在iOS开发中,Delegate是一种非常重要的设计模式,它允许对象之间进行通信,特别是当一个对象(委托者)需要通知另一个对象(委托对象)某些事件发生时。本文将深入讲解“简单的Delegate实现”,并以实际代码为例,帮助你理解这一概念。 Delegate在iOS中的主要作用是传递消息或事件。例如,UITableView需要知道何时应该加载数据,如何显示单元格,用户点击了哪个单元格等。这些事件由UITableView的Delegate来处理。以下是一个简单的Delegate实现过程: 1. **定义协议**: 我们需要定义一个协议(Protocol)。协议定义了委托对象需要遵循的方法。在Objective-C中,我们使用`@protocol`关键字来创建协议。例如: ```objc @protocol SimpleDelegate <NSObject> - (void)didTapButton:(UIButton *)sender; @end ``` 这里定义了一个名为`SimpleDelegate`的协议,包含一个`didTapButton:`方法,用于处理按钮被点击的事件。 2. **实现协议**: 然后,你需要让一个类实现这个协议。在类声明中,使用`<SimpleDelegate>`来表明该类遵循这个协议。例如: ```objc @interface ViewController : UIViewController <SimpleDelegate> ... @end ``` 在这个例子中,ViewController类不仅继承自UIViewController,还遵循了SimpleDelegate协议,因此它必须实现`didTapButton:`方法。 3. **设置Delegate**: 在需要使用委托的对象中,通常会有一个属性来存储Delegate。例如,在UIControl的子类中,我们可以设置一个Delegate属性: ```objc @interface CustomButton : UIButton @property (nonatomic, weak) id<SimpleDelegate> delegate; ... @end ``` 4. **处理事件**: 当事件发生时,比如按钮被点击,我们调用Delegate上的相应方法。在CustomButton的`- (void)touchesEnded:(NSSet<UITouch *> *)touches withEvent:(UIEvent *)event`方法中,我们可以添加如下代码: ```objc - (void)touchesEnded:(NSSet<UITouch *> *)touches withEvent:(UIEvent *)event { [super touchesEnded:touches withEvent:event]; if ([self.delegate respondsToSelector:@selector(didTapButton:)]) { [self.delegate didTapButton:self]; } } ``` 5. **设置Delegate实例**: 在需要响应事件的类(如ViewController)中,我们设置CustomButton的Delegate为自身,并实现协议中的方法: ```objc - (void)viewDidLoad { [super viewDidLoad]; CustomButton *button = [[CustomButton alloc] initWithFrame:CGRectMake(0, 0, 100, 50)]; button.delegate = self; [self.view addSubview:button]; } - (void)didTapButton:(UIButton *)sender { NSLog(@"Button tapped!"); } ``` 通过以上步骤,我们就完成了Delegate的基本实现。当你点击CustomButton时,ViewController的`didTapButton:`方法会被调用,从而实现了对象间的通信。这种设计模式在iOS开发中广泛应用,如UITableView、UICollectionView、UINavigationController等,极大地提高了代码的可维护性和灵活性。 在提供的`DelegateSample`压缩包中,你应该能找到一个具体的Delegate实现示例,包括定义协议、实现协议、设置Delegate以及处理事件的代码。通过阅读和分析这些代码,你会对Delegate有更深入的理解。记得实践是检验真理的唯一标准,动手尝试一下,你将在实践中更好地掌握Delegate的运用。
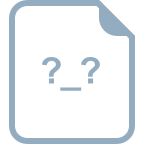
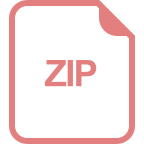
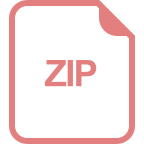
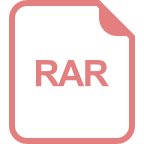
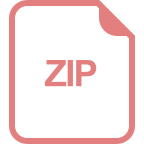
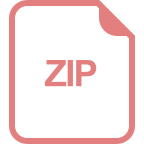
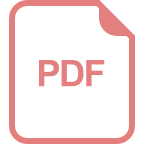
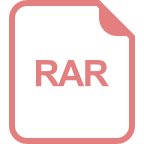
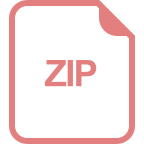
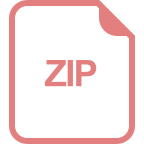
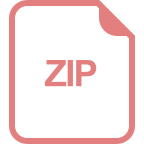
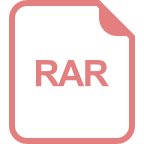
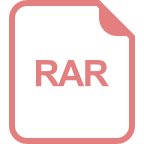
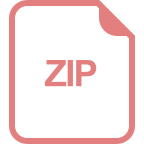
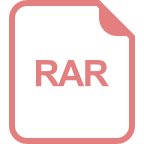
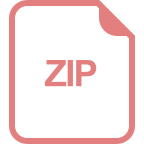
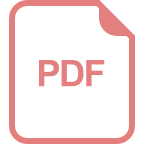
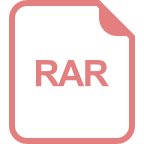
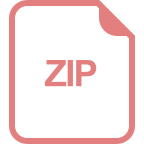
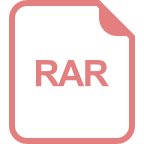
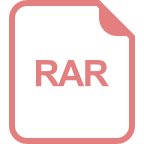
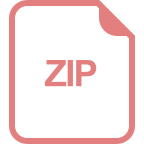
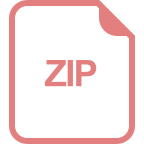


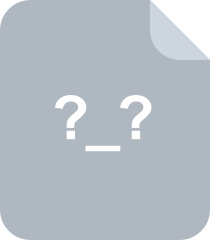



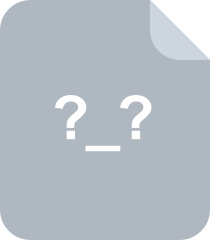
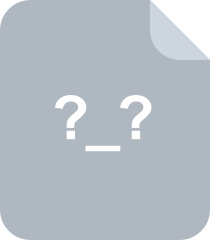
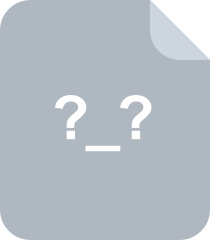
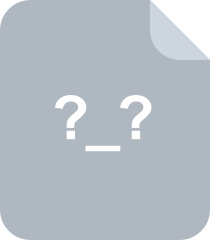
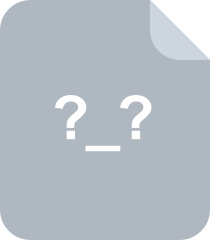
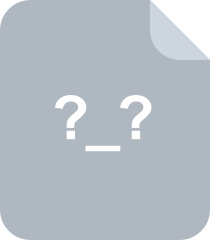




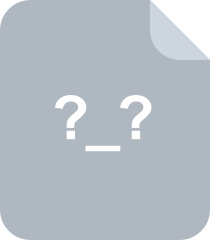
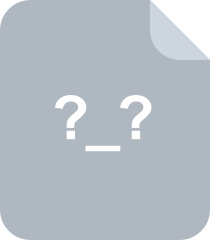



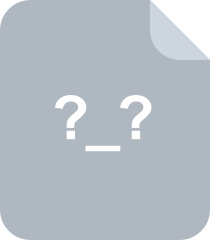
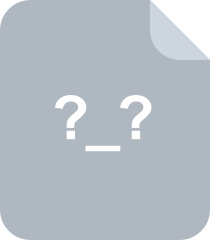


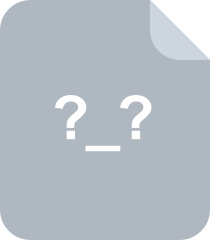
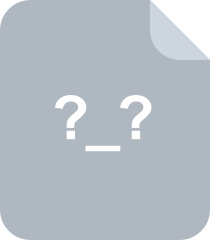
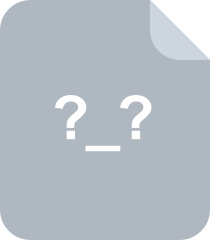
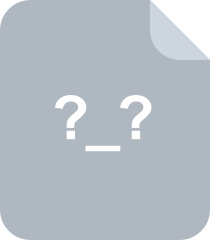
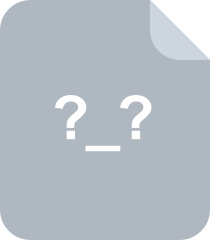
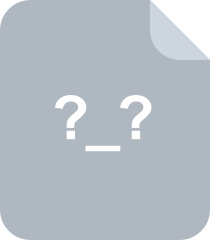
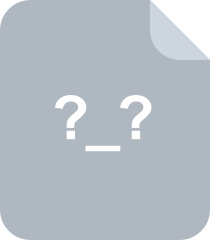
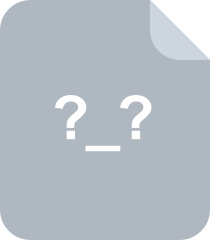
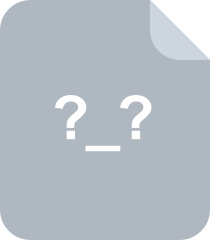
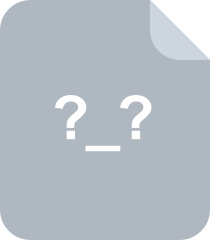
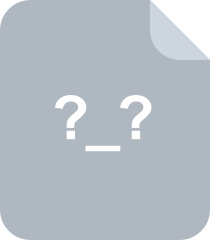
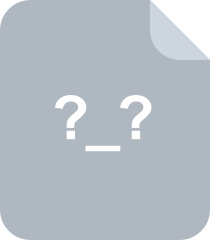
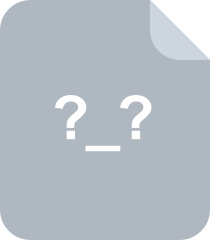
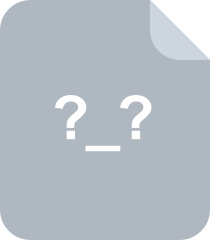

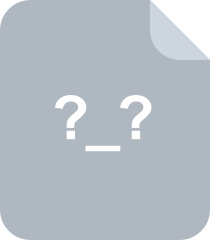
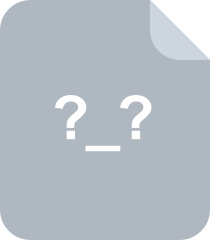
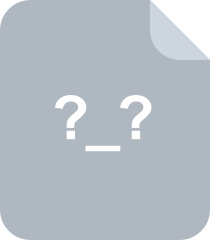
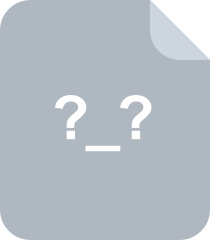
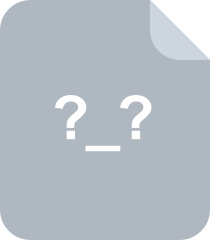
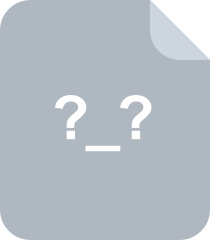
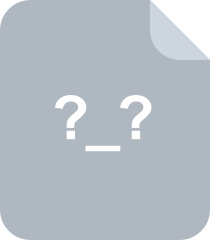
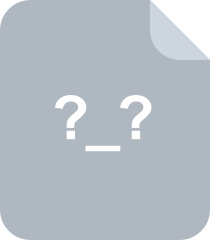
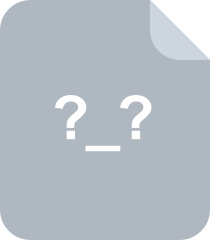

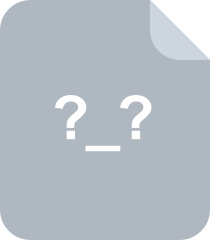
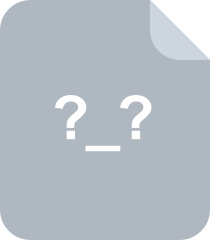
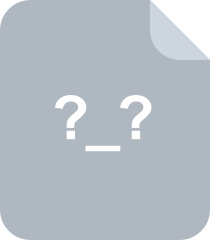
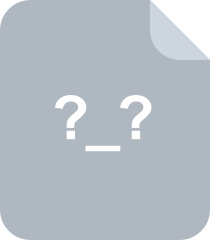

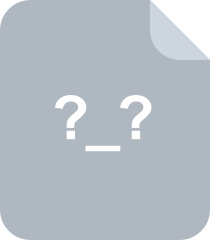
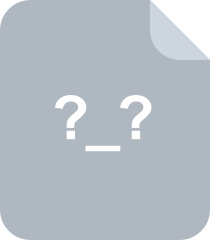
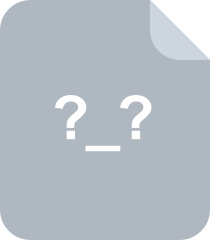
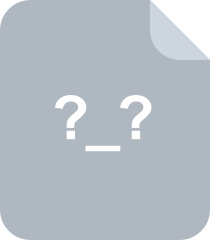
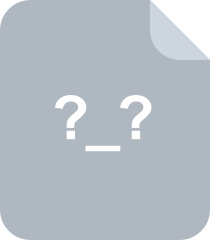
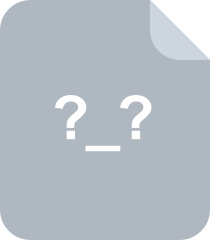
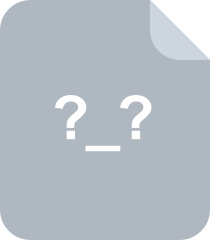
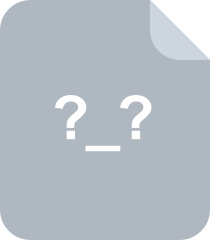

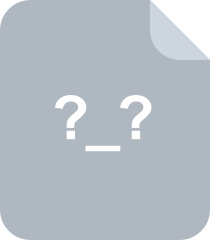
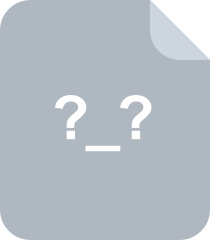
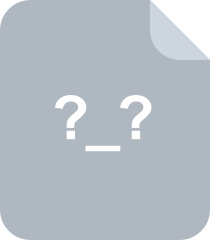
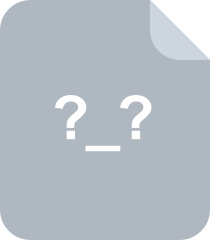
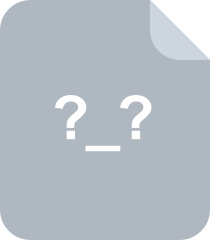
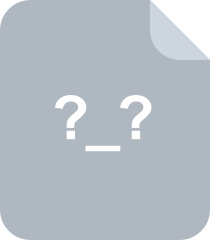
- 1
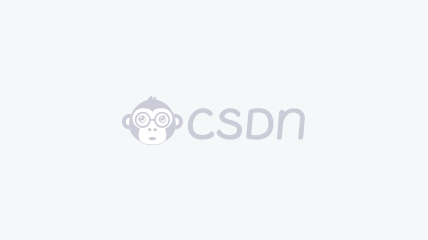
- 杨锘2012-07-16做的是很详细,可惜我对delegate就是不开窍……
- terrorist912013-04-17好吧,还是挺不错的

- 粉丝: 2
- 资源: 15
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

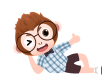
最新资源
- x64dbg-development-2022-09-07-14-52.zip
- 多彩吉安红色旅游网站-JAVA-基于springBoot多彩吉安红色旅游网站的设计与实现
- 本 repo 包含使用新 cv2 接口的 OpenCV-Python 库教程.zip
- 更新框架 (TUF) 的 Python 参考实现.zip
- Qos,GCC,pacing,Nack
- 章节1:Python入门视频
- 无需样板的 Python 类.zip
- ESP32 : 32-bit MCU & 2.4 GHz Wi-Fi & BT/BLE SoCs
- 博物馆文博资源库-JAVA-基于springBoot博物馆文博资源库系统设计与实现
- 旅游网站-JAVA-springboot+vue的桂林旅游网站系统设计与实现

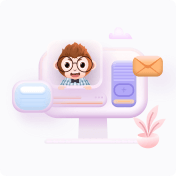
