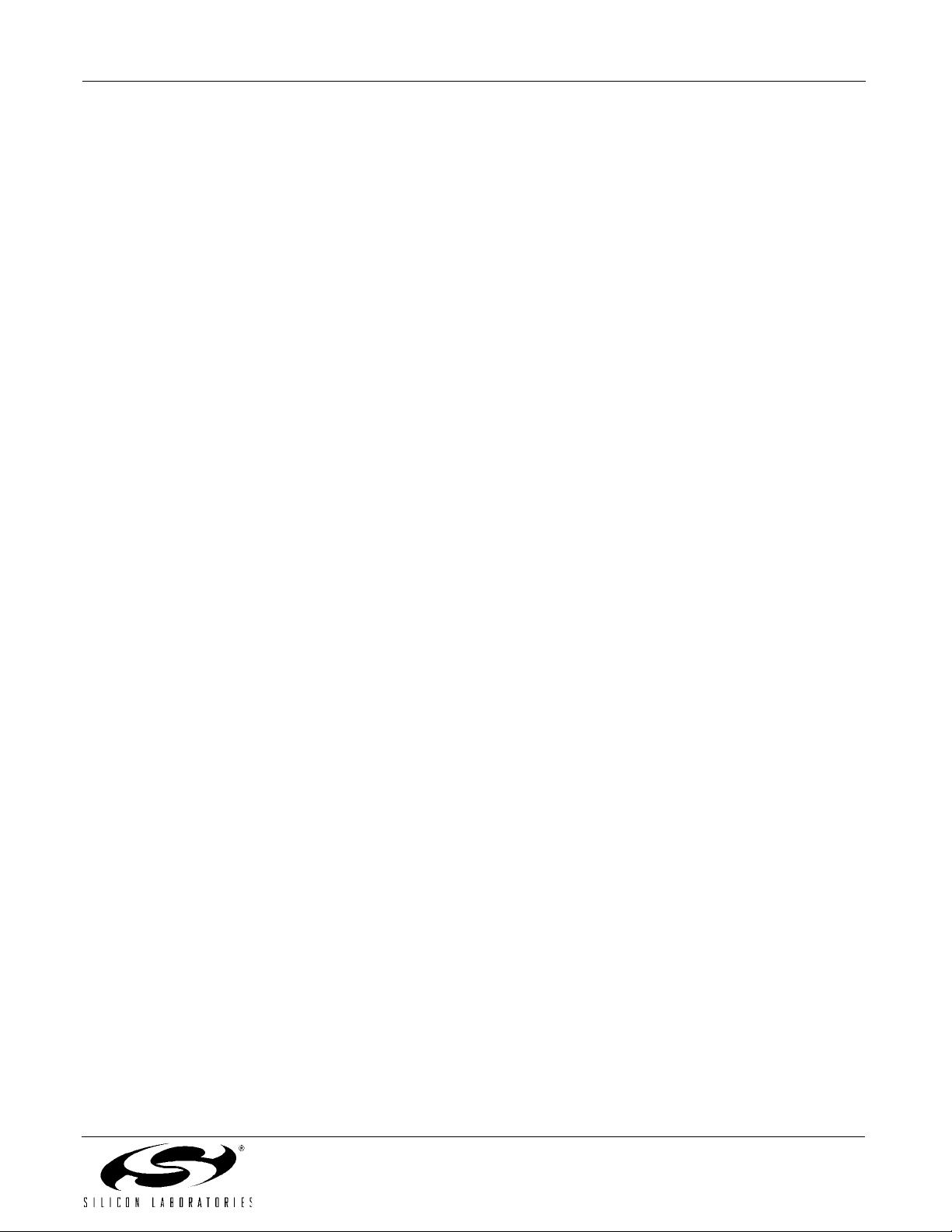
AN128
Rev. 1.1 5
Using the Functions
To use one of the example SPI functions in a ‘C’
program, the file containing the function must first
be assembled or compiled. The resulting object file
then can be added to the build list for the project
and linked to the host (calling) software.
The host software must correctly configure the
GPIO pins of the C8051F30x device to the desired
functionality. See “Hardware Interface” on page 1.
A function prototype for the SPI_Transfer( ) func-
tion also needs to be declared within all files that
call the routine. The ‘C’ prototype for all of the
example functions is:
extern char SPI_Transfer(char);
The “extern” modifier tells the linker that the func-
tion itself will be defined in a separate object file.
The functions can be called with the line:
in_spi = SPI_Transfer(out_spi);
where in_spi and out_spi are variables of type char
that are used for the incoming and outgoing SPI
bytes, respectively.
Sample Usage Code
Two full ‘C’ programs are included that demon-
strate the usage of the SPI routines. The first exam-
ple program, “SPI_F300_Test.c”, demonstrates the
method used to call the SPI routine. The second
example, “SPI_EE_F30x.c”, implements a serial
EEPROM interface using the Mode 0 or Mode 3
SPI routines.
SPI_F300_Test.c
In the file “SPI_F300_Test.c”, a for loop is estab-
lished which counts up from 0 to 255 repeatedly.
The for loop variable test_counter is used as the
outgoing SPI byte, while the SPI_return variable is
the incoming SPI byte. The NSS signal is pulled
low immediately before the function call to select
the slave device, and is pulled high after the func-
tion call to de-select it. After the SPI data transfer,
both the outgoing and incoming SPI bytes are
transmitted to the UART, where the progress can be
viewed from a PC terminal program.
To test the sample code, the files
“SPI_F300_Test.c”, “SPI_defs.h”, and one of the
example function files should be placed in a single
directory. “SPI_defs.h” contains the sbit declara-
tions for the four pins used in the SPI implementa-
tion. The file containing SPI_Transfer( ) and the
“SPI_F300_Test.c” file should be compiled or
assembled separately and included in the build.
Once the code has been programmed into the
FLASH on a C8051F30x device, the MISO and
MOSI pins of the device can be tied together to
verify that what is being shifted out is also being
shifted back in. With a PC terminal (configured for
115,200 Baud, 8 Data Bits, No Parity, 1 Stop Bit,
No Flow Control) connected through an RS-232
level translator to the UART, the terminal program
should display that the numbers being sent out on
MOSI are the same as what is being received on
MISO. A section of the resulting output from this
configuration looks like:
SPI Out = 0xFC, SPI In = 0xFC
SPI Out = 0xFD, SPI In = 0xFD
SPI Out = 0xFE, SPI In = 0xFE
SPI Out = 0xFF, SPI In = 0xFF
SPI Out = 0x00, SPI In = 0x00
SPI Out = 0x01, SPI In = 0x01
SPI Out = 0x02, SPI In = 0x02
SPI Out = 0x03, SPI In = 0x03
SPI Out = 0x04, SPI In = 0x04
SPI Out = 0x05, SPI In = 0x05
Tying the MOSI and MISO pins together does not
completely test the functionality of the SPI imple-
- 1
- 2
前往页