/*
*
* This file is part of the open-source SeetaFace engine, which includes three modules:
* SeetaFace Detection, SeetaFace Alignment, and SeetaFace Identification.
*
* This file is an example of how to use SeetaFace engine for face alignment, the
* face alignment method described in the following paper:
*
*
* Coarse-to-Fine Auto-Encoder Networks (CFAN) for Real-Time Face Alignment,
* Jie Zhang, Shiguang Shan, Meina Kan, Xilin Chen. In Proceeding of the
* European Conference on Computer Vision (ECCV), 2014
*
*
* Copyright (C) 2016, Visual Information Processing and Learning (VIPL) group,
* Institute of Computing Technology, Chinese Academy of Sciences, Beijing, China.
*
* The codes are mainly developed by Jie Zhang (a Ph.D supervised by Prof. Shiguang Shan)
*
* As an open-source face recognition engine: you can redistribute SeetaFace source codes
* and/or modify it under the terms of the BSD 2-Clause License.
*
* You should have received a copy of the BSD 2-Clause License along with the software.
* If not, see < https://opensource.org/licenses/BSD-2-Clause>.
*
* Contact Info: you can send an email to SeetaFace@vipl.ict.ac.cn for any problems.
*
* Note: the above information must be kept whenever or wherever the codes are used.
*
*/
#include <cstdint>
#include <fstream>
#include <iostream>
#include <string>
#include "cv.h"
#include "highgui.h"
#include "/root/SeetaFaceEngine-master/FaceDetection/include/face_detection.h"
#include "/root/SeetaFaceEngine-master/FaceAlignment/include/face_alignment.h"
#ifdef _WIN32
std::string DATA_DIR = "../../data/";
std::string MODEL_DIR = "../../model/";
#else
std::string DATA_DIR = "./data/";
std::string MODEL_DIR = "./model/";
#endif
int main(int argc, char** argv)
{
// Initialize face detection model
//seeta::FaceDetection detector("../../../FaceDetection/model/seeta_fd_frontal_v1.0.bin");
seeta::FaceDetection detector("/root/SeetaFaceEngine-master/FaceDetection/model/seeta_fd_frontal_v1.0.bin");
detector.SetMinFaceSize(40);
detector.SetScoreThresh(2.f);
detector.SetImagePyramidScaleFactor(0.8f);
detector.SetWindowStep(4, 4);
// Initialize face alignment model
//seeta::FaceAlignment point_detector((MODEL_DIR + "seeta_fa_v1.1.bin").c_str());
seeta::FaceAlignment point_detector("/root/SeetaFaceEngine-master/FaceAlignment/model/seeta_fa_v1.1.bin");
//load image
IplImage *img_grayscale = NULL;
//img_grayscale = cvLoadImage((DATA_DIR + "image_0001.png").c_str(), 0);
img_grayscale = cvLoadImage("/root/SeetaFaceEngine-master/imagefilePath/1270343438180.jpg", 0);
if (img_grayscale == NULL)
{
return 0;
}
//IplImage *img_color = cvLoadImage((DATA_DIR + "image_0001.png").c_str(), 1);
IplImage *img_color = cvLoadImage("/root/SeetaFaceEngine-master/imagefilePath/1270343438180.jpg", 1);
int pts_num = 5;
int im_width = img_grayscale->width;
int im_height = img_grayscale->height;
unsigned char* data = new unsigned char[im_width * im_height];
unsigned char* data_ptr = data;
unsigned char* image_data_ptr = (unsigned char*)img_grayscale->imageData;
int h = 0;
for (h = 0; h < im_height; h++) {
memcpy(data_ptr, image_data_ptr, im_width);
data_ptr += im_width;
image_data_ptr += img_grayscale->widthStep;
}
seeta::ImageData image_data;
image_data.data = data;
image_data.width = im_width;
image_data.height = im_height;
image_data.num_channels = 1;
// Detect faces
std::vector<seeta::FaceInfo> faces = detector.Detect(image_data);
int32_t face_num = static_cast<int32_t>(faces.size());
if (face_num == 0)
{
delete[]data;
cvReleaseImage(&img_grayscale);
cvReleaseImage(&img_color);
return 0;
}
// Detect 5 facial landmarks
seeta::FacialLandmark points[5];
point_detector.PointDetectLandmarks(image_data, faces[0], points);
// Visualize the results
cvRectangle(img_color, cvPoint(faces[0].bbox.x, faces[0].bbox.y), cvPoint(faces[0].bbox.x + faces[0].bbox.width - 1, faces[0].bbox.y + faces[0].bbox.height - 1), CV_RGB(255, 0, 0));
for (int i = 0; i<pts_num; i++)
{
cvCircle(img_color, cvPoint(points[i].x, points[i].y), 2, CV_RGB(0, 255, 0), CV_FILLED);
}
cvSaveImage("/root/SeetaFaceEngine-master/result.jpg", img_color);
// cv::namedWindow("Test", cv::WINDOW_AUTOSIZE);
// cv::imshow("Test", img_color);
// cv::waitKey(0);
// cv::destroyAllWindows();
// Release memory
cvReleaseImage(&img_color);
cvReleaseImage(&img_grayscale);
delete[]data;
return 0;
}
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
基于seetaface的人脸识别,本人修改为安卓NDK编译程序,并扩展了,安卓调用接口,包括了检测,对齐,识别,在同一个程序中,附全部源码,含C源码,jni接口,安卓调用,可跟据你的需要随意加接口,首次运行会将几个老师的bin文件自动复制到本地存储的abdosoft.face文件夹下,训练的数据也放在这里,决对好的参考。本人笔记网址www.abdosoft.cn, 至少可以让你节省1个星期时间,包括jni学习也是很好的参考了。
资源推荐
资源详情
资源评论
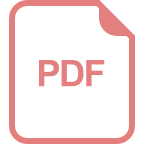
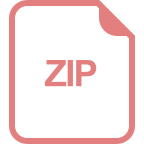
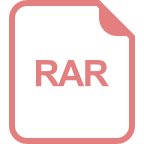
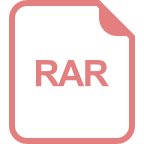
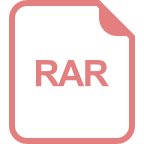
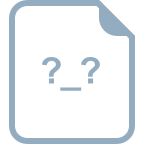
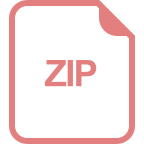
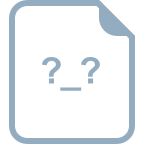
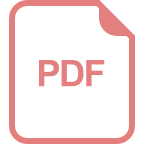
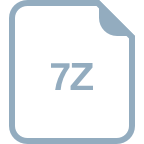
收起资源包目录

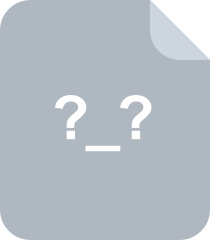
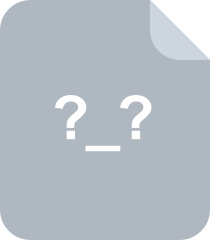
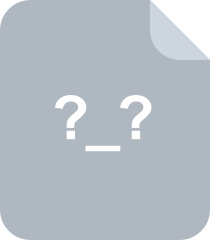
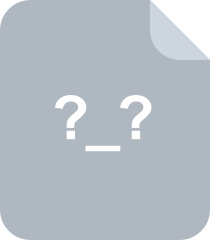
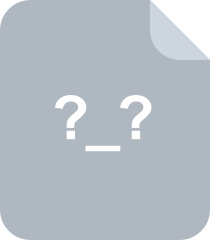
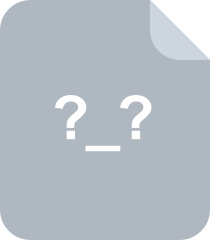
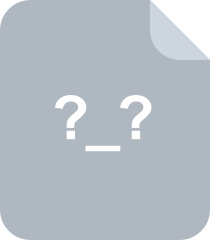
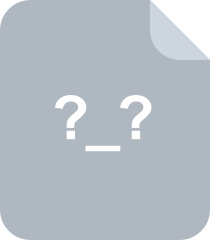
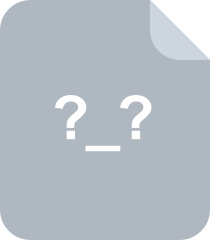
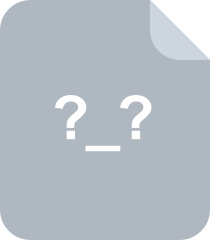
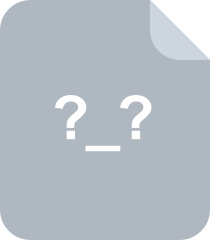
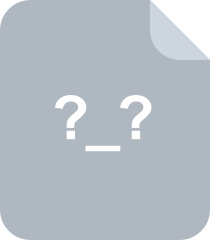
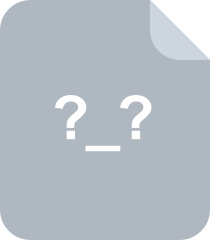
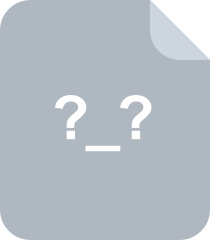
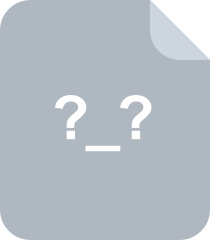
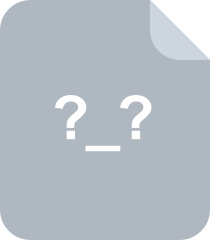
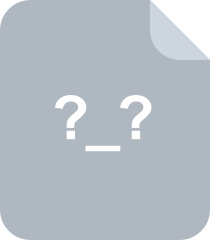
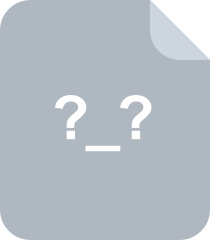
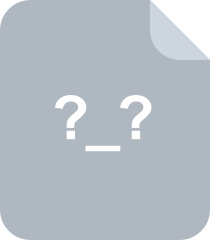
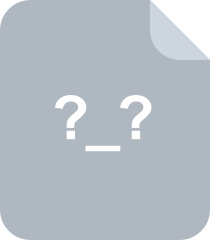
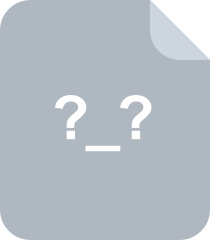
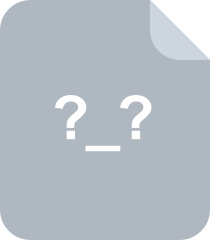
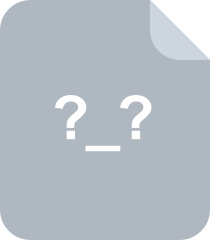
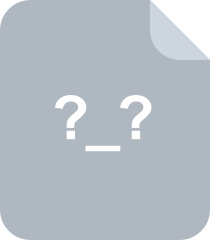
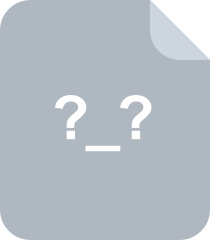
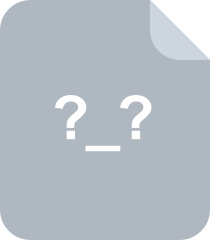
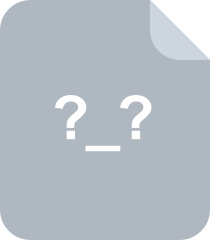
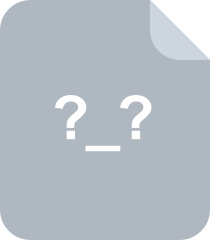
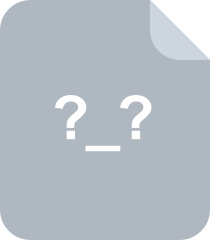
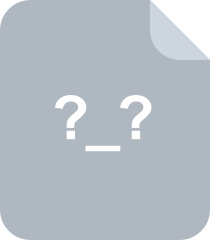
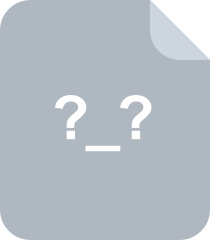
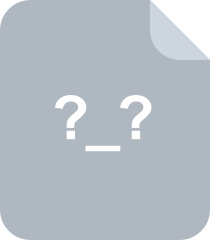
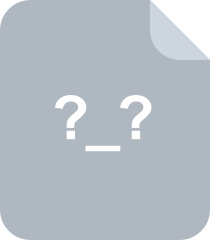
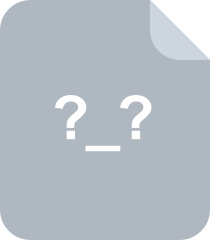
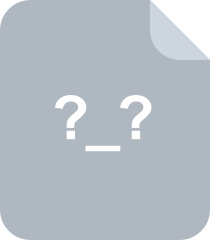
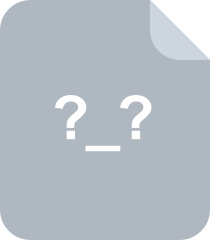
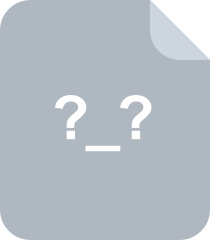
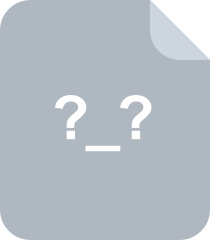
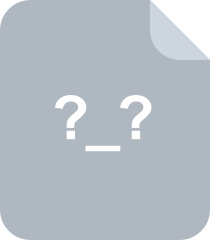
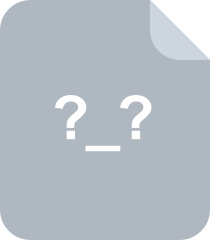
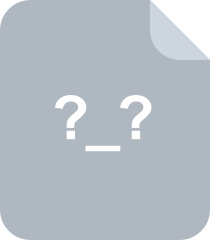
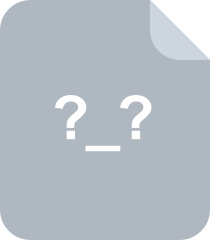
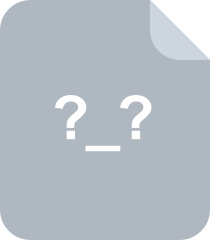
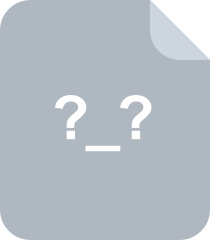
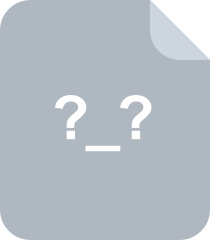
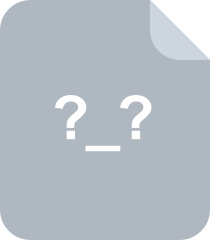
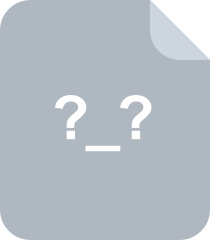
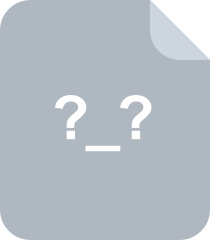
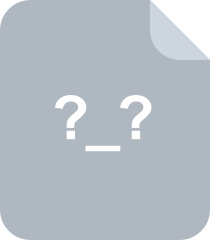
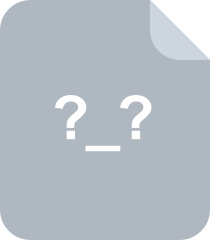
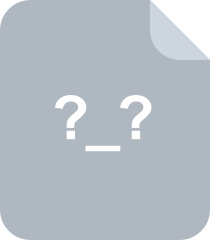
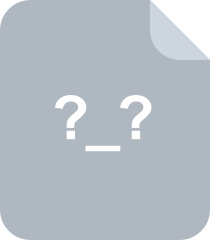
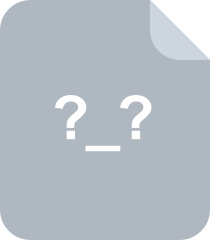
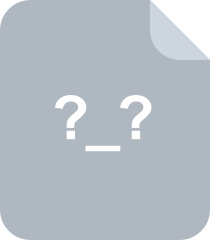
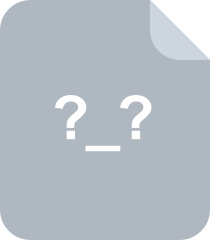
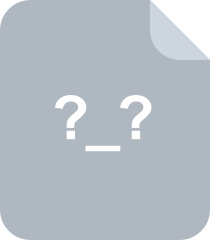
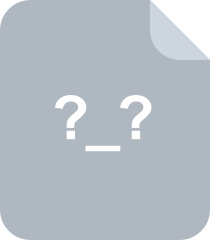
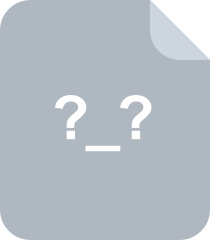
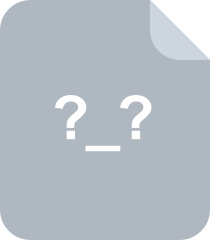
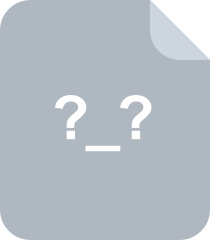
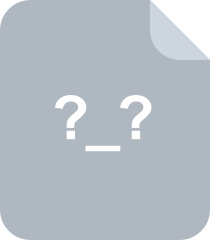
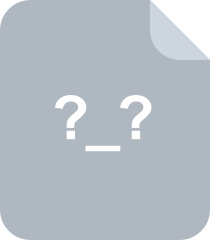
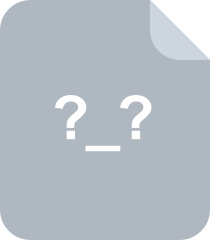
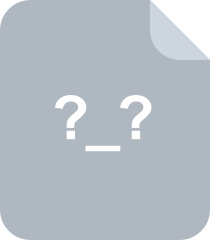
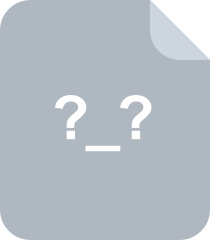
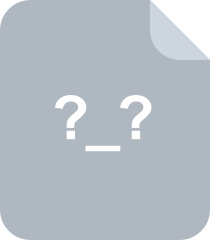
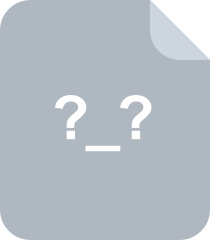
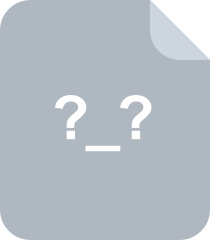
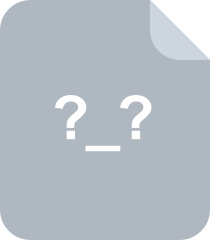
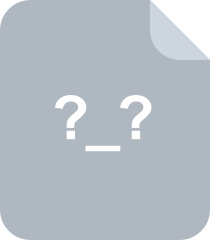
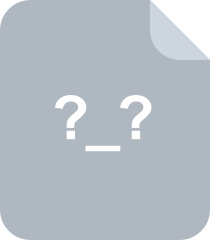
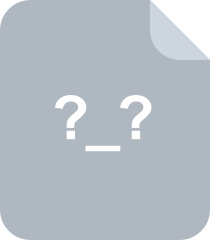
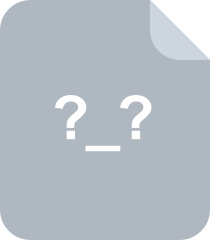
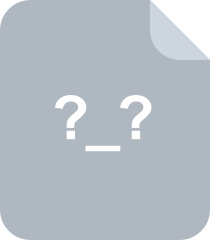
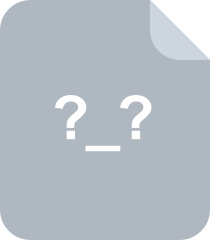
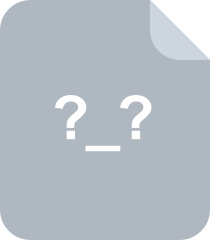
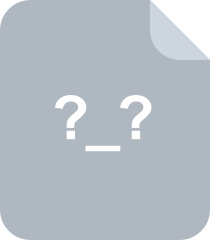
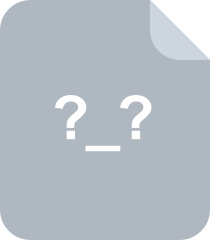
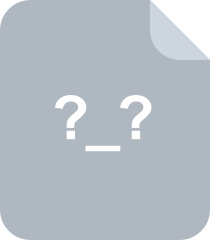
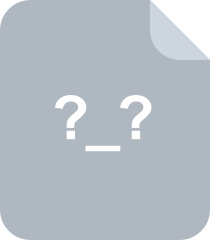
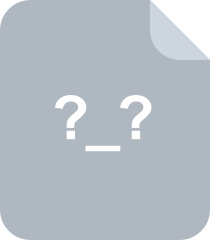
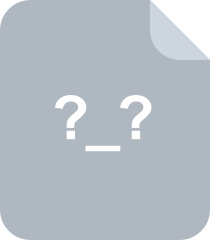
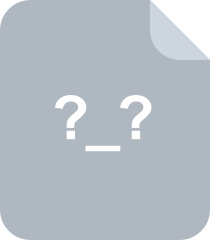
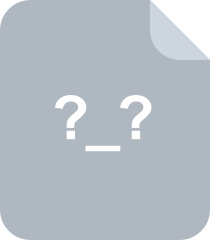
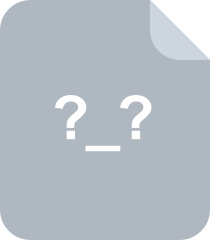
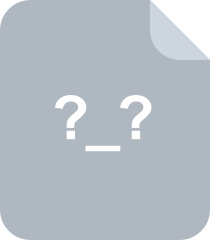
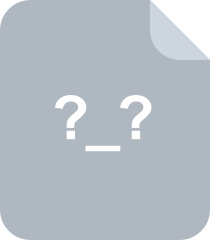
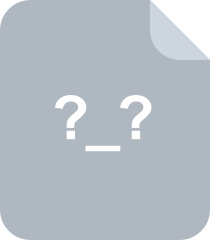
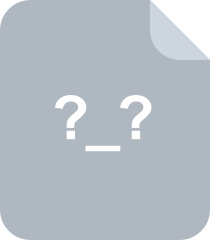
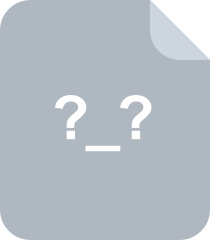
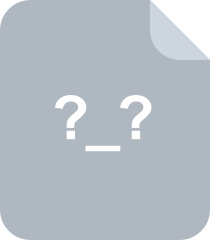
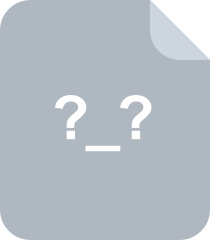
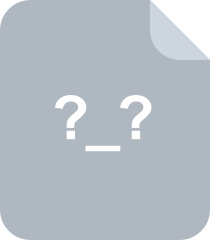
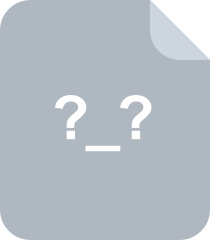
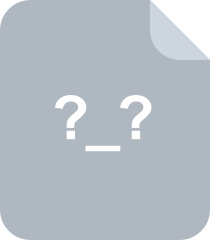
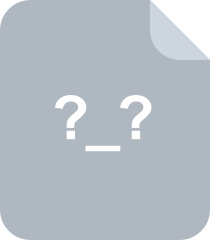
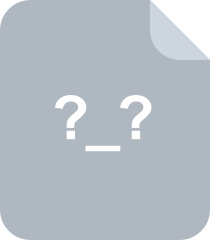
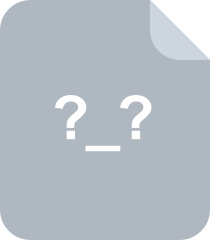
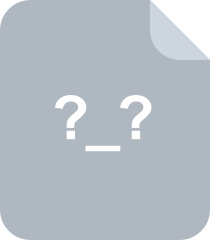
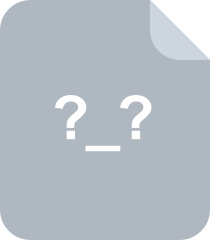
共 489 条
- 1
- 2
- 3
- 4
- 5
资源评论
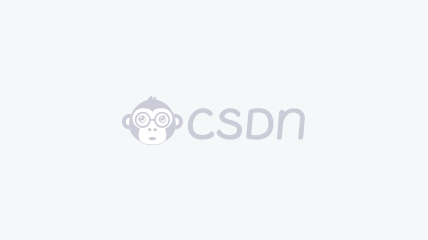

mxeye
- 粉丝: 1
- 资源: 5
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

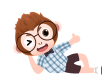
最新资源
- C#/WinForm演示退火算法(源码)
- 如何在 IntelliJ IDEA 中去掉 Java 方法注释后的空行.md
- 小程序官方组件库,内含各种组件实例,以及调用方式,多种UI可修改
- 2011年URL缩短服务JSON数据集
- Kaggle-Pokemon with stats(宠物小精灵数据)
- Harbor 最新v2.12.0的ARM64版离线安装包
- 【VUE网站静态模板】Uniapp 框架开发响应式网站,企业项目官网-APP,web网站,小程序快速生成 多语言:支持中文简体,中文繁体,英语
- 使用哈夫曼编码来对字符串进行编码HuffmanEncodingExample
- Ti芯片C2000内核手册
- c语言实现的花式爱心源码
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


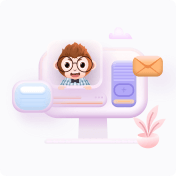
安全验证
文档复制为VIP权益,开通VIP直接复制
