package cc.bean.sport.daoImpl;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.List;
import cc.bean.sport.DBconect.JdbcConnect;
import cc.bean.sport.dao.SportDAO;
import cc.bean.sport.vo.Record;
import cc.bean.sport.vo.Result;
public class SportDAOImpl implements SportDAO
{
// 根据sId查询出该学校的所有信息
public List<Record> queryBysId(String id) throws Exception
{
String sql = null;//sql语句
PreparedStatement pst = null;
JdbcConnect jdbc = null;
ResultSet rs = null;
Record record = null;
List<Record> all = new ArrayList<Record>();
sql = "SELECT sName,proName,proInt,proResult FROM record WHERE sId=?";
try
{
jdbc = new JdbcConnect();
pst = jdbc.getConnection().prepareStatement(sql);
pst.setString(1, id);
rs = pst.executeQuery();
while ( rs.next() )
{
record = new Record();
record.setSName((String) rs.getString("sName"));
record.setProName((String) rs.getString("proName"));
record.setProResult((String) rs.getString("proResult"));
record.setProInt((Integer) rs.getInt("proInt"));
all.add(record);
}
pst.close();
}
catch (Exception e)
{
e.printStackTrace();
}
finally
{
jdbc.close();
}
return all;
}
// 更新学校比赛项目的成绩及单项积分
public void updateBasic(Record record) throws Exception
{
String sql = null;//sql语句
PreparedStatement pst = null;
JdbcConnect jdbc = null;
sql = "UPDATE record SET proResult=?,proInt=? WHERE sId=? and proName=?";
try
{
jdbc = new JdbcConnect();
pst = jdbc.getConnection().prepareStatement(sql);
pst.setString(1, record.getProResult());
pst.setInt(2, record.getProInt());
pst.setString(3, record.getSId());
pst.setString(4, record.getProName());
pst.executeUpdate();
pst.close();
}
catch (Exception e)
{
e.printStackTrace();
}
finally
{
jdbc.close();
}
}
// 统计某个学校的总分
public void updateSumInt(Result result) throws Exception
{
String sql = null;//sql语句
PreparedStatement pst = null;
JdbcConnect jdbc = null;
List<Record> all = new ArrayList<Record>();
Record record = null;
int mInt = 0, //记录男子团体总积分
wInt = 0, //记录女子团体总积分
sumInt = 0;//记录该学校团体总积分
all = queryBysId(result.getSId());//获取某学校的积分情况
Iterator<Record> it = all.iterator();
while ( it.hasNext() )
{
record = it.next();
if ((record.getProName()).startsWith("w"))
{
wInt = wInt + record.getProInt();
}
else
{
mInt = mInt + record.getProInt();
}
}
sumInt = wInt + mInt;
result.setMInt(mInt);
result.setWInt(wInt);
result.setSumInt(sumInt);
sql = "UPDATE result SET mInt=?,wInt=?,sumInt=? WHERE sId=?";
try
{
jdbc = new JdbcConnect();
pst = jdbc.getConnection().prepareStatement(sql);
pst.setInt(1, mInt);
pst.setInt(2, wInt);
pst.setInt(3, sumInt);
pst.setString(4, result.getSId());
pst.executeUpdate();
pst.close();
}
catch (Exception e)
{
e.printStackTrace();
}
finally
{
jdbc.close();
}
}
// 按不同排序方式输出所有学校
public List<Result> querySortsumIntByAll(int id) throws Exception
{
Comp_mIntImpl comp_mInt = new Comp_mIntImpl();
Comp_wIntImpl comp_wInt = new Comp_wIntImpl();
String sql = null;//sql语句
PreparedStatement pst = null;
JdbcConnect jdbc = null;
ResultSet rs = null;
Result result = null;
List<Result> all = new LinkedList<Result>();
sql = "SELECT * FROM result";
try
{
jdbc = new JdbcConnect();
pst = jdbc.getConnection().prepareStatement(sql);
rs = pst.executeQuery();
while ( rs.next() )
{
result = new Result();
result.setSId((String) rs.getString(1));
result.setSName((String) rs.getString(2));
result.setMInt((Integer) rs.getInt(3));
result.setWInt((Integer) rs.getInt(4));
result.setSumInt((Integer) rs.getInt(5));
all.add(result);
}
pst.close();
}
catch (Exception e)
{
e.printStackTrace();
}
finally
{
jdbc.close();
}
if (id == 4)
{
Collections.sort(all);//按学校团体积分排序
return all;
}
else if (id == 2)
{
Collections.sort(all, comp_mInt);//按男子团体积分排序
return all;
}
else if (id == 3)
{
Collections.sort(all, comp_wInt);//按女子团体积分排序
return all;
}
else
{
return all;//按编号
}
}
// 按照项目名称查询,取前三名的学校的情况
public List<Record> querySortIntByPro(String proName) throws Exception
{
String sql = null;//sql语句
PreparedStatement pst = null;
JdbcConnect jdbc = null;
ResultSet rs = null;
Record record = null;
List<Record> all = new LinkedList<Record>();
sql = "SELECT sId,sName,proResult,proInt FROM record WHERE proName=?";
try
{
jdbc = new JdbcConnect();
pst = jdbc.getConnection().prepareStatement(sql);
pst.setString(1, proName);
rs = pst.executeQuery();
while ( rs.next() )
{
record = new Record();
record.setSId((String) rs.getString("sId"));
record.setSName((String) rs.getString("sName"));
record.setProResult((String) rs.getString("proResult"));
record.setProInt((Integer) rs.getInt("proInt"));
all.add(record);
}
pst.close();
}
catch (Exception e)
{
e.printStackTrace();
}
finally
{
jdbc.close();
}
Collections.sort(all);
return all;
}
}

movingbaby
- 粉丝: 27
- 资源: 15
最新资源
- (176497824)Python基于Django+mysql超市进销存销售管理系统设计毕业源码案例设计.zip
- 基于java+springboot+mysql+微信小程序的乡村政务服务系统 源码+数据库+论文(高分毕业设计).zip
- (176954054)基于ssm高校学籍管理系统设计
- 基于java+springboot+mysql+微信小程序的校园保修系统 源码+数据库+论文(高分毕业设计).zip
- (177095634)Microsoft Equation Editor 3.0公式编辑器 安装包
- 基于java+springboot+mysql+微信小程序的微信小程序书店系统 源码+数据库+论文(高分毕业设计).zip
- 最新更新!!!全国各省、市、县城镇化率相关数据2005-2022/2023年
- (177324612)Java课程设计,坦克游戏大战.zip
- 基于java+springboot+mysql+微信小程序的校园资料分享小程序 源码+数据库+论文(高分毕业设计).zip
- (177361822)Java版坦克大战游戏!.zip
- 基于java+springboot+mysql+微信小程序的校园自助打印系统 源码+数据库+论文(高分毕业设计).rar
- 基于java+springboot+mysql+微信小程序的学生管理系统 源码+数据库+论文(高分毕业设计).zip
- (177483040)基于深度学习的车牌识别算法,其中车辆检测网络直接使用YOLO侦测.zip
- 基于java+springboot+mysql+微信小程序的英语互助小程序 源码+数据库+论文(高分毕业设计).zip
- (177506838)华为AP4050DN FAT 固件包
- 基于java+springboot+mysql+微信小程序的研学自习室选座与门禁系统 源码+数据库+论文(高分毕业设计).zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


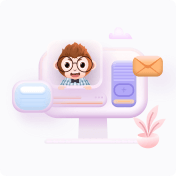