// ==ClosureCompiler==
// @compilation_level SIMPLE_OPTIMIZATIONS
/**
* @license Highcharts JS v3.0.3 (2013-07-31)
*
* (c) 2009-2013 Torstein Hønsi
*
* License: www.highcharts.com/license
*/
// JSLint options:
/*global Highcharts, document, window, navigator, setInterval, clearInterval, clearTimeout, setTimeout, location, jQuery, $, console, each, grep */
(function () {
// encapsulated variables
var UNDEFINED,
doc = document,
win = window,
math = Math,
mathRound = math.round,
mathFloor = math.floor,
mathCeil = math.ceil,
mathMax = math.max,
mathMin = math.min,
mathAbs = math.abs,
mathCos = math.cos,
mathSin = math.sin,
mathPI = math.PI,
deg2rad = mathPI * 2 / 360,
// some variables
userAgent = navigator.userAgent,
isOpera = win.opera,
isIE = /msie/i.test(userAgent) && !isOpera,
docMode8 = doc.documentMode === 8,
isWebKit = /AppleWebKit/.test(userAgent),
isFirefox = /Firefox/.test(userAgent),
isTouchDevice = /(Mobile|Android|Windows Phone)/.test(userAgent),
SVG_NS = 'http://www.w3.org/2000/svg',
hasSVG = !!doc.createElementNS && !!doc.createElementNS(SVG_NS, 'svg').createSVGRect,
hasBidiBug = isFirefox && parseInt(userAgent.split('Firefox/')[1], 10) < 4, // issue #38
useCanVG = !hasSVG && !isIE && !!doc.createElement('canvas').getContext,
Renderer,
hasTouch = doc.documentElement.ontouchstart !== UNDEFINED,
symbolSizes = {},
idCounter = 0,
garbageBin,
defaultOptions,
dateFormat, // function
globalAnimation,
pathAnim,
timeUnits,
noop = function () {},
charts = [],
PRODUCT = 'Highcharts',
VERSION = '3.0.3',
// some constants for frequently used strings
DIV = 'div',
ABSOLUTE = 'absolute',
RELATIVE = 'relative',
HIDDEN = 'hidden',
PREFIX = 'highcharts-',
VISIBLE = 'visible',
PX = 'px',
NONE = 'none',
M = 'M',
L = 'L',
/*
* Empirical lowest possible opacities for TRACKER_FILL
* IE6: 0.002
* IE7: 0.002
* IE8: 0.002
* IE9: 0.00000000001 (unlimited)
* IE10: 0.0001 (exporting only)
* FF: 0.00000000001 (unlimited)
* Chrome: 0.000001
* Safari: 0.000001
* Opera: 0.00000000001 (unlimited)
*/
TRACKER_FILL = 'rgba(192,192,192,' + (hasSVG ? 0.0001 : 0.002) + ')', // invisible but clickable
//TRACKER_FILL = 'rgba(192,192,192,0.5)',
NORMAL_STATE = '',
HOVER_STATE = 'hover',
SELECT_STATE = 'select',
MILLISECOND = 'millisecond',
SECOND = 'second',
MINUTE = 'minute',
HOUR = 'hour',
DAY = 'day',
WEEK = 'week',
MONTH = 'month',
YEAR = 'year',
// constants for attributes
LINEAR_GRADIENT = 'linearGradient',
STOPS = 'stops',
STROKE_WIDTH = 'stroke-width',
// time methods, changed based on whether or not UTC is used
makeTime,
getMinutes,
getHours,
getDay,
getDate,
getMonth,
getFullYear,
setMinutes,
setHours,
setDate,
setMonth,
setFullYear,
// lookup over the types and the associated classes
seriesTypes = {};
// The Highcharts namespace
win.Highcharts = win.Highcharts ? error(16, true) : {};
/**
* Extend an object with the members of another
* @param {Object} a The object to be extended
* @param {Object} b The object to add to the first one
*/
function extend(a, b) {
var n;
if (!a) {
a = {};
}
for (n in b) {
a[n] = b[n];
}
return a;
}
/**
* Deep merge two or more objects and return a third object.
* Previously this function redirected to jQuery.extend(true), but this had two limitations.
* First, it deep merged arrays, which lead to workarounds in Highcharts. Second,
* it copied properties from extended prototypes.
*/
function merge() {
var i,
len = arguments.length,
ret = {},
doCopy = function (copy, original) {
var value, key;
// An object is replacing a primitive
if (typeof copy !== 'object') {
copy = {};
}
for (key in original) {
if (original.hasOwnProperty(key)) {
value = original[key];
// Copy the contents of objects, but not arrays or DOM nodes
if (value && typeof value === 'object' && Object.prototype.toString.call(value) !== '[object Array]'
&& typeof value.nodeType !== 'number') {
copy[key] = doCopy(copy[key] || {}, value);
// Primitives and arrays are copied over directly
} else {
copy[key] = original[key];
}
}
}
return copy;
};
// For each argument, extend the return
for (i = 0; i < len; i++) {
ret = doCopy(ret, arguments[i]);
}
return ret;
}
/**
* Take an array and turn into a hash with even number arguments as keys and odd numbers as
* values. Allows creating constants for commonly used style properties, attributes etc.
* Avoid it in performance critical situations like looping
*/
function hash() {
var i = 0,
args = arguments,
length = args.length,
obj = {};
for (; i < length; i++) {
obj[args[i++]] = args[i];
}
return obj;
}
/**
* Shortcut for parseInt
* @param {Object} s
* @param {Number} mag Magnitude
*/
function pInt(s, mag) {
return parseInt(s, mag || 10);
}
/**
* Check for string
* @param {Object} s
*/
function isString(s) {
return typeof s === 'string';
}
/**
* Check for object
* @param {Object} obj
*/
function isObject(obj) {
return typeof obj === 'object';
}
/**
* Check for array
* @param {Object} obj
*/
function isArray(obj) {
return Object.prototype.toString.call(obj) === '[object Array]';
}
/**
* Check for number
* @param {Object} n
*/
function isNumber(n) {
return typeof n === 'number';
}
function log2lin(num) {
return math.log(num) / math.LN10;
}
function lin2log(num) {
return math.pow(10, num);
}
/**
* Remove last occurence of an item from an array
* @param {Array} arr
* @param {Mixed} item
*/
function erase(arr, item) {
var i = arr.length;
while (i--) {
if (arr[i] === item) {
arr.splice(i, 1);
break;
}
}
//return arr;
}
/**
* Returns true if the object is not null or undefined. Like MooTools' $.defined.
* @param {Object} obj
*/
function defined(obj) {
return obj !== UNDEFINED && obj !== null;
}
/**
* Set or get an attribute or an object of attributes. Can't use jQuery attr because
* it attempts to set expando properties on the SVG element, which is not allowed.
*
* @param {Object} elem The DOM element to receive the attribute(s)
* @param {String|Object} prop The property or an abject of key-value pairs
* @param {String} value The value if a single property is set
*/
function attr(elem, prop, value) {
var key,
setAttribute = 'setAttribute',
ret;
// if the prop is a string
if (isString(prop)) {
// set the value
if (defined(value)) {
elem[setAttribute](prop, value);
// get the value
} else if (elem && elem.getAttribute) { // elem not defined when printing pie demo...
ret = elem.getAttribute(prop);
}
// else if prop is defined, it is a hash of key/value pairs
} else if (defined(prop) && isObject(prop)) {
for (key in prop) {
elem[setAttribute](key, prop[key]);
}
}
return ret;
}
/**
* Check if an element is an array, and if not, make it into an array. Like
* MooTools' $.splat.
*/
function splat(obj) {
return isArray(obj) ? obj : [obj];
}
/**
* Return the first value that is defined. Like MooTools' $.pick.
*/
function pick() {
var args = arguments,
i,
arg,
length = args.length;
for (i = 0; i < length; i++) {
arg = args[i];
if (typeof arg !== 'undefined' && arg !== null) {
return arg;
}
}
}
/**
* Set CSS on a given element
* @param {Object} el
* @param {Object} styles Style object with camel case property names
*/
function css(el, styles) {
if (isIE) {
if (styles && styles.opacity !== UNDEFINED) {
styles.filter = 'alpha(opacity=' + (styles.opacity * 100) + ')';
}
}
extend(el.style, styles);
}
/**
* Utility function to create element with attributes and styles
* @param {Object} tag
* @param {Object} attribs
* @param {Object} styles
* @param {Object} parent
* @param {Object} nopad
*/
function createElement(tag, attribs, styles, parent, nopad) {
var el = doc.createElement(tag);
if (attribs) {
extend(el, attribs);
}
if (nopad) {
css(el, {paddin
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
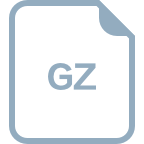
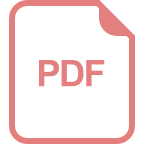
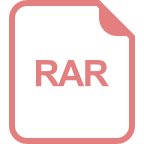
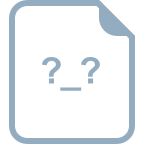
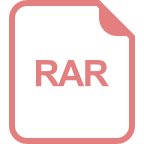
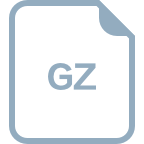
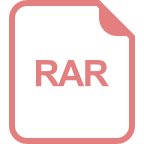
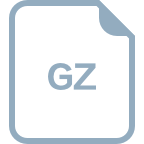
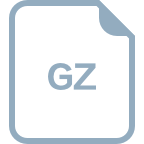
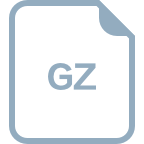
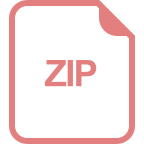
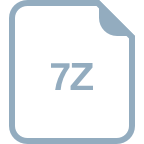
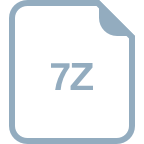
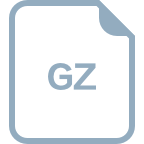
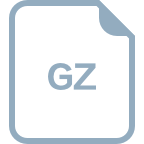
收起资源包目录

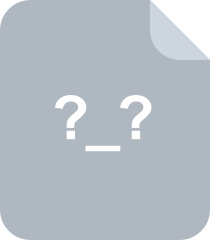
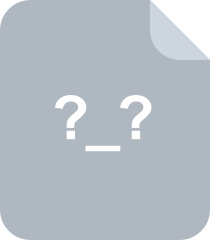
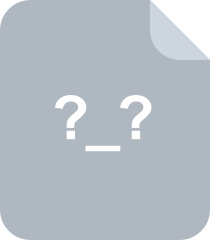
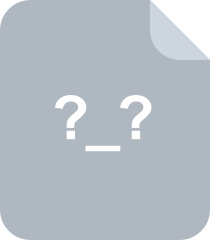
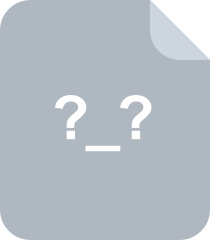
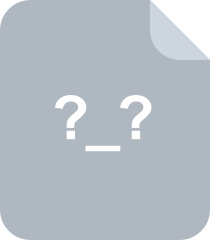
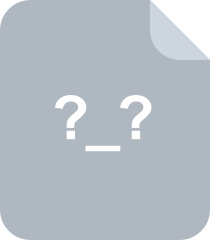
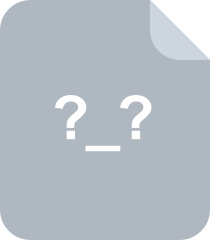
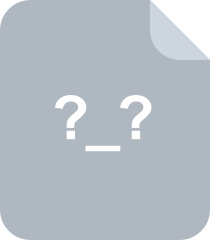
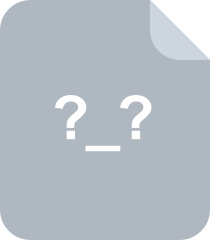
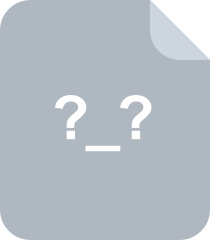
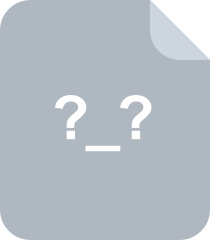
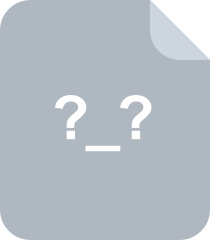
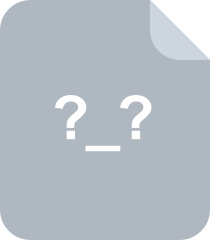
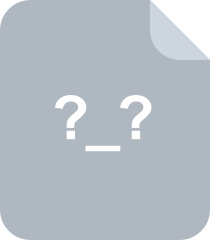
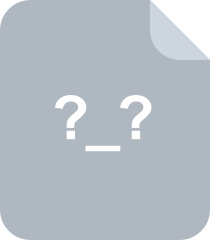
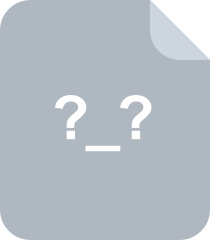
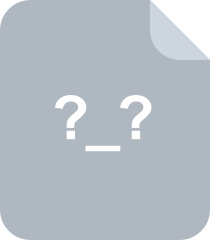
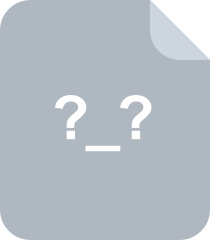
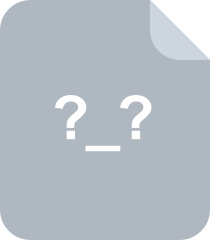
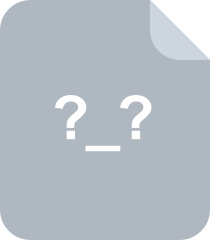
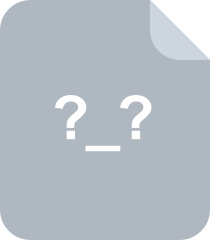
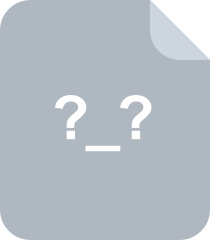
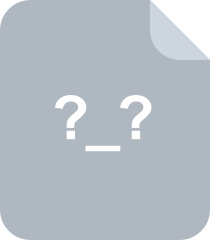
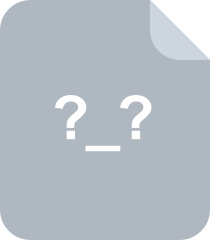
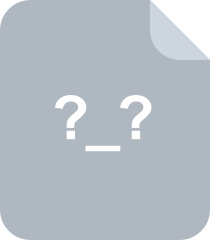
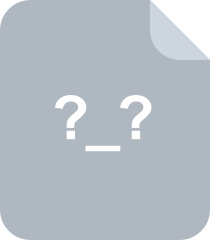
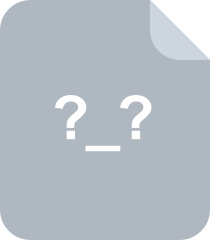
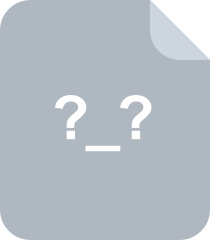
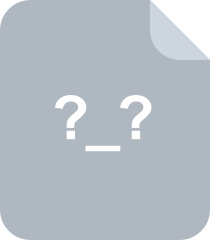
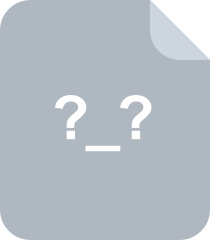
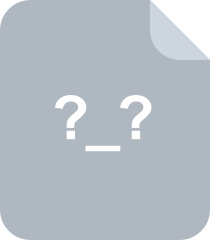
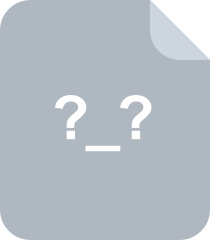
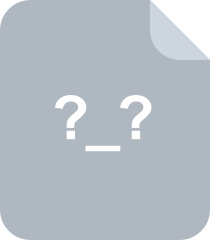
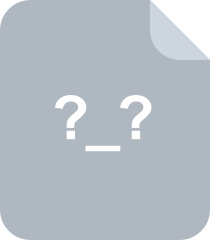
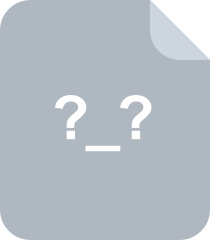
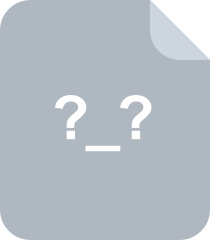
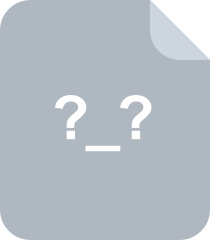
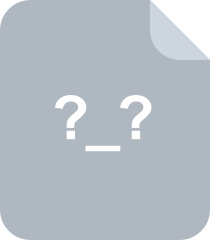
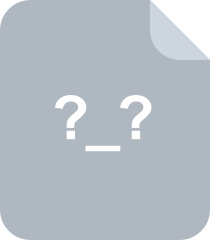
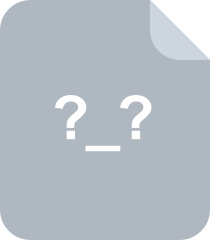
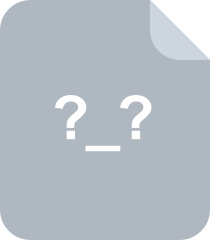
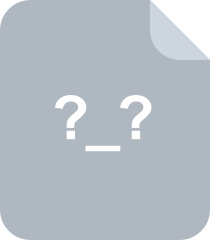
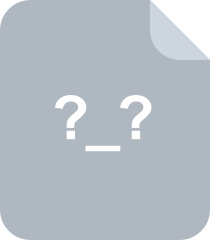
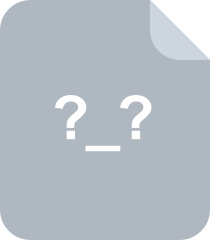
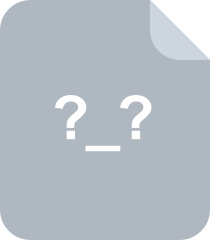
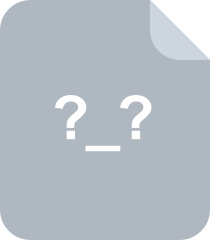
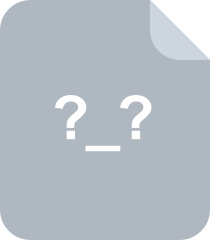
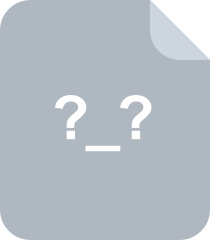
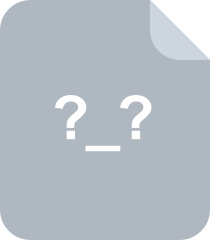
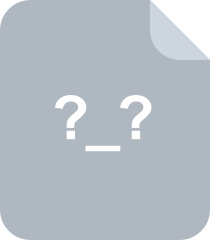
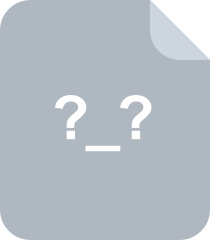
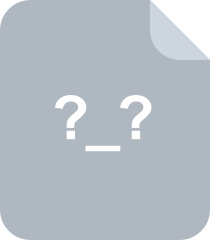
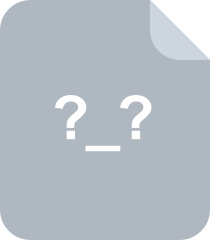
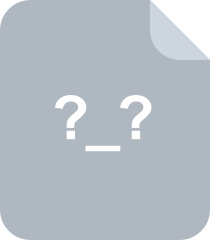
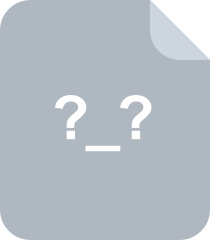
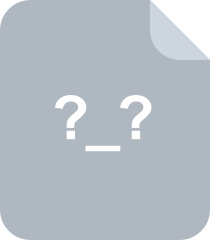
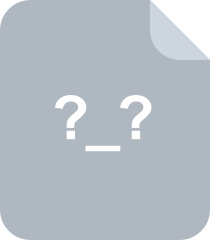
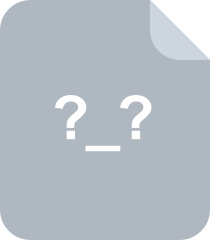
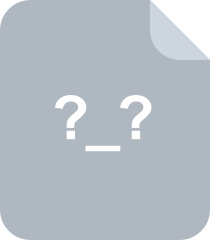
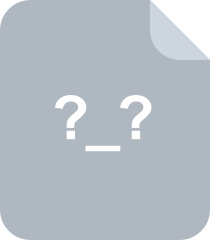
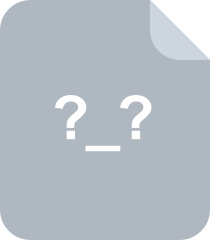
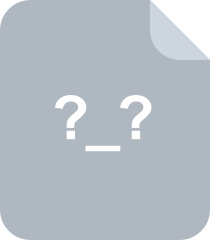
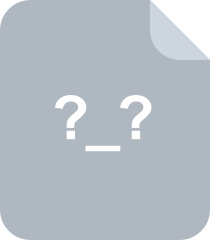
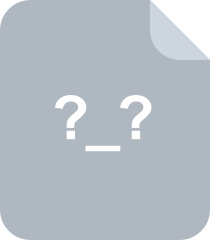
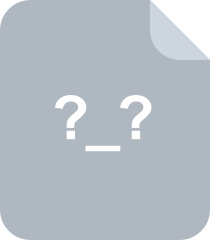
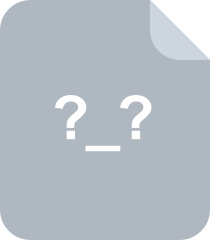
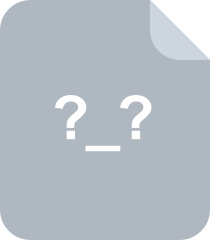
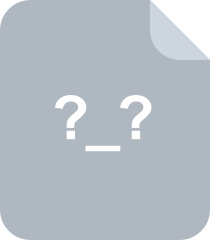
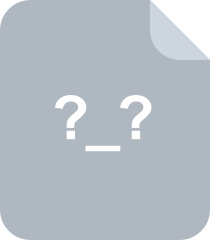
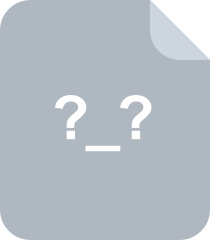
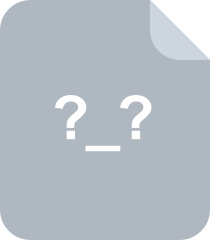
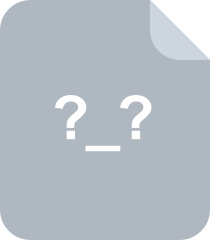
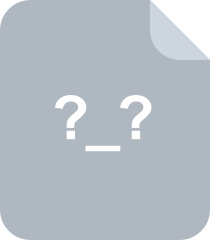
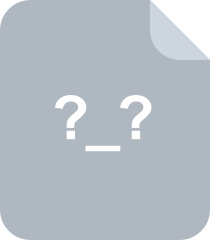
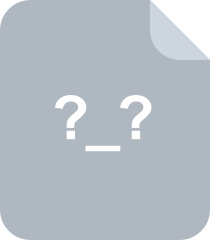
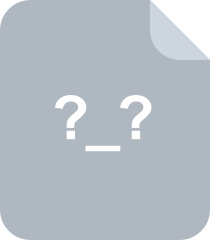
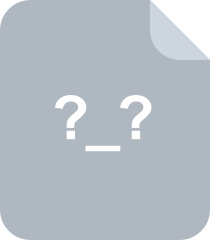
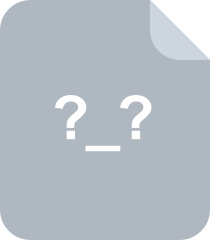
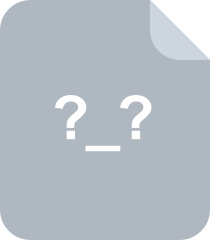
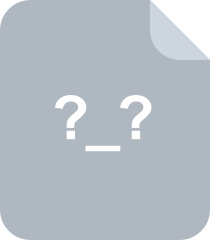
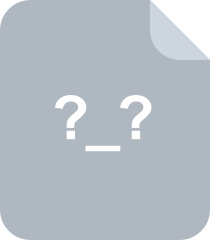
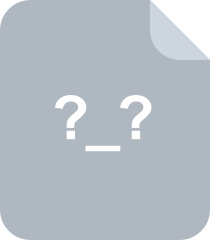
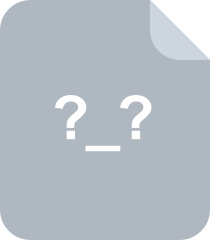
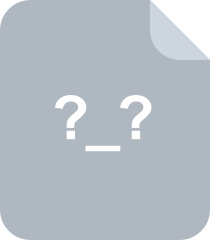
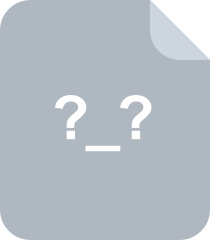
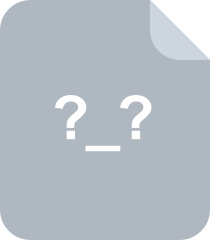
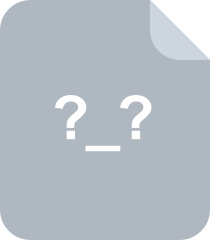
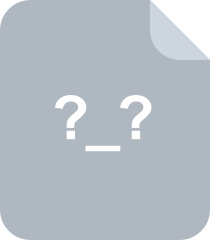
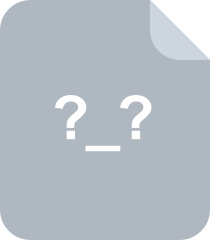
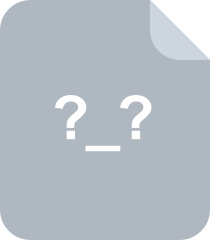
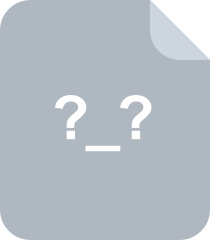
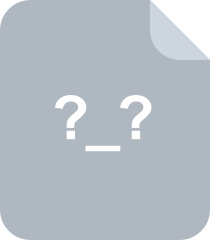
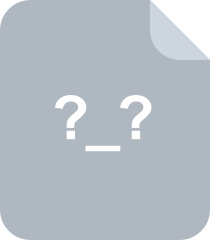
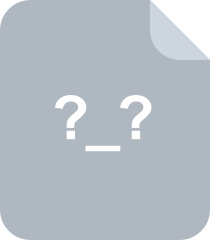
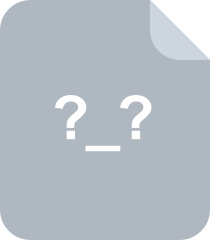
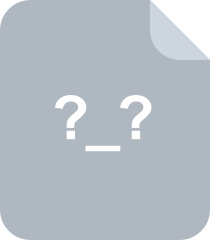
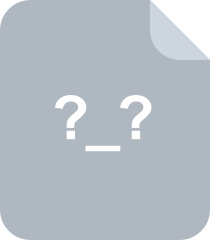
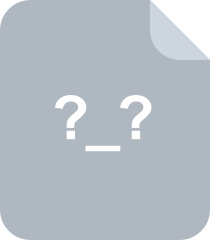
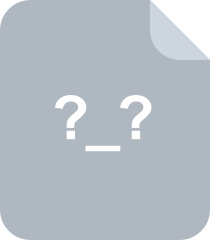
共 1067 条
- 1
- 2
- 3
- 4
- 5
- 6
- 11
资源评论
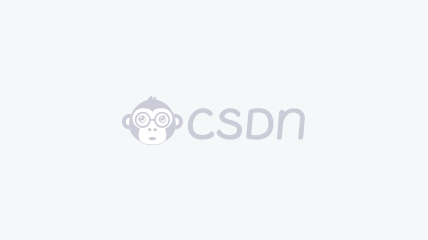

大话JAVA的那些事
- 粉丝: 336
- 资源: 20
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

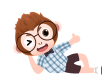
最新资源
- 小说网站-JAVA-基于springBoot“西贝”小说网站的设计与实现
- 游戏分享网站-JAVA-基于springBoot“腾达”游戏分享网站的设计与实现
- 学习交流-JAVA-基于springBoot“非学勿扰”学习交流平台设计与实现
- EDAfloorplanning
- 所有课程均提供 Python 复习部分.zip
- 所有算法均在 Python 3 中实现,是 hacktoberfest2020 的一个项目 - 没有针对 hacktoberfest 2021 的问题或 PR.zip
- OpenCV的用户手册资源.zip
- 用springmvc实现的校园选课管理系统
- 我的所有 Python 代码都存储在这个文件夹中 .zip
- 以下是关于毕业设计项目开发的详细资源.docx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


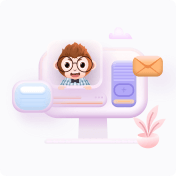
安全验证
文档复制为VIP权益,开通VIP直接复制
