/***************************************************************************
kdmonfuns.cpp - description
-------------------
begin : Thu Nov 14 2002
copyright : (C) 2002 by sys_devp
email : sys_devp@sina.com
***************************************************************************/
/***************************************************************************
* *
* This program is free software; you can redistribute it and/or modify *
* it under the terms of the GNU General Public License as published by *
* the Free Software Foundation; either version 2 of the License, or *
* (at your option) any later version. *
* *
***************************************************************************/
#include "kdmonfuns.h"
KdmonFuns::KdmonFuns(){
}
KdmonFuns::~KdmonFuns(){
}
/** No descriptions */
int KdmonFuns::KdWGetStr(WINDOW *win, char *buffer, const int &n)
{
int x;
int y;
int ch;
int startx;
int buffLength;
char spaces[n+1];
memset (spaces, ' ', sizeof (spaces));
getyx(win, y, x);
x ++;
y ++;
startx = x;
if (buffer != NULL)
{
addstr (buffer);
x += strlen (buffer);
if ( (int)strlen (buffer) == n)
x --;
}
move (y, x);
do {
ch = getch ( );
switch (ch)
{
case '\r': /* key <ENTER>, return */
return 2;
case '\t': /* key <TAB>, disabled */
break;
case 27: /* key <ESC>, return */
return 3;
case KEY_UP: /* key <UP>, return */
return 0;
case KEY_HOME: /* key <HOME>, go to the line head */
x = startx;
break;
case KEY_END: /* key <END>, go to the line end */
buffLength = (int)strlen (buffer);
x = startx + buffLength;
if ( buffLength == n )
--x;
break;
case KEY_DOWN: /* key <DOWN>, return */
return 1;
case KEY_RIGHT: /* key <RIGHT>, move right */
buffLength = (int)strlen (buffer);
if ( (x < (startx + (int)strlen (buffer))) && (x < (startx + n - 1)) )
++ x;
break;
case KEY_LEFT: /* key <LEFT>, move left */
if (x > (startx))
-- x;
break;
case KEY_DC:
buffLength = (int)strlen (buffer);
if (x != (startx + (int)strlen (buffer)))
{
memmove (buffer + x - startx, buffer + x - startx + 1 , buffLength + startx - x - 1);
buffer[buffLength - 1] = '\0';
mvaddstr (y, startx, buffer);
mvaddnstr (y, startx + (int)strlen (buffer), spaces, n - (int)strlen (buffer));
}
break;
case KEY_BACKSPACE: /* key <BACKSPACE>, delete a character */
buffLength = (int)strlen (buffer);
if ( x != startx)
{
memmove (buffer + x - startx - 1, buffer + x - startx, buffLength + startx - x);
buffer[buffLength - 1] = '\0';
-- x;
mvaddstr (y, startx, buffer);
mvaddnstr (y, startx + (int)strlen (buffer), spaces, n - (int)strlen (buffer));
}
break;
default: /* almost receive any other key, and display on screen */
buffer[x - startx] = ch;
if (x < startx + n - 1)
++ x;
mvaddstr (y, startx, buffer);
break;
}
move (y, x);
} while (1);
}
/** ******************************************
return value:
failure ---------- RET_FAILURE
ok ---------- RET_SUCCESS
******************************************* */
int KdmonFuns::msgWin(const int &y,const int &x,const char * msg)
{
int ncols=40;
int nlines;
int beginX;
int beginY;
int i;
char inputCh;
char pressMes[]="Press Key SPACE For Close This Window";
WINDOW *win;
nlines=((int)strlen(msg)/ncols)+5;
if((int)strlen(msg)%ncols){
nlines++;
}
win=newwin(nlines,ncols+4,y,x);
box(win,0,0);
beginX=2;
beginY=2;
for(i=0;i<(nlines-6);i++)
{
mvwaddnstr(win,beginY,beginX,msg,ncols);
msg+=ncols;
beginY++;
}
if(*msg!='\0')
{
mvwaddstr(win,beginY,beginX,msg);
beginY++;
}
beginY++;
beginX=2;
mvwaddstr(win,beginY,beginX,pressMes);
touchwin(win);
wrefresh(win);
topWindowList.addWin(win);
while((inputCh=wgetch(win)))
{
if(inputCh==' ')
break;
}
topWindowList.delWin();
wclear(win);
wrefresh(win);
delwin(win);
return RET_SUCCESS;
}
/** show window where require confirm
return value:
failure ---------- RET_FAILURE
ok ---------- RET_OK
cancel ---------- RET_CANCEL */
int KdmonFuns::confirmWin(const int& y,const int&x,const char * msg)
{
int ncols=40;
int nlines;
int beginX;
int beginY;
int i;
int ret;
int act;
int returnValue=0;
int subWinRow;
int subWinCol;
char key;
WINDOW *win;
WINDOW *subWin;
nlines=((int)strlen(msg)/ncols)+5;
if((int)strlen(msg)%ncols){
nlines++;
}
win=newwin(nlines,ncols+4,y,x);
box(win,0,0);
beginX=2;
beginY=2;
for(i=0;i<(nlines-5);i++)
{
mvwaddnstr(win,beginY,beginX,msg,ncols);
msg+=ncols;
beginY++;
}
beginY++;
ITEM *itemTab[3]={new_item("OK",""),new_item("CANCEL",""),NULL};
MENU *menu;
menu=new_menu(itemTab);
menu_opts_on(menu,O_ROWMAJOR);
scale_menu(menu,&subWinRow,&subWinCol);
subWin=subwin(win,1,subWinRow*(subWinCol+1),beginY+y,beginX+x+10);
set_menu_format(menu,1,subWinRow*(subWinCol+1));
set_menu_win(menu,win);
set_menu_sub(menu,subWin);
set_menu_grey(menu,A_PROTECT);
// touchwin(win);
// wrefresh(win);
touchwin(win);
wrefresh(win);
topWindowList.addWin(win);
touchwin(subWin);
wrefresh(subWin);
post_menu(menu);
while((key=wgetch(subWin)))
{
if(key==13)
break;
ret=0;
switch(key)
{
case 'C':
returnValue++;
act=REQ_RIGHT_ITEM;
ret=menu_driver(menu,REQ_RIGHT_ITEM);
break;
case 'D':
returnValue++;
act=REQ_LEFT_ITEM;
ret=menu_driver(menu,REQ_LEFT_ITEM);
break;
default:
break;
}
if(ret==E_REQUEST_DENIED)
{
switch(act)
{
case REQ_LEFT_ITEM:
menu_driver(menu,REQ_LAST_ITEM);
break;
case REQ_RIGHT_ITEM:
menu_driver(menu,REQ_FIRST_ITEM);
break;
default:
break;
}
}
}
unpost_menu(menu);
free_menu(menu);
fre
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
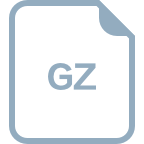
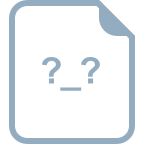
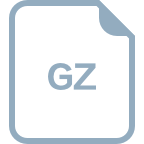
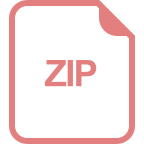
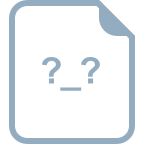
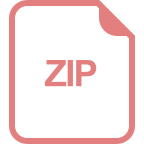
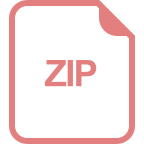
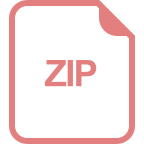
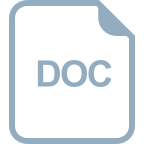
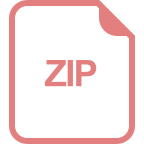
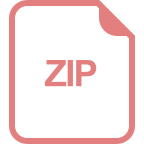
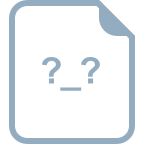
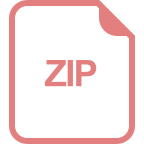
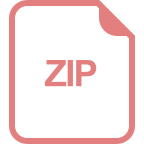
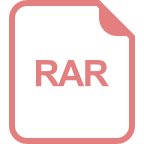
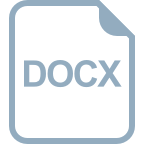
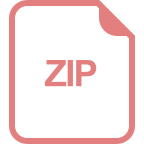
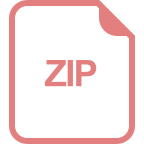
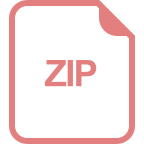
收起资源包目录


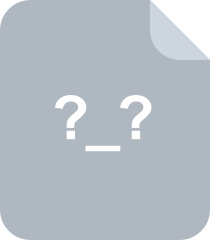
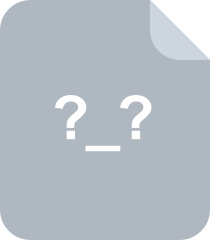
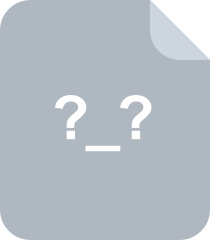
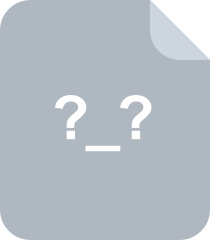
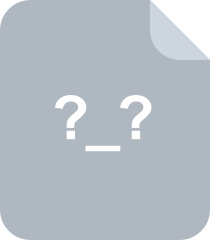
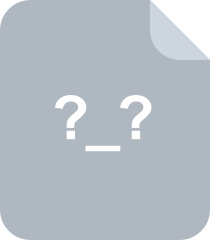
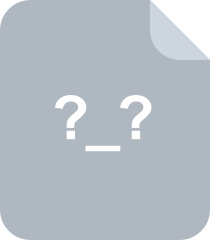
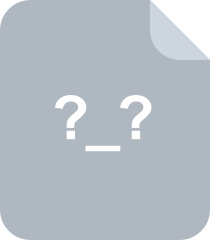
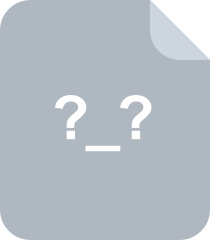
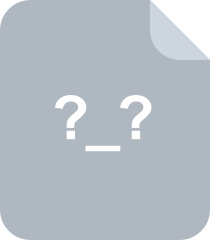
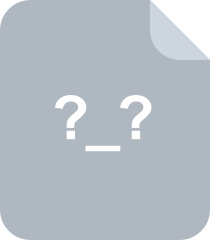
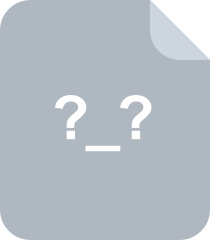
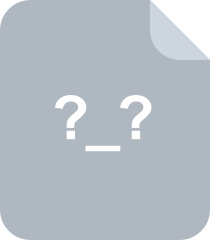
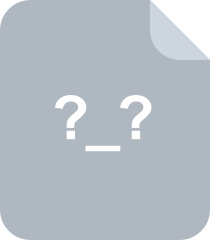
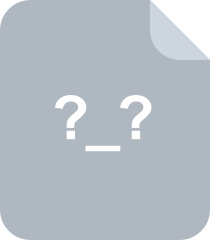
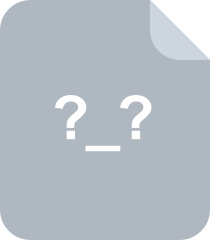
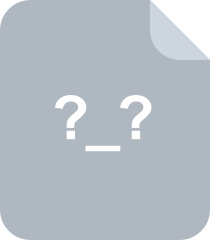
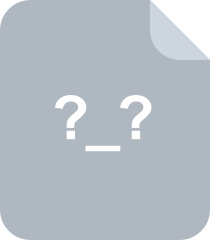

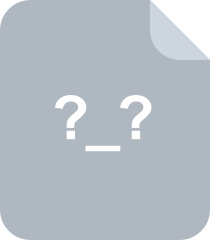
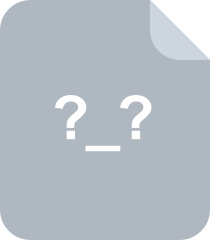
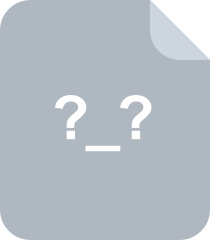
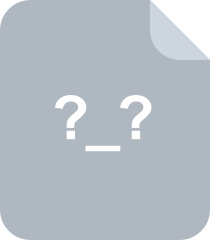
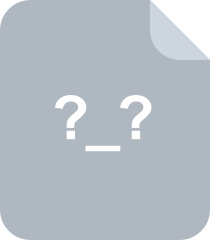
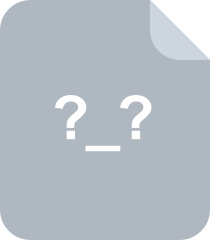
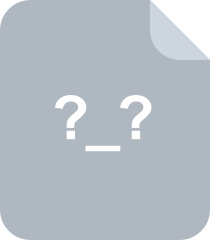

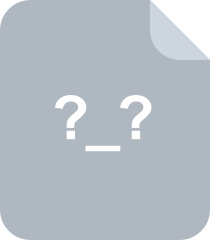
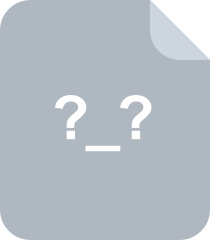
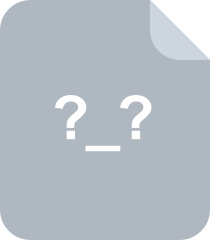

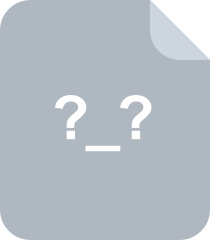
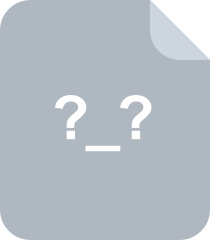
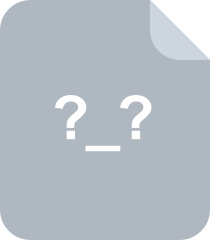
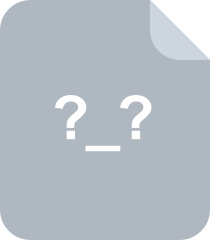


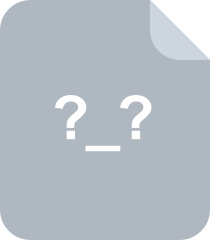
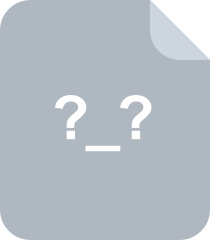
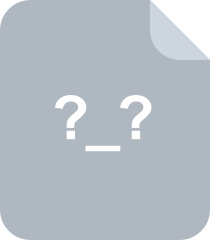
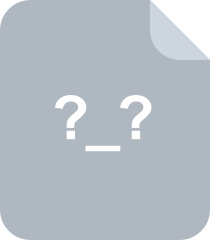
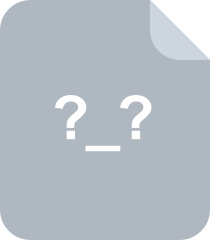
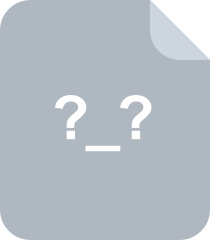
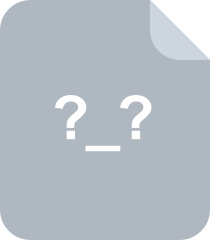
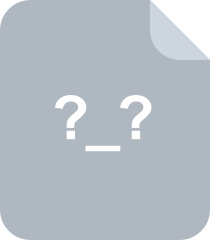
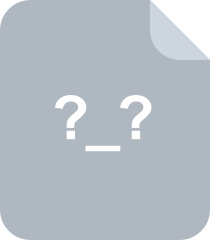
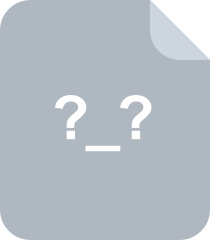

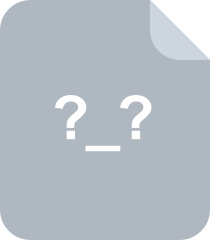
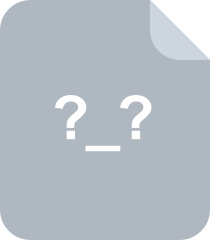
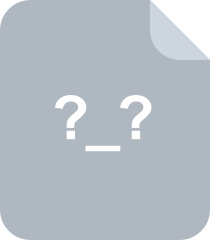
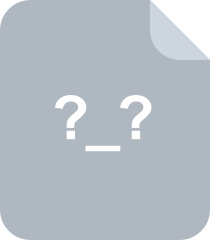
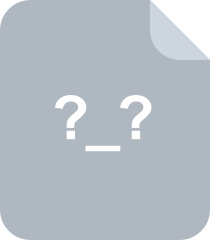
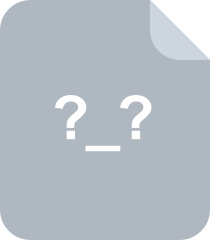
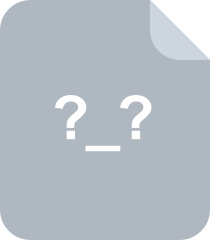
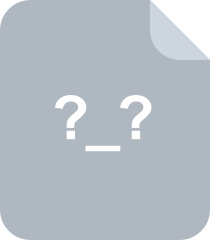
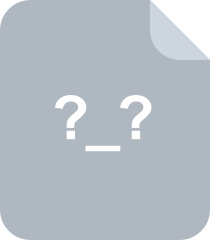
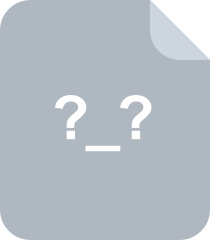
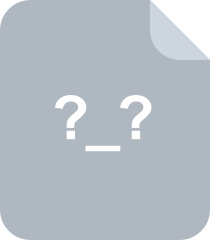
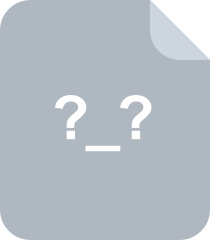
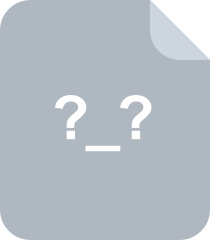
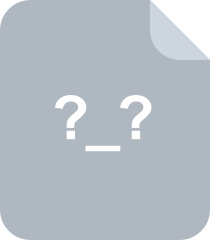
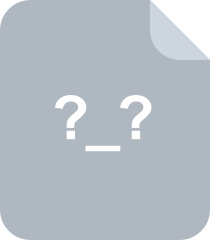
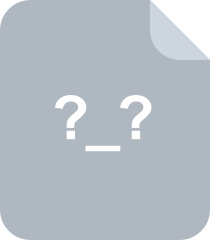
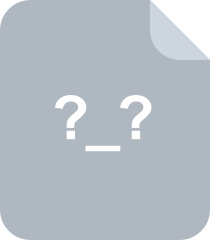
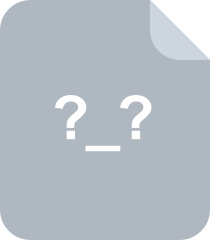
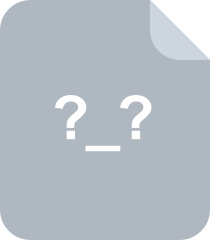
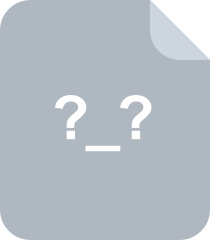
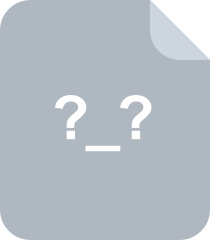
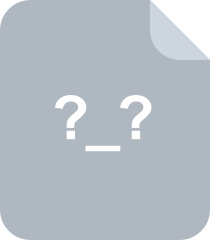
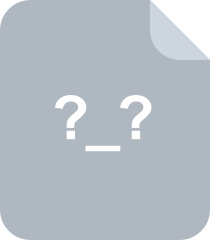
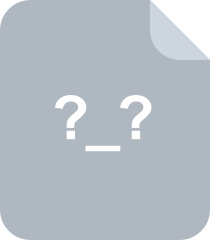
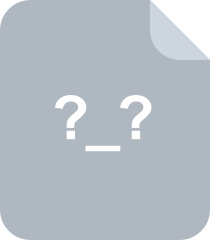
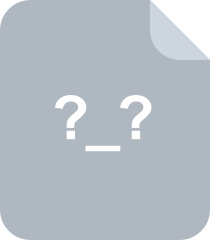
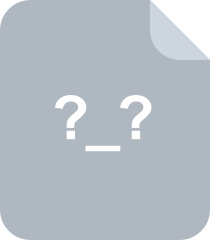
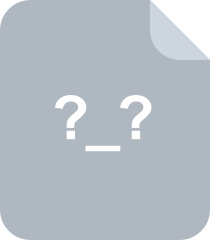
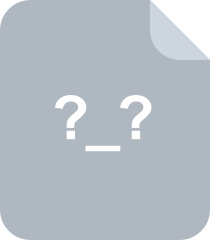
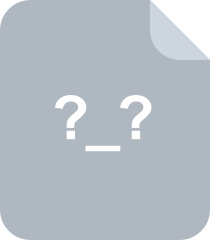
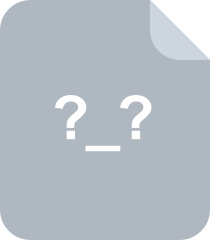
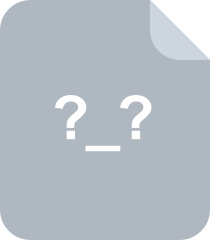
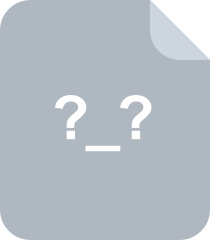
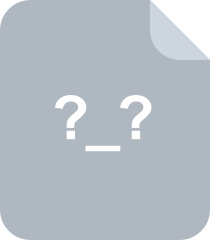
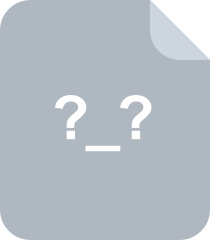
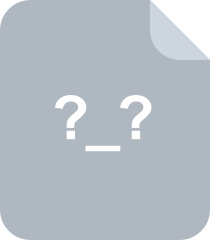
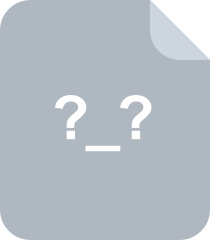
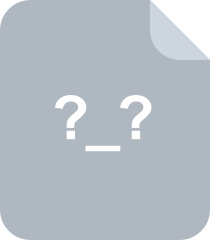
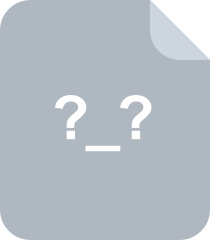
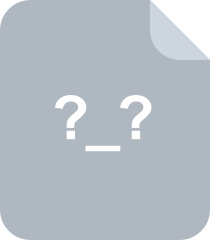
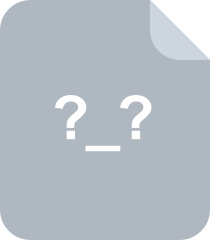
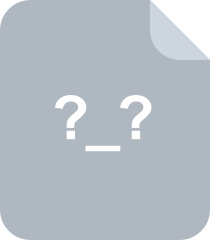
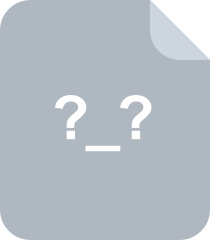
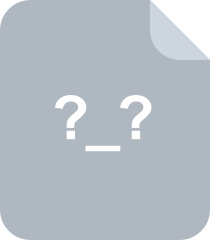
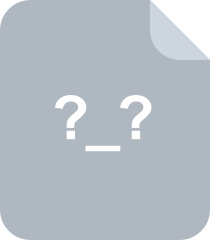
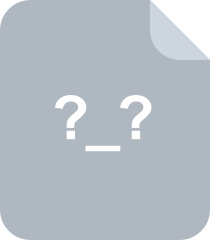
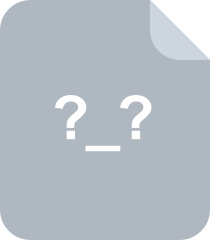
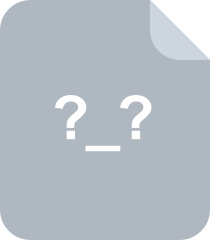
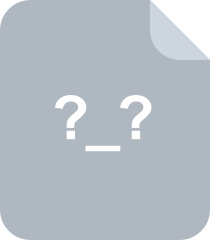
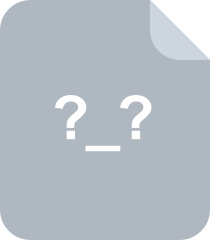
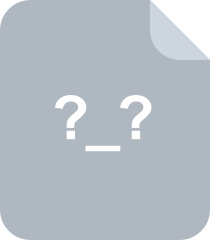
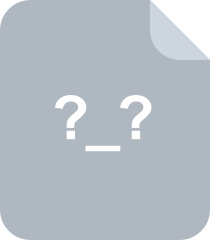
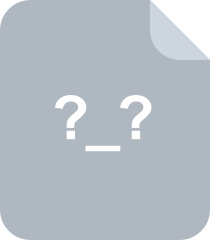
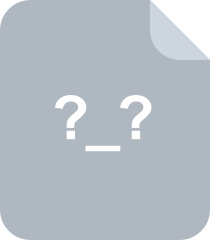
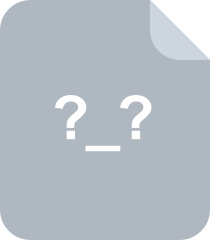
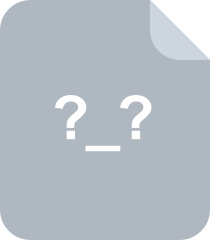
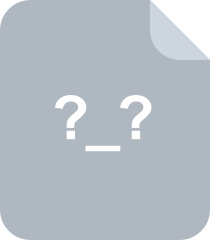
共 99 条
- 1
资源评论
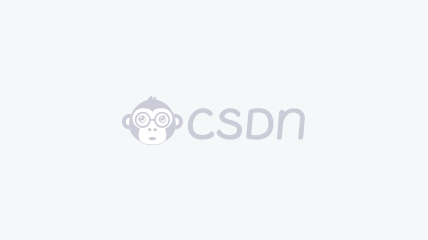

maxiaomx
- 粉丝: 0
- 资源: 3
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

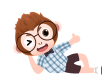
最新资源
- Python项目-自动办公-02 批量生成PPT版荣誉证书.zip
- 2025年十大战略技术趋势-Gartner-2024-27页.pdf
- tsn-imagenet-pretrained-r50-8xb32-1x1x8-100e-kinetics400-rgb-20220906-2692d16c.pth
- Python项目-实例-21 音乐播放器.zip
- 2010-2023年中国地级市绿色金融试点DID数据
- dpdk源码,高性能的网络驱动
- tsn-imagenet-pretrained-r50-8xb32-dense-1x1x5-100e-kinetics400-rgb-20220906-dcbc6e01.pth
- 对matplotlib进行介绍
- cmake-3.30.5.tar.gz
- 有监督的学习-线性回归.ipynb
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


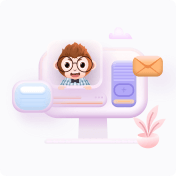
安全验证
文档复制为VIP权益,开通VIP直接复制
