# -*- coding: utf-8 -*-
# Autogenerated by Sphinx on Tue Oct 11 17:41:15 2022
topics = {'assert': 'The "assert" statement\n'
'**********************\n'
'\n'
'Assert statements are a convenient way to insert debugging '
'assertions\n'
'into a program:\n'
'\n'
' assert_stmt ::= "assert" expression ["," expression]\n'
'\n'
'The simple form, "assert expression", is equivalent to\n'
'\n'
' if __debug__:\n'
' if not expression: raise AssertionError\n'
'\n'
'The extended form, "assert expression1, expression2", is '
'equivalent to\n'
'\n'
' if __debug__:\n'
' if not expression1: raise AssertionError(expression2)\n'
'\n'
'These equivalences assume that "__debug__" and "AssertionError" '
'refer\n'
'to the built-in variables with those names. In the current\n'
'implementation, the built-in variable "__debug__" is "True" under\n'
'normal circumstances, "False" when optimization is requested '
'(command\n'
'line option "-O"). The current code generator emits no code for '
'an\n'
'assert statement when optimization is requested at compile time. '
'Note\n'
'that it is unnecessary to include the source code for the '
'expression\n'
'that failed in the error message; it will be displayed as part of '
'the\n'
'stack trace.\n'
'\n'
'Assignments to "__debug__" are illegal. The value for the '
'built-in\n'
'variable is determined when the interpreter starts.\n',
'assignment': 'Assignment statements\n'
'*********************\n'
'\n'
'Assignment statements are used to (re)bind names to values and '
'to\n'
'modify attributes or items of mutable objects:\n'
'\n'
' assignment_stmt ::= (target_list "=")+ (starred_expression '
'| yield_expression)\n'
' target_list ::= target ("," target)* [","]\n'
' target ::= identifier\n'
' | "(" [target_list] ")"\n'
' | "[" [target_list] "]"\n'
' | attributeref\n'
' | subscription\n'
' | slicing\n'
' | "*" target\n'
'\n'
'(See section Primaries for the syntax definitions for '
'*attributeref*,\n'
'*subscription*, and *slicing*.)\n'
'\n'
'An assignment statement evaluates the expression list '
'(remember that\n'
'this can be a single expression or a comma-separated list, the '
'latter\n'
'yielding a tuple) and assigns the single resulting object to '
'each of\n'
'the target lists, from left to right.\n'
'\n'
'Assignment is defined recursively depending on the form of the '
'target\n'
'(list). When a target is part of a mutable object (an '
'attribute\n'
'reference, subscription or slicing), the mutable object must\n'
'ultimately perform the assignment and decide about its '
'validity, and\n'
'may raise an exception if the assignment is unacceptable. The '
'rules\n'
'observed by various types and the exceptions raised are given '
'with the\n'
'definition of the object types (see section The standard type\n'
'hierarchy).\n'
'\n'
'Assignment of an object to a target list, optionally enclosed '
'in\n'
'parentheses or square brackets, is recursively defined as '
'follows.\n'
'\n'
'* If the target list is a single target with no trailing '
'comma,\n'
' optionally in parentheses, the object is assigned to that '
'target.\n'
'\n'
'* Else: The object must be an iterable with the same number of '
'items\n'
' as there are targets in the target list, and the items are '
'assigned,\n'
' from left to right, to the corresponding targets.\n'
'\n'
' * If the target list contains one target prefixed with an '
'asterisk,\n'
' called a “starred” target: The object must be an iterable '
'with at\n'
' least as many items as there are targets in the target '
'list, minus\n'
' one. The first items of the iterable are assigned, from '
'left to\n'
' right, to the targets before the starred target. The '
'final items\n'
' of the iterable are assigned to the targets after the '
'starred\n'
' target. A list of the remaining items in the iterable is '
'then\n'
' assigned to the starred target (the list can be empty).\n'
'\n'
' * Else: The object must be an iterable with the same number '
'of items\n'
' as there are targets in the target list, and the items '
'are\n'
' assigned, from left to right, to the corresponding '
'targets.\n'
'\n'
'Assignment of an object to a single target is recursively '
'defined as\n'
'follows.\n'
'\n'
'* If the target is an identifier (name):\n'
'\n'
' * If the name does not occur in a "global" or "nonlocal" '
'statement\n'
' in the current code block: the name is bound to the object '
'in the\n'
' current local namespace.\n'
'\n'
' * Otherwise: the name is bound to the object in the global '
'namespace\n'
' or the outer namespace determined by "nonlocal", '
'respectively.\n'
'\n'
' The name is rebound if it was already bound. This may cause '
'the\n'
' reference count for the object previously bound to the name '
'to reach\n'
' zero, causing the object to be deallocated and its '
'destructor (if it\n'
' has one) to be called.\n'
'\n'
'* If the target is an attribute reference: The primary '
'expression in\n'
' the reference is evaluated. It should yield an object with\n'
' assignable attributes; if this is not the case, "TypeError" '
'is\n'
' raised. That object is then asked to assign the assigned '
'object to\n'
' the given attribute; if it cannot perform the assignment, it '
'raises\n'
' an exception (usually but not necessarily '
'"AttributeError").\n'
'\n'
' Note: If the object is a class instance and the attribute '
'reference\n'
' occurs on both sides of the assignment operator, the '
'right-hand side\n'
' expression, "a.x" can access either an instance attribute or '
'(if no\n'
' instan
没有合适的资源?快使用搜索试试~ 我知道了~
个人vx机器人集成讯飞、文心一言软件
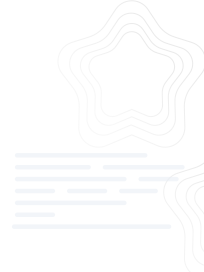
共2000个文件
py:1285个
json:474个
h:184个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 74 浏览量
2024-01-15
17:31:39
上传
评论
收藏 238.86MB ZIP 举报
温馨提示
使用大模型搭建聊天机器人,基于 GPT3.5/GPT4.0/Claude/文心一言/讯飞星火/LinkAI,支持个人微信、公众号、企业微信部署,能处理文本、语音和图片,有丰富的插件体系。
资源推荐
资源详情
资源评论
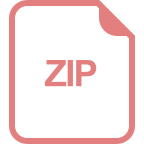
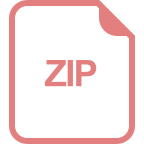
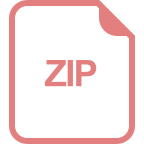
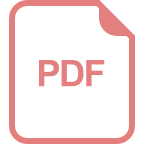
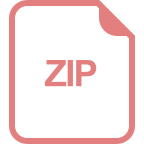
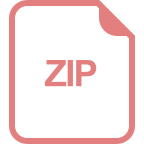
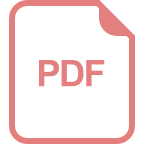
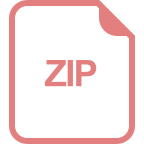
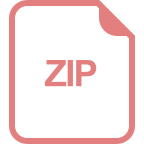
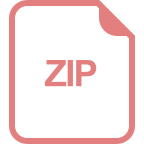
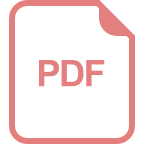
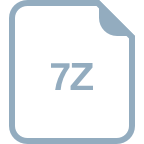
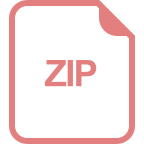
收起资源包目录

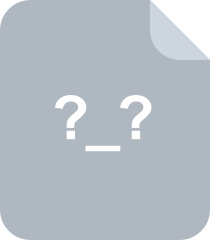
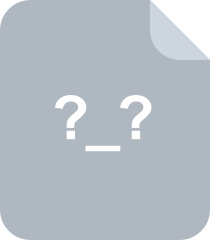
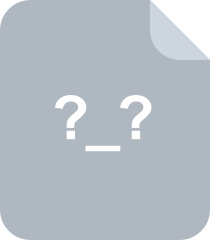
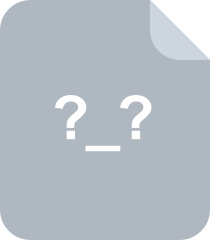
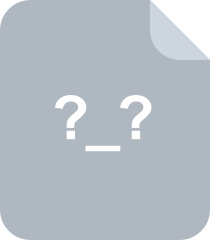
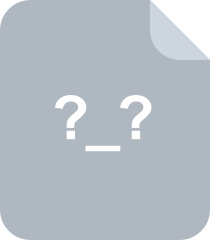
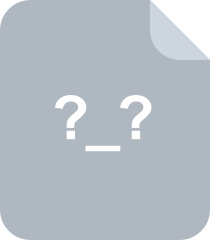
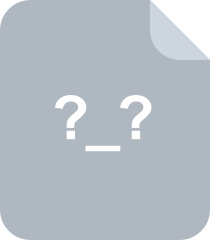
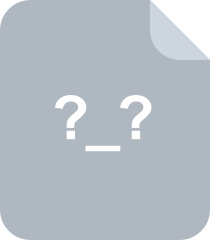
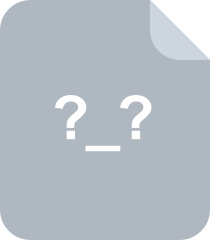
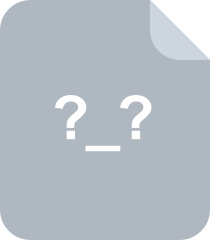
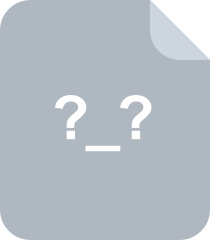
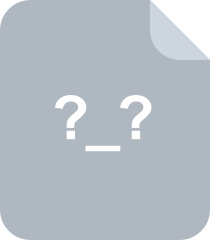
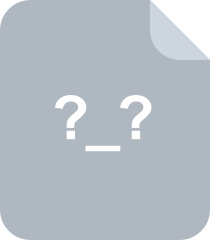
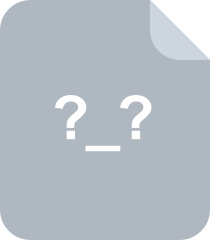
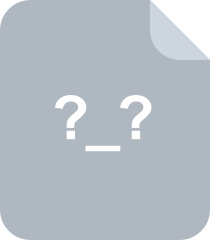
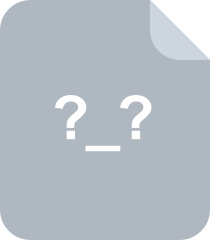
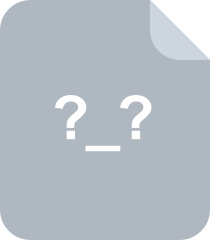
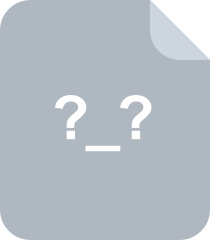
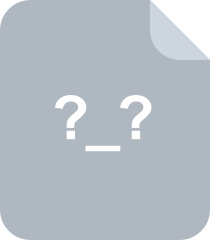
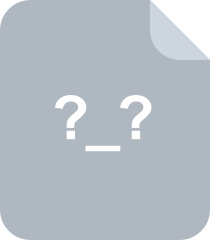
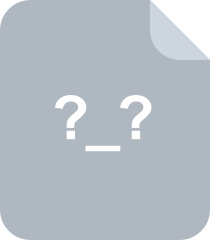
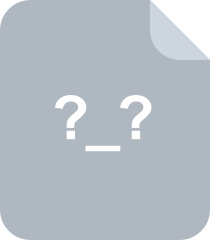
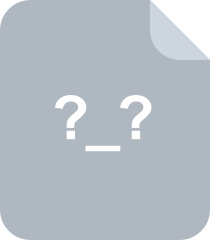
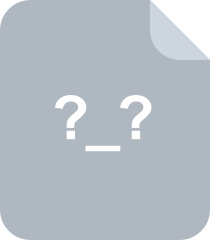
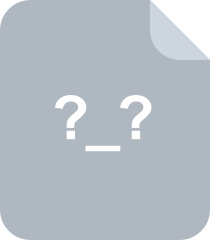
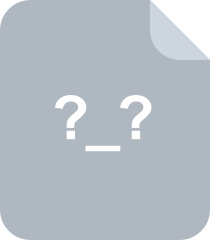
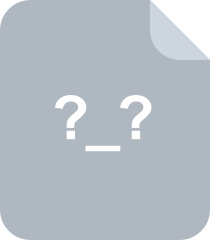
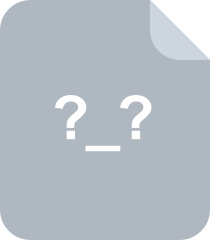
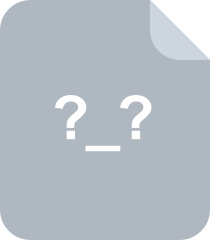
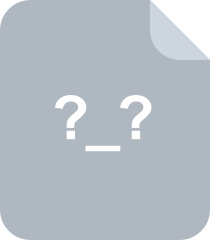
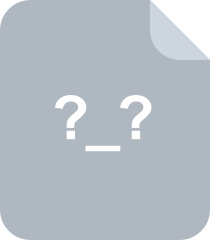
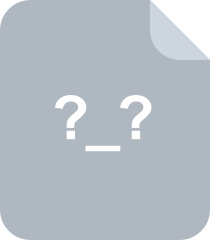
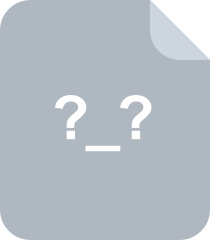
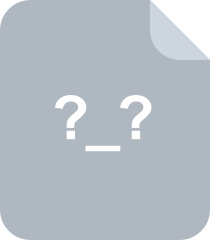
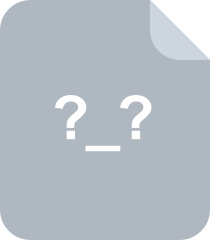
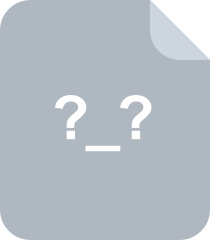
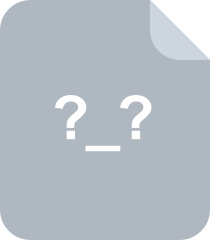
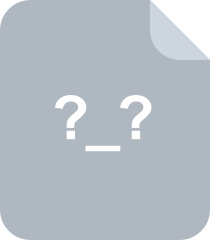
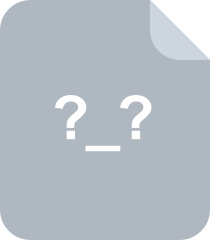
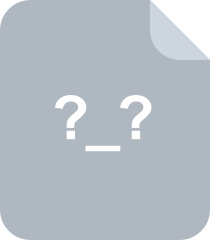
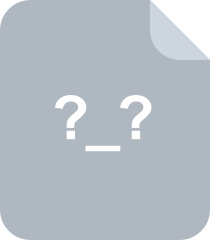
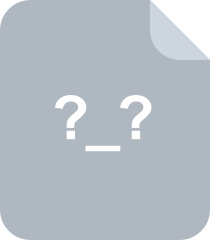
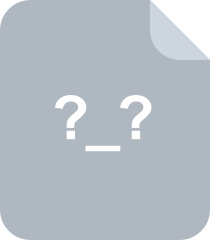
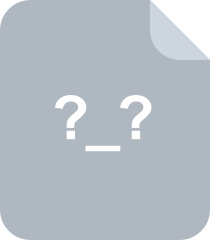
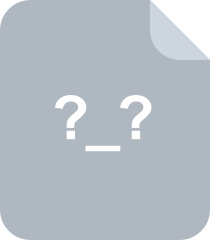
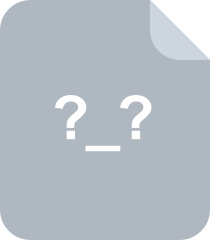
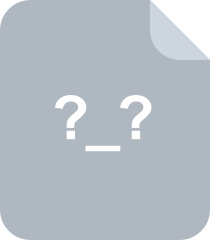
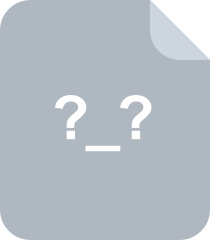
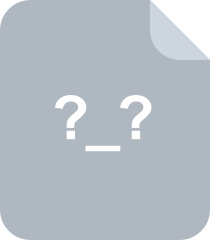
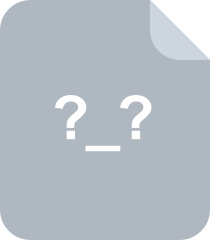
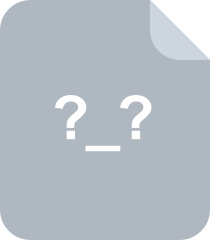
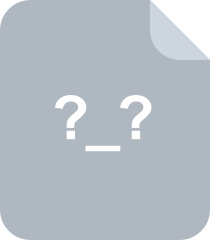
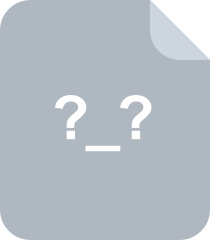
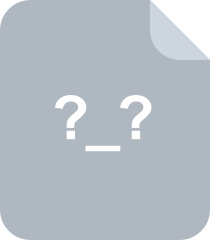
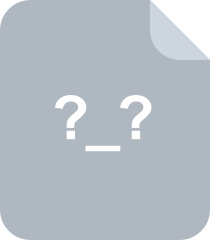
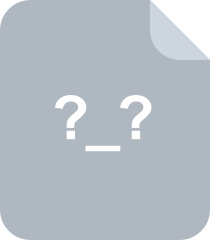
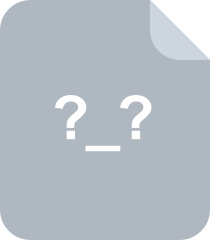
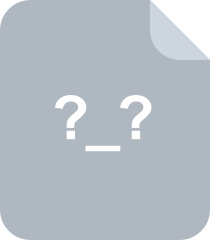
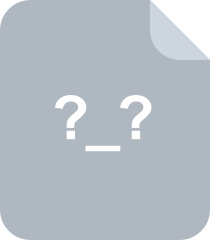
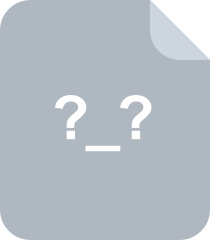
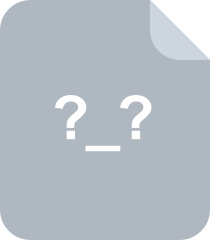
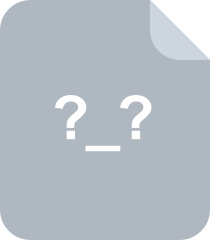
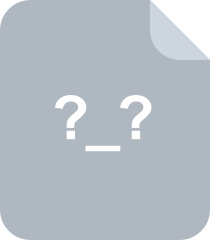
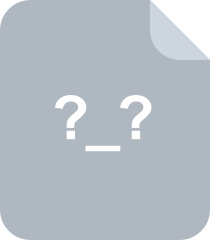
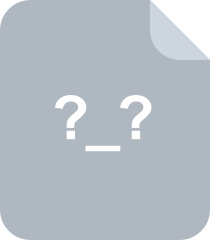
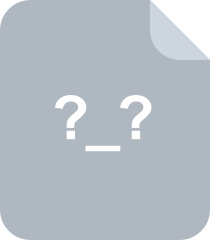
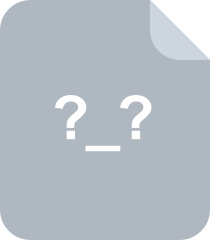
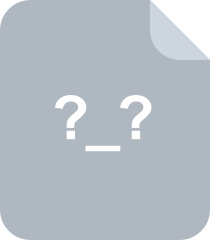
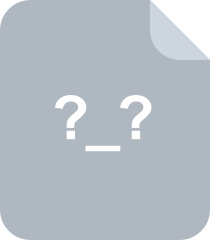
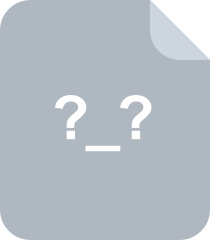
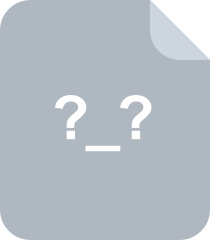
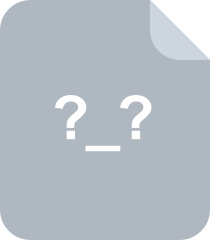
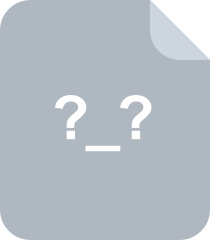
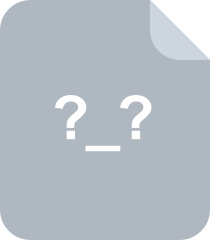
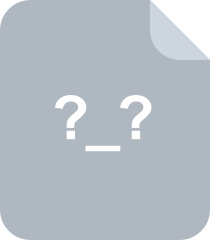
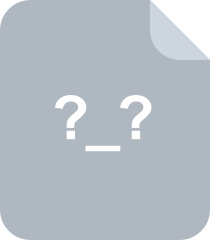
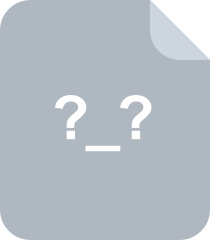
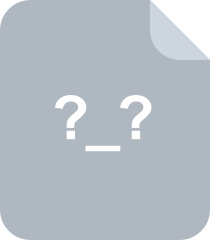
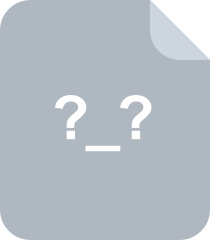
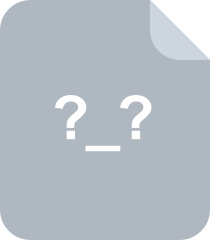
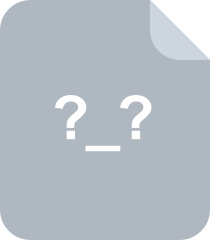
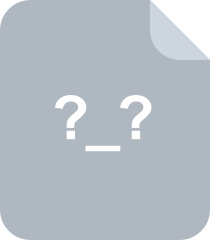
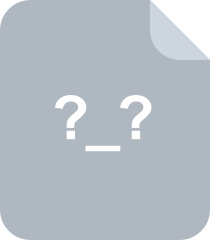
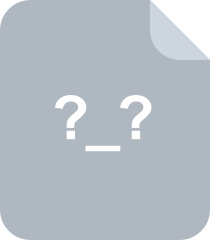
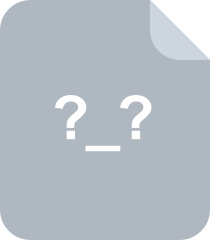
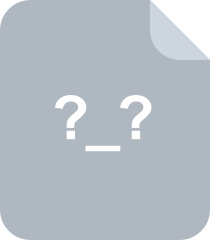
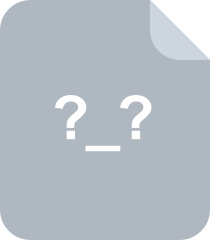
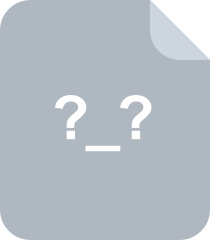
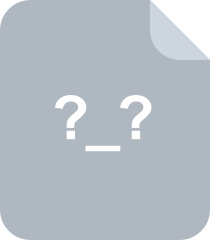
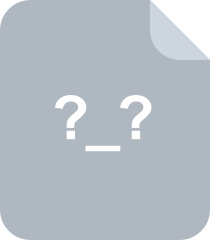
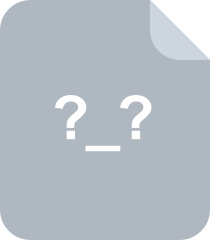
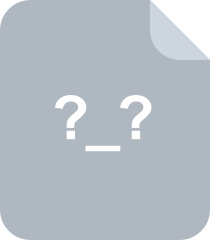
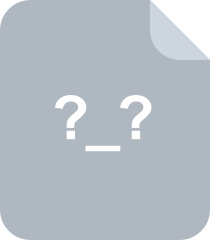
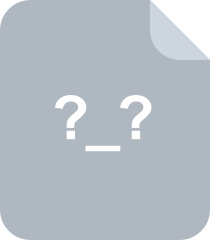
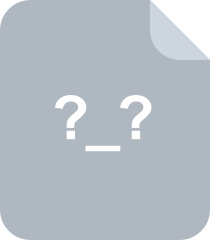
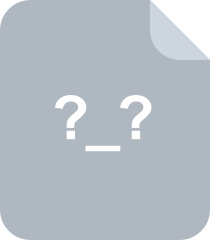
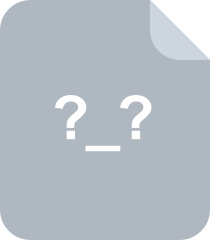
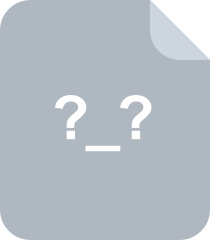
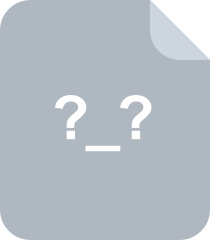
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
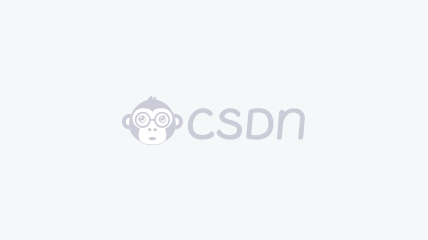

瑆箫
- 粉丝: 835
- 资源: 124
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

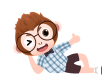
安全验证
文档复制为VIP权益,开通VIP直接复制
