package com.game;
import android.app.Activity;
import android.content.SharedPreferences;
import android.util.Log;
public class Player
{
private GameView gameView;
private GameActivity activity;//保存Activity对象,用于保存数据操作
Ball temp;
private static final int BASENUM = 4;
private static int maxScore;//该游戏历史最高分
private int currentScore = 0;//玩家的当前分数
private int currentBallCount = 0;//当前玩家有多少个球
private static final int ROW = 9;//球盘的行数
private static final int COLUMN = 9;//球盘的列数
private static final int COLORCOUNT = 5;//色球颜色的总数
private Ball[][] map;//map代表球盘,每一元素指向一个色球
private int[][] model;//抽象map模型
private Coordinate[] path;//移动的路径
private Queue[]queue;//队列
private int front = -1;//对头
private int rear = -1;//队尾
int[] tipColor = new int[3];
public Player(GameActivity activity, GameView gameView)
{
this.gameView = gameView;
map = new Ball[ROW][COLUMN];
/*
* 读取该游戏的历史最高分
*/
this.activity = activity;
SharedPreferences setting = this.activity.getPreferences(Activity.MODE_PRIVATE);
maxScore = setting.getInt("MAXSCORE", 0);
}
//游戏开始
public void gameBegin()
{
produceInitBall(5);//刚进入游戏在球盘上产生5个球
prodeceTipColor();
}
public void prodeceTipColor()
{
int i = 0;
do
{
tipColor[i] = (int)(Math.random() * COLORCOUNT) + 1;
System.out.println("tipColor[i]==" + tipColor[i]);
i++;
} while(i < 3);
}
//产生count所指定个数的球 返回实际产生的球的个数
public void produceInitBall(int count)
{
int mCount = 0;
int row, column, color;
do
{
row = (int)(Math.random() * ROW);
column = (int)(Math.random() * COLUMN);
if(map[row][column] == null)
{
color = (int)(Math.random() * COLORCOUNT) + 1;
map[row][column] = new Ball();
map[row][column].setBallColor(color);
map[row][column].setRowIndex(row);
map[row][column].setColumnIndex(column);
mCount++;
this.currentBallCount++;
}
} while(mCount < count);
}
public int produceBall(int count)
{
int mCount = 0;
int row, column, color;
int max = ROW * COLUMN;
System.out.println("produce ing");
do
{
if(this.currentBallCount >= max)//首先判断当前有多少个球存在 如果当前的球超过了或者等于81个,返回
{
break;
}
row = (int)(Math.random() * ROW);
column = (int)(Math.random() * COLUMN);
if(map[row][column] == null)
{
color = tipColor[mCount];
map[row][column] = new Ball();
map[row][column].setBallColor(color);
map[row][column].setRowIndex(row);
map[row][column].setColumnIndex(column);
mCount++;
this.currentBallCount++;
this.clearUpBall(map[row][column]);
}
} while(mCount < count);
prodeceTipColor();
System.out.println("currentBallCount==" + currentBallCount);
return mCount;
}
//检查给定参数所代表的位置有没有色球
public boolean checkBall(int row, int column)
{
if(map[row][column] == null)
{
return false;
}
else
{
return true;
}
}
//游戏结束
public void gameOver()
{
setMaxScore(this.currentScore, Player.maxScore);
}
//获取该游戏历史最高分
public int getMaxScore()
{
return maxScore;
}
//退出游戏时设置最高分
public void setMaxScore(int currentScore, int maxScore)
{
if(currentScore > maxScore)
{
SharedPreferences setting = this.activity.getPreferences(Activity.MODE_PRIVATE);
SharedPreferences.Editor editor = setting.edit();
editor.putInt("MAXSCORE", currentScore);
if(editor.commit())
{
Log.i("game", "save successfully");
}
}
}
//获取玩家当前的分数
public int getCurrentScore()
{
return currentScore;
}
//设置玩家的当前分数
public void setScore(int count)
{
this.currentScore = this.currentScore + (10 + (count - 5) * 5);
System.out.println("currentScore==" + currentScore);
}
public int getCurrentBallCount()
{
return this.currentBallCount;
}
public Ball getBall(int row, int column)
{
return map[row][column];
}
public boolean moveBall(Ball from, int row, int column)
{
model = new int[ROW][COLUMN];
this.getModel();
queue = new Queue[ROW * COLUMN];
front = -1;
rear = -1;
if(this.searchPath(from, row, column) == true)
{
//在gameview上移动球
gameView.setStatus(1);
temp = new Ball();
temp.setBallColor(from.getBallColor());
temp.setRowIndex(row);
temp.setColumnIndex(column);
map[from.getRowIndex()][from.getColumnindex()] = null;
return true;
}
else
{
return false;
}
}
//消去相同的色球
public int clearUpBall(Ball ball)
{
int count = 0;
count = count + clearDirctionOne(ball);
count = count + clearDirctionTwo(ball);
count = count + clearDirctionThree(ball);
count = count + clearDirctionFour(ball);
if(count > 0)
{
count++;
map[ball.getRowIndex()][ball.getColumnindex()] = null;
this.currentBallCount = this.currentBallCount - count;
}
return count;
}
//消除方向1上面的色球
public int clearDirctionOne(Ball ball)
{
int leftCount = 0, rightCount = 0, count = 0;
int row, column, i;
int color;
/*
* 先去的当前要检查的球的颜色和坐标
*/
color = ball.getBallColor();
row = ball.getRowIndex();
column = ball.getColumnindex();
//先检查右边的四个球row不变column递增
for(i = 1; i <= BASENUM; i++)
{
column = column + 1;
if(column >= COLUMN)/* 判读column是否越界 */
{
break;
}
if(map[row][column]== null)
{
break;
}
else if(map[row][column].getBallColor() == color)
{
rightCount++;
}
else
{
break;
}
}
column = ball.getColumnindex();
//检查左边的4个球row不变column递减
for(i = 1; i <= BASENUM; i++)
{
column = column - 1;
if(column < 0)/* 判读column是否越界 */
{
break;
}
if(map[row][column]== null)
{
break;
}
else if(map[row][column].getBallColor() == color)
{
leftCount++;
}
else
{
break;
}
}
count = leftCount + rightCount;
if(count < BASENUM)/*满足消去条件的球的个数不足4个 */
{
return 0;
}
else
{
column = ball.getColumnindex();
for(i = 1; i <= rightCount; i++)
{
column = column + 1;
map[row][column] = null;
}
column = ball.getColumnindex();
for(i = 1; i <= leftCount; i++)
{
column = column - 1;
map[row][column] = null;
}
}
return count;/* 返回消去的球的个数 */
}
//消除方向2上面的色球
public int clearDirctionTwo(Ball ball)
{
int upCount = 0, downCount = 0, count = 0;
int row, column, i;
int color;
/*
* 先去的当前要检查的球的颜色和坐标
*/
color = ball.getBallColor();
row = ball.getRowIndex();
column = ball.getColumnindex();
//先检查当前球上方的四个球row递减column不变
for(i = 1; i <= BASENUM; i++)
{
row = row - 1;
if(row < 0)/* 判读row是否越界 */
{
break;
}
if(map[row][column]== null)
{
break;
}
else if(map[row][column].getBallColor() == color)
{
upCount++;
}
else
{
break;
}
}
row = ball.getRowIndex();
//检查当前球下面的4个球row递增column不变
for(i = 1; i <= BASENUM; i++)
{
row = row + 1;
if(row >= ROW)/* 判读column是否越界 */
{
break;
}
if(map[row][column]== null)
{
break;
}
else if(map[row][column].getBallColor() == color)
{
downCount++;
}
else
{
break;
}
}
count = upCount + downCount;
if(count < BASENUM)/*满足消去条件的球
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
基于andorid平台的游戏 五彩连珠 代码基本都有注释的,能运行,没BUG的,这是我的论文课题。论文就不传上来了。可以给大家参考一下。 另外请注意以为上传的东西不能大于15M所以我吧游戏的背景音乐删除了,请下载的朋友下载之后解压之后在: \mgame\res\raw目录下放置一手MP3音乐文件,音乐文件名字请务必改为bkmusic.mp3,说的这么清楚了还不明白我也很无语的哦。。。。。 游戏画面介绍: 画面中间是游戏区,9行9列共81个方格;左下方是最高分纪录,右下方是本次游戏的计分情况。 游戏规则: 点击彩球移动到期望的位置,每移动一次,画面将随机出现3个新的彩球; 当同一颜色的彩球连成5个一行或一列或一斜线时,这5个彩球同时消失,游戏得分10分,同时本次不产生球。 当画面上每个方格都被彩球占满时,游戏结束,要想决定获得更高分就要使彩球消失的速度尽量快,以延长游戏时间和空间。
资源推荐
资源详情
资源评论
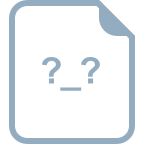
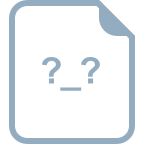
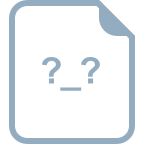
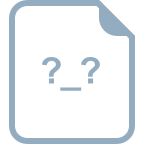
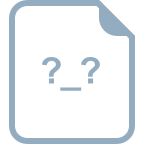
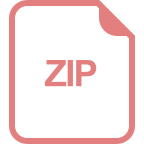
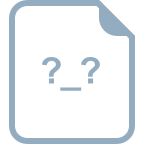
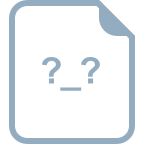
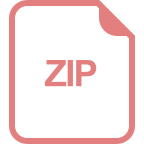
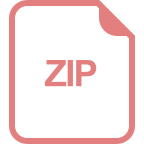
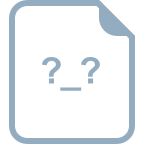
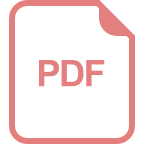
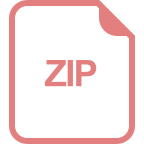
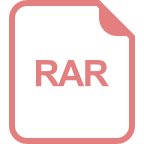
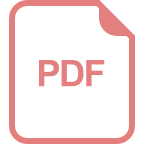
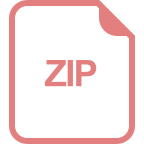
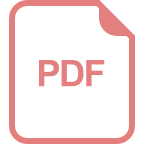
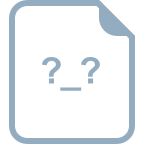
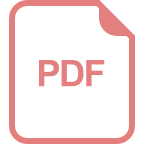
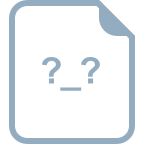
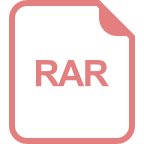
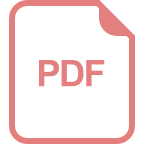
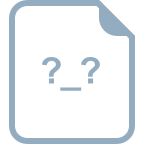
收起资源包目录


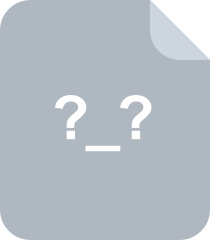
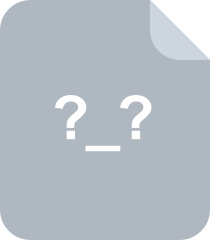

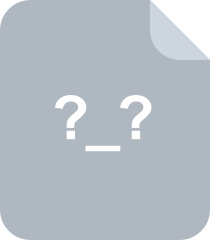


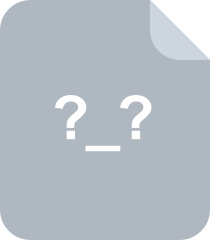
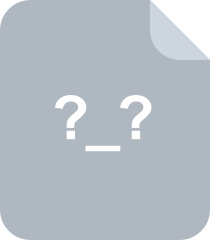
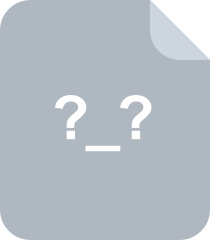
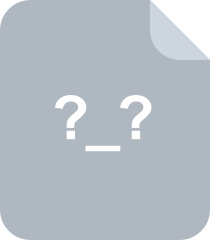
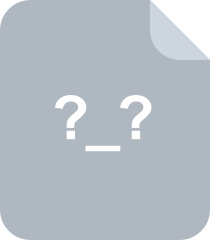
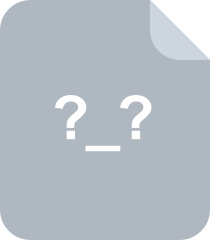
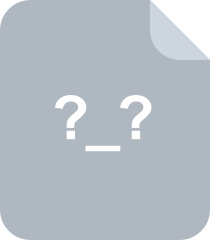
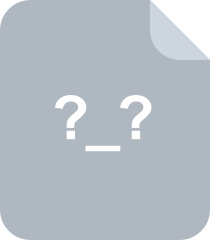
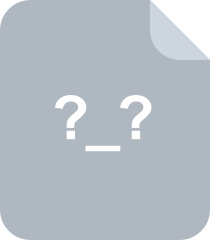
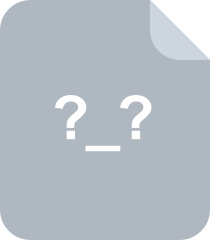
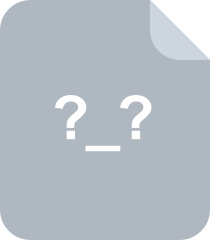
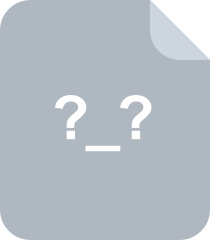
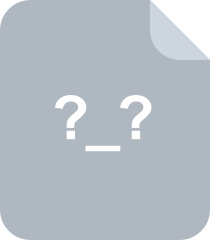
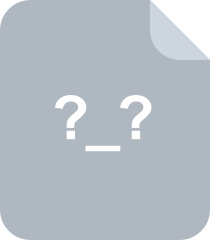
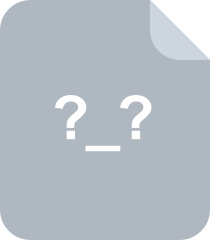
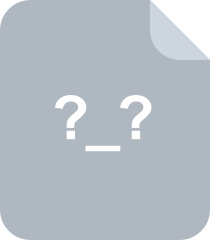
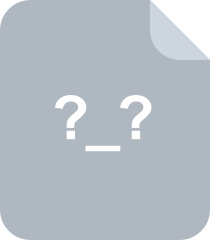
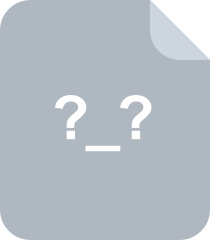
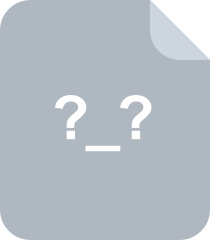
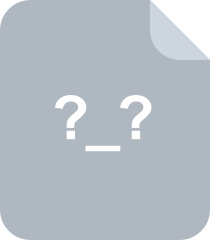
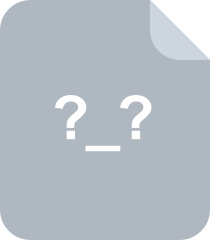



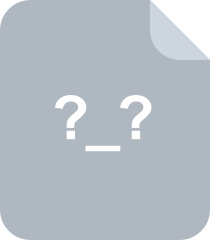
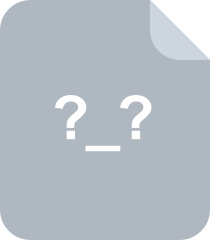
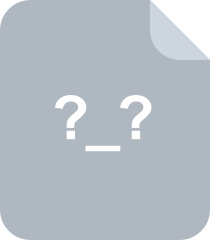
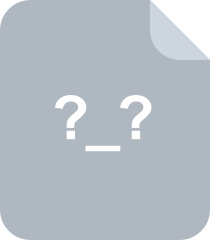
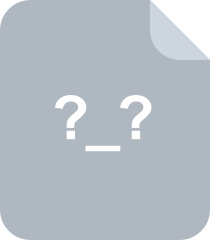
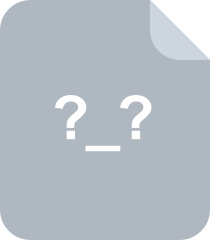
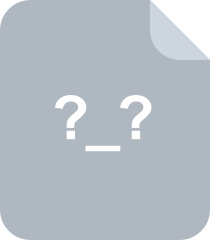
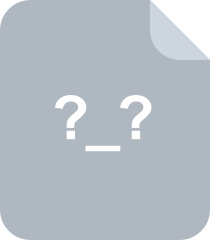
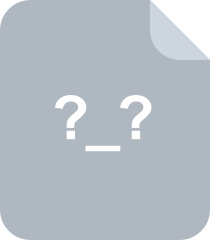


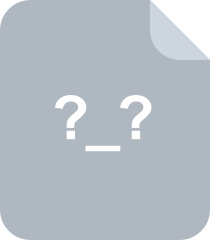

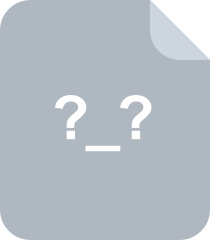




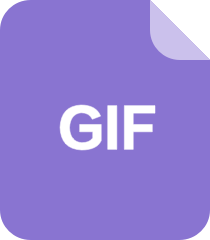
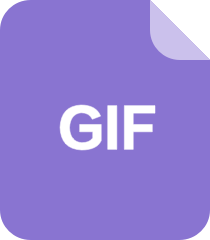
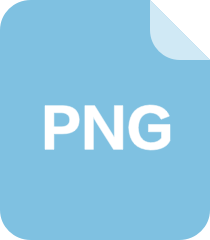
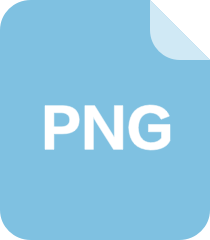
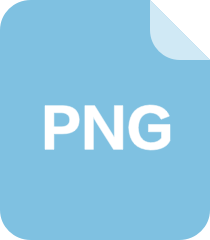
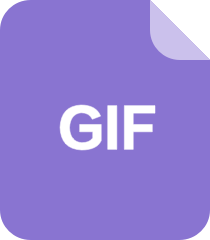
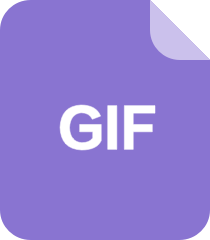
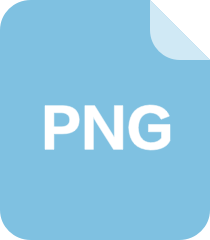
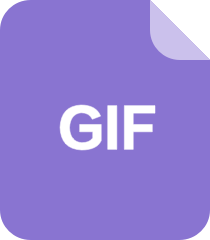
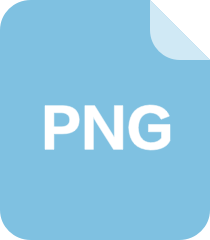
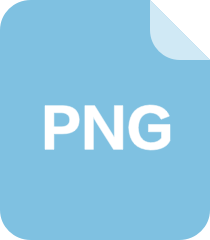
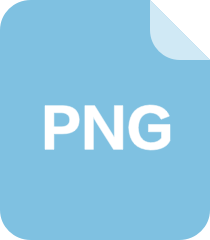
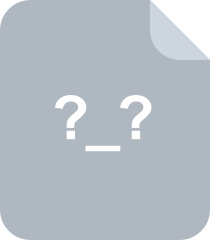



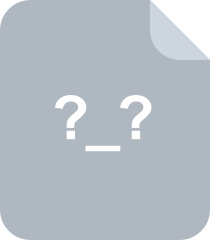

共 49 条
- 1
资源评论
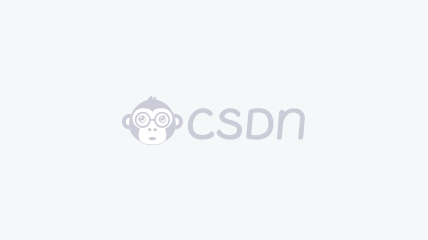
- liucccggg2012-09-13很有用,我参看这个游戏做了一个连连看一类的游戏。
- lindir2012-08-15非常给力,代码很清晰易懂
- daolangdesign2012-11-13可以用,就是游戏不太好玩。研究是有用的
- cs043182012-10-27可以用,代码很清晰易懂

ndl1302732
- 粉丝: 17
- 资源: 8
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

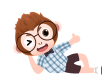
最新资源
- (源码)基于Spring Cloud和Spring Security的微服务权限管理系统.zip
- (源码)基于Java和Jsoup的教务系统爬虫工具.zip
- (源码)基于Spring Boot和Vue的后台权限管理系统.zip
- 坚牢黄玉matlab gui平台的dsp实验平台设计
- 【java毕业设计】五台山景点购票系统源码(ssm+mysql+说明文档+LW).zip
- (源码)基于JFinal框架的Blog管理系统.zip
- 系统架构设计师 历年真题及答案详解一.pdf
- 人物专注性检测《基于深度学习的驾驶员分心驾驶行为(疲劳+危险行为)预警系统【YOLOv5+Deepsort】》+源码+说明
- C#ASP.NET公司年会抽奖程序源码数据库 Access源码类型 WebForm
- (源码)基于SQLite和C++的项目管理系统.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


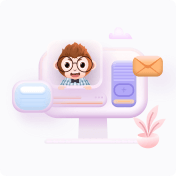
安全验证
文档复制为VIP权益,开通VIP直接复制
