#bxSlider 4.1.1
##The fully-loaded, responsive jQuery content slider
###Why should I use this slider?
* Fully responsive - will adapt to any device
* Horizontal, vertical, and fade modes
* Slides can contain images, video, or HTML content
* Full callback API and public methods
* Small file size, fully themed, simple to implement
* Browser support: Firefox, Chrome, Safari, iOS, Android, IE7+
* Tons of configuration options
For complete documentation, tons of examples, and a good time, visit:
[http://bxslider.com](http://bxslider.com)
Written by: Steven Wanderski - [http://stevenwanderski.com](http://stevenwanderski.com)
###License
Released under the MIT license - http://opensource.org/licenses/MIT
Let's get on with it!
##Installation
###Step 1: Link required files
First and most important, the jQuery library needs to be included (no need to download - link directly from Google). Next, download the package from this site and link the bxSlider CSS file (for the theme) and the bxSlider Javascript file.
```html
<!-- jQuery library (served from Google) -->
<script src="//ajax.googleapis.com/ajax/libs/jquery/1.8.2/jquery.min.js"></script>
<!-- bxSlider Javascript file -->
<script src="/js/jquery.bxslider.min.js"></script>
<!-- bxSlider CSS file -->
<link href="/lib/jquery.bxslider.css" rel="stylesheet" />
```
###Step 2: Create HTML markup
Create a `<ul class="bxslider">` element, with a `<li>` for each slide. Slides can contain images, video, or any other HTML content!
```html
<ul class="bxslider">
<li><img src="/images/pic1.jpg" /></li>
<li><img src="/images/pic2.jpg" /></li>
<li><img src="/images/pic3.jpg" /></li>
<li><img src="/images/pic4.jpg" /></li>
</ul>
```
###Step 3: Call the bxSlider
Call .bxslider() on `<ul class="bxslider">`. Note that the call must be made inside of a $(document).ready() call, or the plugin will not work!
```javascript
$(document).ready(function(){
$('.bxslider').bxSlider();
});
```
##Configuration options
###General
**mode**
Type of transition between slides
```
default: 'horizontal'
options: 'horizontal', 'vertical', 'fade'
```
**speed**
Slide transition duration (in ms)
```
default: 500
options: integer
```
**slideMargin**
Margin between each slide
```
default: 0
options: integer
```
**startSlide**
Starting slide index (zero-based)
```
default: 0
options: integer
```
**randomStart**
Start slider on a random slide
```
default: false
options: boolean (true / false)
```
**slideSelector**
Element to use as slides (ex. <code>'div.slide'</code>).<br />Note: by default, bxSlider will use all immediate children of the slider element
```
default: ''
options: jQuery selector
```
**infiniteLoop**
If <code>true</code>, clicking "Next" while on the last slide will transition to the first slide and vice-versa
```
default: true
options: boolean (true / false)
```
**hideControlOnEnd**
If <code>true</code>, "Prev" and "Next" controls will receive a class <code>disabled</code> when slide is the first or the last<br/>Note: Only used when <code>infiniteLoop: false</code>
```
default: false
options: boolean (true / false)
```
**easing**
The type of "easing" to use during transitions. If using CSS transitions, include a value for the <code>transition-timing-function</code> property. If not using CSS transitions, you may include <code>plugins/jquery.easing.1.3.js</code> for many options.<br />See <a href="http://gsgd.co.uk/sandbox/jquery/easing/" target="_blank">http://gsgd.co.uk/sandbox/jquery/easing/</a> for more info.
```
default: null
options: if using CSS: 'linear', 'ease', 'ease-in', 'ease-out', 'ease-in-out', 'cubic-bezier(n,n,n,n)'. If not using CSS: 'swing', 'linear' (see the above file for more options)
```
**captions**
Include image captions. Captions are derived from the image's <code>title</code> attribute
```
default: false
options: boolean (true / false)
```
**ticker**
Use slider in ticker mode (similar to a news ticker)
```
default: false
options: boolean (true / false)
```
**tickerHover**
Ticker will pause when mouse hovers over slider. Note: this functionality does NOT work if using CSS transitions!
```
default: false
options: boolean (true / false)
```
**adaptiveHeight**
Dynamically adjust slider height based on each slide's height
```
default: false
options: boolean (true / false)
```
**adaptiveHeightSpeed**
Slide height transition duration (in ms). Note: only used if <code>adaptiveHeight: true</code>
```
default: 500
options: integer
```
**video**
If any slides contain video, set this to <code>true</code>. Also, include <code>plugins/jquery.fitvids.js</code><br />See <a href="http://fitvidsjs.com/" target="_blank">http://fitvidsjs.com/</a> for more info
```
default: false
options: boolean (true / false)
```
**responsive**
Enable or disable auto resize of the slider. Useful if you need to use fixed width sliders.
```
default: true
options: boolean (true /false)
```
**useCSS**
If true, CSS transitions will be used for horizontal and vertical slide animations (this uses native hardware acceleration). If false, jQuery animate() will be used.
```
default: true
options: boolean (true / false)
```
**preloadImages**
If 'all', preloads all images before starting the slider. If 'visible', preloads only images in the initially visible slides before starting the slider (tip: use 'visible' if all slides are identical dimensions)
```
default: 'visible'
options: 'all', 'visible'
```
**touchEnabled**
If <code>true</code>, slider will allow touch swipe transitions
```
default: true
options: boolean (true / false)
```
**swipeThreshold**
Amount of pixels a touch swipe needs to exceed in order to execute a slide transition. Note: only used if <code>touchEnabled: true</code>
```
default: 50
options: integer
```
**oneToOneTouch**
If <code>true</code>, non-fade slides follow the finger as it swipes
```
default: true
options: boolean (true / false)
```
**preventDefaultSwipeX**
If <code>true</code>, touch screen will not move along the x-axis as the finger swipes
```
default: true
options: boolean (true / false)
```
**preventDefaultSwipeY**
If <code>true</code>, touch screen will not move along the y-axis as the finger swipes
```
default: false
options: boolean (true / false)
```
###Pager
**pager**
If <code>true</code>, a pager will be added
```
default: true
options: boolean (true / false)
```
**pagerType**
If <code>'full'</code>, a pager link will be generated for each slide. If <code>'short'</code>, a x / y pager will be used (ex. 1 / 5)
```
default: 'full'
options: 'full', 'short'
```
**pagerShortSeparator**
If <code>pagerType: 'short'</code>, pager will use this value as the separating character
```
default: ' / '
options: string
```
**pagerSelector**
Element used to populate the populate the pager. By default, the pager is appended to the bx-viewport
```
default: ''
options: jQuery selector
```
**pagerCustom**
Parent element to be used as the pager. Parent element must contain a <code><a data-slide-index="x"></code> element for each slide. See example <a href="/examples/thumbnail-method-1">here</a>. Not for use with dynamic carousels.
```
default: null
options: jQuery selector
```
**buildPager**
If supplied, function is called on every slide element, and the returned value is used as the pager item markup.<br />See <a href="http://bxslider.com/examples">examples</a> for detailed implementation
```
default: null
options: function(slideIndex)
```
###Controls
**controls**
If <code>true</code>, "Next" / "Prev" controls will be added
```
default: true
options: boolean (true / false)
```
**nextText**
Text to be used for the "Next" control
```
default: 'Next'
options: string
```
**prevText**
Text to be used for the "Prev" control
```
default: 'Prev'
options: string
```
**nextSelector**
Element used to populate the "Next" control
```
default: null
options: jQuery selector
```
**prevSelector**
Element used to populate the "Prev" control
```
default: null
options: jQuery selector
```

柯晓楠
- 粉丝: 2w+
- 资源: 2875
最新资源
- GA-RBF回归预测,基于遗传算法(GA)优化径向基神经网络(RBF)的数据回归预测,多变量输入单输出 优化参数为扩散速度,采用交叉验证防止过拟合 程序已经调试好,无需更改代码替数据集即可运行数据格式
- 三相VIENNA整流器仿真(全网独一份) matlab仿真 T型vienna整流器仿真 双闭环PI控制,中点电位平衡控制,SPWM调制,三相锁相环 图3为三相电流波形,图4THD为1.01%,电感仅
- 半导体PCB冲裁与上料sw18全套技术资料100%好用.zip
- comsol激光双温模型 金属 半导体 【脉冲激光移动烧蚀材料仿真】 1、脉冲激光移动烧蚀材料仿真 2、采用COMSOL固体传热等物理场进行多物理场耦合仿真 3、对皮秒激光烧蚀后的材料进行后处理分析
- 不锈钢填充粉设备stp全套技术资料100%好用.zip
- 城市小型无负压二次供水设备sw17全套技术资料100%好用.zip
- 汽车二、三自由度模型 汽车二、三自由度模型 本人用了三种不同方法搭的汽车线性二自由度simulink模型,文档里包含有具体的车辆数值,还关于汽车simulink仿真实例 适合初学者学习simulin
- csdn_v6.5.5-1.apk
- 薄膜滚胶设备sw20可编辑全套技术资料100%好用.zip
- 自编地震动反应谱matlab计算程序,有使用方法视频 程序具有以下特点: 1.完全来源,参数可以个性化设置 2.可以批量处理大量反应谱
- 光伏储能离网系统simulink仿真 1光照在0.2s时候从1000变成200 光照1000时光伏给蓄电池和负载供电 光照200时,光伏和蓄电池同时给负载供电 2负载电阻的阻值可以修改,系统仍
- CLLC双向谐振变器仿真 输出电压闭环控制 采用CLLC对称结构,正反两个方向的运行对称 模型可以实现自动的正反向运行 如效果图2所示: 在0.2s处,电路由正向传输改为反向传输 有plec
- 手机电池连接器产品设计规范
- 从无序到优雅:为什么无数个点的迭代最终变成椭圆?一分钟带你玩转MATLAB乐趣代码平面中无数个点的首位依次连接,取中点之后再收尾依次连接,反复进行此操作最终无数个点收敛成为椭圆形状
- 三菱电梯全新系统N5平台参数地址LEHY-Pro,比较全
- C#开发MES系统程序源码 c#winform MES管理系统源码1.该系统用C#.net开发,与7台西门子plc以太网通讯,生产数据收集,设备状态显示,生产管理等
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


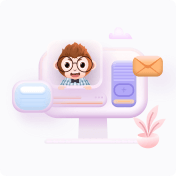