<img width="600" alt="kan_plot" src="https://github.com/KindXiaoming/pykan/assets/23551623/a2d2d225-b4d2-4c1e-823e-bc45c7ea96f9">
# Kolmogorov-Arnold Networks (KANs)
This is the github repo for the paper ["KAN: Kolmogorov-Arnold Networks"](https://arxiv.org/abs/2404.19756) and ["KAN 2.0: Kolmogorov-Arnold Networks Meet Science"](https://arxiv.org/abs/2408.10205). You may want to quickstart with [hellokan](https://github.com/KindXiaoming/pykan/blob/master/hellokan.ipynb), try more examples in [tutorials](https://github.com/KindXiaoming/pykan/tree/master/tutorials), or read the documentation [here](https://kindxiaoming.github.io/pykan/).
Kolmogorov-Arnold Networks (KANs) are promising alternatives of Multi-Layer Perceptrons (MLPs). KANs have strong mathematical foundations just like MLPs: MLPs are based on the universal approximation theorem, while KANs are based on Kolmogorov-Arnold representation theorem. KANs and MLPs are dual: KANs have activation functions on edges, while MLPs have activation functions on nodes. This simple change makes KANs better (sometimes much better!) than MLPs in terms of both model **accuracy** and **interpretability**. A quick intro of KANs [here](https://kindxiaoming.github.io/pykan/intro.html).
## Installation
Pykan can be installed via PyPI or directly from GitHub.
**Pre-requisites:**
```
Python 3.9.7 or higher
pip
```
**For developers**
```
git clone https://github.com/KindXiaoming/pykan.git
cd pykan
pip install -e .
```
**Installation via github**
```
pip install git+https://github.com/KindXiaoming/pykan.git
```
**Installation via PyPI:**
```
pip install pykan
```
Requirements
```python
# python==3.9.7
matplotlib==3.6.2
numpy==1.24.4
scikit_learn==1.1.3
setuptools==65.5.0
sympy==1.11.1
torch==2.2.2
tqdm==4.66.2
```
After activating the virtual environment, you can install specific package requirements as follows:
```python
pip install -r requirements.txt
```
**Optional: Conda Environment Setup**
For those who prefer using Conda:
```
conda create --name pykan-env python=3.9.7
conda activate pykan-env
pip install git+https://github.com/KindXiaoming/pykan.git # For GitHub installation
# or
pip install pykan # For PyPI installation
```
## Computation requirements
Examples in [tutorials](tutorials) are runnable on a single CPU typically less than 10 minutes. All examples in the paper are runnable on a single CPU in less than one day. Training KANs for PDE is the most expensive and may take hours to days on a single CPU. We use CPUs to train our models because we carried out parameter sweeps (both for MLPs and KANs) to obtain Pareto Frontiers. There are thousands of small models which is why we use CPUs rather than GPUs. Admittedly, our problem scales are smaller than typical machine learning tasks, but are typical for science-related tasks. In case the scale of your task is large, it is advisable to use GPUs.
## Documentation
The documentation can be found [here](https://kindxiaoming.github.io/pykan/).
## Tutorials
**Quickstart**
Get started with [hellokan.ipynb](./hellokan.ipynb) notebook.
**More demos**
More Notebook tutorials can be found in [tutorials](tutorials).
## Advice on hyperparameter tuning
Many intuition about MLPs and other networks may not directy transfer to KANs. So how can I tune the hyperparameters effectively? Here is my general advice based on my experience playing with the problems reported in the paper. Since these problems are relatively small-scale and science-oriented, it is likely that my advice is not suitable to your case. But I want to at least share my experience such that users can have better clues where to start and what to expect from tuning hyperparameters.
* Start from a simple setup (small KAN shape, small grid size, small data, no reguralization `lamb=0`). This is very different from MLP literature, where people by default use widths of order `O(10^2)` or higher. For example, if you have a task with 5 inputs and 1 outputs, I would try something as simple as `KAN(width=[5,1,1], grid=3, k=3)`. If it doesn't work, I would gradually first increase width. If that still doesn't work, I would consider increasing depth. You don't need to be this extreme, if you have better understanding about the complexity of your task.
* Once an acceptable performance is achieved, you could then try refining your KAN (more accurate or more interpretable).
* If you care about accuracy, try grid extention technique. An example is [here](https://kindxiaoming.github.io/pykan/Examples/Example_1_function_fitting.html). But watch out for overfitting, see below.
* If you care about interpretability, try sparsifying the network with, e.g., `model.train(lamb=0.01)`. It would also be advisable to try increasing lamb gradually. After training with sparsification, plot it, if you see some neurons that are obvious useless, you may call `pruned_model = model.prune()` to get the pruned model. You can then further train (either to encourage accuracy or encouarge sparsity), or do symbolic regression.
* I also want to emphasize that accuracy and interpretability (and also parameter efficiency) are not necessarily contradictory, e.g., Figure 2.3 in [our paper](https://arxiv.org/pdf/2404.19756). They can be positively correlated in some cases but in other cases may dispaly some tradeoff. So it would be good not to be greedy and aim for one goal at a time. However, if you have a strong reason why you believe pruning (interpretability) can also help accuracy, you may want to plan ahead, such that even if your end goal is accuracy, you want to push interpretability first.
* Once you get a quite good result, try increasing data size and have a final run, which should give you even better results!
Disclaimer: Try the simplest thing first is the mindset of physicists, which could be personal/biased but I find this mindset quite effective and make things well-controlled for me. Also, The reason why I tend to choose a small dataset at first is to get faster feedback in the debugging stage (my initial implementation is slow, after all!). The hidden assumption is that a small dataset behaves qualitatively similar to a large dataset, which is not necessarily true in general, but usually true in small-scale problems that I have tried. To know if your data is sufficient, see the next paragraph.
Another thing that would be good to keep in mind is that please constantly checking if your model is in underfitting or overfitting regime. If there is a large gap between train/test losses, you probably want to increase data or reduce model (`grid` is more important than `width`, so first try decreasing `grid`, then `width`). This is also the reason why I'd love to start from simple models to make sure that the model is first in underfitting regime and then gradually expands to the "Goldilocks zone".
## Citation
```python
@article{liu2024kan,
title={KAN: Kolmogorov-Arnold Networks},
author={Liu, Ziming and Wang, Yixuan and Vaidya, Sachin and Ruehle, Fabian and Halverson, James and Solja{\v{c}}i{\'c}, Marin and Hou, Thomas Y and Tegmark, Max},
journal={arXiv preprint arXiv:2404.19756},
year={2024}
}
```
## Contact
If you have any questions, please contact zmliu@mit.edu
## Author's note
I would like to thank everyone who's interested in KANs. When I designed KANs and wrote codes, I have math & physics examples (which are quite small scale!) in mind, so did not consider much optimization in efficiency or reusability. It's so honored to receive this unwarranted attention, which is way beyond my expectation. So I accept any criticism from people complaning about the efficiency and resuability of the codes, my apology. My only hope is that you find `model.plot()` fun to play with :).
For users who are interested in scientific discoveries and scientific computing (the orginal users intended for), I'm happy to hear your applications and collaborate. This repo will continue remaining mostly for this purpose, p
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
KAN: Kolmogorov-Arnold神经网络,通过引入可学习的激活函数和无线性权重的设计,克服了传统神经网络在处理复杂数据时的局限性。其在准确性、参数效率和可解释性等方面的优势,使其成为对多层感知器(MLP)的有力替代方案,为深度学习模型的进一步发展提供了新的方向和机会。KAN 网络不仅在机器学习领域具有广泛的应用潜力,还能够为科学研究提供重要的支持,促进数学和物理等领域的发现与创新。适用于科研工作者,学生,论文创新点研究,数据拟合,分类领域研究。
资源推荐
资源详情
资源评论
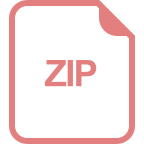
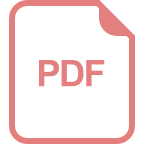
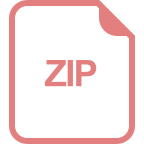
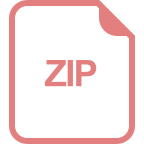
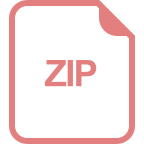
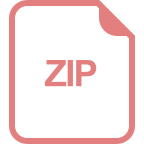
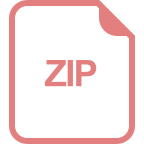
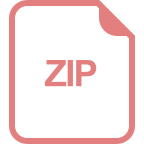
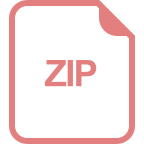
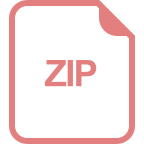
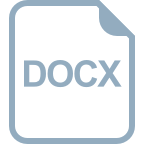
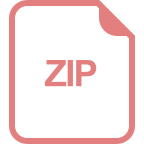
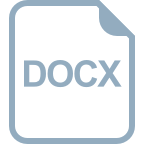
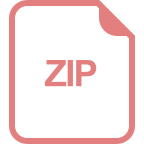
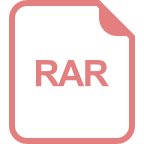
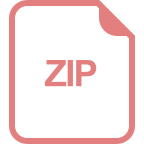
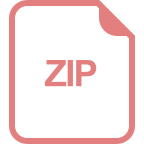
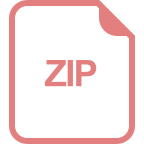
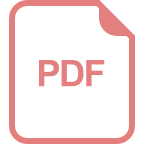
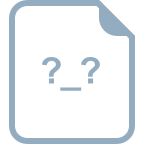
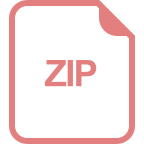
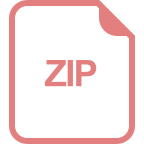
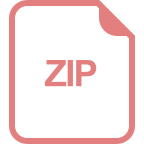
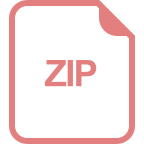
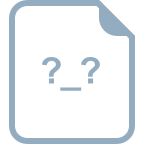
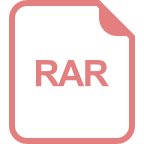
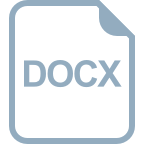
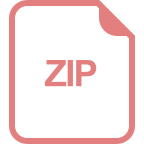
收起资源包目录

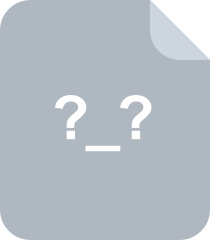
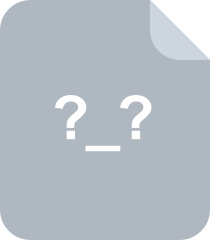
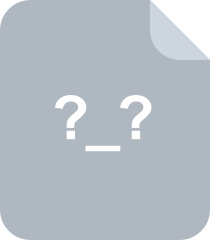
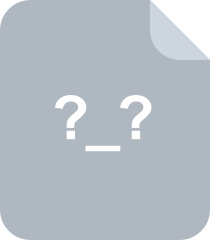
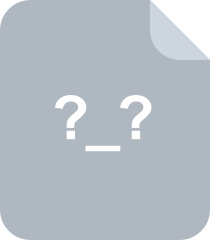
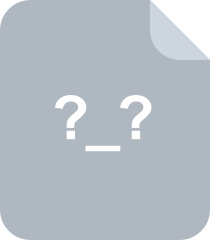
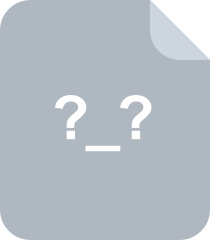
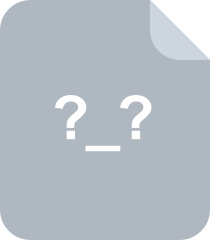
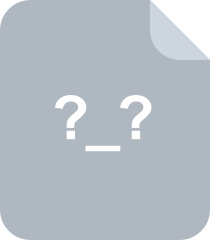
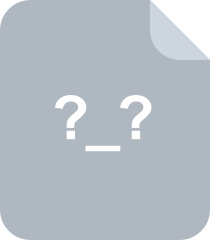
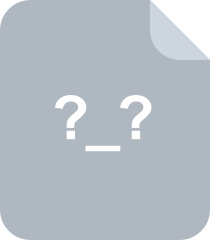
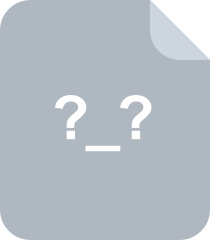
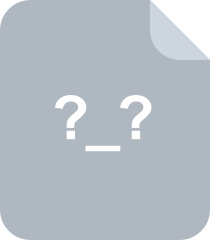
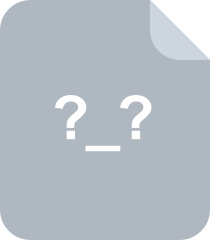
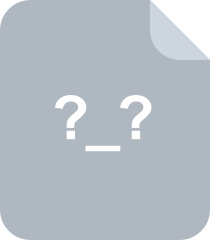
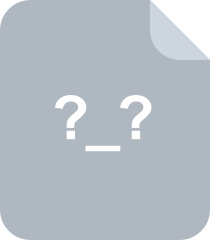
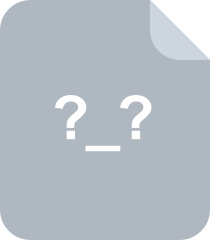
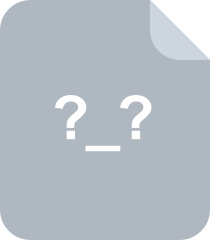
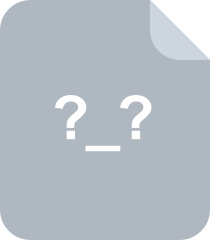
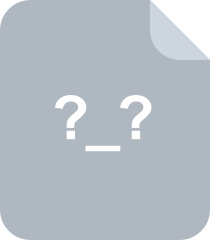
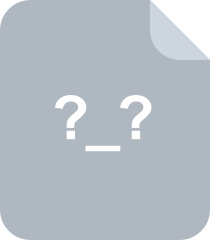
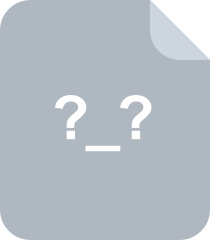
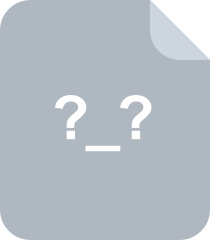
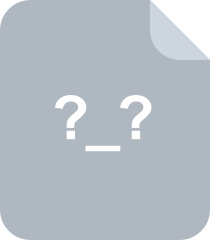
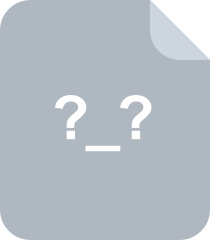
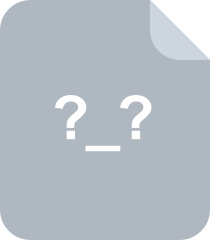
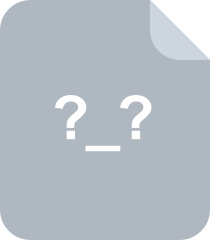
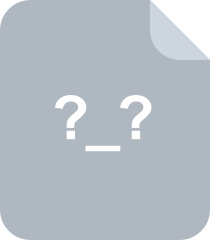
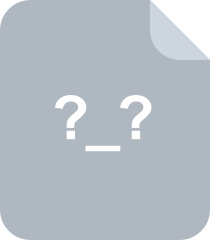
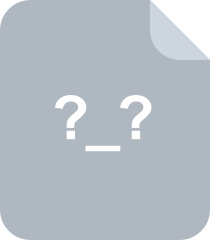
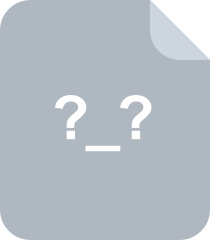
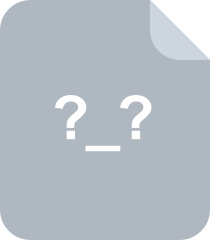
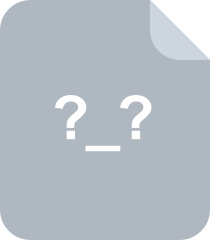
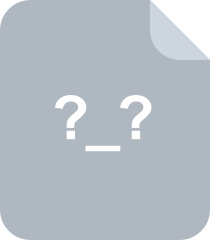
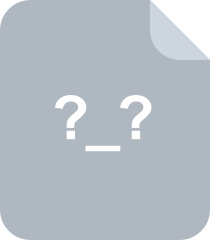
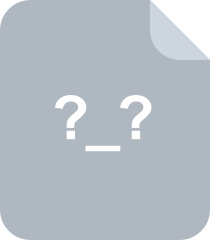
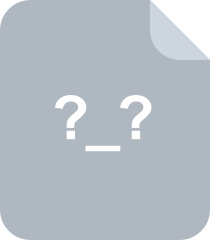
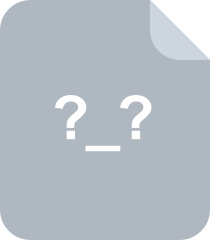
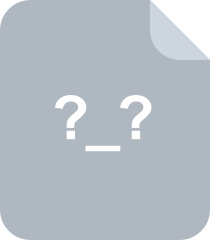
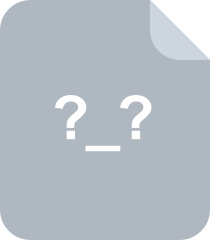
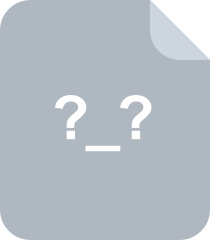
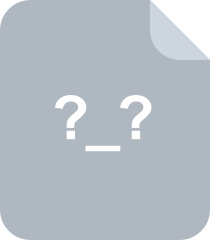
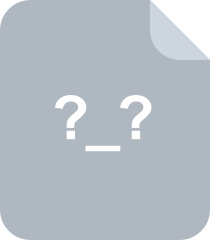
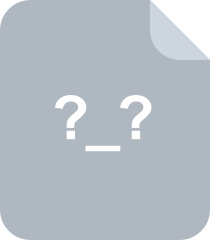
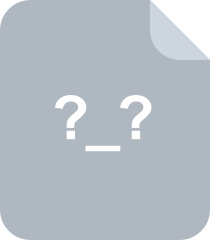
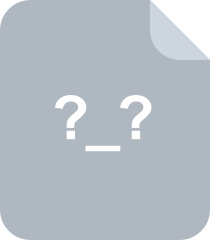
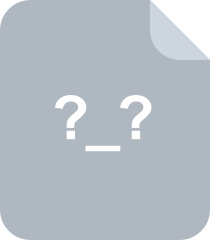
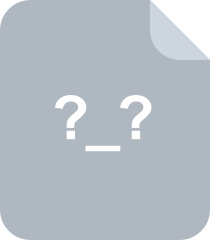
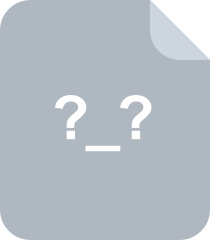
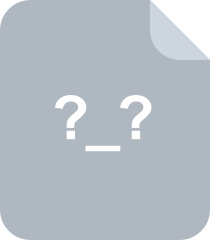
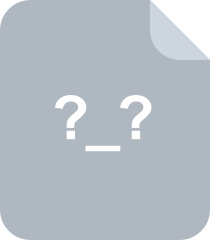
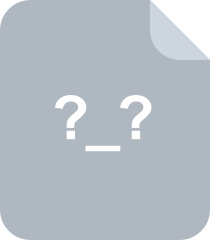
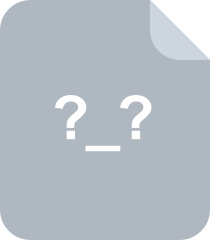
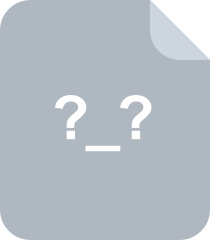
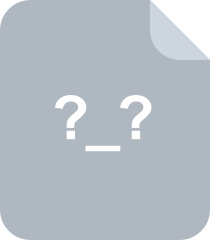
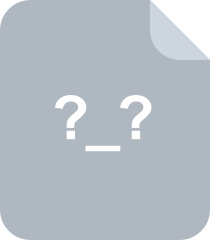
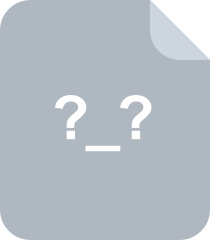
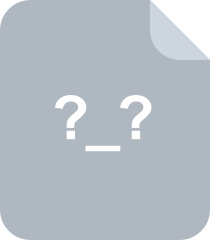
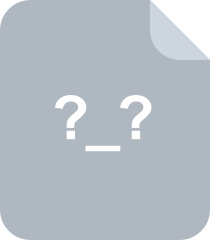
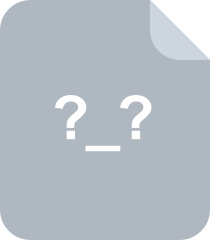
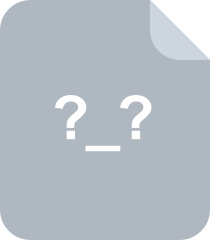
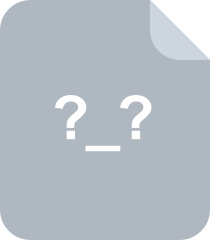
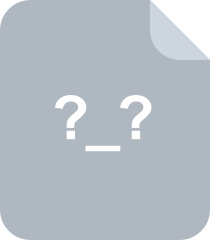
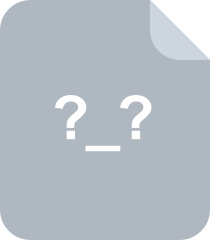
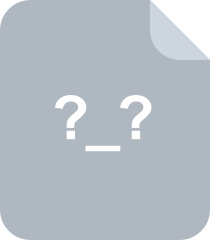
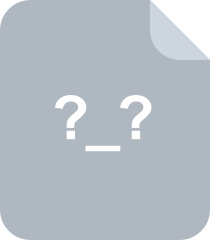
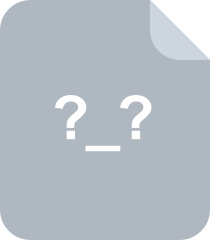
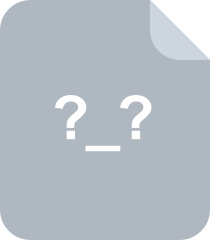
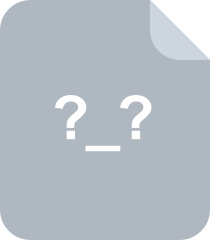
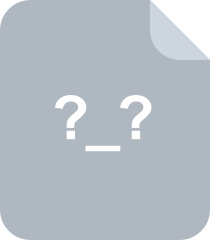
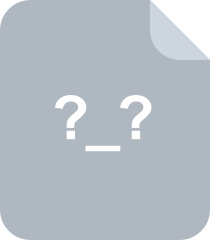
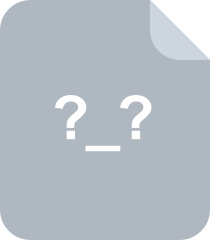
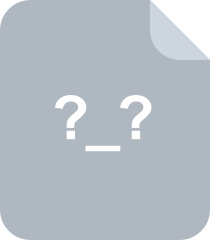
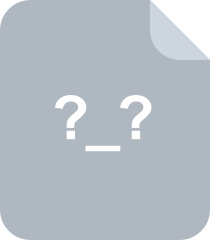
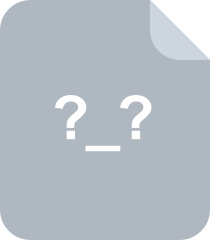
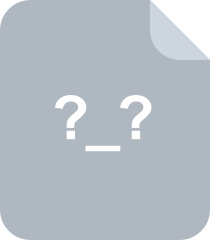
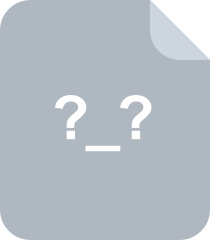
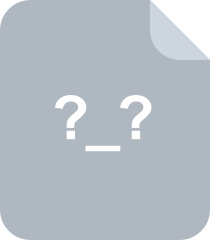
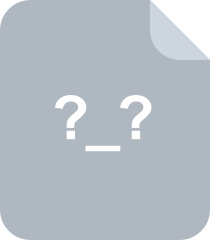
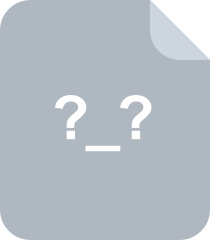
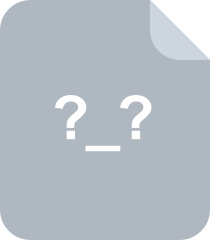
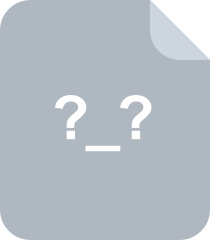
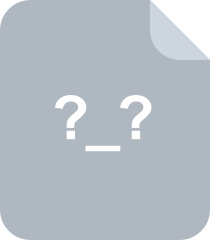
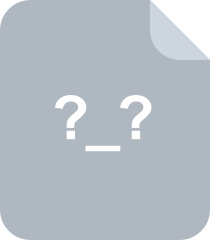
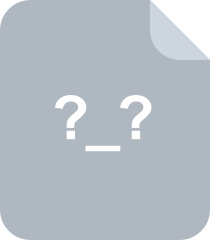
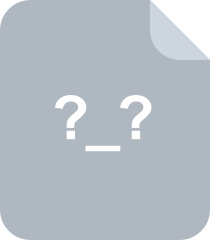
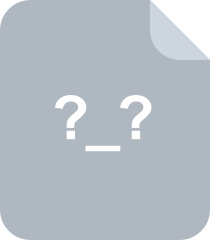
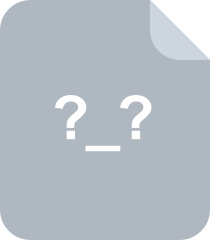
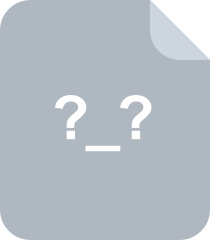
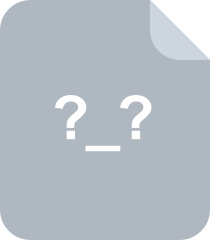
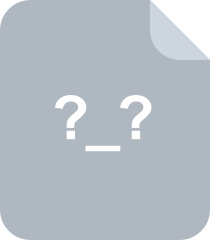
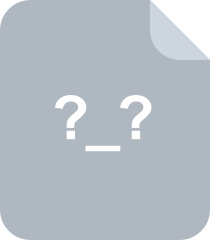
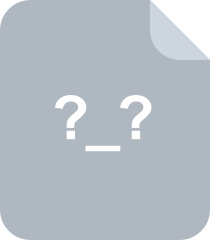
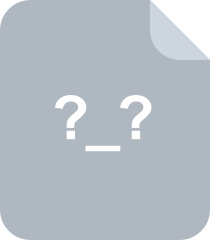
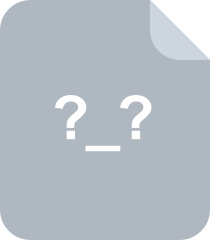
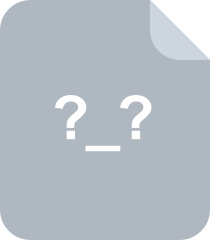
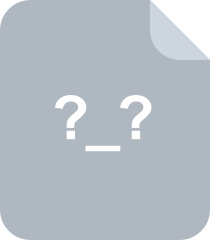
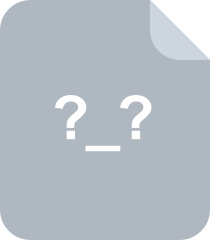
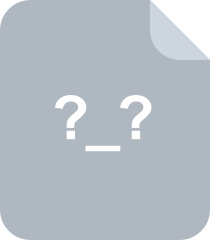
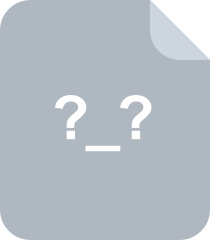
共 461 条
- 1
- 2
- 3
- 4
- 5
资源评论
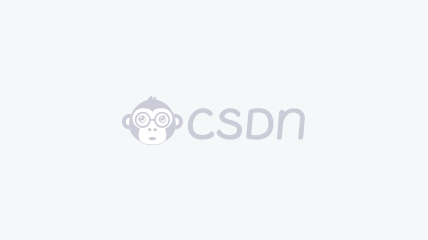

呆头鹅AI工作室
- 粉丝: 218
- 资源: 6
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

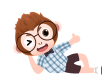
最新资源
- 毕设&课程作业_基于C#的实现宿舍管理系统.zip
- 毕设&课程作业_基于C#的人事工资管理系统.zip
- 毕设&课程作业_基于C#的聊天系统.zip
- 毕设&课程作业_基于C#的一套浏览器系统.zip
- 毕设&课程作业_基于C#的wpf 选课系统 无数据库版本.zip
- 毕设&课程作业_基于C#的请假管理系统 C#.zip
- 毕设&课程作业_基于C#的实现的影院售票系统。.zip
- 毕设&课程作业_基于C#的实现的宿舍管理系统.zip
- 毕设&课程作业_基于C#的体操赛事管理系统。.zip
- 毕设&课程作业_基于C#的图书馆管理系统.zip
- 毕设&课程作业_基于C#的WPF 个人记账系统。.zip
- 毕设&课程作业_基于C#的部门信息管理系统c# mysql.zip
- 毕设&课程作业_基于C#的和SQL-Server实现简易的选课系统.zip
- 毕设&课程作业_基于C#的公寓管理系统.zip
- 毕设&课程作业_基于C#的三层架构图书管理系统.zip
- 毕设&课程作业_基于C#的使用.net asp 和 sql server 使用c#语言开发的学生档案管理系统.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


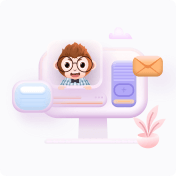
安全验证
文档复制为VIP权益,开通VIP直接复制
