<!-- ---------------------------------------------------------------------
//
// Copyright 2000 Microsoft Corporation. All Rights Reserved.
//
// File: calendar.htc
//
// Description: The calendar behavior provides an easy, declarative way
// to add a standard calendar control to web pages and html
// based applications. It provides a variety of properties
// to customize the look and feel along with a strong set
// events and functionality.
//
//-------------------------------------------------------------------- -->
<script language="javascript">
//------------------------------------------------------------------------
// Attach to element events
//------------------------------------------------------------------------
element.attachEvent("onselectstart", fnOnSelectStart)
element.attachEvent("onclick", fnOnClick)
element.attachEvent("onpropertychange", fnOnPropertyChange)
element.attachEvent("onreadystatechange", fnOnReadyStateChange)
//------------------------------------------------------------------------
// Create the arrays of days & months for different languages
//------------------------------------------------------------------------
var gaMonthNames = new Array(
new Array('Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun',
'Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec'),
new Array('January', 'February', 'March', 'April', 'May', 'June', 'July',
'August', 'September', 'October', 'November', 'December')
);
var gaDayNames = new Array(
new Array('S', 'M', 'T', 'W', 'T', 'F', 'S'),
new Array('Sun', 'Mon', 'Tue', 'Wed', 'Thu', 'Fri', 'Sat'),
new Array('Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday')
);
var gaMonthDays = new Array(
/* Jan */ 31, /* Feb */ 29, /* Mar */ 31, /* Apr */ 30,
/* May */ 31, /* Jun */ 30, /* Jul */ 31, /* Aug */ 31,
/* Sep */ 30, /* Oct */ 31, /* Nov */ 30, /* Dec */ 31 )
var StyleInfo = null // Style sheet with rules for this calendar
var goStyle = new Object() // A hash of style sheet rules that apply to this calendar
var gaDayCell = new Array() // an array of the table cells for days
var goDayTitleRow = null // The table row containing days of the week
var goYearSelect = null // The year select control
var goMonthSelect = null // The month select control
var goCurrentDayCell = null // The cell for the currently selected day
var giStartDayIndex = 0 // The index in gaDayCell for the first day of the month
var gbLoading = true // Flag for if the behavior is loading
var giDay // day of the month (1 to 31)
var giMonth // month of the year (1 to 12)
var giYear // year (1900 to 2099)
var giMonthLength = 1 // month length (0,1)
var giDayLength = 1 // day length (0 to 2)
var giFirstDay = 0 // first day of the week (0 to 6)
var gsGridCellEffect = 'raised' // Grid cell effect
var gsGridLinesColor = 'black' // Grid line color
var gbShowDateSelectors = true // Show date selectors (0,1)
var gbShowDays = true // Show the days of the week titles (0,1)
var gbShowTitle = true // Show the title (0,1)
var gbShowHorizontalGrid = true // Show the horizontal grid (0,1)
var gbShowVerticalGrid = true // Show the vertical grid (0,1)
var gbValueIsNull = false // There is no value selected (0,1)
var gbReadOnly = false // The user can not interact with the control
var giMinYear = 1900 // Minimum year (1 is the lowest possible value)
var giMaxYear = 2099 // Maximum year
// Load the property values defined on the element to replace defaults
fnGetPropertyDefaults()
// Create the style sheets needed for the calendar display
fnCreateStyleSheets()
// Insert the HTML elements needed for the calendar display
fnCreateCalendarHTML()
// Update the title with the month and year
fnUpdateTitle()
// Fill in the days of the week
fnUpdateDayTitles()
// Build the month select control
fnBuildMonthSelect()
// Build the year select control
fnBuildYearSelect()
// Fill in the cells with the days of the month and set style values
fnFillInCells()
// **********************************************************************
// PROPERTY GET/SET FUNCTIONS
// **********************************************************************
//------------------------------------------------------------------------
//
// Function: fnGetDay / fnPutDay
//
// Synopsis: The day property is used to set the day of the month. The
// valid range is from 1 to the maximum day of the selected
// month & year. If a number is given outside that range, it
// is set to the closest valid value. Invalid input will cause
// an exception.
//
// Arguments: The put method requires an integer value for the day
//
// Returns: The get method will return the selected day of the month
// If the valueIsNull property is set, null is returned
//
// Notes: none
//
//------------------------------------------------------------------------
function fnGetDay()
{
return (gbValueIsNull) ? null : giDay
}
function fnPutDay(iDay)
{
if (gbLoading) return // return if the behavior is loading
iDay = parseInt(iDay)
if (isNaN(iDay)) throw 450
fnSetDate(iDay, giMonth, giYear)
}
//------------------------------------------------------------------------
//
// Function: fnGetMonth / fnPutMonth
//
// Synopsis: The month property is used to set the month of the year.
// The valid range is from 1 to 12. If a value is given
// outside that range, it is set to the closest valid value.
// Invalid input will cause an exception.
//
// Arguments: The put method requires an integer value for the month
//
// Returns: The get method will return the selected month value
// If the valueIsNull property is set, null is returned
//
// Notes: Setting the year can cause the selected "day" value to be
// reduced to the highest day in the selected month if needed.
//
//------------------------------------------------------------------------
function fnGetMonth()
{
return (gbValueIsNull) ? null : giMonth
}
function fnPutMonth(iMonth)
{
if (gbLoading) return // return if the behavior is loading
iMonth = parseInt(iMonth)
if (isNaN(iMonth)) throw 450
fnSetDate(giDay, iMonth, giYear)
}
//------------------------------------------------------------------------
//
// Function: fnGetYear / fnPutYear
//
// Synopsis: The year property is used to set the current year.
// The valid range is from minYear to maxYear. If a value is given
// outside that range, it is set to the closest valid value.
// Invalid input will cause an exception.
//
// Arguments: The put method requires an integer value for the year
//
// Returns: The get method will return the selected year value
// If the valueIsNull property is set, null is returned.
//
// Notes: Setting the year can cause the selected "day" value to be
// reduced to the highest day in the selected month if needed.
//
//------------------------------------------------------------------------
function fnGetYear()
{
return (gbValueIsNull) ? null : giYear
}
function fnPutYear(iYear)
{
if (gbLoading) return // return if the behavior is loading
iYear = parseInt(iYear)
if (isNaN(iYear)) throw 450
fnSetDate(giDay, giMonth, iYear)
}
//-------------------
没有合适的资源?快使用搜索试试~ 我知道了~
js特效大全。新手必备。老手省力
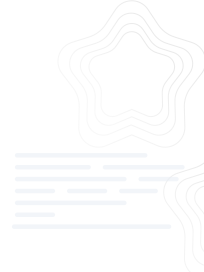
共1006个文件
gif:451个
htm:371个
jpg:68个


温馨提示
js特效大全 新手必备 老手省力的宝贝 收藏起来吧。。。
资源推荐
资源详情
资源评论
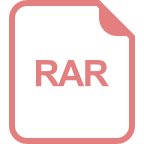
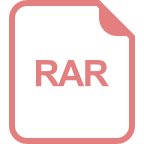
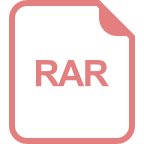
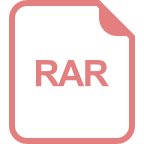
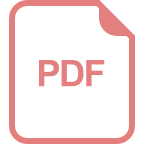
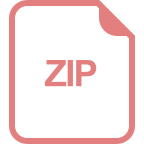
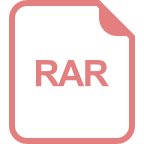
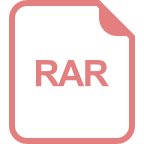
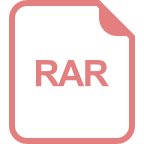
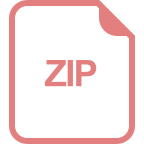
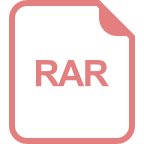
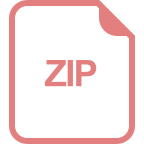
收起资源包目录

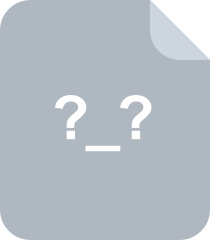
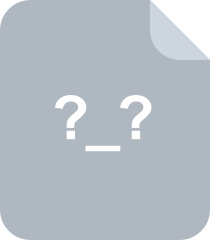
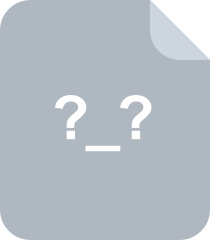
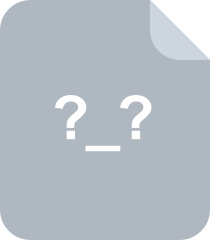
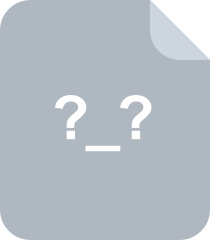
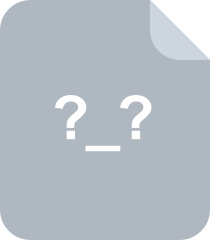
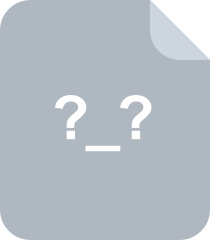
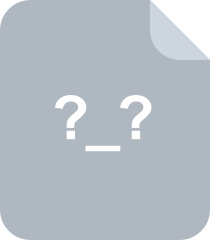
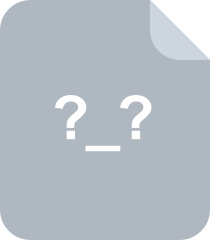
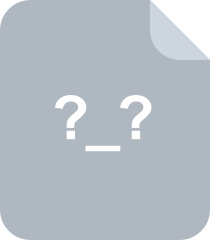
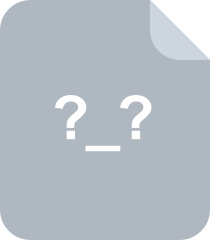
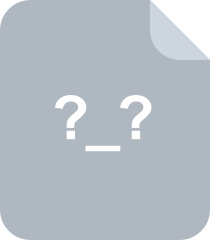
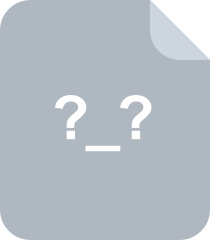
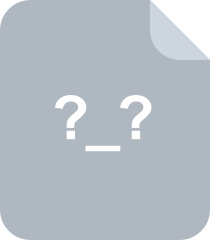
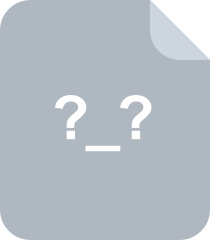
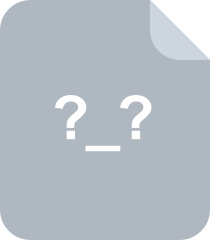
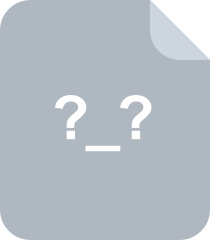
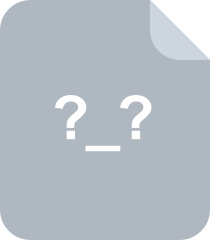
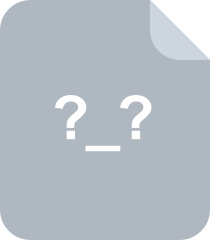
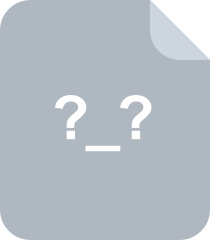
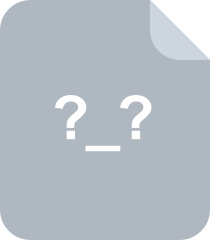
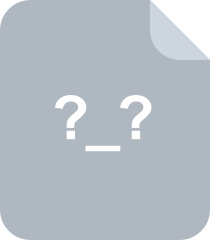
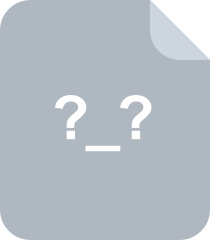
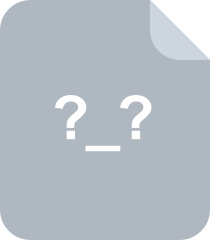
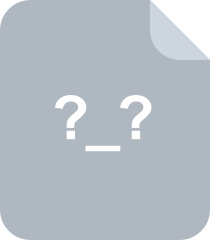
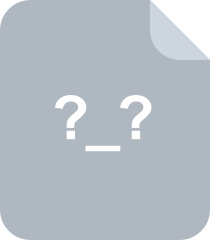
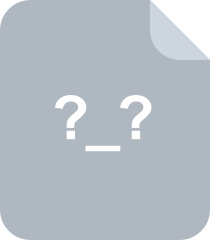
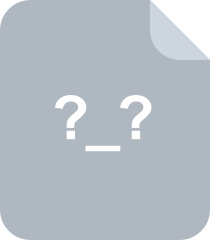
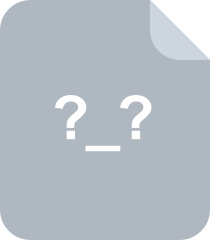
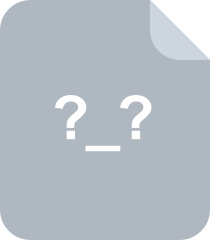
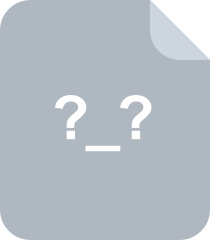
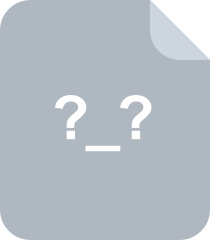
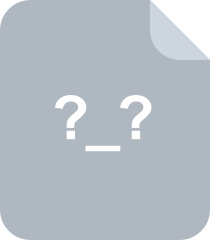
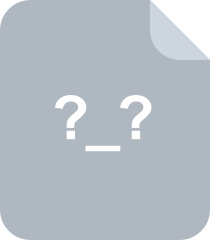
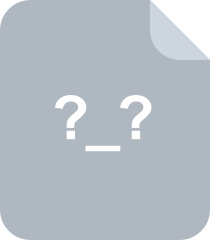
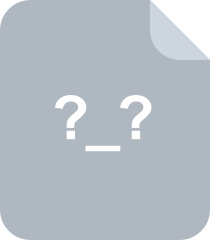
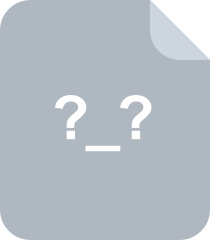
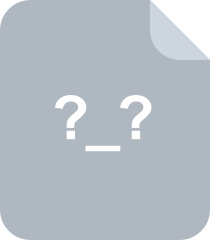
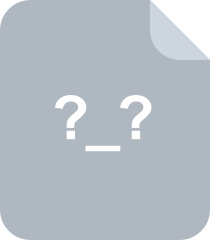
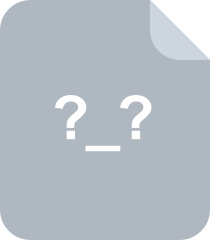
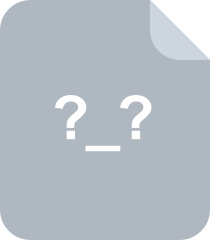
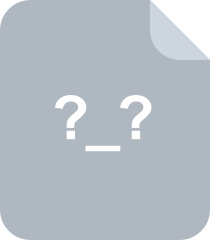
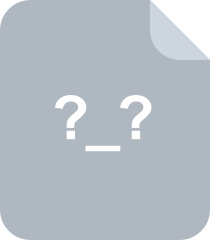
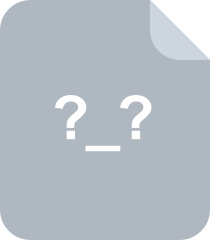
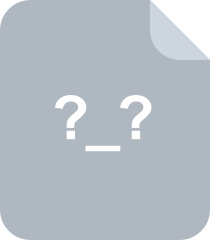
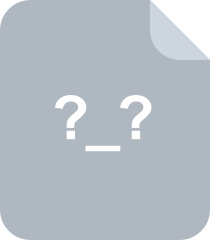
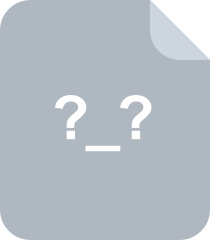
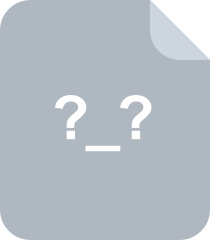
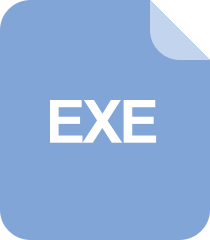
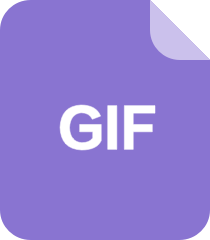
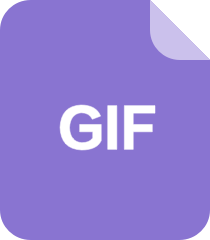
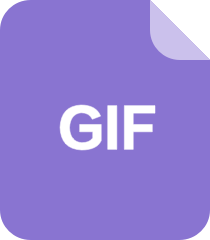
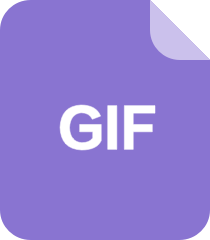
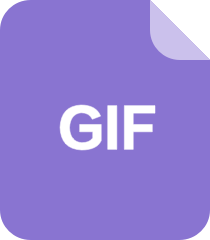
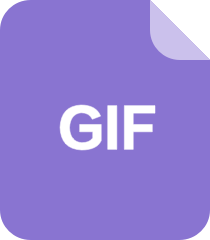
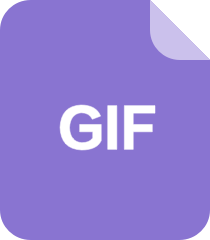
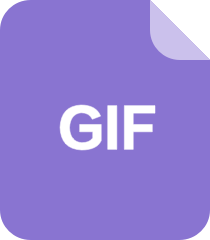
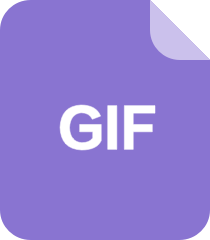
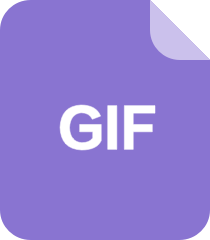
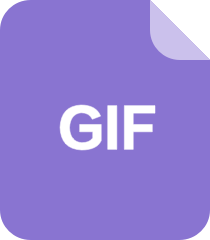
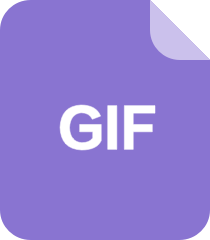
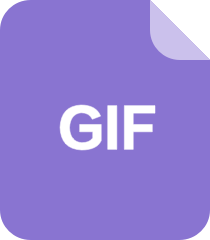
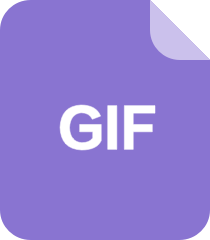
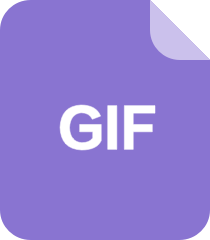
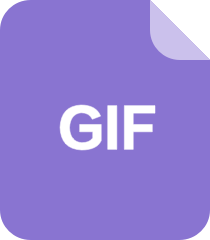
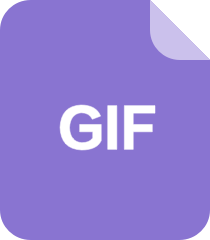
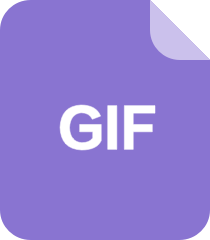
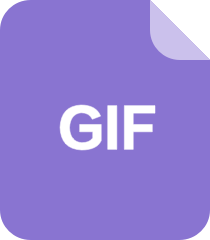
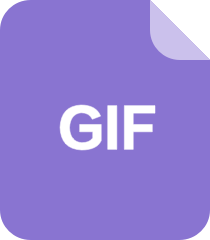
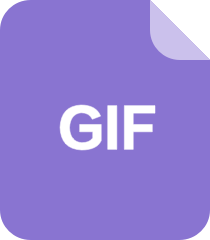
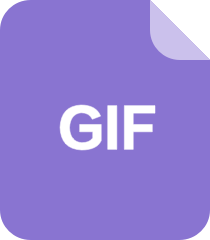
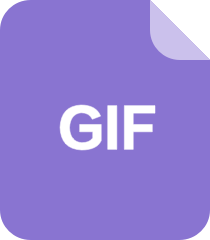
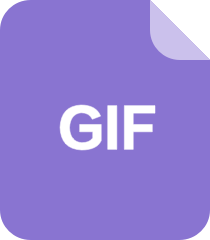
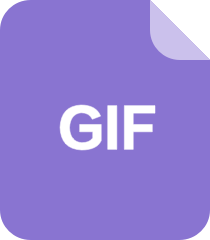
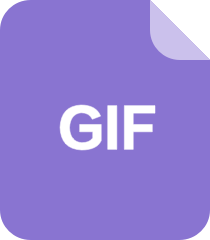
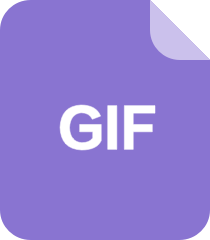
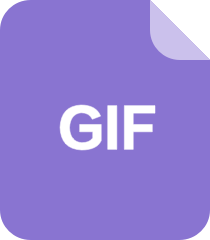
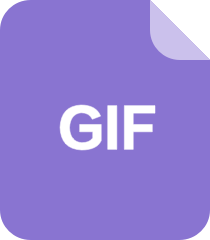
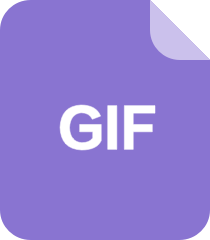
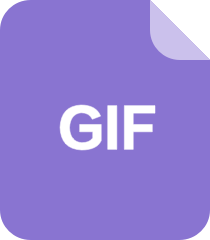
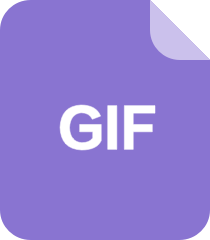
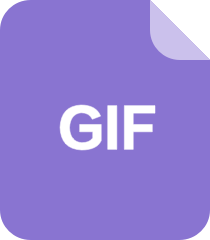
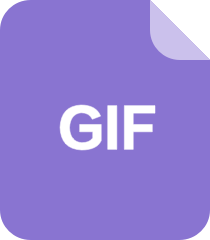
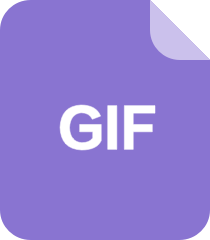
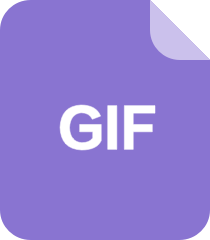
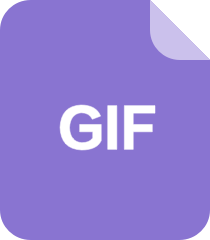
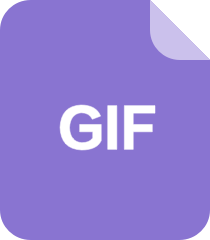
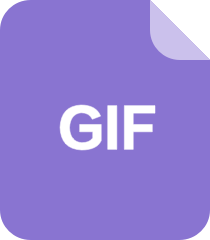
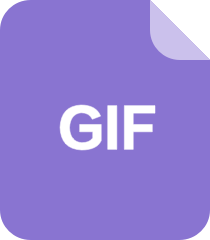
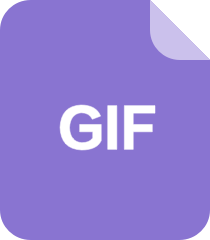
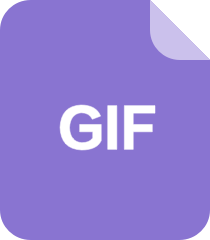
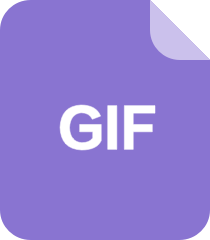
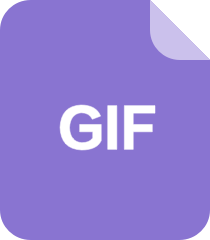
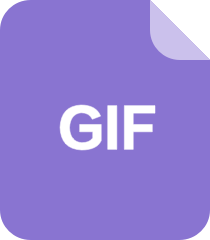
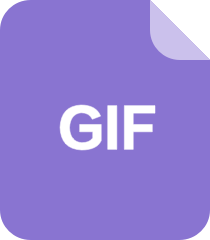
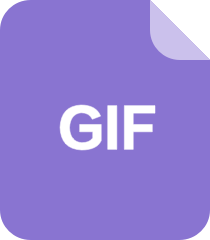
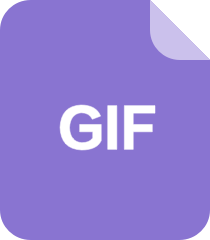
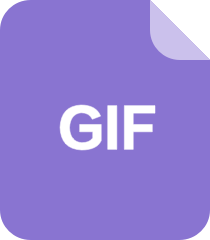
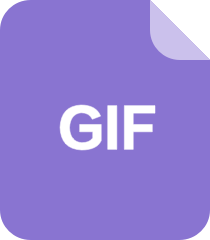
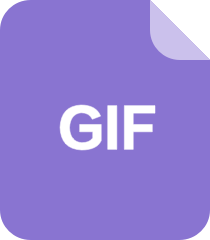
共 1006 条
- 1
- 2
- 3
- 4
- 5
- 6
- 11
资源评论
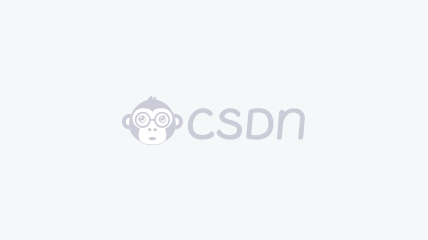
- JudeHo2012-05-17功能挺多,不过只是IE上看好,到了火狐谷歌之类的就乱了

mxpayy198369
- 粉丝: 0
- 资源: 2
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

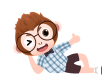
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


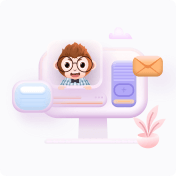
安全验证
文档复制为VIP权益,开通VIP直接复制
