HttpClient入门

HttpClient 是apache 组织下面的一个用于处理HTTP 请求和响应的开源工具。它不是一个浏览器,也不处 理客户端缓存等浏览器的功能。它只是一个类库!它在JDK 的基本类库基础上做了更好的封装! HttpClient 目前(写作本文日期:2010 年8 月)最新版本是4.0.1,官方网址: http://hc.apache.org/httpcomponents-client-4.0.1/index.html HttpClient 项目依赖于HttpCore(处理核心的HTTP 协议)、commons-codec(处理与编码有关的问题的项 目)和commons-logging(处理与日志记录有关问题的项目)。 如果你希望能够通过HttpClient 向服务器上传文件等与multipart 编码类型有关的请求,以及其它复杂的 MIME 类型,那么,你需要另外一个依赖包:HttpMime(它是专门处理与MIME 类型有关问题的项目),在 下载的HttpClient 包中(下载地址为:http://hc.apache.org/downloads.cgi)已经包含了HttpMime。 ### HttpClient入门知识点详解 #### 一、HttpClient概述 **HttpClient** 是 Apache 组织下的一个开源工具库,主要用于处理 HTTP 请求和响应。它不同于浏览器,不处理客户端缓存等功能,而是一个专注于网络通信的类库。它在 JDK 基础类库的基础上进行了更好的封装,使得开发人员能够更便捷地进行 HTTP 通信操作。 - **版本**: 截至 2010 年 8 月,HttpClient 最新版本为 4.0.1。 - **官方网站**: [http://hc.apache.org/httpcomponents-client-4.0.1/index.html](http://hc.apache.org/httpcomponents-client-4.0.1/index.html) - **依赖库**: - **HttpCore**: 处理核心的 HTTP 协议。 - **commons-codec**: 处理与编码相关的问题。 - **commons-logging**: 处理与日志记录相关的问题。 - **HttpMime**: 专门处理与 MIME 类型相关的问题,如 multipart 编码类型的请求。 #### 二、了解JDK中有关HTTP URL处理的API ##### 2.1 最简单的获取网页内容的示例 在使用 HttpClient 之前,了解 Java 标准库中的相关 API 非常重要,这有助于理解 HttpClient 的封装逻辑。 ```java try { String urlString = "http://localhost:8080/cms/"; URL url = new URL(urlString); // 创建 URL 对象 InputStream is = url.openStream(); // 打开连接并获取输入流 BufferedReader br = new BufferedReader(new InputStreamReader(is, "UTF-8")); String line; while ((line = br.readLine()) != null) { System.out.println(line); } is.close(); } catch (Exception e) { e.printStackTrace(); } ``` 此示例展示了如何使用 `java.net.URL` 和 `java.net.URLConnection` 类来发送 HTTP GET 请求并读取响应体。这是 Java 标准库中最基本的 HTTP 操作方法之一。 ##### 2.2 `URLConnection` 的基本用法 `URLConnection` 是 `URL` 类的一个抽象子类,它提供了打开到指定 URL 的连接的方法。`URLConnection` 类的主要功能包括: - 设置请求属性(例如 User-Agent 或 Accept-Language)。 - 获取响应头信息。 - 控制连接超时。 - 获取输入/输出流。 ```java URL url = new URL("http://example.com"); URLConnection connection = url.openConnection(); connection.setConnectTimeout(5000); // 设置连接超时时间 connection.setReadTimeout(5000); // 设置读取超时时间 ``` ##### 2.3 `HttpURLConnection` 的用法 `HttpURLConnection` 是 `URLConnection` 的一个具体实现,它专门用来处理 HTTP 协议的请求和响应。 ```java URL url = new URL("http://example.com"); HttpURLConnection connection = (HttpURLConnection) url.openConnection(); connection.setRequestMethod("GET"); // 设置请求方法 connection.setRequestProperty("User-Agent", "Mozilla/5.0"); // 设置 User-Agent connection.setDoInput(true); // 允许输入流 connection.setDoOutput(false); // 不允许输出流 int responseCode = connection.getResponseCode(); // 获取响应码 ``` #### 三、使用 HttpClient 获取网页内容 ##### 3.1 使用 GET 方式向后台递交请求 ```java CloseableHttpClient httpClient = HttpClients.createDefault(); HttpGet httpGet = new HttpGet("http://example.com"); CloseableHttpResponse response = httpClient.execute(httpGet); try { System.out.println(response.getStatusLine()); HttpEntity entity = response.getEntity(); if (entity != null) { System.out.println(EntityUtils.toString(entity)); } } finally { response.close(); } ``` 上述代码演示了如何使用 HttpClient 发送一个 GET 请求并打印出响应的状态行和响应体。 ##### 3.2 自动获得响应的编码信息 HttpClient 能够自动解析响应头中的字符集编码信息,并正确地解码响应体。 ```java CloseableHttpResponse response = httpClient.execute(httpGet); try { HttpEntity entity = response.getEntity(); if (entity != null) { String content = EntityUtils.toString(entity); System.out.println(content); } } finally { response.close(); } ``` ##### 3.3 设置代理服务器,访问网站 当需要通过代理服务器访问目标网站时,可以使用以下方法: ```java Proxy proxy = new Proxy(Proxy.Type.HTTP, new InetSocketAddress("proxy.example.com", 8080)); HttpGet httpGet = new HttpGet("http://example.com"); httpGet.setConfig(RequestConfig.custom().setProxy(proxy).build()); CloseableHttpResponse response = httpClient.execute(httpGet); ``` ##### 3.4 获得重定向之后的网址信息 HttpClient 可以自动处理重定向,并返回最终的 URL 地址。 ```java HttpGet httpGet = new HttpGet("http://example.com"); httpGet.addHeader("User-Agent", "Mozilla/5.0"); CloseableHttpResponse response = httpClient.execute(httpGet); try { System.out.println(response.getStatusLine()); HttpEntity entity = response.getEntity(); if (entity != null) { System.out.println(EntityUtils.toString(entity)); } Header location = response.getFirstHeader("Location"); if (location != null) { System.out.println("Redirected to: " + location.getValue()); } } finally { response.close(); } ``` ##### 3.5 自动 Cookie 处理 HttpClient 支持自动管理和处理 Cookie。 ```java HttpClientBuilder httpClientBuilder = HttpClients.custom(); httpClientBuilder.setDefaultCookieStore(new BasicCookieStore()); CloseableHttpClient httpClient = httpClientBuilder.build(); HttpGet httpGet = new HttpGet("http://example.com"); CloseableHttpResponse response = httpClient.execute(httpGet); try { System.out.println(response.getStatusLine()); HttpEntity entity = response.getEntity(); if (entity != null) { System.out.println(EntityUtils.toString(entity)); } } finally { response.close(); } ``` 通过以上示例可以看出,HttpClient 提供了丰富的功能来简化 HTTP 请求的处理过程。无论是基本的 GET 请求还是复杂的 POST 请求,甚至是处理重定向、代理服务器配置等问题,HttpClient 都能提供简便高效的解决方案。
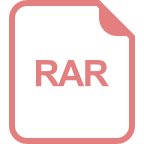
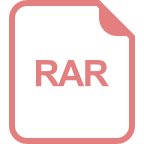
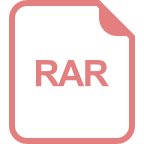
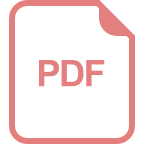
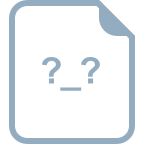
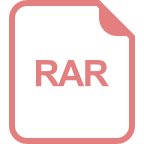
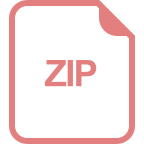
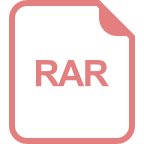
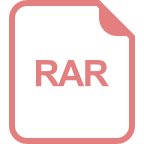
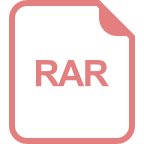
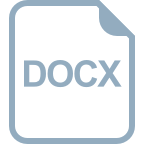
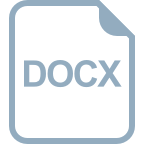
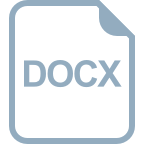
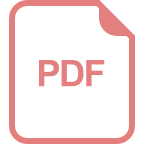
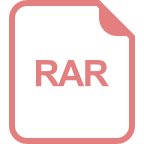
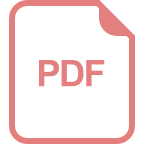
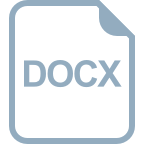
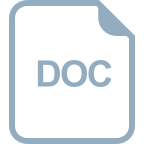
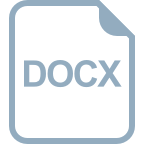
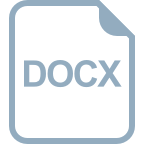


- 粉丝: 1
- 资源: 7
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

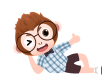
最新资源

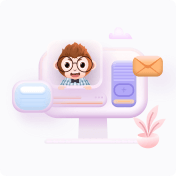

- 1
- 2
前往页