<?php if ( ! defined('BASEPATH')) exit('No direct script access allowed');
/**
* CodeIgniter
*
* An open source application development framework for PHP 4.3.2 or newer
*
* @package CodeIgniter
* @author ExpressionEngine Dev Team
* @copyright Copyright (c) 2008 - 2010, EllisLab, Inc.
* @license http://codeigniter.com/user_guide/license.html
* @link http://codeigniter.com
* @since Version 1.0
* @filesource
*/
// ------------------------------------------------------------------------
/**
* CodeIgniter Email Class
*
* Permits email to be sent using Mail, Sendmail, or SMTP.
*
* @package CodeIgniter
* @subpackage Libraries
* @category Libraries
* @author ExpressionEngine Dev Team
* @link http://codeigniter.com/user_guide/libraries/email.html
*/
class CI_Email {
var $useragent = "CodeIgniter";
var $mailpath = "/usr/sbin/sendmail"; // Sendmail path
var $protocol = "mail"; // mail/sendmail/smtp
var $smtp_host = ""; // SMTP Server. Example: mail.earthlink.net
var $smtp_user = ""; // SMTP Username
var $smtp_pass = ""; // SMTP Password
var $smtp_port = "25"; // SMTP Port
var $smtp_timeout = 5; // SMTP Timeout in seconds
var $wordwrap = TRUE; // TRUE/FALSE Turns word-wrap on/off
var $wrapchars = "76"; // Number of characters to wrap at.
var $mailtype = "text"; // text/html Defines email formatting
var $charset = "utf-8"; // Default char set: iso-8859-1 or us-ascii
var $multipart = "mixed"; // "mixed" (in the body) or "related" (separate)
var $alt_message = ''; // Alternative message for HTML emails
var $validate = FALSE; // TRUE/FALSE. Enables email validation
var $priority = "3"; // Default priority (1 - 5)
var $newline = "\n"; // Default newline. "\r\n" or "\n" (Use "\r\n" to comply with RFC 822)
var $crlf = "\n"; // The RFC 2045 compliant CRLF for quoted-printable is "\r\n". Apparently some servers,
// even on the receiving end think they need to muck with CRLFs, so using "\n", while
// distasteful, is the only thing that seems to work for all environments.
var $send_multipart = TRUE; // TRUE/FALSE - Yahoo does not like multipart alternative, so this is an override. Set to FALSE for Yahoo.
var $bcc_batch_mode = FALSE; // TRUE/FALSE Turns on/off Bcc batch feature
var $bcc_batch_size = 200; // If bcc_batch_mode = TRUE, sets max number of Bccs in each batch
var $_safe_mode = FALSE;
var $_subject = "";
var $_body = "";
var $_finalbody = "";
var $_alt_boundary = "";
var $_atc_boundary = "";
var $_header_str = "";
var $_smtp_connect = "";
var $_encoding = "8bit";
var $_IP = FALSE;
var $_smtp_auth = FALSE;
var $_replyto_flag = FALSE;
var $_debug_msg = array();
var $_recipients = array();
var $_cc_array = array();
var $_bcc_array = array();
var $_headers = array();
var $_attach_name = array();
var $_attach_type = array();
var $_attach_disp = array();
var $_protocols = array('mail', 'sendmail', 'smtp');
var $_base_charsets = array('us-ascii', 'iso-2022-'); // 7-bit charsets (excluding language suffix)
var $_bit_depths = array('7bit', '8bit');
var $_priorities = array('1 (Highest)', '2 (High)', '3 (Normal)', '4 (Low)', '5 (Lowest)');
/**
* Constructor - Sets Email Preferences
*
* The constructor can be passed an array of config values
*/
function CI_Email($config = array())
{
if (count($config) > 0)
{
$this->initialize($config);
}
else
{
$this->_smtp_auth = ($this->smtp_user == '' AND $this->smtp_pass == '') ? FALSE : TRUE;
$this->_safe_mode = ((boolean)@ini_get("safe_mode") === FALSE) ? FALSE : TRUE;
}
log_message('debug', "Email Class Initialized");
}
// --------------------------------------------------------------------
/**
* Initialize preferences
*
* @access public
* @param array
* @return void
*/
function initialize($config = array())
{
$this->clear();
foreach ($config as $key => $val)
{
if (isset($this->$key))
{
$method = 'set_'.$key;
if (method_exists($this, $method))
{
$this->$method($val);
}
else
{
$this->$key = $val;
}
}
}
$this->_smtp_auth = ($this->smtp_user == '' AND $this->smtp_pass == '') ? FALSE : TRUE;
$this->_safe_mode = ((boolean)@ini_get("safe_mode") === FALSE) ? FALSE : TRUE;
}
// --------------------------------------------------------------------
/**
* Initialize the Email Data
*
* @access public
* @return void
*/
function clear($clear_attachments = FALSE)
{
$this->_subject = "";
$this->_body = "";
$this->_finalbody = "";
$this->_header_str = "";
$this->_replyto_flag = FALSE;
$this->_recipients = array();
$this->_headers = array();
$this->_debug_msg = array();
$this->_set_header('User-Agent', $this->useragent);
$this->_set_header('Date', $this->_set_date());
if ($clear_attachments !== FALSE)
{
$this->_attach_name = array();
$this->_attach_type = array();
$this->_attach_disp = array();
}
}
// --------------------------------------------------------------------
/**
* Set FROM
*
* @access public
* @param string
* @param string
* @return void
*/
function from($from, $name = '')
{
if (preg_match( '/\<(.*)\>/', $from, $match))
{
$from = $match['1'];
}
if ($this->validate)
{
$this->validate_email($this->_str_to_array($from));
}
// prepare the display name
if ($name != '')
{
// only use Q encoding if there are characters that would require it
if ( ! preg_match('/[\200-\377]/', $name))
{
// add slashes for non-printing characters, slashes, and double quotes, and surround it in double quotes
$name = '"'.addcslashes($name, "\0..\37\177'\"\\").'"';
}
else
{
$name = $this->_prep_q_encoding($name, TRUE);
}
}
$this->_set_header('From', $name.' <'.$from.'>');
$this->_set_header('Return-Path', '<'.$from.'>');
}
// --------------------------------------------------------------------
/**
* Set Reply-to
*
* @access public
* @param string
* @param string
* @return void
*/
function reply_to($replyto, $name = '')
{
if (preg_match( '/\<(.*)\>/', $replyto, $match))
{
$replyto = $match['1'];
}
if ($this->validate)
{
$this->validate_email($this->_str_to_array($replyto));
}
if ($name == '')
{
$name = $replyto;
}
if (strncmp($name, '"', 1) != 0)
{
$name = '"'.$name.'"';
}
$this->_set_header('Reply-To', $name.' <'.$replyto.'>');
$this->_replyto_flag = TRUE;
}
// --------------------------------------------------------------------
/**
* Set Recipients
*
* @access public
* @param string
* @return void
*/
function to($to)
{
$to = $this->_str_to_array($to);
$to = $this->clean_email($to);
if ($this->validate)
{
$this->validate_email($to);
}
if ($this->_get_protocol() != 'mail')
{
$this->_set_header('To', implode(", ", $to));
}
switch ($this->_get_protocol())
{
case 'smtp' : $this->_recipients = $to;
break;
case 'sendmail' : $this->_recipients = implode(", ", $to);
break;
case 'mail' : $this->_recipients = implode(", ", $to);
break;
}
}
// --------------------------------------------------------------------
/**
* Set CC
*
* @access public
* @param string
* @return void
*/
function cc($cc)
{
$cc = $this->_str_to_array($cc);
$cc = $this->clean_email($cc);
if ($this->validate)
{
$this->validate_email($cc);
}
$this->_set_header('Cc', implode(", ", $cc));
if ($this->_get_protocol() == "smtp")
{
$this->_cc_array = $cc;
}
}
// --------------------------------------------------------------------
/**
* Set BCC
*
* @access public
* @param string
* @param string
* @return void
*/
function bcc($bcc, $limit = '')
{
if ($limit != '' && is_numeric($limit))
{
$this->bcc_batch_mode = TRUE;
$this->bcc_batch_size = $limit;
}
$bcc = $this->_str_to_array($bcc);
$bcc = $t
没有合适的资源?快使用搜索试试~ 我知道了~
php轻量级框架CodeIgniter_1.7.3
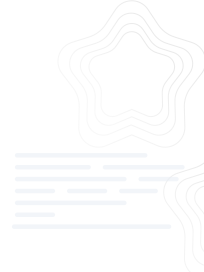
共172个文件
php:136个
html:32个
jpg:2个

需积分: 10 10 下载量 180 浏览量
2011-04-27
13:30:38
上传
评论
收藏 371KB RAR 举报
温馨提示
你想要一个小巧的框架。 你需要出色的性能。 你需要广泛兼容标准主机上的各种 PHP 版本和配置。 你想要一个几乎只需 0 配置的框架。 你想要一个不需使用命令行的框架。 你想要一个不需坚守限制性编码规则的框架。 你对 PEAR 这种大规模集成类库不感兴趣。 你不希望被迫学习一门模板语言(虽然可以选择你喜欢的模板解析器)。 你不喜欢复杂,热爱简单。 你需要清晰、完善的文档。
资源推荐
资源详情
资源评论
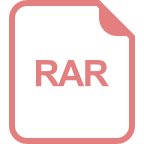
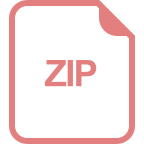
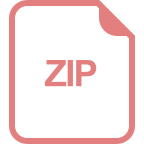
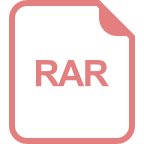
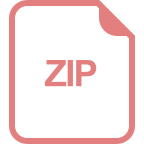
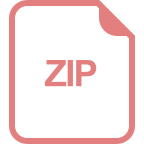
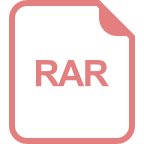
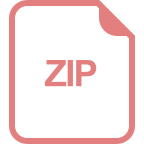
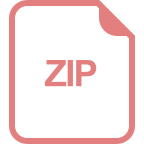
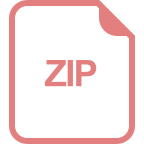
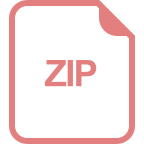
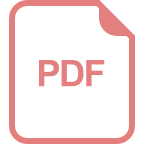
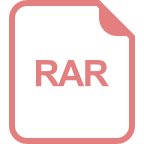
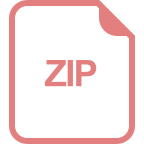
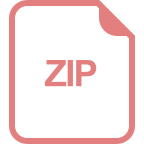
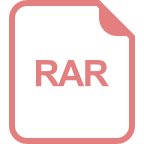
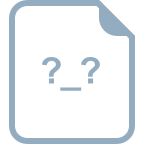
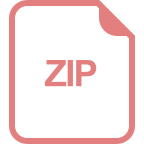
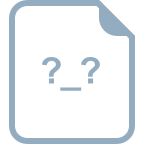
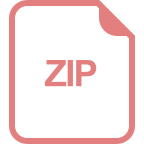
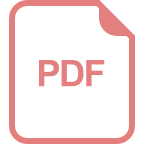
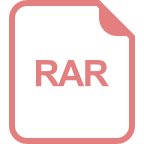
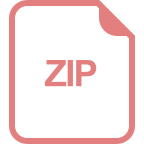
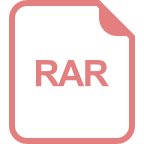
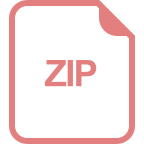
收起资源包目录

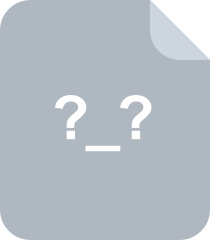
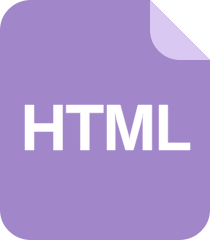
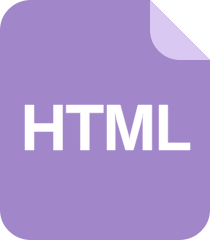
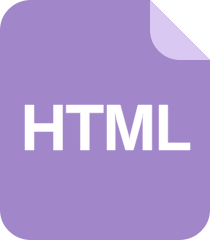
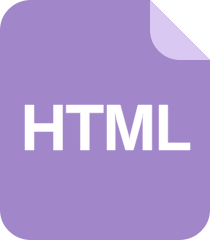
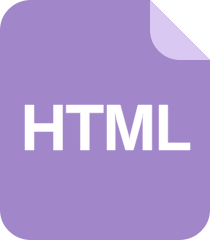
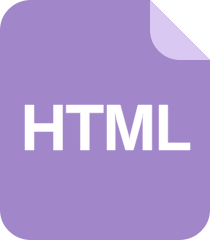
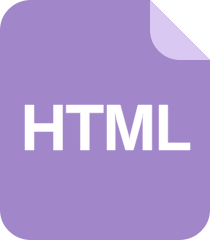
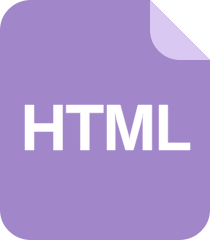
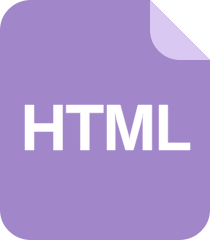
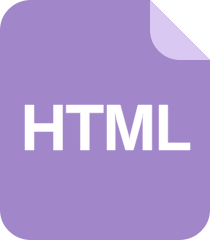
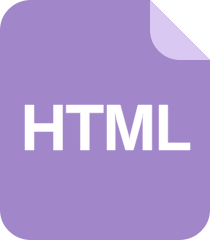
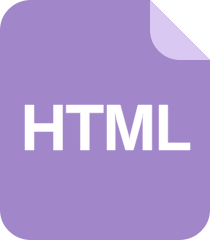
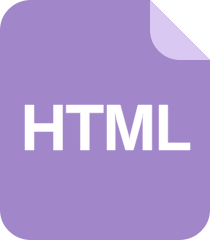
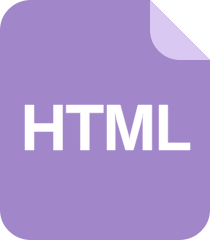
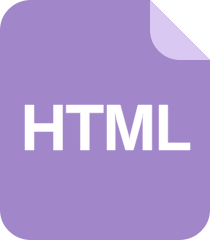
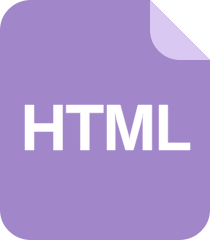
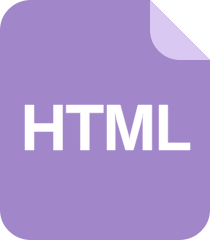
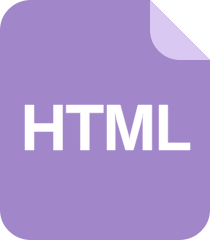
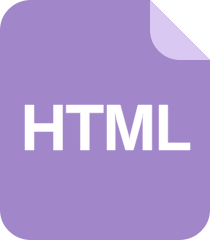
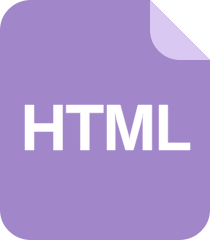
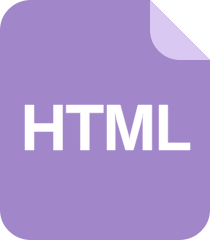
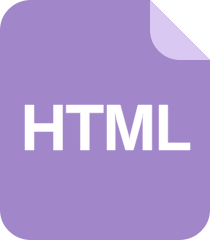
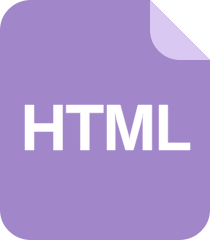
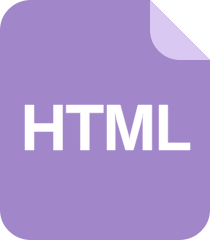
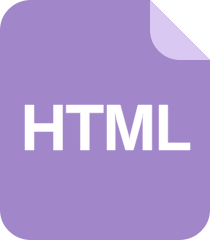
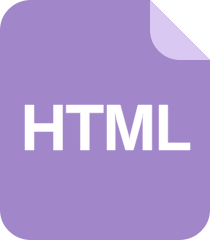
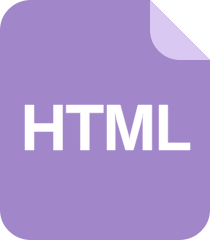
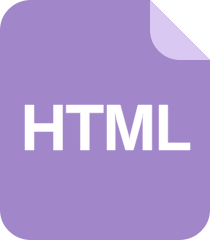
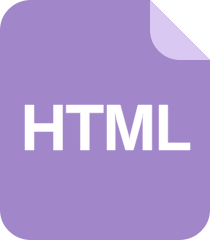
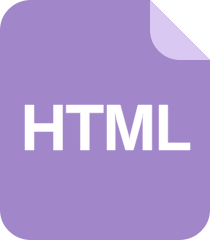
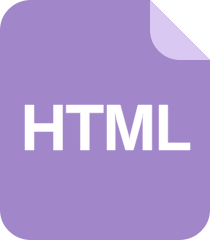
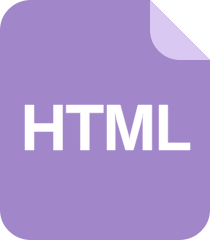
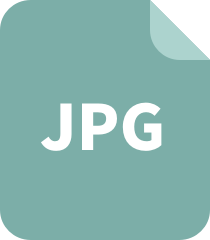
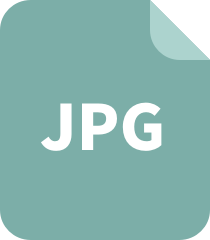
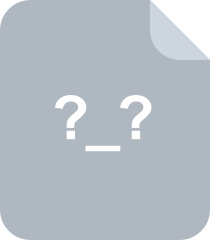
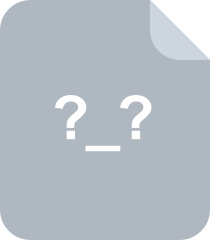
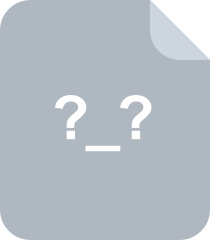
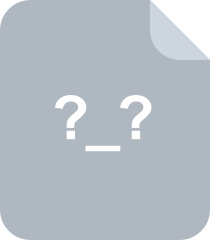
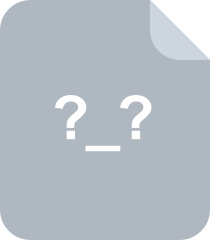
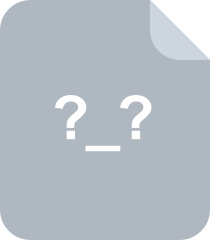
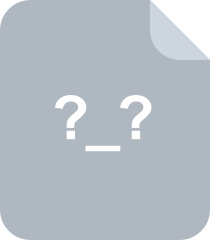
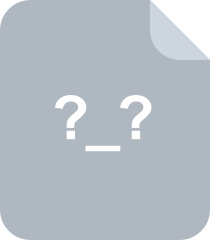
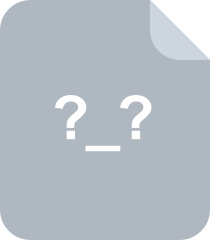
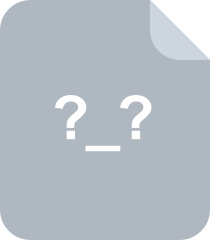
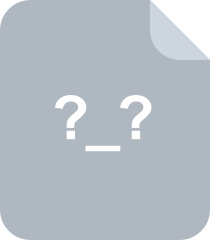
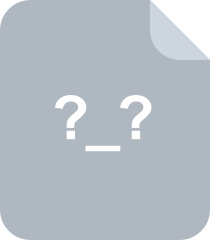
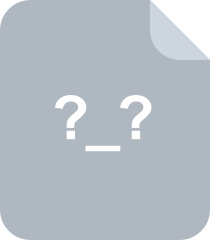
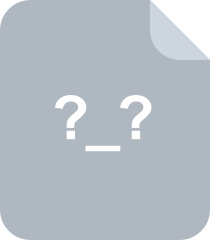
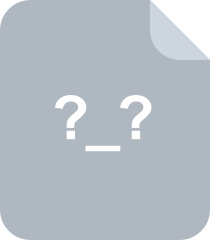
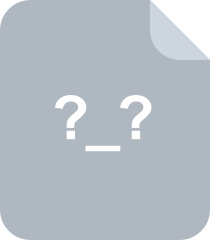
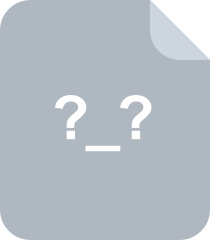
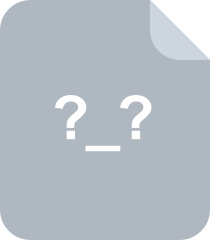
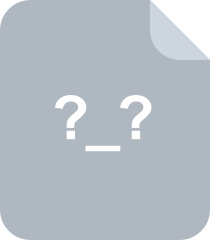
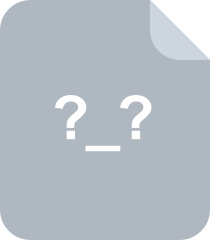
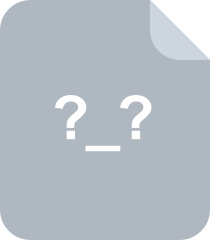
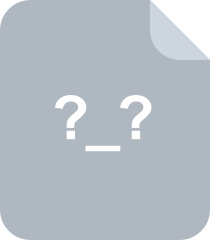
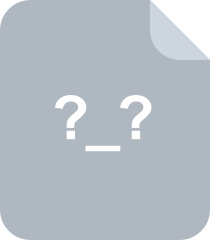
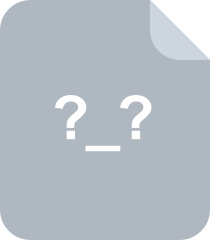
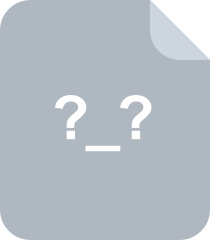
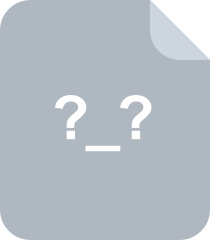
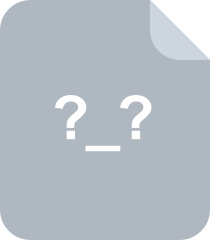
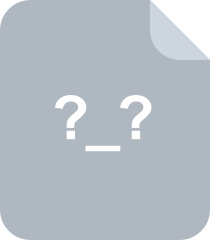
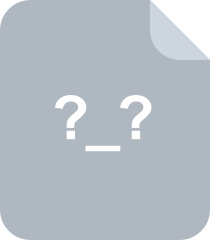
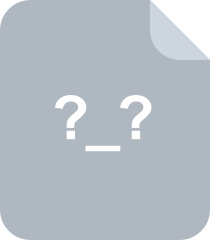
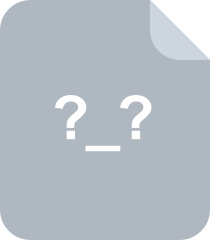
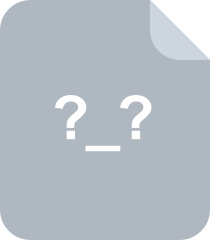
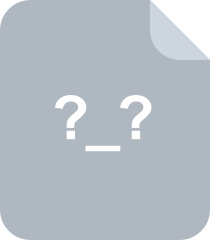
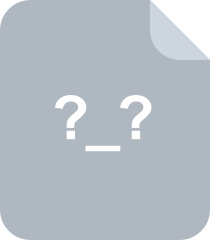
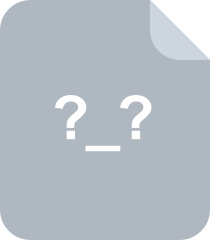
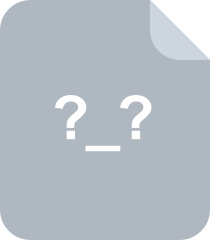
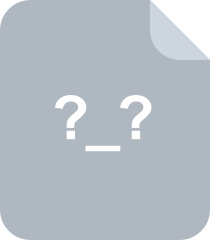
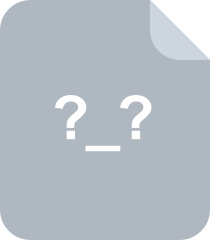
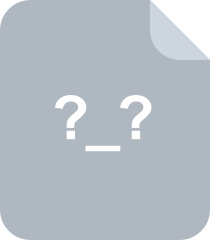
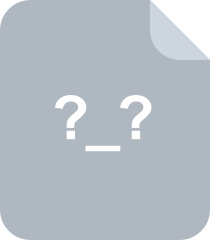
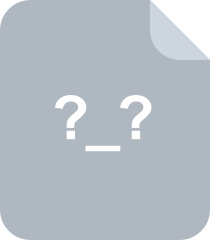
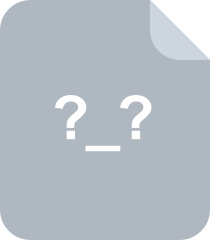
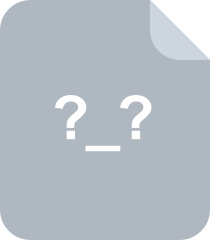
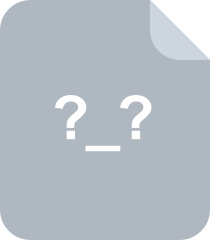
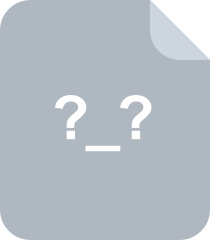
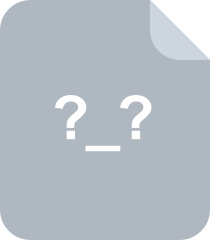
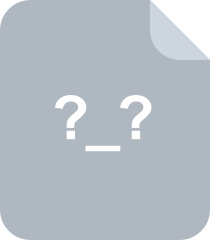
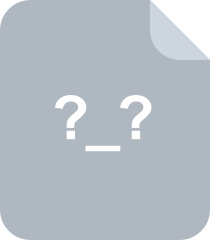
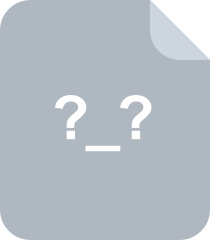
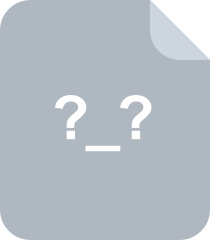
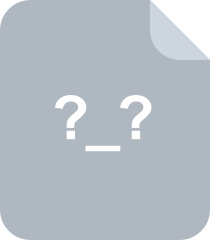
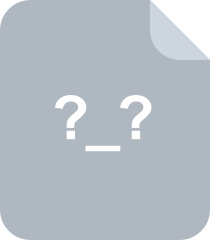
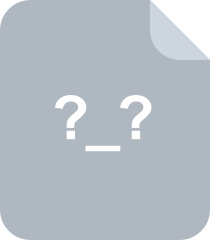
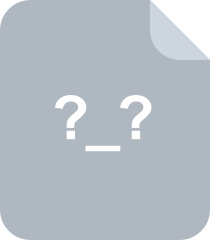
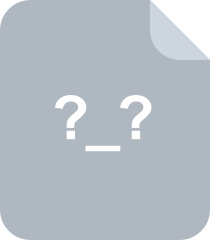
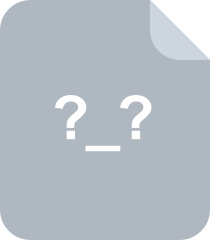
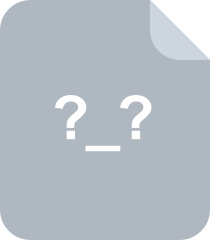
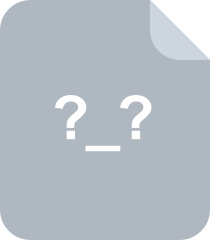
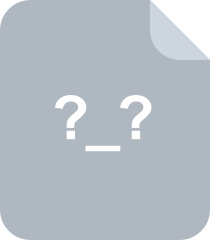
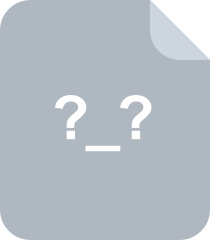
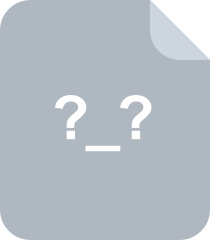
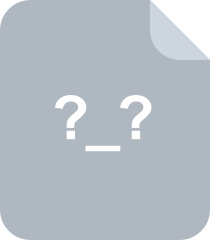
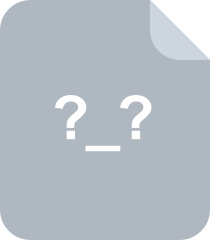
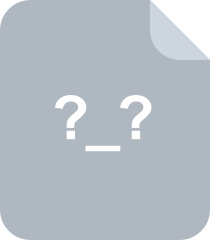
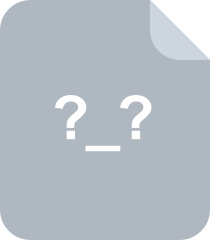
共 172 条
- 1
- 2
资源评论
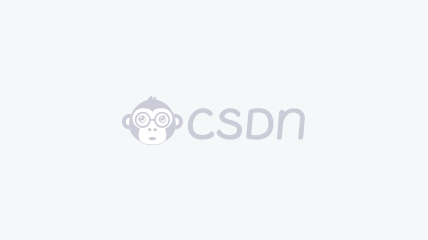

nokia19860924
- 粉丝: 1
- 资源: 3
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

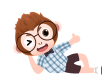
最新资源
- 网上书城系统(Struts+Hibernate+Mysql).rar
- 网上书店(struts+hibernate+css+mysql).rar
- 网上书店系统(论文+jsp源程序)130220.rar
- 网上书店系统(论文+jsp源程序).rar
- 网上书店(struts+hibernate+css+mysql)130223.rar
- 系统详细配置方法.rar
- 文本编辑器.rar
- 项目申报系统(Struts2+Spring+Hibernate+Jsp+Mysql5).rar
- 纯电动汽车再生制动策略,Cruise和Simulink联合仿真,提供Cruise整车模型和simuink策略模型,有详细解析文档,可运行
- 学生成绩管理系统(SSH+MYSQL)130221.rar
- 学生成绩管理系统(SSH+MYSQL).rar
- 项目申报系统(Struts2+Spring+Hibernate+Jsp+Mysql5)130223.rar
- 移动ssh项目(struts+spring+hibernate+oracle).rar
- 阳光酒店管理系统(javaapplet+SQL)130425.rar
- 移动ssh项目(struts+spring+hibernate+oracle)130222.rar
- 音乐网站(JSP+SERVLET)130222.rar
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


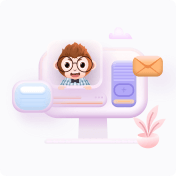
安全验证
文档复制为VIP权益,开通VIP直接复制
