Delphi7与C#语法的比较

### Delphi7与C#语法的比较 #### 一、概述 本文旨在详细对比Delphi7与C#的语法特点,以帮助Delphi开发者更好地理解并过渡到C#开发环境。Delphi是一款由Borland公司开发的快速应用开发工具,而C#则是微软为.NET框架设计的一种面向对象的编程语言。 #### 二、注释(Comments) 在Delphi中,支持单行注释`//`以及多行注释`{...}`或`(*...*)`。 ```delphi // 这是一条单行注释 { 这是多行注释 } (* 这也是多行注释 *) ``` 而在C#中,单行注释同样使用`//`,多行注释则使用`/*...*/`。 ```csharp // 这是一条单行注释 /* 这是多行注释 */ ``` 此外,C#还支持XML文档注释,这些注释可以被编译器用来生成API文档。 ```csharp /// <summary> /// 这是一个XML文档注释 /// </summary> ``` #### 三、数据类型(Data Types) - **简单类型(Value Types)** - Delphi中包含`Boolean`、`Byte`、`Char`等基本类型。 - C#同样提供了类似的简单类型,如`bool`、`byte`、`char`等。 - Delphi中的`Integer`类型相当于C#中的`int`。 - `Real`类型在Delphi中对应C#中的`float`或`double`,取决于精度需求。 - Delphi中特有类型`TDate`和`TDateTime`用于处理日期时间,在C#中可以通过`DateTime`来实现相同功能。 - **引用类型(Reference Types)** - Delphi中的引用类型包括`Object`、`String`等。 - C#中的引用类型主要为`object`和`string`。 - Delphi中的`String`类型有多种形式,如`ShortString`、`AnsiString`、`WideString`,而C#中统一使用`string`。 #### 四、常量(Constants) - Delphi中定义常量的方式: ```delphi const MyConst = 10; ``` - C#中定义常量的方法: ```csharp const int MyConst = 10; ``` #### 五、枚举(Enumerations) - Delphi中定义枚举: ```delphi type TColor = (Red, Green, Blue); ``` - C#中定义枚举: ```csharp enum Color { Red, Green, Blue }; ``` #### 六、运算符(Operators) - Delphi和C#都支持大多数常见的算术、比较和逻辑运算符。 - 例如,加法`+`、减法`-`、乘法`*`等在两种语言中都是相同的。 - 对于赋值操作符,Delphi使用`:=`,而C#使用`=`。 #### 七、选择语句(Choices) - Delphi中使用`if`语句进行条件判断: ```delphi if x > 0 then ShowMessage('Positive'); ``` - C#中的`if`语句: ```csharp if (x > 0) Console.WriteLine("Positive"); ``` #### 八、循环语句(Loops) - Delphi中使用`for`循环: ```delphi for i := 1 to 10 do ShowMessage(IntToStr(i)); ``` - C#中的`for`循环: ```csharp for (int i = 1; i <= 10; i++) Console.WriteLine(i.ToString()); ``` #### 九、数组(Arrays) - Delphi中定义数组: ```delphi var A: array [1..10] of Integer; ``` - C#中定义数组: ```csharp int[] A = new int[10]; ``` #### 十、函数(Functions) - Delphi中定义函数: ```delphi function Square(x: Integer): Integer; begin Result := x * x; end; ``` - C#中定义函数: ```csharp int Square(int x) { return x * x; } ``` #### 十一、异常处理(Exception Handling) - Delphi中使用`try...except`块: ```delphi try // 可能抛出异常的代码 except on E: Exception do ShowMessage(E.Message); end; ``` - C#中使用`try...catch`块: ```csharp try { // Code that may throw an exception } catch (Exception e) { Console.WriteLine(e.Message); } ``` #### 十二、命名空间(Namespace) - Delphi中通常没有命名空间的概念,而是通过单元(unit)来组织代码。 - C#中使用命名空间来避免名称冲突: ```csharp namespace MyNamespace { class MyClass { // ... } } ``` #### 十三、类与接口(Class/Interface) - Delphi和C#都支持面向对象编程(OOP),包括类(class)和接口(interface)。 - Delphi中定义一个简单的类: ```delphi type TMyClass = class private FValue: Integer; public procedure SetValue(AValue: Integer); end; procedure TMyClass.SetValue(AValue: Integer); begin FValue := AValue; end; ``` - C#中定义一个简单的类: ```csharp class MyClass { private int _value; public void SetValue(int value) { _value = value; } } ``` #### 十四、构造与析构(Constructors/Destructors) - Delphi中构造器和析构器分别称为构造方法(constructor)和销毁方法(destructor)。 - C#中使用`class`关键字定义构造函数,无需显式定义析构函数(unless using unmanaged resources)。 - Delphi中构造方法: ```delphi constructor TMyClass.Create; begin inherited Create; FValue := 0; end; ``` - C#中构造函数: ```csharp class MyClass { public MyClass() { _value = 0; } } ``` #### 十五、对象(Object) - 在Delphi中创建对象实例: ```delphi var Obj: TMyClass; begin Obj := TMyClass.Create; end; ``` - 在C#中创建对象实例: ```csharp MyClass obj = new MyClass(); ``` #### 十六、结构体(Structs) - Delphi和C#都支持结构体(struct),用于存储一组相关联的数据。 - Delphi中定义结构体: ```delphi type TPoint = record X, Y: Integer; end; ``` - C#中定义结构体: ```csharp struct Point { public int X; public int Y; } ``` #### 十七、属性(Properties) - Delphi中定义属性: ```delphi type TMyClass = class private FValue: Integer; public property Value: Integer read FValue write FValue; end; ``` - C#中定义属性: ```csharp class MyClass { private int _value; public int Value { get { return _value; } set { _value = value; } } } ``` #### 十八、委托与事件(Delegates/Events) - Delphi中使用`TNotifyEvent`等预定义类型的委托。 - C#中定义委托和事件: ```csharp delegate void MyDelegate(); class MyClass { public event MyDelegate MyEvent; } ``` #### 十九、控制台输入输出(Console I/O) - Delphi中使用`ReadLn`和`WriteLn`进行控制台输入输出。 - C#中使用`Console.ReadLine()`和`Console.WriteLine()`进行控制台输入输出。 #### 二十、文件输入输出(File I/O) - Delphi中使用`TFileStream`等类进行文件操作。 - C#中使用`StreamReader`和`StreamWriter`等类进行文件操作。 以上是Delphi7与C#语法的主要差异和相似之处的总结,对于希望从Delphi过渡到C#的开发者来说,掌握这些差异将有助于更平滑地完成技术迁移。
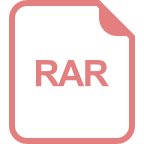
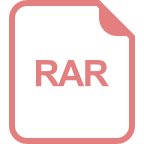
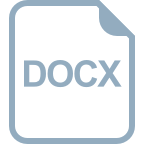
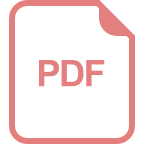
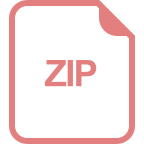
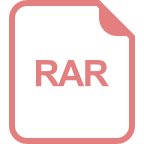
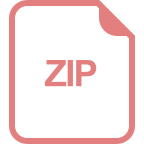
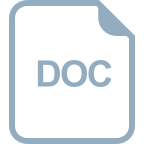
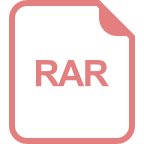
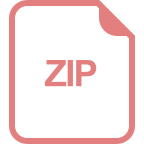
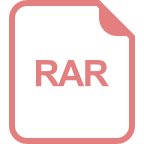
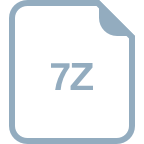
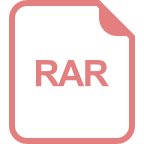
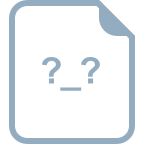
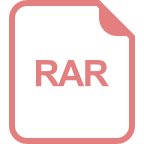
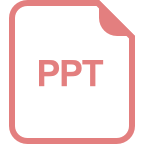
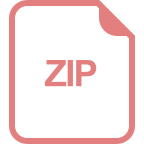
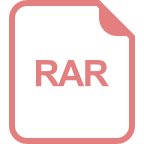
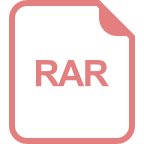
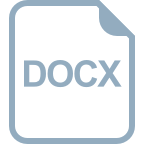
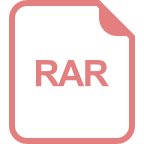
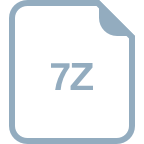
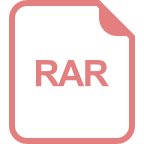
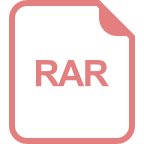
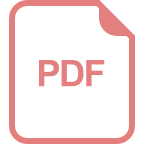
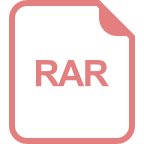
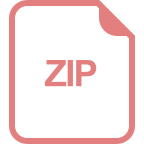
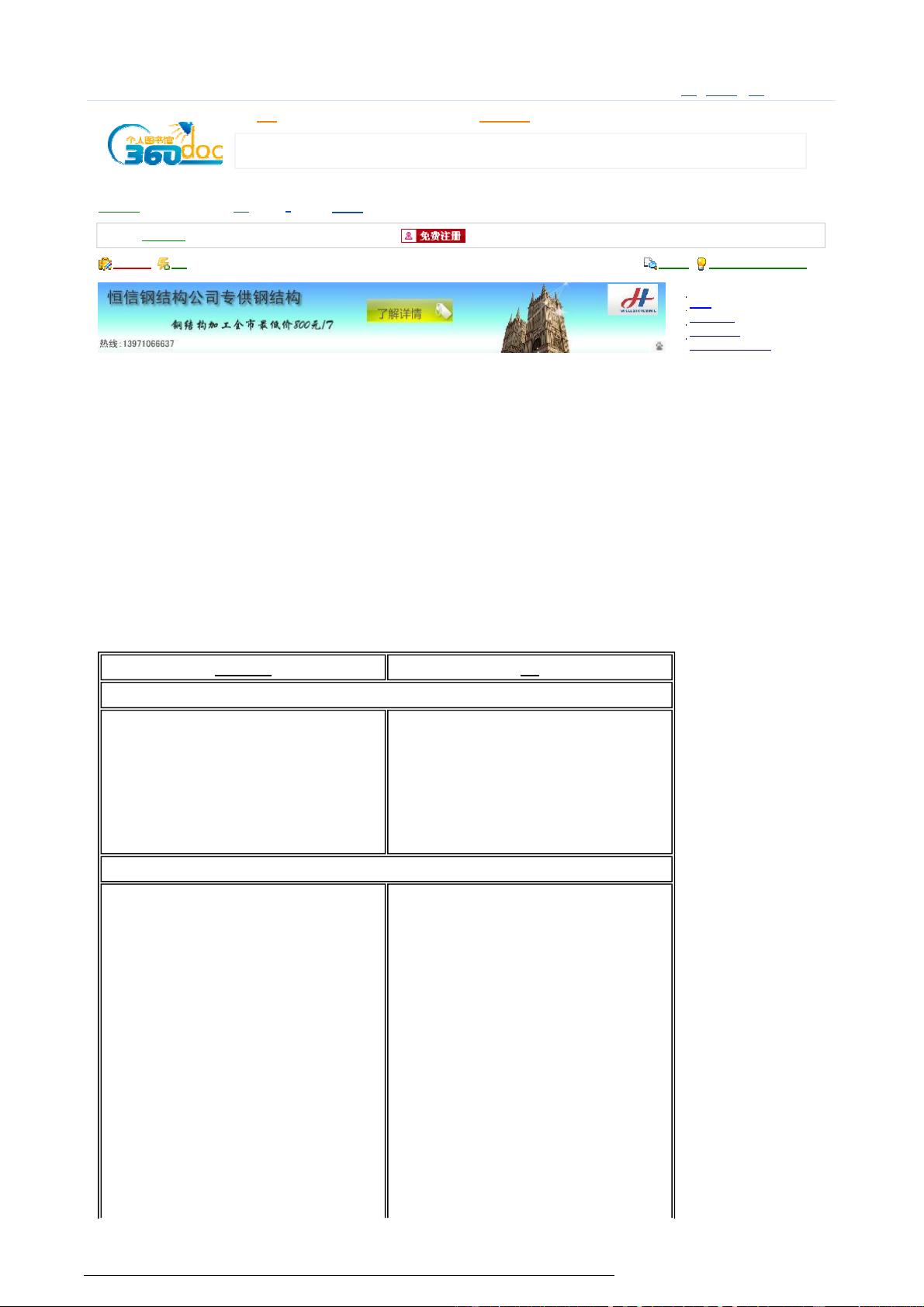
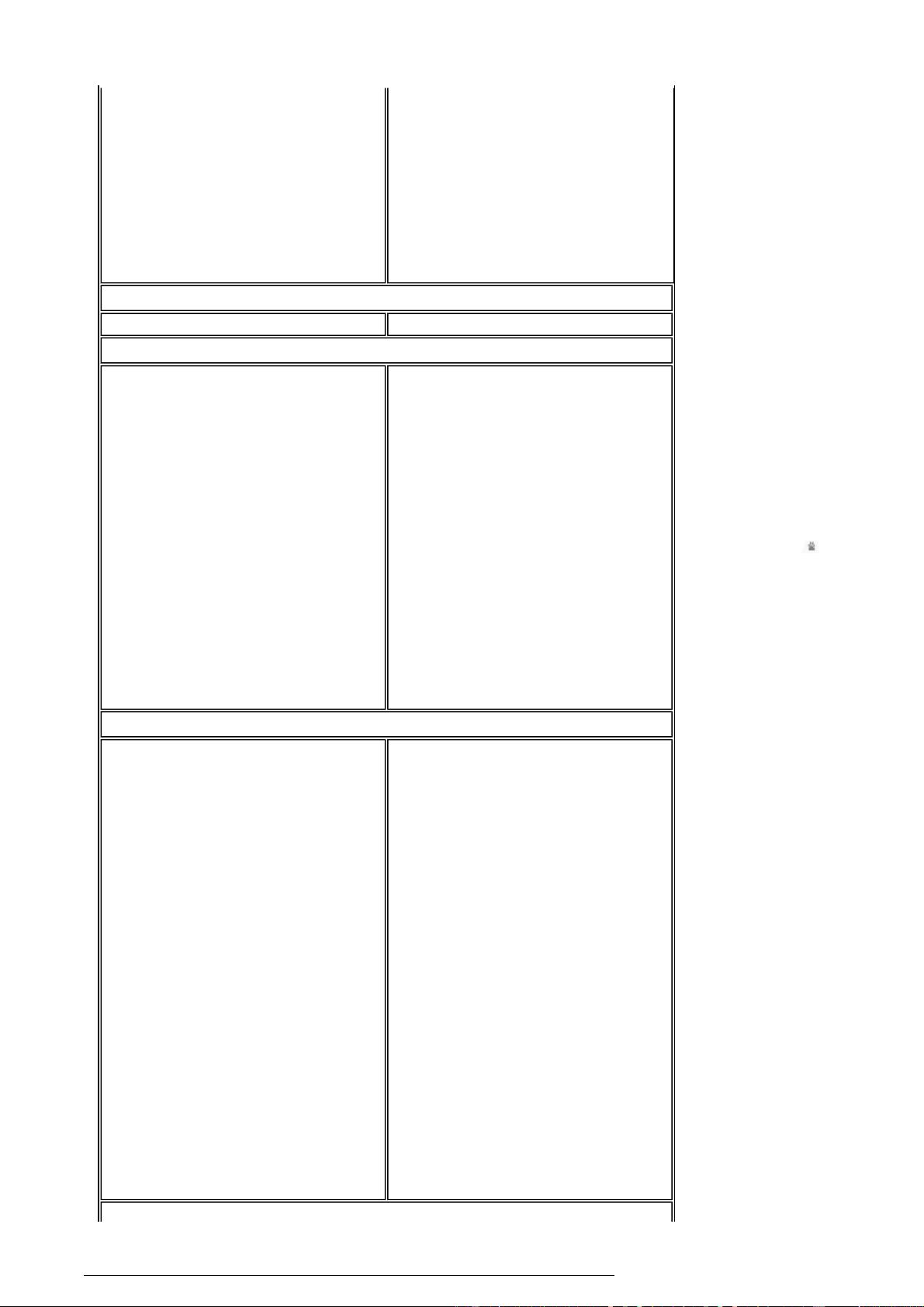
剩余9页未读,继续阅读
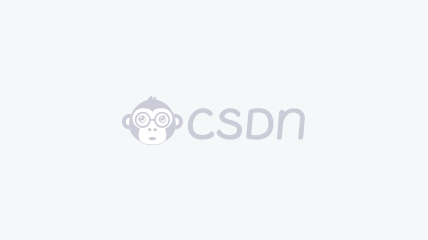
- nick22152013-10-12内容一般 ,没有什么参考价值。

- 粉丝: 0
- 资源: 5
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

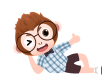
最新资源

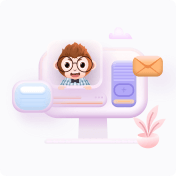
