【JavaScript源代码】jquery+springboot实现文件上传功能.docx
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
jquery+springboot实现文件上传功能 本文实例为大家分享了jquery+springboot实现文件上传功能的具体代码,供大家参考,具体内容如下 前端 <!DOCTYPE html> <html lang="zh"> <head> <title></title> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta name="renderer" content="webkit ### 使用jQuery与SpringBoot实现文件上传功能 #### 一、前言 在现代Web开发中,文件上传是一项常见的需求。无论是用户头像上传还是文档管理,都需要开发者掌握如何处理文件上传的技术。本篇文章将详细介绍如何利用jQuery前端库与后端框架SpringBoot来实现文件上传的功能。我们将从HTML表单的基本配置出发,逐步深入到使用SpringBoot处理文件上传的后端逻辑,并探讨不同场景下的文件上传方式。 #### 二、HTML表单设计 我们需要构建一个HTML表单来让用户选择并上传文件。这里我们使用标准的`<form>`标签,并指定`enctype`属性为`multipart/form-data`,这是为了支持文件上传而必须设置的。 ```html <!DOCTYPE html> <html lang="zh"> <head> <title>文件上传示例</title> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta name="renderer" content="webkit"> </head> <body> <!-- 单文件上传 --> <form action="/metadata/metaTables/single-file" method="post" enctype="multipart/form-data"> <input type="file" name="meFile"/> <br> <input type="submit" value="提交"/> <input type="reset" value="清空"/> </form> <!-- 单文件加额外参数 --> <form action="/metadata/metaTables/single-file-param" method="post" enctype="multipart/form-data"> <input type="file" name="meFile"/> <label for="name">Name:</label> <input type="text" name="name" id="name"/> <br> <input type="submit" value="提交"/> <input type="reset" value="清空"/> </form> <!-- 单文件加多个额外参数 --> <form action="/metadata/metaTables/single-file-object" method="post" enctype="multipart/form-data"> <input type="file" name="meFile"/> <label for="aaa">AAA:</label> <input type="text" name="aaa" id="aaa"/> <label for="bbb">BBB:</label> <input type="text" name="bbb" id="bbb"/> <label for="ccc">CCC:</label> <input type="text" name="ccc" id="ccc"/> <br> <input type="submit" value="提交"/> <input type="reset" value="清空"/> </form> <!-- 多文件上传 --> <form action="/metadata/metaTables/many-file" method="post" enctype="multipart/form-data"> <input type="file" name="meFile" multiple="multiple"/> <br> <input type="submit" value="提交"/> <input type="reset" value="清空"/> </form> <!-- 文件夹上传 --> <form action="/metadata/metaTables/dir" method="post" enctype="multipart/form-data"> <input type="file" name="meFile" webkitdirectory directory/> <br> <input type="submit" value="提交"/> <input type="reset" value="清空"/> </form> </body> </html> ``` #### 三、SpringBoot后端处理 接下来,我们来看看SpringBoot后端是如何处理这些请求的。确保在项目中添加了文件上传的相关依赖。 ##### 1. 添加Maven依赖 ```xml <!-- Spring Boot Starter Web --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <!-- Commons FileUpload --> <dependency> <groupId>commons-fileupload</groupId> <artifactId>commons-fileupload</artifactId> <version>1.4</version> </dependency> ``` ##### 2. 配置文件上传 在`application.properties`或`application.yml`中配置文件上传的最大大小等限制。 ```properties spring.http.multipart.max-file-size=10MB spring.http.multipart.max-request-size=50MB ``` ##### 3. 创建控制器处理文件上传 ```java import org.springframework.web.bind.annotation.*; import org.springframework.web.multipart.MultipartFile; import javax.servlet.http.HttpServletRequest; import java.io.File; import java.io.IOException; @RestController @RequestMapping("/metadata/metaTables") public class FileUploadController { @PostMapping("/single-file") public String handleSingleFileUpload(@RequestParam("meFile") MultipartFile file) { // 保存文件逻辑 try { file.transferTo(new File("/path/to/save/" + file.getOriginalFilename())); } catch (IOException e) { e.printStackTrace(); return "Error occurred while uploading file."; } return "File uploaded successfully."; } @PostMapping("/single-file-param") public String handleSingleFileUploadWithParams( @RequestParam("meFile") MultipartFile file, @RequestParam("name") String name) { // 保存文件逻辑 try { file.transferTo(new File("/path/to/save/" + file.getOriginalFilename())); } catch (IOException e) { e.printStackTrace(); return "Error occurred while uploading file."; } return "File and parameter received: " + name; } @PostMapping("/single-file-object") public String handleSingleFileUploadWithObject( @RequestParam("meFile") MultipartFile file, @RequestParam("aaa") String aaa, @RequestParam("bbb") String bbb, @RequestParam("ccc") String ccc) { // 保存文件逻辑 try { file.transferTo(new File("/path/to/save/" + file.getOriginalFilename())); } catch (IOException e) { e.printStackTrace(); return "Error occurred while uploading file."; } return "File and parameters received: " + aaa + ", " + bbb + ", " + ccc; } @PostMapping("/many-file") public String handleManyFilesUpload(@RequestParam("meFile") MultipartFile[] files) { for (MultipartFile file : files) { try { file.transferTo(new File("/path/to/save/" + file.getOriginalFilename())); } catch (IOException e) { e.printStackTrace(); return "Error occurred while uploading file."; } } return "Multiple files uploaded successfully."; } @PostMapping("/dir") public String handleDirectoryUpload(@RequestParam("meFile") MultipartFile file, HttpServletRequest request) { // 这里需要处理目录上传逻辑 return "Directory upload not implemented yet."; } } ``` #### 四、总结 通过以上步骤,我们可以使用jQuery前端库与SpringBoot后端框架实现文件上传的功能。本文详细介绍了HTML表单的设计、SpringBoot后端处理逻辑,并给出了具体的代码实现。希望本文能够帮助你在实际开发中解决文件上传的问题。
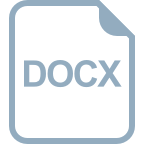
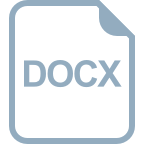
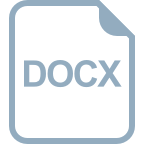
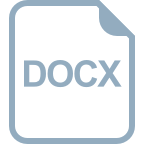
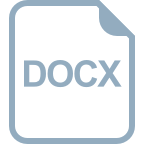
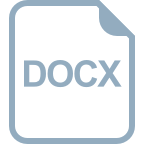
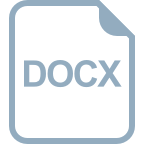
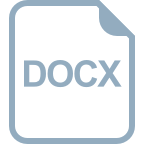
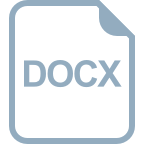
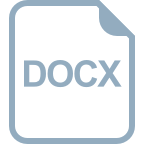
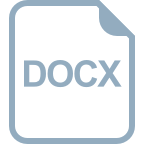
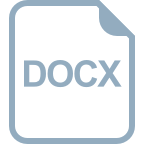
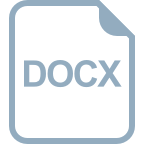
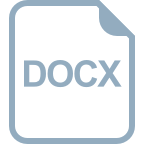
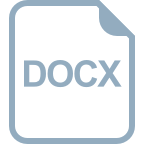
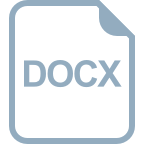
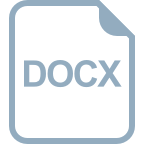
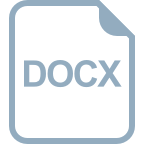
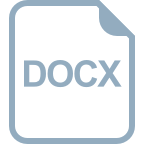
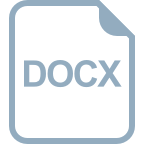
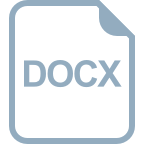
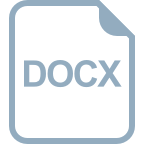
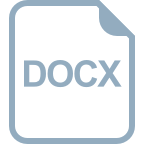
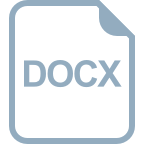
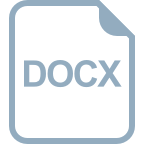
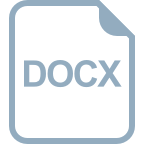
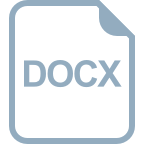
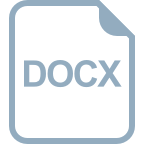
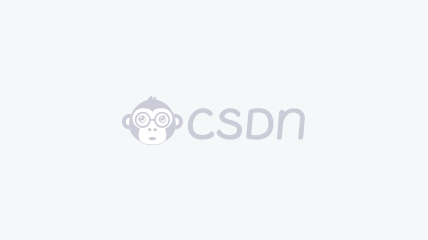

- 粉丝: 6241
- 资源: 1万+
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

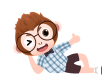
最新资源

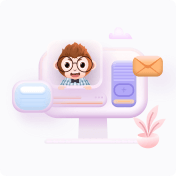
