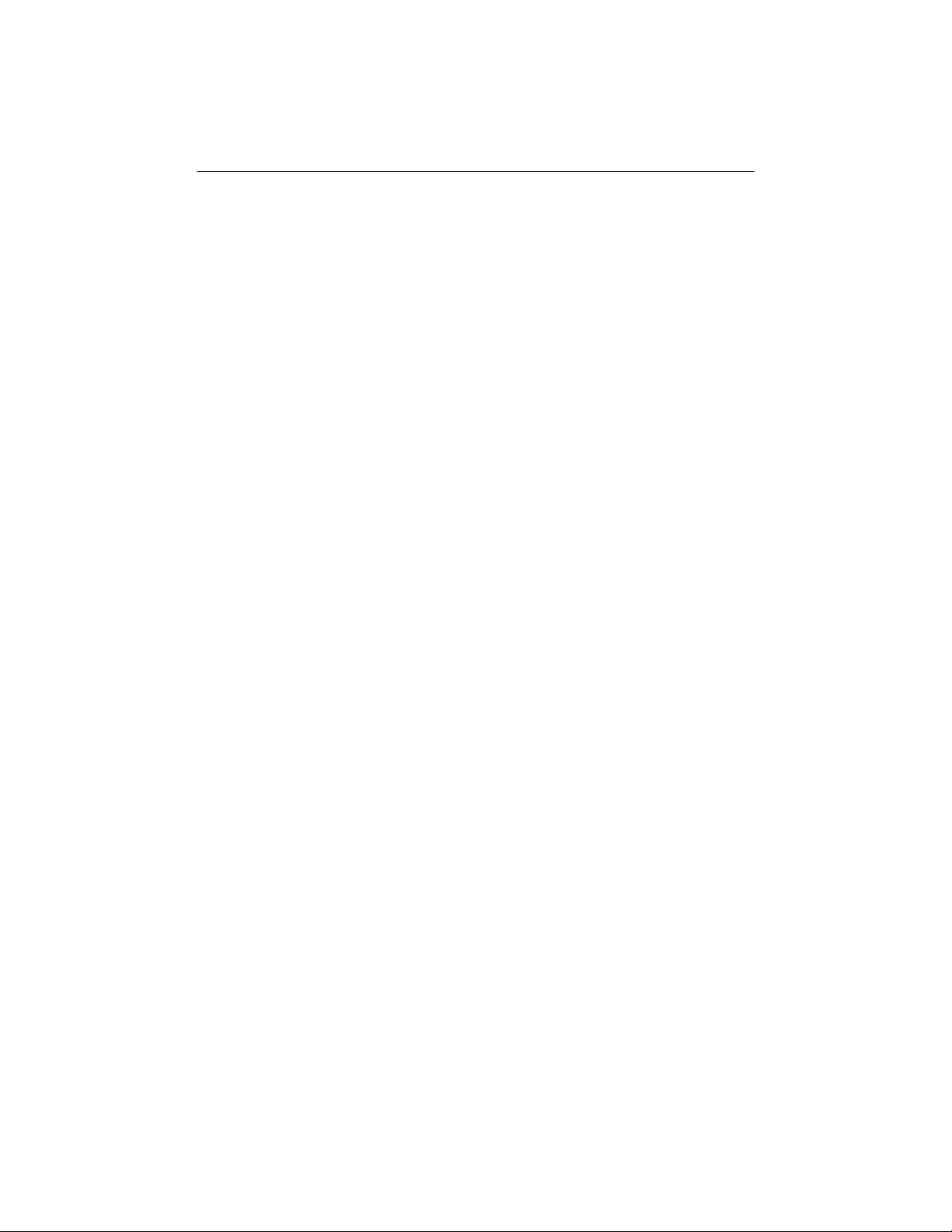
剩余34页未读,继续阅读
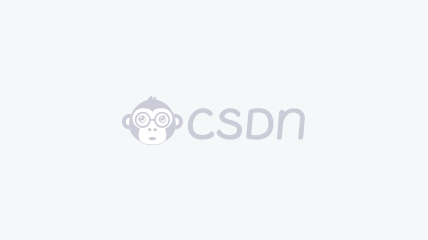

- 粉丝: 1
- 资源: 12
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

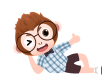
最新资源
- 使用Python和Pygame实现圣诞节动画效果
- 数据分析-49-客户细分-K-Means聚类分析
- 企业可持续发展性数据集,ESG数据集,公司可持续发展性数据(可用于多种企业可持续性研究场景)
- chapter9.zip
- 使用Python和Pygame库创建新年烟花动画效果
- 国际象棋检测10-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- turbovnc-2.2.6.x86-64.rpm
- 艾利和iriver Astell&Kern SP3000 V1.30升级固件
- VirtualGL-2.6.5.x86-64.rpm
- dbeaver-ce-24.3.1-x86-64-setup.exe

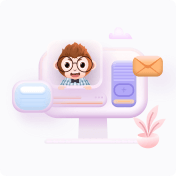
