s = "hello"
s.concat(" world") # Synonym for <<. Mutating append to s. Returns new s.
s.insert(5, " there") # Same as s[5] = " there". Alters s. Returns new s.
s.slice(0,5) # Same as s[0,5]. Returns a substring.
s.slice!(5,6) # Deletion. Same as s[5,6]="". Returns deleted substring.
s.eql?("hello world") # True. Same as ==.
---------------------------
s.length # => 5: counts characters in 1.9, bytes in 1.8
s.size # => 5: size is a synonym
s.bytesize # => 5: length in bytes; Ruby 1.9 only
s.empty? # => false
"".empty? # => true
---------------------------
s = "hello"
# Finding the position of a substring or pattern match
s.index('l') # => 2: index of first l in string
s.index(?l) # => 2: works with character codes as well
s.index(/l+/) # => 2: works with regular expressions, too
s.index('l',3) # => 3: index of first l in string at or after position 3
s.index('Ruby') # => nil: search string not found
s.rindex('l') # => 3: index of rightmost l in string
s.rindex('l',2) # => 2: index of rightmost l in string at or before 2
# Checking for prefixes and suffixes: Ruby 1.9 and later
s.start_with? "hell" # => true. Note singular "start" not "starts"
s.end_with? "bells" # => false
# Testing for presence of substring
s.include?("ll") # => true: "hello" includes "ll"
s.include?(?H) # => false: "hello" does not include character H
# Pattern matching with regular expressions
s =~ /[aeiou]{2}/ # => nil: no double vowels in "hello"
s.match(/[aeiou]/) {|m| m.to_s} # => "e": return first vowel
# Splitting a string into substrings based on a delimiter string or pattern
"this is it".split # => ["this", "is", "it"]: split on spaces by default
"hello".split('l') # => ["he", "", "o"]
"1, 2,3".split(/,\s*/) # => ["1","2","3"]: comma and optional space delimiter
# Split a string into two parts plus a delimiter. Ruby 1.9 only.
# These methods always return arrays of 3 strings:
"banana".partition("an") # => ["b", "an", "ana"]
"banana".rpartition("an") # => ["ban", "an", "a"]: start from right
"a123b".partition(/\d+/) # => ["a", "123", "b"]: works with Regexps, too
# Search and replace the first (sub, sub!) or all (gsub, gsub!)
# occurrences of the specified string or pattern.
# More about sub and gsub when we cover regular expressions later.
s.sub("l", "L") # => "heLlo": Just replace first occurrence
s.gsub("l", "L") # => "heLLo": Replace all occurrences
s.sub!(/(.)(.)/, '\2\1') # => "ehllo": Match and swap first 2 letters
s.sub!(/(.)(.)/, "\\2\\1") # => "hello": Double backslashes for double quotes
# sub and gsub can also compute a replacement string with a block
# Match the first letter of each word and capitalize it
"hello world".gsub(/\b./) {|match| match.upcase } # => "Hello World"
---------------------------
# Case modification methods
s = "world" # These methods work with ASCII characters only
s.upcase # => "WORLD"
s.upcase! # => "WORLD"; alter s in place
s.downcase # => "world"
s.capitalize # => "World": first letter upper, rest lower
s.capitalize! # => "World": alter s in place
s.swapcase # => "wORLD": alter case of each letter
# Case insensitive comparison. (ASCII text only)
# casecmp works like <=> and returns -1 for less, 0 for equal, +1 for greater
"world".casecmp("WORLD") # => 0
"a".casecmp("B") # => -1 (<=> returns 1 in this case)
---------------------------
s = "hello\r\n" # A string with a line terminator
s.chomp! # => "hello": remove one line terminator from end
s.chomp # => "hello": no line terminator so no change
s.chomp! # => nil: return of nil indicates no change made
s.chomp("o") # => "hell": remove "o" from end
$/ = ";" # Set global record separator $/ to semicolon
"hello;".chomp # => "hello": now chomp removes semicolons and end
# chop removes trailing character or line terminator (\n, \r, or \r\n)
s = "hello\n"
s.chop! # => "hello": line terminator removed. s modified.
s.chop # => "hell": last character removed. s not modified.
"".chop # => "": no characters to remove
"".chop! # => nil: nothing changed
# Strip all whitespace (including \t, \r, \n) from left, right, or both
# strip!, lstrip! and rstrip! modify the string in place.
s = "\t hello \n" # Whitespace at beginning and end
s.strip # => "hello"
s.lstrip # => "hello \n"
s.rstrip # => "\t hello"
# Left-justify, right-justify, or center a string in a field n-characters wide.
# There are no mutator versions of these methods. See also printf method.
s = "x"
s.ljust(3) # => "x "
s.rjust(3) # => " x"
s.center(3) # => " x "
s.center(5, '-') # => "--x--": padding other than space are allowed
s.center(7, '-=') # => "-=-x-=-": multicharacter padding allowed
---------------------------
s = "A\nB" # Three ASCII characters on two lines
s.each_byte {|b| print b, " " } # Prints "65 10 66 "
s.each_line {|l| print l.chomp} # Prints "AB"
# Sequentially iterate characters as 1-character strings
# Works in Ruby 1.9, or in 1.8 with the jcode library:
s.each_char { |c| print c, " " } # Prints "A \n B "
# Enumerate each character as a 1-character string
# This does not work for multibyte strings in 1.8
# It works (inefficiently) for multibyte strings in 1.9:
0.upto(s.length-1) {|n| print s[n,1], " "}
# In Ruby 1.9, bytes, lines, and chars are aliases
s.bytes.to_a # => [65,10,66]: alias for each_byte
s.lines.to_a # => ["A\n","B"]: alias for each_line
s.chars.to_a # => ["A", "\n", "B"] alias for each_char
---------------------------
"10".to_i # => 10: convert string to integer
"10".to_i(2) # => 2: argument is radix: between base-2 and base-36
"10x".to_i # => 10: nonnumeric suffix is ignored. Same for oct, hex
" 10".to_i # => 10: leading whitespace ignored
"ten".to_i # => 0: does not raise exception on bad input
"10".oct # => 8: parse string as base-8 integer
"10".hex # => 16: parse string as hexadecimal integer
"0xff".hex # => 255: hex numbers may begin with 0x prefix
" 1.1 dozen".to_f # => 1.1: parse leading floating-point number
"6.02e23".to_f # => 6.02e+23: exponential notation supported
"one".to_sym # => :one -- string to symbol conversion
"two".intern # => :two -- intern is a synonym for to_sym
---------------------------
# Increment a string:
"a".succ # => "b": the successor of "a". Also, succ!
"aaz".next # => "aba": next is a synonym. Also, next!
"a".upto("e") {|c| print c } # Prints "abcde. upto iterator based on succ.
# Reverse a string:
"hello".reverse # => "olleh". Also reverse!
# Debugging
"hello\n".dump # => "\"hello\\n\"": Escape special characters
"hello\n".inspect # Works much like dump
# Translation from one set of characters to another
"hello".tr("aeiou", "AEIOU") # => "hEllO": capitalize vowels. Also tr!
"hello".tr("aeiou", " ") # => "h ll ": convert vowels to spaces
"bead".tr_s("aeiou", " ") # => "b d": convert and remove duplicates
# Checksums
"hello".sum # => 532: weak 16-bit checksum
"hello".sum(8) # => 20: 8 bit checksum instead of 16 bit
"hello".crypt("ab") # => "abl0JrMf6tlhw": one way cryptographic checksum
# Pass two alphanumeric characters as "salt"
# The result may be platform-dependent
# Counting letters, deleting letters, and removing duplicates
"hello".count('aeiou') # => 2: count lowercase vowels
"hello".delete('aeiou') # => "hll": delete lowercase vowels. Also delete!
"hello".squeeze('a-z') # => "helo": remove runs of letters. Also squeeze!
# When there is more than one argument, take the intersection.
# Arguments
没有合适的资源?快使用搜索试试~ 我知道了~
Ruby程序设计语言 (涵盖Ruby 1.8和1.9)源代码
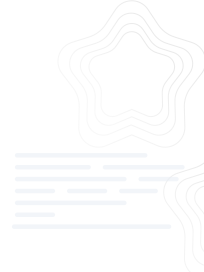
共26个文件
rb:15个
txt:10个
readme:1个

需积分: 9 13 下载量 149 浏览量
2011-06-09
19:20:02
上传
评论
收藏 107KB ZIP 举报
温馨提示
《Ruby程序设计语言》是Ruby的权威指南,全面涵盖该语言的1.8版和1.9版。本书详尽但并不拘泥于语言规范,既适合首次接触Ruby的资深程序员,同样也适合那些想要挑战对这门语言的理解并更深入掌握它的Ruby程序员。本书首先通过一个快速指南带您熟悉这门语言,然后彻底解释它的细节,包括: Ruby程序的词法和句法结构 数据类型和对象 表达式和操作符 语句和控制结构 Method、proc、lambda和closure 类和模块 反射和元编程
资源推荐
资源详情
资源评论
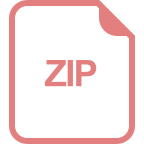
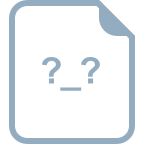
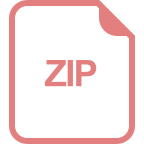
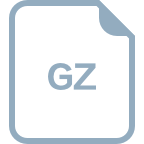
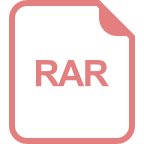
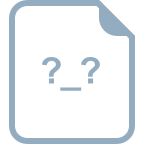
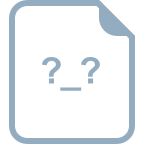
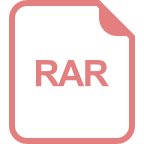
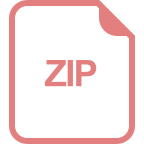
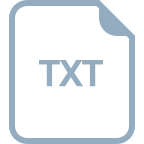
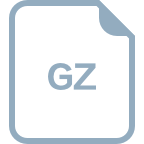
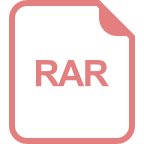
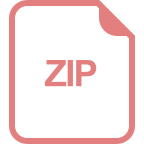
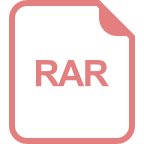
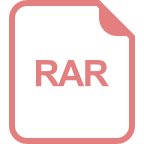
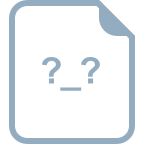
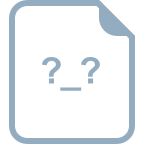
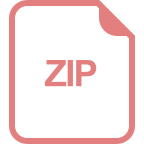
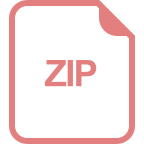
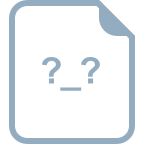
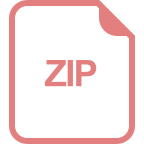
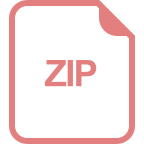
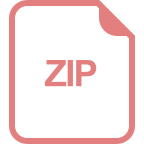
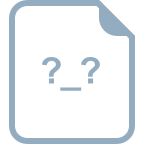
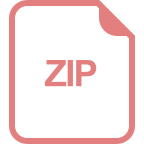
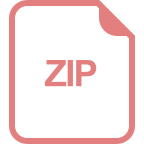
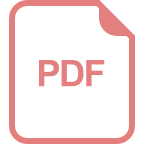
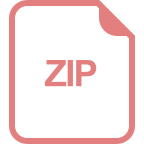
收起资源包目录


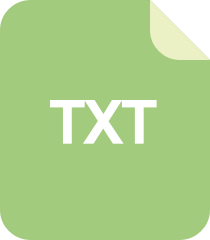
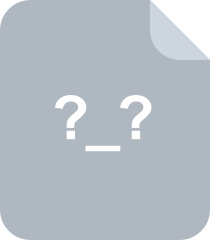
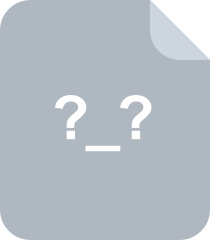
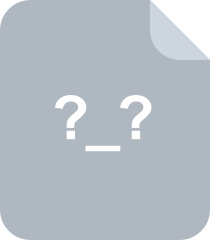
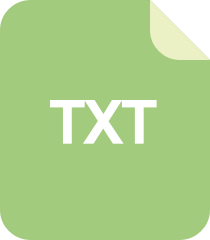
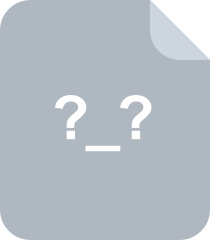
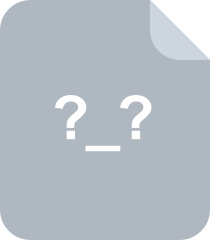
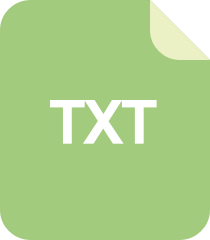
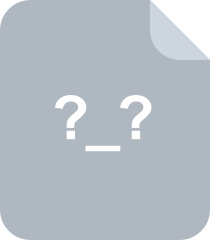
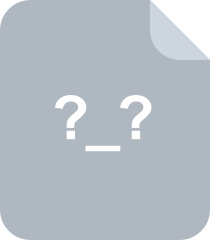
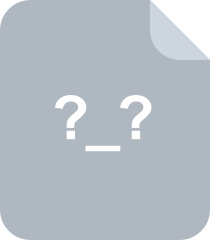
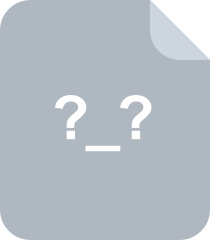
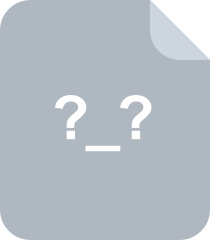
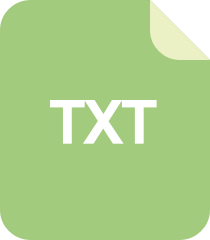
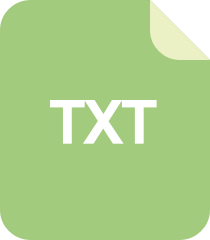
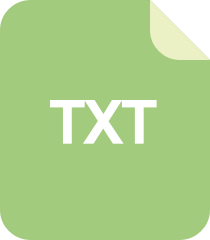
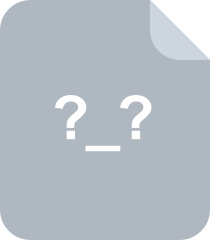
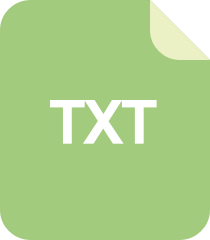
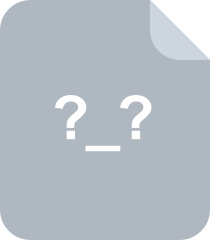
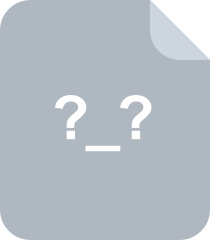
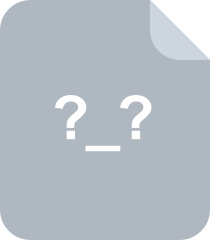
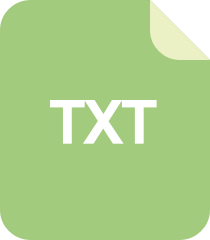
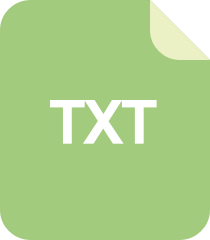
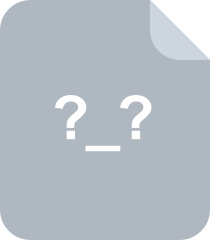
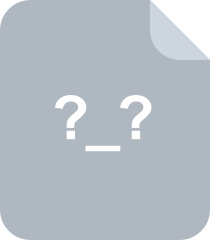
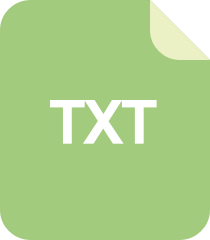
共 26 条
- 1
资源评论
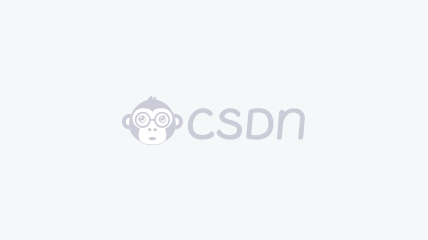

猴子搬来的救兵Castiel
- 粉丝: 3573
- 资源: 881
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

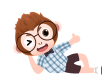
最新资源
- (174717862)有源滤波电路1-模电Multisim仿真实验
- (3822212)单片机Proteus仿真
- (481250)Proteus 与单片机 仿真
- (179979052)基于MATLAB车牌识别系统【带界面GUI】.zip
- 计算机网络四次实验报告
- (175549404)基于微信小程序的十二神鹿点餐(外卖小程序)(毕业设计,包括数据库,源码,教程).zip
- (179941432)基于MATLAB车牌识别系统【GUI含界面】.zip
- (179941434)基于MATLAB车牌识别系统【含界面GUI】.zip
- (178021462)基于Javaweb+ssm的医院在线挂号系统的设计与实现.zip
- (178047214)基于springboot图书管理系统.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


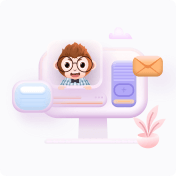
安全验证
文档复制为VIP权益,开通VIP直接复制
