python MapReduce的wordcount
### Python 实现 MapReduce 的 WordCount 示例详解 #### 一、引言 MapReduce 是 Hadoop 生态系统中的一种编程模型,主要用于大规模数据集的并行处理。它通过两个主要阶段——`Map` 和 `Reduce` 来实现数据处理任务。在本篇文章中,我们将深入探讨如何使用 Python 实现一个简单的 MapReduce WordCount 应用。 #### 二、MapReduce 基本思想 MapReduce 的工作原理依赖于将大数据集分割成多个小块,然后将这些小块并行地处理。具体来说: 1. **Map 阶段**:该阶段的主要任务是对输入数据进行分解,将其转换为键值对形式,每个键值对都是输入数据的一部分。 2. **Reduce 阶段**:在 Map 阶段完成后,键值对会被传递到 Reduce 阶段。在这个阶段,相同键的键值对被组合在一起处理,通常用于汇总或聚合操作。 在使用 Python 实现 MapReduce 时,我们主要利用了 Hadoop Streaming API,通过标准输入和输出(`STDIN` 和 `STDOUT`)来传递数据。 #### 三、Python 实现 MapReduce WordCount 下面我们将通过具体的代码示例来实现一个简单的 WordCount 应用。 ##### 1. Mapper 函数 Mapper 函数负责读取输入数据,并将其转换为键值对形式,这里的键为单词,值为出现次数。 ```python import sys for line in sys.stdin: line = line.strip() words = line.split() for word in words: print('%s\t%s' % (word, 1)) ``` - **代码解析**: - 首先导入 `sys` 模块,用于处理标准输入输出。 - 对每行输入数据去除首尾空白字符后,按空格分割成单词。 - 输出每个单词及其出现次数,格式为 “单词,1”。 - **测试**: ```bash echo "aabbccddaacc" | python mapper.py ``` ##### 2. Reducer 函数 Reducer 函数的任务是接收 Mapper 函数的输出,并对相同单词的出现次数进行汇总。 ```python import sys current_word = None current_count = 0 word = None for line in sys.stdin: line = line.strip() word, count = line.split('\t', 1) try: count = int(count) except ValueError: continue if current_word == word: current_count += count else: if current_word: print('%s\t%s' % (current_word, current_count)) current_count = count current_word = word if current_word == word: print('%s\t%s' % (current_word, current_count)) ``` - **代码解析**: - 导入 `sys` 模块。 - 初始化变量 `current_word` 和 `current_count`。 - 读取 Mapper 的输出,解析出单词和对应的计数。 - 如果当前单词与之前的不同,则输出前一个单词的统计结果,并更新当前单词及其计数。 - **测试**: ```bash echo "aaaabbccdddd" | python mapper.py | python reducer.py ``` ##### 3. 部署与运行 为了使上述程序能够在 Hadoop 环境下运行,我们需要做一些准备工作: 1. **创建 HDFS 目录**: ```bash bin/hdfs dfs -mkdir /temp/ bin/hdfs dfs -mkdir /temp/hdin ``` 2. **上传文件至 HDFS**: ```bash bin/hdfs dfs -copyFromLocal LICENSE.txt /temp/hdin ``` 3. **编写并运行 Shell 脚本**: ```bash #!/bin/bash export CURRENT=/usr/local/working $HADOOP_HOME/bin/hdfs dfs -rm -r /temp/hdout $HADOOP_HOME/bin/hadoop jar $HADOOP_HOME/share/hadoop/tools/lib/hadoop-streaming-2.7.3.jar \ -input "/temp/hdin/*" \ -output "/temp/hdout" \ -mapper "python mapper.py" \ -reducer "python reducer.py" \ -file "$CURRENT/mapper.py" \ -file "$CURRENT/reducer.py" ``` 4. **查看结果**: ```bash bin/hdfs dfs -cat /temp/hdout/* ``` 通过以上步骤,我们成功实现了基于 Python 的 MapReduce WordCount 示例。这种方法不仅有助于理解 MapReduce 的基本概念,还能够帮助开发者快速上手使用 Python 进行大数据处理任务。
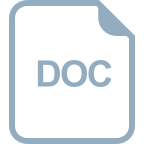
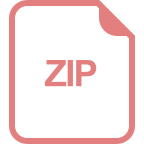
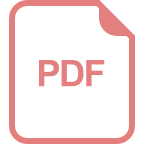
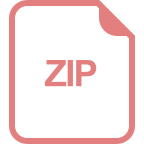
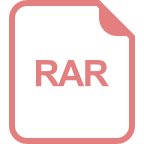
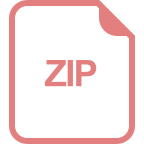
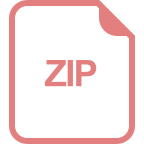
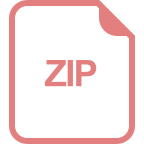
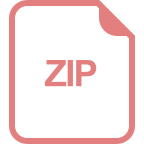
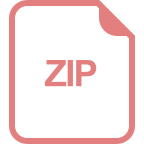
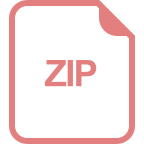
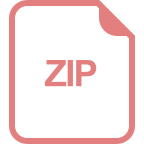
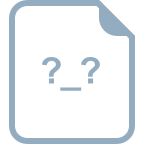
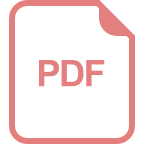
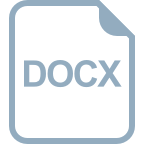
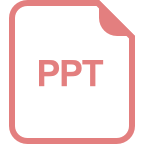
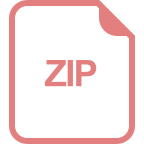
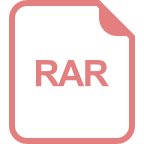
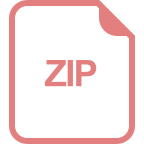
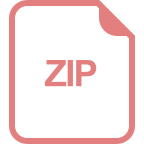
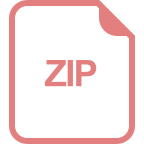
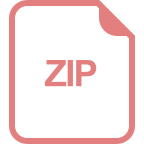
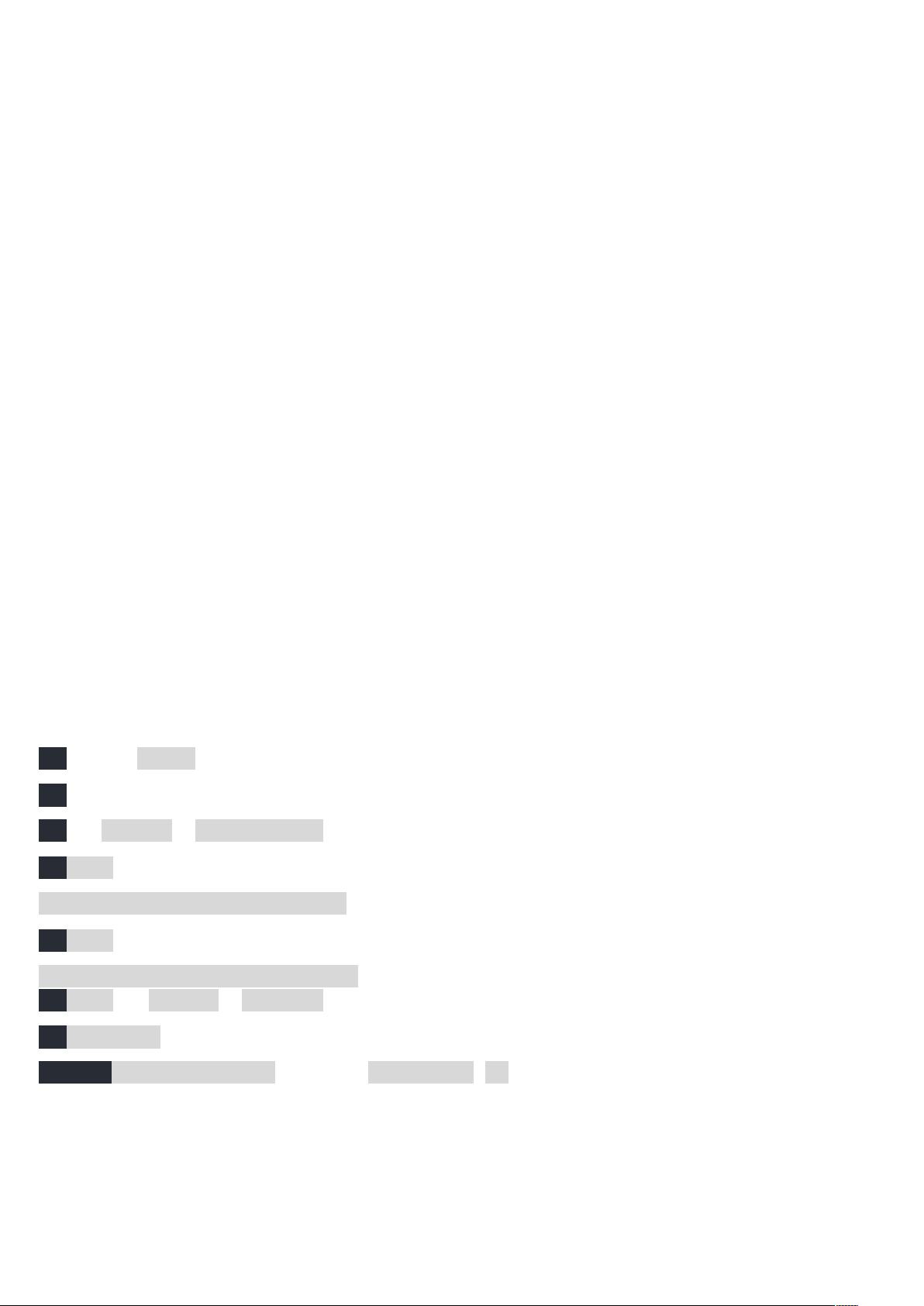
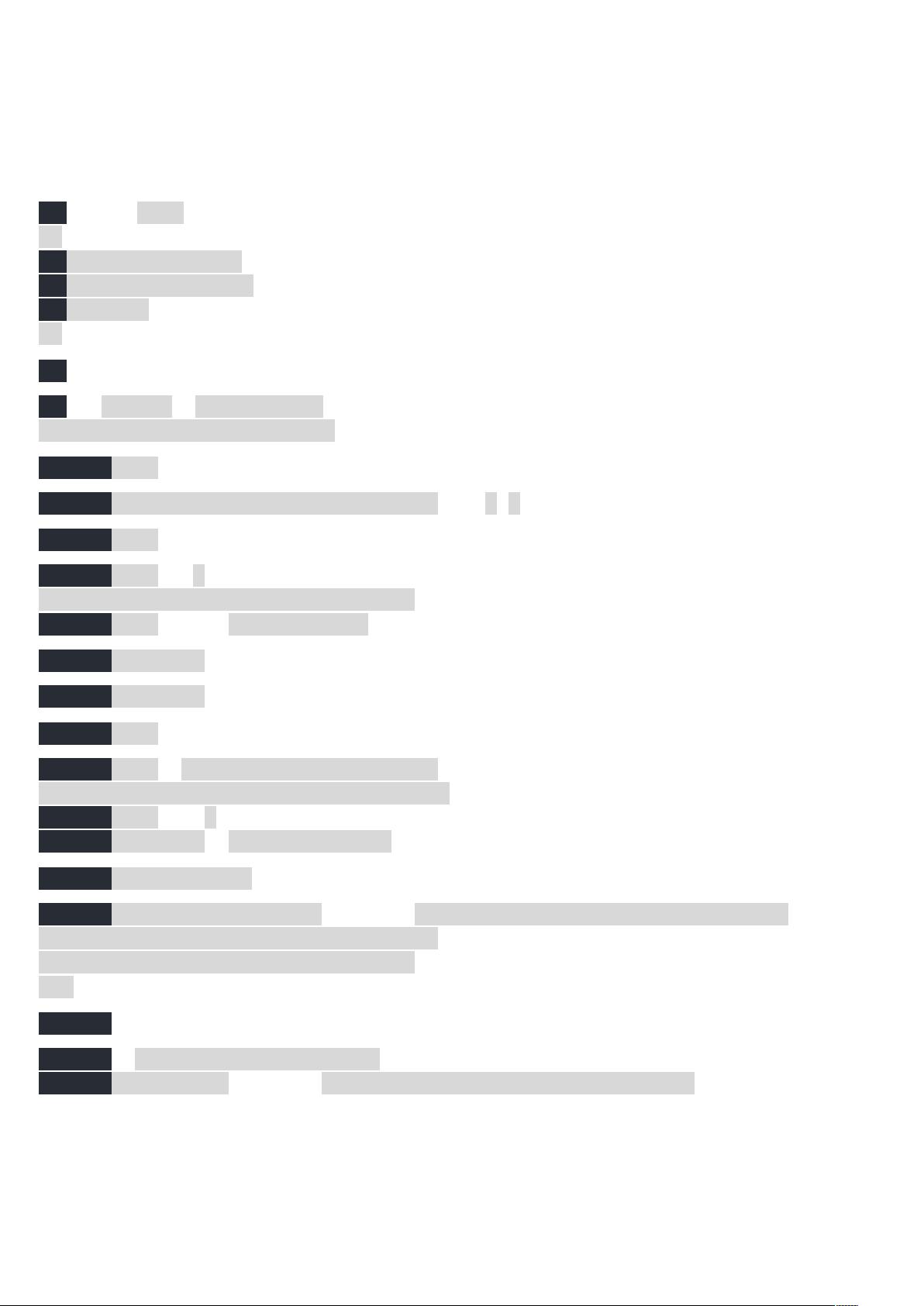
剩余7页未读,继续阅读
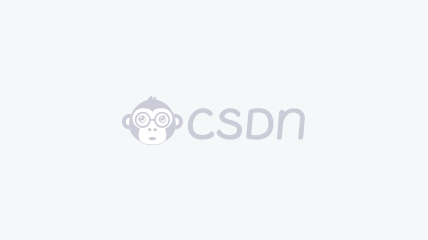

- 粉丝: 2
- 资源: 27
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

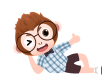
最新资源

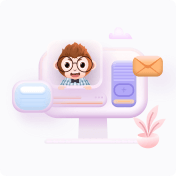
