#include "palette.h"
#include <QHBoxLayout>
#include <QGridLayout>
Palette::Palette(QWidget *parent)
: QDialog(parent)
{
createCtrlFrame();
createContentFrame();
QHBoxLayout *mainLayout = new QHBoxLayout(this);
mainLayout->addWidget(ctrlFrame);
mainLayout->addWidget(contentFrame);
}
Palette::~Palette()
{
}
void Palette::createCtrlFrame()
{
ctrlFrame = new QFrame;
windowLabel = new QLabel(tr("QPalette::Window: "));
windowComboBox = new QComboBox;
fillColorList(windowComboBox); //向下拉列表中插入各种不同的颜色选项
connect(windowComboBox, SIGNAL(activated(int)), this, SLOT(ShowWindow())); //连接 改变背景色的槽函数
windowTextLabel = new QLabel(tr("QPalette::WindowText: "));
windowTextComboBox = new QComboBox;
fillColorList(windowTextComboBox);
connect(windowTextComboBox, SIGNAL(activated(int)), this, SLOT(ShowWindowText()));
buttonLabel = new QLabel(tr("QPalette::Button: "));
buttonComboBox = new QComboBox;
fillColorList(buttonComboBox);
connect(buttonComboBox, SIGNAL(activated(int)), this, SLOT(ShowButton()));
buttonTextLabel = new QLabel(tr("QPalette::ButtonText: "));
buttonTextComboBox = new QComboBox;
fillColorList(buttonTextComboBox);
connect(buttonTextComboBox, SIGNAL(activated(int)), this, SLOT(ShowButtonText()));
baseLabel = new QLabel(tr("QPalette::Base: "));
baseComboBox = new QComboBox;
fillColorList(baseComboBox);
connect(baseComboBox, SIGNAL(activated(int)), this, SLOT(ShowBase()));
QGridLayout *mainLayout = new QGridLayout(ctrlFrame);
mainLayout->setSpacing(20);
mainLayout->addWidget(windowLabel, 0 ,0);
mainLayout->addWidget(windowComboBox, 0 ,1);
mainLayout->addWidget(windowTextLabel, 1 ,0);
mainLayout->addWidget(windowTextComboBox, 1 ,1);
mainLayout->addWidget(buttonLabel, 2 ,0);
mainLayout->addWidget(buttonComboBox, 2 ,1);
mainLayout->addWidget(buttonTextLabel, 3 ,0);
mainLayout->addWidget(buttonTextComboBox, 3 ,1);
mainLayout->addWidget(baseLabel, 4 ,0);
mainLayout->addWidget(baseComboBox, 4 ,1);
}
void Palette::createContentFrame()
{
contentFrame = new QFrame;
contentFrame->setAutoFillBackground(true); //* 后加内容
label1 = new QLabel(tr("请选择一个值: "));
comboBox1 = new QComboBox;
label2 = new QLabel(tr("请输入字符串: "));
lineEdit2 = new QLineEdit;
textEdit = new QTextEdit;
QGridLayout *TopLayout = new QGridLayout;
TopLayout->addWidget(label1, 0, 0);
TopLayout->addWidget(comboBox1, 0, 1);
TopLayout->addWidget(label2, 1, 0);
TopLayout->addWidget(lineEdit2, 1, 1);
TopLayout->addWidget(textEdit, 2, 0, 1, 2);
okBtn = new QPushButton(tr("确认"));
CancelBtn = new QPushButton(tr("取消"));
CancelBtn->setAutoFillBackground(true); //* 后加内容 相当于开关 打开可设置按钮背景色 按钮下的一小块部分
CancelBtn->setFlat(true); //* 后加内容 相当于开关 打开可设置按钮背景色 按钮表面的那部分
QHBoxLayout *BottomLayout = new QHBoxLayout;
BottomLayout->addStretch(1);
BottomLayout->addWidget(okBtn);
BottomLayout->addWidget(CancelBtn);
QVBoxLayout *mainLayout = new QVBoxLayout(contentFrame);
mainLayout->addLayout(TopLayout);
mainLayout->addLayout(BottomLayout);
}
void Palette::ShowWindow()
{
QStringList colorList = QColor::colorNames();
QColor color = QColor(colorList[windowComboBox->currentIndex()]); //获取当前选择的颜色值
QPalette p = contentFrame->palette(); //获取右部分窗体的调色板信息
p.setColor(QPalette::Window, color); //设置contentFrame窗体的Window类颜色 即背景色 参数1:设置的主题色 参数2:具体的颜色值
contentFrame->setPalette(p); //将获取到的颜色值进行应用
contentFrame->update();
}
void Palette::ShowWindowText()
{
QStringList colorList = QColor::colorNames();
QColor color = colorList[windowTextComboBox->currentIndex()]; //获取当前选择的颜色值
QPalette p = contentFrame->palette();
p.setColor(QPalette::WindowText, color);
contentFrame->setPalette(p);
}
void Palette::ShowButton()
{
QStringList colorList = QColor::colorNames();
QColor color = QColor(colorList[buttonComboBox->currentIndex()]); //获取当前选择的颜色值
QPalette p = contentFrame->palette();
p.setColor(QPalette::Button, color);
contentFrame->setPalette(p);
contentFrame->update();
}
void Palette::ShowButtonText()
{
QStringList colorList = QColor::colorNames();
QColor color = QColor(colorList[buttonTextComboBox->currentIndex()]); //获取当前选择的颜色值
QPalette p = contentFrame->palette();
p.setColor(QPalette::ButtonText, color);
contentFrame->setPalette(p);
}
void Palette::ShowBase()
{
QStringList colorList = QColor::colorNames();
QColor color = QColor(colorList[baseComboBox->currentIndex()]); //获取当前选择的颜色值
QPalette p = contentFrame->palette();
p.setColor(QPalette::Base, color);
contentFrame->setPalette(p);
}
void Palette::fillColorList(QComboBox *comboBox)
{
QStringList colorList = QColor::colorNames(); //获得Qt所有导致名称的颜色列表 返回的是一个字符串列表colorlist
QString color;
foreach(color, colorList)
{
QPixmap pix(QSize(70, 20)); //新建一个QPixmap对象 pix作为显示颜色的图标
pix.fill(QColor(color)); //为 pix 填充当前的颜色
comboBox->addItem(QIcon(pix), NULL); // 调用QcomboBox 的addItem 为下拉列表插入一个条目 用准备好的pix作为插入条目的图标 名称为Null 不显示名称
comboBox->setIconSize(QSize(70, 20)); //设置图标尺寸
comboBox->setSizeAdjustPolicy(QComboBox::AdjustToContents); // 下拉表框的调整策略为AdjustToContents 符合内容的大小
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
QPalette QColor 窗体颜色主题案例
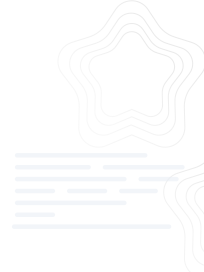
共5个文件
cpp:2个
user:1个
pro:1个

需积分: 5 3 下载量 99 浏览量
2023-01-17
10:25:47
上传
评论
收藏 5KB 7Z 举报
温馨提示
展示不同 QPalette颜色角色所改变的颜色内容(QPalette::ColorRole)
资源推荐
资源详情
资源评论
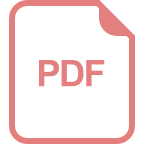
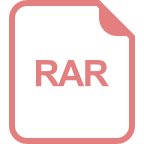
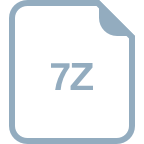
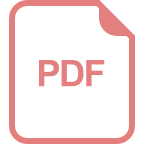
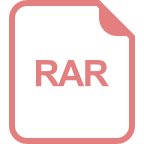
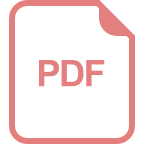
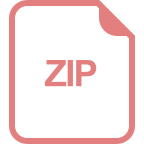
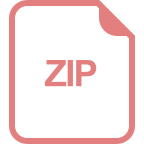
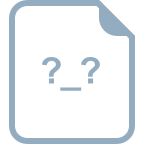
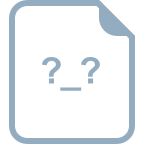
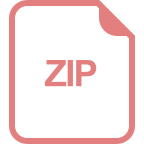
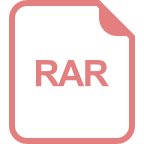
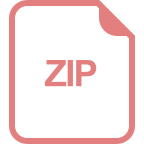
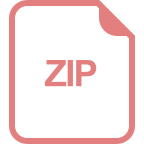
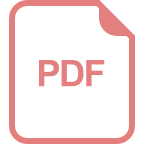
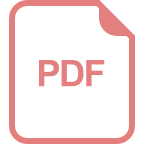
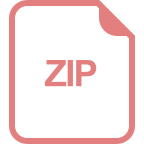
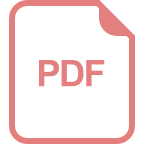
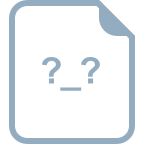
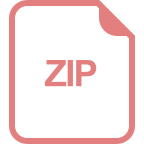
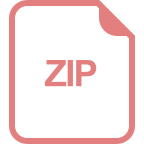
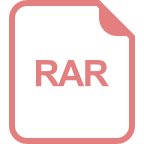
收起资源包目录

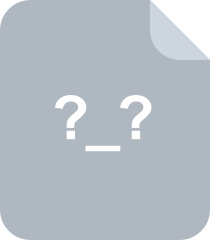
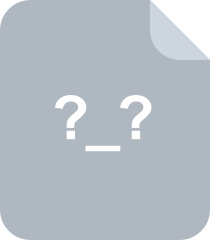
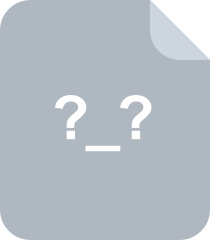
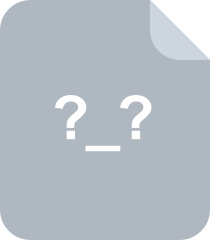
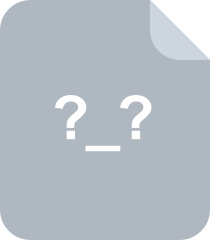
共 5 条
- 1
资源评论
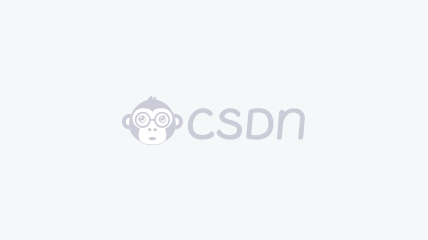

Evans_Y
- 粉丝: 151
- 资源: 4
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

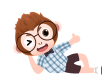
安全验证
文档复制为VIP权益,开通VIP直接复制
