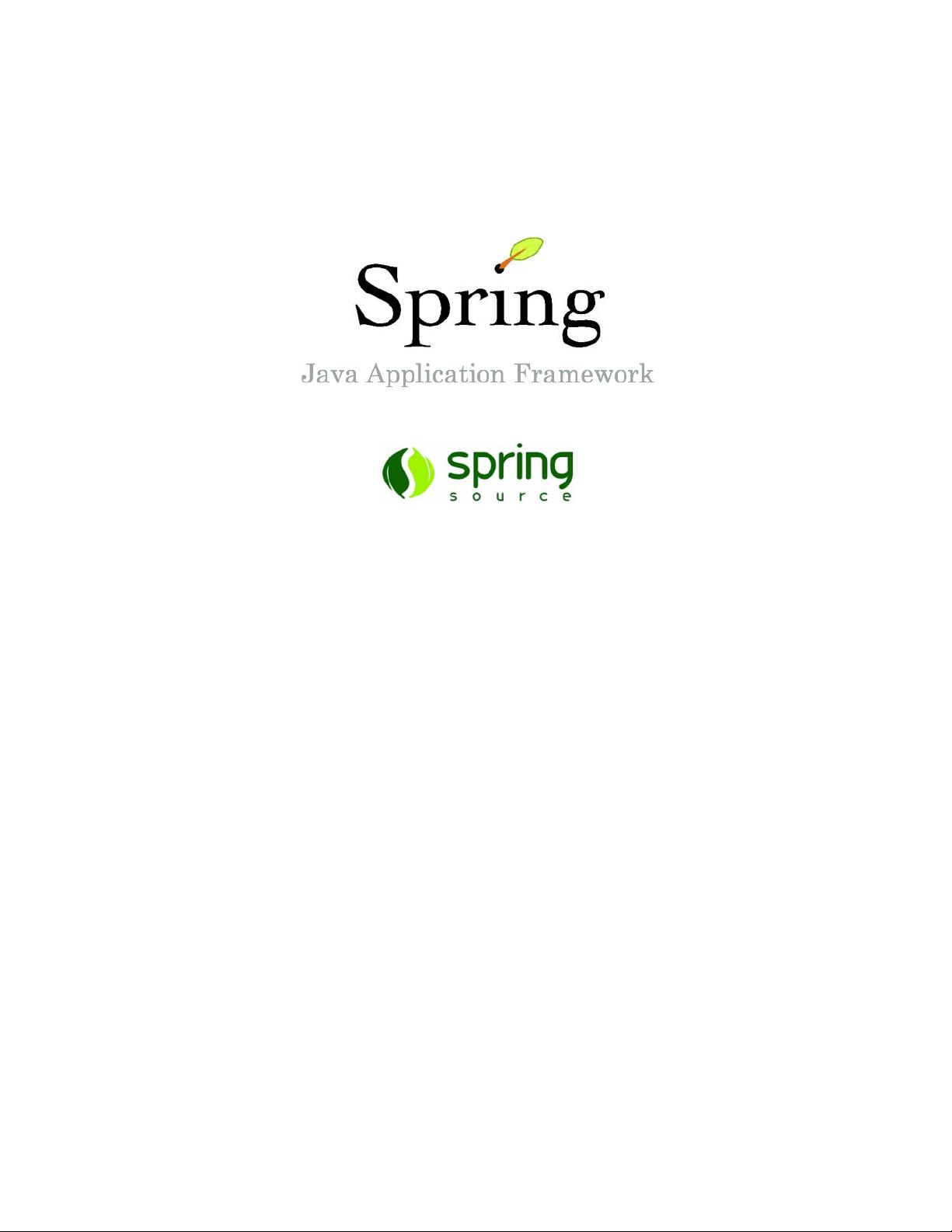
Reference Documentation
3.1
Copyright © 2004-2011 Rod Johnson, Juergen Hoeller, Keith Donald, Colin Sampaleanu, Rob
Harrop, Alef Arendsen, Thomas Risberg, Darren Davison, Dmitriy Kopylenko, Mark Pollack,
Thierry Templier, Erwin Vervaet, Portia Tung, Ben Hale, Adrian Colyer, John Lewis, Costin
Leau, Mark Fisher, Sam Brannen, Ramnivas Laddad, Arjen Poutsma, Chris Beams, Tareq
Abedrabbo, Andy Clement, Dave Syer, Oliver Gierke, Rossen Stoyanchev
Copies of this document may be made for your own use and for distribution to others, provided
that you do not charge any fee for such copies and further provided that each copy contains this
Copyright Notice, whether distributed in print or electronically.
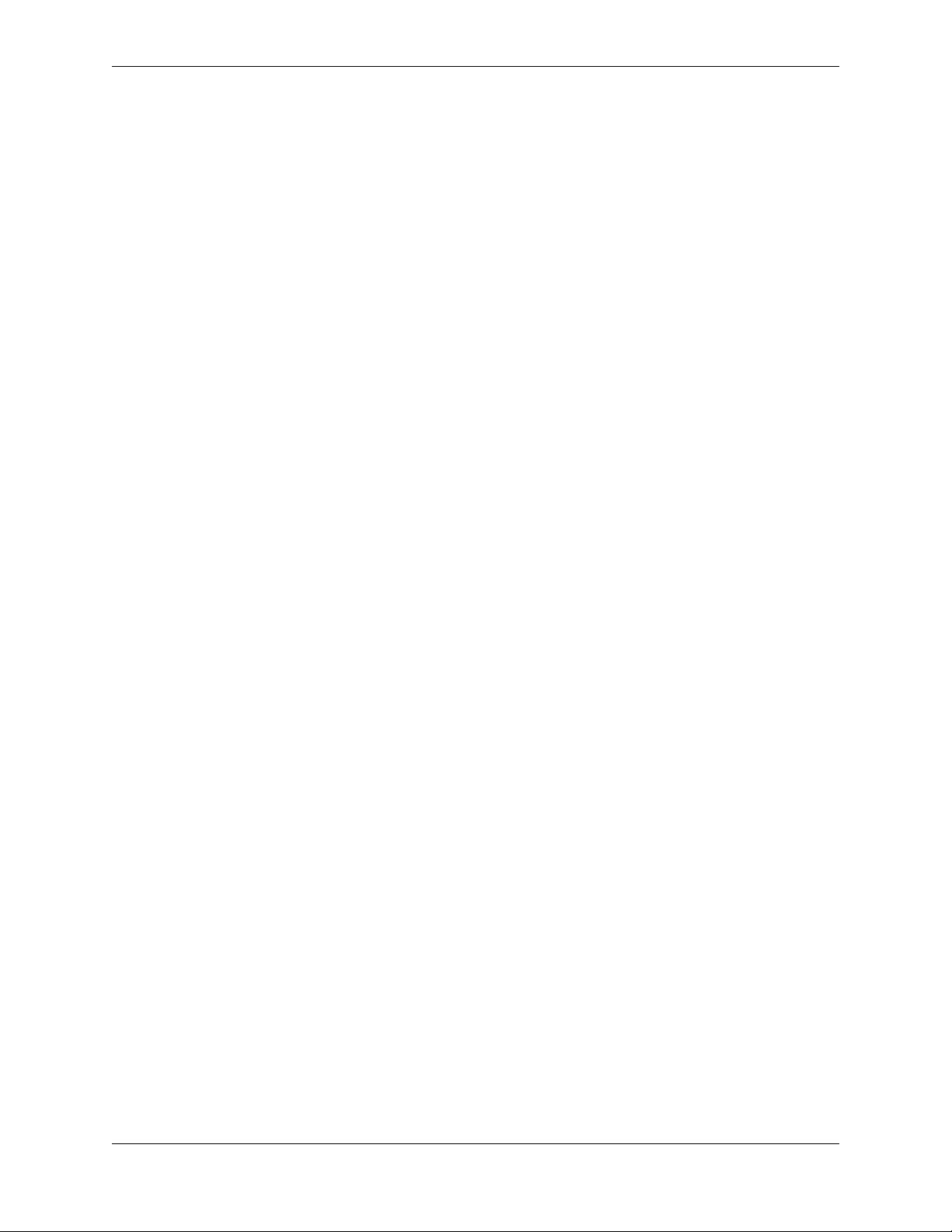
Table of Contents
I. Overview of Spring Framework ..............................................................................................1
1. Introduction to Spring Framework ..................................................................................2
1.1. Dependency Injection and Inversion of Control ....................................................2
1.2. Modules ............................................................................................................3
Core Container .................................................................................................3
Data Access/Integration ....................................................................................4
Web .................................................................................................................4
AOP and Instrumentation ..................................................................................5
Test .................................................................................................................5
1.3. Usage scenarios .................................................................................................5
Dependency Management and Naming Conventions ...........................................9
Spring Dependencies and Depending on Spring ........................................11
Maven Dependency Management ............................................................12
Ivy Dependency Management .................................................................13
Logging .........................................................................................................14
Not Using Commons Logging .................................................................14
Using SLF4J ..........................................................................................15
Using Log4J ...........................................................................................16
II. What's New in Spring 3 ......................................................................................................18
2. New Features and Enhancements in Spring 3.0 .............................................................19
2.1. Java 5 ..............................................................................................................19
2.2. Improved documentation ..................................................................................19
2.3. New articles and tutorials ..................................................................................19
2.4. New module organization and build system .......................................................20
2.5. Overview of new features .................................................................................21
Core APIs updated for Java 5 ..........................................................................22
Spring Expression Language ...........................................................................22
The Inversion of Control (IoC) container ..........................................................23
Java based bean metadata ........................................................................23
Defining bean metadata within components ..............................................24
General purpose type conversion system and field formatting system .................24
The Data Tier .................................................................................................24
The Web Tier .................................................................................................25
Comprehensive REST support .................................................................25
@MVC additions ...................................................................................25
Declarative model validation ...........................................................................25
Early support for Java EE 6 .............................................................................25
Support for embedded databases ......................................................................25
3. New Features and Enhancements in Spring 3.1 .............................................................26
3.1. Overview of new features .................................................................................26
Spring Framework
3.1 Reference Documentation ii
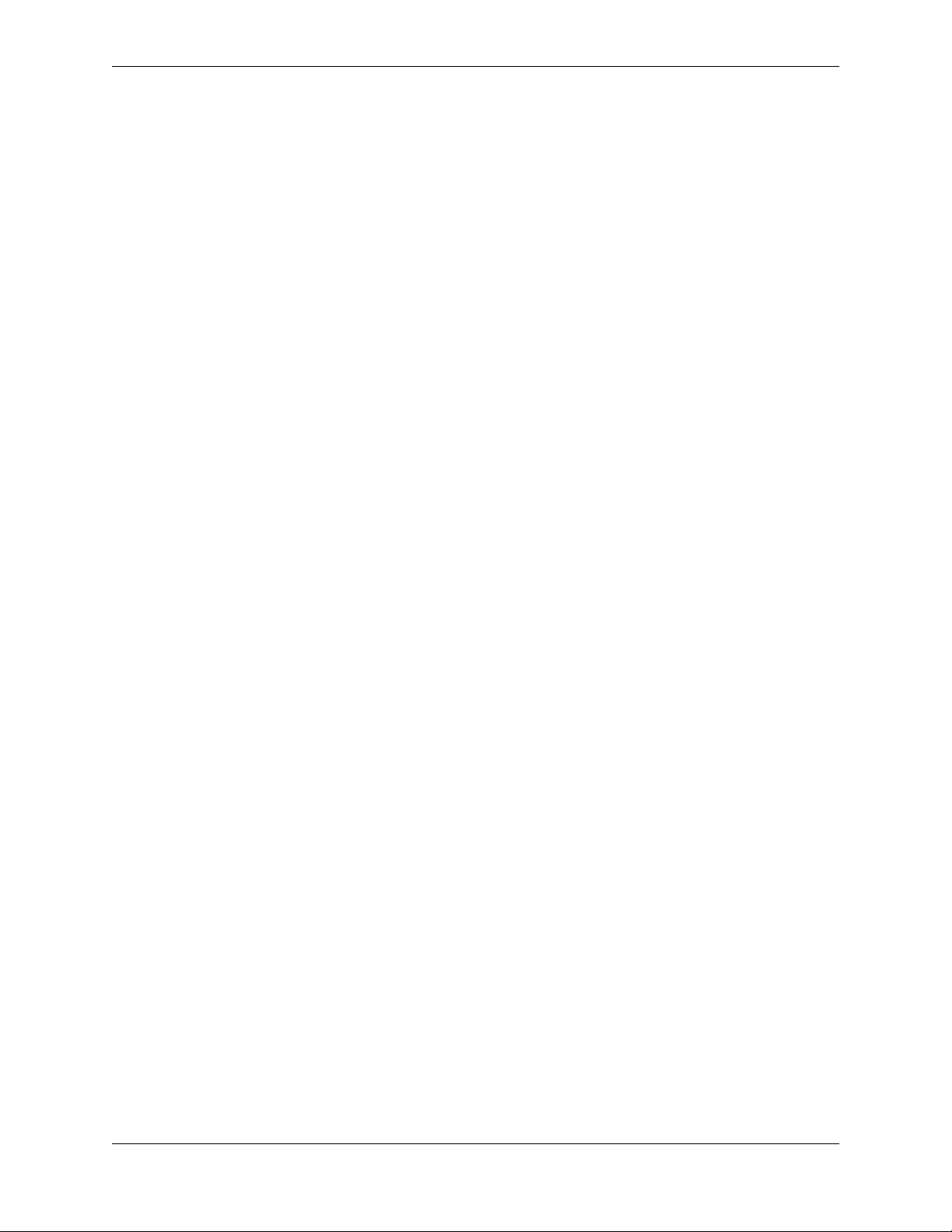
Cache Abstraction ..........................................................................................26
Bean Definition Profiles ..................................................................................26
Environment Abstraction ................................................................................26
PropertySource Abstraction .............................................................................26
Code equivalents for Spring's XML namespaces ...............................................27
Support for Hibernate 4.x ................................................................................27
TestContext framework support for @Configuration classes and bean definition
profiles ..........................................................................................................27
c: namespace for more concise constructor injection .........................................28
Support for injection against non-standard JavaBeans setters .............................28
Support for Servlet 3 code-based configuration of Servlet Container ..................28
Support for Servlet 3 MultipartResolver ...........................................................28
JPA EntityManagerFactory bootstrapping without persistence.xml ....................28
New HandlerMethod-based Support Classes For Annotated Controller Processing
.......................................................................................................................29
"consumes" and "produces" conditions in @RequestMapping ...........................30
Flash Attributes and RedirectAttributes ............................................................30
URI Template Variable Enhancements .............................................................30
@Valid On @RequestBody Controller Method Arguments ...............................30
@RequestPart Annotation On Controller Method Arguments ............................30
UriComponentsBuilder and UriComponents .....................................................31
III. Core Technologies ............................................................................................................32
4. The IoC container .......................................................................................................33
4.1. Introduction to the Spring IoC container and beans .............................................33
4.2. Container overview ..........................................................................................33
Configuration metadata ...................................................................................34
Instantiating a container ..................................................................................36
Composing XML-based configuration metadata .......................................37
Using the container .........................................................................................38
4.3. Bean overview .................................................................................................38
Naming beans .................................................................................................40
Aliasing a bean outside the bean definition ...............................................40
Instantiating beans ..........................................................................................41
Instantiation with a constructor ................................................................42
Instantiation with a static factory method .................................................42
Instantiation using an instance factory method ..........................................43
4.4. Dependencies ...................................................................................................44
Dependency injection ......................................................................................44
Constructor-based dependency injection ...................................................44
Setter-based dependency injection ...........................................................47
Dependency resolution process ................................................................48
Examples of dependency injection ...........................................................49
Dependencies and configuration in detail .........................................................51
Straight values (primitives, Strings, and so on) .........................................51
References to other beans (collaborators) .................................................53
Spring Framework
3.1 Reference Documentation iii
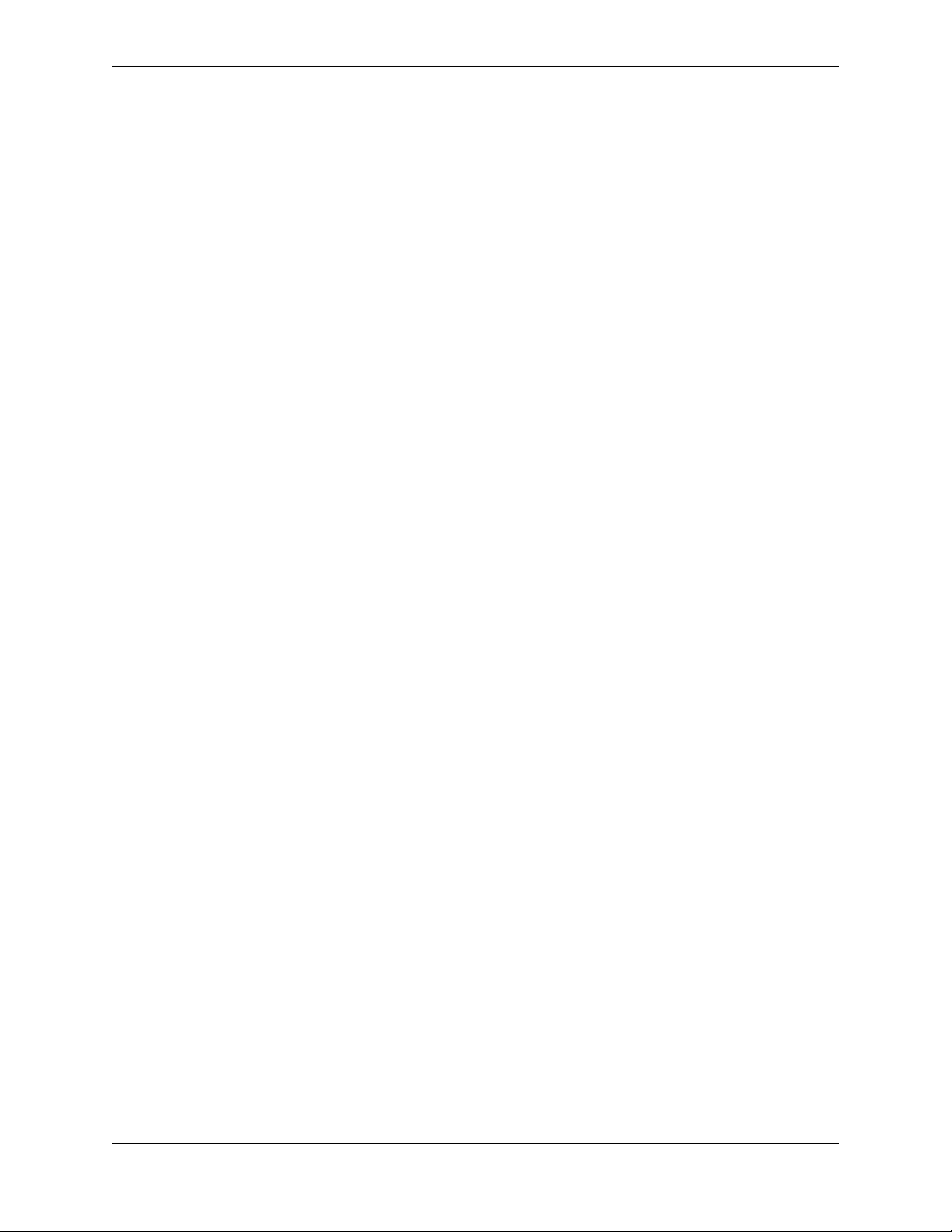
Inner beans .............................................................................................54
Collections .............................................................................................54
Null and empty string values ...................................................................57
XML shortcut with the p-namespace ........................................................57
XML shortcut with the c-namespace ........................................................58
Compound property names ......................................................................59
Using depends-on ...........................................................................................59
Lazy-initialized beans .....................................................................................60
Autowiring collaborators .................................................................................61
Limitations and disadvantages of autowiring ............................................62
Excluding a bean from autowiring ...........................................................63
Method injection ............................................................................................63
Lookup method injection .........................................................................64
Arbitrary method replacement .................................................................66
4.5. Bean scopes .....................................................................................................67
The singleton scope ........................................................................................68
The prototype scope ........................................................................................69
Singleton beans with prototype-bean dependencies ...........................................70
Request, session, and global session scopes ......................................................70
Initial web configuration .........................................................................70
Request scope .........................................................................................71
Session scope .........................................................................................72
Global session scope ...............................................................................72
Scoped beans as dependencies .................................................................72
Custom scopes ...............................................................................................74
Creating a custom scope ..........................................................................75
Using a custom scope ..............................................................................75
4.6. Customizing the nature of a bean .......................................................................77
Lifecycle callbacks .........................................................................................77
Initialization callbacks ............................................................................77
Destruction callbacks ..............................................................................78
Default initialization and destroy methods ................................................79
Combining lifecycle mechanisms ............................................................80
Startup and shutdown callbacks ...............................................................80
Shutting down the Spring IoC container gracefully in non-web applications 82
ApplicationContextAware and BeanNameAware ..............................................83
Other Aware interfaces ...................................................................................84
4.7. Bean definition inheritance ...............................................................................86
4.8. Container Extension Points ...............................................................................87
Customizing beans using a BeanPostProcessor .................................................87
Example: Hello World, BeanPostProcessor-style ......................................89
Example: The RequiredAnnotationBeanPostProcessor ..............................90
Customizing configuration metadata with a BeanFactoryPostProcessor ..............90
Example: the PropertyPlaceholderConfigurer ...........................................91
Example: the PropertyOverrideConfigurer ...............................................93
Spring Framework
3.1 Reference Documentation iv
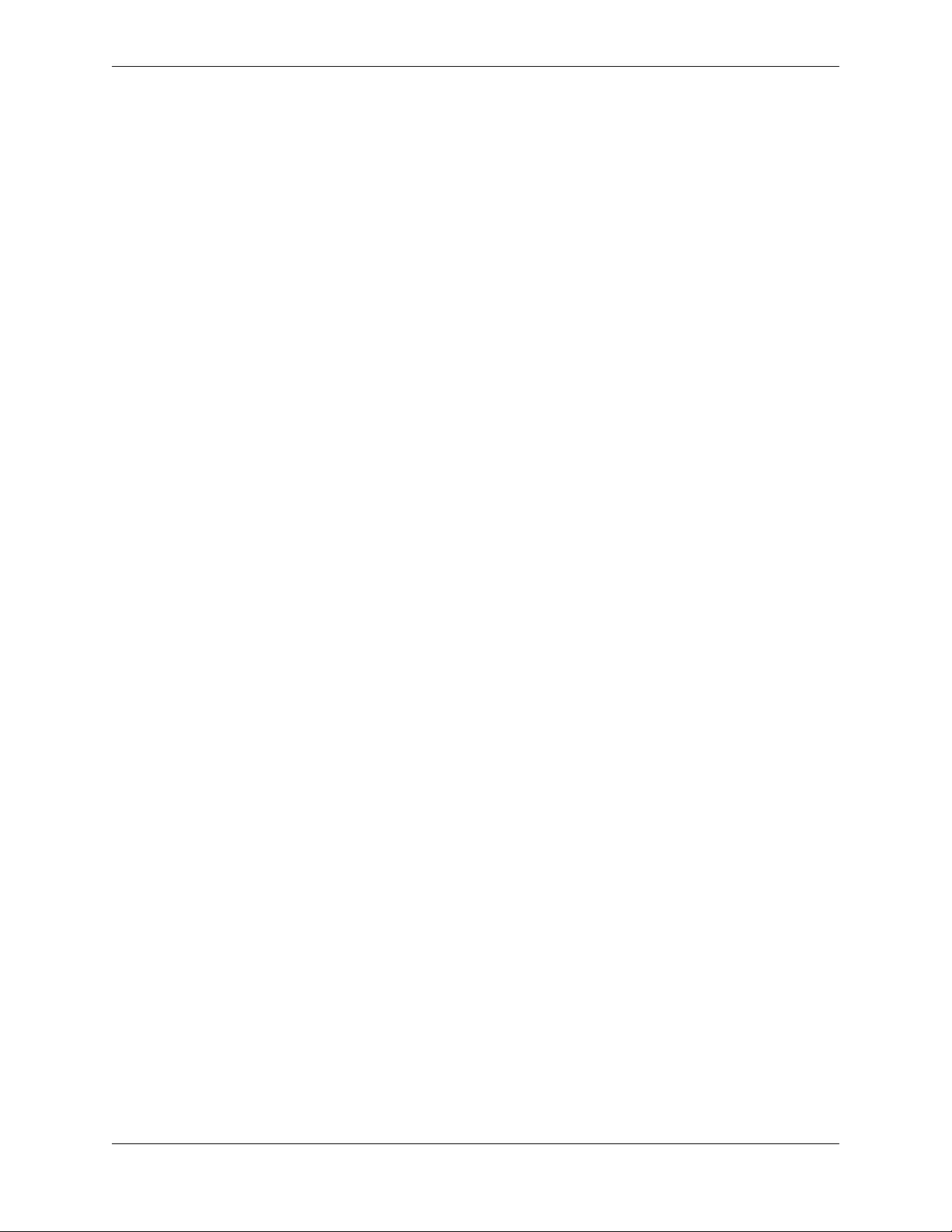
Customizing instantiation logic with a FactoryBean ..........................................94
4.9. Annotation-based container configuration ..........................................................95
@Required .....................................................................................................96
@Autowired ...................................................................................................97
Fine-tuning annotation-based autowiring with qualifiers ...................................99
CustomAutowireConfigurer ..........................................................................104
@Resource ...................................................................................................105
@PostConstruct and @PreDestroy .................................................................106
4.10. Classpath scanning and managed components ................................................107
@Component and further stereotype annotations ............................................107
Automatically detecting classes and registering bean definitions ......................107
Using filters to customize scanning ................................................................109
Defining bean metadata within components ....................................................110
Naming autodetected components ..................................................................111
Providing a scope for autodetected components ..............................................112
Providing qualifier metadata with annotations ................................................112
4.11. Using JSR 330 Standard Annotations .............................................................113
Dependency Injection with @Inject and @Named ..........................................114
@Named: a standard equivalent to the @Component annotation ......................114
Limitations of the standard approach ..............................................................115
4.12. Java-based container configuration ................................................................116
Basic concepts: @Configuration and @Bean ..................................................116
Instantiating the Spring container using AnnotationConfigApplicationContext .116
Simple construction ..............................................................................117
Building the container programmatically using register(Class<?>...) ........117
Enabling component scanning with scan(String...) ..................................117
Support for web applications with AnnotationConfigWebApplicationContext
.............................................................................................................118
Composing Java-based configurations ............................................................119
Using the @Import annotation ...............................................................119
Combining Java and XML configuration ................................................122
Using the @Bean annotation .........................................................................124
Declaring a bean ...................................................................................124
Injecting dependencies ..........................................................................125
Receiving lifecycle callbacks .................................................................125
Specifying bean scope ...........................................................................126
Customizing bean naming .....................................................................128
Bean aliasing ........................................................................................128
Further information about how Java-based configuration works internally ........128
4.13. Registering a LoadTimeWeaver ....................................................................129
4.14. Additional Capabilities of the ApplicationContext ..........................................130
Internationalization using MessageSource ......................................................130
Standard and Custom Events .........................................................................133
Convenient access to low-level resources .......................................................136
Convenient ApplicationContext instantiation for web applications ...................137
Spring Framework
3.1 Reference Documentation v