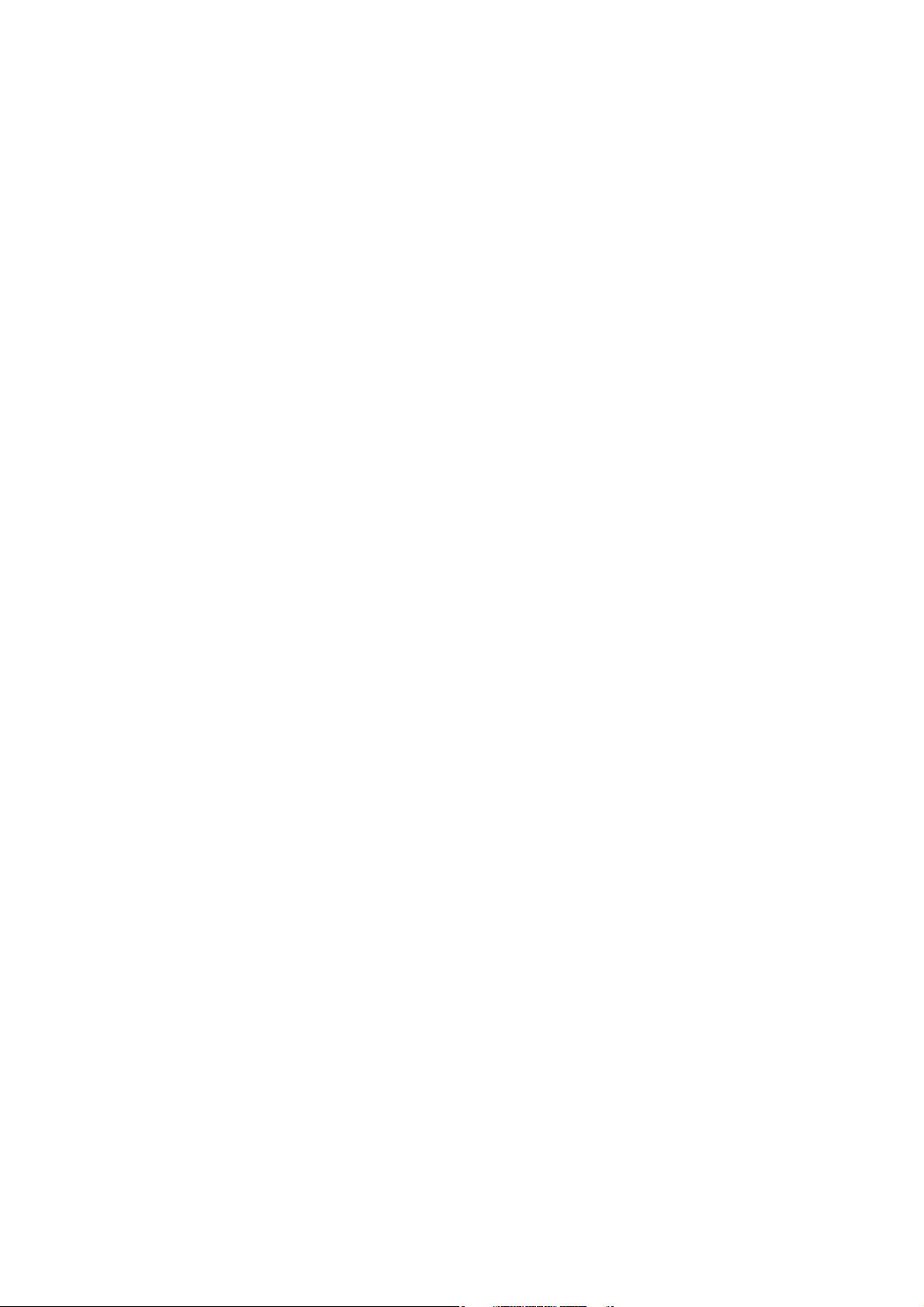
EFSL
Embedded Filesystem Library
Lennart Ysboodt - Michael De Nil
c
2005
1
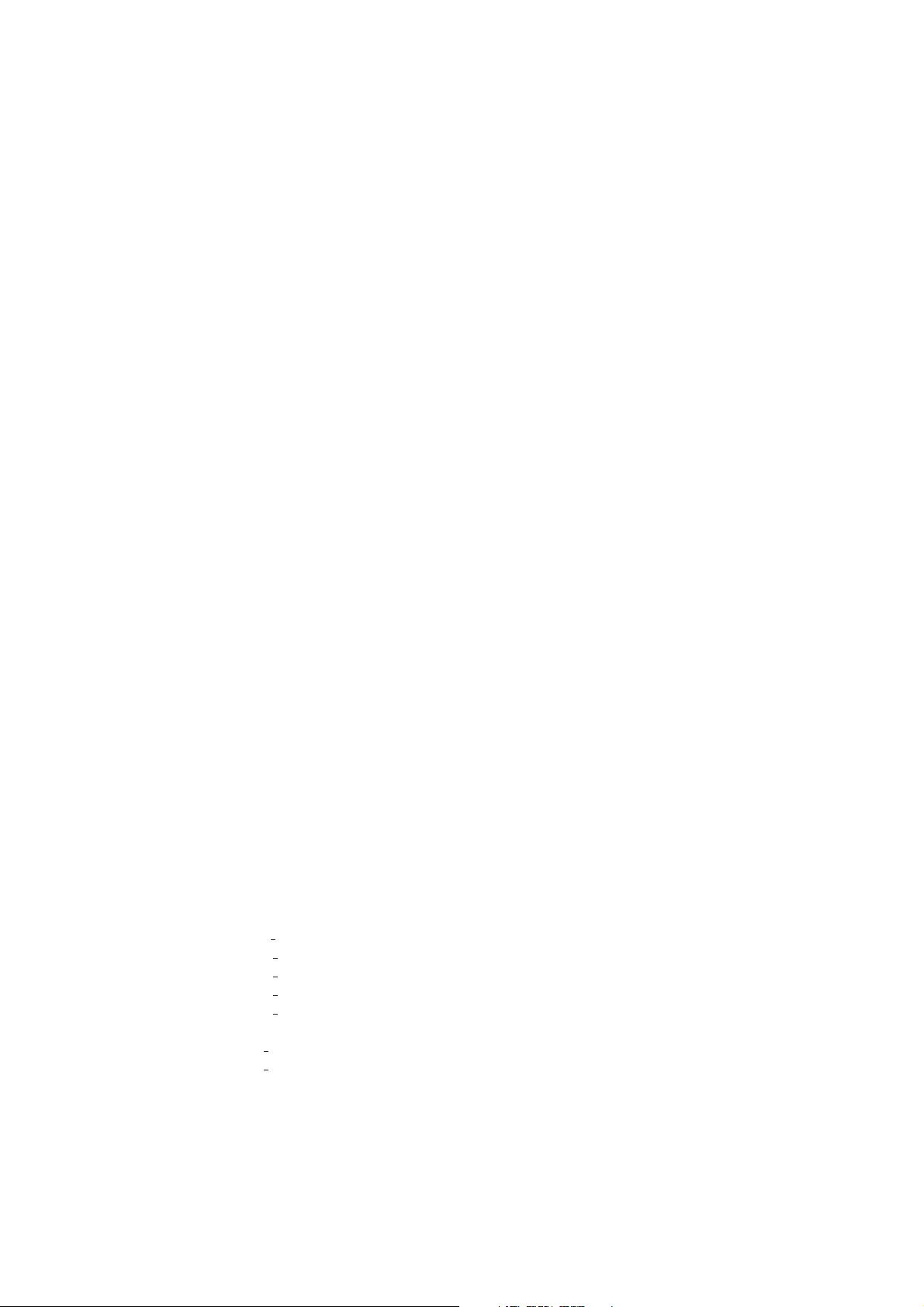
Contents
1 Preface 3
1.1 Project aims ............................. 3
1.2 Project status ............................. 3
1.3 License ................................. 3
1.4 Contact ................................ 3
2 Getting started 4
2.1 On Linux (file) ............................ 4
2.1.1 Download & Compile .................... 4
2.1.2 Example ............................ 4
2.1.3 Testing ............................ 6
2.2 On AVR (SD-Card) ......................... 7
2.2.1 Hardware ........................... 7
2.2.2 Download & Compile .................... 8
2.2.3 Example ............................ 8
2.2.4 Testing ............................ 11
2.3 On DSP (SD-Card) .......................... 12
2.3.1 Hardware ........................... 12
2.3.2 McBSP configuration .................... 13
2.4 On ARM7 (SD-Card) ........................ 14
2.4.1 License ............................ 14
2.4.2 General information about the ARM7 port ........ 14
2.4.3 Example code ......................... 14
2.4.4 Test Hard- and Software ................... 15
2.4.5 Credits ............................ 15
3 Configuring EFSL 16
3.1 Hardware target ........................... 16
3.2 Memory configuration ........................ 16
3.3 Cache configuration ......................... 17
3.4 Pre-allocation ............................. 18
3.5 Endianness .............................. 19
3.6 Date and time ............................ 19
3.7 Errors ................................. 19
3.8 Debug ................................. 19
4 EFSL Functions 21
4.1 Date and time support ........................ 21
4.2 efs
init ................................. 22
4.3 file
fopen ............................... 23
4.4 file
fclose ............................... 25
4.5 file
read ................................ 27
4.6 file
write ................................ 29
4.7 mkdir ................................. 31
4.8 ls
openDir ............................... 33
4.9 ls
getNext ............................... 34
4.10 rmfile ................................. 36
4.11 Getting the free space ........................ 38
2
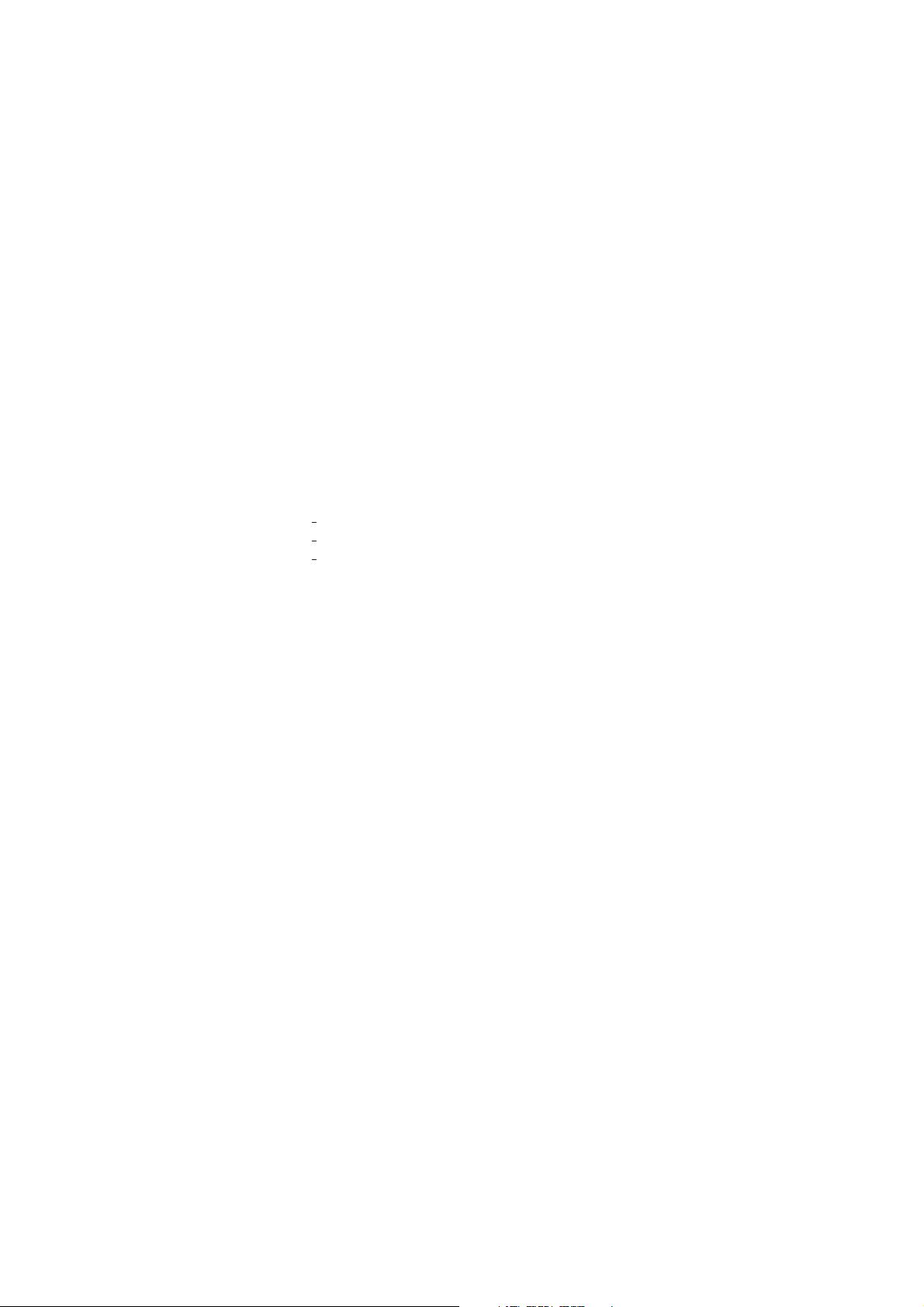
5 EFSL utilities 39
5.1 Notations ............................... 39
5.2 cpo................................... 39
5.3 cpi................................... 39
5.4 cpa................................... 40
5.5 list ................................... 40
5.6 mkdir ................................. 40
5.7 rmfile ................................. 41
6 Developer notes 42
6.1 Integer types ............................. 42
6.2 Debugging ............................... 42
6.2.1 Debugging on Linux ..................... 42
6.2.2 Debugging on AVR ...................... 43
6.2.3 Debugging on DSP ...................... 43
6.3 Adding support for a new endpoint ................. 43
6.3.1 hwInterface .......................... 45
6.3.2 if
initInterface ........................ 45
6.3.3 if
readBuf ........................... 46
6.3.4 if
writeBuf .......................... 46
6.4 I/O Manager ............................. 47
6.4.1 General operation ...................... 47
6.4.2 Cache decisions ........................ 47
6.4.3 Functions ........................... 48
6.5 C library for EFSL .......................... 51
7 Legal notes 52
7.1 GNU Lesser General Public License ................ 52
3
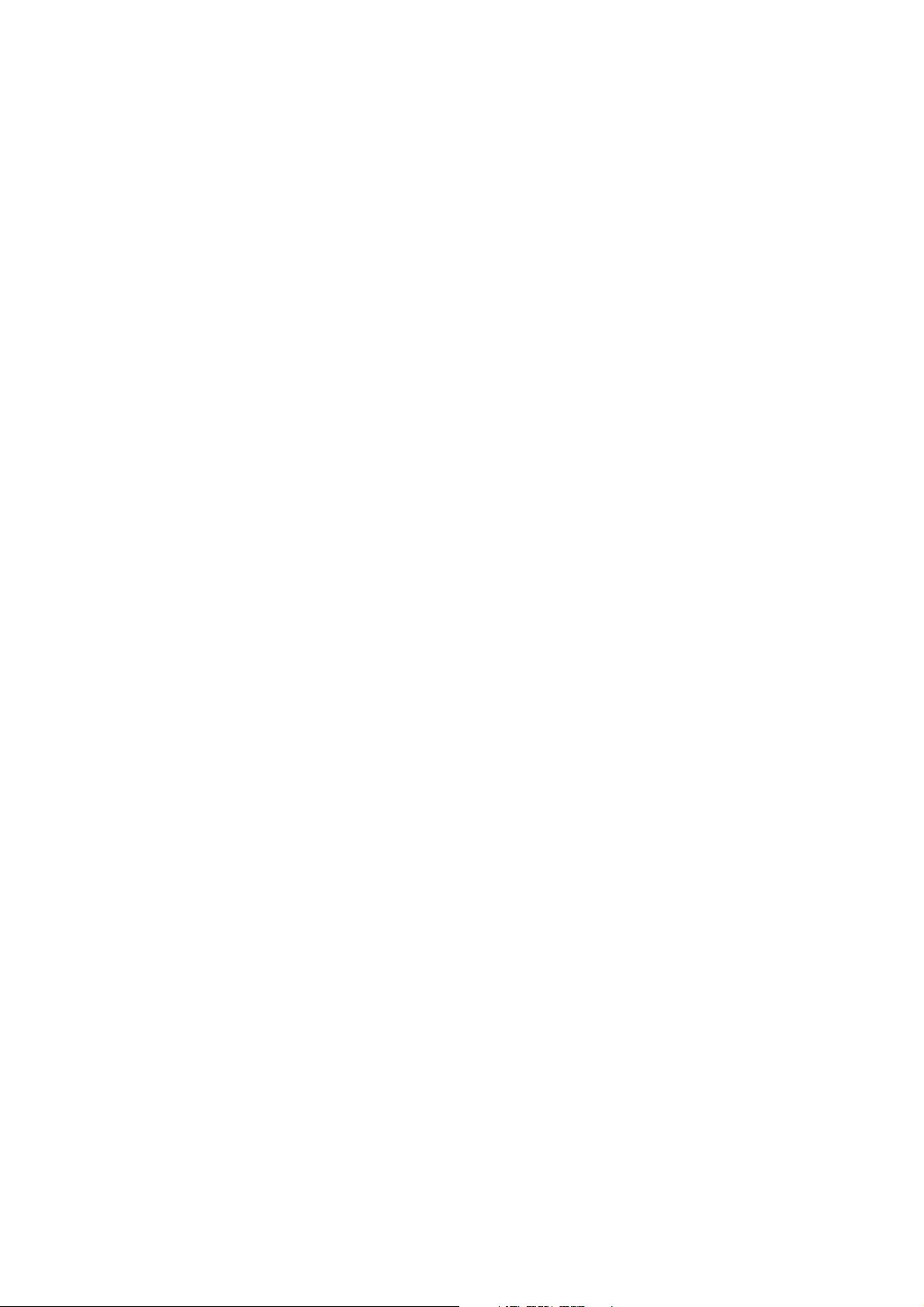
1 Preface
1.1 Project aims
The EFSL project aims to create a library for filesystems, to be used on various
embedded systems. Currently we support the Microsoft FAT filesystem family.
It is our intention to create pure ANSI C code that compiles on anything that
bears the name ’C compiler’. We don’t make assumptions about endianness or
how the memory alignment is arranged on your architecture.
Adding code for your specific hardware is straightforward, just add code that
fetches or writes a 512 byte sector, and the library will do the rest. Existing code
can be used, writing your own code is only required when you have hardware
for which no target exists.
1.2 Project status
Efsl currently supports FAT12, FAT16 and FAT32. Read and write has been
tested and is stable. Efsl runs on PC (GNU/Linux, development environment),
TMS C6000 DSP’s from Texas instruments, and ATMega’s from Atmel. You
can use this code with as little as 1 kilobyte RAM, however if you have more
at your disposal, an infinite amount can be used as cache memory. The more
memory you commit, the better the performance will be.
1.3 License
This project is released under the Lesser General Public license, which means
that you may use the library and it’s sourcecode for any purpose you want, that
you may link with it and use it commercially, but that ANY change to the code
must be released under the same license. We would appreciate if you would send
us a patch when you add support for new hardware, but this is not obligatory,
since it falls under linking as far as the LGPL is concerned.
1.4 Contact
You can reach us by email:
Michael De Nil michael@flex-it.be
Lennart Yseboodt len@belf.be
4
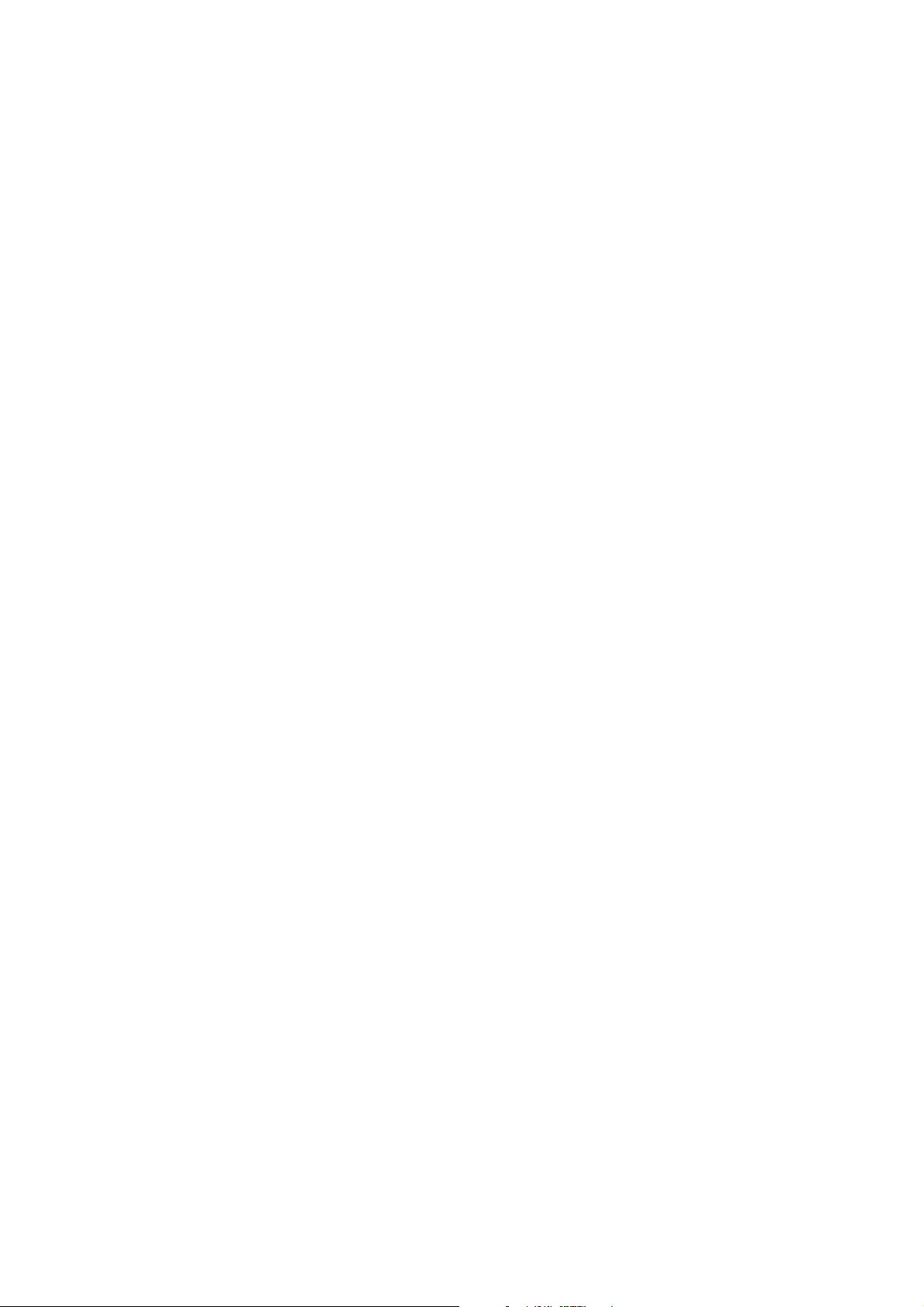
2 Getting started
2.1 On Linux (file)
Debugging efsl on embedded devices is a rather hard job, because you can’t just
printf debug strings or watch memory maps easily. Because of that, core devel-
opment has been performed under the Linux operating system. Under Linux,
efsl can be compiled as library and used as a userspace filesystem handler. On
Unix- style operating system (like Linux), all devices (usb stick, disc, . . . ) can
be seen as a file, and as such been opened by efsl.
In the following section, we will explain how to get started using efsl as userspace
filesystem handler. However, please note that the main focus for efsl is to sup-
port embedded systems, which usually don’t even have 1% of the memory you
have on a PC. Accessing files on a FAT-filesystem with efsl will be much slower
than when accessing these files with the Linux FAT kernel modules.
2.1.1 Download & Compile
Let’s get started:
1. Get the latest release of efsl on http://www.sf.net/projects/efsl/ and put
it in your homedir
2. Unpack the library (tar xvfj efsl-version.tar.bz2)
3. Get inside the directory (cd ∼/efsl)
4. Create a symlink from Makefile-LINUX to Makefile (ln -s Makefile-LINUX
Makefile)
5. Copy conf/config-sample-linux.h to conf/config.h (cp conf/config-sample-
linux.h conf/config.h)
6. Compile the library (make lib)
7. Find the compiled filesystem library (libefsl.a) in the current directory
If you got any errors with the steps above, please check that that you have the
following packages installed: tar, gcc, libgcc, binutils & make.
2.1.2 Example
Since efsl itself is only a library, it’s not supposed to do anything out of the box,
than just compile. To get started, we’ll show here a small example program
that opens a file on a disc/usb-stick/floppy that contains a FAT-filesystem and
prints it’s content to stdout.
First, create a new directory in which you put the compiled efsl-library ( libefsl.a
) and create a new file called linuxtest.c containing:
5