package com.chen.mvnbook.account.service;
import com.chen.mvnbook.account.captcha.AccountCaptchaException;
import com.chen.mvnbook.account.captcha.AccountCaptchaService;
import com.chen.mvnbook.account.captcha.RandomGenerator;
import com.chen.mvnbook.account.email.AccountEmailException;
import com.chen.mvnbook.account.email.AccountEmailService;
import com.chen.mvnbook.account.persist.Account;
import com.chen.mvnbook.account.persist.AccountPersistException;
import com.chen.mvnbook.account.persist.AccountPersistService;
import java.util.HashMap;
import java.util.Map;
/**
* Created by YouZeng on 2015-09-30.
*/
public class AccountServiceImpl implements AccountService {
private AccountPersistService accountPersistService;
private AccountEmailService accountEmailService;
private AccountCaptchaService accountCaptchaService;
private Map<String ,String> activationMap = new HashMap<String, String>();
@Override
public String generateCaptchaKey() throws AccountServiceException {
try{
return accountCaptchaService.generateCaptchaKey();
}catch (AccountCaptchaException e){
throw new AccountServiceException("Unable to generate Captcha Key.", e);
}
}
@Override
public byte[] generateCaptchaImage(String captchaKey) throws AccountServiceException {
try {
return accountCaptchaService.generateCaptchaImage(captchaKey);
} catch (AccountCaptchaException e) {
throw new AccountServiceException("Unable to generate Captcha Image", e);
}
}
@Override
public void signUp(SignUpRequest signUpRequest) throws AccountServiceException {
try{
if(!signUpRequest.getPassword().equals(signUpRequest.getConfirmPassword())){
throw new AccountServiceException("2 passwords do not match.");
}
if(!accountCaptchaService.validateCaptcha(signUpRequest.getCaptchaKey(), signUpRequest.getCaptchaValue())){
throw new AccountServiceException("Incorrect Captcha");
}
Account account = new Account();
account.setId(signUpRequest.getId());
account.setEmail(signUpRequest.getEmail());
account.setName(signUpRequest.getName());
account.setPassword(signUpRequest.getPassword());
account.setActivated(false);
accountPersistService.createAccount(account);
String activationId = RandomGenerator.getRandomString();
activationMap.put(activationId, account.getId());
String link = signUpRequest.getActivateServiceUrl().endsWith("/") ?
signUpRequest.getActivateServiceUrl() + activationId :
signUpRequest.getActivateServiceUrl() + "?key=" + activationId;
accountEmailService.sendMail(account.getEmail(), "Please Activate Your Account", link);
} catch (AccountCaptchaException e) {
e.printStackTrace();
} catch (AccountPersistException e) {
e.printStackTrace();
} catch (AccountEmailException e) {
e.printStackTrace();
}
}
@Override
public void activate(String activationNumber) throws AccountServiceException {
String accountId = activationMap.get(activationNumber);
if(accountId == null){
throw new AccountServiceException("Invalid account activation ID.");
}
try{
Account account = accountPersistService.readAccount(accountId);
account.setActivated(true);
accountPersistService.updateAccount(account);
} catch (AccountPersistException e) {
e.printStackTrace();
}
}
@Override
public void login(String id, String password) throws AccountServiceException {
try{
Account account = accountPersistService.readAccount(id);
if(account == null){
throw new AccountServiceException("account does not exists.");
}
if(!account.isActivated()){
throw new AccountServiceException("account is not disabled.");
}
if(!account.getPassword().equals(password)){
throw new AccountServiceException("incorrect password.");
}
} catch (AccountPersistException e) {
throw new AccountServiceException("Can't log in");
}
}
public AccountPersistService getAccountPersistService() {
return accountPersistService;
}
public void setAccountPersistService(AccountPersistService accountPersistService) {
this.accountPersistService = accountPersistService;
}
public AccountEmailService getAccountEmailService() {
return accountEmailService;
}
public void setAccountEmailService(AccountEmailService accountEmailService) {
this.accountEmailService = accountEmailService;
}
public AccountCaptchaService getAccountCaptchaService() {
return accountCaptchaService;
}
public void setAccountCaptchaService(AccountCaptchaService accountCaptchaService) {
this.accountCaptchaService = accountCaptchaService;
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
Maven实战学习代码.zip
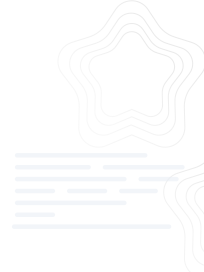
共54个文件
java:25个
xml:15个
properties:5个

需积分: 1 0 下载量 29 浏览量
2024-06-19
16:37:06
上传
评论
收藏 183KB ZIP 举报
温馨提示
Maven实战学习代码.zip account-aggregator account-captcha account-email account-parent account-persist account-service account-web hello-world src/main/webapp
资源推荐
资源详情
资源评论
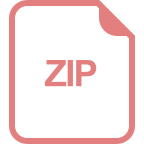
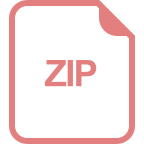
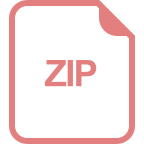
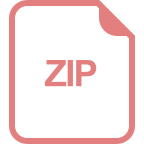
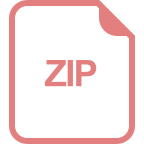
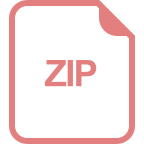
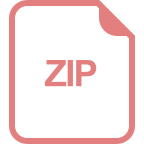
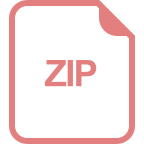
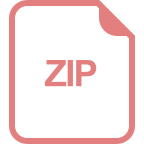
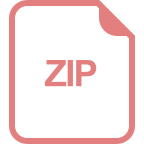
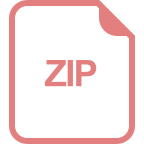
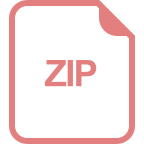
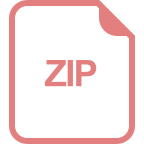
收起资源包目录

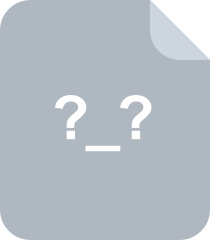

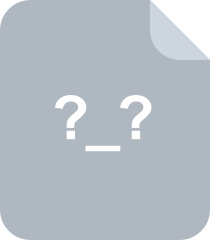

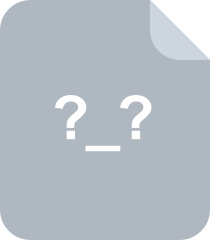








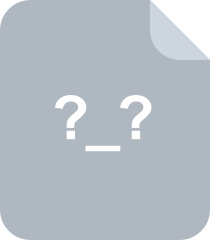
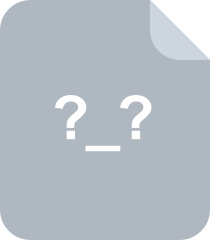


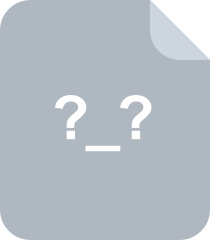
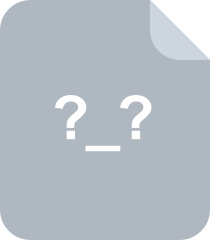

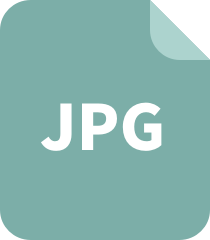
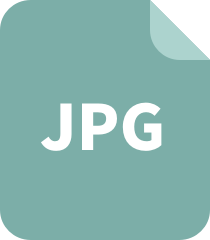

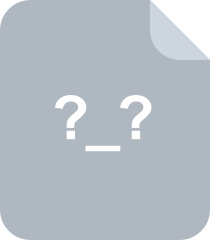

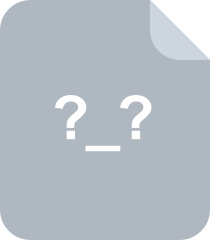
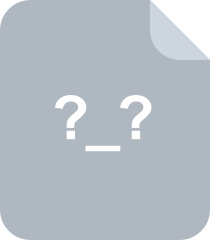




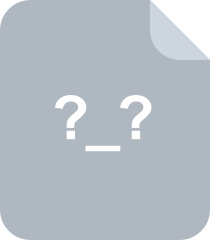
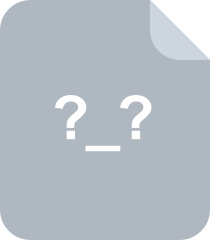

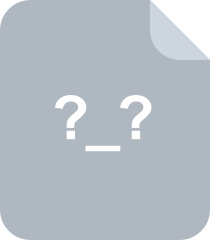

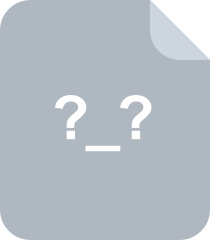

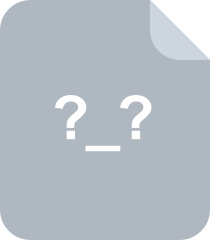



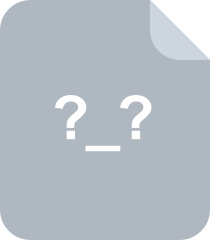






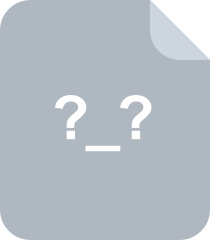


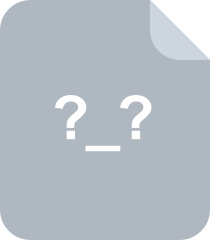
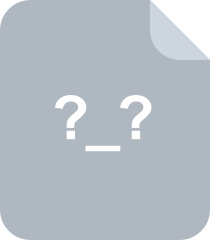






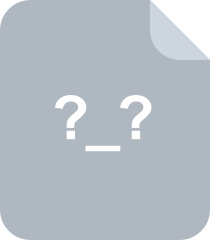
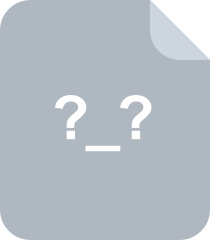
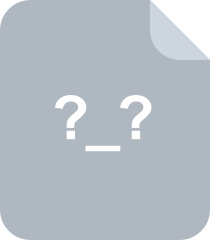
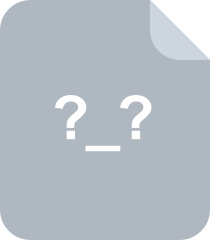

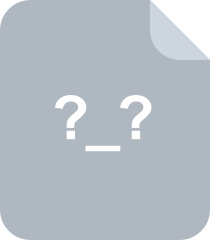



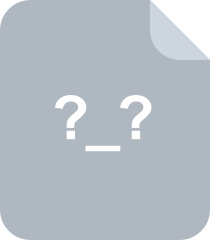





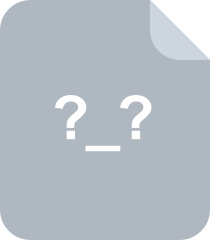


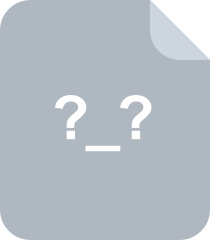
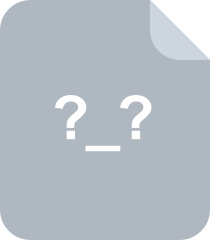






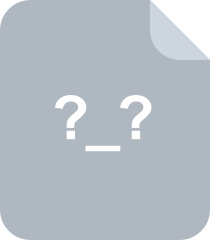
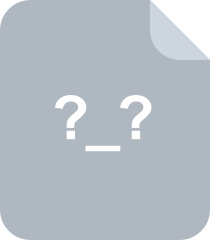
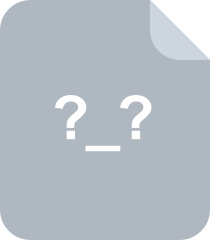

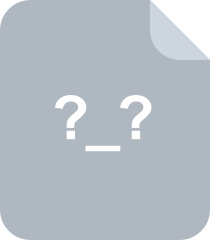







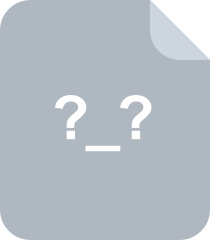


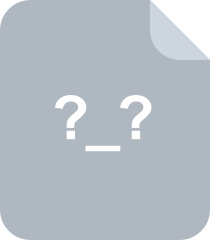






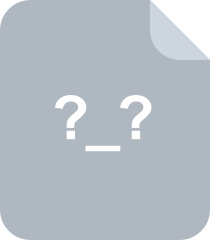
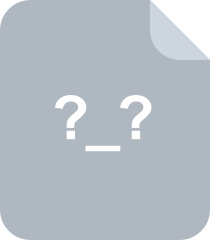
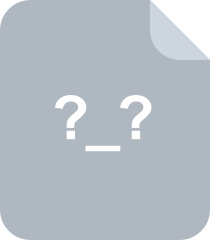
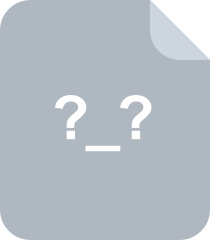

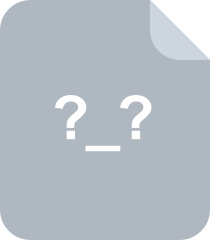








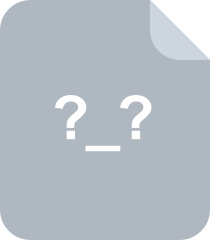
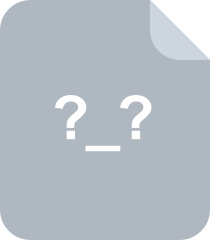
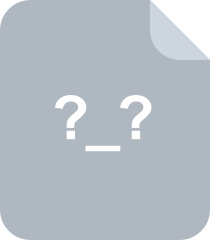


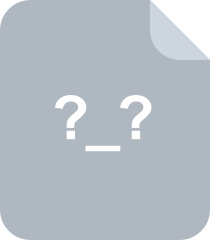






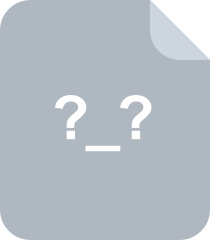
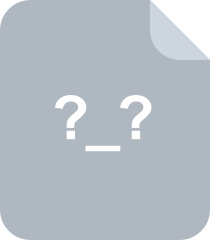
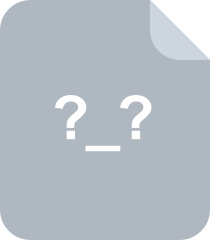
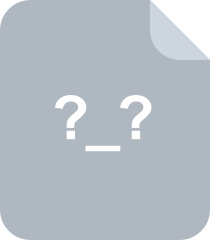
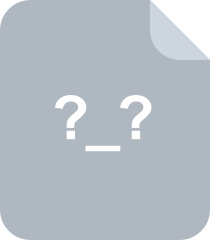

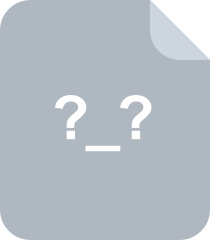







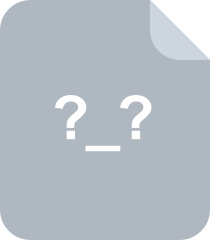


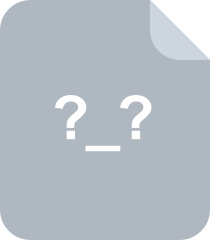





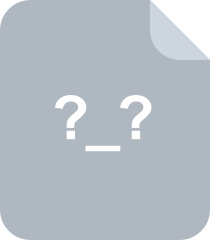
共 54 条
- 1
资源评论
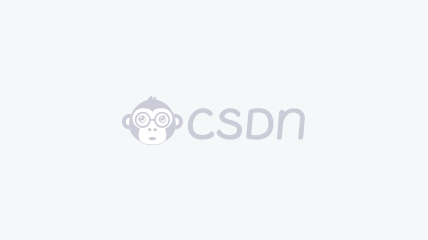

泡芙萝莉酱
- 粉丝: 2402
- 资源: 959
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

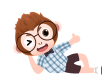
最新资源
- 基于c语言的线性链表的实现和应用
- 艾利和iriver Astell&Kern SP3000 V1.20升级固件
- 律师事务所网站建设与管理功能概述
- Python 端口访问邮件提醒工具
- 基于springboot的抗疫物资管理系统
- 基于C语言的二叉树构建及遍历
- 587833617736230KEY_C0091 STM32简易交通灯仿真设计.zip
- 垃圾废物检测19-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- 专项资金申报平台需求规范文档解析及关键技术要求
- TMS320F28377原理图
- Docker以及Docker-Compose的安装与卸载
- 艾利和iriver Astell&Kern SP3000 V1.31升级固件
- 基于C语言的图的实现和遍历
- 周勤富恒升职业学校网络安全渗透测试及解决方案第2版.doc
- images(5).zip
- 计算机程序设计员三级(选择题)
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


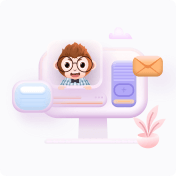
安全验证
文档复制为VIP权益,开通VIP直接复制
