libpng-manual.txt - A description on how to use and modify libpng
libpng version 1.5.1 - February 3, 2011
Updated and distributed by Glenn Randers-Pehrson
<glennrp at users.sourceforge.net>
Copyright (c) 1998-2011 Glenn Randers-Pehrson
This document is released under the libpng license.
For conditions of distribution and use, see the disclaimer
and license in png.h
Based on:
libpng versions 0.97, January 1998, through 1.5.1 - February 3, 2011
Updated and distributed by Glenn Randers-Pehrson
Copyright (c) 1998-2011 Glenn Randers-Pehrson
libpng 1.0 beta 6 version 0.96 May 28, 1997
Updated and distributed by Andreas Dilger
Copyright (c) 1996, 1997 Andreas Dilger
libpng 1.0 beta 2 - version 0.88 January 26, 1996
For conditions of distribution and use, see copyright
notice in png.h. Copyright (c) 1995, 1996 Guy Eric
Schalnat, Group 42, Inc.
Updated/rewritten per request in the libpng FAQ
Copyright (c) 1995, 1996 Frank J. T. Wojcik
December 18, 1995 & January 20, 1996
I. Introduction
This file describes how to use and modify the PNG reference library
(known as libpng) for your own use. There are five sections to this
file: introduction, structures, reading, writing, and modification and
configuration notes for various special platforms. In addition to this
file, example.c is a good starting point for using the library, as
it is heavily commented and should include everything most people
will need. We assume that libpng is already installed; see the
INSTALL file for instructions on how to install libpng.
For examples of libpng usage, see the files "example.c", "pngtest.c",
and the files in the "contrib" directory, all of which are included in
the libpng distribution.
Libpng was written as a companion to the PNG specification, as a way
of reducing the amount of time and effort it takes to support the PNG
file format in application programs.
The PNG specification (second edition), November 2003, is available as
a W3C Recommendation and as an ISO Standard (ISO/IEC 15948:2003 (E)) at
<http://www.w3.org/TR/2003/REC-PNG-20031110/
The W3C and ISO documents have identical technical content.
The PNG-1.2 specification is available at
<http://www.libpng.org/pub/png/documents/>. It is technically equivalent
to the PNG specification (second edition) but has some additional material.
The PNG-1.0 specification is available
as RFC 2083 <http://www.libpng.org/pub/png/documents/> and as a
W3C Recommendation <http://www.w3.org/TR/REC.png.html>.
Some additional chunks are described in the special-purpose public chunks
documents at <http://www.libpng.org/pub/png/documents/>.
Other information
about PNG, and the latest version of libpng, can be found at the PNG home
page, <http://www.libpng.org/pub/png/>.
Most users will not have to modify the library significantly; advanced
users may want to modify it more. All attempts were made to make it as
complete as possible, while keeping the code easy to understand.
Currently, this library only supports C. Support for other languages
is being considered.
Libpng has been designed to handle multiple sessions at one time,
to be easily modifiable, to be portable to the vast majority of
machines (ANSI, K&R, 16-, 32-, and 64-bit) available, and to be easy
to use. The ultimate goal of libpng is to promote the acceptance of
the PNG file format in whatever way possible. While there is still
work to be done (see the TODO file), libpng should cover the
majority of the needs of its users.
Libpng uses zlib for its compression and decompression of PNG files.
Further information about zlib, and the latest version of zlib, can
be found at the zlib home page, <http://www.info-zip.org/pub/infozip/zlib/>.
The zlib compression utility is a general purpose utility that is
useful for more than PNG files, and can be used without libpng.
See the documentation delivered with zlib for more details.
You can usually find the source files for the zlib utility wherever you
find the libpng source files.
Libpng is thread safe, provided the threads are using different
instances of the structures. Each thread should have its own
png_struct and png_info instances, and thus its own image.
Libpng does not protect itself against two threads using the
same instance of a structure.
II. Structures
There are two main structures that are important to libpng, png_struct
and png_info. Both are internal structures that are no longer exposed
in the libpng interface (as of libpng 1.5.0).
The png_info structure is designed to provide information about the
PNG file. At one time, the fields of png_info were intended to be
directly accessible to the user. However, this tended to cause problems
with applications using dynamically loaded libraries, and as a result
a set of interface functions for png_info (the png_get_*() and png_set_*()
functions) was developed.
The png_struct structure is the object used by the library to decode a
single image. As of 1.5.0 this structure is also not exposed.
Almost all libpng APIs require a pointer to a png_struct as the first argument.
Many (in particular the png_set and png_get APIs) also require a pointer
to png_info as the second argument. Some application visible macros
defined in png.h designed for basic data access (reading and writing
integers in the PNG format) break this rule, but it's almost always safe
to assume that a (png_struct*) has to be passed to call an API function.
The png.h header file is an invaluable reference for programming with libpng.
And while I'm on the topic, make sure you include the libpng header file:
#include <png.h>
Types
The png.h header file defines a number of integral types used by the
APIs. Most of these are fairly obvious; for example types corresponding
to integers of particular sizes and types for passing color values.
One exception is how non-integral numbers are handled. For application
convenience most APIs that take such numbers have C (double) arguments,
however internally PNG, and libpng, use 32 bit signed integers and encode
the value by multiplying by 100,000. As of libpng 1.5.0 a convenience
macro PNG_FP_1 is defined in png.h along with a type (png_fixed_point)
which is simply (png_int_32).
All APIs that take (double) arguments also have an matching API that
takes the corresponding fixed point integer arguments. The fixed point
API has the same name as the floating point one with _fixed appended.
The actual range of values permitted in the APIs is frequently less than
the full range of (png_fixed_point) (-21474 to +21474). When APIs require
a non-negative argument the type is recorded as png_uint_32 above. Consult
the header file and the text below for more information.
Special care must be take with sCAL chunk handling because the chunk itself
uses non-integral values encoded as strings containing decimal floating point
numbers. See the comments in the header file.
Configuration
The main header file function declarations are frequently protected by C
preprocessing directives of the form:
#ifdef PNG_feature_SUPPORTED
declare-function
#endif
The library can be built without support for these APIs, although a
standard build will have all implemented APIs. Application programs
should check the feature macros before using an API for maximum
portability. From libpng 1.5.0 the feature macros set during the build
of libpng are recorded in the header file "pnglibconf.h" and this file
is always included by png.h.
If you don't need to change the library configuration from the default skip to
the next section ("Reading").
Notice that some of the makefiles in the 'scripts' directory and (in 1.5.0) all
of the build project files in the 'projects' directory simply copy
scripts/pnglibconf.h.prebuilt to pnglibconf.h. This means that these build
systems do not permit easy auto-configuration of the library - they only
support the default configuration.
The easiest way to make minor changes to the libpng configuration when
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
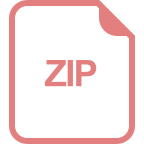
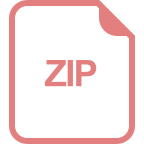
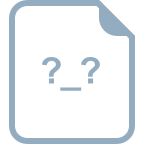
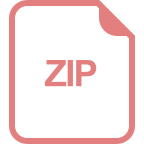
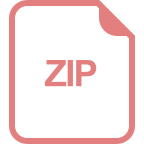
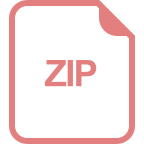
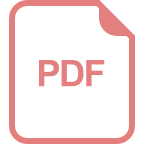
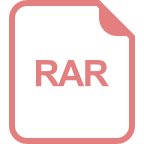
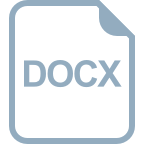
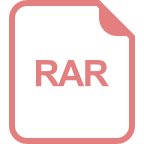
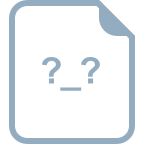
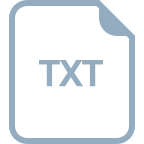
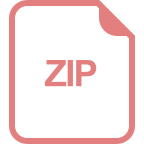
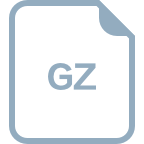
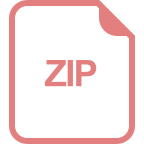
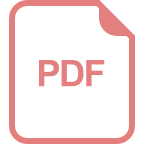
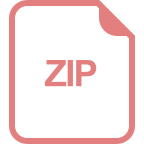
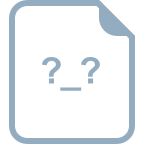
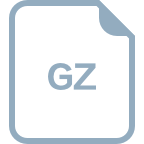
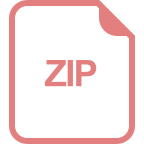
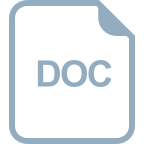
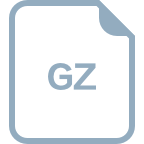
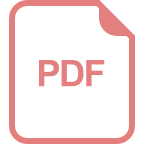
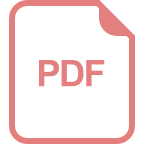
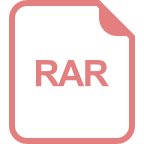
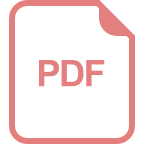
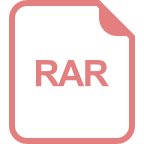
收起资源包目录

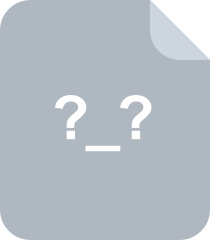
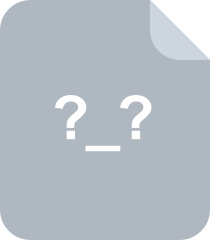
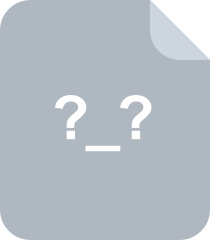
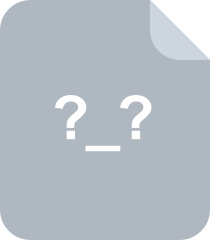
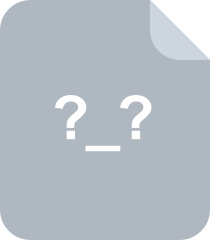
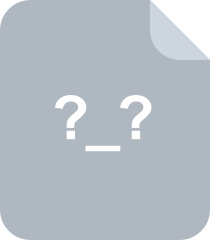
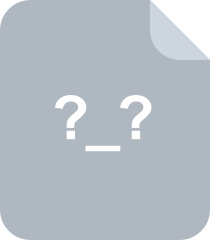
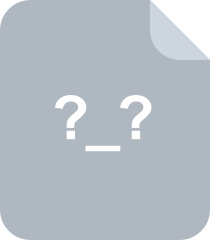
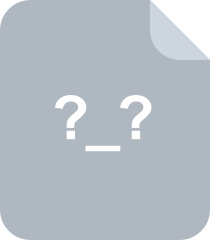
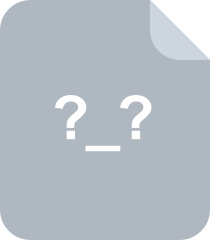
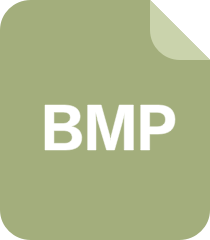
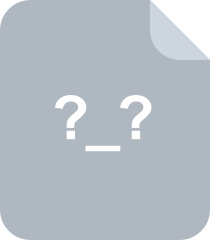
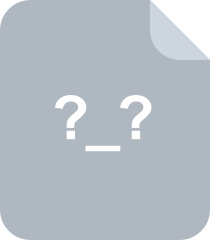
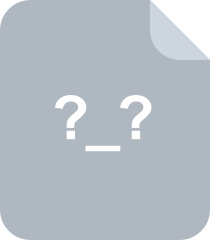
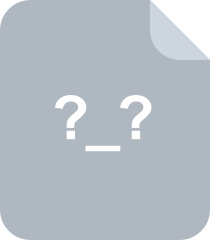
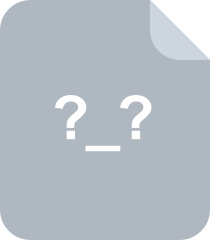
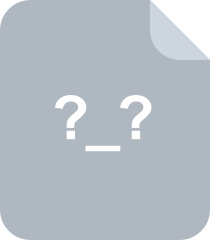
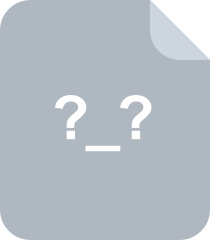
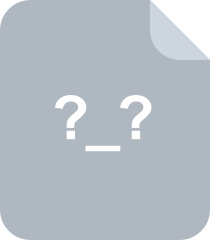
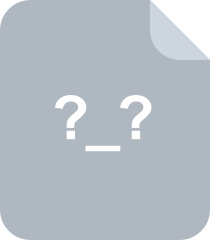
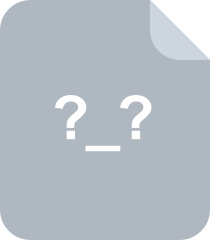
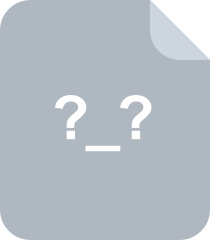
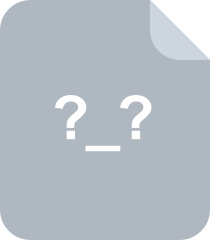
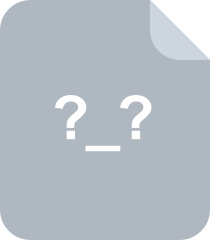
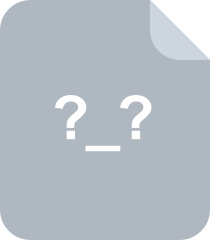
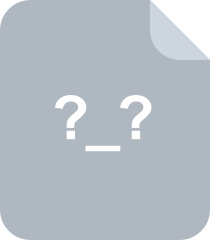
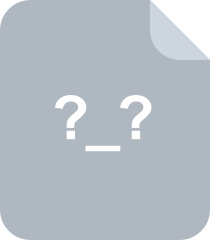
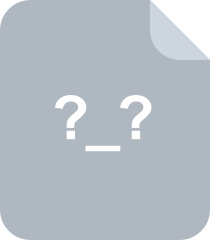
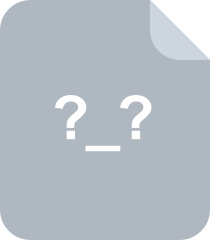
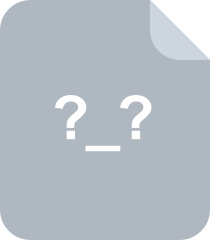
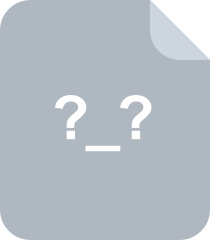
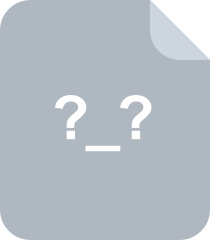
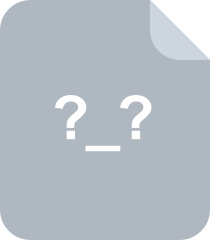
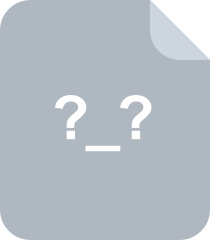
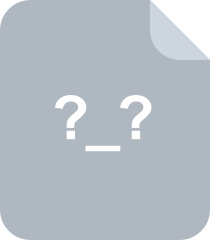
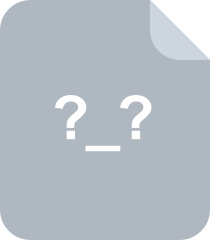
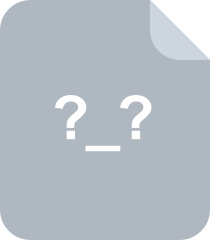
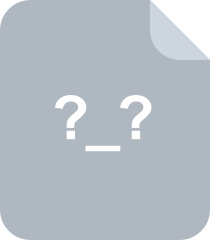
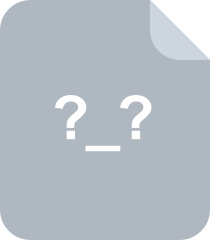
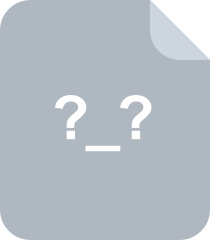
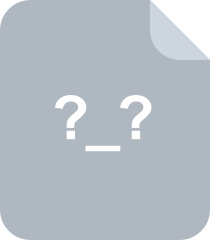
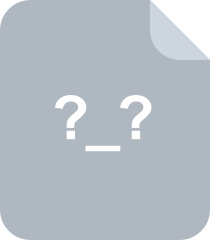
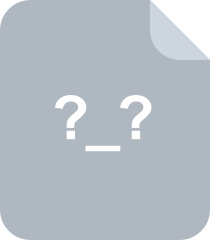
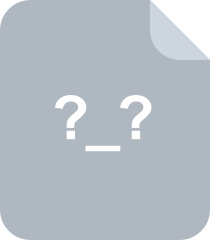
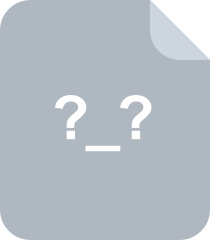
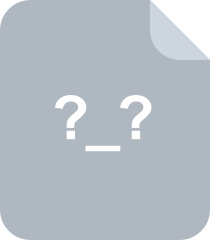
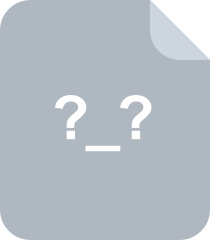
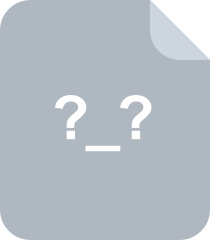
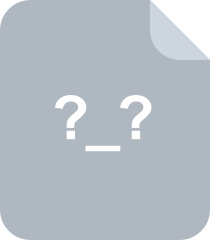
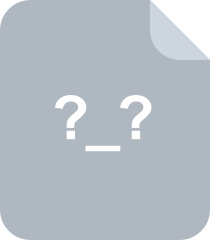
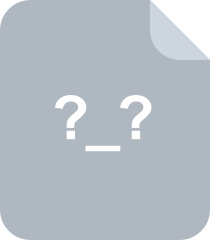
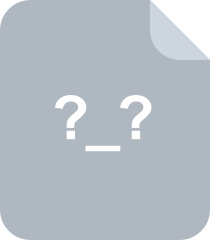
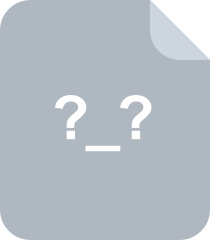
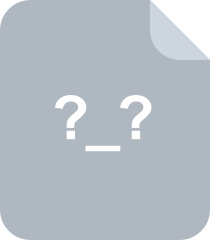
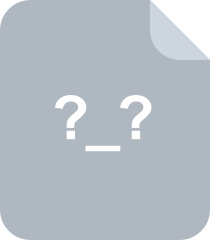
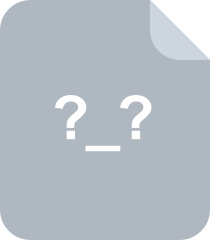
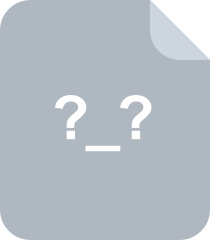
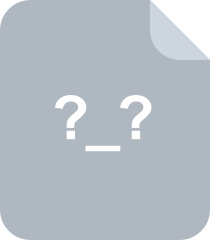
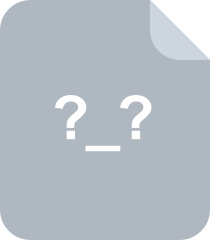
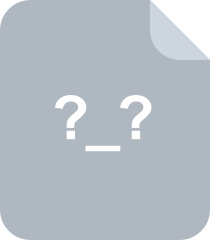
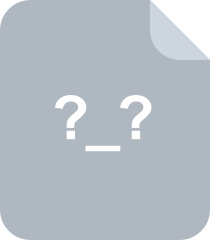
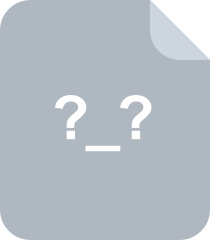
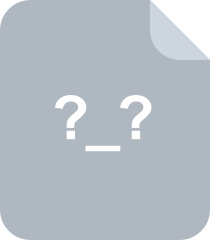
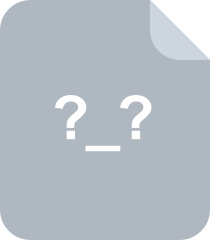
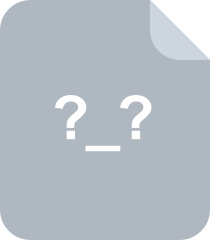
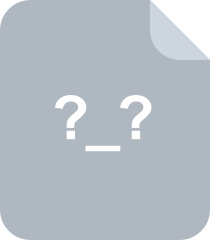
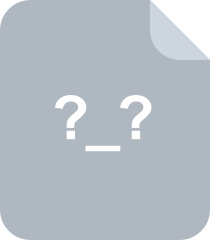
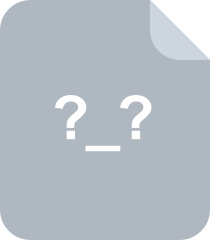
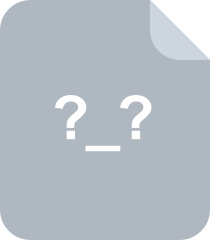
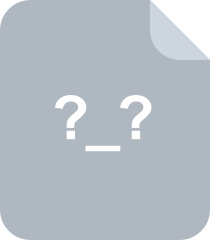
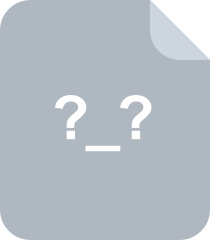
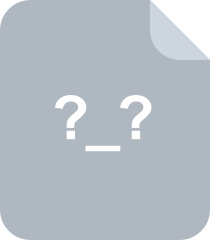
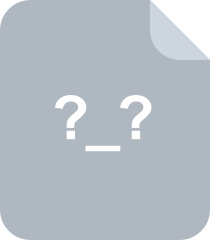
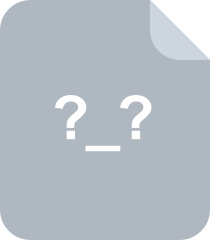
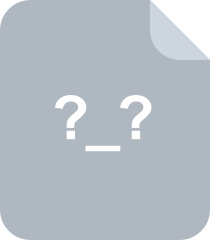
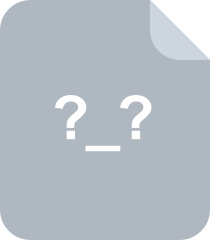
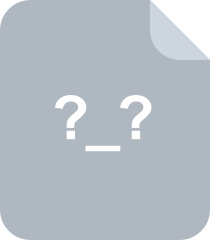
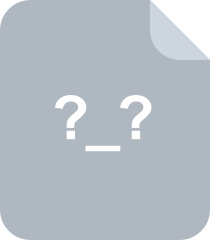
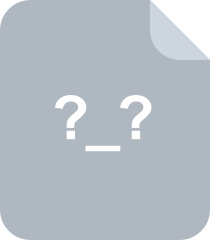
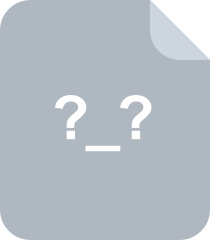
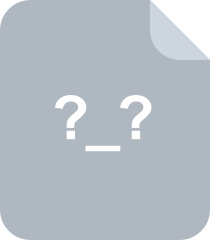
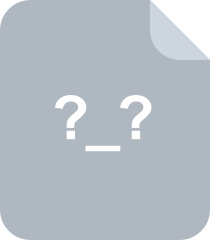
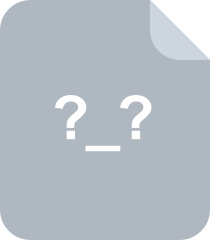
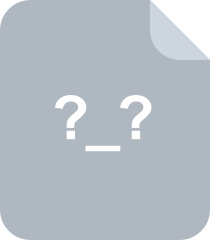
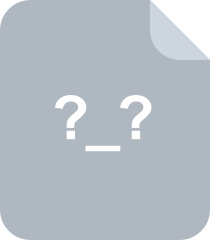
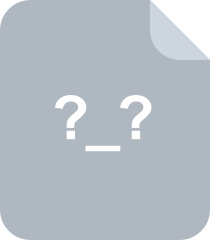
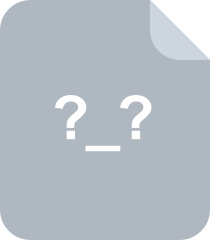
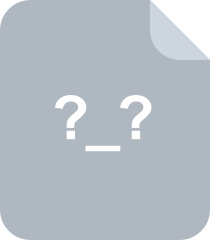
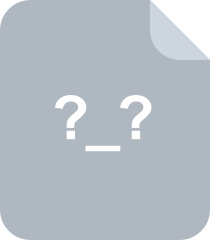
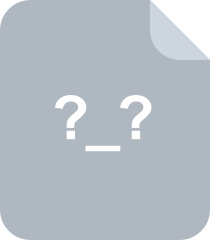
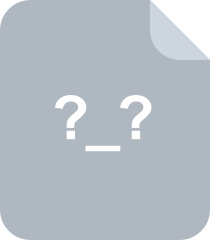
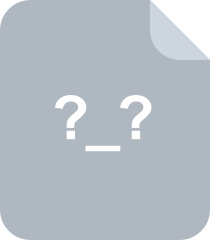
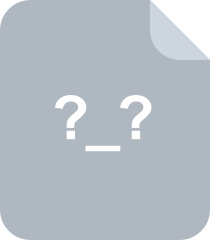
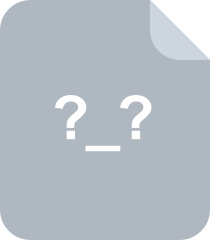
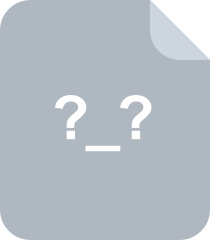
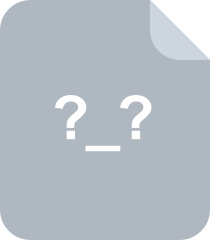
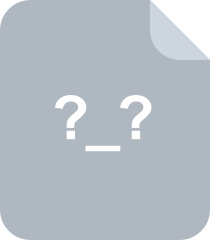
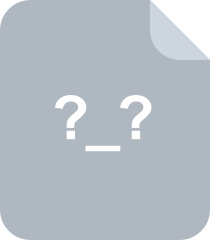
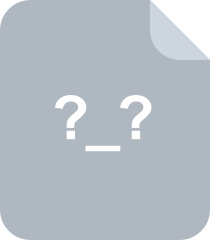
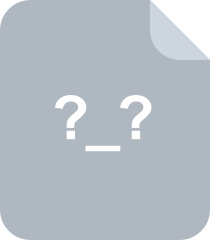
共 1221 条
- 1
- 2
- 3
- 4
- 5
- 6
- 13
资源评论
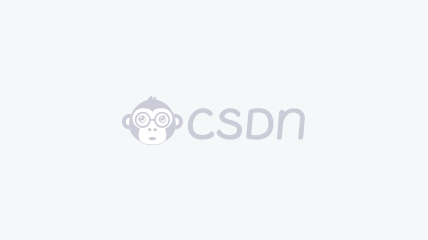


新华
- 粉丝: 1w+
- 资源: 628
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

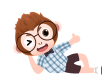
最新资源
- java超市订单管理系统源码数据库 MySQL源码类型 WebForm
- 记录windows安装nvm:nvm-setup-2024-11-16.exe.zip
- 同济大学数字信号处理实验(包含实验报告)
- Kettle 是Kettle E.T.T.L. Envirnonment只取首字母的缩写,这意味着它被设计用来帮助你实现你的
- java微信小程序B2C商城 H5+APP源码 前后端分离数据库 MySQL源码类型 WebForm
- matplotlib 绘制随机漫步图
- java版快速开发框架后台管理系统源码数据库 MySQL源码类型 WebForm
- Java实现植物大战僵尸简易版
- matplotlib 绘制随机漫步图
- ijkplayer播放rtsp延时越来越高处理方案
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


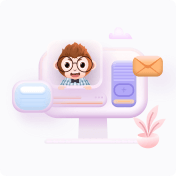
安全验证
文档复制为VIP权益,开通VIP直接复制
