没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
THIS book covers the Java™ Native Interface (JNI). It will be useful to you if you are interested in any of the following: • integrating a Java application with legacy code written in languages such as C or C++ • incorporating a Java virtual machine implementation into an existing application written in languages such as C or C++ • implementing a Java virtual machine • understanding the technical issues in language interoperability, in particular how to handle features such as garbage collection and multithreading
资源推荐
资源详情
资源评论
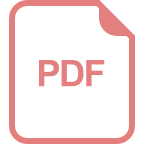
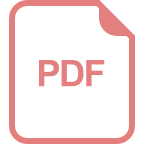
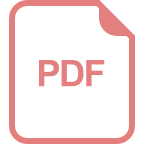
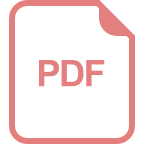
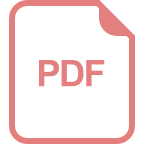
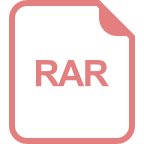
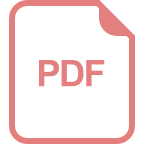
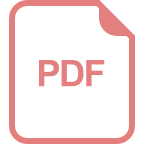
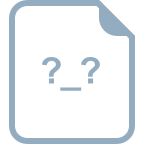
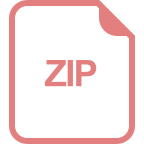
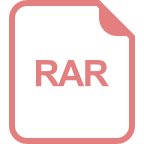
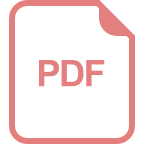
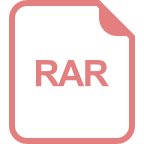
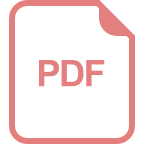
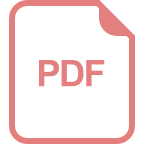
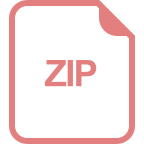
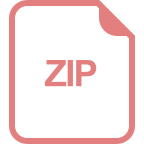
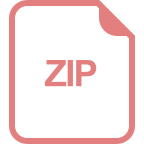
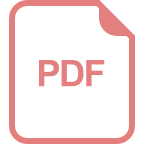
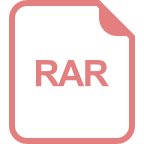
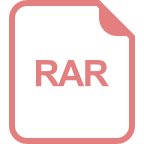
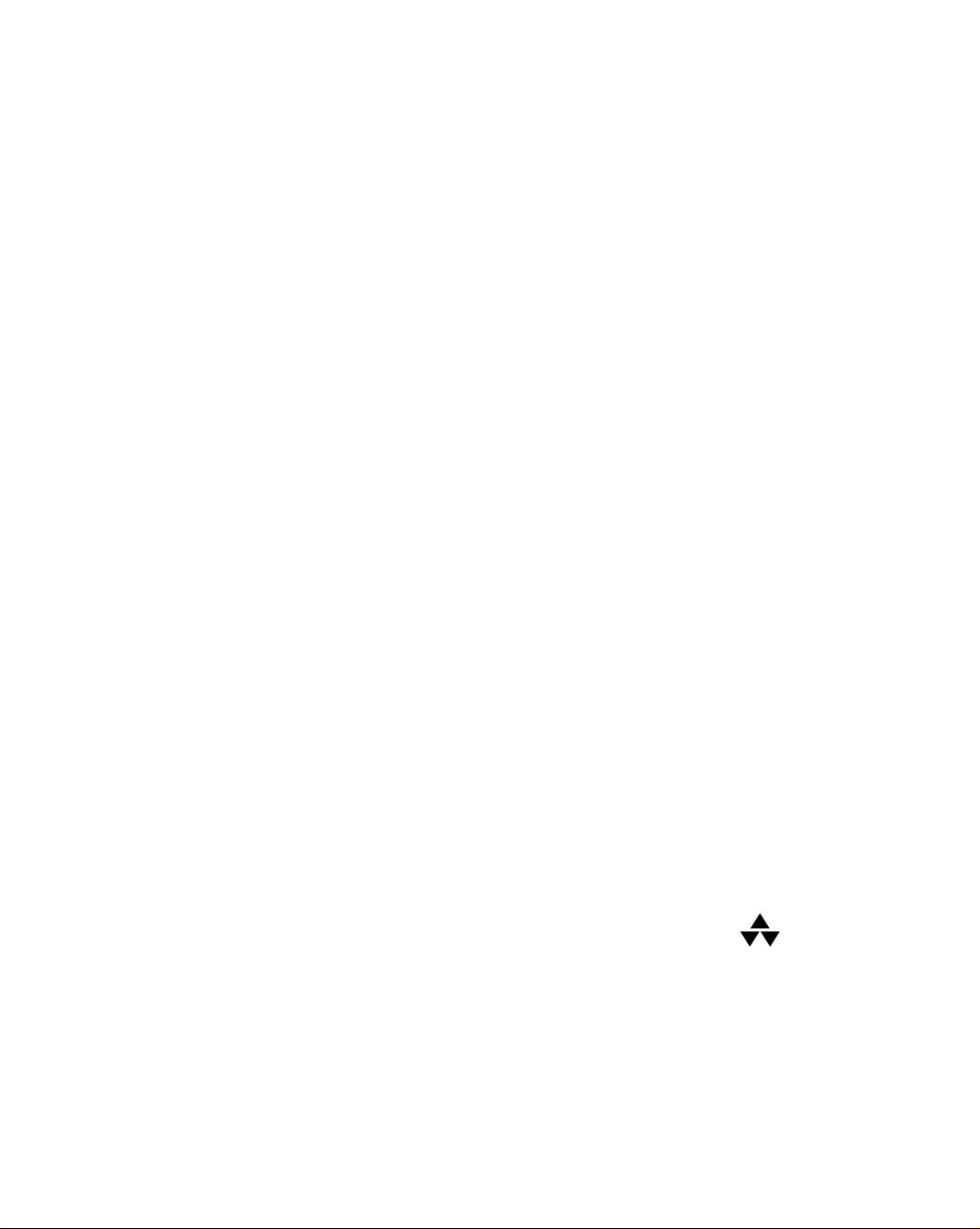
The Java
™
Native
Interface
Programmer’s Guide and Specification
Sheng Liang
ADDISON-WESLEY
An imprint of Addison Wesley Longman, Inc.
Reading, Massachusetts • Harlow, England • Menlo Park, California
Berkeley, California • Don Mills, Ontario • Sydney
Bonn • Amsterdam • Tokyo • Mexico City
jni.book Page 3 Thursday, February 21, 2002 4:36 PM
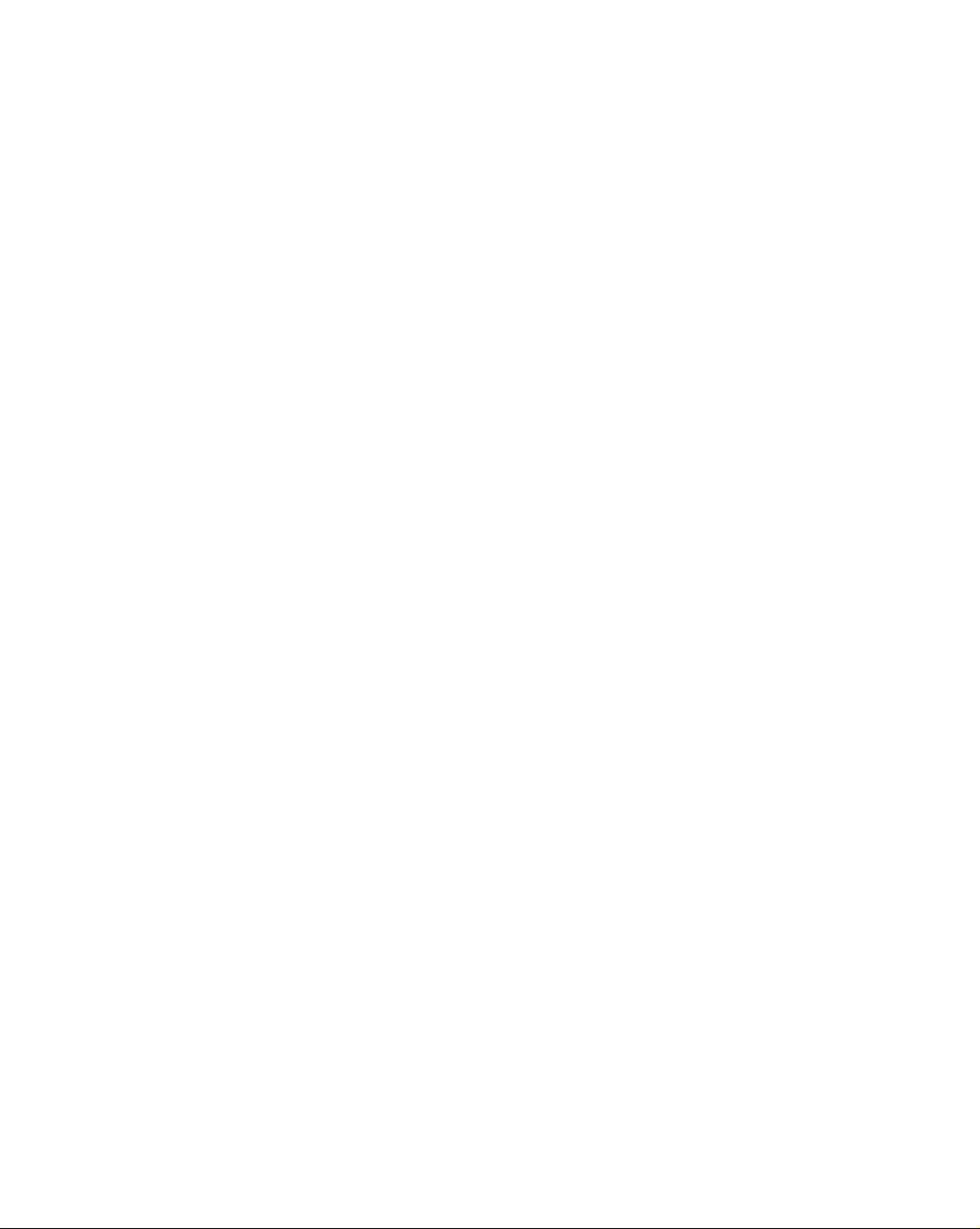
Copyright © 1999 Sun Microsystems, Inc.
901 San Antonio Road, Palo Alto, CA 94303 USA.
All rights reserved.
Duke™ designed by Joe Palrang.
Sun Microsystems, Inc. has intellectual property rights relating to implementations of the technology
described in this publication. In particular, and without limitation, these intellectual property rights
may include one or more U.S. patents, foreign patents, or pending applications. Sun, Sun
Microsystems, the Sun logo, and all Sun, Java, Jini, and Solaris based trademarks and logos are
trademarks or registered trademarks of Sun Microsystems, Inc. in the United States and other
countries. UNIX is a registered trademark in the United States and other countries, exclusively licensed
through X/Open Company, Ltd.
Sun Microsystems, Inc. (SUN) hereby grants to you a fully paid, nonexclusive, nontransferable,
perpetual, worldwide limited license (without the right to sublicense) under SUN’s intellectual
property rights that are essential to practice this specification. This license allows and is limited to the
creation and distribution of clean room implementations of this specification that: (i) include a
complete implementation of the current version of this specification without subsetting or supersetting;
(ii) implement all the required interfaces and functionality of the Java™ 2 Platform, Standard Edition,
as defined by SUN, without subsetting or supersetting; (iii) do not add any additional packages,
classes, or interfaces to the java.* or javax.* packages or their subpackages; (iv) pass all test suites
relating to the most recent published version of the specification of the Java™ 2 Platform, Standard
Edition, that are available from SUN six (6) months prior to any beta release of the clean room
implementation or upgrade thereto; (v) do not derive from SUN source code or binary materials; and
(vi) do not include any SUN source code or binary materials without an appropriate and separate
license from SUN.
U.S. GOVERNMENT USE: This specification relates to commercial items, processes, or software.
Accordingly, use by the United States Government is subject to these terms and conditions, consistent
with FAR 12.211 and 12.212.
THIS PUBLICATION IS PROVIDED “AS IS” WITHOUT WARRANTY OF ANY KIND, EITHER
EXPRESS OR IMPLIED, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES
OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE, OR NON-INFRINGE-
MENT.
THIS PUBLICATION COULD INCLUDE TECHNICAL INACCURACIES OR TYPOGRAPHI-
CAL ERRORS. CHANGES ARE PERIODICALLY ADDED TO THE INFORMATION HEREIN;
THESE CHANGES WILL BE INCORPORATED IN NEW EDITIONS OF THE PUBLICATION.
SUN MICROSYSTEMS, INC. MAY MAKE IMPROVEMENTS AND/OR CHANGES IN ANY
TECHNOLOGY, PRODUCT, OR PROGRAM DESCRIBED IN THIS PUBLICATION AT ANY
TIME.
The publisher offers discounts on this book when ordered in quantity for special sales. For more
information, please contact the Corporate, Government, and Special Sales Group, CEPUB, Addison
Wesley Longman, Inc., One Jacob Way, Reading, Massachusetts 01867.
ISBN 0-201-32577-2
1 2 3 4 5 6 7 8 9-MA-0302010099
First printing, June 1999
jni.book Page 4 Thursday, February 21, 2002 4:36 PM
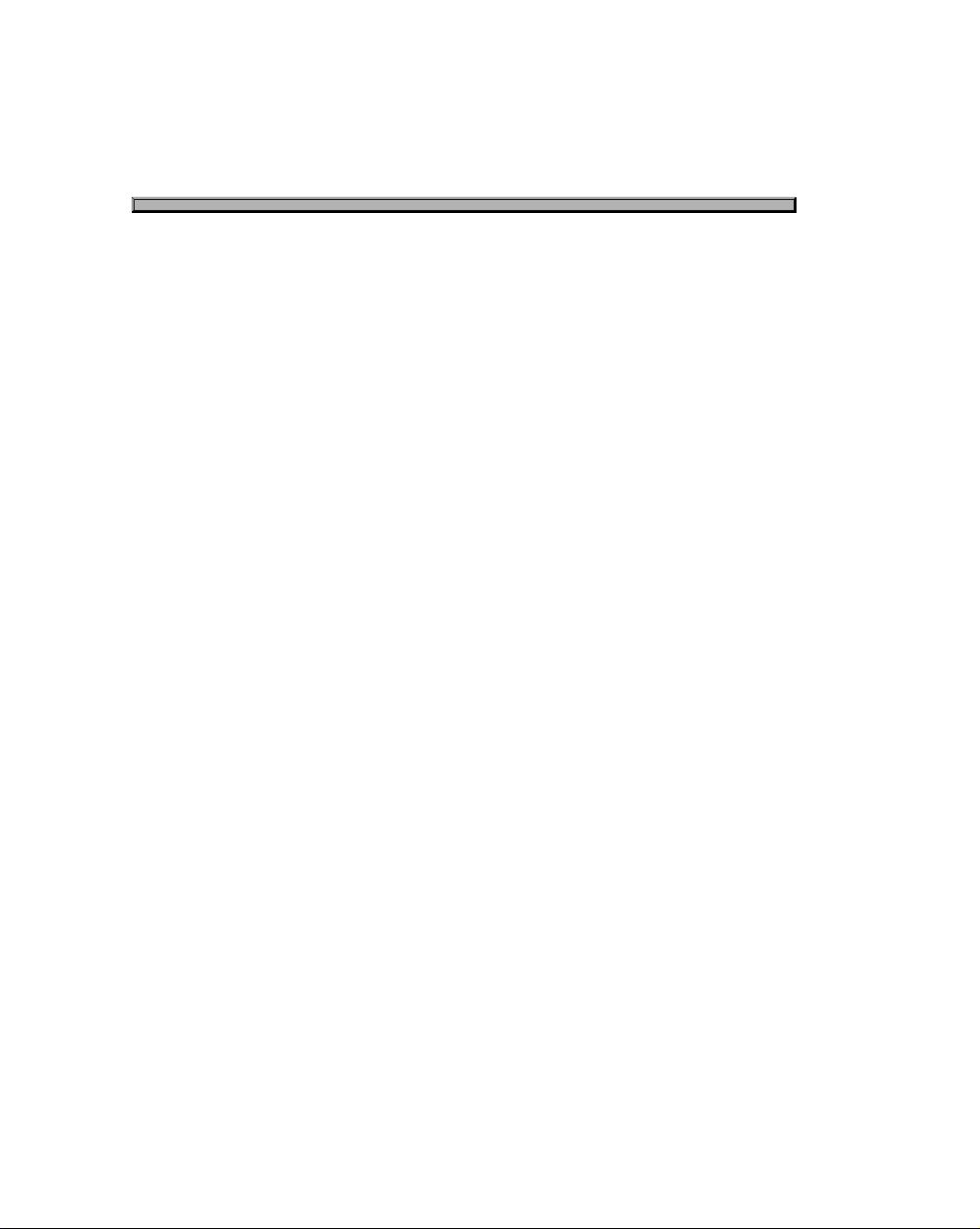
vii
Contents
Preface . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . xiii
Part One: Introduction and Tutorial
1
Introduction. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 3
1.1 The Java Platform and Host Environment . . . . . . . . . . . . . . . . . . . . . . . . . . 4
1.2 Role of the JNI . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 4
1.3 Implications of Using the JNI. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 6
1.4 When to Use the JNI. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 6
1.5 Evolution of the JNI . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 7
1.6 Example Programs . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 8
2 Getting Started . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 11
2.1 Overview . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 11
2.2 Declare the Native Method. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 13
2.3 Compile the HelloWorld Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 14
2.4 Create the Native Method Header File. . . . . . . . . . . . . . . . . . . . . . . . . . . . 14
2.5 Write the Native Method Implementation . . . . . . . . . . . . . . . . . . . . . . . . . 15
2.6 Compile the C Source and Create a Native Library . . . . . . . . . . . . . . . . . . 15
2.7 Run the Program. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 16
Part Two: Programmer’s Guide
3
Basic Types, Strings, and Arrays. . . . . . . . . . . . . . . . . . . . . . . . . . 21
3.1 A Simple Native Method . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 21
3.1.1 C Prototype for Implementing the Native Method. . . . . . . . . . . . 22
3.1.2 Native Method Arguments. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 22
3.1.3 Mapping of Types . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 23
3.2 Accessing Strings . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 24
3.2.1 Converting to Native Strings . . . . . . . . . . . . . . . . . . . . . . . . . . . . 24
3.2.2 Freeing Native String Resources . . . . . . . . . . . . . . . . . . . . . . . . . 25
3.2.3 Constructing New Strings . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 26
3.2.4 Other JNI String Functions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 26
3.2.5 New JNI String Functions in Java 2 SDK Release 1.2 . . . . . . . . 27
jni.book Page vii Thursday, February 21, 2002 4:36 PM
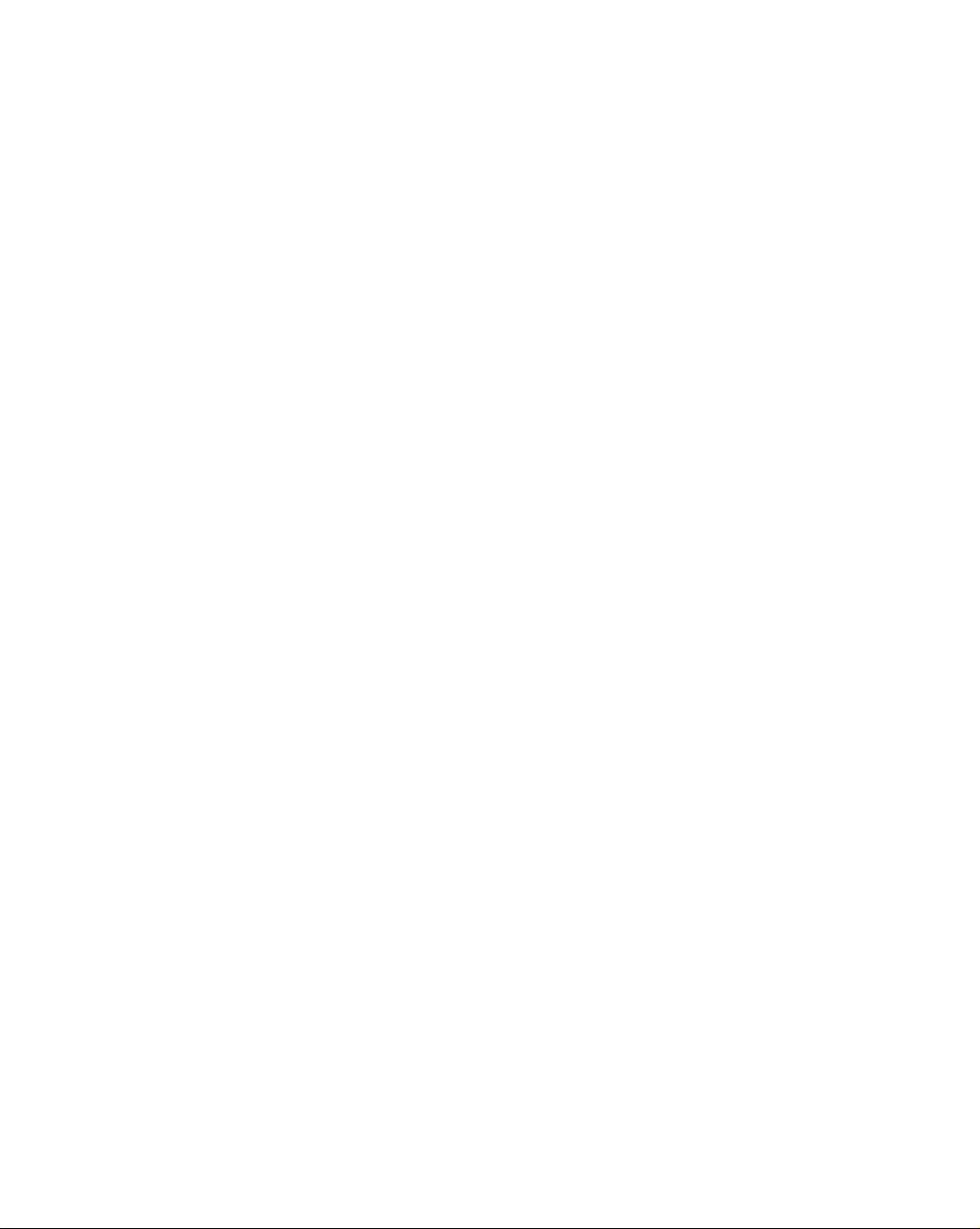
CONTENTS
viii
3.2.6 Summary of JNI String Functions. . . . . . . . . . . . . . . . . . . . . . . . 29
3.2.7 Choosing among the String Functions . . . . . . . . . . . . . . . . . . . . 31
3.3 Accessing Arrays. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 33
3.3.1 Accessing Arrays in C. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 34
3.3.2 Accessing Arrays of Primitive Types . . . . . . . . . . . . . . . . . . . . . 34
3.3.3 Summary of JNI Primitive Array Functions . . . . . . . . . . . . . . . . 35
3.3.4 Choosing among the Primitive Array Functions. . . . . . . . . . . . . 36
3.3.5 Accessing Arrays of Objects. . . . . . . . . . . . . . . . . . . . . . . . . . . . 38
4 Fields and Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 41
4.1 Accessing Fields . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 41
4.1.1 Procedure for Accessing an Instance Field . . . . . . . . . . . . . . . . . 43
4.1.2 Field Descriptors . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 44
4.1.3 Accessing Static Fields . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 44
4.2 Calling Methods. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 46
4.2.1 Calling Instance Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 47
4.2.2 Forming the Method Descriptor . . . . . . . . . . . . . . . . . . . . . . . . . 48
4.2.3 Calling Static Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 49
4.2.4 Calling Instance Methods of a Superclass. . . . . . . . . . . . . . . . . . 51
4.3 Invoking Constructors . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 51
4.4 Caching Field and Method IDs . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 53
4.4.1 Caching at the Point of Use. . . . . . . . . . . . . . . . . . . . . . . . . . . . . 53
4.4.2 Caching in the Defining Class’s Initializer . . . . . . . . . . . . . . . . . 56
4.4.3 Comparison between the Two Approaches to Caching IDs . . . . 57
4.5 Performance of JNI Field and Method Operations . . . . . . . . . . . . . . . . . . 58
5 Local and Global References . . . . . . . . . . . . . . . . . . . . . . . . . . . . 61
5.1 Local and Global References. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 61
5.1.1 Local References. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 62
5.1.2 Global References . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 64
5.1.3 Weak Global References . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 65
5.1.4 Comparing References . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 66
5.2 Freeing References . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 66
5.2.1 Freeing Local References . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 67
5.2.2 Managing Local References in Java 2 SDK Release 1.2 . . . . . . 68
5.2.3 Freeing Global References . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 69
5.3 Rules for Managing References. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 70
6 Exceptions. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 73
6.1 Overview . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 73
6.1.1 Caching and Throwing Exceptions in Native Code . . . . . . . . . . 73
6.1.2 A Utility Function . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 75
6.2 Proper Exception Handling . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 76
6.2.1 Checking for Exceptions. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 76
6.2.2 Handling Exceptions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 78
6.2.3 Exceptions in Utility Functions. . . . . . . . . . . . . . . . . . . . . . . . . . 79
jni.book Page viii Thursday, February 21, 2002 4:36 PM
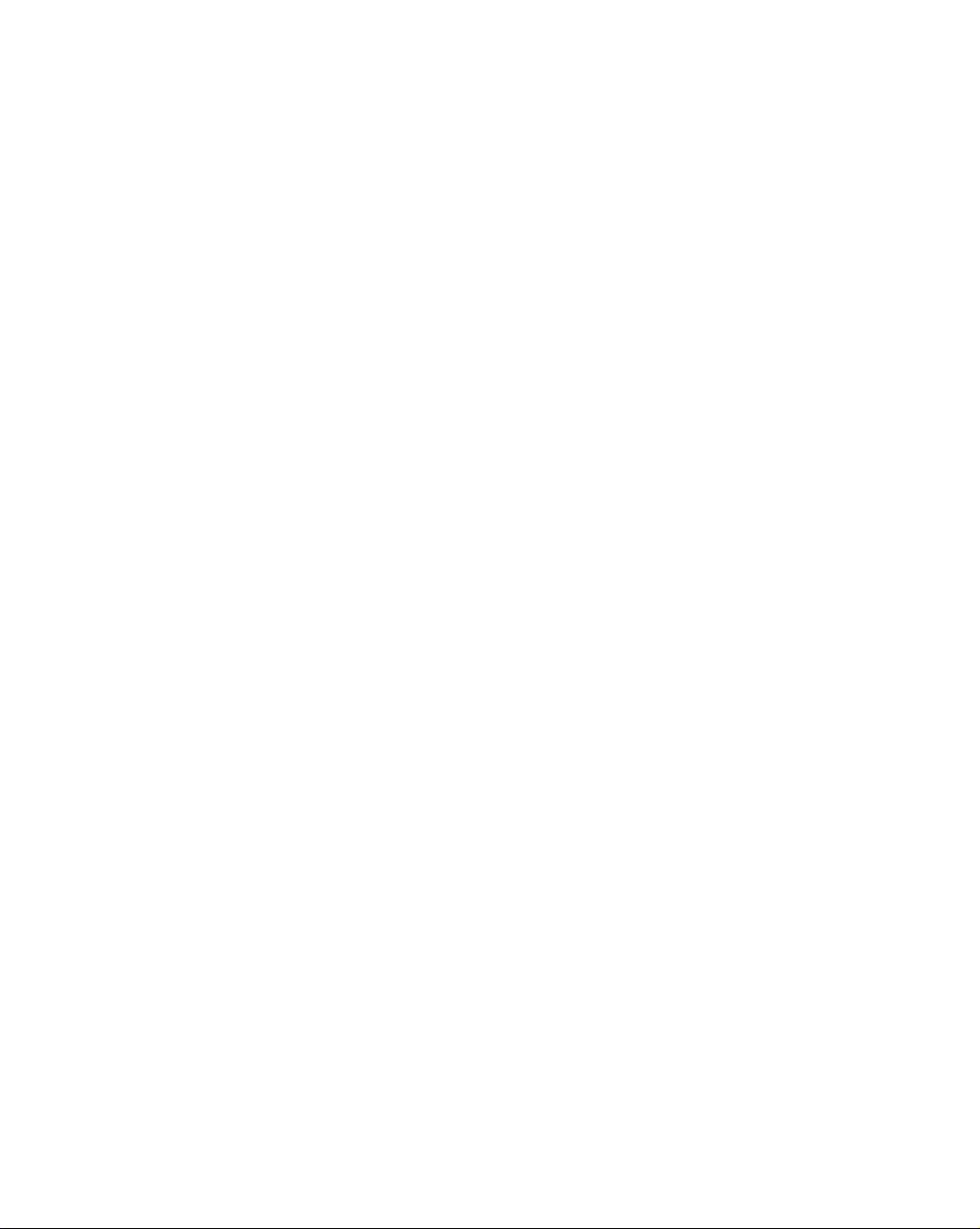
CONTENTS
ix
7 The Invocation Interface . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 83
7.1 Creating the Java Virtual Machine . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 83
7.2 Linking Native Applications with the Java Virtual Machine . . . . . . . . . . . 86
7.2.1 Linking with a Known Java Virtual Machine . . . . . . . . . . . . . . . 86
7.2.2 Linking with Unknown Java Virtual Machines . . . . . . . . . . . . . . 87
7.3 Attaching Native Threads. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 89
8 Additional JNI Features. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 93
8.1 JNI and Threads . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 93
8.1.1 Constraints . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 93
8.1.2 Monitor Entry and Exit . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 94
8.1.3 Monitor Wait and Notify . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 95
8.1.4 Obtaining a JNIEnv Pointer in Arbitrary Contexts. . . . . . . . . . . 96
8.1.5 Matching the Thread Models . . . . . . . . . . . . . . . . . . . . . . . . . . . . 97
8.2 Writing Internationalized Code . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 99
8.2.1 Creating jstrings from Native Strings . . . . . . . . . . . . . . . . . . . 99
8.2.2 Translating jstrings to Native Strings . . . . . . . . . . . . . . . . . . 100
8.3 Registering Native Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 101
8.4 Load and Unload Handlers. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 102
8.4.1 The JNI_OnLoad Handler. . . . . . . . . . . . . . . . . . . . . . . . . . . . . 102
8.4.2 The JNI_OnUnload Handler. . . . . . . . . . . . . . . . . . . . . . . . . . . 104
8.5 Reflection Support . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 105
8.6 JNI Programming in C++. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 106
9 Leveraging Existing Native Libraries . . . . . . . . . . . . . . . . . . . . . 109
9.1 One-to-One Mapping . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 109
9.2 Shared Stubs. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 113
9.3 One-to-One Mapping versus Shared Stubs . . . . . . . . . . . . . . . . . . . . . . . 116
9.4 Implementation of Shared Stubs . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 116
9.4.1 The CPointer Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 117
9.4.2 The CMalloc Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 117
9.4.3 The CFunction Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 118
9.5 Peer Classes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 123
9.5.1 Peer Classes in the Java Platform. . . . . . . . . . . . . . . . . . . . . . . . 124
9.5.2 Freeing Native Data Structures . . . . . . . . . . . . . . . . . . . . . . . . . 125
9.5.3 Backpointers to Peer Instances . . . . . . . . . . . . . . . . . . . . . . . . . 127
10 Traps and Pitfalls . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 131
10.1 Error Checking . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 131
10.2 Passing Invalid Arguments to JNI Functions . . . . . . . . . . . . . . . . . . . . . . 131
10.3 Confusing jclass with jobject . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 132
10.4 Truncating jboolean Arguments . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 132
10.5 Boundaries between Java Application and Native Code . . . . . . . . . . . . . 133
10.6 Confusing IDs with References . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 134
10.7 Caching Field and Method IDs. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 135
10.8 Terminating Unicode Strings . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 137
jni.book Page ix Thursday, February 21, 2002 4:36 PM
剩余312页未读,继续阅读
资源评论
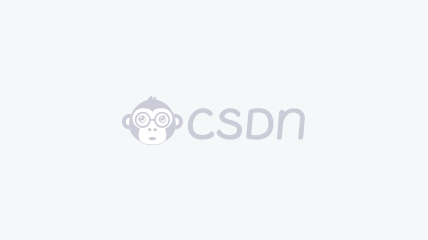

nn123456789
- 粉丝: 14
- 资源: 128
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

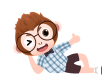
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


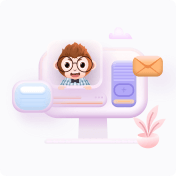
安全验证
文档复制为VIP权益,开通VIP直接复制
