[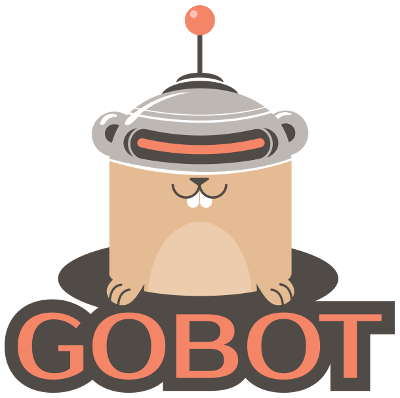](http://gobot.io/)
[](https://godoc.org/gobot.io/x/gobot/v2)
[](https://circleci.com/gh/hybridgroup/gobot/tree/dev)
[](https://ci.appveyor.com/project/deadprogram/gobot/branch/dev)
[](https://codecov.io/gh/hybridgroup/gobot)
[](https://goreportcard.com/report/hybridgroup/gobot)
[](https://github.com/hybridgroup/gobot/blob/master/LICENSE.txt)
Gobot (<https://gobot.io/>) is a framework using the Go programming language (<https://golang.org/>) for robotics, physical
computing, and the Internet of Things.
It provides a simple, yet powerful way to create solutions that incorporate multiple, different hardware devices at the
same time.
Want to run Go directly on microcontrollers? Check out our sister project TinyGo (<https://tinygo.org/>)
## Getting Started
### Get in touch
Get the Gobot source code by running this commands:
```sh
git clone https://github.com/hybridgroup/gobot.git
git checkout release
```
Afterwards have a look at the [examples directory](./examples). You need to find an example matching your platform for your
first test (e.g. "raspi_blink.go"). Than build the binary (cross compile), transfer it to your target and run it.
`env GOOS=linux GOARCH=arm GOARM=5 go build -o ./output/my_raspi_bink examples/raspi_blink.go`
> Building the code on your local machine with the example code above will create a binary for ARMv5. This is probably not
> what you need for your specific target platform. Please read also the platform specific documentation in the platform
> subfolders.
### Create your first project
Create a new folder and a new Go module project.
```sh
mkdir ~/my_gobot_example
cd ~/my_gobot_example
go mod init my.gobot.example.com
```
Copy your example file besides the go.mod file, import the requirements and build.
```sh
cp /<path to gobot folder>/examples/raspi_blink.go ~/my_gobot_example/
go mod tidy
env GOOS=linux GOARCH=arm GOARM=5 go build -o ./output/my_raspi_bink raspi_blink.go
```
Now you are ready to modify the example and test your changes. Start by removing the build directives at the beginning
of the file.
## Examples
### Gobot with Arduino
```go
package main
import (
"time"
"gobot.io/x/gobot/v2"
"gobot.io/x/gobot/v2/drivers/gpio"
"gobot.io/x/gobot/v2/platforms/firmata"
)
func main() {
firmataAdaptor := firmata.NewAdaptor("/dev/ttyACM0")
led := gpio.NewLedDriver(firmataAdaptor, "13")
work := func() {
gobot.Every(1*time.Second, func() {
led.Toggle()
})
}
robot := gobot.NewRobot("bot",
[]gobot.Connection{firmataAdaptor},
[]gobot.Device{led},
work,
)
robot.Start()
}
```
### Gobot with Sphero
```go
package main
import (
"fmt"
"time"
"gobot.io/x/gobot/v2"
"gobot.io/x/gobot/v2/platforms/sphero"
)
func main() {
adaptor := sphero.NewAdaptor("/dev/rfcomm0")
driver := sphero.NewSpheroDriver(adaptor)
work := func() {
gobot.Every(3*time.Second, func() {
driver.Roll(30, uint16(gobot.Rand(360)))
})
}
robot := gobot.NewRobot("sphero",
[]gobot.Connection{adaptor},
[]gobot.Device{driver},
work,
)
robot.Start()
}
```
### "Metal" Gobot
You can use the entire Gobot framework as shown in the examples above ("Classic" Gobot), or you can pick and choose from
the various Gobot packages to control hardware with nothing but pure idiomatic Golang code ("Metal" Gobot). For example:
```go
package main
import (
"gobot.io/x/gobot/v2/drivers/gpio"
"gobot.io/x/gobot/v2/platforms/intel-iot/edison"
"time"
)
func main() {
e := edison.NewAdaptor()
e.Connect()
led := gpio.NewLedDriver(e, "13")
led.Start()
for {
led.Toggle()
time.Sleep(1000 * time.Millisecond)
}
}
```
### "Master" Gobot
You can also use the full capabilities of the framework aka "Master Gobot" to control swarms of robots or other features
such as the built-in API server. For example:
```go
package main
import (
"fmt"
"time"
"gobot.io/x/gobot/v2"
"gobot.io/x/gobot/v2/api"
"gobot.io/x/gobot/v2/platforms/sphero"
)
func NewSwarmBot(port string) *gobot.Robot {
spheroAdaptor := sphero.NewAdaptor(port)
spheroDriver := sphero.NewSpheroDriver(spheroAdaptor)
spheroDriver.SetName("Sphero" + port)
work := func() {
spheroDriver.Stop()
spheroDriver.On(sphero.Collision, func(data interface{}) {
fmt.Println("Collision Detected!")
})
gobot.Every(1*time.Second, func() {
spheroDriver.Roll(100, uint16(gobot.Rand(360)))
})
gobot.Every(3*time.Second, func() {
spheroDriver.SetRGB(uint8(gobot.Rand(255)),
uint8(gobot.Rand(255)),
uint8(gobot.Rand(255)),
)
})
}
robot := gobot.NewRobot("sphero",
[]gobot.Connection{spheroAdaptor},
[]gobot.Device{spheroDriver},
work,
)
return robot
}
func main() {
master := gobot.NewMaster()
api.NewAPI(master).Start()
spheros := []string{
"/dev/rfcomm0",
"/dev/rfcomm1",
"/dev/rfcomm2",
"/dev/rfcomm3",
}
for _, port := range spheros {
master.AddRobot(NewSwarmBot(port))
}
master.Start()
}
```
## Hardware Support
Gobot has a extensible system for connecting to hardware devices. The following robotics and physical computing
platforms are currently supported:
- [Arduino](http://www.arduino.cc/) <=> [Package](https://github.com/hybridgroup/gobot/tree/master/platforms/firmata)
- Audio <=> [Package](https://github.com/hybridgroup/gobot/tree/master/platforms/audio)
- [Beaglebone Black](http://beagleboard.org/boards) <=> [Package](https://github.com/hybridgroup/gobot/tree/master/platforms/beaglebone)
- [Beaglebone PocketBeagle](http://beagleboard.org/pocket/) <=> [Package](https://github.com/hybridgroup/gobot/tree/master/platforms/beaglebone)
- [Bluetooth LE](https://www.bluetooth.com/what-is-bluetooth-technology/bluetooth-technology-basics/low-energy) <=> [Package](https://github.com/hybridgroup/gobot/tree/master/platforms/ble)
- [C.H.I.P](http://www.nextthing.co/pages/chip) <=> [Package](https://github.com/hybridgroup/gobot/tree/master/platforms/chip)
- [C.H.I.P Pro](https://docs.getchip.com/chip_pro.html) <=> [Package](https://github.com/hybridgroup/gobot/tree/master/platforms/chip)
- [Digispark](http://digistump.com/products/1) <=> [Package](https://github.com/hybridgroup/gobot/tree/master/platforms/digispark)
- [DJI Tello](https://www.ryzerobotics.com/tello) <=> [Package](https://github.com/hybridgroup/gobot/tree/master/platforms/dji/tello)
- [DragonBoard](https://developer.qualcomm.com/hardware/dragonboard-410c) <=> [Package](https://github.com/hybridgroup/gobot/tree/master/platforms/dragonboard)
- [ESP8266](http://esp8266.net/) <=> [Package](https://github.com/hybridgroup/gobot/tree/master/platforms/firmata)
- [GoPiGo 3](https://www.dexterindustries.com/gopigo3/) <=> [Package](https://github.com/hybridgroup/gobot/tree/master/platforms/dexter/gopigo3)
- [Intel Curie](https://www.intel.com/content/www/us/en/products/boards-kits/curie.html) <=> [Package](https://github.com/hybridgroup/gobot/tree/master/platforms/intel-iot/curie)
- [Intel Edison](http://www.intel.com/content/www/us/en/do-it-yourself/edison.html) <=> [Package](https://github.com/hybridgroup/gobot/tree/master/platforms/intel-iot/edison)
- [Intel Joule](http://intel.com/joule/getstarted) <=> [Package](https://github.com/hybridgroup/gobot/tree/master/platforms/intel-iot/joule)
- [Jetson Nano](https://developer.nvidia.com/embedded/jetson-nano/) <=>


新华
- 粉丝: 1w+
- 资源: 628
最新资源
- 前端分析-2023071100789
- 基于纯手工HTML与CSS构建的田东家乡介绍网站设计源码
- 基于鸿蒙操作系统的芯片评估板自检系统源码
- manatee电磁噪声振动计算softwareManatee 1.09 电机电磁振动噪声NVH终结者 带教程,带教程,带教程重要的话说3遍 史上最强后处理软件,甩jmag、Maxwell、flux几
- 基于Python的Django框架开发的subaoApi设计源码
- 基于Owin+融云的LayIM3.0 .NET版本设计源码
- 单向光伏并网逆变器 图一单向光伏并网逆变器整体结构图 图二并网电流与电压曲线图 图三mppt控制最大功率追踪图 图四直流母线电压曲线图
- 基于Python Django框架的旅游网站后端设计源码
- 基于Kotlin语言的Gradle统一依赖管理设计源码
- 基于PyQt5框架的ExcelDiffer设计源码
- COMSOL裂缝地层的THM耦合,离散裂缝模型,随机复杂裂缝,适合地热能研究 增强地热系统,热流固耦合的开采过程
- 基于2020.02.06快照的xadmin JavaScript/Python/HTML/CSS/Shell五语言混合设计源码
- 基于Vue框架的计算机协会招新系统前端微服务设计源码
- BUCK多种控制策略对比 图一BUCK主电路图与控制策略方法 图二采用开环控制波形 图三开环调节过程 图四单电压闭环控制波形 图五单电压调节过程
- 永磁同步电机(PMSM)MATLAB仿真 直接转矩控制 转速外环 转矩跟磁链内环控制 转矩脉动去下图 能够明显减小电机转矩脉动
- 基于JavaScript的UscIoV跨链交互区块链平台设计源码
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


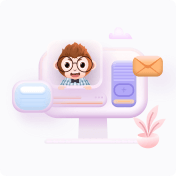