/**
* @copyright
* ====================================================================
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
* ====================================================================
* @endcopyright
*/
package org.apache.subversion.javahl;
import org.apache.subversion.javahl.callback.*;
import org.apache.subversion.javahl.types.*;
import java.io.File;
import java.io.FileOutputStream;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
import java.io.PrintWriter;
import java.io.ByteArrayOutputStream;
import java.text.ParseException;
import java.util.Collection;
import java.util.Arrays;
import java.util.ArrayList;
import java.util.Date;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import java.util.Map;
import java.text.DateFormat;
import java.text.SimpleDateFormat;
/**
* Tests the basic functionality of javahl binding (inspired by the
* tests in subversion/tests/cmdline/basic_tests.py).
*/
public class BasicTests extends SVNTests
{
/**
* Base name of all our tests.
*/
public final static String testName = "basic_test";
public BasicTests()
{
init();
}
public BasicTests(String name)
{
super(name);
init();
}
/**
* Initialize the testBaseName and the testCounter, if this is the
* first test of this class.
*/
private void init()
{
if (!testName.equals(testBaseName))
{
testCounter = 0;
testBaseName = testName;
}
}
/**
* Test LogDate().
* @throws Throwable
*/
public void testLogDate() throws Throwable
{
String goodDate = "2007-10-04T03:00:52.134992Z";
String badDate = "2008-01-14";
LogDate logDate;
try
{
logDate = new LogDate(goodDate);
assertEquals(1191466852134992L, logDate.getTimeMicros());
} catch (ParseException e) {
fail("Failed to parse date " + goodDate);
}
try
{
logDate = new LogDate(badDate);
fail("Failed to throw exception on bad date " + badDate);
} catch (ParseException e) {
}
}
/**
* Test SVNClient.getVersion().
* @throws Throwable
*/
public void testVersion() throws Throwable
{
try
{
Version version = client.getVersion();
String versionString = version.toString();
if (versionString == null || versionString.trim().length() == 0)
{
throw new Exception("Version string empty");
}
}
catch (Exception e)
{
fail("Version should always be available unless the " +
"native libraries failed to initialize: " + e);
}
}
/**
* Test the JNIError class functionality
* @throws Throwable
*/
public void testJNIError() throws Throwable
{
// build the test setup.
OneTest thisTest = new OneTest();
// Create a client, dispose it, then try to use it later
ISVNClient tempclient = new SVNClient();
tempclient.dispose();
// create Y and Y/Z directories in the repository
addExpectedCommitItem(null, thisTest.getUrl().toString(), "Y", NodeKind.none,
CommitItemStateFlags.Add);
Set<String> urls = new HashSet<String>(1);
urls.add(thisTest.getUrl() + "/Y");
try
{
tempclient.mkdir(urls, false, null, new ConstMsg("log_msg"), null);
}
catch(JNIError e)
{
return; // Test passes!
}
fail("A JNIError should have been thrown here.");
}
/**
* Tests Mergeinfo and RevisionRange classes.
* @since 1.5
*/
public void testMergeinfoParser() throws Throwable
{
String mergeInfoPropertyValue =
"/trunk:1-300,305,307,400-405\n/branches/branch:308-400";
Mergeinfo info = new Mergeinfo(mergeInfoPropertyValue);
Set<String> paths = info.getPaths();
assertEquals(2, paths.size());
List<RevisionRange> trunkRange = info.getRevisionRange("/trunk");
assertEquals(4, trunkRange.size());
assertEquals("1-300", trunkRange.get(0).toString());
assertEquals("305", trunkRange.get(1).toString());
assertEquals("307", trunkRange.get(2).toString());
assertEquals("400-405", trunkRange.get(3).toString());
List<RevisionRange> branchRange =
info.getRevisionRange("/branches/branch");
assertEquals(1, branchRange.size());
}
/**
* Test the basic SVNClient.status functionality.
* @throws Throwable
*/
public void testBasicStatus() throws Throwable
{
// build the test setup
OneTest thisTest = new OneTest();
// check the status of the working copy
thisTest.checkStatus();
// Test status of non-existent file
File fileC = new File(thisTest.getWorkingCopy() + "/A", "foo.c");
MyStatusCallback statusCallback = new MyStatusCallback();
client.status(fileToSVNPath(fileC, false), Depth.unknown, false, true,
false, false, null, statusCallback);
if (statusCallback.getStatusArray().length > 0)
fail("File foo.c should not return a status.");
}
/**
* Test the "out of date" info from {@link
* org.apache.subversion.javahl.SVNClient#status()}.
*
* @throws SubversionException
* @throws IOException
*/
public void testOODStatus() throws SubversionException, IOException
{
// build the test setup
OneTest thisTest = new OneTest();
// Make a whole slew of changes to a WC:
//
// (root) r7 - prop change
// iota
// A
// |__mu
// |
// |__B
// | |__lambda
// | |
// | |__E r12 - deleted
// | | |__alpha
// | | |__beta
// | |
// | |__F r9 - prop change
// | |__I r6 - added dir
// |
// |__C r5 - deleted
// |
// |__D
// |__gamma
// |
// |__G
// | |__pi r3 - deleted
// | |__rho r2 - modify text
// | |__tau r4 - modify text
// |
// |__H
// |__chi r10-11 replaced with file
// |__psi r13-14 replaced with dir
// |__omega
// |__nu r8 - added file
File file, dir;
PrintWriter pw;
Status status;
MyStatusCallback statusCallback;
long rev; // Resulting rev from co or update
long expectedRev = 2; // Keeps track of the latest rev committed
// ----- r2: modify file A/D/G/rho --------------------------
file = new File(thisTest.getWorkingCopy(), "A/D/G/rho");
pw = new Prin
没有合适的资源?快使用搜索试试~ 我知道了~
Subversion for Windows 1.7.3 最新绿色版

温馨提示
Subversion是一个自由/开源的版本控制系统。也就是说,在Subversion管理下,文件和目录可以超越时空。也就是Subversion允许你数据恢复到早期版本,或者是检查数据修改的历史。正因为如此,许多人将版本控制系统当作一种神奇的“时间机器”。
资源推荐
资源详情
资源评论
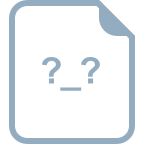
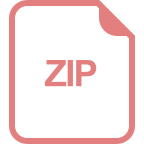
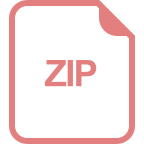
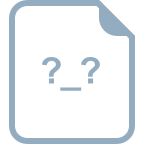
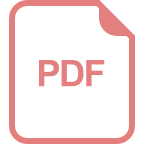
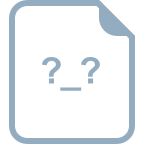
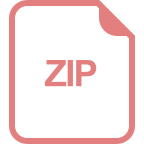
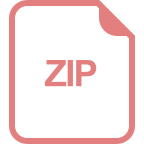
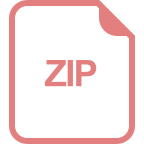
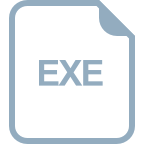
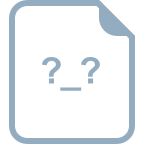
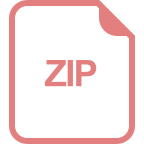
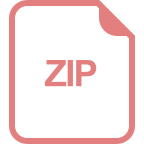
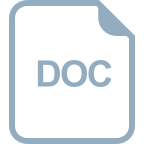
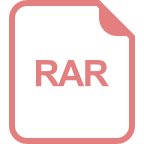
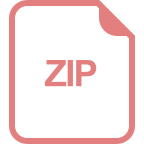
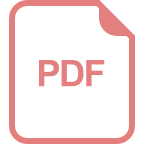
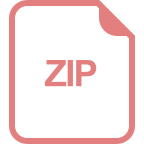
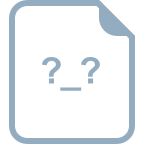
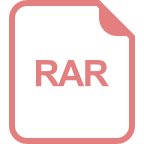
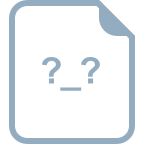
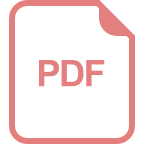
收起资源包目录

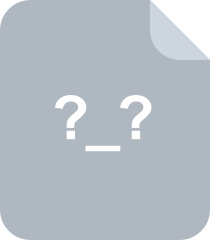
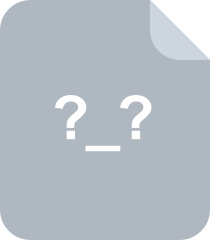
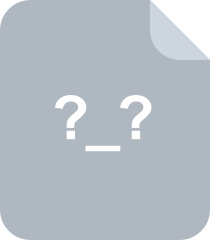
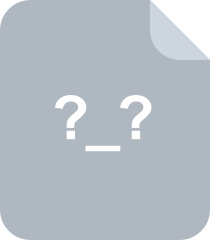
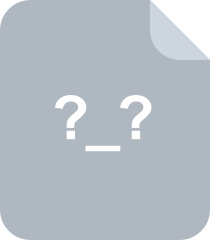
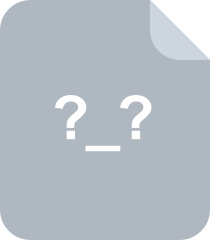
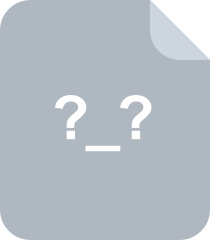
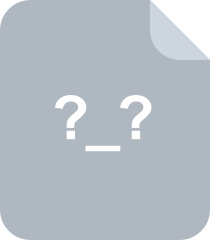
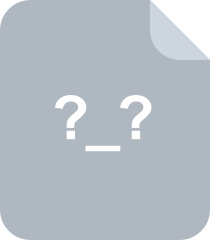
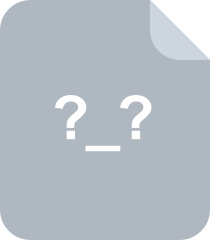
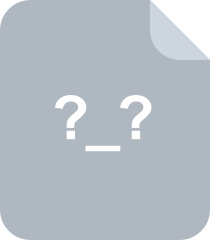
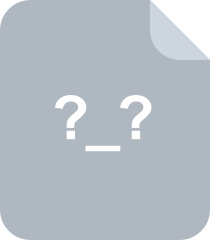
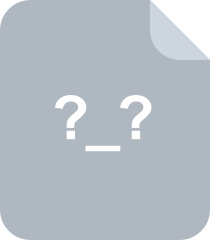
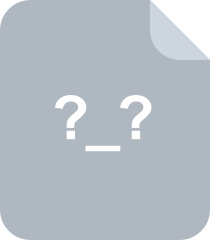
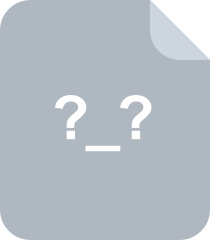
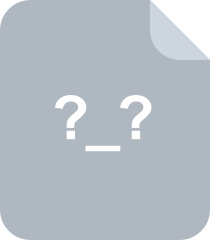
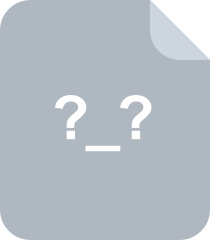
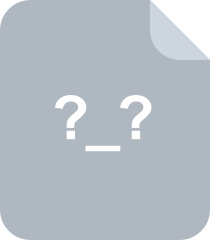
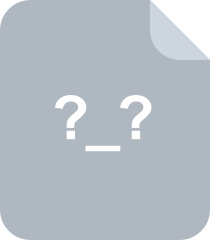
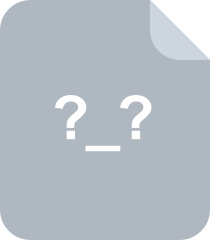
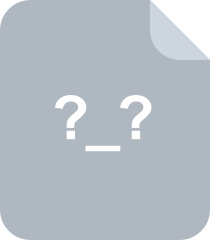
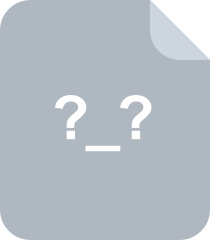
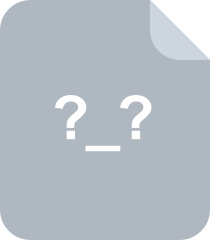
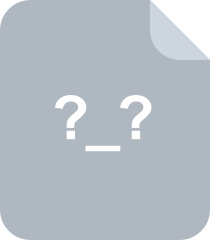
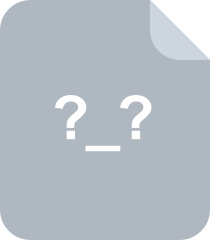
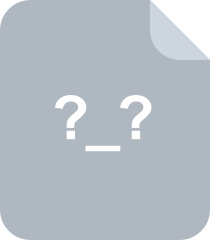
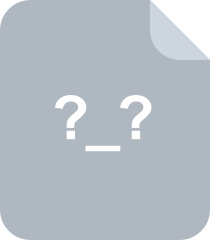
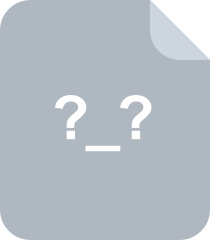
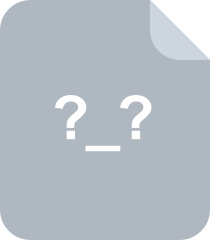
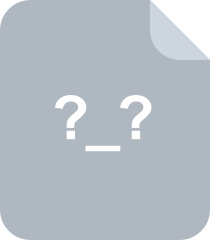
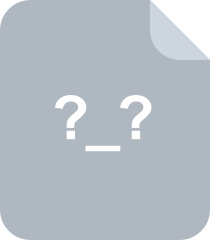
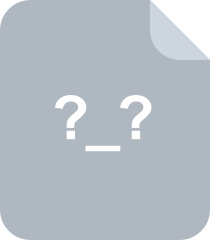
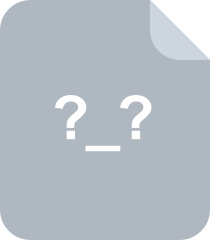
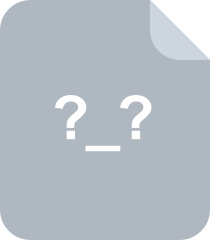
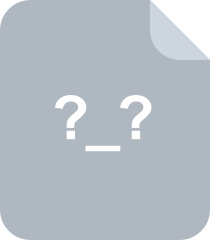
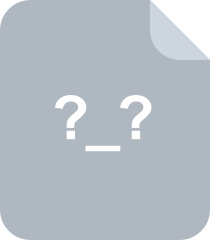
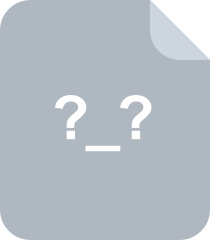
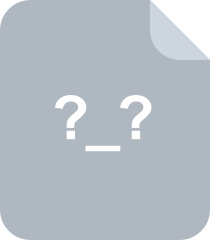
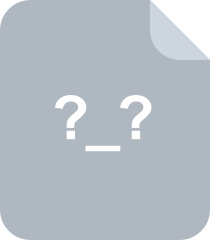
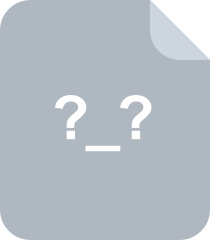
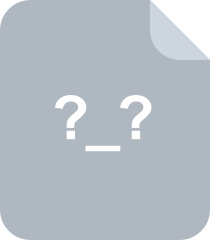
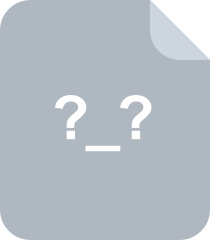
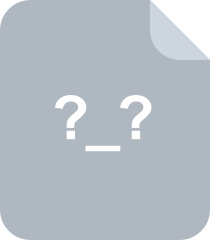
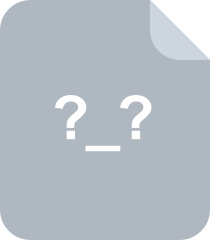
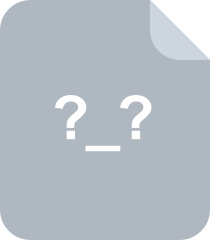
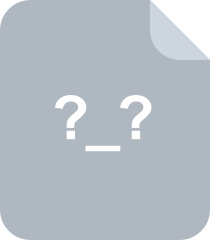
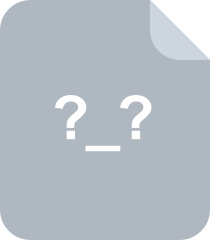
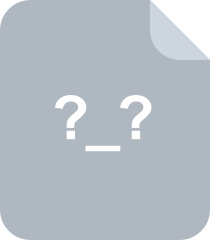
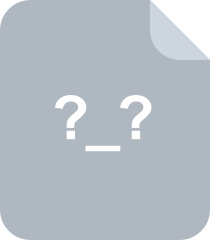
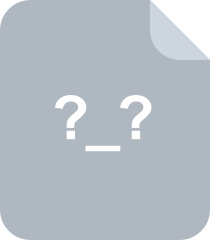
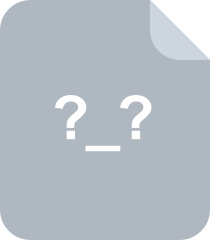
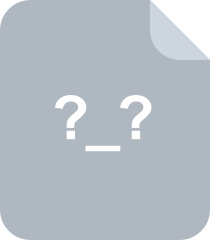
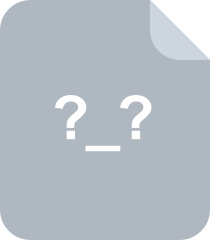
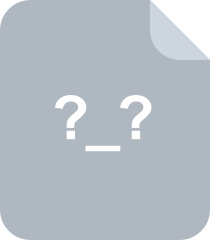
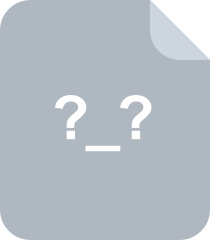
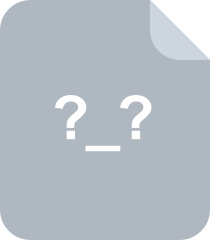
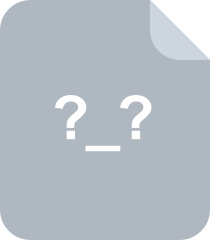
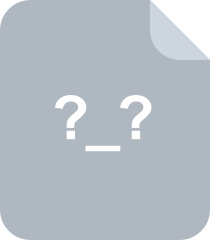
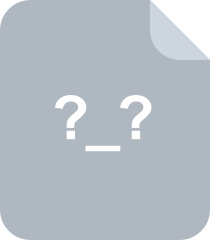
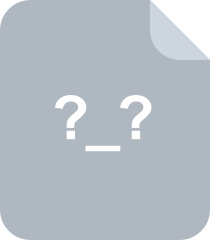
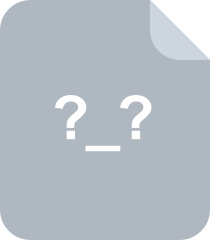
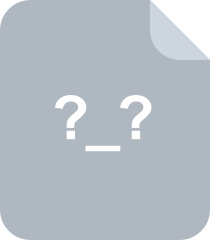
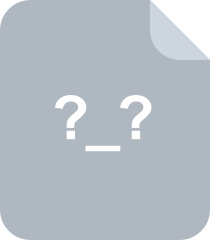
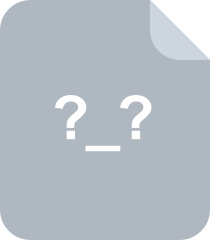
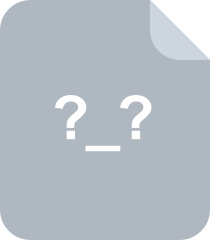
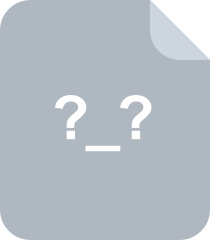
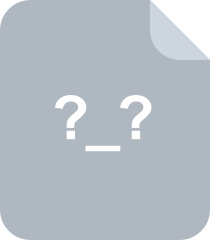
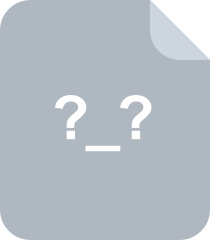
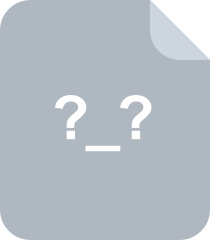
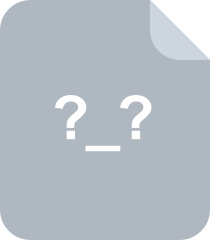
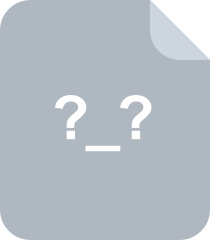
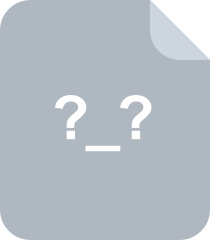
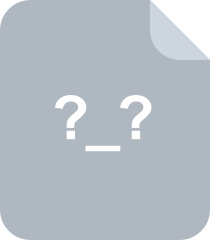
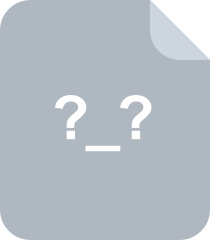
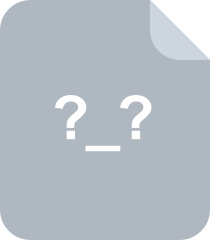
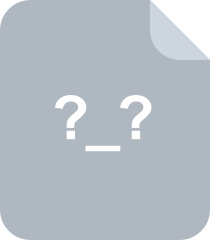
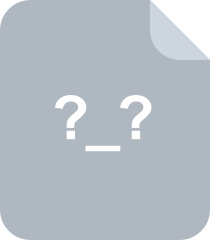
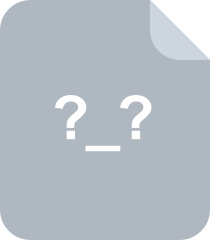
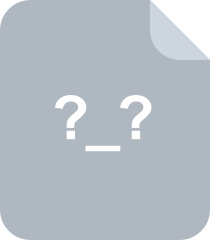
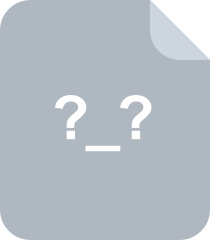
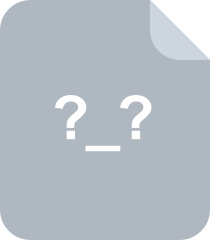
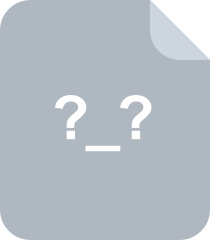
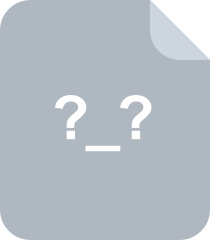
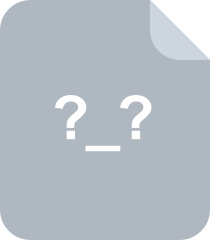
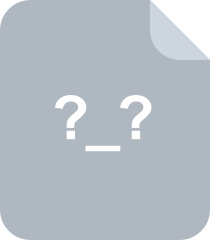
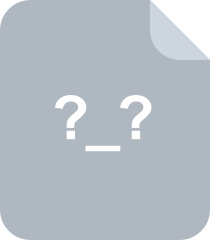
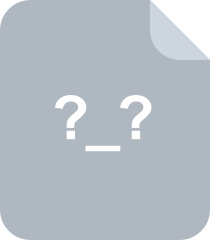
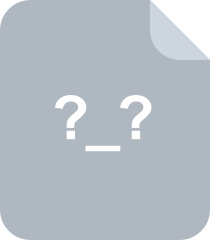
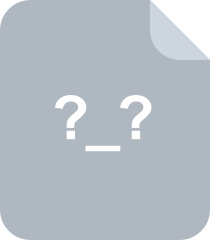
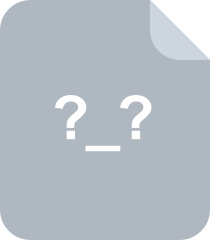
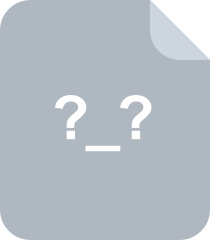
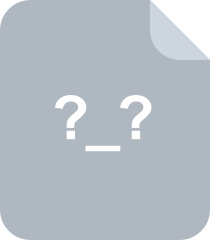
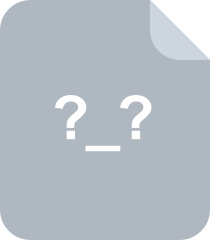
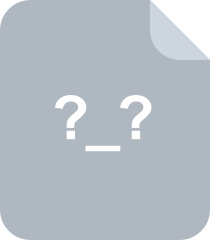
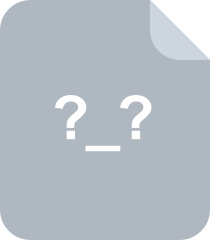
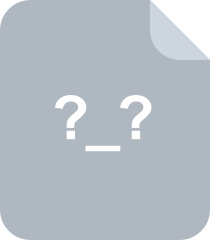
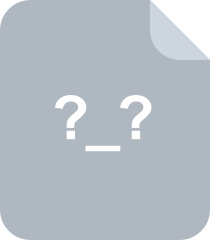
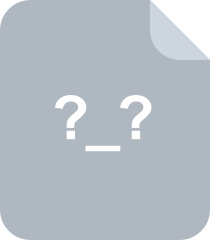
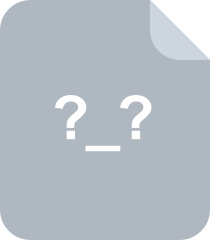
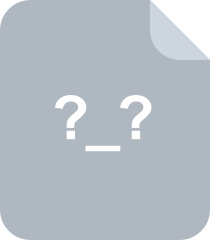
共 1575 条
- 1
- 2
- 3
- 4
- 5
- 6
- 16
资源评论
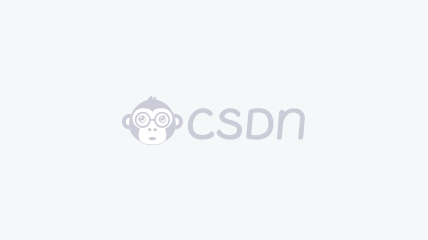
- thinkn2017-04-01是 linux版本的,不存在的绿色。。。。。
- simonyesh19842013-09-18是linux版本的,我想要win版本的
- cbwindboy2015-12-16找好很多地方,最终还是CSDN上靠谱,给力

nicksean
- 粉丝: 18
- 资源: 14
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

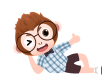
最新资源
- YoloX目标检测算法的结构原理及其应用优化
- java-leetcode题解之Populating Next Right Pointers in Each Node.java
- java-leetcode题解之Plus One.java
- java-leetcode题解之Play with Chips.java
- java-leetcode题解之PIO.java
- java-leetcode题解之Permutation Sequence.java
- java-leetcode题解之Permutation in String.java
- java-leetcode题解之Perfect Squares.java
- java-leetcode题解之Path with Maximum Gold.java
- java-leetcode题解之Path Sum III.java
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


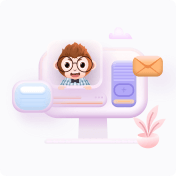
安全验证
文档复制为VIP权益,开通VIP直接复制
