<?php
/*
日期:2022年7月25日
作者:cy
概要:与商砼交互有关的配置
*/
//本地配置
$TO_ST_URL="http://xyis.cy/api/oa_api.index";
$ST_SAP_URL="https://xyis.cy/api/sap_api.search/search";
$ZUO_ST_URL="http://xyis.cy/api/oa_api.index/cprod";
//生产配置
//$TO_ST_URL="http://10.0.0.253:8008/api/oa_api.index";
//$ST_SAP_URL="http://xyis.iwoyou.cn:8008/api/sap_api.search/search";//访问商砼接口,请求SAP主数据,仅在生产环境下连接正常访问。
$MD5_KEY='20220801#CY:为天地立心,为生民立命,为往圣继绝学,为万世开太平';
function getStationByTop($top_dept_id){
$station=array();
switch($top_dept_id){
case "21":
$station=array(
"station_code"=>"1000",
"station_name"=>"环保站",
"station_zm"=>"A"
);
break;
case "7":
$station=array(
"station_code"=>"2000",
"station_name"=>"兴业站",
"station_zm"=>"B"
);
break;
case "30":
$station=array(
"station_code"=>"3000",
"station_name"=>"好特站",
"station_zm"=>"D"
);
break;
case "39":
$station=array(
"station_code"=>"5000",
"station_name"=>"润旺站",
"station_zm"=>"C"
);
break;
case "88":
$station=array(
"station_code"=>"6000",
"station_name"=>"圣特兰",
"station_zm"=>"E"
);
break;
default:
$station=array(
"station_code"=>"无",
"station_name"=>"无",
"station_zm"=>"无");
break;
}
return $station;
}
function arrEncodeChange($arr,$toEncode='UTF-8',$defEncode='UTF-8'){
$encodeArr=array('toEncode'=>$toEncode,'defEncode'=>$defEncode);
array_walk_recursive($arr,function(&$value,$key,$encodeArr){
$encode=mb_detect_encoding($value,array('UTF-8','ASCII','GB2312','GBK','BIG5','EUC-CN'));
if($encode!=$encodeArr['defEncode']){
$value=iconv($encode,$encodeArr['toEncode'],$value);
}else{
return $value;
}
},$encodeArr);
return $arr;
}
function strEncodeChange($str,$toEncode='UTF-8',$defEncode='UTF-8'){
$encode=mb_detect_encoding($str,array('UTF-8','ASCII','GB2312','GBK','BIG5','EUC-CN'));
return iconv($encode,$toEncode,$str);
}
function posturl($url,$data){
$data = json_encode(arrEncodeChange($data,'UTF-8','UTF-8'));
$headerArray =array("Content-type:application/json;charset='utf-8'","Accept:application/json");
$curl = curl_init($url);
//curl_setopt($curl, CURLOPT_URL, $url);
curl_setopt($curl, CURLOPT_HTTPHEADER, array('Expect:'));
//php curl使用的liburl在发送数据时,如果数据量大于1KB时,会先发送包含header Expect:100-continue的请求与远程服务器协商,得到能够继续接收数据的确认后才开始发送真正数据,如果远程服务器没有正确返回,则此次请求失败,当然也就获取不到返回结果了。
curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, FALSE);
curl_setopt($curl, CURLOPT_SSL_VERIFYHOST,FALSE);
curl_setopt($curl, CURLOPT_POST, 1);
curl_setopt($curl, CURLOPT_POSTFIELDS, $data);
curl_setopt($curl,CURLOPT_HTTPHEADER,$headerArray);
curl_setopt($curl, CURLOPT_RETURNTRANSFER, 1);
$output = curl_exec($curl);
curl_close($curl);
return json_decode($output,true);
}
function strFilter($str){
$str = str_replace('`', '', $str);
$str = str_replace('·', '', $str);
$str = str_replace('~', '', $str);
$str = str_replace('!', '', $str);
$str = str_replace('!', '', $str);
$str = str_replace('@', '', $str);
$str = str_replace('#', '', $str);
$str = str_replace('$', '', $str);
$str = str_replace('¥', '', $str);
$str = str_replace('%', '', $str);
$str = str_replace('^', '', $str);
$str = str_replace('……', '', $str);
$str = str_replace('&', '', $str);
$str = str_replace('*', '', $str);
$str = str_replace('(', '', $str);
$str = str_replace(')', '', $str);
$str = str_replace('(', '', $str);
$str = str_replace(')', '', $str);
$str = str_replace('-', '', $str);
$str = str_replace('_', '', $str);
$str = str_replace('——', '', $str);
$str = str_replace('+', '', $str);
$str = str_replace('=', '', $str);
$str = str_replace('|', '', $str);
$str = str_replace('\\', '', $str);
$str = str_replace('[', '', $str);
$str = str_replace(']', '', $str);
$str = str_replace('【', '', $str);
$str = str_replace('】', '', $str);
$str = str_replace('{', '', $str);
$str = str_replace('}', '', $str);
$str = str_replace(';', '', $str);
$str = str_replace(';', '', $str);
$str = str_replace(':', '', $str);
$str = str_replace(':', '', $str);
$str = str_replace('\'', '', $str);
$str = str_replace('"', '', $str);
$str = str_replace('“', '', $str);
$str = str_replace('”', '', $str);
$str = str_replace(',', '', $str);
$str = str_replace(',', '', $str);
$str = str_replace('<', '', $str);
$str = str_replace('>', '', $str);
$str = str_replace('《', '', $str);
$str = str_replace('》', '', $str);
$str = str_replace('.', '', $str);
$str = str_replace('。', '', $str);
$str = str_replace('/', '', $str);
$str = str_replace('、', '', $str);
$str = str_replace('?', '', $str);
$str = str_replace('?', '', $str);
return trim($str);
}
// without SQL.
function safeData($data) {
$str=trim($data);
$str = str_replace('`', '', $str);
$str = str_replace('·', '', $str);
$str = str_replace('!', '', $str);
$str = str_replace('!', '', $str);
$str = str_replace('@', '', $str);
$str = str_replace('\\', '/', $str);
$str = str_replace('\'', '', $str);
$str = str_replace('"', '', $str);
$str = str_replace('<', '', $str);
$str = str_replace('>', '', $str);
$str = str_replace('=', '', $str);
$returnData = strip_tags($str);
return $returnData;
}
/*
日期:2022年7月27日
作者:cy
概要:流程中心的流程,在流转过程中,读取表单里的控件名对应的控件值。
替用原来的gerRunData函数的原因:
OA系统安装后,没有原工作流的数据表,导致原有函数getRunData运行报错。
涉及表:bpm_type,bpm_variable,bpm_variable_sort、业务表(bpm_data_xxx,bpm_data_xxx_child,bpm_data_xxx_list_xxx)
*/
function getRunDataCy($RUN_ID, $ELEMENT_ARRAY = array())
{
$OUTPUT_ARRAY = array();
if (!$RUN_ID) {
return NULL;
}
$query = 'select FORM_ID,bpm_type.FLOW_ID from bpm_type,bpm_RUN WHERE RUN_ID="' . $RUN_ID . '" AND bpm_RUN.FLOW_ID=bpm_type.FLOW_ID';
$cursor = exequery(TD::conn(), $query);
if ($ROW = mysql_fetch_array($cursor)) {
$FORM_ID = $ROW[0];
$FLOW_ID = $ROW['FLOW_ID'];
}
$table_name = 'bpm_data_' . $FLOW_ID;
$query = ' select * from ' . $table_name . ' where run_id="' . $RUN_ID . '" limit 1';
$cursor = exequery(TD::conn(), $query);
if ($ROW = mysql_fetch_assoc($cursor)) {
foreach ($ROW as $key => $value) {
if (strtolower(substr($key, 0, 6)) == 'data_m') {
$STR = strtoupper($key);
${$STR} = $value;
}
}
}
$table_name2=$table_name.'_child';
$query = ' select * from ' .$table_name2. ' where run_id="' . $RUN_ID . '" limit 1';
$cursor = exequery(TD::conn(), $query);
if ($ROW = mysql_fetch_assoc($cursor)) {
foreach ($ROW as $key => $value) {
if (strtolower(substr($key, 0, 6)) == 'data_m') {
$STR = strtoupper($key);
${$STR} = $value;
}
}
}
$tmp_arr=array();
$sql="select ID from bpm_variable_sort where FLOW_ID='".$FLOW_ID."'";
$res=exequery(TD::conn(), $sql);
while($row=mysql_fetch_assoc($res)){
$tmp_arr[]=$row['ID'];
}
$idstr=implode(',',$tmp_arr);
$WORKFLOW_ELEMENT_ARRAY = TD::get_cache('bpm/form/ELEMENT_ARRAY_' . $FORM_ID);
foreach ($WORKFLOW_ELEMENT_ARRAY as $ENAME => $ELEMENT_ARR) {
$ETITLE = "";
if(isset($ELEMENT_ARR['TITLE'])&&!empty($ELEMENT_ARR['TITLE'])){
if(strpos($ELE

67号人生
- 粉丝: 90
- 资源: 16
最新资源
- (源码)基于Django和OpenCV的智能车视频处理系统.zip
- (源码)基于ESP8266的WebDAV服务器与3D打印机管理系统.zip
- (源码)基于Nio实现的Mycat 2.0数据库代理系统.zip
- (源码)基于Java的高校学生就业管理系统.zip
- (源码)基于Spring Boot框架的博客系统.zip
- (源码)基于Spring Boot框架的博客管理系统.zip
- (源码)基于ESP8266和Blynk的IR设备控制系统.zip
- (源码)基于Java和JSP的校园论坛系统.zip
- (源码)基于ROS Kinetic框架的AGV激光雷达导航与SLAM系统.zip
- (源码)基于PythonDjango框架的资产管理系统.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


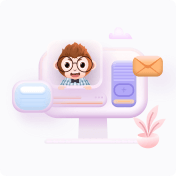
评论0