/*++ BUILD Version: 0004 // Increment this if a change has global effects
Copyright (c) 1985-96, Microsoft Corporation
Module Name:
gl.h
Abstract:
Procedure declarations, constant definitions and macros for the OpenGL
component.
--*/
#ifndef __gl_h_
#ifndef __GL_H__
#define __gl_h_
#define __GL_H__
#ifdef __cplusplus
extern "C" {
#endif
/*
** Copyright 1996 Silicon Graphics, Inc.
** All Rights Reserved.
**
** This is UNPUBLISHED PROPRIETARY SOURCE CODE of Silicon Graphics, Inc.;
** the contents of this file may not be disclosed to third parties, copied or
** duplicated in any form, in whole or in part, without the prior written
** permission of Silicon Graphics, Inc.
**
** RESTRICTED RIGHTS LEGEND:
** Use, duplication or disclosure by the Government is subject to restrictions
** as set forth in subdivision (c)(1)(ii) of the Rights in Technical Data
** and Computer Software clause at DFARS 252.227-7013, and/or in similar or
** successor clauses in the FAR, DOD or NASA FAR Supplement. Unpublished -
** rights reserved under the Copyright Laws of the United States.
*/
typedef unsigned int GLenum;
typedef unsigned char GLboolean;
typedef unsigned int GLbitfield;
typedef signed char GLbyte;
typedef short GLshort;
typedef int GLint;
typedef int GLsizei;
typedef unsigned char GLubyte;
typedef unsigned short GLushort;
typedef unsigned int GLuint;
typedef float GLfloat;
typedef float GLclampf;
typedef double GLdouble;
typedef double GLclampd;
typedef void GLvoid;
/*************************************************************/
/* Version */
#define GL_VERSION_1_1 1
/* AccumOp */
#define GL_ACCUM 0x0100
#define GL_LOAD 0x0101
#define GL_RETURN 0x0102
#define GL_MULT 0x0103
#define GL_ADD 0x0104
/* AlphaFunction */
#define GL_NEVER 0x0200
#define GL_LESS 0x0201
#define GL_EQUAL 0x0202
#define GL_LEQUAL 0x0203
#define GL_GREATER 0x0204
#define GL_NOTEQUAL 0x0205
#define GL_GEQUAL 0x0206
#define GL_ALWAYS 0x0207
/* AttribMask */
#define GL_CURRENT_BIT 0x00000001
#define GL_POINT_BIT 0x00000002
#define GL_LINE_BIT 0x00000004
#define GL_POLYGON_BIT 0x00000008
#define GL_POLYGON_STIPPLE_BIT 0x00000010
#define GL_PIXEL_MODE_BIT 0x00000020
#define GL_LIGHTING_BIT 0x00000040
#define GL_FOG_BIT 0x00000080
#define GL_DEPTH_BUFFER_BIT 0x00000100
#define GL_ACCUM_BUFFER_BIT 0x00000200
#define GL_STENCIL_BUFFER_BIT 0x00000400
#define GL_VIEWPORT_BIT 0x00000800
#define GL_TRANSFORM_BIT 0x00001000
#define GL_ENABLE_BIT 0x00002000
#define GL_COLOR_BUFFER_BIT 0x00004000
#define GL_HINT_BIT 0x00008000
#define GL_EVAL_BIT 0x00010000
#define GL_LIST_BIT 0x00020000
#define GL_TEXTURE_BIT 0x00040000
#define GL_SCISSOR_BIT 0x00080000
#define GL_ALL_ATTRIB_BITS 0x000fffff
/* BeginMode */
#define GL_POINTS 0x0000
#define GL_LINES 0x0001
#define GL_LINE_LOOP 0x0002
#define GL_LINE_STRIP 0x0003
#define GL_TRIANGLES 0x0004
#define GL_TRIANGLE_STRIP 0x0005
#define GL_TRIANGLE_FAN 0x0006
#define GL_QUADS 0x0007
#define GL_QUAD_STRIP 0x0008
#define GL_POLYGON 0x0009
/* BlendingFactorDest */
#define GL_ZERO 0
#define GL_ONE 1
#define GL_SRC_COLOR 0x0300
#define GL_ONE_MINUS_SRC_COLOR 0x0301
#define GL_SRC_ALPHA 0x0302
#define GL_ONE_MINUS_SRC_ALPHA 0x0303
#define GL_DST_ALPHA 0x0304
#define GL_ONE_MINUS_DST_ALPHA 0x0305
/* BlendingFactorSrc */
/* GL_ZERO */
/* GL_ONE */
#define GL_DST_COLOR 0x0306
#define GL_ONE_MINUS_DST_COLOR 0x0307
#define GL_SRC_ALPHA_SATURATE 0x0308
/* GL_SRC_ALPHA */
/* GL_ONE_MINUS_SRC_ALPHA */
/* GL_DST_ALPHA */
/* GL_ONE_MINUS_DST_ALPHA */
/* Boolean */
#define GL_TRUE 1
#define GL_FALSE 0
/* ClearBufferMask */
/* GL_COLOR_BUFFER_BIT */
/* GL_ACCUM_BUFFER_BIT */
/* GL_STENCIL_BUFFER_BIT */
/* GL_DEPTH_BUFFER_BIT */
/* ClientArrayType */
/* GL_VERTEX_ARRAY */
/* GL_NORMAL_ARRAY */
/* GL_COLOR_ARRAY */
/* GL_INDEX_ARRAY */
/* GL_TEXTURE_COORD_ARRAY */
/* GL_EDGE_FLAG_ARRAY */
/* ClipPlaneName */
#define GL_CLIP_PLANE0 0x3000
#define GL_CLIP_PLANE1 0x3001
#define GL_CLIP_PLANE2 0x3002
#define GL_CLIP_PLANE3 0x3003
#define GL_CLIP_PLANE4 0x3004
#define GL_CLIP_PLANE5 0x3005
/* ColorMaterialFace */
/* GL_FRONT */
/* GL_BACK */
/* GL_FRONT_AND_BACK */
/* ColorMaterialParameter */
/* GL_AMBIENT */
/* GL_DIFFUSE */
/* GL_SPECULAR */
/* GL_EMISSION */
/* GL_AMBIENT_AND_DIFFUSE */
/* ColorPointerType */
/* GL_BYTE */
/* GL_UNSIGNED_BYTE */
/* GL_SHORT */
/* GL_UNSIGNED_SHORT */
/* GL_INT */
/* GL_UNSIGNED_INT */
/* GL_FLOAT */
/* GL_DOUBLE */
/* CullFaceMode */
/* GL_FRONT */
/* GL_BACK */
/* GL_FRONT_AND_BACK */
/* DataType */
#define GL_BYTE 0x1400
#define GL_UNSIGNED_BYTE 0x1401
#define GL_SHORT 0x1402
#define GL_UNSIGNED_SHORT 0x1403
#define GL_INT 0x1404
#define GL_UNSIGNED_INT 0x1405
#define GL_FLOAT 0x1406
#define GL_2_BYTES 0x1407
#define GL_3_BYTES 0x1408
#define GL_4_BYTES 0x1409
#define GL_DOUBLE 0x140A
/* DepthFunction */
/* GL_NEVER */
/* GL_LESS */
/* GL_EQUAL */
/* GL_LEQUAL */
/* GL_GREATER */
/* GL_NOTEQUAL */
/* GL_GEQUAL */
/* GL_ALWAYS */
/* DrawBufferMode */
#define GL_NONE 0
#define GL_FRONT_LEFT 0x0400
#define GL_FRONT_RIGHT 0x0401
#define GL_BACK_LEFT 0x0402
#define GL_BACK_RIGHT 0x0403
#define GL_FRONT 0x0404
#define GL_BACK 0x0405
#define GL_LEFT 0x0406
#define GL_RIGHT 0x0407
#define GL_FRONT_AND_BACK 0x0408
#define GL_AUX0 0x0409
#define GL_AUX1 0x040A
#define GL_AUX2 0x040B
#define GL_AUX3 0x040C
/* Enable */
/* GL_FOG */
/* GL_LIGHTING */
/* GL_TEXTURE_1D */
/* GL_TEXTURE_2D */
/* GL_LINE_STIPPLE */
/* GL_POLYGON_STIPPLE */
/* GL_CULL_FACE */
/* GL_ALPHA_TEST */
/* GL_BLEND */
/* GL_INDEX_LOGIC_OP */
/* GL_COLOR_LOGIC_OP */
/* GL_DITHER */
/* GL_STENCIL_TEST */
/*

muyi0421
- 粉丝: 0
- 资源: 4
最新资源
- 计算机毕业设计:python+爬虫+cnki网站爬
- nyakumi-lewd-snack-3-4k_720p.7z.002
- 现在微信小程序能用的mqtt.min.js
- 基于MPC的非线性摆锤系统轨迹跟踪控制matlab仿真,包括程序中文注释,仿真操作步骤
- 基于MATLAB的ITS信道模型数值模拟仿真,包括程序中文注释,仿真操作步骤
- 基于Java、JavaScript、CSS的电子产品商城设计与实现源码
- 基于Vue 2的zjc项目设计源码,适用于赶项目需求
- 基于跨语言统一的C++头文件设计源码开发方案
- 基于MindSpore 1.3的T-GCNTemporal Graph Convolutional Network设计源码
- 基于Java的贝塞尔曲线绘制酷炫轮廓背景设计源码
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


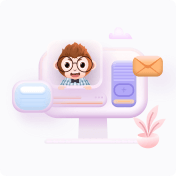