/*
pygame - Python Game Library
Copyright (C) 2000-2001 Pete Shinners
This library is free software; you can redistribute it and/or
modify it under the terms of the GNU Library General Public
License as published by the Free Software Foundation; either
version 2 of the License, or (at your option) any later version.
This library is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
Library General Public License for more details.
You should have received a copy of the GNU Library General Public
License along with this library; if not, write to the Free
Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
Pete Shinners
pete@shinners.org
*/
#ifndef _PYGAME_H
#define _PYGAME_H
/** This header file includes all the definitions for the
** base pygame extensions. This header only requires
** SDL and Python includes. The reason for functions
** prototyped with #define's is to allow for maximum
** python portability. It also uses python as the
** runtime linker, which allows for late binding. For more
** information on this style of development, read the Python
** docs on this subject.
** http://www.python.org/doc/current/ext/using-cobjects.html
**
** If using this to build your own derived extensions,
** you'll see that the functions available here are mainly
** used to help convert between python objects and SDL objects.
** Since this library doesn't add a lot of functionality to
** the SDL libarary, it doesn't need to offer a lot either.
**
** When initializing your extension module, you must manually
** import the modules you want to use. (this is the part about
** using python as the runtime linker). Each module has its
** own import_xxx() routine. You need to perform this import
** after you have initialized your own module, and before
** you call any routines from that module. Since every module
** in pygame does this, there are plenty of examples.
**
** The base module does include some useful conversion routines
** that you are free to use in your own extension.
**
** When making changes, it is very important to keep the
** FIRSTSLOT and NUMSLOT constants up to date for each
** section. Also be sure not to overlap any of the slots.
** When you do make a mistake with this, it will result
** is a dereferenced NULL pointer that is easier to diagnose
** than it could be :]
**/
#if defined(HAVE_SNPRINTF) /* defined in python.h (pyerrors.h) and SDL.h \
(SDL_config.h) */
#undef HAVE_SNPRINTF /* remove GCC redefine warning */
#endif
// This must be before all else
#if defined(__SYMBIAN32__) && defined(OPENC)
#include <sys/types.h>
#if defined(__WINS__)
void *
_alloca(size_t size);
#define alloca _alloca
#endif
#endif
#define PG_STRINGIZE_HELPER(x) #x
#define PG_STRINGIZE(x) PG_STRINGIZE_HELPER(x)
#define PG_WARN(desc) message(__FILE__ "(" PG_STRINGIZE(__LINE__) "): WARNING: " #desc)
/* This is unconditionally defined in Python.h */
#if defined(_POSIX_C_SOURCE)
#undef _POSIX_C_SOURCE
#endif
#include <Python.h>
/* the version macros are defined since version 1.9.5 */
#define PG_MAJOR_VERSION 1
#define PG_MINOR_VERSION 9
#define PG_PATCH_VERSION 6
#define PG_VERSIONNUM(MAJOR, MINOR, PATCH) (1000*(MAJOR) + 100*(MINOR) + (PATCH))
#define PG_VERSION_ATLEAST(MAJOR, MINOR, PATCH) \
(PG_VERSIONNUM(PG_MAJOR_VERSION, PG_MINOR_VERSION, PG_PATCH_VERSION) >= \
PG_VERSIONNUM(MAJOR, MINOR, PATCH))
/* Cobjects vanish in Python 3.2; so we will code as though we use capsules */
#if defined(Py_CAPSULE_H)
#define PG_HAVE_CAPSULE 1
#else
#define PG_HAVE_CAPSULE 0
#endif
#if defined(Py_COBJECT_H)
#define PG_HAVE_COBJECT 1
#else
#define PG_HAVE_COBJECT 0
#endif
#if !PG_HAVE_CAPSULE
#define PyCapsule_New(ptr, n, dfn) PyCObject_FromVoidPtr(ptr, dfn)
#define PyCapsule_GetPointer(obj, n) PyCObject_AsVoidPtr(obj)
#define PyCapsule_CheckExact(obj) PyCObject_Check(obj)
#endif
/* Pygame uses Py_buffer (PEP 3118) to exchange array information internally;
* define here as needed.
*/
#if !defined(PyBUF_SIMPLE)
typedef struct bufferinfo {
void *buf;
PyObject *obj;
Py_ssize_t len;
Py_ssize_t itemsize;
int readonly;
int ndim;
char *format;
Py_ssize_t *shape;
Py_ssize_t *strides;
Py_ssize_t *suboffsets;
void *internal;
} Py_buffer;
/* Flags for getting buffers */
#define PyBUF_SIMPLE 0
#define PyBUF_WRITABLE 0x0001
/* we used to include an E, backwards compatible alias */
#define PyBUF_WRITEABLE PyBUF_WRITABLE
#define PyBUF_FORMAT 0x0004
#define PyBUF_ND 0x0008
#define PyBUF_STRIDES (0x0010 | PyBUF_ND)
#define PyBUF_C_CONTIGUOUS (0x0020 | PyBUF_STRIDES)
#define PyBUF_F_CONTIGUOUS (0x0040 | PyBUF_STRIDES)
#define PyBUF_ANY_CONTIGUOUS (0x0080 | PyBUF_STRIDES)
#define PyBUF_INDIRECT (0x0100 | PyBUF_STRIDES)
#define PyBUF_CONTIG (PyBUF_ND | PyBUF_WRITABLE)
#define PyBUF_CONTIG_RO (PyBUF_ND)
#define PyBUF_STRIDED (PyBUF_STRIDES | PyBUF_WRITABLE)
#define PyBUF_STRIDED_RO (PyBUF_STRIDES)
#define PyBUF_RECORDS (PyBUF_STRIDES | PyBUF_WRITABLE | PyBUF_FORMAT)
#define PyBUF_RECORDS_RO (PyBUF_STRIDES | PyBUF_FORMAT)
#define PyBUF_FULL (PyBUF_INDIRECT | PyBUF_WRITABLE | PyBUF_FORMAT)
#define PyBUF_FULL_RO (PyBUF_INDIRECT | PyBUF_FORMAT)
#define PyBUF_READ 0x100
#define PyBUF_WRITE 0x200
#define PyBUF_SHADOW 0x400
typedef int (*getbufferproc)(PyObject *, Py_buffer *, int);
typedef void (*releasebufferproc)(Py_buffer *);
#endif /* #if !defined(PyBUF_SIMPLE) */
/* Flag indicating a pg_buffer; used for assertions within callbacks */
#ifndef NDEBUG
#define PyBUF_PYGAME 0x4000
#endif
#define PyBUF_HAS_FLAG(f, F) (((f) & (F)) == (F))
/* Array information exchange struct C type; inherits from Py_buffer
*
* Pygame uses its own Py_buffer derived C struct as an internal representation
* of an imported array buffer. The extended Py_buffer allows for a
* per-instance release callback,
*/
typedef void (*pybuffer_releaseproc)(Py_buffer *);
typedef struct pg_bufferinfo_s {
Py_buffer view;
PyObject *consumer; /* Input: Borrowed reference */
pybuffer_releaseproc release_buffer;
} pg_buffer;
/* Operating system specific adjustments
*/
// No signal()
#if defined(__SYMBIAN32__) && defined(HAVE_SIGNAL_H)
#undef HAVE_SIGNAL_H
#endif
#if defined(HAVE_SNPRINTF)
#undef HAVE_SNPRINTF
#endif
#ifdef MS_WIN32 /*Python gives us MS_WIN32, SDL needs just WIN32*/
#ifndef WIN32
#define WIN32
#endif
#endif
/// Prefix when initializing module
#define MODPREFIX ""
/// Prefix when importing module
#define IMPPREFIX "pygame."
#ifdef __SYMBIAN32__
#undef MODPREFIX
#undef IMPPREFIX
// On Symbian there is no pygame package. The extensions are built-in or in
// sys\bin.
#define MODPREFIX "pygame_"
#define IMPPREFIX "pygame_"
#endif
#include <SDL.h>
/* Pygame's SDL version macros:
* IS_SDLv1 is 1 if SDL 1.x.x, 0 otherwise
* IS_SDLv2 is 1 if at least SDL 2.0.0, 0 otherwise
*/
#if (SDL_VERSION_ATLEAST(2, 0, 0))
#define IS_SDLv1 0
#define IS_SDLv2 1
#else
#define IS_SDLv1 1
#define IS_SDLv2 0
#endif
/*#if IS_SDLv1 && PG_MAJOR_VERSION >= 2
#error pygame 2 requires SDL 2
#endif*/
#if IS_SDLv2
/* SDL 1.2 constants removed from SDL 2 */
typedef enum {
SDL_HWSURFACE = 0,
SDL_RESIZABLE = SDL_WINDOW_RESIZABLE,
SDL_ASYNCBLIT = 0,
SDL_OPENGL = SDL_WINDOW_OPENGL,
SDL_OPENGLBLIT = 0,
SDL_ANYFORMAT = 0,
SDL_HWPALETTE = 0,
SDL_DOUBLEBUF = 0,
SDL_FULLSCREEN = SDL_WINDOW_FULLSCREEN,
SDL_HWACCEL = 0,
SDL_SRCCOLORKEY = 0,
SDL_RLEACCELOK = 0,
SDL_SRCALPHA = 0,
SDL_NOFRAME = SDL_WINDOW_BORDERLESS,
SDL_GL_SWAP_CONTROL = 0,
TIMER_RESOLUTION = 0
} PygameVideoFlags;
/* the wheel button constants were removed from SDL 2 */
typedef enum {
PGM_BUTTON_LEFT = SDL_BUTTON_LEFT,
PGM_BUTTON_RIGHT = SD
没有合适的资源?快使用搜索试试~ 我知道了~
蛇蛇闯关Adapted-game-snake-master
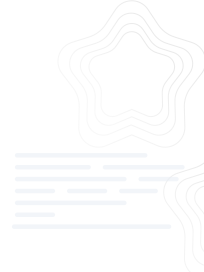
共1118个文件
py:693个
png:108个
pyd:76个

需积分: 5 0 下载量 141 浏览量
2023-11-30
17:15:09
上传
评论
收藏 54.57MB ZIP 举报
温馨提示
独特的闯关模式,更加平滑的360度移动,炫酷的攻击动画,耐听又贴切的背景音乐 欢迎你,新的挑战者! 一共七关 每关都有不同的挑战 激光,大炮…… 但是对于一个吃货蛇来说,这都不算什么 为了获得无限的食物而奋斗吧!
资源推荐
资源详情
资源评论
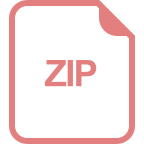
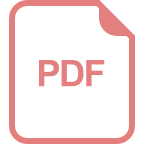
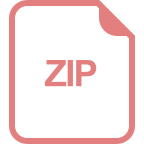
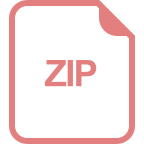
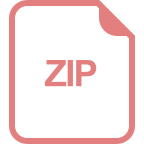
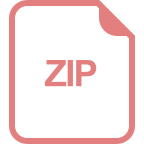
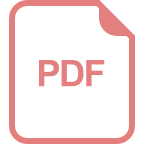
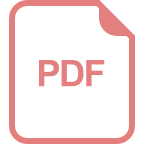
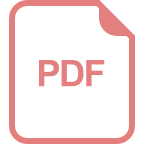
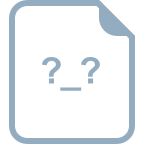
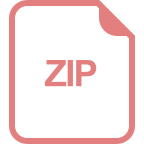
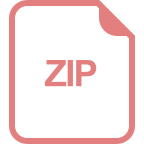
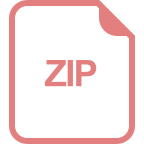
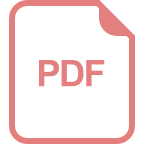
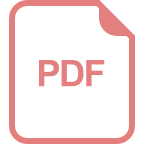
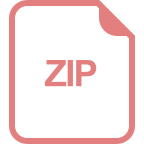
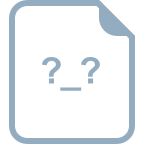
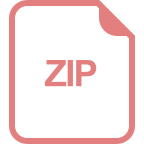
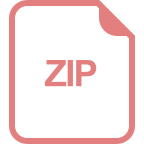
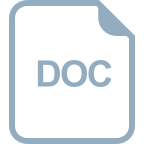
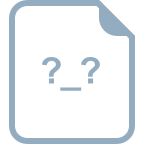
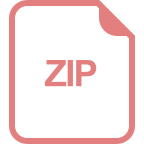
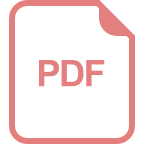
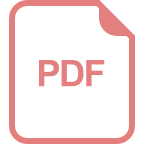
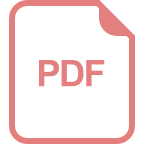
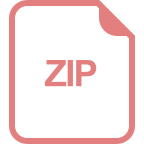
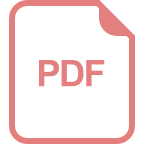
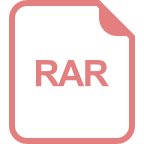
收起资源包目录

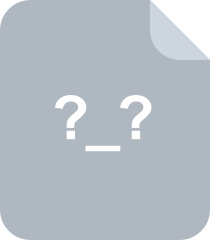
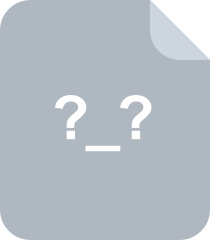
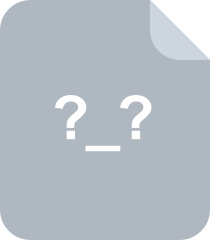
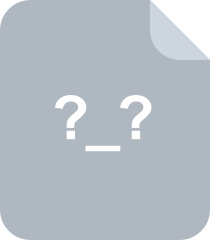
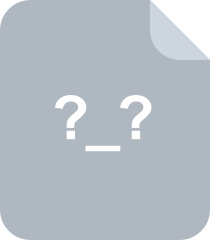
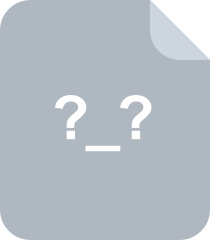
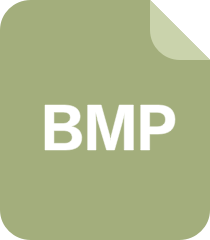
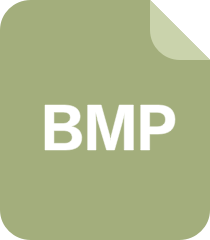
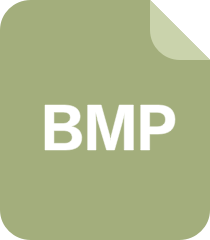
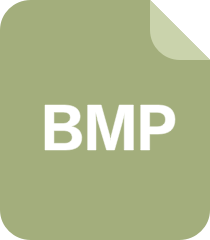
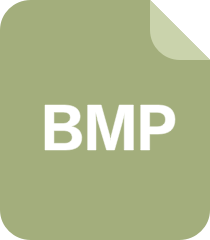
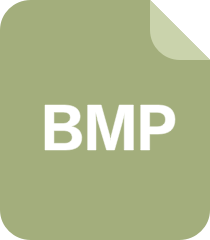
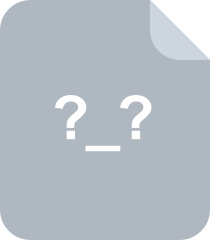
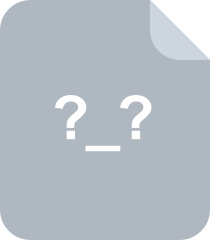
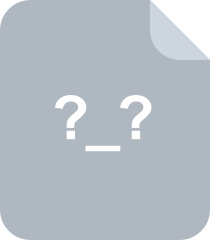
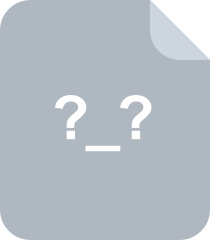
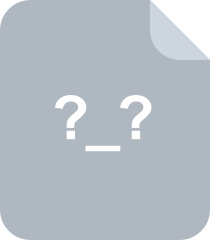
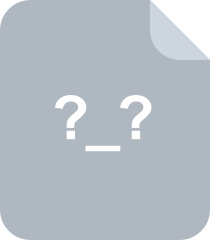
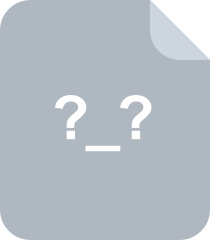
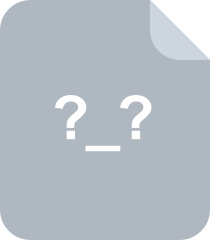
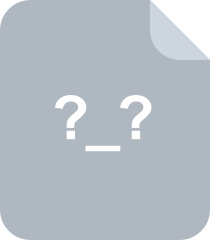
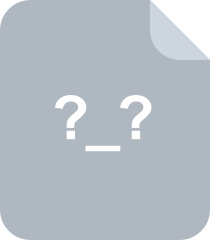
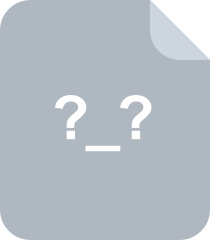
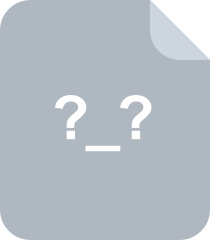
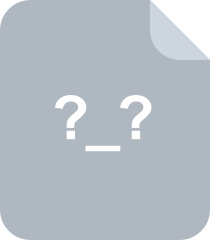
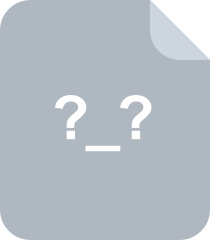
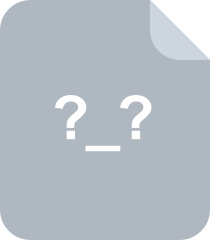
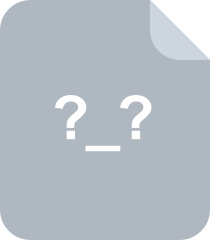
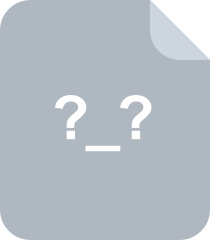
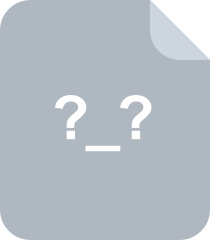
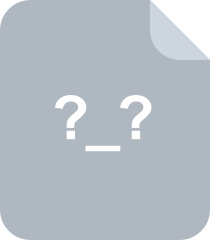
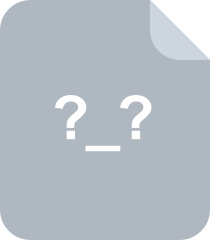
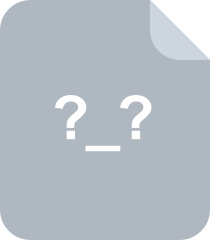
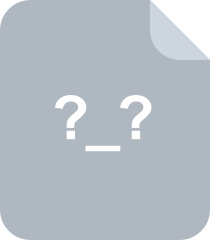
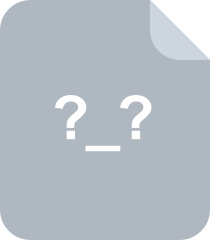
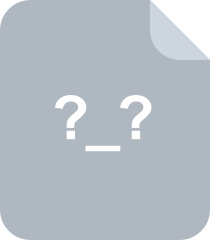
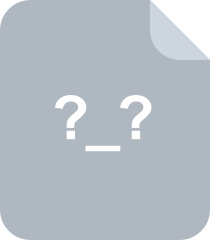
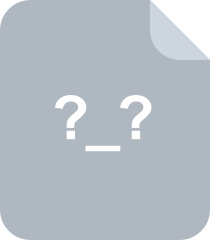
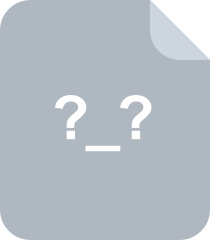
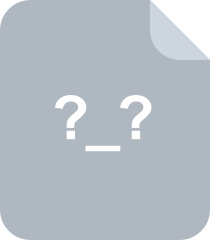
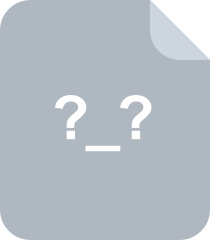
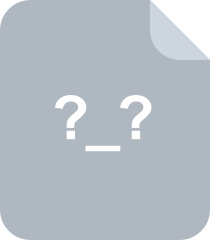
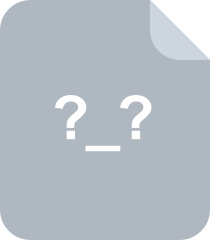
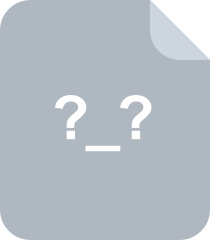
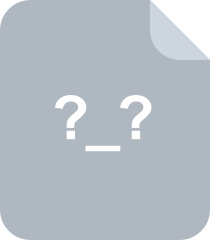
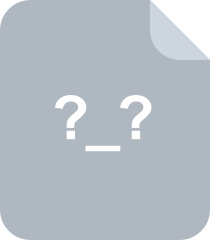
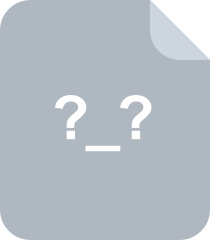
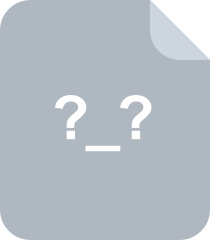
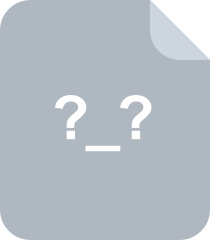
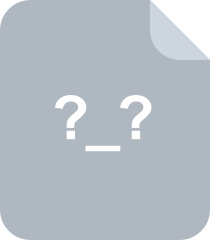
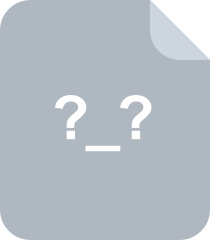
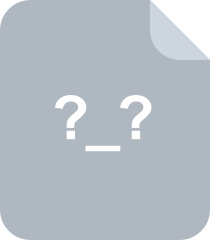
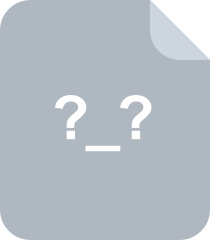
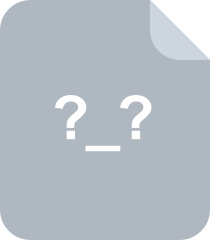
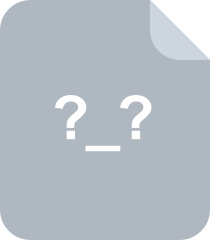
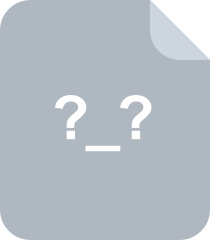
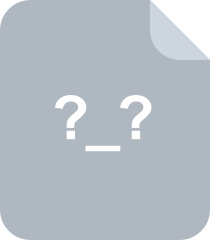
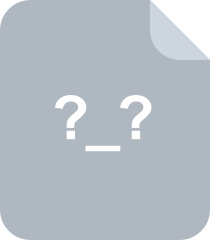
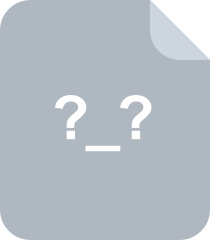
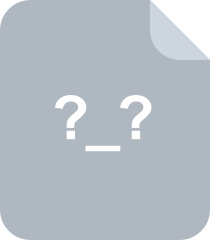
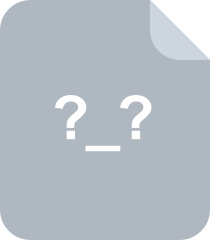
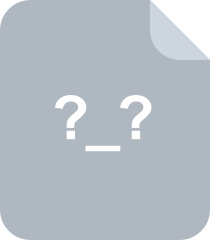
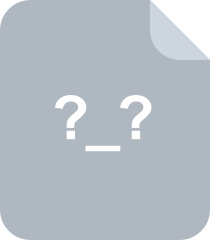
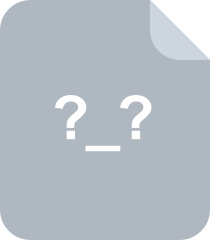
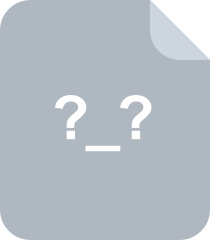
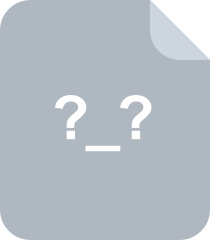
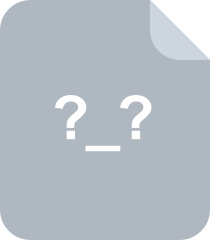
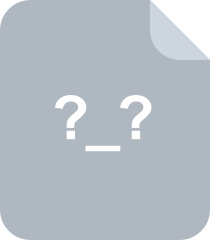
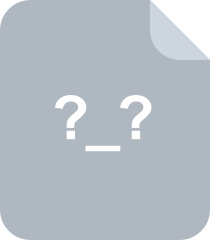
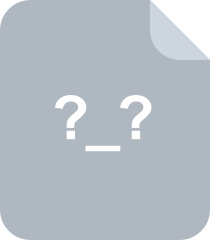
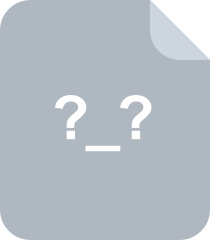
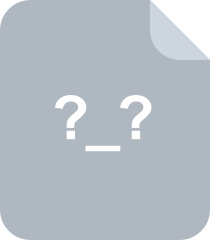
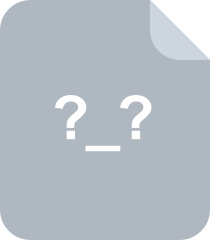
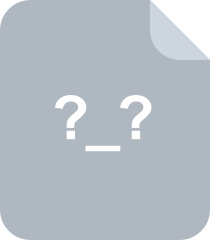
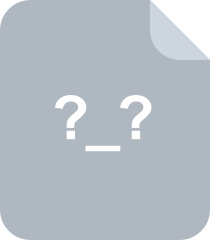
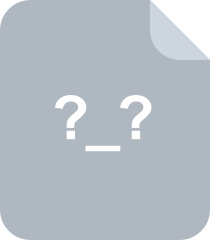
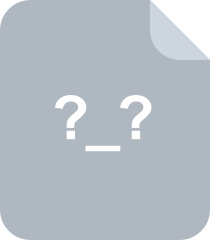
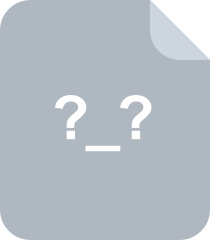
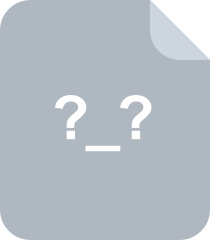
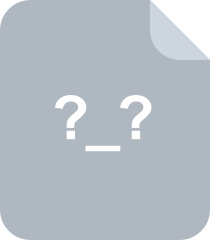
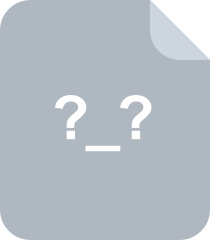
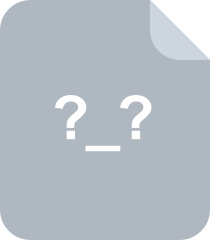
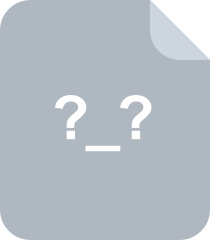
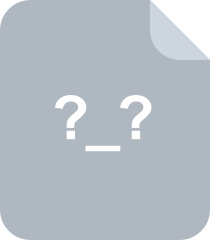
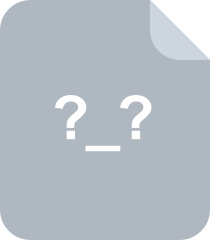
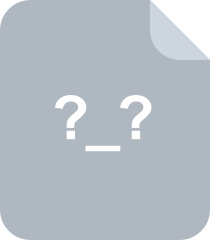
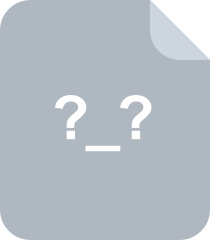
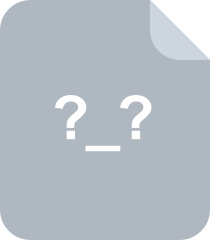
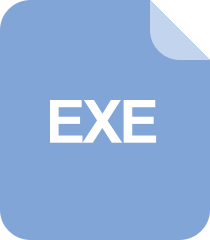
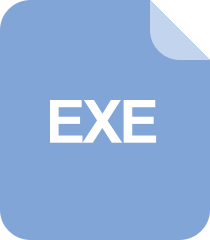
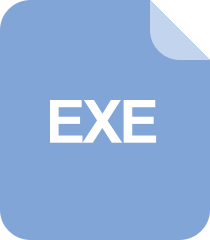
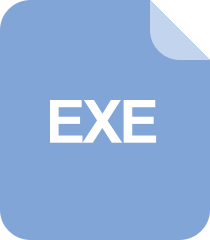
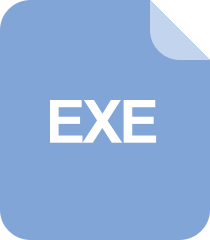
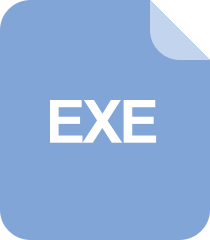
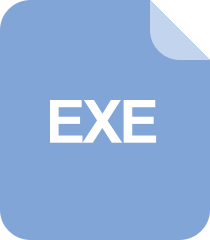
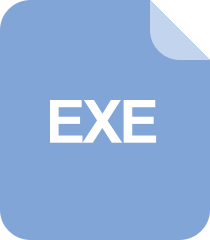
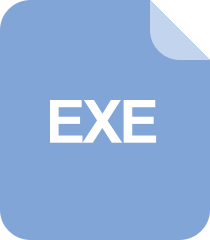
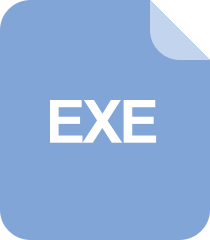
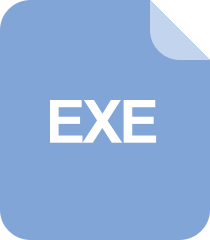
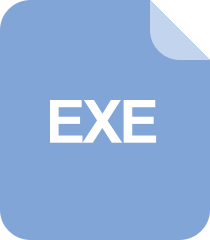
共 1118 条
- 1
- 2
- 3
- 4
- 5
- 6
- 12
资源评论
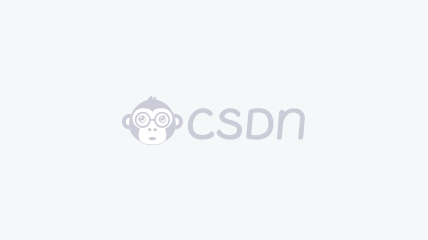

流华追梦
- 粉丝: 9354
- 资源: 3842
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

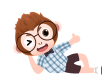
最新资源
- 基于C++和 Qt 的影院票务系统源码(高分项目代码)
- 解析抖音视频 下载直接按照就可用
- 【java毕业设计】springboot智能健康饮食系统(springboot+vue+mysql+说明文档).zip
- 【java毕业设计】springboot基于java的网上订餐系统(springboot+vue+mysql+说明文档).zip
- 2633996324RUSH_TANK_RACE_SA2.1_48CH.json
- 【java毕业设计】springbootJava区社区停车信息管理系统(springboot+mysql+说明文档).zip
- mobilenetv2-12.onnx
- 2024最新股票系统源码 附详细教程
- 2048游戏(附源码)
- 4%2Fpf%2Ffiles%2FKKDuiZhanDownloader-v1.0.1.409.exe
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


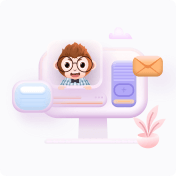
安全验证
文档复制为VIP权益,开通VIP直接复制
