在本文中,我们将深入探讨如何在嵌入式Linux系统中使用C/C++实现AirPlay接收器,以便将苹果设备(如iPhone或iPad)上的视频和图片无线投屏至电视机。AirPlay是Apple Inc.推出的一项功能,允许用户将多媒体内容从iOS、macOS设备流式传输到兼容的设备,如智能电视或扬声器。 我们需要理解嵌入式Linux系统的基本概念。嵌入式Linux是指在各种嵌入式设备中运行的定制版Linux操作系统,如机顶盒。这些系统通常具有资源受限的硬件,因此需要轻量级内核和应用程序。C和C++编程语言因其高效性和对底层硬件的直接访问能力,常被用于开发嵌入式软件。 AirPlay接收器的实现涉及到多个关键组件和技术: 1. **网络通信**:AirPlay协议基于TCP/IP,因此需要熟悉socket编程来建立和维护网络连接。开发者需要实现一个服务器端程序,监听特定端口,等待客户端(即苹果设备)的连接请求。 2. **音频/视频流处理**:AirPlay支持实时音视频流传输,接收器需要能够解码并播放这些流。这可能涉及使用开源的多媒体库,如FFmpeg,它提供了音频和视频编解码器以及容器格式的支持。 3. **加密与安全**:为了确保数据的安全传输,AirPlay协议采用了加密技术。接收器需要实现相应的解密算法,例如AES-128,以解析加密的媒体数据。 4. **设备控制**:与电视机的交互可能需要通过HDMI CEC(消费电子控制)或其他遥控协议。这需要理解相关的硬件接口,并编写驱动程序来控制电视的开关、音量和输入源。 5. **事件处理与用户界面**:在机顶盒上展示AirPlay的状态和控制选项,可能需要一个简单的用户界面。可以使用C或C++库,如GTK+或Qt,来创建图形用户界面。 6. **多线程编程**:为了保证用户界面的响应性和媒体流的流畅性,通常会采用多线程设计。一个线程负责接收和解码媒体流,另一个线程处理用户输入和界面更新。 7. **性能优化**:考虑到嵌入式系统的资源限制,开发者需要关注代码的性能和内存占用。使用适当的数据结构和算法,以及内存管理策略,可以提高程序效率。 在"airplay_receiver"这个项目中,我们可以推测这是实现上述功能的源代码。通过分析和编译这个项目,开发者可以将AirPlay接收功能集成到他们的嵌入式Linux设备,让用户体验到与Apple设备无缝连接的便捷性。 构建一个AirPlay接收器是一项涉及网络编程、多媒体处理、加密、硬件交互和用户界面设计等多方面技能的任务。在嵌入式Linux环境中,开发者需要充分利用C/C++的灵活性和效率,以适应硬件限制,同时提供稳定且高性能的解决方案。
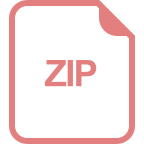
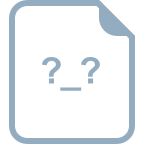
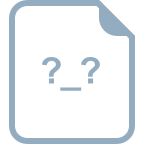
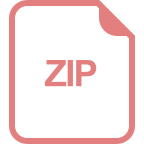
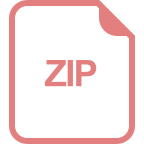
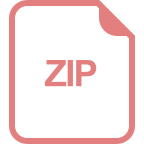
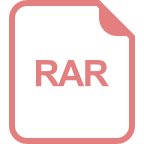
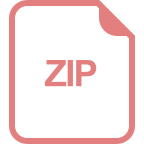
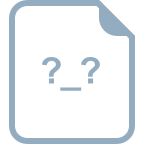
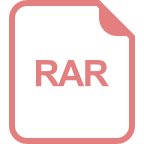
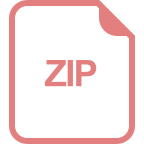
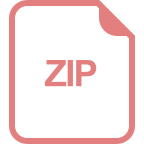
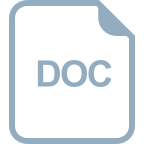
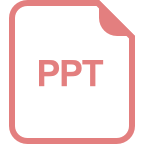
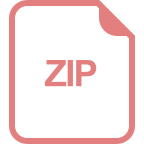
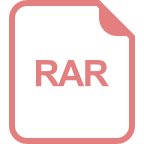
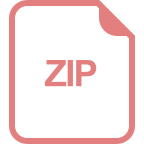
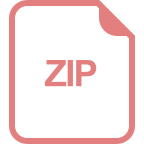


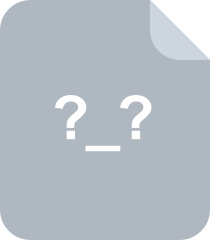
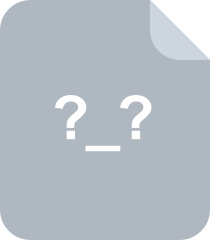
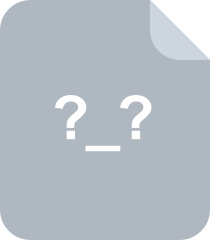
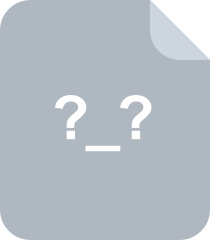
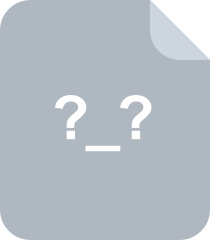
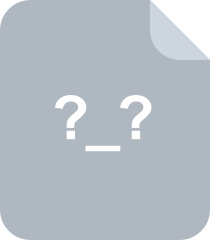
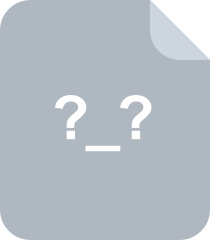
- 1
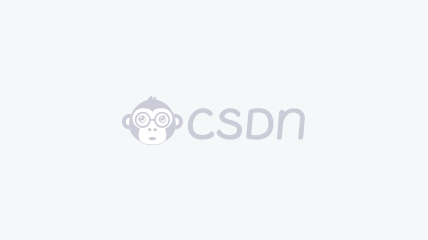
- DS1MGT2022-03-12用户下载后在一定时间内未进行评价,系统默认好评。

- 粉丝: 50
- 资源: 4万+
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

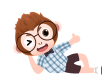
最新资源
- TMS320F28069控制500-1000Vdc 0-60A 30KW 三相PFC充电桩程序
- 双馈风机 DFIG 低电压穿越 MATLAB仿真模型 LVRT 双馈异步风力 Crowbar电路 (1)转子侧变器采用基于定子电压定向的矢量控制策略,有功无功解耦,具备MPPT能力,采用功率外环电
- 电机控制器,FPGA 硬件电流环 基于FPGA的永磁同步伺服控制系统的设计,在FPGA实现了伺服电机的矢量控制 有坐标变,电流环,速度环,位置环,电机反馈接口,SVPWM Verilog
- 鲁棒优化多阶段规划 利用列和约束生成(C&CG)算法进行求解 提升了配电网对可再生能源的消纳能力且改善了配电网的运行指标,同时又保证了微电网投资商的经济利益,有效实现了配电网与微电网的协调发展
- 脉振方波HFI HFI脉振方波高频注入模型代码和matlab仿真 码基于TI283x,:::仿真和相关文档齐全,有仿真的代码,仿真也可以生成代码
- 主机欧姆龙CP1H,主机带四轴,从机CP1H带数轴进行运动控制 全自动CE锂电极片多极耳连续冲切机 欧姆龙CP1H+MCGS昆仑通态触摸屏 伺服电机控制,电阻尺应用控制,电芯极耳间距定长冲切控制,涵盖
- 物流中心选址规划 帝企鹅优化调度算法 基于帝企鹅优化算法的全国物流中心选址规划算法MATLAB程序源代码及完整数据表格(Excel文件,详见附图) 程序到手可运行 关联词:备选点选址规划,调度
- 风机变桨控制FAST与MATLAB SIMULINK联合仿真模型非线性风力发电机的 PID独立变桨和统一变桨控制下仿真模型,对于5WM非线性风机风机进行控制 链接simulink的scope出转速对比
- 逆变器光伏并网逆变器资料,包含原理图,pcb,源码以及元器件明细表 如下: 1) 功率接口板原理图和pcb,元器件明细表 2) 主控DSP板原理图(pdf);如果有需要,可发mentor版
- PSASP环境下基于PMU同步测量的分区惯量估计方法,附资料 对应主要模式下的频率、分区联络线功率测量,做为PMU计算的依据: 1、恒功率负荷模式; 2、感应电动机负荷模式; 3、1模式基础上叠加2
- TMS320F28069控制500-1000Vdc 0-60A 30KW 三相PFC充电桩硬件设计
- 基于simulink的FCV燃料电池电动汽车模型 包含3个汽车模型,双输入DCDC模型,电池管理系统模型 模型建模清晰,运行良好,部分内容如截图所示 需要matlab2015b
- 基于Html与C#、CSS、JavaScript的Blazor入门课程设计源码
- 西门子1500PLC大型立体仓库堆垛机输送机程序项目,具体为智能物流实际项目案例,成熟并且稳定的运行现场,有一万多个库位,输送机一百多个,堆垛机八个,仓库分楼下和楼上两层,以西门子1500plc为控制
- 24V 65W 120W 350W反激电源 全套资料(原理图+PCB+变压器规格+测试报告)
- PMSM模型预测电流控制集(MPCC):单矢量,双矢量,三矢量;单步预测,两步预测,三步预测;两点平,三电平;无差拿预测...... 仿真模型和文档包括且不限于:见图

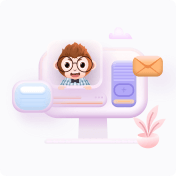
