// Borland C++ Builder
// Copyright (c) 1995, 1999 by Borland International
// All rights reserved
// (DO NOT EDIT: machine generated header) 'FrmGridSet.pas' rev: 5.00
#ifndef FrmGridSetHPP
#define FrmGridSetHPP
#pragma delphiheader begin
#pragma option push -w-
#pragma option push -Vx
#include <DBGridEh.hpp> // Pascal unit
#include <GridsEh.hpp> // Pascal unit
#include <RzCmboBx.hpp> // Pascal unit
#include <ComCtrls.hpp> // Pascal unit
#include <CheckLst.hpp> // Pascal unit
#include <ExtCtrls.hpp> // Pascal unit
#include <Buttons.hpp> // Pascal unit
#include <StdCtrls.hpp> // Pascal unit
#include <Dialogs.hpp> // Pascal unit
#include <Forms.hpp> // Pascal unit
#include <Controls.hpp> // Pascal unit
#include <Graphics.hpp> // Pascal unit
#include <Classes.hpp> // Pascal unit
#include <SysUtils.hpp> // Pascal unit
#include <Messages.hpp> // Pascal unit
#include <Windows.hpp> // Pascal unit
#include <SysInit.hpp> // Pascal unit
#include <System.hpp> // Pascal unit
//-- user supplied -----------------------------------------------------------
namespace Frmgridset
{
//-- type declarations -------------------------------------------------------
typedef DynamicArray<Dbgrideh::TColumnEh* > FrmGridSet__1;
struct TGrids
{
int ColCount;
bool AutoFitColWidths;
bool Ctl3D;
bool MultiTitle;
int SumList;
int FrozenCols;
Graphics::TFont* NRFont;
Graphics::TFont* TitleFont;
Graphics::TFont* FooterFont;
Graphics::TColor NRColor;
Graphics::TColor TitleColor;
Graphics::TColor FooterColor;
Graphics::TColor OddRowColor;
Graphics::TColor EvenRowColor;
DynamicArray<Dbgrideh::TColumnEh* > Items;
} ;
class DELPHICLASS TFrm_GridSet;
class PASCALIMPLEMENTATION TFrm_GridSet : public Forms::TForm
{
typedef Forms::TForm inherited;
__published:
Extctrls::TPanel* Panel1;
Buttons::TBitBtn* btn_Ok;
Buttons::TBitBtn* btn_CCL;
Extctrls::TPanel* Panel2;
Stdctrls::TGroupBox* GroupBox1;
Stdctrls::TGroupBox* GroupBox4;
Checklst::TCheckListBox* CLB;
Stdctrls::TLabel* Label1;
Buttons::TSpeedButton* sb_zd;
Buttons::TSpeedButton* sb_sy;
Buttons::TSpeedButton* sb_xy;
Buttons::TSpeedButton* sb_zy;
Buttons::TSpeedButton* btn_fx;
Buttons::TSpeedButton* sb_qx;
Stdctrls::TCheckBox* ChkSort;
Stdctrls::TCheckBox* ChkReadOnly;
Extctrls::TRadioGroup* rg1;
Comctrls::TUpDown* ud1;
Stdctrls::TGroupBox* GroupBox2;
Stdctrls::TLabel* Label2;
Stdctrls::TCheckBox* ChkSumList;
Stdctrls::TCheckBox* chkAutoColWidth;
Stdctrls::TCheckBox* ChkMultiTitle;
Stdctrls::TCheckBox* chkAutoListWidth;
Stdctrls::TCheckBox* chkCtl3D;
Stdctrls::TEdit* edtFix;
Comctrls::TUpDown* udFix;
Dialogs::TFontDialog* dlgFont1;
Stdctrls::TButton* btn_sj;
Stdctrls::TButton* btn_bt;
Stdctrls::TButton* btn_jz;
Stdctrls::TButton* btn_jzbjs;
Stdctrls::TButton* btn_sjbjs;
Stdctrls::TButton* btn_btbjs;
Dialogs::TColorDialog* dlgColor1;
Buttons::TBitBtn* btn_EvenRowColor;
Stdctrls::TLabel* Label3;
Buttons::TSpeedButton* btn_OddRowColor;
Stdctrls::TEdit* edtEdtFieldTitle;
Stdctrls::TLabel* Label4;
Stdctrls::TEdit* lbledt1;
Stdctrls::TLabel* Label5;
void __fastcall sb_zdClick(System::TObject* Sender);
void __fastcall sb_syClick(System::TObject* Sender);
void __fastcall sb_xyClick(System::TObject* Sender);
void __fastcall sb_zyClick(System::TObject* Sender);
void __fastcall sb_qxClick(System::TObject* Sender);
void __fastcall btn_fxClick(System::TObject* Sender);
void __fastcall lbledt1KeyPress(System::TObject* Sender, char &Key);
void __fastcall FormShow(System::TObject* Sender);
void __fastcall CLBClickCheck(System::TObject* Sender);
void __fastcall CLBClick(System::TObject* Sender);
void __fastcall lbledtEdtFieldTitleChange(System::TObject* Sender);
void __fastcall lbledt1Change(System::TObject* Sender);
void __fastcall chkAutoListWidthClick(System::TObject* Sender);
void __fastcall chkAutoColWidthClick(System::TObject* Sender);
void __fastcall chkCtl3DClick(System::TObject* Sender);
void __fastcall ChkMultiTitleClick(System::TObject* Sender);
void __fastcall ChkSumListClick(System::TObject* Sender);
void __fastcall edtFixChange(System::TObject* Sender);
void __fastcall ChkReadOnlyClick(System::TObject* Sender);
void __fastcall ChkSortClick(System::TObject* Sender);
void __fastcall rg1Click(System::TObject* Sender);
void __fastcall btn_OkClick(System::TObject* Sender);
void __fastcall FormClose(System::TObject* Sender, Forms::TCloseAction &Action);
void __fastcall btn_sjClick(System::TObject* Sender);
void __fastcall btn_CCLClick(System::TObject* Sender);
void __fastcall btn_btClick(System::TObject* Sender);
void __fastcall btn_jzClick(System::TObject* Sender);
void __fastcall btn_btbjsClick(System::TObject* Sender);
void __fastcall btn_sjbjsClick(System::TObject* Sender);
void __fastcall btn_jzbjsClick(System::TObject* Sender);
void __fastcall btn_EvenRowColorClick(System::TObject* Sender);
void __fastcall btn_OddRowColorClick(System::TObject* Sender);
void __fastcall FormCreate(System::TObject* Sender);
private:
TGrids HYGrid;
int SelIndex;
bool ShowFlag;
bool CloseFlag;
bool ReadSet;
void __fastcall CloseInit(void);
public:
Dbgrideh::TDBGridEh* grid;
__fastcall TFrm_GridSet(System::TObject* Sender, Dbgrideh::TDBGridEh* Grids, bool ReadSet);
public:
#pragma option push -w-inl
/* TCustomForm.CreateNew */ inline __fastcall virtual TFrm_GridSet(Classes::TComponent* AOwner, int
Dummy) : Forms::TForm(AOwner, Dummy) { }
#pragma option pop
#pragma option push -w-inl
/* TCustomForm.Destroy */ inline __fastcall virtual ~TFrm_GridSet(void) { }
#pragma option pop
public:
#pragma option push -w-inl
/* TWinControl.CreateParented */ inline __fastcall TFrm_GridSet(HWND ParentWindow) : Forms::TForm(ParentWindow
) { }
#pragma option pop
};
//-- var, const, procedure ---------------------------------------------------
extern PACKAGE TFrm_GridSet* Frm_GridSet;
} /* namespace Frmgridset */
#if !defined(NO_IMPLICIT_NAMESPACE_USE)
using namespace Frmgridset;
#endif
#pragma option pop // -w-
#pragma option pop // -Vx
#pragma delphiheader end.
//-- end unit ----------------------------------------------------------------
#endif // FrmGridSet
没有合适的资源?快使用搜索试试~ 我知道了~
delphi中checklistbox构造sql语句中in实例
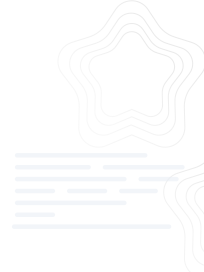
共74个文件
dcu:23个
dfm:5个
pas:5个

需积分: 16 13 下载量 60 浏览量
2014-11-01
11:16:30
上传
评论 1
收藏 7.48MB ZIP 举报
温馨提示
在delphi查询功能设计过程中,对于sql语句中的in的构造,网上没有现成的示例,就自己写了一个简单的用checklistbox来生成in()括号里的记录,最终构造的SQL为 :select * from 表1 where name in (aaaa,cccc); 这个简单功能的难点在于逗号的生成; 第一次上传,如果有更好的实现方法,请勿见笑。
资源推荐
资源详情
资源评论
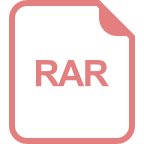
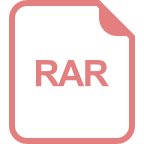
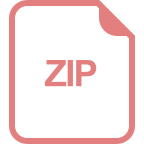
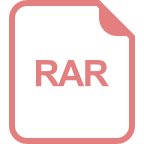
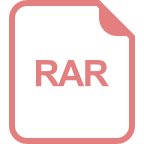
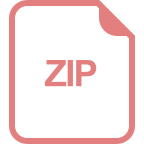
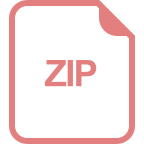
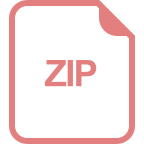
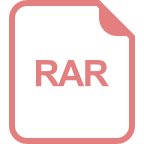
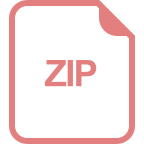
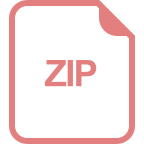
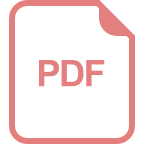
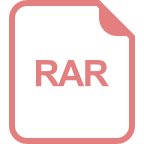
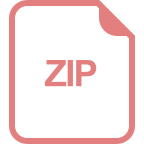
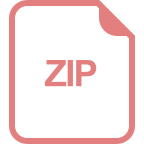
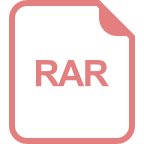
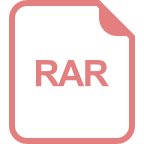
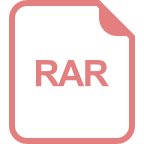
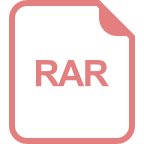
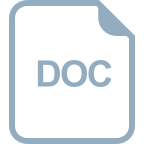
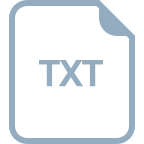
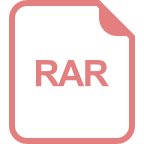
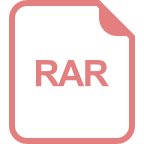
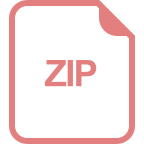
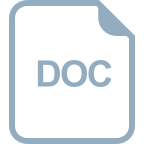
收起资源包目录



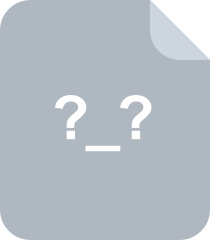
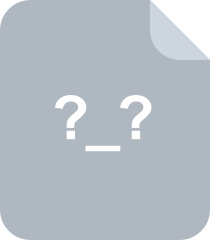
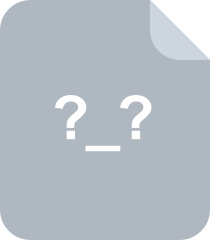
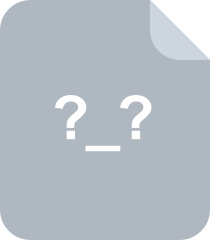
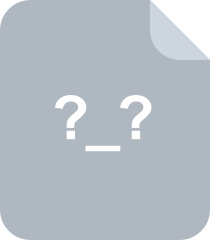
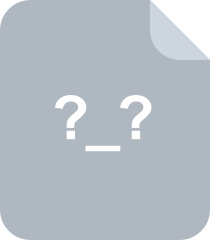
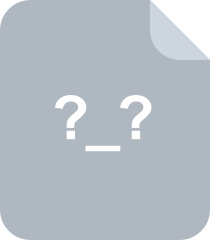
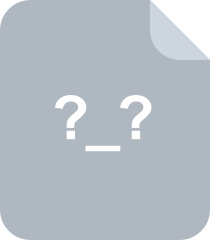
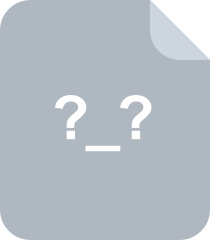
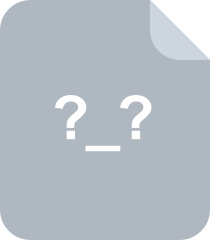
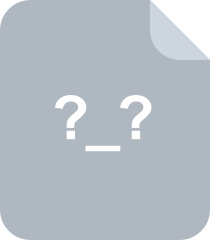
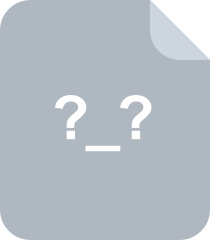
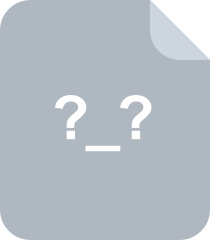
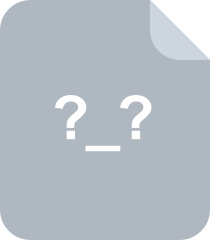
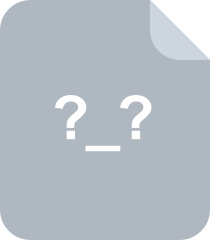
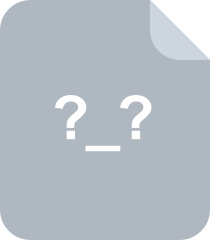
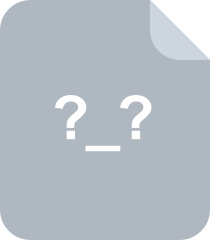
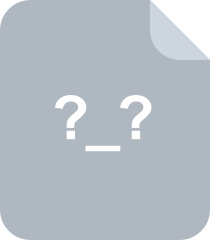
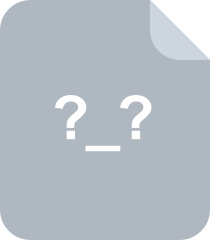
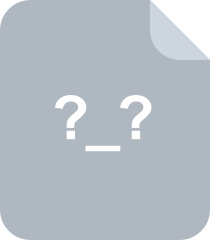
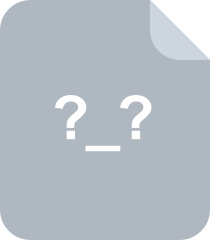
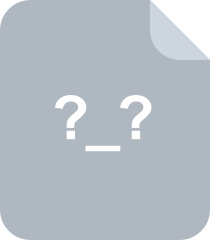
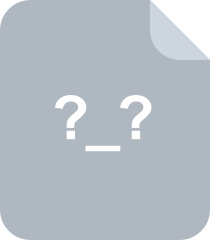
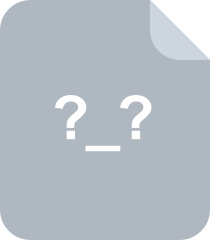
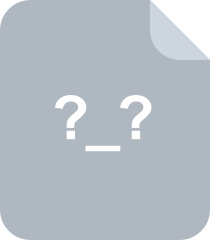
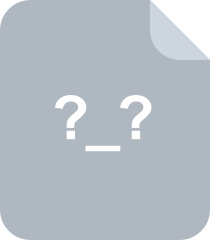
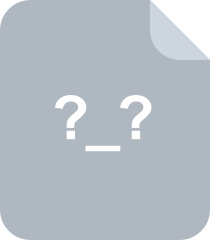
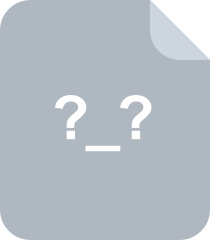
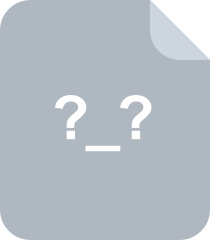
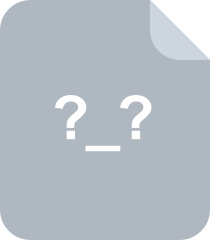
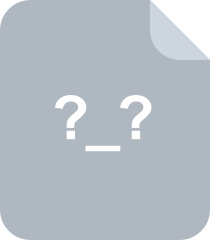
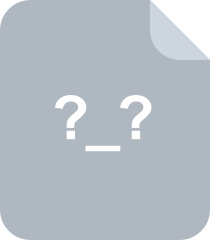
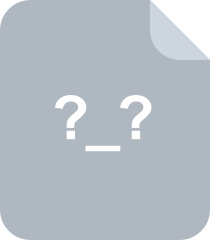
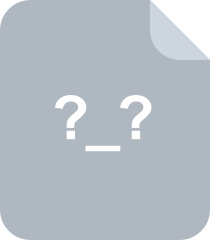
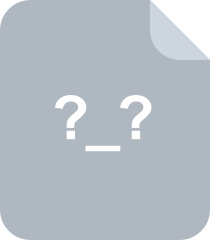


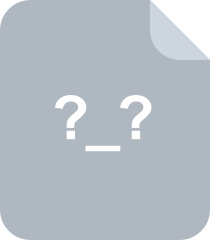
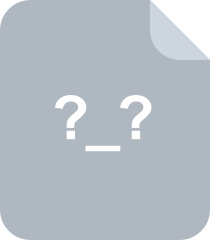
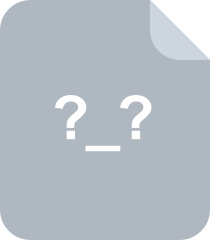
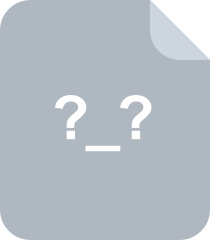
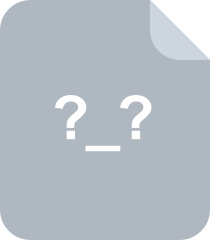
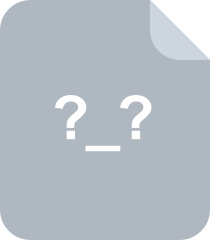
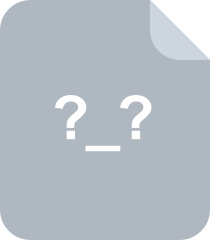
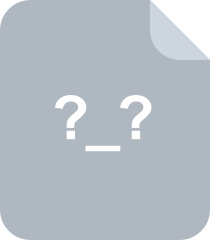
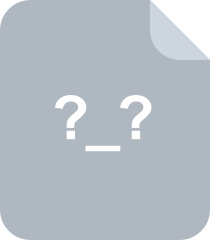
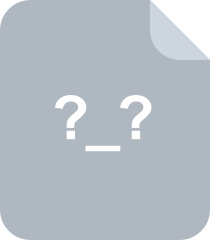
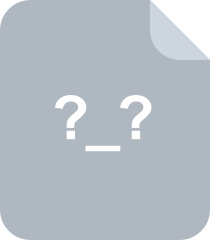
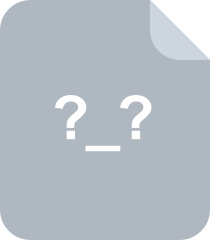
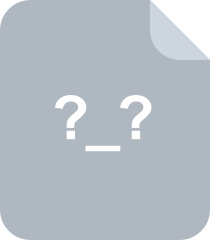
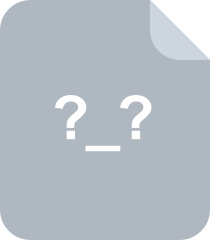
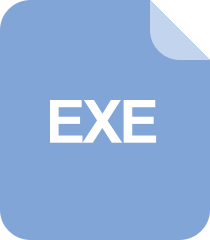
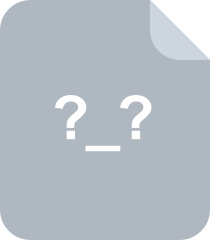
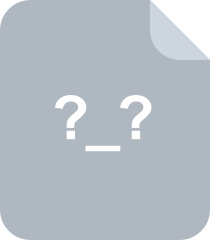
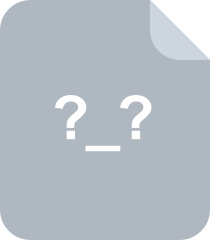
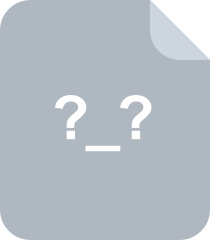
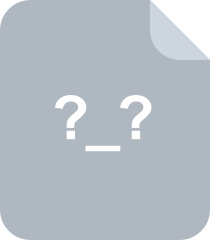
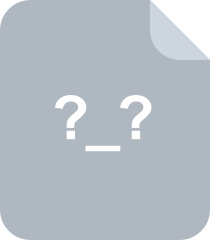
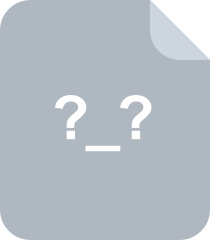
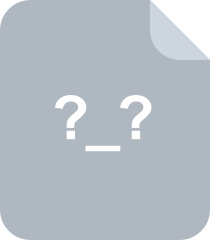
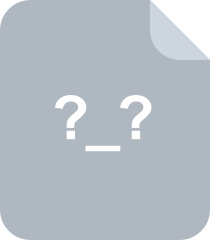
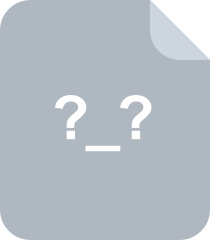
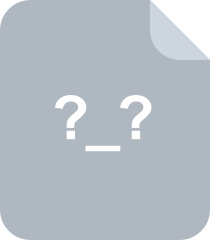
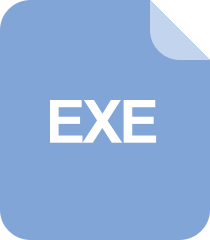
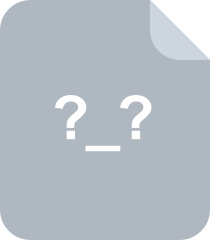
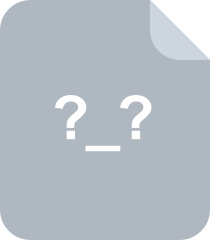
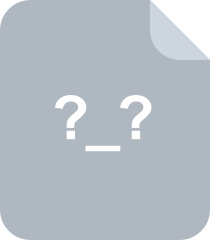
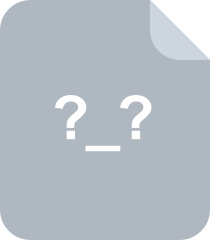
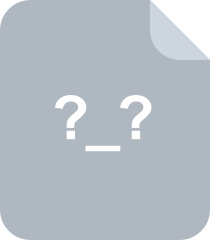
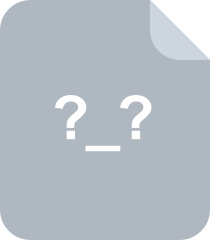
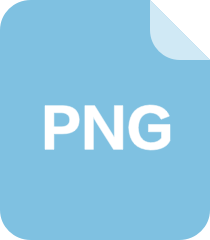
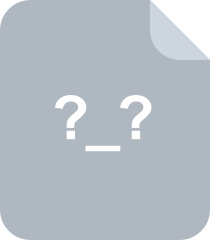
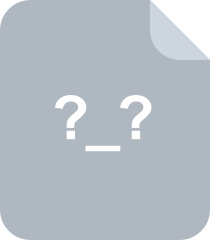
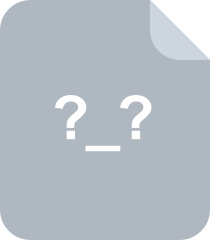
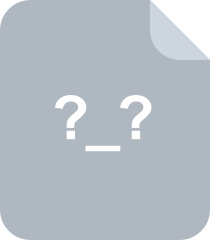
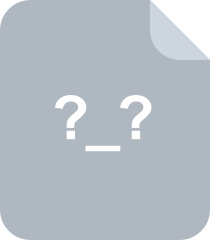
共 74 条
- 1
资源评论
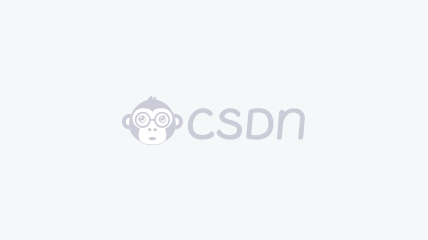

maxthonghh
- 粉丝: 0
- 资源: 4
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

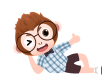
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


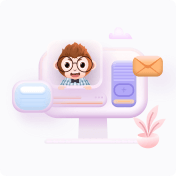
安全验证
文档复制为VIP权益,开通VIP直接复制
