R for beginner
### R for Beginner: Basic Introduction of Using R #### Preamble This document serves as an introductory guide to the R programming language, specifically designed for beginners. It covers essential concepts and operations that users need to familiarize themselves with to effectively use R in data analysis and statistical computing. #### A Few Concepts Before Starting ##### How R Works R is an interpreted language that operates on a command-line interface. Users interact with R by entering commands, which are then executed directly without the need for compilation. This interactive nature makes it easy to test out small pieces of code and see immediate results. Here’s a brief overview of how R works: - **Interactive Mode**: In this mode, you can type commands one by one and see the output immediately. This is useful for exploring data and testing hypotheses. - **Script Mode**: For more complex tasks, you can write a series of commands in a script file and run them all at once. This approach is ideal for reproducible research and larger projects. - **Packages**: R has a vast ecosystem of packages that extend its functionality. These packages can be installed and loaded into your R session to access additional functions and features. ##### Creating, Listing, and Deleting Objects in Memory In R, objects are fundamental components that store data and information. You create objects using assignment operators such as `<-` or `=`. To list the objects currently in memory, you can use the `ls()` function. If you want to delete an object from memory, you can use the `rm()` function. For example: ```r x <- 10 # Create an object 'x' ls() # List all objects in memory rm(x) # Remove the object 'x' ``` ##### The On-Line Help R provides comprehensive documentation through its help system. You can access help on any function by typing `?function_name` or `help(function_name)` in the console. Additionally, the `help.search()` function allows you to search for help on specific topics or keywords. For example: ```r ?mean # Get help on the mean function help.search("ANOVA") # Search for ANOVA-related topics ``` #### Data with R ##### Objects In R, data is stored in various types of objects, including vectors, matrices, arrays, data frames, and lists. Each type of object has its own structure and is suited for different purposes. For example: - **Vectors**: One-dimensional arrays that can hold numeric, character, or logical data. - **Matrices**: Two-dimensional arrays that must have the same data type for all elements. - **Arrays**: Multi-dimensional data structures that can hold elements of the same data type. - **Data Frames**: Similar to matrices but can hold different data types in different columns. - **Lists**: Flexible containers that can hold a mix of different types of objects. ##### Reading Data in a File R supports reading data from various file formats, such as CSV, Excel, and text files. The most commonly used function for reading CSV files is `read.csv()`. For example: ```r data <- read.csv("data.csv") ``` ##### Saving Data To save data created within R, you can use the `write.table()` or `write.csv()` functions. For example: ```r write.csv(data, "saved_data.csv", row.names = FALSE) ``` ##### Generating Data R provides several functions to generate data programmatically: - **Regular Sequences**: Use the `seq()` function to generate regular sequences. - **Random Sequences**: Functions like `rnorm()`, `runif()`, etc., can be used to generate random numbers following specific distributions. ##### Manipulating Objects R offers a wide range of functions and operators to manipulate objects. Some common operations include: - **Creating Objects**: Use assignment operators (`<-` or `=`) to create new objects. - **Converting Objects**: Functions like `as.numeric()`, `as.character()`, etc., can be used to convert one data type to another. - **Operators**: Arithmetic (`+`, `-`, `*`, `/`), relational (`<`, `>`, `==`), and logical (`&&`, `||`, `!`) operators are available. - **Indexing System**: Access elements of an object using indexing (e.g., `data[1,2]` to access the element in the first row and second column of a matrix). - **Accessing Values with Names**: Use named indexing (e.g., `data$name`) to access values based on names. - **Data Editor**: The `edit()` function opens an interactive editor to modify data. - **Arithmetic and Simple Functions**: Common mathematical operations and functions are supported (e.g., `sum()`, `mean()`, `sd()`). - **Matrix Computation**: Functions like `t()` (transpose), `solve()` (matrix inversion), and `%*%` (matrix multiplication) are available for matrix computations. #### Graphics with R ##### Managing Graphics R provides flexible tools for creating graphics. You can open multiple graphical devices and partition a single device into multiple plots. Functions like `par(mfrow=c(2,2))` can be used to specify the layout of multiple plots in a single device. ##### Graphical Functions R includes numerous built-in functions for generating graphs, such as `plot()`, `hist()`, `boxplot()`, etc. These functions allow you to create various types of plots, including line graphs, bar charts, histograms, and scatter plots. ##### Low-Level Plotting Commands For more advanced customization, low-level plotting commands like `points()`, `lines()`, `text()`, and `polygon()` can be used to add elements to existing plots. ##### Graphical Parameters You can customize the appearance of plots using graphical parameters. These parameters control aspects like colors, fonts, and line styles. For example: ```r plot(x, y, col="red", pch=19, cex=2) ``` ##### A Practical Example Let’s create a simple scatter plot: ```r x <- rnorm(50) y <- rnorm(50) plot(x, y, main="Scatter Plot", xlab="X-axis", ylab="Y-axis") ``` ##### The grid and lattice Packages The `grid` and `lattice` packages provide advanced graphics capabilities. The `grid` package offers low-level functions for creating complex graphical layouts, while the `lattice` package provides high-level functions for creating trellis displays, which are particularly useful for visualizing relationships in multivariate data. #### Statistical Analyses with R ##### A Simple Example of Analysis of Variance R provides powerful tools for conducting statistical analyses, such as ANOVA (Analysis of Variance). For example, you can perform a one-way ANOVA using the `aov()` function: ```r fit <- aov(y ~ group, data=mydata) summary(fit) ``` ##### Formulae In R, formulae are used to specify the model structure in statistical analyses. They are written in the form `response ~ predictors`, where `response` is the dependent variable and `predictors` are the independent variables. ##### Generic Functions R uses generic functions to handle different types of objects and perform specific actions based on the class of the object. For example, the `print()` function can print the contents of various types of objects, while the `summary()` function provides a summary of statistical models. ##### Packages R relies heavily on packages to extend its functionality. Popular packages for statistical analysis include `ggplot2` for data visualization, `tidyverse` for data manipulation, and `lme4` for mixed-effects modeling. #### Programming with R in Practice ##### Loops and Vectorization While loops are a fundamental part of programming, R emphasizes vectorized operations, which are more efficient and easier to read. For example, instead of using a loop to sum the elements of a vector, you can simply use the `sum()` function: ```r v <- c(1, 2, 3, 4, 5) # Using a loop total <- 0 for (i in v) { total <- total + i } # Using vectorization total_vectorized <- sum(v) ``` This document provides a comprehensive introduction to R, covering essential concepts and operations. By mastering these basics, you will be well-equipped to tackle more advanced topics and applications in R programming.
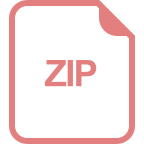
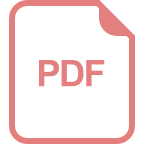
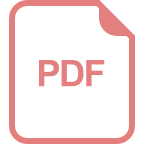
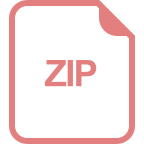
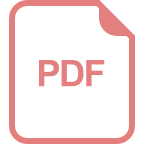
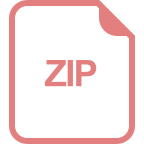
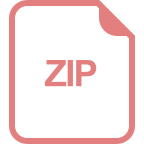
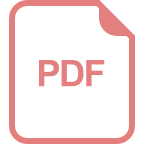
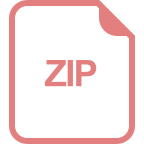
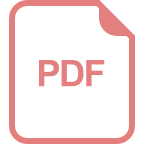
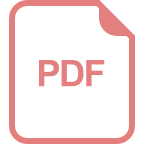
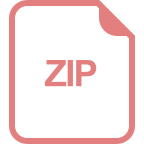
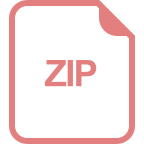
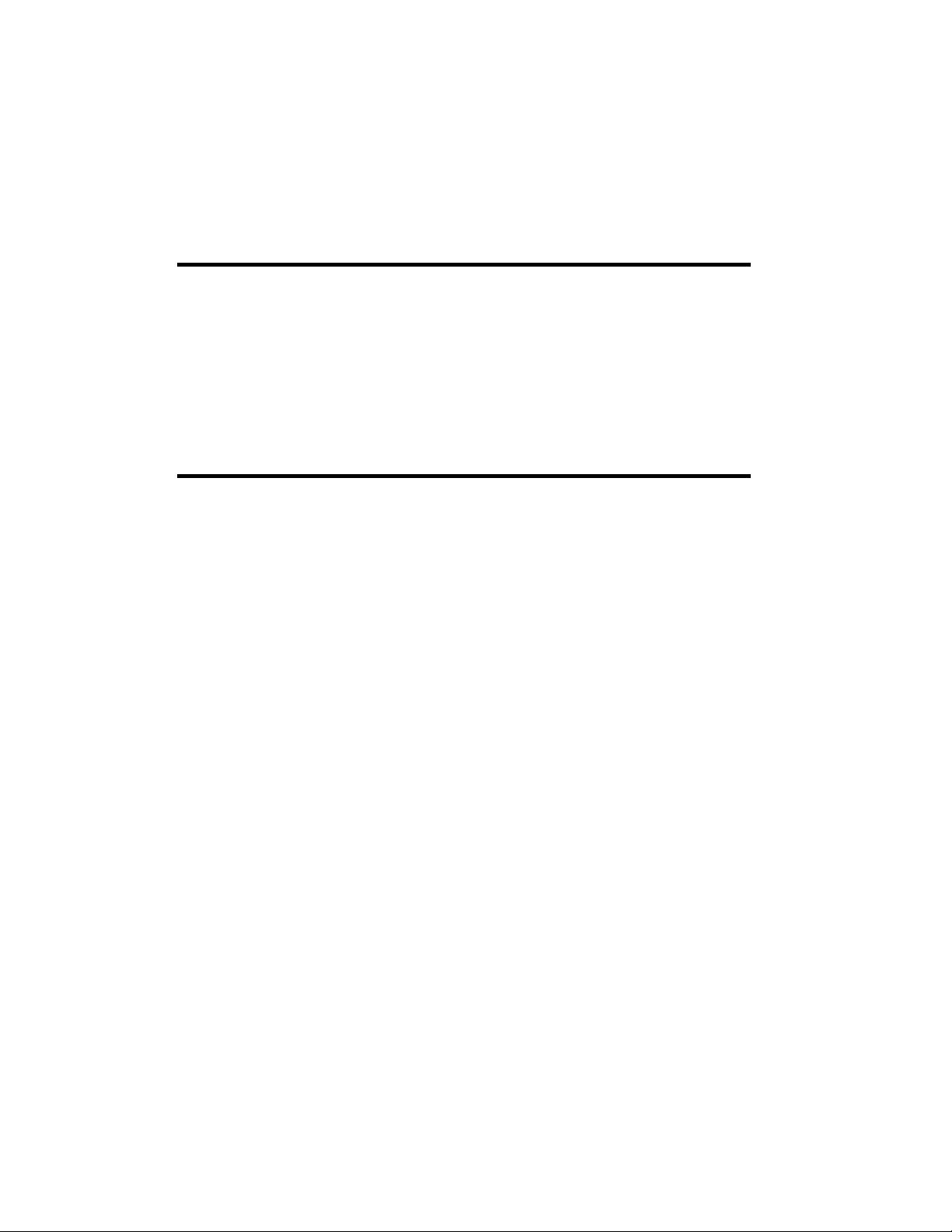
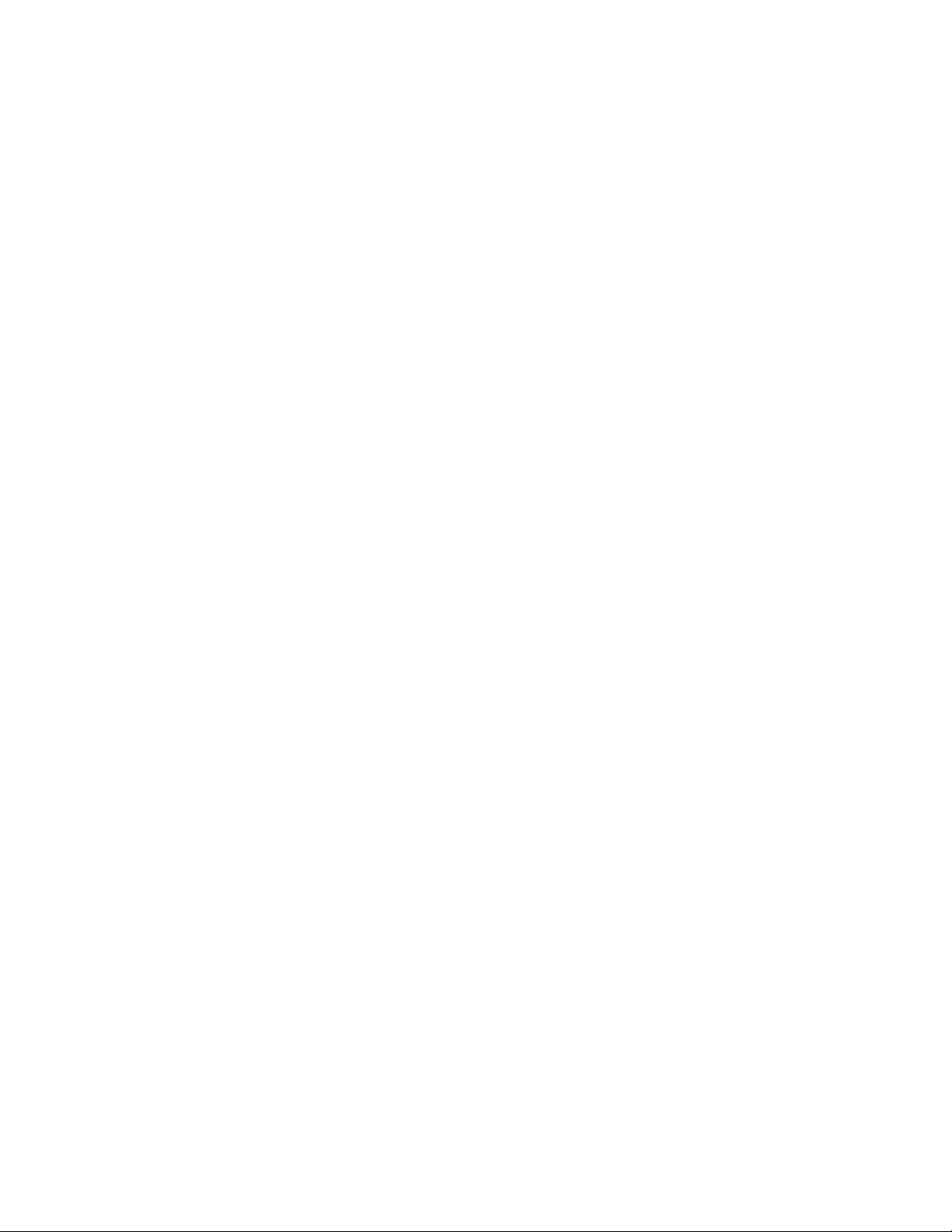
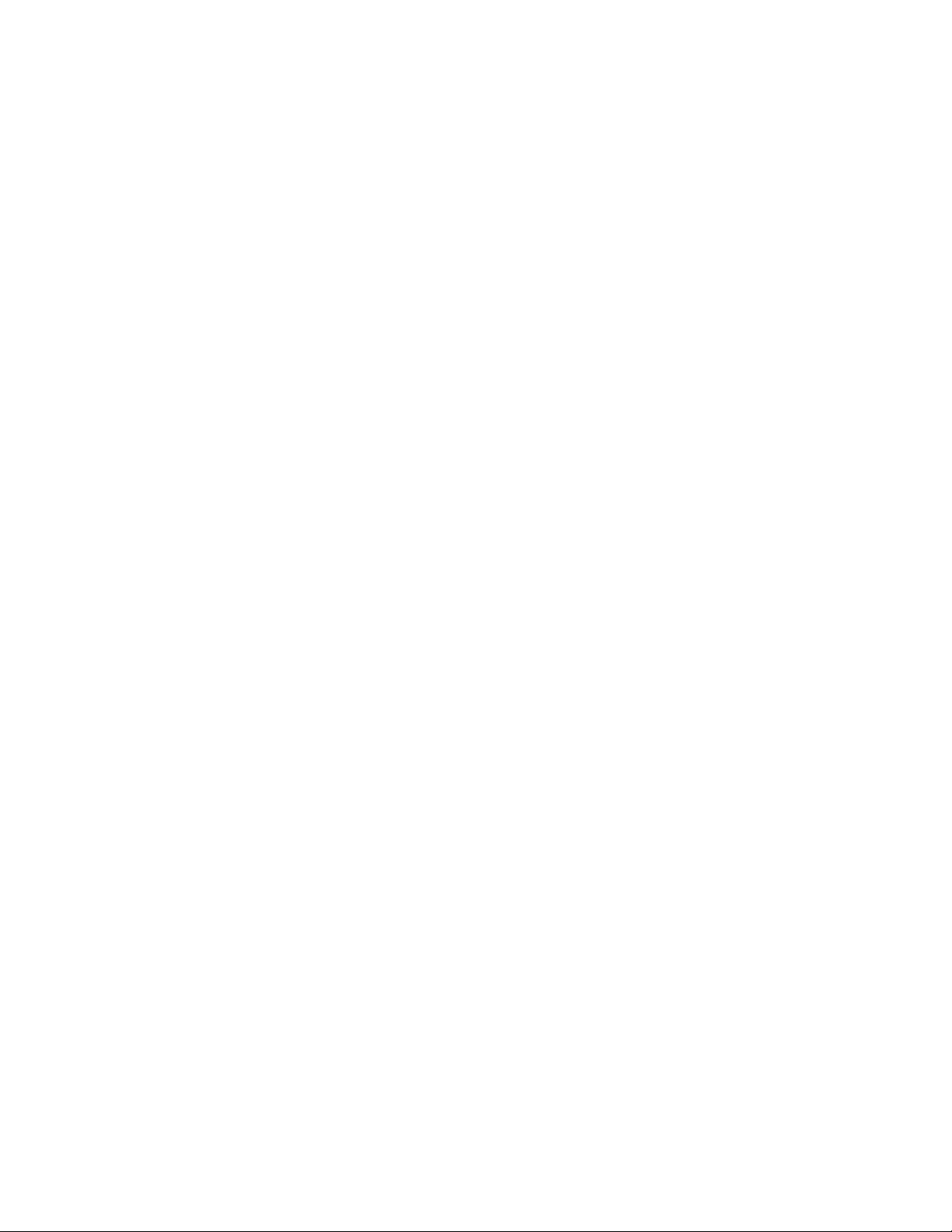
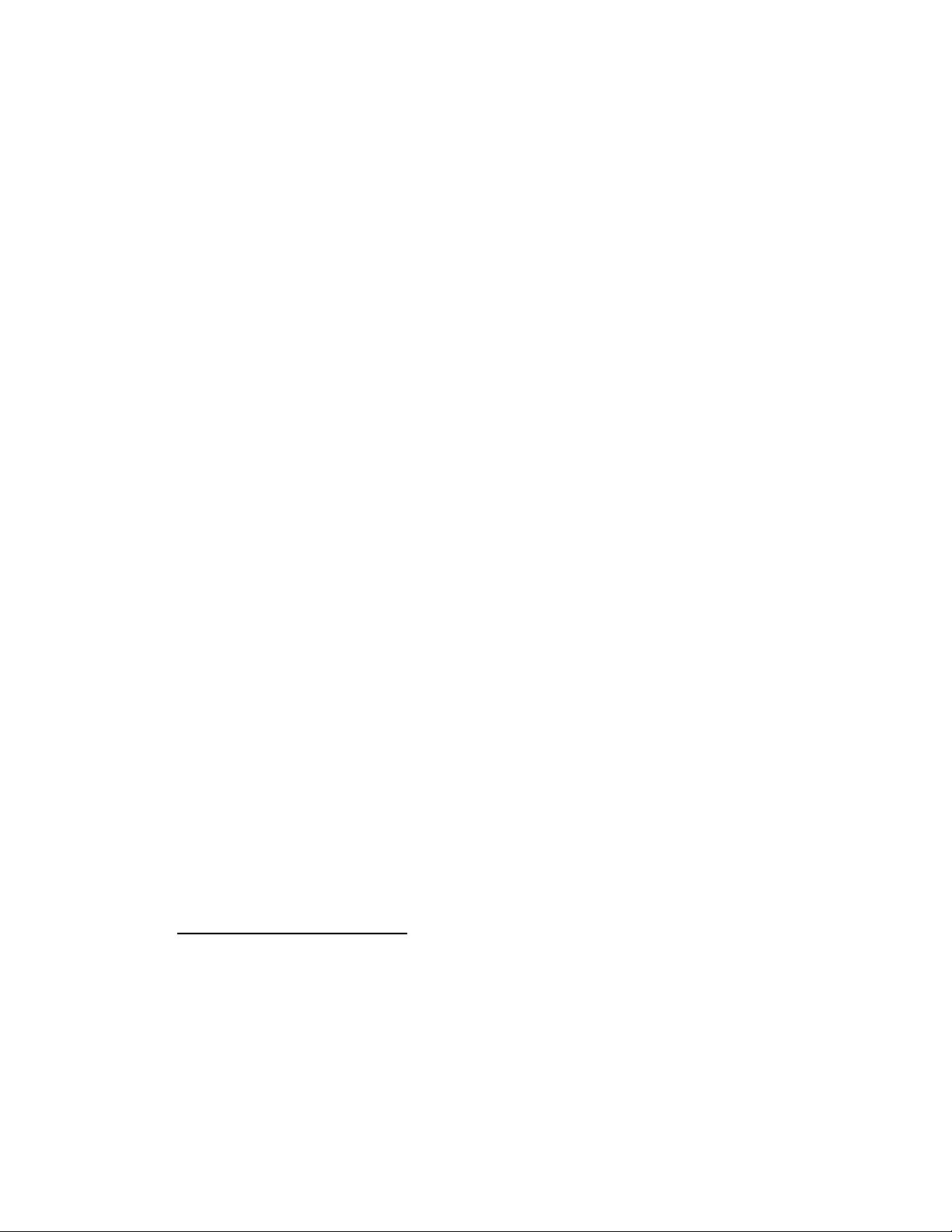
剩余75页未读,继续阅读
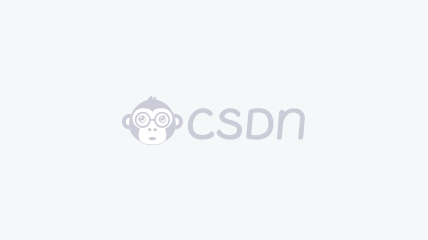

- 粉丝: 1
- 资源: 3
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

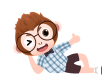
最新资源

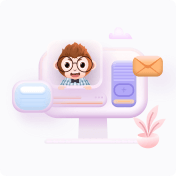
