package com.meession.am.dao.impl;
import java.sql.Connection;
import java.sql.Date;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.util.ArrayList;
import java.util.List;
import com.meession.am.dao.RouteDAO;
import com.meession.am.entity.Route;
public class RouteImpl implements RouteDAO {
private Connection conn = null;
public RouteImpl(Connection conn) {
this.conn = conn;
}
public boolean doCreateRoute(Route route) throws Exception { // 车次信息
boolean flag = false;
String sql = "INSERT INTO Route(name,trainType,fromStation,toStation,departureTime,arrivalTime,businessSeatCount,bPrice,specialSeatCount,spPrice,softSeatCount,sfPrice,hardSeatCount,hPrice,noSeatCount,nPrice,remark,fromStationId,toStationId) VALUES (?, ?, ?, ?, ?, ?, ?, ?, ?, ?, ? , ? , ? , ? , ? , ? , ?,(select id from Station where name = ?),(select id from Station where name = ? ))";
PreparedStatement pstmt = null;
try {
pstmt = this.conn.prepareStatement(sql);
pstmt.setString(1, route.getName());
pstmt.setString(2, route.getType());
pstmt.setString(3, route.getFromStation());
pstmt.setString(4, route.getToStation());
pstmt.setTimestamp(5, new java.sql.Timestamp(route.getDepartureTime().getTime()));
pstmt.setTimestamp(6, new java.sql.Timestamp(route.getArrivalTime().getTime()));
pstmt.setInt(7, route.getBusinessSeatCount());
pstmt.setInt(8, route.getbPrice());
pstmt.setInt(9,route.getSpecialSeatCount());
pstmt.setInt(10, route.getSpPrice());
pstmt.setInt(11, route.getSoftSeatCount());
pstmt.setInt(12, route.getSfPrice());
pstmt.setInt(13, route.getHardSeatCount());
pstmt.setInt(14, route.gethPrice());
pstmt.setInt(15, route.getNoSeatCount());
pstmt.setInt(16, route.getnPrice());
pstmt.setString(17, route.getRemark());
pstmt.setString(18, route.getFromStation());
pstmt.setString(19, route.getToStation());
if (pstmt.executeUpdate() > 0) {
flag = true;
}
} catch (Exception e) {
e.printStackTrace();
} finally {
if (pstmt != null) {
try {
pstmt.close();
} catch (Exception e2) {
e2.printStackTrace();
}
}
}
return flag;
}
public boolean doUpdateRoute(Route route) throws Exception { //更新车次
boolean flag = false;
PreparedStatement pstmt = null;
String sql = "UPDATE Route SET name = ?, trainType = ?, fromStation = ?, toStation = ?, departureTime = ?, arrivalTime = ?, businessSeatCount = ?, bPrice = ?, specialSeatCount = ?, spPrice = ?, softSeatCount = ?,sfPrice = ?,hardSeatCount = ?,hPrice = ?,noSeatCount = ?,nPrice = ? ,remark = ? WHERE id = ?";
try {
pstmt = this.conn.prepareStatement(sql);
pstmt.setString(1, route.getName());
pstmt.setString(2, route.getType());
pstmt.setString(3, route.getFromStation());
pstmt.setString(4, route.getToStation());
pstmt.setTimestamp(5, new java.sql.Timestamp(route.getDepartureTime().getTime()));
pstmt.setTimestamp(6, new java.sql.Timestamp(route.getArrivalTime().getTime()));
pstmt.setInt(7, route.getBusinessSeatCount());
pstmt.setInt(8, route.getbPrice());
pstmt.setInt(9,route.getSpecialSeatCount());
pstmt.setInt(10, route.getSpPrice());
pstmt.setInt(11, route.getSoftSeatCount());
pstmt.setInt(12, route.getSfPrice());
pstmt.setInt(13, route.getHardSeatCount());
pstmt.setInt(14, route.gethPrice());
pstmt.setInt(15, route.getNoSeatCount());
pstmt.setInt(16, route.getnPrice());
pstmt.setString(17, route.getRemark());
pstmt.setLong(18, route.getId());
if (pstmt.executeUpdate() > 0) {
flag = true;
}
} catch (Exception e) {
e.printStackTrace();
} finally {
if (pstmt != null) {
try {
pstmt.close();
} catch (Exception e2) {
e2.printStackTrace();
}
}
}
return flag;
}
public boolean doDeleteRoute(Long routeid) throws Exception {
boolean flag = false;
PreparedStatement pstmt = null;
String sql = "DELETE FROM Route WHERE id = ?";
try {
pstmt = this.conn.prepareStatement(sql);
pstmt.setLong(1, routeid);
if (pstmt.executeUpdate() > 0) {
flag = true;
}
} catch (Exception e) {
e.printStackTrace();
} finally {
if (pstmt != null) {
try {
pstmt.close();
} catch (Exception e2) {
e2.printStackTrace();
}
}
}
return flag;
}
public List<Route> findRoute(String fromStation,String toStation,Date departureTime) throws Exception { //查询车次
List<Route> all = new ArrayList<Route>();
PreparedStatement pstmt = null;
String sql = "SELECT * FROM route WHERE fromStation = ? and toStation = ? and departureTime LIKE ? ";
try {
pstmt = this.conn.prepareStatement(sql);
pstmt.setString(1, fromStation);
pstmt.setString(2, toStation);
pstmt.setString(3, departureTime+"%");
ResultSet rs = pstmt.executeQuery();
while (rs.next()) {
Route route = new Route();
route.setId(rs.getLong(1));
route.setName(rs.getString(2));
route.setType(rs.getString(3));
route.setFromStation(rs.getString(4));
route.setToStation(rs.getString(5));
route.setDepartureTime(rs.getTimestamp(6));
route.setArrivalTime(rs.getTimestamp(7));
route.setBusinessSeatCount(rs.getInt(8));
route.setbPirce(rs.getInt(9));
route.setSpecialSeatCount(rs.getInt(10));
route.setSpPrice(rs.getInt(11));
route.setSoftSeatCount(rs.getInt(12));
route.setSfPrice(rs.getInt(13));
route.setHardSeatCount(rs.getInt(14));
route.sethPrice(rs.getInt(15));
route.setNoSeatCount(rs.getInt(16));
route.setnPrice(rs.getInt(17));
route.setRemark(rs.getString(18));
route.setFromStationId(rs.getLong(19));
route.setToStationId(rs.getLong(20));
all.add(route);
}
rs.close();
} catch (Exception e) {
e.printStackTrace();
} finally {
if (pstmt != null) {
try {
pstmt.close();
} catch (Exception e2) {
e2.printStackTrace();
}
}
}
return all;
}
public List<Route> findAllRoute() throws Exception { //查询车次
List<Route> all = new ArrayList<Route>();
PreparedStatement pstmt = null;
String sql = "SELECT * FROM route ";
try {
pstmt = this.conn.prepareStatement(sql);
ResultSet rs = pstmt.executeQuery();
while (rs.next()) {
Route route = new Route();
route.setId(rs.getLong(1));

OldWinePot
- 粉丝: 9012
- 资源: 428
最新资源
- 养老院管理系统(小程序--论文pf-微信小程序毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 微信小程序软件缺陷管理系统ssm-微信小程序毕业项目,适合计算机毕-设、实训项目、大作业学习.rar
- 微信课堂助手小程序+php-微信小程序毕业项目,适合计算机毕-设、实训项目、大作业学习.rar
- 医笙小程序设计与前端开发_0d26l-微信小程序毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 医院预约挂号系统小程序--论文-微信小程序毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 医院核酸检测预约挂号微信小程序--论文-微信小程序毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 疫情核酸预约小程序-微信小程序毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 优选驾考小程序+ssm-微信小程序毕业项目,适合计算机毕-设、实训项目、大作业学习.rar
- 音乐播放器小程序--论文pf-微信小程序毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 微信外卖小程序+ssm-微信小程序毕业项目,适合计算机毕-设、实训项目、大作业学习.rar
- 游乐园智慧向导小程序-微信小程序毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 文章管理系统+ssm-微信小程序毕业项目,适合计算机毕-设、实训项目、大作业学习.rar
- 消防隐患在线举报系统开发+ssm-微信小程序毕业项目,适合计算机毕-设、实训项目、大作业学习.rar
- 微信阅读网站小程序+ssm-微信小程序毕业项目,适合计算机毕-设、实训项目、大作业学习.rar
- 小说实体书商城+ssm-微信小程序毕业项目,适合计算机毕-设、实训项目、大作业学习.rar
- 语言课学习系统的设计与实现--微信小程序-微信小程序毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


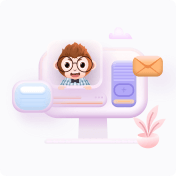